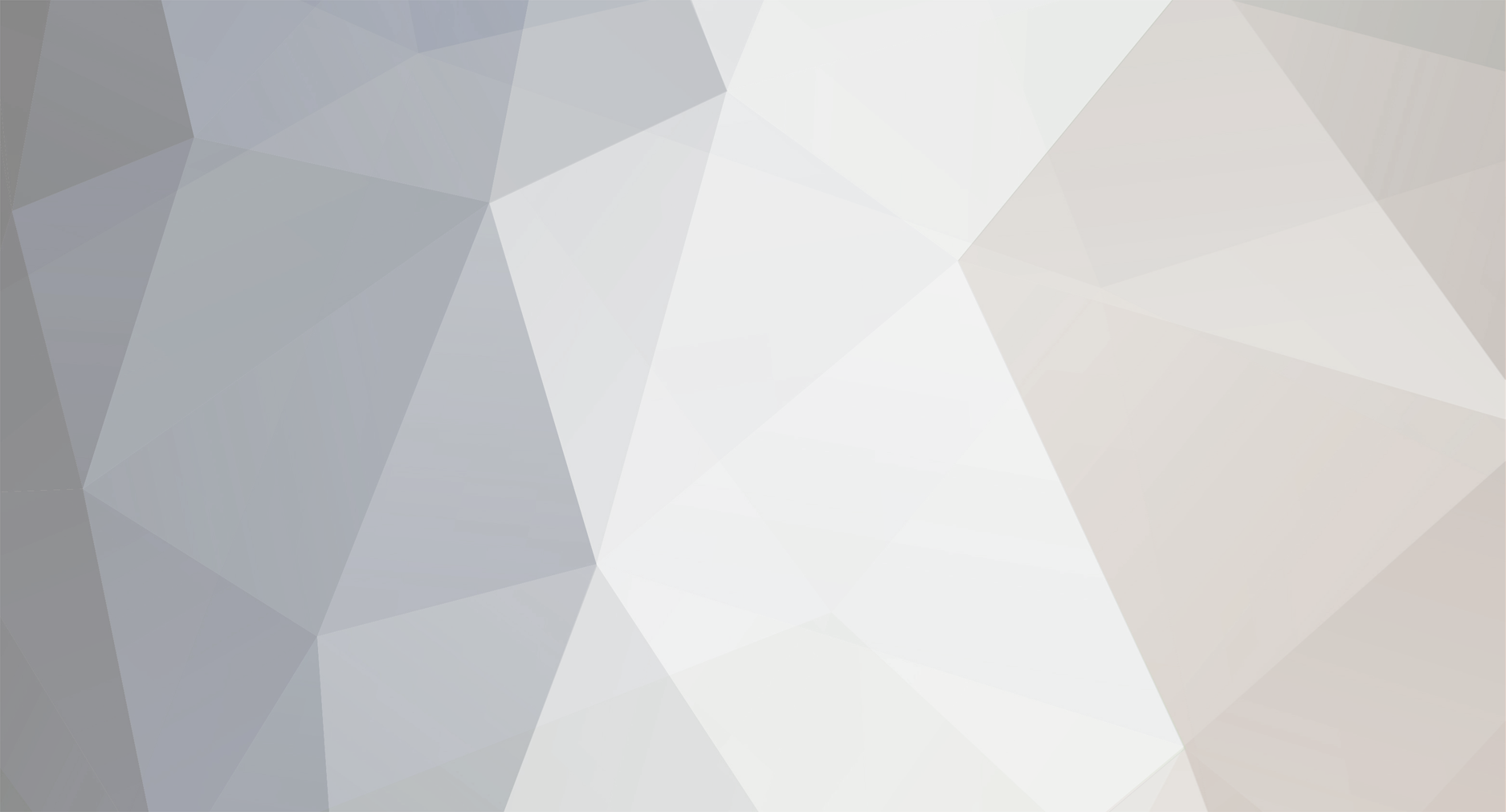
ngreenwood6
Members-
Posts
1,356 -
Joined
-
Last visited
Everything posted by ngreenwood6
-
First of all your code is really inefficient. You are querying pretty much the same data 3 different times when you could simply just use a join. Without being able to see the page its kinda hard to tell what the issue is but I have cleaned up your code a bit and hopefully it will help or resolve the issue. You may have to fix some of the variables but it should get you on the right path. <?php include "../../includes/mysql_connect.php"; include "../../includes/info_files.php"; $bottom_links = ''; $javascript = ''; $result = mysql_query("SELECT nt.id, ns.name AS sub_name, ns.url AS sub_url FROM nav_title nt LEFT JOIN nav_sub ns ON ns.titlereg=nt.reg"); $num_rows = mysql_num_rows($result); $count = 0; while($row = mysql_fetch_array($result)){ //increment the counter $count++; //build your bottom links $bottom_links .= '<a href="" class="menuanchorclass" rel="' . $row['id'] . '"><img src="http://www.controlhabbo.net/layout/images/buttons/' . $row['id'] . '.png" style="border-width:0" /></a>'; //build the javascript $javascript .= 'var ' . $row['id'] . '={divclass:\'anylinkmenu\', inlinestyle:\'width:150px; border: solid black 1px; background:#ebebeb\', linktarget:\'\'}' . $row['id'] . '.items=['; //add onto the javascript $javascript .= '["'.$row['sub_name'].'", "'.$row['sub_url'].'"]'; //add ending bracket ? if($num_rows == $count){ $javascript .= ']'; } //add a comma if there is more than one row and its not the last one add a comma ? if($num_rows > 1 && $num_rows != $count){ $javascript .= ','; } } ?> <link rel="stylesheet" href="http://www.controlhabbo.net/layout/global.css" type="text/css" /> <link rel="stylesheet" type="text/css" href="http://www.controlhabbo.net/layout/menu/anylinkmenu.css" /> <script type="text/javascript" src="http://www.controlhabbo.net/layout/menu/anylinkmenu.js"></script> <script type="text/javascript"> <?php echo $javascript; ?> //anylinkmenu.init("menu_anchors_class") //Pass in the CSS class of anchor links (that contain a sub menu) anylinkmenu.init("menuanchorclass"); </script> <div id="navbar"> <div id="navbardropdown"><?php echo $bottom_links; ?></div> </div>
-
I actually just figured it out. When I put the second statement in parenthesis it then works....Can anyone explain...I would have thought it would have stopped when it met the first condition but it seems like it was checking the second no matter what for some reason? <?php for($i=0;$i<7;$i++){ $class = ($i==0) ? 'cal_item cal_item_first' : (($i==6) ? 'cal_item cal_item_last' : 'cal_item'); echo $class.'<br />'; } ?>
-
I have a loop like this: <?php for($i=0;$i<7;$i++){ $class = ($i==0) ? 'cal_item cal_item_first' : ($i==6) ? 'cal_item cal_item_last' : 'cal_item'; echo $class; } ?> I dont see anything wrong with it but for some reason when it hits the first item it is saying cal_item_last instead of cal_item_first. Any ideas?
-
I dont know if I am misunderstanding your question or what but from what it looks like to me you dont need to do this throught mysql you can do it through php. You could create an array of words that you didnt want to be included in the search. Then you could just do a str_replace(or str_ireplace - is case insensitive) and remove those words. For example: //users search $data = "Chateau wine another x de keyword"; //search and replace $search = array("Chateau","another","keyword"); $replace = ""; //run the replace $data = str_replace($search,$replace,$data); //users search after would be "wine x de" So if you look at the code that I provided it will just remove the keywords that you dont want to be included. You can add as many as you want and you can also make the replace an array and tell it to replace the keywords with other words if you wanted to. Please let me know if I was wrong in your question.
-
Well actually your for loop is correct however because you did not use my code your code is not correct. The input fields that you have look like "username" or "password" mine looked like "username[]" or "password[]". The reason for that is that it then sends that data as a multi-dimensional array with all of the users info instead of just one user like yours. With a multi dimensional array your data would look like: Array ( [0]=>Array( [username] => cookiemonster => [email][email protected] [member] => 0 [referrer] => [level] => 1 [exp] => 0 [credits] => 50.000 [submit] => Submit Changes ) [1]=>Array(//another user here) ) That is what you would loop through to update each user. Also you didnt add the hidden field for the id so you would not be able to update the database correctly. I took the time to update and fix your code to make it more readable and made alot of fixes to the code. You may want to try using mine and troubleshooting any errors you get at that point. If you dont update your code, then I will not be able to help you and unwilling.
-
at the very top of the page do a: print_r($_POST); if you are unfamiliar with this what it does is it prints out the value of the array to the page. Make sure you submit the form before doing this or it will not have any values - Then right click the page somewhere and do view page source. You should be able to then see the values. Make sure that you are seeing the values there, then the problem lies somewhere in your loop....can you post your new code?
-
Sorry about that, I missed something. You should change the form to have a (method="post") so that they are passed in a POST instead of a GET. I added a hidden field called user_id which is the users id. The data posts all the users data like you wanted to but it posts all the data as a multi-dimensional array. Which means that it will look like something this: $_POST[0]['user_id'] = 1; $_POST[0]['username'] = username here; $_POST[1]['user_id'] = 2 $_POST[1]['username'] = username here; so with that data what you can do is perform an update query on each of the rows to update the users information while you perform a loop. It will look something like this: for($i=0;$i<count($_POST);$i++){ $update_query = "UPDATE users SET username='".mysql_real_escape_string($_POST[$i]['username'])."' WHERE id=".(int)$_POST[$i]['user_id']; mysql_query($update_query); } The important part in that is the WHERE section. The id is how you are telling it which username belongs to which user in the database. You would have to also tell it to set all of the other values but I think you can get a general sensus of what to do with the data. Before you start running queries, you can try setting up the loop and just outputting the data to the page to ensure that you are going to update the database correctly.
-
Ok here is some information that should help you. I fixed alot of your code to be alot easier to read and did this to help you so that you may in the future use these methods. I added in the ability to only delete an ban users if yes is selected, and I also added in the ability for you to be able to loop through and update the users. I have it printing out the array at this time but you should be able to loop through that data and insert what is necessary. The last one I left up to you to at least take an attempt at it. One thing of help I can give you for that is that you can use what is called pagination (google it). What I would do is set a page through the url or the session and then you can limit the query using the LIMIT keyword. Please at least attempt it and I might be willing to help further. Please let me know if you have any issues with my code. <?php session_start(); include_once('../inc/connect.php'); //set the sort counter if(!isset($_SESSION['sort_counter'])) $_SESSION['sort_counter'] = 1; //get the sort if(($_SESSION['sort_counter']%2) == 0){ $sortcount = "DESC"; } else { $sortcount = "ASC"; } //start the query $query = "SELECT * FROM users LEFT JOIN userstats ON userstats.id = users.id ORDER BY "; //get the date $today = date("Y-m-d"); //get values $sort = $_GET['sort']; $delete = $_GET['delete']; $ban = $_GET['ban']; $submit = $_POST['submit']; //check for post update if(isset($submit)){ //print the array print_r($_POST); } //get the sort type switch($sort){ case 'username': $query .= " users.username"; $_SESSION['sort_counter'] += 1; break; case 'email': $query .= " users.email"; $_SESSION['sort_counter'] += 1; break; case 'type': $query .= " users.member"; $_SESSION['sort_counter'] += 1; break; case 'referrer': $query .= " users.referrer"; $_SESSION['sort_counter'] += 1; break; case 'level': $query .= " userstats.level"; $_SESSION['sort_counter'] += 1; break; case 'exp': $query .= " userstats.exp"; $_SESSION['sort_counter'] += 1; break; case 'credits': $query .= " userstats.credits"; $_SESSION['sort_counter'] += 1; break; default: $query .= "users.id"; $_SESSION['sort_counter'] += 1; break; } //add the sort type $query .= " $sortcount"; //check if delete is set and id is numeric if (isset($delete) && is_numeric($_GET['id'])){ //delete the user and the user stats (dont need (int) because we made sure it was numeric) mysql_query('DELETE FROM users WHERE id='.$_GET['id']); mysql_query('DELETE FROM userstats WHERE id='.$_GET['id']); //redirect header("Location:users.php"); } //check if ban is set to true and id is numeric if ($ban=="true" && is_numeric($_GET['id'])){ //ban the user mysql_query('UPDATE users SET active="no" WHERE id='.$_GET['id']); //redirect header("Location:users.php"); } //check if ban is set to false and id is numeric if ($ban=="false" && isset($_GET['id'])){ //unban the user mysql_query('UPDATE users SET active="yes" WHERE id=',$_GET['id']); //redirect header("Location:users.php"); } ?> <html> <head> <title>Users</title> <style> a:link{ text-decoration: none; color: #519904; } a:visited{ text-decoration: none; color: #519904; } a:hover{ text-decoration: none; color: #4296ce; } #joined{ position: relative; width: 97px; margin-left: auto; margin-right: auto; top: -550px; } </style> </head> <body> <h2 align='center'>Users</h2> <br /> <table border='1' align='center'> <tr> <th bgcolor='#cccccc'><a href='users.php?sort=id'>ID</a></th> <th bgcolor='#cccccc'><a href='users.php?sort=username'>Username</a></th> <th bgcolor='#cccccc'><a href='users.php?sort=email'>Email</a></th> <th bgcolor='#cccccc'><a href='users.php?sort=type'>Type</a></th> <th bgcolor='#cccccc'><a href='users.php?sort=referrer'>Referrer</a></th> <!-- Level, Exp, and Credits are in the table called userstats --> <th bgcolor='#cccccc'><a href='users.php?sort=level'>Level</a></th> <th bgcolor='#cccccc'><a href='users.php?sort=exp'>Exp</a></th> <th bgcolor='#cccccc'><a href='users.php?sort=credits'>Credits</a></th> <th bgcolor='#cccccc'><a href='users.php'>Delete</a></th> <th bgcolor='#cccccc'><a href='users.php'>Ban</a></th> </tr> <form> <?php //make the query $results = mysql_query($query); //initialize recent members $recentmembers = 0; //loop through the results while($row = mysql_fetch_array($result)){ //get options $joined = $row['joindate']; $active = $row['active']; //add recent members if ($joined==$today){ $recentmembers += 1; } //set options based on ban if ($active=='no'){ $color = "#f43636"; $active = false; $ban = "true"; } else { $color = "#ffffff"; $active = true; $ban = "false"; } switch($row['member']){ case 0: $typecolor = "#eeeeee"; break; case 1: $typecolor = "#72A4D2"; break; case 9: $typecolor = "#00cc00"; break; } ?> <tr> <input type="hidden" name="user_id[]" value="<?php echo $row['id'];?>" /> <td align="center" width="40" bgcolor="<?php echo $color;?>"><?php echo $row['id'];?></td> <td align="center" width="130"><input type="text" name="username[]" value="<?php echo $row['username'];?>"></td> <td align="center" width="230"><input type="text" name="email[]" value="<?php echo $row['email'];?>" size="35"></td> <td align="center" width="10"><input type="text" name="member[]" value="<?php echo $row['member'];?>" size="2" style="background-color: <?php echo $typecolor; ?>;"></td> <td align="center" width="130"><input type="text" name="referrer[]" value="<?php echo $row['referrer'];?>"></td> <td align="center" width="10"><input type="text" name="level[]" value="<?php echo $row['level'];?>" size="2"></td> <td align="center" width="10"><input type="text" name="exp[]" value="<?php echo $row['exp'];?>" size="10"></td> <td align="center" width="10"><input type="text" name="credits[]" value="<?php echo $row['credits'];?>" size="20"></td> <td align="center" width="10"><a href="javascript:void(0);" onclick="show_delete('<?php echo $row['id'];?>');">Delete</a></td> <td align="center" width="10"><a href="javascript:void(0);" onclick="show_ban('<?php echo $ban;?>','<?php echo $row['id'];?>');"><?php echo ($active==true) ? "Ban" : "Unban";?></a></td> </tr> <?php } //end while ?> </table> <br /> <center><input type="submit" name="submit" value="Submit Changes"><input type="reset" name="reset" value="Reset"></center> </form> <br /> <div id="joined">Joined Today: <?php echo $recentmembers;?></div> </body> <script type="text/javascript"> function show_delete(user_id){ var answer = confirm("Delete User?"); if(answer){ window.location = 'users.php?delete&id='+user_id; } } function show_ban(ban,user_id){ var ban_type = (ban=="true") ? 'Ban' : 'Unban'; var answer = confirm(ban_type+' user?'); if(answer){ window.location = 'users.php?ban='+ban+'&id='+user_id; } } </script> </html>
-
Can you link us to another page where you dont have to register to see what you are talking about? That seems to me like what you wanted as well.
-
Looks to me like there is only one form on that page so the form would be forms[0] not one. Could be a problem with something else on the page but wont be able to tell until the first error is fixed.
-
In mysql you can perform select statements inside of select statements. So you could do something like this: $sql = "select idnum, lname, fname, location from employees, (SELECT something_here FROM sites WHERE location=sites.sitename LIMIT 1) AS something_here where temp <> '1' and ( idnum in ($idnumstring) AND ( idnum in (select idnum from CurrentLDAP) or idnum in (select idnum from shifts where intime >= '$start' and outtime <= '$stop')) ) and salary = 'hourly' order by lname, fname"; You will have to replace both of the something_here 's with the value that you actually want. If you notice I also put limit 1 in the query, that is because each one can only return one value. so you will have to do multiple selects to get all the values that you need. A join would be a better choice but if you cant seem to figure it out this will work for you.
-
Thats actually pretty simple. I assume you are creating your users in the database with a unique id. What you can do is when the form that you create for the voting is posted you can then insert the user that is logged in user id and vote into a new table storing there user_id and there selection. Then when you display the form if the users id is in the new table you will just display something saying that they have already voted.
-
That is awesome lol. My personal favorite about that article is the 2 MHz processor vs the 9,320 MHz processor lol.
-
yeah i would do like mike said and put it above the root directory so that there is no direct access to the file. Also a good idea would be to not actually show the file in the url for example instead of having: http://www.yoururl.com/pdf/somepdf.pdf you would have a url like http://www.yoururl.com/pdf?id=23&somerandom=2234325 obviously that is just an example but you get the picture.
-
ok so why dont you just create a class yourself? imagine if programmer out there just tried to have something done for them automatically. we would have NO good programmers. every programmer out there that i know has gone through some tedious work to validate fields. you could make a simple database class that you could pass values to to check. <?php class db{ function __construc(){ mysql_connect('host','user','pass'); mysql_select_db('db'); } function validate($array){ switch($array[0]){ case 'integer': if(is_numeric($array[1])) return true; case 'email': return eregi("^[_a-z0-9-]+(\.[_a-z0-9-]+)*@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$", $array[1]); } } } //making use of the class $db = new db(); //initialize a number $number = 23; //check that it is a number $error = $db->validate(array('integer',$number)); //check the error if($error == true){ echo 'there was an error'; } else { echo 'your good to go'; } See how easy that was and you could just extend that validate method or create one for each type of validation you needed. Then you could also create methods in that class for inserting and updating data.
-
I dont know why you would want something to do that for you when it is simple enough to do yourself. Not to mention the fact that you arent going to know if the validation is meeting what you need to validate. I do not know of any software out there that can do this and as far as I know there is no such thing. My suggestion is to write your own classes to manage your database.
-
you can try going to the url of the pdf. so if you have your pdfs like this pdf/somepdf.pdf go to http://yoururl.com/pdf/somepdf.pdf and i bet you can view it.
-
what would be the results for this for loop?
ngreenwood6 replied to co.ador's topic in PHP Coding Help
6 but it would only go to 5 -
is this what you are looking for; $cur_date = '14-12-2009'; SELECT * FROM table WHERE start_date <= $cur_date AND end_date >= $cur_date
-
Returning results before and after the actual result?
ngreenwood6 replied to Kryptix's topic in MySQL Help
Thats what I was originally thinking but I cant think of a way to do that in mysql when you are using just the orderby. If you actually had the users position you could do it but without knowing the position your kinda sol lol. Maybe someone else knows a better method but i just offered mine as a solution. -
try commenting out the line under your Meta classes getBootedServers function or better just set it to return test or something. I think the problem is with how you are trying to call that function.
-
Returning results before and after the actual result?
ngreenwood6 replied to Kryptix's topic in MySQL Help
I cant seem to think of a way to do this with the current query that you are performing but you can use php to help you display. There is a php function called array_search that you can use to search for the value in the array which will give you the key. You could then loop through that key - 50 to the key +50. here is an example: $array = array('player1','player2','player3'); $array_key = array_search('player2',$array); //$array_key will be 1 //loop through the array - 50 to + 50 for($i = $array_key - 50; $i <= $array_key + 50; $i++){ echo $array[$i]; //display the results } Obviously there is some error checking (making sure there is a key - 50 and + 50) to do here but that is a basic example. Also you will have to create a new array of the values that are output from your query in order for this to work so that they will get a numbered key. -
pass the toolbar as 0 to the iframe....if you do this the toolbar will be hidden... however user always has final control over this and can change it.....but for most users they wont know how to do it: <iframe frameborder="0" id="myFrame" src="http://www.hbo.com/guidepdf/2009-12/hbo_dec09_ee.pdf#toolbar=0" style="display:block" width="800" height="600"> <div style="position:absolute; background-color:#000;height:80px;width:800px;"></div> </iframe>
-
Returning results before and after the actual result?
ngreenwood6 replied to Kryptix's topic in MySQL Help
do you mind if I see the query so that I can see if I can give you a hand or not. -
try this: <?php class Meta { var $base; var $meta; function __construct(){ Ice_loadProfile(); $base = $ICE->stringToProxy("Meta:tcp -h 127.0.0.1 -p 6502"); $meta = $base->ice_checkedCast("::Murmur::Meta"); } function getServer($serverid) { return $this->meta->getServer($serverid); } function newServer() { return $this->meta->newServer(); } function getBootedServers() { return $this->meta->getBootedServers(); } function getAllServers() { return $this->meta->getAllServers(); } } $meta = new Meta(); echo $meta->getBootedServers(); ?>