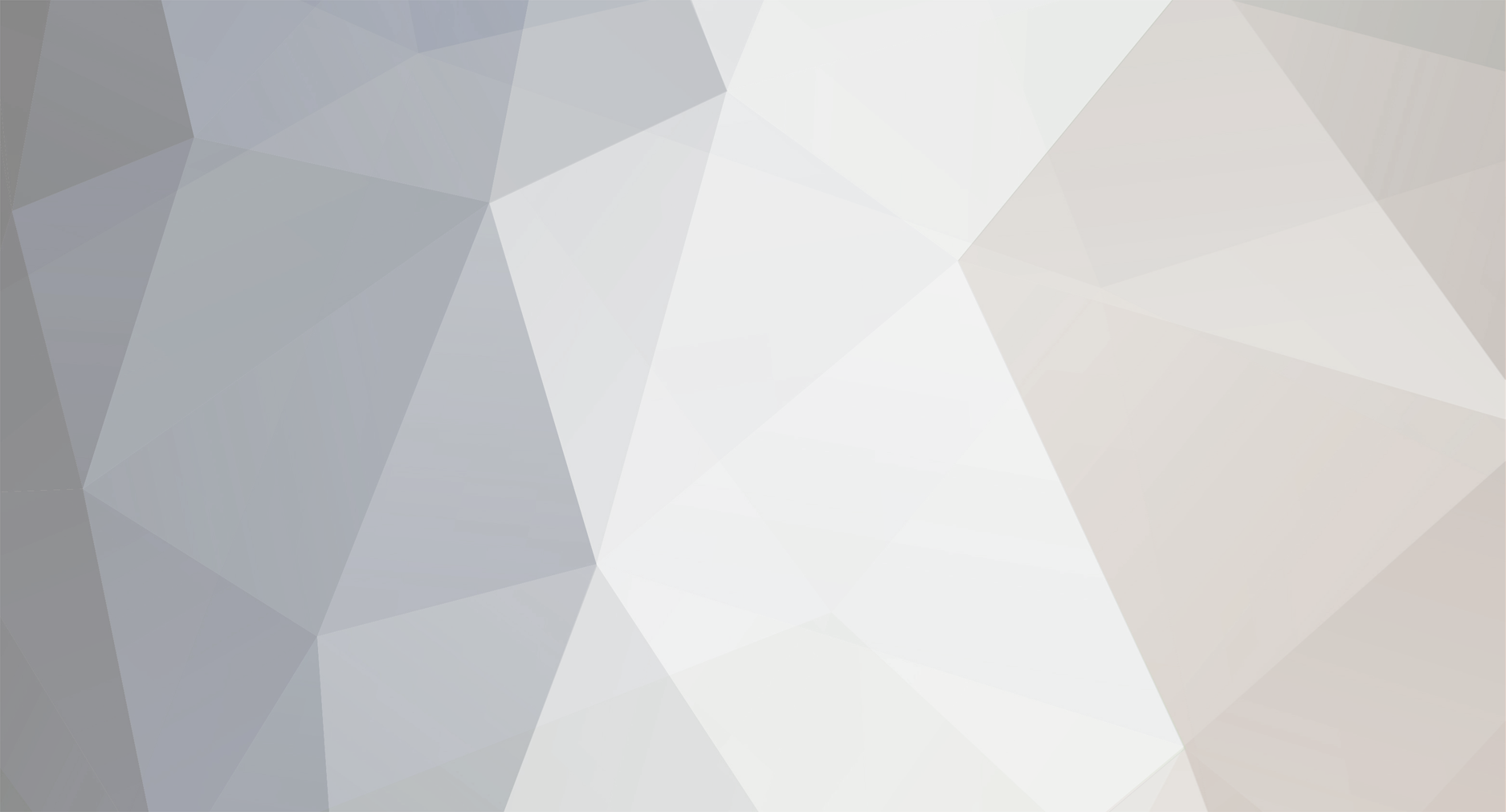
Wolphie
-
Posts
682 -
Joined
-
Last visited
Never
Posts posted by Wolphie
-
-
Eh, not the best practice. You should instead use whatever escape mechanism is appropriate for the db you're using (e.g., mysql_real_escape_string).
That wasn't intended to sanitize the input data. It was simply a solution to his problem (i.e. removing the slashes from his input string).
mysql_real_escape_string() prepends backslashes, which I assume he was already using since he was trying to remove the backslashes from his quotes.
-
What about using cookies? I've never heard of a session failing, however if the users browser doesn't accept sessions then this could be a problem. I'd attempt using cookies and then as a fallback I'd temporarily store their form data in a database.
Although, personally I'd rather store the data in the URL as a fallback.
-
Here's a very simple way of doing it without using sessions
<?php $rand = rand(1, 10); if (isset($_POST['submit'])) { if (preg_match('/[0-9]/', $_POST['user_input']) && preg_match('/[0-9]/', $_POST['user_input'])) { if ($_POST['user_input'] == $_POST['number']) { echo '<p>Correct!</p>'; } else { echo '<p>In-correct!</p>'; } } else { echo '<p>Please enter a valid number.</p>'; } } ?> <html> <body> <?php echo '<p>'. $rand .'</p>'; ?> <form name="form_sent" method="POST"> <input type="text" name="user_input" /><br /> <input type="hidden" name="number" value="<?php echo $rand; ?>" /> <input type="submit" name="submit" value="Submit" /> </form> </body> </html>
-
Not a problem, although you didn't mention that you were trying to draw out the postcode using PHP.
What is it *exactly* you're trying to do?
-
<?php function sanitize($input) { if (magic_quotes_gpc()) { $input = stripslashes($input); } else { $input = strip_tags($input); $input = htmlspecialchars($input); $input = trim($input); } return mysql_real_escape_string($input); } ?>
That's generally what I use to completely sanitise a string.
-
This should be in the JavaScript forum.
-
As PFMaBiSmAd said, store the number in a session variable..
<?php $_SESSION['number'] = rand(1, 10); // This will make the number absolute until changed by you if ($_REQUEST['number'] == $_SESSION['number']) { // correct } else { // false } ?>
-
Use stripslashes() before inserting the data into the database.
-
You would need to store the number in a session variable.
Web servers are stateless. They don't know or care what happened on any page request before or after the current page request. All resources used on any page, such as a variable, are destroyed when the server finishes with that page request.
In other words, every time the script is ran, the $number variable becomes a new random number. It isn't saved or stored in any way. So with each page request it is likely (but not absolute) to be different.
-
Can you post the other three images please?
-
I'm setting up a copy of your database and images. Can I have the other 3 images please?
-
Can you post the HTML source? You can upload images for free to http://tinypic.com/ and post the link here.
-
Are you sure the images are there? Have you checked the permissions on them? Is the path right?
-
<?php // Connect to server and select database. mysql_connect("$host", "$username", "$password")or die("cannot connect"); mysql_select_db("$db_name")or die("cannot select DB"); // Location where the images are stored $file_path = 'http://www.mysite.com/holidays/files/photo_big/'; $sql = mysql_query("SELECT photo_id, photo_caption_1, photo_listing FROM listing_photo WHERE photo_listing = 127 LIMIT 10"); if (mysql_num_rows($sql) > 0) { // Default numver of columns $num_cols = 2; while ($row = mysql_fetch_array($sql)) { $items[] = array('photo_id' => $row['photo_id'], 'photo_caption_1' => $row['photo_caption_1']); } // Number of items in the array $num_items = count($items); // Number of rows $num_rows = ceil($num_items / $num_cols); // Begin HTML table echo '<table width="100%">'; for ($row = 1; $row < $num_rows; $row++) { $cell = 0; // Start each new row echo '<tr>'; for ($col = 1; $col <= $num_cols; $col++) { echo '<td>'; if ($col === 1) { $cell += $row; echo '<div class="Image"><img src="'. $file_path . $items[$cell - 1]['photo_id'] .'.jpg" alt="'. $items[$cell - 1]['photo_caption_1'] .'" title="'. $items[$cell - 1]['photo_caption_1'] .'" />'; echo '<br />'; echo '<span><font face="Verdana, Arial, Helvetica, sans-serif" size="2" color="#000000">'. $items[$cell - 1]['photo_caption_1'] .'</font></span></div>'; } else { $cell += $row; echo '<div class="Image"><img src="'. $file_path . $items[$cell - 1]['photo_id'] .'.jpg" alt="'. $items[$cell - 1]['photo_caption_1'] .'" title="'. $items[$cell - 1]['photo_caption_1'] .'" />'; echo '<br />'; echo '<span><font face="Verdana, Arial, Helvetica, sans-serif" size="2" color="#000000">'. $items[$cell - 1]['photo_caption_1'] .'</font></span></div>'; } echo '</td>'; } echo '</tr>'; } echo '</table>'; } ?>
-
Try that
<?php // Connect to server and select database. mysql_connect("$host", "$username", "$password")or die("cannot connect"); mysql_select_db("$db_name")or die("cannot select DB"); // Location where the images are stored $file_path = 'http://www.mysite.com/holidays/files/photo_big/'; $sql = mysql_query("SELECT photo_id, photo_caption_1, photo_listing FROM listing_photo WHERE photo_listing = 127 LIMIT 10"); if (mysql_num_rows($sql) > 0) { // Default numver of columns $num_cols = 2; while ($row = mysql_fetch_array($sql)) { $items[] = array('photo_id' => $row['photo_id'], 'photo_caption_1' => $row['photo_caption_1']); } // Number of items in the array $num_items = count($items); // Number of rows $num_rows = ceil($num_items / $num_cols); // Begin HTML table echo '<table width="100%">'; for ($row = 1; $row < $num_rows; $row++) { $cell = 0; // Start each new row echo '<tr>'; for ($col = 1; $col <= $num_cols; $col++) { echo '<td>'; if ($col === 1) { $cell += $row; echo '<div class="Image"><img src="'. $file_path . $items[$cell - 1]['photo_id'] .'.jpg'" alt="'. $items[$cell - 1]['photo_caption_1'] .'" title="'. $items[$cell - 1]['photo_caption_1'] .'" />'; echo '<br />'; echo '<span><font face="Verdana, Arial, Helvetica, sans-serif" size="2" color="#000000">'. $items[$cell - 1]['photo_caption_1'] .'</font></span></div>'; } else { $cell += $row; echo '<div class="Image"><img src="'. $file_path . $items[$cell - 1]['photo_id'] .'.jpg" alt="'. $items[$cell - 1]['photo_caption_1'] .'" title="'. $items[$cell - 1]['photo_caption_1'] .'" />'; echo '<br />'; echo '<span><font face="Verdana, Arial, Helvetica, sans-serif" size="2" color="#000000">'. $items[$cell - 1]['photo_caption_1'] .'</font></span></div>'; } echo '</td>'; } echo '</tr>'; } echo '</table>'; } ?>
-
Okay, this should work for you.
<?php // Connect to server and select database. mysql_connect("$host", "$username", "$password")or die("cannot connect"); mysql_select_db("$db_name")or die("cannot select DB"); // Location where the images are stored $file_path = 'http://www.mysite.com/holidays/files/photo_big/'; $sql = mysql_query("SELECT photo_id, photo_caption_1, photo_listing FROM listing_photo WHERE photo_listing = 127 LIMIT 10"); if (mysql_num_rows($sql) > 0) { // Default numver of columns $num_cols = 2; while ($row = mysql_fetch_array($sql)) { $items[] = array('photo_id' => $row['photo_id'], 'photo_caption_1' => $row['photo_caption_1']); } // Number of items in the array $num_items = count($items); // Number of rows $num_rows = ceil($num_items / $num_cols); // Begin HTML table echo '<table width="100%">'; for ($row = 1; $row < $num_rows; $row++) { $cell = 0; // Start each new row echo '<tr>'; for ($col = 1; $col <= $num_cols; $col++) { echo '<td>'; if ($col === 1) { $cell += $row; echo '<div class="Image"><img src="'. $file_path . $items[$cell - 1]['photo_id'] .'.jpg'; .'" alt="'. $items[$cell - 1]['photo_caption_1'] .'" title="'. $items[$cell - 1]['photo_caption_1'] .'" />'; echo '<br />'; echo '<span><font face="Verdana, Arial, Helvetica, sans-serif" size="2" color="#000000">'. $items[$cell - 1]['photo_caption_1'] .'</font></span></div>'; } else { $cell += $row; echo '<div class="Image"><img src="'. $file_path . $items[$cell - 1]['photo_id'] .'.jpg'; .'" alt="'. $items[$cell - 1]['photo_caption_1'] .'" title="'. $items[$cell - 1]['photo_caption_1'] .'" />'; echo '<br />'; echo '<span><font face="Verdana, Arial, Helvetica, sans-serif" size="2" color="#000000">'. $items[$cell - 1]['photo_caption_1'] .'</font></span></div>'; } echo '</td>'; } echo '</tr>'; } echo '</table>'; } ?>
-
Or an improved version:
<?php /** * This script allows people to get a data set from * a single table in the database and display the data * in multiple columns of an HTML table. * * * Example usage: * * $sql = "SELECT url_links FROM websites"; * echo display_columns($sql, 'url_links', 3, 'my_table_class'); */ /** * This function will allow you to display a single * data set into multiple columns of an HTML table. * * @param $query * This is the MySQL query that is ran to return the * data set. * * @param $field * This is the name of the table field we should columnise. * * @param $num_cols * The number of columns to split the data set up into. * * @param $class * The CSS class of the table so that it can be styled. * This is optional. * * @return * Returns a formatted HTML string containing the tabulated * data from the data set. */ function display_columns($query, $field, $num_cols, $class = '') { // Execute query $sql = mysql_query($query); if (mysql_num_rows($sql) > 0) { // Stick the results in another array while ($row = mysql_fetch_array($sql)) { $items[] = array(1 => $row[$field]); } // Number of items in the array $num_items = count($items); // Number of rows $num_rows = ceil($num_items / $num_cols); // Begin HTML table $output = '<table width="100%" class="'. $class .'">'; for ($row = 1; $row < $num_rows; $row++) { // Start at cell 0 $cell = 0; // Start each new row $output .= '<tr>'; for ($col = 1; $col <= $num_cols; $col++) { $output .= '<td>'; if ($col === 1) { // For each iteration, add the row number so that we know what element to get data from $cell += $row; // Use the row number subtract one to get the correct element we're after $output .= $items[$cell - 1][1]; } else { // For each iteration, add the row number so that we know what element to get data from $cell += $row; // Use the row number subtract one to get the correct element we're after $output .= $items[$cell - 1][1]; } $output .= '</td>'; } $output .= '</tr>'; } // End HTML table $output .= '</table>'; } // Return the data return $output; } ?>
-
I think that's what you're after.
<?php // Database connection info // Execute query to retrieve data $sql = mysql_query("SELECT column1 FROM tblname"); if (mysql_num_rows($sql) > 0) { // Default numver of columns $num_cols = 2; while ($row = mysql_fetch_array($sql)) { $items[] = array(1 => $row['names']); } // Number of items in the array $num_items = count($items); // Number of rows $num_rows = ceil($num_items / $num_cols); // Begin HTML table echo '<table width="100%">'; for ($row = 1; $row < $num_rows; $row++) { $cell = 0; // Start each new row echo '<tr>'; for ($col = 1; $col <= $num_cols; $col++) { echo '<td>'; if ($col === 1) { $cell += $row; echo $items[$cell - 1][1]; } else { $cell += $row; echo $items[$cell - 1][1]; } echo '</td>'; } echo '</tr>'; } echo '</table>'; } ?>
-
This would be done in JavaScript/AJAX. I strongly suggest that you use jQuery for this, go to http://jquery.com/ and take a look around. It makes nice effects and AJAX scripting A LOT easier.
-
Check out http://swfupload.org/, I think this is what you're looking for.
-
That seems like a legit reason. However, when you're doing this nothing else should be echo'd on the page where this code belongs.
If you have all of the text you want to place in the file you can use this:
<?php // Once the user visits this page, the code below will be ran and a file transfer will be initiated by the browser. // Name of the file $filename = 'filename.txt'; // Set the appropriate headers to tell the browser that it's a file to downlaod header('Content-Type: application/octet-stream'); header('Content-Description: File Transfer'); header('Content-Disposition: attachment; filename="'. $filename .'"'); header('Pragma: no-cache'); header('Expires: 0'); // $output contains the text that you want to place into the .txt file. echo $output; ?>
-
You check the profile picture field in the user table for a value.
For example
<?php $sql = mysql_query("SELECT avatar FROM users ORDER BY RAND() LIMIT 12"); if (mysql_num_rows($sql) > 0) { while ($item = mysql_fetch_array($sql)) { if ($item['avatar'] == '') { echo 'nopic.png'; } else { echo 'profilepic.png'; } } } ?>
-
Me either really, I just took a shot in the dark.
-
<?php $val1 = 5; $val2 = 10; $val3 = 7; if (($val3 > $val1) && ($val3 < $val2)) { echo 'icon.png'; } else { echo 'othericon.png'; } ?>
problem with a FOR within a FOR
in PHP Coding Help
Posted
Could you please just post the code that is relevant to your problem?