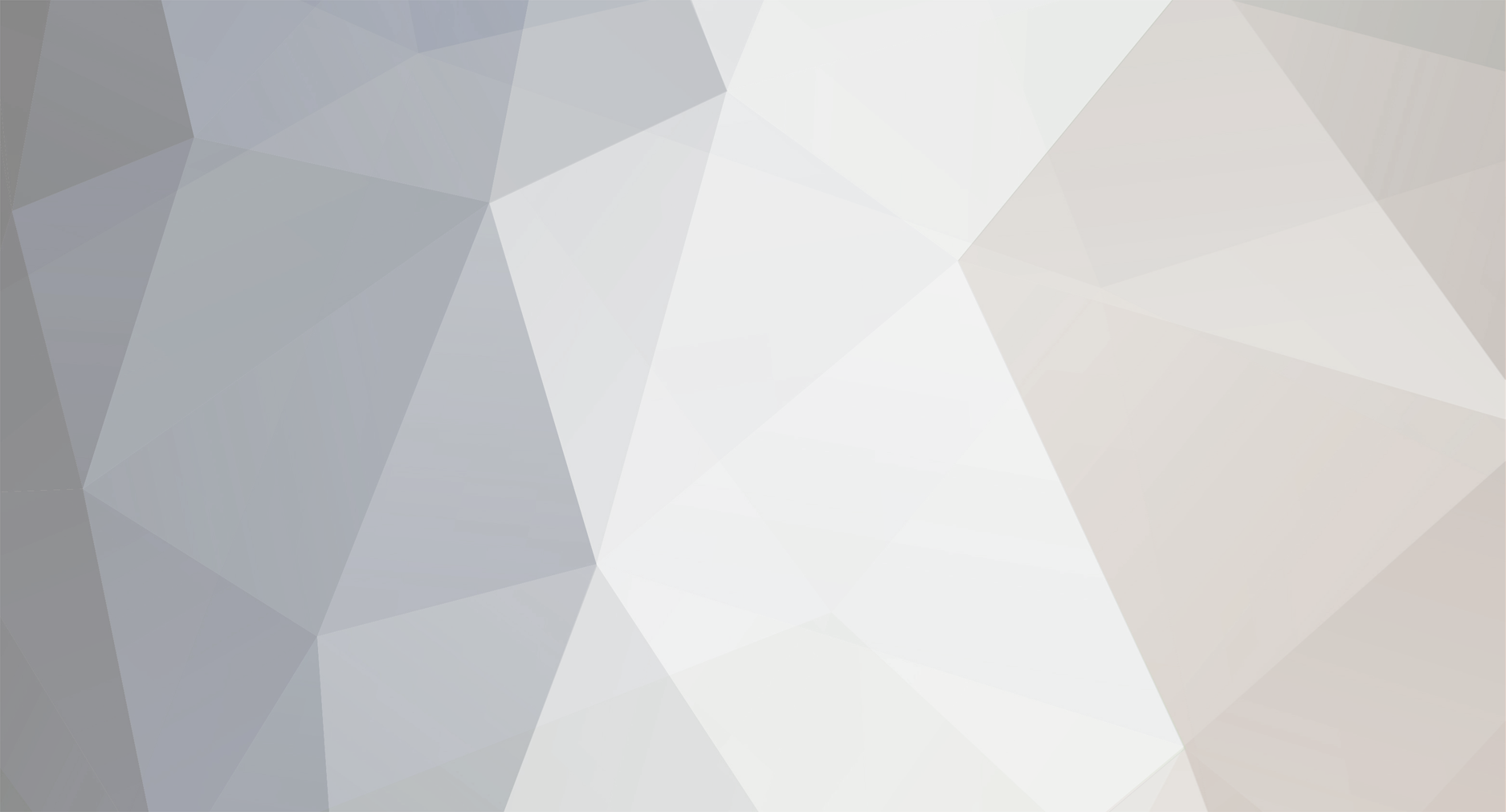
Wolphie
-
Posts
682 -
Joined
-
Last visited
Never
Posts posted by Wolphie
-
-
Why is
require_once 'DbVars.php';
placed outside of the <?php ?> tags?
-
Generators aren't always reliable. If I were you, I'd start where thorpe suggested, reading the free PHP book on hudzilla. You don't need a book for reference when you already have awesome PHP documentation at www.php.net
-
Post the code for DbConnector.php, it appears that the DbConnector class isn't defined.
-
You're going to have to change every local HREF to match your new URL scheme. Have fun
Yes, you will.
As I mentioned before, this really isn't a sufficient method to use for a scale of 200 files give or take. Hence why you should use Clean URLs (mod_rewrite) when starting an application, that way you can put contingency and fail safes in place.
For a pre-built website with 200 files or more and hundreds if not thousands of redirects and anchor links, I'd for sure advise against this. On the other hand, if you were just starting this application. I'd support you all the way and advise you to use Clean URLs provided you have fail safes and contingency in place.
For a dynamic URL you would use something like..
-
Please do clarify how exactly I'm mistaken? If that is the case, it's due to your poor explanations and descriptions.
If you're asking for help on something specific, state it as clearly as you can, with a description on what you want and what you're trying to achieve. Thank you.
As a matter of elaboration, they're both "select" box elements. A "jump" box as you so describe is just a select box with some JavaScript implemented into it.
Now please, explain as best you can what you want, and what you're trying to achieve.
-
This is what I use:
RewriteEngine on RewriteCond %{REQUEST_URI} ^/[^\.]+[^/]$ RewriteRule ^(.*)$ http://%{HTTP_HOST}/$1/ [R=301,L] RewriteRule ^([^/\.]+)/?$ /index.php?q=$1 [L] RewriteRule ^([^/\.]+)/([^/\.]+)/?$ /index.php?view=$1&id=$2 [L] # RewriteRule ^([^/\.]+)/([^/\.]+)/([^/\.]+)/?$ /index.php?section=$1&subsection=$2&page=$3 [L] # These rewrite rules can be used over and over for different master pages. # For example, see below. # RewriteRule ^([^/\.]+)/?$ /profile.php?user_id=$1 [L] # RewriteRule ^([^/\.]+)/([^/\.]+)/?$ /profile.php?category=$1&page=$2 [L] # RewriteRule ^([^/\.]+)/([^/\.]+)/([^/\.]+)/?$ /profile.php?category=$1&subcategory=$2&page=$3 [L]
However I doubt that would be sufficient enough for over 200 different filenames. However, it should help you out a bit. Hopefully.
-
It's
<select multiple></select>
Example:
<select multiple size="4"> <option>Option 1</option> <option>Option 2</option> <option>Option 3</option> <option>Option 4</option> </select>
-
Then why even show .xxx at all? Why not just use Clean URLs? That way nobody can see any filetype/extension except for images etc...
-
OK, now "None" is being set. However, it doesn't seem to get past
if($completed != 0) { ... }
So no values are being pushed into the array.
-
Yes it should, thanks for pointing that out for me. Although doing that, I now have another problem. How will I be able to determine whether I should use "None" or not? Since $completed will always return TRUE to being an array.
-
Ok so,
If have a group of checkboxes, and I know how to get the values from each of them in an array. However, I can't seem to find a solution to
only return a default value if "none" of the checkboxes are checked, and only return the values that are checked.
I've tried:
foreach($form_values['completed'] as $complete) { if($complete != 0) { $completed = array(); $completed = array_push($completed, $complete); } } if (is_array($completed)) { $completed = implode(', ', $completed); } else { $completed = 'None'; }
-
http://irjaws.com/zymn/uploads//phpinfo.php
Lol,
I'd seriously advise against allowing .php file extensions.
-
What are the errors?
-
Could you please paste the code relevant to that error.
I.e. lines 1430 - 1440 and 1060 - 1070
-
It'd be useful if you provided some code.
-
This is called pagination. There is a tutorial for it on the main PHP Freaks website.
-
Try this:
$query = sprintf("SELECT * FROM `table` WHERE `City`, `State`, `ZipCode` LIKE '%s' AND `%s` = '1'", mysql_real_escape_string('%'. $_POST['mainSearch'] .'%'), mysql_real_escape_string($_POST['Type']) ); $result = mysql_query($query) or trigger_error(mysql_error());
That's how I'd do it any way, assuming the value of $_POST['Type'] will be equal to the name of a field.
-
Welcome to PHP Freaks, I'm sure you'll find what you're looking for.
-
If it was me I'd look into using sockets, however you could just use file_get_contents().
PHP Reference: http://uk.php.net/manual/en/function.file-get-contents.php
-
This is how I would do it:
$query = "UPDATE `jtablegrid` SET $tmp_name = '$username' WHERE `game_no` = '$game_no_tempo'";
Just out of curiosity, what is $tmp_name? Is it just a variable storing a field name?
-
or just write
<input type="checkbox" name="check[]" value="1" />
in php you could access it in this manner:
<?php
echo $_POST['check'][0]; // 1
?>
ohhh smart
I prefer to use implode(', ', $_POST['check'])); which will turn the array into a string, with the values being separated by comma's. Or you could loop through them using a foreach loop, if you want to automate things a bit.
-
I've never had any problem writing and retreiving data from the database in any character case. Have you tried just writing a value to the database using different cases throughout and then tried validating it using lower case / upper case?
<?php $sql = "SELECT pwd FROM users WHERE LOWER (`username`) = '".strtolower($username)."'"; ?>
Field names should not be in single quotes, surroung them with bck ticks..try and let me know
A back tick is just another form of a quote, it doesn't matter what you surround them in, it's strictly for clarity and easy reading.
-
How exactly are you going to store user information? Sessions and cookies only last so long.
-
Do you mean you want it to go the other way around? I.e. Use Clean URLs?
By the way, how is this relevant to the PHP forum?
Parse error: parse error, unexpected '\"', expecting T_STRING or T_VARIABLE or T
in PHP Coding Help
Posted
Then as you would normally: