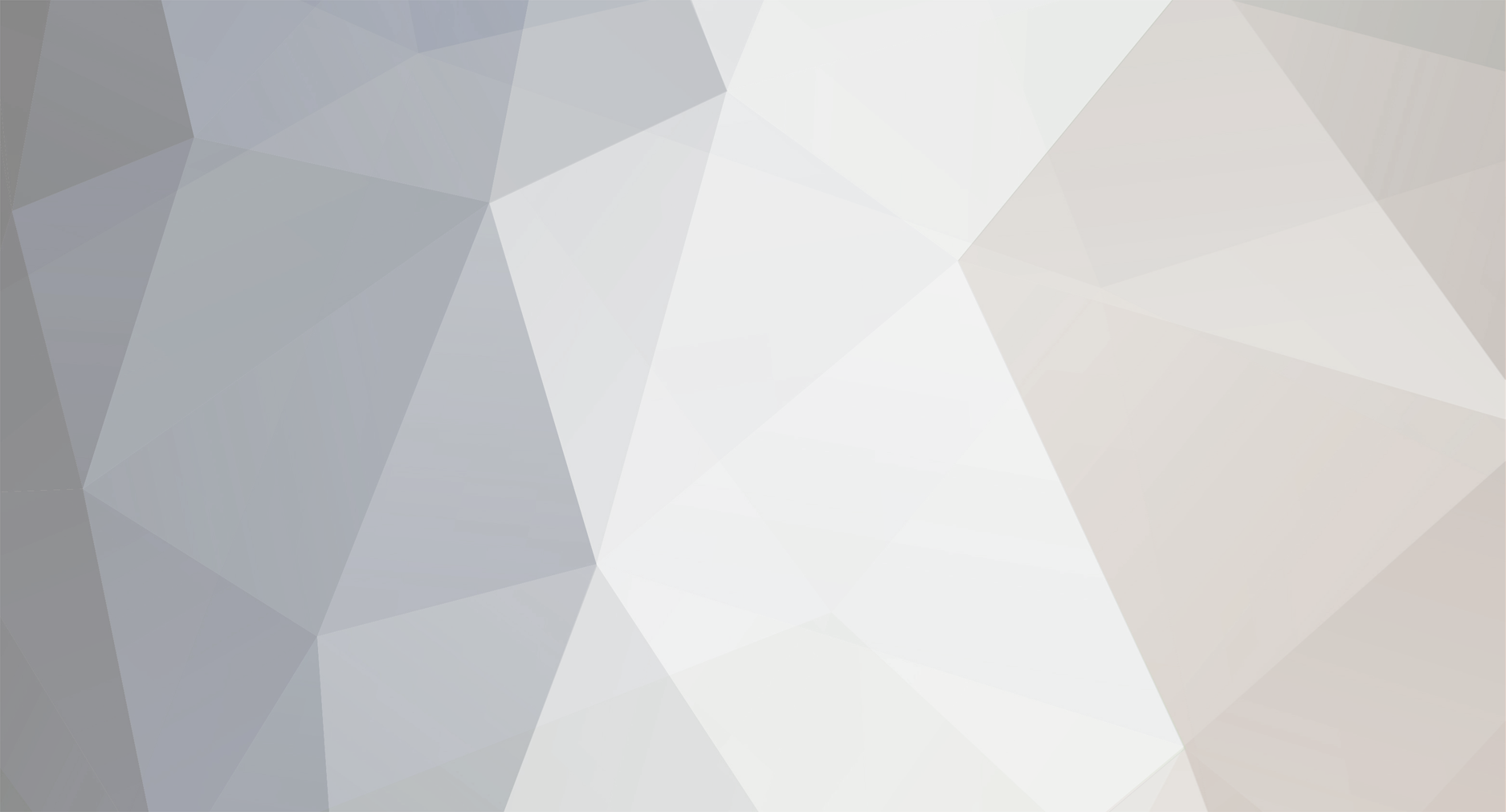
alexweber15
-
Posts
238 -
Joined
-
Last visited
Posts posted by alexweber15
-
-
Being a purist, I'm not an __autoload() fan, so I'm not the one to ask.
[rant]damn purists![\rant]
dont even know anything about it to shed some light on the debate?
seems like it could be awfully useful
-
you might be right...
hey, since you've killed every question i've thrown at this board so far and your on the prowl ATM, care to settle this one please??
http://www.phpfreaks.com/forums/index.php?topic=214757.new;topicseen#new
-
I think it's better to use spl_autoload_register().
For example, I have this code
function classAutoload($class_name) { require_once("class.".$class_name.".php"); } spl_autoload_register('classAutoload');
in a file called autoload.php, which I require at the beginning of each php file that might use these classes.
correct me if i'm wrong but isn't the exact same thing as I posted at the start of the thread?
AFAIK spl_autoload() is basically an alias to __autoload() if you don't change any parameters or behaviors via the other sp_autoload_etc....() functions... also calling it every time you might use a function kinda defeats the purpose IMO....
in fact, you just basically created a function that does the exact same thing as __autoload() originally does and used spl_autoload_register to overwrite the __autoload() function cache...
just did some reading up on the manual:
http://www.php.net/manual/en/function.spl-autoload.php
void spl_autoload ( string $class_name [, string $file_extensions ] )
By default it checks all include paths to contain filenames built up by the lowercase class name appended by the filename extensions .inc and .php.
so if i understood this properly:
1) its NOT case sensitive
2) the advantage of using spl_autoload() instead of __autoload() is that you can customize it further:
Example:
; spl_autoload($classname,'.class.php');
Again, please correct me if i'm wrong, but inserting the above line anywhere in your code will make sure that if you try to create an instance of $classname not included/required anywhere in your code it will look in your include_path for matching files with $classname."class.php" ... right?
thus eliminating the need to explicitly create the __autoload() function and with no other code necessary?
thx
-Alex
-
Well, sure, but.. then you add complexity. If there's a good reason to add this complexity (I'm not sure there is, hence my original post,) no one is going to fault you for doing it.
In the end, it's your decision to make. I'm just trying to get you to make the right one
i know and i appreciate it!
...plus all those stars under your name suggest you know what you're talking about...
plus i just ran into this nice snippet of code that iterates through class attributes in exactly the same way as i hoped to do with an array, thus rendering the array approach completely useless
class MyClass { public $var1 = 'value 1'; public $var2 = 'value 2'; public $var3 = 'value 3'; protected $protected = 'protected var'; private $private = 'private var'; function iterateVisible() { foreach($this as $key => $value) { echo "$key => $value\n"; } } } // print only public attributes $foo = new MyClass(); foreach($foo as $key=>$value){ echo "$key => $value\n"; } //print ALL attributes $foo->iterateVisible();
didn't realize you could use foreach to iterate through an object's attributes
thanks again for your attention!
-
been reading about the wonders of __autoload and spl_autoload and they sound great specially if you have lots of classes....
two questions:
1) is it case-sensitive?
function __autoload ($className) { require_once ($className.'.class.php'); }
so for example i need a class called "Product" but the source code is in a file called "product.class.php"... will this work? or should i name my .class.php files in the same case as the actually class declaration?
2) WHERE do i define it????
in the first .php file that gets executed? (index.php) or what?
assume i use a 3 layer design, should i define it at the top of the business/logic layer?
thanks... !
-Alex
-
thanks for the links
-
KeeB thanks for the detailed reply dude!
i completely agree with you on all points but fail to see how this impacts the decision on whether or not to store the data as a private array or as multiple private variables?
the concept of storing private attributes and public get and set methods remains in either case... !
-
sounds good!
i usually find that storing in an array is easier to manipulate in the case of repetitive operations and whatnot, also helps when storing in a database if you name they assoc array keys properly
-
his main argument was talking about project layers and that the logic layer should communicate directly with the DAO layer, passing back and forth the data objects and that the GUI layer should have no knowledge of how the DAO layer works, interacting directly with the logic layer and the data objects passed to it.
i agree with him on that point.
he also mentioned for example that if i were to create a separate branch of development such as creating a web-service (this is a dynamic website) i would run into problems because of the excessive dependancy on the Lead class and the lack of cohesion.
-
in a nutshell i had the following classes planned out:
abstract class Lead{ $attributes=array(); function __construct($params){ // if params is array copy to internal array attribute } // some other abstract and other not methods like validation for each attribute, getters and setters and so on } class InsuranceLead extends Lead{ // basically the same functionality as a Lead with 1 additional attributes } class FinanceLead extends Lead{ // basically the same funcionality as a Lead with 5 additional attributes }
so basically it made sense to me to make both InsuranceLead and Finance Lead inherit from Lead because they share most of the same attributes and also common getter and setter methods
I recently had to have this diagram reviewed by a veteran VB.NET coder and he crushed it saying that the dependance on the parent Lead class was a fundamental weakness and that instead I should have the two seperate classes (FinanceLead and InsuranceLead) and have the original Lead object as an attribute instead of superclass.
Is this a good idea? It kinda makes sense but I kinda just gotta let it settle in a bit and think about it before taking any action...
ANY feedback is GREATLY appreciated, thanks!
-Alex
-
just wondering what the consensus on best practices for storing a multitude of class attributes is?
for example i have a Person class that has the following attributes, age, height, hair color, etc the basic php tutorial stuff..
is it better to store the attributes as individual variables, ie:
public $age, $height, $hairColor;
or in an array, ie:
public $attributes = array();
$this->attributes[age] = xxxx';
$this->attributes[height] = xxxx';
$this->attributes[hairColor] = xxxx';
i realize that using an array is more practical in terms of traversing the attributes and performing operations to them collectively such as validation or strip_tags(), etc... but is there any performance downside?
thanks!
Alex
-
Hi all,
I'm working on a simple shopping cart implementation for learning purposes.
I have a Product Class and a Shopping Cart class, each in a seperate .php file
Naturally, the Shopping Cart Class is a collection of Product Objects.
Now in the Shopping Cart Class at some point I'll need to call Product Object methods, how does this work as far as includes go?
Where do I include the Product class in my Shopping Cart code? Or by including both classes in my index this isn't necessary?
Thanks!
Alex
where to define __autoload() function???
in PHP Coding Help
Posted
duly noted!
thanks!
(gonna hold off on using this feature for now)