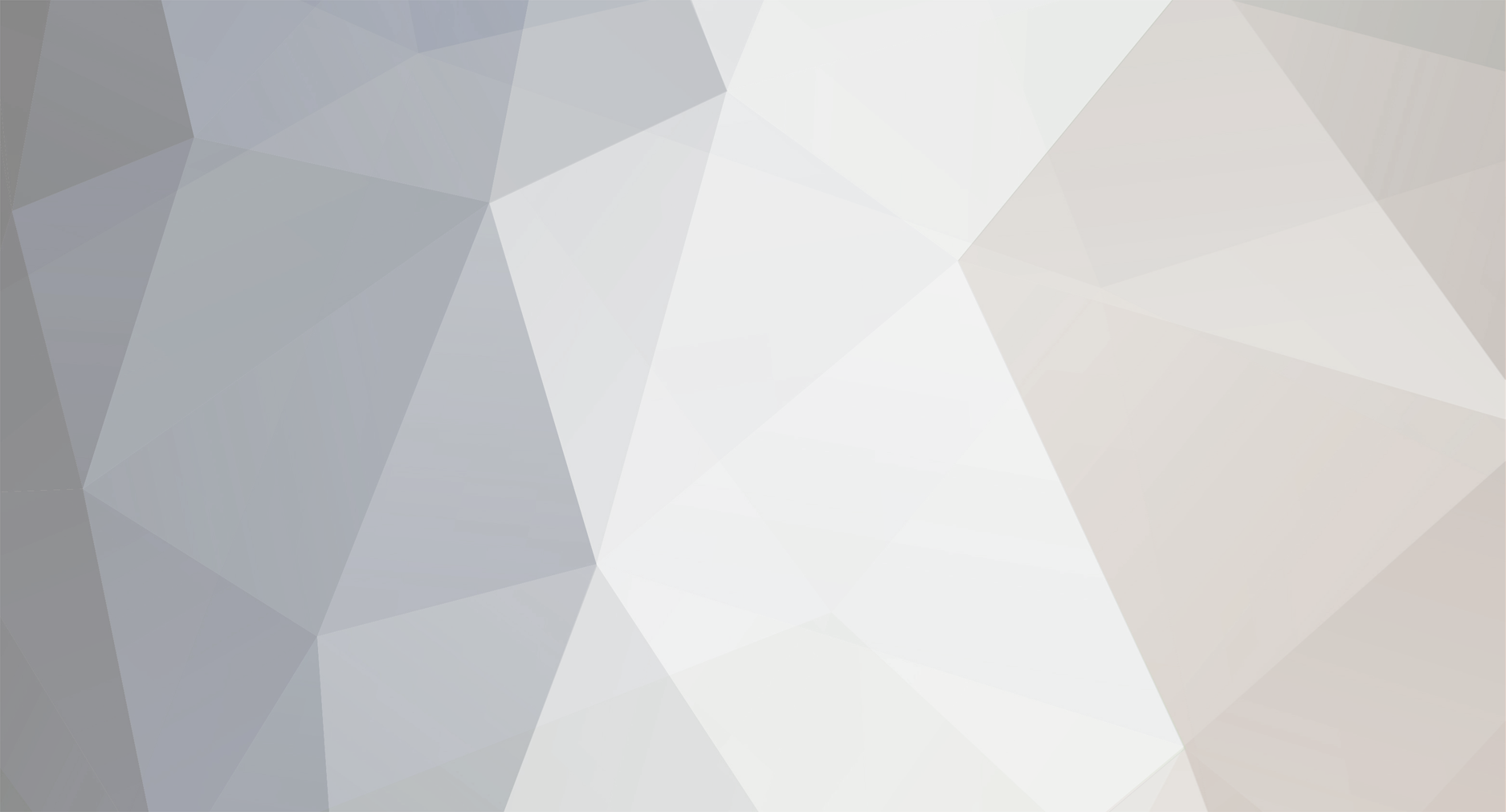
alexweber15
-
Posts
238 -
Joined
-
Last visited
Posts posted by alexweber15
-
-
thanks im gonna try the exceptions for error-checking...
but what about the fact that it autocommits anyway? yeah mysql has it on by default but according to the docs if you explicitly call PDO::beginTransaction() it will turn off autocommitting until PDO::commit() is called.
in this case even without the commit() at the end it still commits them on-the-fly as in if i kill the script right after the foreach (or even in the middle of it) it will have created the tables without me having to call commit()....
??? ???
-
learning about transactions, prepared queries and whatnot but i cant get transactions to work...
$test = new PDO(...); $test->beginTransaction(); $query[] = 'INSERT x'; $query[] = 'INSERT y'; $query[] = 'INSERT z'; foreach($query as $statement){ $test->exec($statement); } $test->commit();
thats what i wanna do... but it just executed the statements directly without waiting for commit() to be called...
what am i doing wrong??
also it doesnt return any errors or nothing, just stops silently whenever an exec statement fails... is there any way to find which statement failed?
i tried:
$test->exec($statement) or die(var_dump($test->errorInfo()));
but it just printed an empty error array after the first insert and if i removed the "or die" it then worked normally...
im kinda confused!! (using mysql btw)
thanks
Alex
-
one of those situations where I can't decide between 2 similar ways of doing something so just wondering about efficiency and best-practices... thanks!
class LoginHelper{ private $email; private $pass; function __construct($email, $pass){ $this->email = $email; $this->pass = $pass; $this->validate() ? $this->authenticate() : $this->showErr(); } private function validate(){ if(!eregi("pattern here",$this->email){ return false; // for the sake of simplicity } return true; } private function authenticate(){ $result = new LoginController($this->email, $this->pass); } }
this is the way it is right now because i'm considering renaming the functions to something a bit more generic and make them all implement a 'Helper' and 'Controller' interface ('login' is obviously just a small part of the entire thing)
another way of doing this would be not storing the values in the class and instead just pass them on to every function ie:
private function validate($email, $pass)is there any particular reason why I should use one or the other approach?
(apart from having less specific function parameters so that I can generalize and make it so that every Helper class has to have a validate() method for example and do some design by contract stuff for the other classes)
thanks!
-
-
ZF is intense and intimidating unless you know what you're doing/looking for, but very solid. It also doesn't hold your hand structurally - so you can use the components it provides how you see fit, rather than having a rigid structure enforced out the box. My biggest gripe (which also could be (and has been pointed out to be that it could be) considered a positive) is some of the classnames are very large, so I find it fairly easy to get lost or confused. However - I've not played with it extensively aside from the introduction tutorial (which WAS easy to follow, granted) so this is more than likely just a case of yet another learning curve rather than any fault of the framework.
i second that... i started trying to learn it for a project im working on but realized as few things: 1) its probably the most solid out there (although this isnt based on personal experience but rather on stuff ive read and others experiences) 2) the learning curve is steep enough that i had to back out and do this project with that i already have available in my personal library because there was no way i could learn ZF and still have time to finish the job on time
-
just recently started trying to learn Zend Framework although I've heard great things about CodeIgniter and CakePHP...
oh and for js.... jQuery!
i've been programming PHP for 2 years now but i've only started getting into the OO stuff and design patterns and whatnot relatively recently so i've been staying away from Frameworks to make sure I had a solid foundation before learning something new
-
Hey its not hard at all
Actually, as a rule of thumb you should always validate user input before doing anything with it because that's the easiest way for anyone to mess with your script... just have something like this at the start of the form handler for example:
if($_POST['email']==''){ // empty email entered // send the user back and print error message... you can do this in a number of ways for example with sessions if you're using them: $_SESSION['errmsg'] = 'email is required'; header('Location: formurl.php'); }
theres tons of other better or worse ways to do this for example if you're not using sessions you could just pass the error message back via GET
header('Location: formurl.php?errmsg=invalidemail');
and back in the form page have something to check for an error message and display it if necessary:
if(isset($_SESSION['errmsg'])){ echo $_SESSION['errmsg']; }
this is just a simple example hope it'll help to get ya started!
PS:
- if you know any javascript its always a good idea to validate client-side before sending stuff over
- you should really validate and clean ALL fields (except maybe select boxes) before doing anything with them, i'd be happy to show some more examples if you want just dont wanna make this post too long!
-
$_FILES is a superglobal so it should be available anywhere in your application's scope, as in you don't really need to pass it to the function because it just exists in the global scope anyway
-
I hardly ever use exceptions besides DB stuff.... hehe
lol same here!
-
I personally use exception [the way I'm understood they're supposed to be used] only in the case that something out of the ordinary happens. For example, if a DB connection fails I would throw an exception. On the other hand, if user input didn't validate, I wouldn't since that is expected.
you're right as far as the theoretical part goes... Exceptions should in fact be used! I just feel that they're not as polished as in other OO languages so far but from what I've read in PHP6 they should be better. It's also good practice to get into it right now so that when they do become less "bulky" you don't have to play any catch-up
-
true enough
i avoid using Exceptions unless i really have to... if statements might be less elegant but are definitely more efficient
but i guess it comes down to personal preference in the end
-
"the way i see it whereas type hinting might be useful for documentation purposes as it makes it more obvious what kind of type you expect the passed parameter to be, the fact that it generates a fatal error = less flexibility."
Less flexibility is good sometimes. If you're expecting an object a with methods B and you get an object b with methods C such that C != B, you have some major problems if you try to use a method from B that does not exist in C.
true, but with instanceof you could also avoid the fatal error and throw an exception instead for example
-
-
thanks, i guess its all good, since im gonna have to load the data everytime i might as well store it somewhere so no need for cronstuff just yet
Why wouldn't you pull this from a database?
overhead? i'd hate to have to query the db every time someone new visited the site... how about caching or serializing the query? or maybe reading from XML or something... better for a small amount of data and concurrent reads afaik!
Ever been to Google? You know when you type in their box, and they do an auto complete, those are database hits?
You know when you go to Ebay, and search for anything, there's tons of database hits?
You know most Oracle/Postgres databases can hit hundreds of thousands of transactions per second?
Overhead. lol..
i guess they have just a tiny comparable advantage over me in terms of server capacity compared to my shared hosting
but yeah whereas mysql can handle tons of hits a second i'd rather do something like Zend does and store the application settings in an ini file or something instead and save that extra DB juice for when i need it
-
thanks, i guess its all good, since im gonna have to load the data everytime i might as well store it somewhere so no need for cronstuff just yet
Why wouldn't you pull this from a database?
overhead? i'd hate to have to query the db every time someone new visited the site... how about caching or serializing the query? or maybe reading from XML or something... better for a small amount of data and concurrent reads afaik!
but thanks its coming together a bit more now...
follow up:
so thumbs up for storing system state in a DB and/or File and retrieving it and creating a Singleton with the info every time a user signs in! i guess the same applies to his personal preferences, although i could also just fetch the stuff when i perform the 'log in' query and stick it in a $_SESSION array or something...
-
Personally type hinting is better, it allows for interfaces/abstract classes to be implemented with stricter controls, and will error early giving you advanced warning of production code that might not function. Also, if you need to create 20 classes and each one has a "instanceof" check in it, are you 100% sure you're going to right the if/else statement and handle the errors correctly each time?
If it doesn't conform to the type hint then you really don't want your code to function at all, because it's usually an object dependency that'll have knock-on affects.
Just my 2 pence.
fair enough! i personally prefer type-hinting too but I've had one or another situation where an object failed to get properly instantiated and then i got a fatal error but i guess that's just PHP for "create your damn object properly next time"
-
got myself in a bit of a knot here trying to figure this out...
say i have a "real-time" system like an auction or game or whatever, something where i need to constantly know the state of the entire system, or at least parts of it. lets assume an auction for the purpose of simplicity:
the auction ends in 24 hours and only 10 products are left.
how would i keep track of this info for all visitors? i read about using singletons to store this kind of stuff but they are only as good as the user's session, so basically if i had 200 visitors at any given time then i'd have 200 seperate singleton instances... all containing the same information... sounds silly to me!
maybe i could write to a file and read off it, but either way thats still one file read for every user... i guess each new user has to get the information from somewhere but isn't there a way to have like an uber-global singleton that resides in the application's scope that every user could just read from or something? or any way to not depend on active sessions to keep track of the system's state... know what i mean?
basically as far as i know when the last user logs out thats it, the application doesn't have a life of its own... ???
-
Ah thanks a lot
!! I was almost disappointed from PHP not to have such a feature. Is this the common way to implement methods outside the class? Or professionals just type them in the class itself.
There's another reason for this than elegancy, in C++ if you implement the method inside a class it's an inline function and outside the class it's not inline (don't know if such a concept is in PHP at all)
as far as i know there's no such concept in PHP... C++ is kinda weird in that sense having stuff like multiple inheritance that almost no other languages have...
in PHP you can have similar functionality using interfaces and/or abstract classes, the key difference being that in an interface you can only specify method signatures and you implement it and in an abstract class you can specify properties (variables) and also actually have full method bodies that you can either overload or just use as is without defining it in a subclass that extends the abstract class.
in both cases you can't instantiate them directly though.
-
do you mean "extensions" maybe?
as in stuff that adds additional functionality to the PHP core, like SimpleXML, Mysqli and whatnot?
if not then you probably mean frameworks... either way hotscripts is a good place to start looking, there's a lot of already made classes and stuff for creating video galleries and stuff like that
-
Lol, glad I could help. Was there any particular reason that you wanted to do this little test? =P
no really it was more of a learning exercise, just testing out some not-so-well documented OO behaviors
-
sounds like magic quotes is on on linux
-
Any luck?
hey thanks for your reply and effort!
I get it now... makes sense! I thought that somehow having an instance of class Foo as a property would allow class Bar access to its protected methods but now that I think about it its the other way round...
as in: if Bar contains an instance of Foo, then Foo should be able to theoretically access Bar's protected variables, because they're in the same scope... but then again i can't think of a way to test this because Foo would have no knowledge that it is one of Bar's properties to begin with
and thats a bit too much Foo and Bar for one thread
-
Not at all... in 'good practise,' you should have very few variables actually sitting in the global name space, and the chances of them colliding with PHP predefined is extremely low.
Usually I see $_varname being used in an object, which should eliminate the possibility of collisions.
In the global space, you should use $_prefix_varname.
wait so what you're saying is that when you're told to avoid using underscored to prefix variable names it only applies to the global namespace? still don't see the point of using that particular prefix when theres so many other possibilities like just, god forbid, naming variables according to what they store
-
I mean manually pass $this instead of self inside of the function.
yup thats what i did...
i don't get the "not foo" error anymore (so its analogous to doing what i did in ex2 except its done inside the first class instead of outside all classes) but i still cant access the private/protected properties...
again, i realize this is probably not very good practice because of the really strong coupling but I'm kind of disappointed you apparently can't do this...
PDO always autocommits even when beginTransaction() ??? wtf!
in PHP Coding Help
Posted
InnoDB cause of the FKs...hmm never occurred to me but afaik its actually MyISAM that can't disable autocommitting...