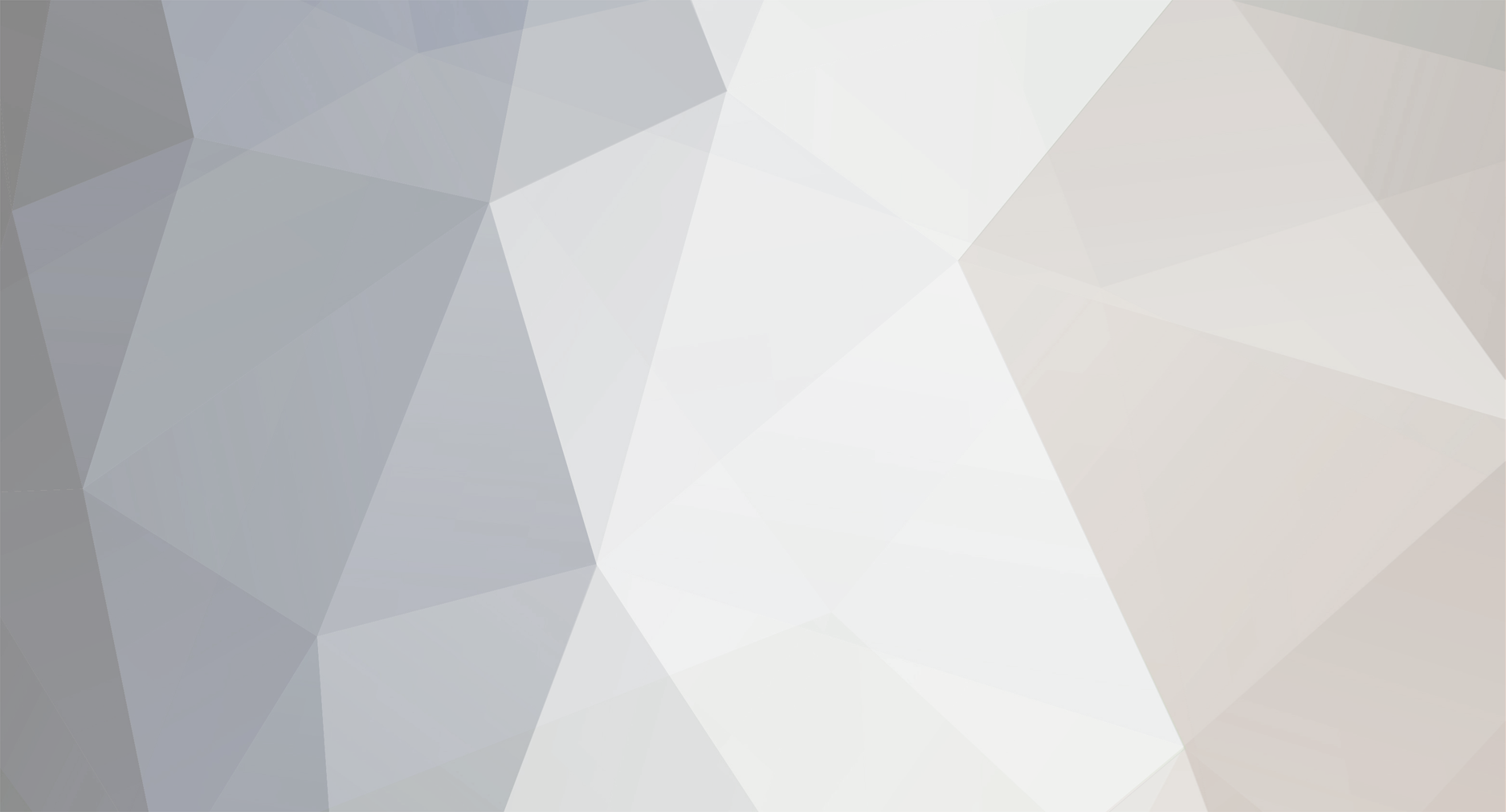
ngreenwood6
Members-
Posts
1,356 -
Joined
-
Last visited
Everything posted by ngreenwood6
-
just move what is not needed to be inside the print statement outside....try this: require_once('captc/recaptchalib.php'); $publickey = '*******************************************'; print("<form name='form1' method='post' action='create-b.php' enctype='application/x-www-form-urlencoded' style='margin:0px'> <input type='hidden' name='time' value='werwRG/443gooa22a04c0c2d46c802cc7e3b262398852$glg/WE?F'> <input name='name' value='Anonymous' type='text' MAXLENGTH='15' style='position:absolute;width:650px;left:67px;top:142px;z-index:11'> <input name='subject' value='(No subject)' MAXLENGTH='15' type='text' style='position:absolute;width:650px;left:67px;top:166px;z-index:11'> <textarea name='body' MAXLENGTH='255' type='text' style='position:absolute;left:67px;top:191px;width:650px;height:98px;z-index:11'></textarea> <input name='submit' type='submit' value='Create thread' style='position:absolute;left:614px;top:291px;z-index:11'> <div id='captcha' style='position:absolute; overflow:hidden; left:10px; top:296px; z-index:10'>".recaptcha_get_html($publickey)." </form> </div>");
-
If this is only text then you could do something like: $text = 'Your text here'; $truncated_text = substr($text,0,500); However if you have tags in there its not as simple as that because if you cut it off in the middle of a tag it may break your layout. A simple workaround for this is to remove the tags before doing so like this: $text = 'Your text here'; $text = strip_tags($text); $truncated_text = substr($text,0,500); If you need to keep the formatting then a more complex solution will need to be put in place and can be quite confusing. Hopefully this helps.
-
calling multiple functions in single call from class
ngreenwood6 replied to ngreenwood6's topic in PHP Coding Help
Thanks for the replies guys. I am actually setting up these functions to be a string builder of sort. They do not need to be called in order, when the "execute" function is called it pieces it all together. -
calling multiple functions in single call from class
ngreenwood6 replied to ngreenwood6's topic in PHP Coding Help
Thanks so basically I am doing it right. Kinda just discovered that on my own because I didnt know what to google but now I do "method chaining". Is this efficient? I assume so because $this should be passed as a reference correct? -
This is a bit of a more advanced question so I am hoping there are some advanced programmers online at this time . Anyways basically I want to be able to "queue" functions from a class in a single call and am wondering if I am doing it correctly or if there is a better way to do it. Here is my code: <?php class test { private static $one; private static $two; private static $three; public function callOne($val){ $one .= $val; return $this; } public function callTwo($val){ $two .= $val; return $this; } public function callThree($val){ $three .= $val; return $this; } public function print(){ echo $this->one.' '.$this->two.' '.$this->three; } } ?> now when I want to call this I can do: $test = new test(); $test->callOne('one')->callTwo('two')->callThree('three'); $test->callOne('another one'); $test->print(); Is there a better way to do this or is there a different method of doing this? Just wanna make sure I am doing it right lol.
-
unable to submit php form on IE browser
ngreenwood6 replied to smitagodbole's topic in PHP Coding Help
That is the correct markup and should work in ie. Maybe there is something else on the page causing the problem. Any more code we can see? -
Quotes inside double and single quotes (triple quotes)?
ngreenwood6 replied to eevan79's topic in PHP Coding Help
sorry I didnt notice that you didnt have quotes around the img tag. I never pass stuff through a function like that. Why dont you just send the hero name and the hero image path and then in the tooltip function have it so that it will just put the image path inside of the img tag inside of that function. here is an example: PHP CODE $hero = '<a onMouseout="hidetooltip();" onMouseover="tooltip(\''.$heroname.'\',\'img/heroes/'.$hero.'\.gif');" href="hero.php?hero='.$hero.'"><img alt="" width="28px" src="./img/heroes/'.$hero.'.gif" border=0></a>'; JAVASCRIPT function tooltip(hero_name,hero){ var img_path = '<img src="'+hero+'" />'; var data = hero_name+'<br />'+img_path; alert(data); } Wasnt tested but hopefully you get the idea of what I was getting at. -
Dropdown generated from Database information
ngreenwood6 replied to Ryflex's topic in PHP Coding Help
that is actually a javascript question but I will answer that as well. on your select tag you need to add an onchange event handler. so it would look something like this: <select onchange="this.form.submit();"> -
Quotes inside double and single quotes (triple quotes)?
ngreenwood6 replied to eevan79's topic in PHP Coding Help
Shows you werent using my code lol. My mouseover was encapsed by double quotes. Quoted from my previous post -
also you should be using mysql_real_escape_string() because if any of those variables have a quote in them it will also break the query.
-
I have a string like this: Something,Somthing Else,"keyword1,keyword2,keyword3",Yet another I need to split that string by the ,. However, I need the data that is n the quotes to remain as one even though it has commas because it is one item. I assume I need to use preg_split and have tried some code but none of it seems to work properly. If anyone has a better method or knows how I can split this up using preg_split the help is appreciated.
-
Sorry I think I misunderstood. I thought you wanted anything between the iframe tags left as well. I found this function on php.net in the comments for strip_tags http://us3.php.net/manual/en/function.strip-tags.php: function strip_only($str, $tags, $stripContent = false) { $content = ''; if(!is_array($tags)) { $tags = (strpos($str, '>') !== false ? explode('>', str_replace('<', '', $tags)) : array($tags)); if(end($tags) == '') array_pop($tags); } foreach($tags as $tag) { if ($stripContent) $content = '(.+</'.$tag.'[^>]*>|)'; $str = preg_replace('#</?'.$tag.'[^>]*>'.$content.'#is', '', $str); } return $str; } Example use: $string = strip_only($string,array('iframe'),true); echo $string; If you want the content between the tag to remain just change the last argument to false.
-
Quotes inside double and single quotes (triple quotes)?
ngreenwood6 replied to eevan79's topic in PHP Coding Help
I find it funny that you ask for help, I give you help, and then you try to tell me how to write code. I also find it funny that I copied exactly what I posted to you and put it on my dev server and it works just fine so there must be something that you are not sharing with us. My code is completely valid. If you notice the single quote is escaped using the \ so it does not end the single quotes. If you were referring the the quote before $hero that just exits the current string literal and puts out the variable and the on after it start the string literal back. Maybe you should post your full code or try mine again because it works perfect on my machine. -
"if (self == top)" php equivalent or alternative?
ngreenwood6 replied to Rhialto's topic in PHP Coding Help
I like PFMaBiSmAd's solution as it seems the best. Can't believe I didnt think of that but that is probaly the best solution. @pawn - the problem with that is if another variable is named that or a global is using that variable name it will take precedence over the one that you used. -
Quotes inside double and single quotes (triple quotes)?
ngreenwood6 replied to eevan79's topic in PHP Coding Help
ok so a lesson on quotes...one of my favorites....first off in any case that you can use single quotes use those as php process them faster than double quotes. here are some examples: //will not work - variables need to be in double quotes or outside of quotes$string = 'Some String $test';//correct way for example above$string = 'Some String'.$test;//another way for example above$string = "Some String $test";//yet another way for example above - method I use if I have to so that the variables dont get confused with other characters$string = "Some String {$test}";//using double quotes inside of single quotes$string = 'Some String "with a quote"';//using double quotes and single quotes inside of string - whichever quotes you start with can be used inside of itself but must be escaped using a \ (backslash) character$string = 'Some String "with some quote\'s"';//Yours fixed$string = '<a onMouseout="hidetooltip();" onMouseover="tooltip(\'<img src=img/heroes/'.$hero.'.gif\');" href="hero.php?hero='.$hero.'">Text</a>'; Hopefully that helps. If you have any questions feel free to ask. -
"if (self == top)" php equivalent or alternative?
ngreenwood6 replied to Rhialto's topic in PHP Coding Help
The defined constant is the best option that you have listed. You could also store it in a session variable if you wanted to. Store the session variable in index.php and clear it in the file.php so when you navigate to another page the variable is clear again to get set again. I would not use the eregi funcion as it is deprecated in 5.3.0 http://us.php.net/manual/en/function.eregi.php -
MySQL Sessions or Filesystem Sessions
ngreenwood6 replied to j.smith1981's topic in Application Design
Would you please clarify what you are referring to when you are saying mysql sessions and file system sessions. Do you mean storing the info in a database or in files? Or are you talking about storing the logged in users data while they are on the page? -
does converting a website restart search engines?
ngreenwood6 replied to corbeeresearch's topic in Application Design
Depending on how you do it will be how the outcome is. You could optimize the meta tags once again on the new site. All of the old pages you will want to create 301 redirects for so that when the search engine tries to go to that page it will redirect them to the correct page so that it updates itself so that it knows it has been moved. I dont think it should have too much effect as long as you do it properly. I am no expert so take this with a grain of assault and if any one who knows better please correct. -
Ok I think I have worked it out for you...try this: <?$string = 'code1<iframe id="test"> <a href="testing">test</a> </iframe>code2<iframe id="test2" > link </iframe>code3';$search = '@(<iframe[^>]+>)@i';$replace = '';$string = preg_replace($search,$replace,$string);echo $string;?>
-
I am not sure how many values you can give an enum column off hand but I am sure it is more than you would ever need. That sql builder is pretty neat that you linked to. If you want an easy to use tool to manage databases you should checkout navicat for mysql. Its a good tool in performing complex queries as it can save alot of time building them with its drag and drop builder.
-
Ok going off the example you gave instead of doing the three tables like this: Equipment_assoc (equip_ID, category_ID) People_assoc (people_ID, category_ID) Company_assoc (company_ID, category_ID) You could do something like this: assoc (link_ID, category_ID,link_TYPE) So a some test entries may be : 1,1,'equipment' 1,1,'people' So when you save it in the link table just save the type along with it INSERT INTO link_table (link_ID,category_ID,link_TYPE) VALUES('1','1','equipment') To get the data just do something like SELECT * FROM link_table WHERE link_TYPE='equipment' Also you can use an enum type for a table, in case you werent aware.
-
Thanks roopurt18....I am fairly new to regexp myself and didnt know you could use the @ symbol as a delimiter, I just escape them using the back slash but that does make it easier to manage.
-
I briefly reviewed your code but couldnt you take some of the processing out of that query and just do the processing via php. Most of the time you can and can save you a big headache of trying to figure out complex queries.
-
Not sure if I am following correctly but maybe I can at least give you an idea if I am incorrect in what I am thinking you are doing. So you have the categories table: Categories (id,name) -Food -Fun Then you have an items table (id,name) -Dogs -Golf Now you can have a third table that just pretty much stores the data of which categories those items are assigned to like this Items_V_Categories (item_id,category_id) -1 "Dogs" - hot dogs , 1 "Food" -1 "Dogs", 2 "Fun" -2"Golf", 2 "Fun" Then you can just pull the items from that table for each category. Hopefully that makes sense.
-
You could use something like (not tested): $string = file_get_contents('somefile.php'); $search = '/<iframe(.*)\/iframe>/is'; $replace = ''; $string = preg_replace($search,$replace,$string); Only problem with that is that it if you forget the ending iframe tag it will break the layout.