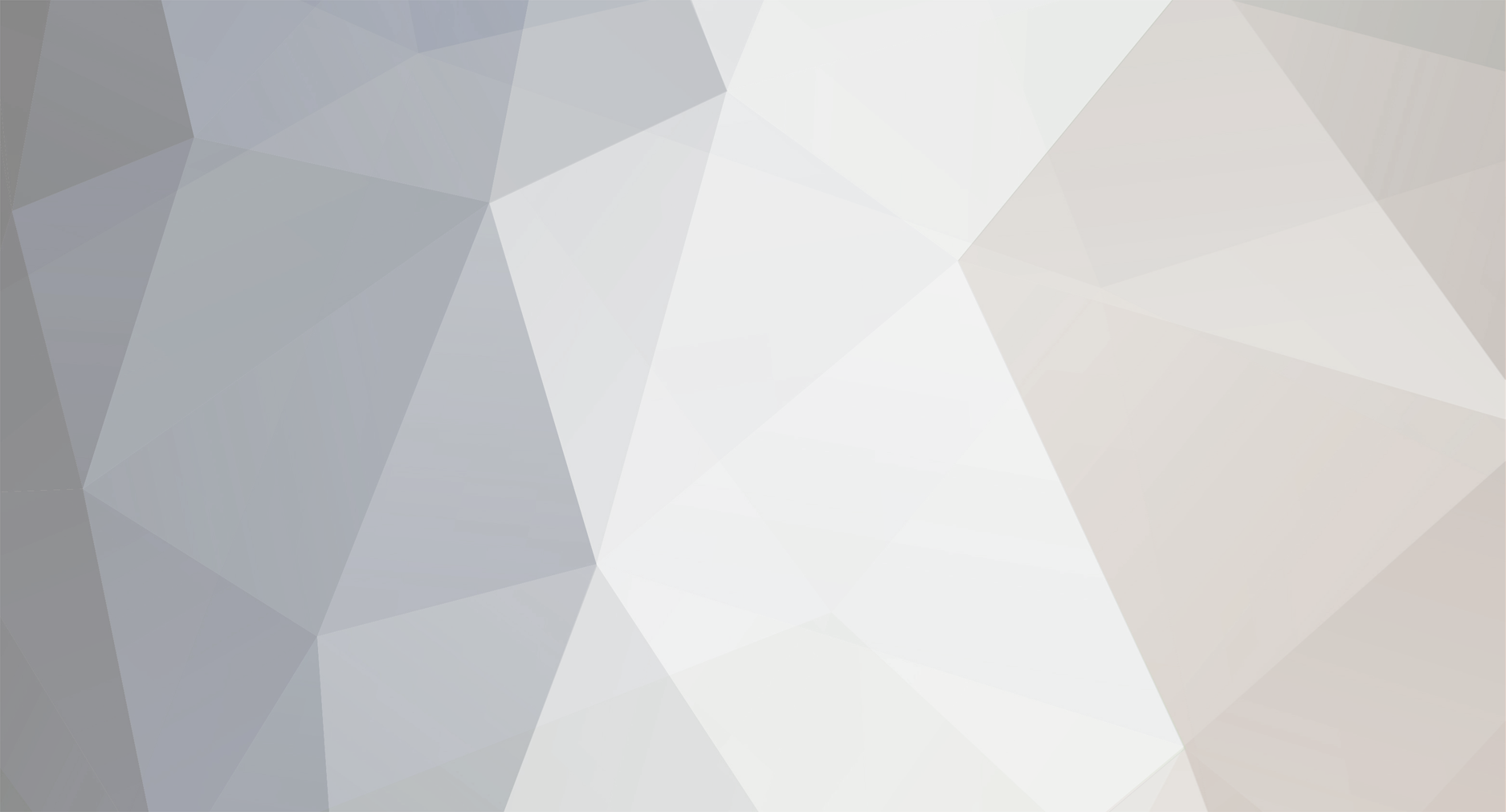
aschk
Staff Alumni-
Posts
1,245 -
Joined
-
Last visited
Never
Everything posted by aschk
-
As a quick example you don't HAVE to number your text input field boxes like field_1, field_2 etc... Just name them ALL field[] . e.g. <input type="text" name="field[]" /> <input type="text" name="field[]" /> <input type="text" name="field[]" /> <input type="text" name="field[]" /> <input type="text" name="field[]" /> This way you don't have to worry about naming them all differently. Then in your PHP you ALREADY have an array ($_POST['field']), so just do $values = implode(",",$_POST['field'])) And use $values in your SQL statement.
-
What you would have to do would be generate that same page in PDF format (using the PDFLib module), then open that page in their browser. However, there's no way to print "directly to PDF". Printing really is just an image of the text. So you might be better just to offer a print.css so it prints out nicely. The only reason to have PDF would be to pass around a digital copy once downloaded (or made available online).
-
mysite.com/username -- How to redirect to profile using htaccess file ??
aschk replied to dilum's topic in PHP Coding Help
In htaccess you would have something like this : RewriteRule ^[a-zA-Z]*$ get_user.php?username=$1 The only problem with this is that it will rewrite ALL your urls to be like that. -
[SOLVED] how is this not working???!!! simple
aschk replied to mybluehair's topic in PHP Coding Help
There are plenty of ways of achieving what you need : 1) Using double quotes (") - echo "welcome ".$one." to here"; 2) Using double quotes (") - echo "welcome {$one} to here"; 3) Using double quotes (") - echo "welcome $one to here"; 4) Using single quotes (') - echo 'welcome '.$one.' to here'; 5) Using sprintf replacement - echo sprintf("welcome %s to here",$one); 6) Using printf replacement - printf("welcome %s to here",$one); 7) Using print - print "welcome $one to here"; Hope those help identify your options. -
Of course it's possible: <?php $to = "theemail@address.com"; $subject = "whole page"; $message = file_get_contents("htmlpage.html"); mail($to,$subject,$message); ?> You'll probably want to be setting the content headers for the mail function to html, but there's nothing different to a normal mail function call. If i'm missing something here (i.e. not understanding your requirements) let me know.
-
As far as I know, ReflectionClass is buggy (as of 5.1) and hasn't had a stable release yet? Btw this is a question rather than definitive answer. I also thought that Reflection would only get public variables. Or at least that was the problem I was having with it last time I used it. Hopefully that's all fixed now. I do feel however that you might as well make the internal members public if they are needed to be accessible, else you are sort of circumventing the reasoning behind member typing. Might as well go back to PHP 4 I don't know for sure as i can't see what it is you're attemping to do.
-
That last part if supposed to be a header Take it out and i bet it works. You probably need to add \n or \n\n at the end of it.
-
Would PHP and MySQL be the best for this type of application?
aschk replied to nscherneck's topic in PHP Coding Help
I think the major part of the project is the code conversion, as you'll be moving from a stateful (VB) desktop environment to a stateless web environment. Although if you just ditch the VB code and start from scratch i gues you can design it however you want. The first process in your changeover should be to convert the database. This should be a relatively simple job as you can just dump the database in SQL text format and reimport it into MySQL (with some minor alterations I expect). Or maybe you want to restructure the database entirely (depending on current flaws). Also to make this a nice staged change you could alter the VB code to utilise MySQL first, then develop your PHP app while your VB system runs from the new database system. -
Adding to $_POST array is not reflected in $_REQUEST array
aschk replied to snype's topic in PHP Coding Help
No there isn't a function to do this, but you could rewrite the $_REQUEST superglobal to be a reference to $_POST, but then you lose all the information from the $_GET inside it. Really there should be NO reason under the sun to do this, but what the hey, here's the code so you can mess your scripts up and then ask why they don't work as expected $_REQUEST =& $_POST; -
Haha, yeah I know. Thought i'd get some patterns into the mix there. Quite a lot to digest, but i've checked it and the example works ok when run. So hopefully we can start opening up some good discussions on patterns in PHP soon.
-
[SOLVED] I really don't understand abstract classes
aschk replied to Demonic's topic in PHP Coding Help
Ah, hate to be sticking the knife in here, but it's very important to make sure you declare internal variables correctly. I realise you've posted an example but for clarity's sake I think they have a valid point. Just worth pointing out to n00bies that when you inherit from another class, ONLY protected and public variables are inherited. Any in the base class that are private are NOT accessible. Either provide a protected or public accessor function getErrorMsg(), allowing you to grab the internal base variable $error_msg. It's a common pitfall so definitely mention-worthy. -
Lol, sounds a bit like intrusion to me. So you want to have your website read the clients pc and see what they're doing, files they're accessing, system processes they're running? A crackers dream
-
[SOLVED] no data being transferred with my php script
aschk replied to stupid girl!'s topic in PHP Coding Help
Yeah I thought so , having just checked your script and your phpinfo information. You're using the much antiquated global variables, so when you submit a form you're expecting $firstname to be a variable. This is OLD syntax and I hurriedly recommend you move onto the newer format which has been present since 4.1.0 . Use $_POST['firstname'] instead of $firstname. Currently your php information shows that you have register_globals set to "Off" and too rightly too. It's a security nightmare. Start using the correct $_POST & $_GET parameters now please. No wonder it didn't work, we all thought you were converting your local variables like $firstname = $_POST['firstname'] , lol -
What you're trying to stop is someone just typing the URL directly into their browser right? So if they type it into their browser answer me these questions : 1) Are they performing a POST action? 2) If they are what does isset($_POST['SellHouse']) equal? 3) If they are NOT what does isset($_POST['SellHouse']) equal? 4) What is FALSE && TRUE? 5) What is FALSE && FALSE? 6) What is TRUE && TRUE?
-
How about you modify your code slightly for a minute and see. Change it to this : if (true && $_SESSION['referer'] == 'estateagents.php'){ echo "OMG, both my tests evaluated to true"; }else{ // Redirect another page. $_SESSION['referer'] = 'invalid'; header("location: estateagents.php"); } I think you'll find that may well work.
-
The reason your code is redirecting is because one of these two is NOT true (i.e. false), you work out which 1) isset($_POST['SellHouse'])) 2) $_SESSION['referer'] == 'estateagents.php'
-
[SOLVED] no data being transferred with my php script
aschk replied to stupid girl!'s topic in PHP Coding Help
That's interesting, considering my code is only about 10 lines long... Put all that code ONLY into your sendemail.php file. Backup your old one and run mine in it's place. Also you might like to put this at the bottom before the last ?> print_r($_POST); This will tell you what you have in your post from the previous form. -
Well, is isset($_POST['SellHouse']) true ? And what does $_SESSION['referer'] equal when process.php is run? How about you echo it out and found out (comment out the header so you don't redirect...)
-
[SOLVED] no data being transferred with my php script
aschk replied to stupid girl!'s topic in PHP Coding Help
Why not just try something simple in your sendemail.php script like : <?php $to = "youremail@yourdomain.com"; $subject = " my subject "; $message = " blah blah blah $_POST['firstname'] "; if(mail($to, $subject, $message)){ echo "mail sent"; } ?> At least this way you're going to see the firstname -
[SOLVED] Calling variables in an extended class.
aschk replied to noidtluom's topic in PHP Coding Help
I think you misunderstand inheritance. There you have created 2 DIFFERENT objects, one of type foo and another of type bar. Inside foo there is a variable called asdf, by calling test('lol') you are setting asdf inside foo ONLY to 'lol'. Now inside bar you also have a variable called asdf, which when you do $bar = new bar() is set to nothing (null), you then call anothertest() which grabs that internal variable from $bar and echo's it. It's value is null there nothing is output. What you really want to do is : $bar = new bar(); $bar->test('lol'); $bar->anothertest(); How does this work? the bar class inherited the method test(). Understand any better now? -
Here's something to ponder over: Let's try the composite + iterator design patterns <?php // Exception for Unsupported Actions. class UnsupportedAction extends Exception { public function __construct(){ parent::__construct("This action is unsupported"); } } /** * Abstract html component. * */ abstract class htmlComponent { public function addComponent(htmlComponent $component){ throw new UnsupportedAction(); } abstract public function getHtml(); } /** * Concrete implementation of html page. * */ class htmlPage extends htmlComponent { private $name; private $components; public function __construct($name){ $this->name = $name; $this->components = array(); } /** * get the Html. * */ public function getHtml(){ $html = "$this->name<br/>"; foreach($this->components as $component){ $html .= $component->getHtml(); } return $html; } /** * Add an html component to our composite. * * @param htmlComponent $component */ public function addComponent(htmlComponent $component){ $this->components[] = $component; } } /** * Concrete implementation of html div. * */ class htmlDiv extends htmlComponent { private $html; public function __construct($text){ $this->setHtml($text); } public function setHtml($text){ $this->html = "<div>".$text."</div>"; } public function getHtml(){ return $this->html; } } /** * Concrete implementation of html p */ class htmlP extends htmlComponent { private $html; public function __construct($text){ $this->setHtml($text); } public function setHtml($text){ $this->html = "<p>".$text."</p>"; } public function getHtml(){ return $this->html; } } $test = new htmlPage("my page"); $test->addComponent(new htmlDiv("some text")); $test->addComponent(new htmlDiv("more text")); $test->addComponent(new htmlP("futher text")); $test->addComponent(new htmlP("and some more")); $test->addComponent(new htmlDiv("another div?")); echo $test->getHtml(); ?>
-
I think you just amply demonstrated that method chaining for no good reason can be counter-intuitive and introduce errors
-
In answer to your question regarding "r" and "d". These are alises to the database table, i.e. shortnames.
-
The reason to avoid using distinct is that most people don't understand how it works, and also that it involves a roll-up after a roll-out. Here is your query without a JOIN: SELECT r.id AS 'ID' , r.date_when , (SELECT name FROM division d WHERE r.div_id = d.id ) as name , r.head_host , r.category FROM report r WHERE DATE(r.date_when) BETWEEN '{$start_date}' AND '{$end_date}' ORDER BY r.date_when DESC