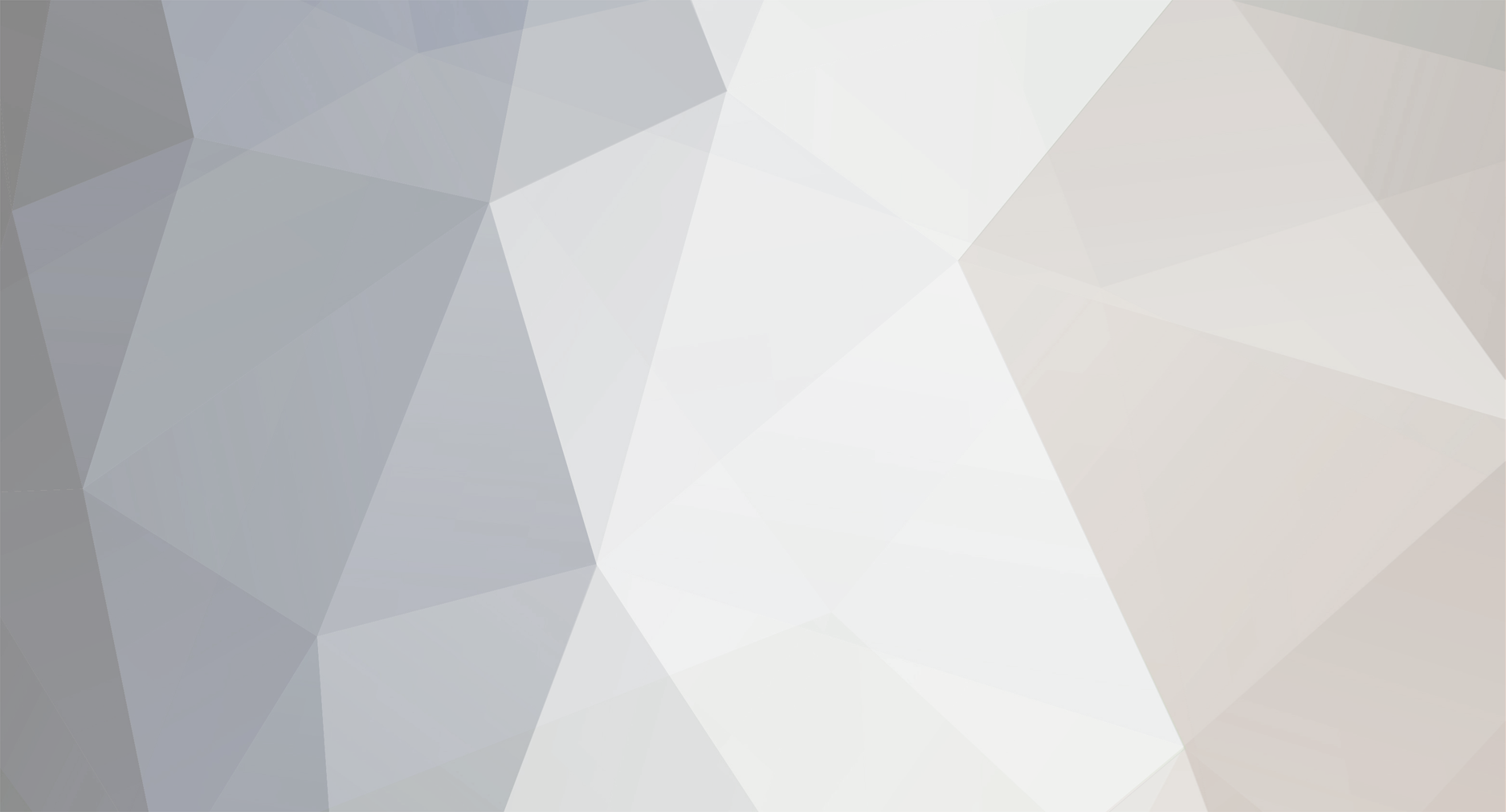
ngreenwood6
Members-
Posts
1,356 -
Joined
-
Last visited
Everything posted by ngreenwood6
-
take out the exit statement and if that doesnt work post your new code.
-
you should probably be using the % in your like statement. try this: <?php ini_set ("display_errors", "1"); error_reporting(E_ALL); session_start(); $db_name = "arscnaor_meetings"; //connect to server and select database $connection = mysql_connect("localhost", "username", "password") or die(mysql_error()); $db = mysql_select_db($db_name, $connection) or die(mysql_error()); //formats from add_meetings $formats = array("Discussion", "Literature Study", "Book Study", "Basic Text", "It Works", "Step Study Guides", "IP Study", "Step Study", "Traditions Study", "Concepts Study", "Just For Today", "New Comer", "Participation", "Topic", "Candlelight", "Speaker", "Birthday", "Serenity", "Men", "Women", "Varies", "Other"); foreach($formats as $format){ $format_sql = "SELECT format FROM meeting WHERE format LIKE '%$format%'"; $format_query = mysql_query($format_sql) or trigger_error(mysql_error()); $format_num = mysql_num_rows($format_query); echo $format . ": " . $format_num . "<br />"; } ?> However, that is alot of queries you are performing there. I would do something like this: <?php ini_set ("display_errors", "1"); error_reporting(E_ALL); session_start(); $db_name = "arscnaor_meetings"; //connect to server and select database $connection = mysql_connect("localhost", "username", "password") or die(mysql_error()); $db = mysql_select_db($db_name, $connection) or die(mysql_error()); //formats from add_meetings $formats = array("Discussion", "Literature Study", "Book Study", "Basic Text", "It Works", "Step Study Guides", "IP Study", "Step Study", "Traditions Study", "Concepts Study", "Just For Today", "New Comer", "Participation", "Topic", "Candlelight", "Speaker", "Birthday", "Serenity", "Men", "Women", "Varies", "Other"); //make only one query $format_sql = "SELECT format FROM meeting"; $format_query = mysql_query($format_sql) or trigger_error(mysql_error()); //initialize an array for formats $returned_formats = array(); while($format_row = mysql_fetch_array($format_query)){ $returned_formats[$format_row['format']] = ($returned_formats[$format_row['format']]) ? $returned_formats[$format_row['format']] + 1 : $returned_format[$format_row['format']] = 0; } echo $returned_formats['Discussion']; ?> This just performs one query and then assigns a count to the format based on the name of the format that you cna use through its own array. Please let me know if you have any questions.
-
There is no real good solution for this unless you use javascript or jquery(javascript library). If you are new to javascript and dont mind a little overhead by a library i would suggest looking into jquery if you want a quick solution. It simplifies ajax requests and is a little easier to get things done. However, I would not suggest strictly learning jquery. Jquery is simply a "wrapper" for javascript because it in reality uses javascript for all the code that is performed. So I would suggest that you learn javascript and then if you decide to use jquery it is always a great addition to your knowledge
-
it is a placeholder for mysql_affected_rows(). the printf() function takes 2 or more arguments the first one is a string, the second is variables. anthing that is set with a % sign infront of it is a placeholder for a variable. the d after the % means that it is going to be an integer (see sprintf() for format http://www.php.net/manual/en/function.sprintf.php) heres a few examples: $name = 'nick'; $age = 23; printf('My name is %s and my age is %d.', $name, $age); So this would show this on the screen:
-
WHYISNT THIS MYSQL STATEMNT NOT WORKING? (php)
ngreenwood6 replied to emopoops's topic in PHP Coding Help
no this is a count. so if you have 5 entries in the database that match the criteria that you queried it will show 5 as the count. it doesnt add up those 5 values in the fields just tells you how many rows were returned. -
For the time being you could just simply set a meta refresh on the page once it is submitted: if($_POST){ echo '<meta http-equiv="refresh" content="5">'; } That would make it so that when the page is posted it will refresh the page after 5 seconds. Because this is in an iframe it should just reload the page. Try it and let me know.
-
you could change your form to something like this: <form id="form1" action="somepage.php" method="post"> <input type="text" id="textbox1"> <input type="button" onclick="someFunction()"> </form> then your javascript would look like this: function someFunction(){ //get the form var form1 = document.getElementById('form1'); //check the form if(document.getElementById('textbox1').value != ''){ //submit the form form1.submit(); } else { //show alert on error alert('Please fill in text box'); } } Basically all this does is submit the form after checking that your criteria is met. It will submit it as a POST instead of a GET. Please ask if you have any questions.
-
you could do something like this: $flush55 = mysql_query("DELETE FROM dumps WHERE id='$flushing' AND time = '$wow'"); $flush2 = mysql_query("DELETE FROM tp WHERE dump = '$flushing'"); if($flush55 && mysql_affected_rows() > 0){ echo "SUCCESSFULLY FLUSHED THE DUMP HON!<br>"; } else { echo "no rows affected"; } The mysql_affected_rows is just a count of the number of rows that were changed. so all I did was add a check to see if the affect rows is greater than 0 (basically a row was changed).
-
WHYISNT THIS MYSQL STATEMNT NOT WORKING? (php)
ngreenwood6 replied to emopoops's topic in PHP Coding Help
no your right when you are in your while loop you can access the variable like you would a field like this: while($row = mysql_fetch_array($query)){ echo $row['count_age']; } I had just omitted it out of my example -
associating field and ID in database
ngreenwood6 replied to pioneerx01's topic in Microsoft SQL - MSSQL
something like this: //some name here $name1 = 'something'; //just insert the first name for now $query = mysql_query("INSERT INTO table(name1) VALUES ('$name1')"); //gets the last id $last_id = mysql_insert_id(); //once we have the id and the record there we can just update it with the name1 and last_id $another_query = mysql_query("UPDATE table SET name2 ='$name1.$last_id' WHERE id='$last_id'"); hopefully that helps. There is a mysql function called last_insert_id() but I think that will be the same type of method i did here, but figured id mention it if you wanted to look into it. -
i would check if there are any javascript errors. if there are post them here. in IE you can see javascript errors by looking in the bottom left hand corner. if there is an error there will be a yellow exclamation point. also if you like using firebug in FF there is firebug lite that can be used in IE to help troubleshoot.
-
WHYISNT THIS MYSQL STATEMNT NOT WORKING? (php)
ngreenwood6 replied to emopoops's topic in PHP Coding Help
count isnt used to get values from the database. essentially it does the same thing as mysql_num_rows. If you were to do this: "SELECT name, count(age) as count_age FROM people" you could then access the count in your code by doing: echo $row['count_age']; This would give you the count of the rows with age, but instead of counting all the results you are just counting the age row. -
it seems to me like the query is being successful. keep in mind that just because it is successful does not mean that it actually did anything. It could have went through the database and looked for values that matched your criteria but didnt find any so it did nothing. However, that would show as successful because it didnt get any errors along the way. But if there was an error in there then it would not show as successful. You should try passing values that you know meet your criteria and check the data then because hte query looks fine. you could always do an or die(mysql_error()) after your query to see if there was an error.
-
yeah this is pretty much turning into an html/css issue rather than a php issue. The problem I can tell you though is because you are using the tags. if you just layout a page and use an h1 tag with some text and and h2 tag with some more text it will go to the next line. if you remove those tags you will probably notice that it all goes on the same line.
-
should look like this: $selected = ($row['id'] == $select_value) ? 'selected' : '';
-
I agree with mchl on this one. Just making errors go away is never a good idea IMO. There is a reason why it is giving you an error and maybe you should look into why it is giving you that error. If you write good code you should never see any php errors. You may not get the results that you want but you wont see an error for it. For example if you were to do this: $test = ''; foreach($test as $t){ //do something here } it will give you an error saying that there was invalid argument supplied (or something to that effect) because it is expecting an array but is getting a single value. But if you were to do this: $test = ''; if(is_array($test)){ foreach($test as $t){ //do something here } } else { echo 'not array'; } You will not get an error. That is called error handling. It first checks the variable test to see if it is an array and if it is it will loop. If it is not then it will just say not an array. But at no point will this throw an error and you can run it with ERRORS ON. But like I said you arent going to get the expected result if you dont pass it an array.
-
oh you know what its late and im not thinking. in the head you are using color=#ff0000 it should read color:#ff000000 css assigns using :'s not ='s edit: you will have to change the ones for font-size and text-align as well.
-
here is some code that should help you: $sql = "SELECT * FROM table"; $query = mysql_query($sql); $columns = mysql_num_fields($query); echo mysql_field_name($query,$columns-1); The columns will be the count of the fields. Then when you need to get the field name, you pass it the resource and the column that you want which in this case is the last one but since you are starting with 0 instead of 1 we subtract one...hope that helps.
-
it could be that you are putting in a ' or a " in the field? you should always run data that is going into a database through mysql_real_escape_string() ex: $id = mysql_real_escape_string($_POST['somethinghere']); $query = "INSERT INTO table (id) VALUES ('$id')"; That code will escape all ' or " making it "safe" for the database. it also prevents errors when users try to use a ' or a " for data going into the database.
-
is it possible to pass an array variable through the URL?
ngreenwood6 replied to co.ador's topic in PHP Coding Help
you can either pass it by serializing it like rajivgonsalves said or you could actually pass it as an array. ex. serialize $pass = serialize($array); echo '<a href="test.php'.$pass.'">test</>'; ex. array echo '<a href="test.php?arr[]=test1&arr[]=test2">test</a>'; -
try this: <html> <head> <style> h1{ font-size=14; color=red; text-align=center; } h2{ font-size=14; color=orange; text-align=center; } </style> </head> <body> <?php echo "Here's one:"; $data = file_get_contents('**URL here**'); $regex = '/"**What I am matching**"/'; preg_match($regex,$data,$match); if ($match[1] == '**Found string**') { echo '<h1>**words here <a href="**link here**">**link name here**</a></h1>'; } elseif ($match[1] != '**something**'){ echo '<h2>**more text here**</h2>'; } I am not sure if the orange one will show up or not. you shouldnt use colors in the form of text you should use there code (ex. white = #FFFFFF). You were assigning a font-color when you should just be assigning a color for the css. Also your h1 tag was closing before the a tag was closing you also were missing a } after the elseif statement. You were missing a closing head tag. You should really get more experience with the basic html coding as I noticed alot of syntax errors.
-
WHYISNT THIS MYSQL STATEMNT NOT WORKING? (php)
ngreenwood6 replied to emopoops's topic in PHP Coding Help
in mysql to is a reserved keyword http://dev.mysql.com/doc/refman/5.0/en/reserved-words.html -
am i in the right direction with this function?
ngreenwood6 replied to justAnoob's topic in Javascript Help
not if you call the function that mjdamato suggests passing it the elements name or in your case $row['item_name'] so it would look like this: <script type="text/javascript"> clicked($row['item_name']); </script> -
Using INT or VARCHAR to store numbers, or convert to HEX?
ngreenwood6 replied to jeremyhowell's topic in MySQL Help
I dont think that it is really necessary to save them as hex. If you were to convert them to hex it would take extra time to run, then when you needed to query it back you would have to decrypt it (taking extra time) and its not really going to take up that much space in the database. You could however always store them as a varchar if you choose though.