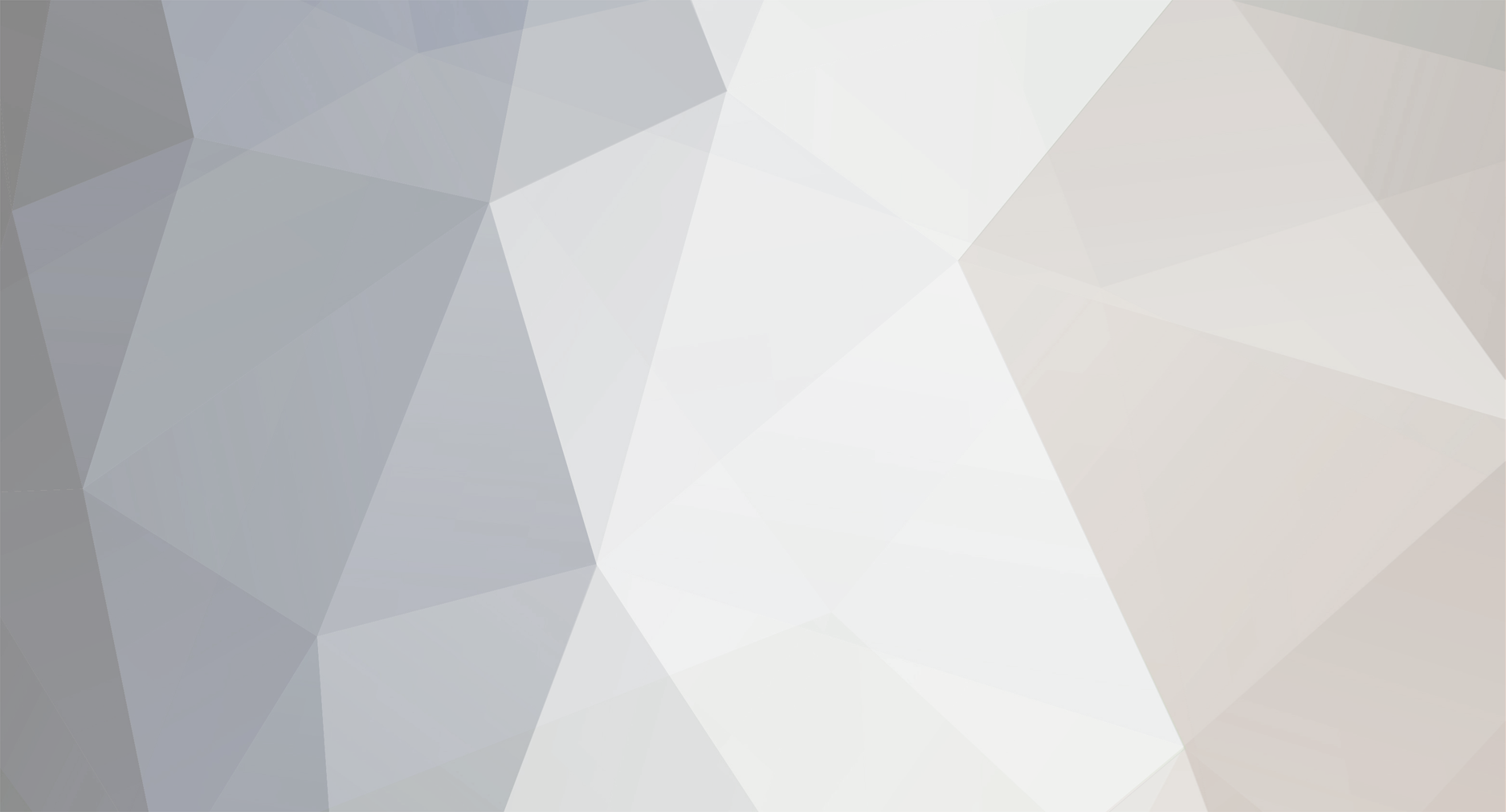
alexweber15
-
Posts
238 -
Joined
-
Last visited
Posts posted by alexweber15
-
-
CroNiX probably nailed it!
(i woulda said the same thing but im cool because i use jQuery so it would be $('#dataxml').val(); )
Although i'm baffled as to how it works in all other browsers...???? ???
-
Will, I'm surprised you didn't get any header-related errors!
The problem is that you echo first and redirect after
So basically what happens is that its so fast that you won't see the echo at all.
If you are using Sessions, store the message in a session and then if the corresponding Session variable is set after reloading, echo the message.
If not, you could use GET to pass the message and it would be a similar implementation.
Example using GET for simplicity but I recommend Sessions instead.
// handles posts if($_POST){ // do all your stuff if( // condition met ){ $message = 'thank you'; }else{ $message = 'you lose'; } // do any other stuff before redirecting header("Location: ".$_SERVER['PHP_SELF']."?msg=".$message); }elseif($_GET['msg']){ echo $_GET['msg']; }
havent tested but it should work and hopefully you get the idea!
Important: Using PHP_SELF can be dangerous when used as form action parameters *doesn't apply to your case specifically but always good to know anyway
Alex
-
please include the loop that currently fails, along with the query and the echo section, in the same way they appear!
a lot is left vague:
- do you execute the query every iteration?
- what condition do you iterate upon? (presumably ->rowcount() but its not clear)
here's a quote from the php manual on PDOStatement->rowCount (i assume you are using PDO by the sql-related syntax)
For most databases, PDOStatement::rowCount() does not return the number of rows affected by a SELECT statement. Instead, use PDO::query() to issue a SELECT COUNT(*) statement with the same predicates as your intended SELECT statement, then use PDOStatement::fetchColumn() to retrieve the number of rows that will be returned. Your application can then perform the correct action.maybe this could be your problem?
* also personally (depends on context and i don't know yours), but I would retrieve ALL values
// assume DB handler ($dbh) and formed query ($sql) $result = $dbh->query($sql); // check if query returned anything if($result->fetchColumn()){ $resultset = $result->fetchAll(PDO::FETCH_ASSOC); }else{ die('no results matched query'); } // echo table foreach($resultset as $colname => $colvalue){ ... }
i know it doesnt use prepared statements but you get the idea right?
Alex
-
just browsing through my profile and i saw the stats: "posts per time of day" thats kinda cool!
how about adding "posts in the last x days" (doesnt need to be variable but it'd be cool to see who's been more and less active recently (since posts/day takes registration date into account and a lot of users lurk for a while before posting, take vacations, etc)
Alex
-
Ops can reply to their own posts, but it has to be approved by a mod/admin.
thats cool!
can they mark topics as solved though? would make it a lot easier to find people that still need coders and also avoid having them receive offers after they no longer need help!
-
apologies in advance if this had been addressed already I took a quick look (more like a perusal) and couldn't find anything...
- would be VERY useful if the OPs could mark job requests as completed
- reply to their own posts or edit the original post to mention WHO did the job
- and maybe even briefly review the experience/coder
might be a but outside the scope of the forum but i think it would add a lot of quality.
again sorry if all of this exists and i just didn't pay enough attention!
Alex
-
448191,
If indeed the interface is consistent among the Factory's subjects, why create a dependency on an enum, when some simple class name mapping would be all a factory needs?Duly noted on your observation, but I might have a counter-argument depending on a clarification in your code:
public static function storageFactory($name) { $className = self::CLASS_PREFIX . $name; if(!class_exists(className) { throw new RuntimeException('Class ' . $className . ' not found'); } return new $className($whatever); }
The main point in my example of using enums for factories was not having to change the base class. (which your example addresses perfectly).
Now another issue is control over what classes are allowed to be constructed...
- In your example you allow any class with the prefix "storageFactory" to be instantiated.
- I won't insult your intelligence (after all you have 10 more stars over your username than I do
) so I'll assume that all classes with the prefix "storageFactory" would extend or implement a common class.
My argument for enums is that you could achieve control over class instantiation by for example commenting out constant declarations.
Also, due to its class structure, you could (although it wouldn't really make any tangible difference) specify the inheritance/implementation directly in the enum's declaration (since it appears to have standard class notation).... and having "const __default" is also nice
but either way, my "counter-arguments" have the intent of discussing possible alternatives to your solution, as opposed to of criticizing it or pointing out flaws/replacing it.
PS - at the risk of sounding dorky, I have to admit that I'm actually really enjoying reading and commenting this thread
-
How do you plan to use them?
I don't see them as useful at all.
frankly, I'm personally disappointed regarding the alleged implementation I posted earlier (that's pretty much exactly what I do without the extends part and without the __default value)
I guess it could be a little bit useful if they could just extend off of the is_object function to include a class name
for instance
Is Object of ClassName
is_object("ClassName", $var);
but really I'm sure there's already a work around for that anyway now, and the lack of type specifiers for functions hasn't really halted many projects as far as I can see.
If I understand what you mean... it would be so much simpler if PHP used the same concept as Java (all objects inherit from a base 'object class', in this case StdClass) but this is not the case (and if it happens all the anti-strongly-typed PHPers might collectively commit suicide)
But come to think of it why don't you just use instanceof ? (it allows both ClassNames, $variables and "strings") lol at the stupid decoration used for each word
if($var instanceof MyClass) { .. }
I don't see enums as all that useful either. Too often developers forget the enumeration itself needs to be constant, not just the enumerators. And how often does that really happen? Days in a week, months in a year, seasons in year, suits in a deck of cards? Not too much extra value IMO. SplEnum is even more useless, as it's not type safe.
ENUMS can be useful for a lot of things in my opinion. For example in implementing SimpleFactories (instead of hard-coding the constants in the factory class you could reference an external Enum Class, preserving the open-closed principle for that particular factory class)
In my personal experience I've emulated ENUM functionality for possible Storage Types (again a factory context, for example have the following constants "XML", "JSON", "YAML" and "MySQL")
Hmmmm what else... well I guess mainly for factories and there's workarounds such as strict Type-Hinting or verification (instanceof) that also work. I personally find ENUMs more elegant and the fact that you can have a separate Class with constants for factories is a plus because it reduces the probability of having to change the actual factory class every time you want to add a new 'product' (assuming the Enumerated types represent objects that implement a common interface or extend from the same base class - which is kind of implicit anyways in this context)
ok im rambling. time to stop!
Alex
-
guess I'm a bit late but better late than never right?
My name in case you haven't already figured it out is Alex;
[list]
[*]I'm 24 years old
[*]I live in São Paulo Brazil
[*]I'm single at the moment (was engaged to a great girl in the very recent past but we broke it off)
[*]I live alone, have no pets (wish i had a dalmatian!) and finishing Uni (comp-sci)
[*]I work at a web-based startup backed by a venture capital fund (which is an awesome and exciting experience)
[*]I still do freelance web-related development in my spare time (lately it's been mostly personal projects though)
[*]I've been programming since I was 13 (QBasic/VB 3.0) but was only introduced to OOP in 2003 (Java) and have been focused on web-based stuff (*AMP, JS, XHTML, CSS, etc) since 2006 but only really got serious about using the OO features early this year
[*]I can't design for the life of me... my photoshop skills limited to hiding layers and cropping
[/list]
and I think that's already borderline too much! :)
Anyway [b]HI![/b]
I hope I've been helpful to others and I'm very thankful to all who've helped me (sorry if i forget some of the more complicated screen-names (you know who you are!), but KeeB, DarkWater and corbin come to mind as some of the guys who've really helped me mature as a programmer!
See you around!
Alex -
its a shame SMF seems to have some kinda issue with long poll options which makes them that much harder to read but keep 'em coming i'm looking forwards to writing a wrap-up afterwards
-
Oh, really? Enums are quite handy. I bet it'll be a construct similar to arrays:
$enum = enum('red', 'blue', 'green');
After a bit of digging I found my source!
Translating from portuguese from the book "PHP Profissional", 1st published in 1997 and apparently revised or reprinted in 2008: (i apologize in advance for any poor english here I'm pretty much translating word-by-word with little regard to context):
A feature that's been promised for PHP6 (or even for a future 5.x build) is support for the concept of "Enumerations", which basically allow you to work with class constants in a more efficient way.
Another feature that's been promised for PHP6 are Object Wrappers for primitive types (similar to Java), making it easier to work with databases, since the best way to insert a value of NULL into a database is to insert an Object that represents NULL, as opposed to the primitive value itself.
This will all be made possible with the introduction of the class splType, which will be abstract and provide basic methods to achieve the aforementioned wrapper functionality.
He made an interesting point regarding storing NULL values in a database and this actually came up in a discussion today (on usenet: comp.lang.php):
Inserting the primitive type NULL into a mysql database can sometimes (maybe always im not sure) end up being stored as a blank string.
* The correct implementation would be to actually NOT pass the PHP variable and to instead just pass the MySQL datatype NULL instead (notice the second parameter passed):
$myvar1 = 'foo'; $myvar2 = null; $query = "INSERT INTO mytable (col1,col2) VALUES ('$myvar1', null)";
This actually works well, but in order to avoid depending on database-specific implementations I suppose passing a "NULL Object Wrapper" (SplNull?) would be a more versatile and future-proof alternative.
As far as ENUMs go, much to DarkWater's disappointment, the author gives the following code example of a PHP Enumeration (sic):
class WeekDays extends SplEnum{ const Sunday = 0, Monday = 1, Tuesday = 2; const Wednesday = 3, Thursday = 4, Friday = 5; const Saturday = 6; const __default = WeekDays::Monday; } $dayObject = new WeekDays(WeekDays::Sunday); // check if the created object corresponds to an existing constant foreach(WeekDays::getConstList() as $key => $val){ echo $key . ": " . ($dayObject == $val ? "yes" : "no"); }
Some more observations:
- notice the "const __default" feature
- notice the static method getConstList() which doesn't yet exist (according to the definition of get_defined_constants() it only works for constants defined by, you guessed it, define() but the top-most comment in the documentation suggests a workaround to get this working inside the Class Scope
and a BONUS for everyone: apparently if you do some scavenging you'll find that quite a few of the PHP6 or future PHP5 features are already listed in the API (albeit with scarce if any documentation): for example: SplTypes seems to have already been defined, along with SplFloat, SplBool, SplInt and, wait for it, SplEnum!
(no mention of SplNull though....)
*phew!* wow this post alone is worthy of its own thread lol, shame its hidden so deep in the middle of this thread
*DISCLAIMER*
I'm merely translating directly from a PHP Book mentioned above and guarantee absolutely none of the quoted material so don't go flaming me if they implement it differently!
-
Based on those meeting notes, they will only support return types for objects.
That's cool with me
I searched the meeting notes and couldn't find anything about it (except that classes won't be able to cast to primitive types) but I read elsewhere (and i'm not sure if this is contradictory or not but i dont think so) that they would also introduce Class Wrappers for primitive types (identical to what Java's been doing for years) so that's even cooler with me
And ENUMS too... boy PHP6 is sure become more java-like
as long as we dont have to compile anything and can still use all the loosely-typed goodness i'm happy with it (although sometimes its good to have features that other more strongly-typed lanaguages have)
-
SQLite
SQLite is a software library that implements a self-contained, serverless, zero-configuration, transactional SQL database engine.transactional SQL, no server, no config... can you really go wrong?
and firefox uses it which automatically makes it even cooler
-
What ticks me off is when devs mix camelHump() and under_score() naming
you mean mixing between the two for variables and/or functions? or in general?
as in would it tick you off if ALL variables used under_score() naming and ALL functions used camelCase() ?
just wondering
-
What are yall's opinions on the prefix-private/protected-attributes/methods-with-underscore convention?
private function _whatever()
private $_whatever;
etc.
for functions: practically never.
for variables: i don't like it (i even posted a thread about it a while ago) but its a good way to distinguish between private and public variables. but in reality, if you are coding something half-decent NONE of your variables will be public (except maybe static ones) so its kind of contradictory.
after reading this i decided to adopt the use underscores to prefix variables that are of strictly internal use. all variables are protected/private but the ones that i don't allow __get() to return i will try to prefix with an underscore.
how's that for a life-changing thread!
yeah, I always use "getters and setters" when it comes to class attributes, no matter the language.
despite reading about how you should NOT use __get() and __set() magic methods lately, I've resorted to using __get() for attribute retrieval (allows more natural syntax, simulates public visibility) and using multiple setVariable() for setters. Not sure why I diverged there but it just seems more natural to me:
$testclass = new Foo(); // set variable $testclass->setX('bar'); // get variable $testvar = $testclass->x;
-
(note to mods: despite mentioning sqlite as an alternative this doesn't quite qualify as a RDBMS thread so i posted here)
I'm looking for a file-based alternative to store small amounts of data (1 table, 2-10 columns, couple thousand entries MAX and most likely reside in the hundreds)
My options are:
- FlatFile
- XML
- JSON
Or maybe even:
- Sqlite
- Firebird
Basically I'm aiming for ease of distributions... I've read up a bit on the sqlite vs json vs xml debate and it only really added fuel to the fire....
Can anyone shed some light (from personal experience preferably) on the following aspects:
- Speed (and whether the difference between one or another is negligible)
- Ease of use (install, setup, GO!)
- Scalability (can it handle hundreds? what about thousands? or even more?)
- The only thing I'm pretty sure of is that JSON is not the best choice (more suited for small amounts of data; AJAX stuff)
- XML + XPath could be powerful but how does it rank against sqlite?
- I'd never heard of "FlatFile" before but it seems robust.
- Sqlite is intriguing heard a lot about but never used it.
- Firebird just occured to me as I was writing this post (afaik its a similar concept as sqlite)
thanks for any and all info!
i realize its hard to pick "THE BEST" but I'd like to hear some personal experiences if you have them and also recommendations and suggestions!
ps - i'm not ruling out MySQL at all - i just want an alternative for a "lite distribution"
-
thank you sir!
-
as long as someFunction(), somefunction() and SomeFunction() all work the same... is there really a need for convention?
without convention anarchy would ensure!
-
oh and this is a shocker to me:
Some_class;
??? i guess i'm just pedantic (except with capitalization when im posting this late at night) but my classes are 100% strict ProperCase
When I was first learning OOP, whatever I was reading (some book) mentioned that all classes should start with a capitol letter. That's how I learned it and it's just habit now, whether it's correct or not.
the weird thing about that is not the capital letter at the start... its the underscore!
Java has the cleanest conventions/syntax rules in my opinion
Java
ClassName
functionName()
varName
-
interesting reply KeeB I hadn't considered the compilation factor...
From what I saw I thought they were already including type-hinted return values in php6?
great news! any links?
I'm not entirely sure why you'd want to type hint function return values... It wouldn't do any more than a comment above the function noting the return type. If anything, I like that a function I design could return an array or string, depending on the parameters I give it.
regarding not wanting to specify a return value that's what i meant by making it optional!! if you dont want to use it then dont!
(also you could just specify "mixed" which if im not mistaken basically accepts any kind of type and pseudo-type (someone please correct me if im wrong)
as for why? basically: programming/design by contract
i usually comment expected return values in interface method signatures but then i'd have to go and enforce this in the method's return value.
similarly to type-hinting, this is a powerful yet optional feature that can help produce more robust code and eliminate the need for so much type checking...
-
Code as if the flag doesn't exist
Even with case-sensitivity, there's no way in hell you should have 2 constants with the same name in different cases.
define('FOOBAR', 'someval'); define('FooBar', 'anotherval');
could lead to some major confusion.
I like case insensitivity for function and class names. They're both designed as a modular way to share code.. whether it be with your own script or others. Some people prefer to code using camelHump(), or UppercaseFirst() or sometimeslower(). Having case-insensitive function/class names allows multiple developers to use the same code without having to worry about the other dev's case standards.
This also goes with my above comment that you should never have two functions or classes with the same name, regardless of case differences
good points, like i said "personally i think that all constants should be capitalized, end of story."
as far as function and variable names I think that there should be some kind of convention (made a poll here: http://www.phpfreaks.com/forums/index.php/topic,221997.0.html)
Alex
-
yet another rant about certain PHP inconsistencies that have been bugging me lately.
i realize that if i'm looking for a strongly-typed language (which im not) then PHP is not for me but consider this:
Type-Hinting was recently introduced right? (ok its limited to arrays and objects but still it exists)
and its optional, so if you dont know about it or dont use it thats fine.
1) So why not take the next step and introduce function return values? (after presumably implementing type-hinting at for all types, excluding maybe pseudo-types)
it would be optional so again, if you dont know about it or chose not to use it thats fine, it would be just like today.
but it sure would make writing interfaces and programming by contract so much easier in my opinion...
2) oh and for the record, if no return statement is specified or if its just "return;" then null is returned right?
*puts on flame suit*
-
1) Essentially, it's not a singleton. It produces new instances of the database object each time the static factory method is called. static =/= singleton
2) Already addressed, it's not a singleton. He could keep making new database connections.
3) Yes, a Config object is often good to keep settings in one place, and passing it in instead of global using it aids in decoupling the classes, because in reality, a database factory has nothing to do with a Config, it should just do what it's told, so the config data is passed in.
thanks for clearing that up and yeah duh, i missed the fact that the constructor wasnt private and because of the static way of getting instances i assumed singleton
im currently building a DAO class for an application im writing using PDO (which is kinda silly i guess since its creating a DB Layer class for another DB Layer Class in a way) but i just want to have more control over the PDO class.
This just occurred to me: keep writing the class or extend PDO and intercept and block functionality i don't want to be able to use? (im guessing the later but who knows)
Also, i now conclude that having a singleton DB Layer is a bad idea!
(time to change private to public on my constructor...
)
-
lol i could have sworn i already said this here but its 3am and ive posted about this on google groups too but i really dont care about case-sensitivity. i think PHP is fine as it is, in fact its GREAT!
i'm just a stickler for consistency. and having a case-sensitive flag (albeit optional) in "define()" and not having the same option in "const" is just wrong. constants are constants. so it makes no sense to me to allow case-insensitivity in one context and disallow it in another.
personally i think that all constants should be capitalized, end of story.
PHP session id to Javascript in Internet Explorer
in PHP Coding Help
Posted
ditto. (i know i already said it). but still... wow! (no offence to OP) but i just didn't realize this was even possible!!!
:o 
