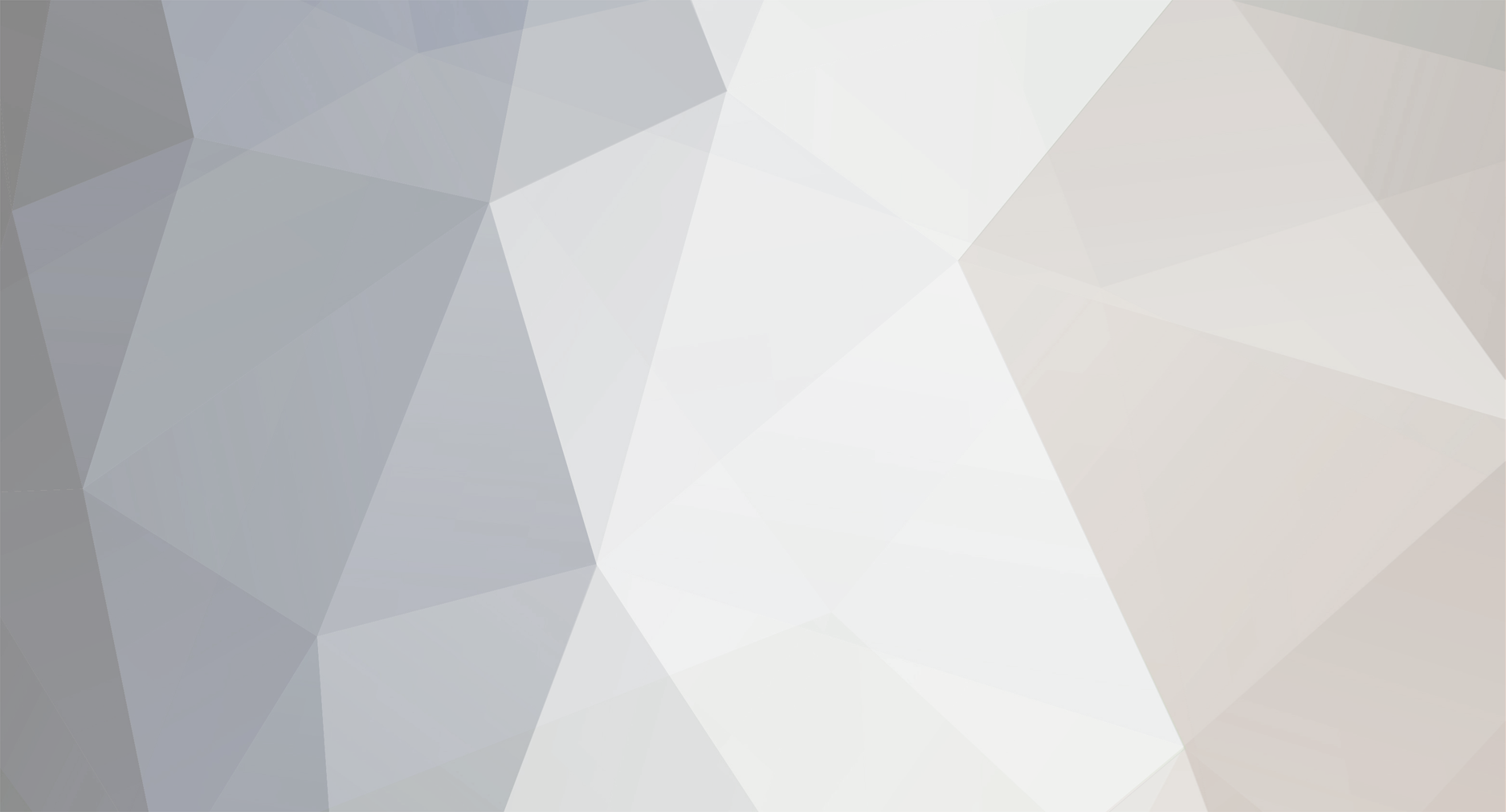
Wolphie
-
Posts
682 -
Joined
-
Last visited
Never
Posts posted by Wolphie
-
-
Please, when posting code remember to use the code BB Code.
-
Well, I still don't entirely understand what you're trying to achieve, but this is my best guess:
<?php $validate = array('var1', 'var2'); foreach ($validate as $key) { if (!isset($_REQUEST[$key]) || empty($_REQUEST[$key])) { // empty or not set } else { // They're fine } ?>
-
Oh, sorry I misunderstood. As alpine pointed out, using $_REQUEST will do both if you're unsure which to expect.
-
Well as you know, isset() literally just checks if the variable has been set, it has no kind of input validation on it. For example you would use it to check to see if a session variable had been set when a user logs in, the value may not be important.
However, in a user-submitted form the value is important, i'd prefer to use the empty() function to check against form element inputs. But on the other hand, I would use isset() to check for checkboxes, and radio buttons.
-
Alpines solution is currently the most widely-used and elegant way of doing what you're trying to achieve.
-
Are you using a primary key for the records in the database?
-
-
I'm pretty sure this isn't PHP related.
-
Of course there is, first check that it's an array you're trying to loop using
-
-
Personally, I'd recommend taking a look at how regular expressions work and how they could help you accomplish what you're trying to do. I've looked at your code, and this works for me although I'm not entirely sure if it's exactly what you're after.
<?php $content = "Crazy Random Apple 43 Left Apple Truck Plane Turtle Duck"; $needles = array("Random", "Apple", "Duck"); $words = split(" ", $content); foreach($words as $word) { foreach ($needles as $needle) { if ($word == $needle) { // Flag regular word flag($word); $alert = 1; } } if ($alert == 1) { if (is_numeric($word)) { $alert = 0; } else { flag($word); } } } ?> [code]
-
Before looking behind the scenes at the code of a content management system and getting into the complexities, I suggest you install one locally and play around with it so that you can understand the processes in which content is dynamically added and changed/removed.
-
I can't really see a shorter way except concatenating it into a single string.
-
This isn't really possible unless you have shell access, or telnet access... and even then I think it would be difficult. Those are the only 2 ways without actually downloading the file to the local machine, whether it's an automated process or not. FTP would require a connection, retrieving the file, opening it, making the changes and then uploading it again.
I hope someone can correct me on that, because I'd be interested to know also.
-
Honestly, there are so many bulletin board softwares out there, it's pointless writing one yourself unless it has a unique feature which none other has. Which is unlikely since most bulletin boards support plug-ins etc...
I'd stick with one that's maintained, open-source and supported.
-
<?php $sql = mysql_query("SELECT * FROM starters"); echo '<table>'; while ($item = mysql_fetch_object($sql)) { echo '<td>'. $item->starter .'</td>'; echo '<td><input type="checkbox" name="selected[\''. $item->starter .'\']" />'; } echo '</table>'; ?>
-
Why can't you just place a check box next to it?
<?php $sql = mysql_query("SELECT * FROM menu"); echo '<table>'; while ($item = mysql_fetch_object($sql)) { echo '<td>'. $item->name .'</td>'; echo '<td><input type="checkbox" name="selected[\''. $item->name .'\']" />'; } echo '</table>'; ?>
-
Well first, what are you trying to achieve?
-
You'd need to get the values from the database and put them in the $offline array.
E.g.
<?php $offline = array(); // Execute query $sql = mysql_query("SELECT a, b, c FROM some_table LIMIT 1"); // Get the key and the corresponding value and place them into the $offline array foreach (mysql_fetch_assoc($sql) as $key => $val) { $offline[$key] = $val; } ?>
I think that's what you're looking for.
-
<?php if (get_env("HTTP_X_FORWARDED_FOR")) { $ip = get_env("HTTP_X_FORWARDED_FOR"); // They're behind a proxy } else { $ip = getenv("REMOTE_ADDR"); // Proxy doesn't exist } ?>
-
Where is the HTML coming from? Are you opening a different file and reading the contents or is it hard coded as a string in the PHP script itself? or perhaps somewhere in a database?
-
Sorry, no I didn't see that.
I'm assuming, $theme_parssed contained all of the HTML to begin with?
-
{username}
is not the same as
{=username}
-
Well, first of all $images_total should be an integer, not a string. Adding the quotes make it a string so using the greater than or less than comparison operators won't work here.
<tr> <td colspan="2"><input type="submit" class="button" value="<?php if ( $images_total < 3 ) { echo "_UPLOAD_SUBMIT"; { else { echo "You have reached your upload limit"; } { } ?>" /></td> </tr>
NooB Alert - Optimize Playlist player
in PHP Coding Help
Posted
Possibly a good idea to enable some form of caching too.