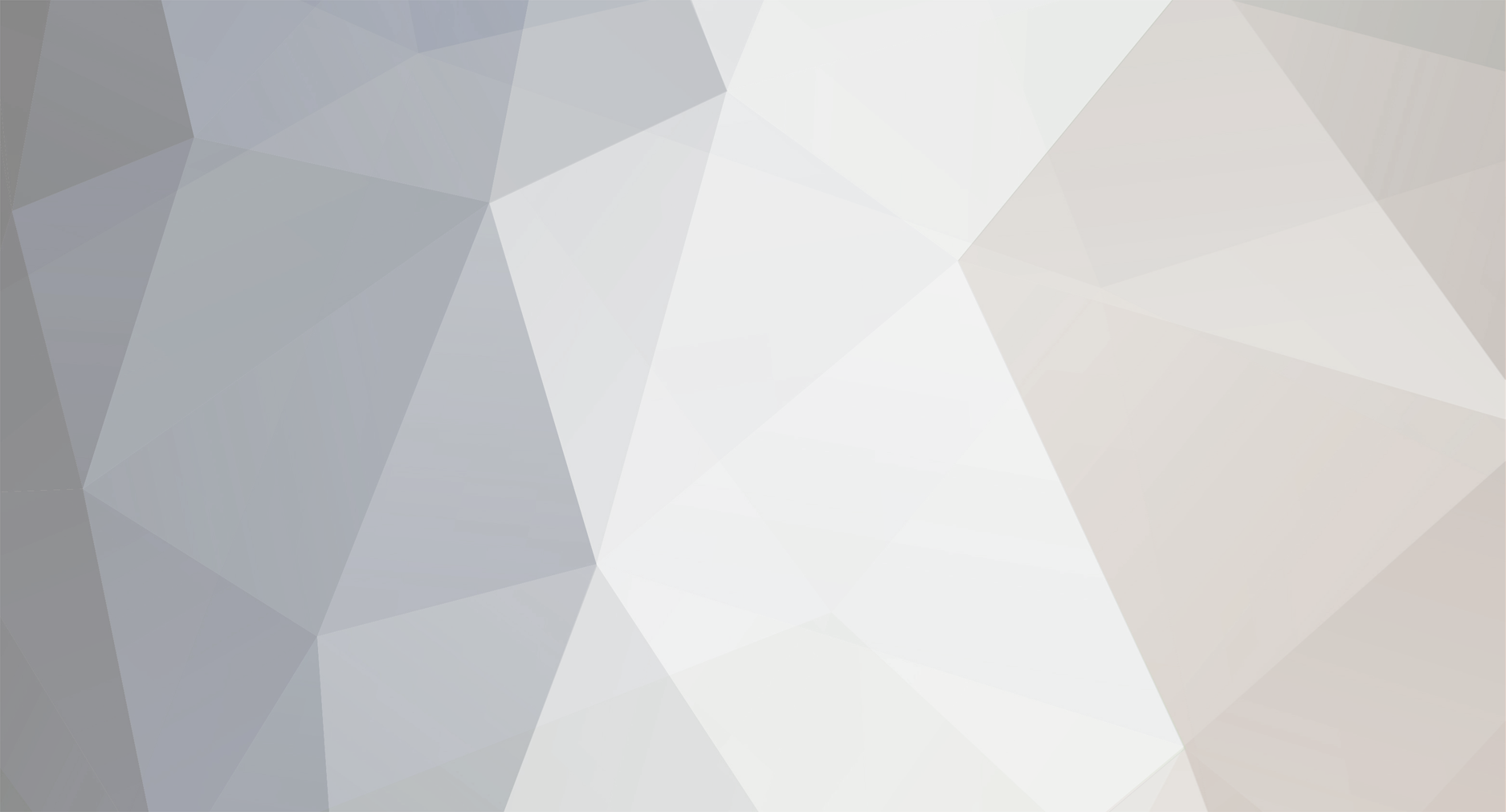
xtopolis
Members-
Posts
1,422 -
Joined
Everything posted by xtopolis
-
FYI, you should never store the true password, store the hashed version instead. But whatever works for you. Mark this solved if you're satisfied.
-
I don't know about using GoogleMaps for IPs, but you could use a GeoLocation database to determine the area yourself, and then supply the location name to google. Some info http://us.php.net/manual/en/book.geoip.php I don't have much experience with this specifically, but I think I saw another thread on this on this forum. Try search this forum for more info.
-
PHP Date-Time to SQL Date-Time and vice versa?
xtopolis replied to scott.stephan's topic in PHP Coding Help
Are you saying you manage PHP dates in a format different than: YYYY-MM-DD HH:MM:SS ? What are you trying to do? Afaik, sticking with the DATETIME format of MySQL is simple for PHP as well. -
delete.php would primitively be something like this: //database connection stuff //grab postid from the url $id = intval($_GET['id']); //check if it exists $sql = "SELECT PostID FROM posts WHERE id='$id'"; $result = $db->query($sql); if(mysql_num_rows($result) == 1){ $delete = "UPDATE posts SET deleted=1 WHERE PostID='$id'"; //exists, "delete" it if(!$db->query($delete)) { echo mysql_error(); }else{ echo 'Post has been deleted.'; } }else{ //post doesn't exist, or multiple matches (shouldn't have multiple on auto inc ..) echo 'No post exists with that post id.'; }
-
md5 returns an alpha numeric string. Only letters and numbers. stripslashes is unnecessary here. Your other inputs, however, could use some sanitizing. $_POST['username'] should later be: mysql_escape_real_string($_POST['username']); First, try outputting the results of the form to your screen. (print out the username and the md5 version of the password as it is when you submit the form). Then copy that directly into mysql / phpmyadmin and test the query. This way you can help determine where your problem is. Secondly, you may want to restructure your code. You have several points where it continues processing even though the result will be a failed login. The first occurrence is when you check if the fields have been entered (!$_POST['username'] | !$_POST['password']). I reccommend changing ! => empty to read as: if(empty($_POST['username']) | empty($_POST['password'])) ... and if they are, don't even both doing anything else because it's a waste of processing. This is seen again when you check how many rows matched ($check2). Obviously if the username doesn't exist, a password won't match... so don't even bother processing further. Lastly, you may want to keep the error message ($message) separate from "is allowed" (you check if empty($message) to determine if the login is successful) Perhaps have a separate boolean to check if login was successful, set after the database result. Overall a good start, but I think you're limiting yourself by sending HTML output and then doing processing.
-
You would probably use a $_GET argument at the end of the url. When you loop through the stories, select the auto increment column as well and have it print something like this: //... while (/* database select stuff */) { echo $row['PostTitle'] . ' <a href="delete.php?id=' . $row['PostID'] . '">[Delete]</a>'; echo '<br>'; } And have delete.php "Delete" the post with that PostID (assuming PostID is the name of the auto increment column). Btw, don't delete things, rather set a flag on or off showing it as "delete", but keep it in the system. When you select, just add a condition "WHERE deleted = 0" or something.
-
I doubt this is part of the problem, but why are you using stripslashes on a password when you're just going to md5 it?
-
This is why you don't mess with programmers. We will find you.
-
I use the free Notepad2 Syntax Highlighting, better undo, tabbing, etc is good enough for me. It's lightweight and free.
-
Hah... I went to post this and got caught up reading about MySQL optimization.. Possible Method One [using 2 tables, 1 category per product]: SELECT c.catName, COUNT(p.pid) FROM categories c JOIN products p USING(cid) /*WHERE c.cid = 2*/ GROUP BY c.cid I included a commented line for specifying just one category. Table 1 - categories -- Table structure for table `categories` -- CREATE TABLE `categories` ( `cid` int(2) NOT NULL auto_increment, `catName` varchar(255) collate latin1_german2_ci NOT NULL, PRIMARY KEY (`cid`) ) ENGINE=MyISAM AUTO_INCREMENT=4 DEFAULT CHARSET=latin1 COLLATE=latin1_german2_ci AUTO_INCREMENT=4 ; -- -- Dumping data for table `categories` -- INSERT INTO `categories` VALUES (1, 'Toyota'); INSERT INTO `categories` VALUES (2, 'Ford'); INSERT INTO `categories` VALUES (3, 'Mercedes'); Table 2 - products -- Table structure for table `products` -- CREATE TABLE `products` ( `pid` int(5) NOT NULL auto_increment, `prodName` varchar(255) collate latin1_german2_ci NOT NULL, `cid` int(11) NOT NULL, PRIMARY KEY (`pid`) ) ENGINE=MyISAM AUTO_INCREMENT=7 DEFAULT CHARSET=latin1 COLLATE=latin1_german2_ci AUTO_INCREMENT=7 ; -- -- Dumping data for table `products` -- INSERT INTO `products` VALUES (1, 'Focus', 1); INSERT INTO `products` VALUES (2, 'Scion', 2); INSERT INTO `products` VALUES (3, 'M-Class', 3); INSERT INTO `products` VALUES (4, 'CoolCar', 1); INSERT INTO `products` VALUES (5, 'RedCar', 2); INSERT INTO `products` VALUES (6, 'Blue Car', 1); ####################################################### Possible Method Two [using 3 tables, multiple categories per product]: SELECT c.catName,COUNT(p.prodName) FROM categories c JOIN cat_prod cp USING(cid) JOIN products p USING(pid) /* WHERE c.cid = 2 */ GROUP BY c.cid I included the commented WHERE line so that you could specify one type if you wanted. Table 1 - categories -- Table structure for table `categories` -- CREATE TABLE `categories` ( `cid` int(2) NOT NULL auto_increment, `catName` varchar(255) collate latin1_german2_ci NOT NULL, PRIMARY KEY (`cid`) ) ENGINE=MyISAM AUTO_INCREMENT=4 DEFAULT CHARSET=latin1 COLLATE=latin1_german2_ci AUTO_INCREMENT=4 ; -- -- Dumping data for table `categories` -- INSERT INTO `categories` VALUES (1, 'Toyota'); INSERT INTO `categories` VALUES (2, 'Ford'); INSERT INTO `categories` VALUES (3, 'Mercedes'); Table 2 - cat_prod -- Table structure for table `cat_prod` -- CREATE TABLE `cat_prod` ( `cid` int(11) NOT NULL, `pid` int(11) NOT NULL ) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_german2_ci; -- -- Dumping data for table `cat_prod` -- INSERT INTO `cat_prod` VALUES (1, 2); INSERT INTO `cat_prod` VALUES (2, 1); INSERT INTO `cat_prod` VALUES (3, 3); INSERT INTO `cat_prod` VALUES (1, 4); INSERT INTO `cat_prod` VALUES (1, 5); INSERT INTO `cat_prod` VALUES (2, 6); Table 3 - products -- Table structure for table `products` -- CREATE TABLE `products` ( `pid` int(5) NOT NULL auto_increment, `prodName` varchar(255) collate latin1_german2_ci NOT NULL, PRIMARY KEY (`pid`) ) ENGINE=MyISAM AUTO_INCREMENT=7 DEFAULT CHARSET=latin1 COLLATE=latin1_german2_ci AUTO_INCREMENT=7 ; -- -- Dumping data for table `products` -- INSERT INTO `products` VALUES (1, 'Focus'); INSERT INTO `products` VALUES (2, 'Scion'); INSERT INTO `products` VALUES (3, 'M-Class'); INSERT INTO `products` VALUES (4, 'CoolCar'); INSERT INTO `products` VALUES (5, 'RedCar'); INSERT INTO `products` VALUES (6, 'Blue Car');
-
date and time that are auto inserted into my database are wrong
xtopolis replied to sandrine's topic in PHP Coding Help
You can't create your own php.ini on a per folder basis even? Perhaps this might work: http://us.php.net/manual/en/function.date-default-timezone-set.php -
I prefer to have a big arrow pointing down to the taskbar that says "Look here in case you don't know what time it is!" Other people just use: <script type="text/javascript"> document.write(Date()); </script> My way is much cooler.
-
How can I add a page that pops up when link is clicked?
xtopolis replied to iconicCreator's topic in Javascript Help
However, those are the exact same concepts applied in a similar manner. An event is trigger (click the picture). A div(usually) is displayed, content is loaded(the larger image), and displayed in a pleasant manner (the growing animation). When the user is done, they click the "close" link which (possibly removes content) hides the div again. The only difference is that instead of following the mouse while it is over the element, they chose to make something happen when a link is clicked. -
It is better to create "groups" with said permissions and then assign users to groups rather than uniquely assign permissions in most cases. I am not an expert on this my self, however you may want to look into the bitwise permissions threads on these boards as well as elsewhere. http://www.phpfreaks.com/forums/index.php/topic,113143.0.html http://www.phpfreaks.com/forums/index.php/topic,110890.0.html
-
This is fairly inconvenient to guess at without table structures.
-
First, thanks for providing your code in a convenient manner. (Copy-pasteable for the most part). That really helped. I only get the issue you describe when accessing the page without supplying a cat_id via GET superglobal. Line12 $cat_id = $_GET['cat_id']; could be changed to $cat_id = (isset($_GET['cat_id'])) ? intval($_GET['cat_id']) : 1; which would default the category to 1 if the page is accessed without selecting a category. I also had an error around line 117 with your mysql_result function. It requires another argument. Let us know if that doesn't fix what you want to happen.
-
How can I add a page that pops up when link is clicked?
xtopolis replied to iconicCreator's topic in Javascript Help
Your link doesn't work for me, but I think I know what you're talking about. I guess the best name for it is a "tooltip", but the idea behind it is a hidden page element that gets shown/populated on some window event. Scroll down to the demo here: http://boxover.swazz.org/ See if that's what you're talking about. As for turning off Javascript and it still working... it's probably just appropriate use of <noscript> tags -
div's don't use value, they use innerHTML function clear() { document.getElementById("txtHint").innerHTML = ''; } Would probably work for your example.
-
Performance wise, it sucks. Having to read through each CSV entry, parsing the string and determining if it is or isn't in a category... Changing/updating/deleting the categories in a csv method is semi unreliable. Why risk changing references to similar things such as "hat" and "chat", when you can edit them individually in one place? If you had a few rows that had csv categories like "house, hat, baseball" and another "school, chat, fire"... I would be skeptical that EVERY time would would perfectly type a mysql query to update only things that are == hat, rather than %hat or something similar. Take fenway's advice, it's good advice.
-
I wish there were some examples, easily seen, about what I might be able to do referenced at the beginning.
-
What about parsing it as an int? var reply = parseInt(xhro.repsonseText);
-
Read example #4, the top lines of code. http://us3.php.net/manual/en/function.mail.php
-
Data submitted by a form can be accessed via the superglobals. $_POST and $_GET You access the data via the name attribute of the field being sent, in your case it would either be: $_POST['firstName'] or $_GET['firstName'] (since you didn't specify your method). This is a small example tutorial. To check the length of a string, use the strlen function. In PHP, you cannot "do nothing" when a form is submitted, and I assume likewise in .NET. The "do nothing" event would be handled by javascript intercepting the submit and acting accordingly. You can however direct the application flow after submission based on whether it meets your requirements (ie strlen > 1). That part is just common logic statements: if(strlen($_POST['firstName']) > 1) { //do something }else{ //could show the form again, with fields filled in, or an error } As for redirecting, you can use header to redirect as long as nothing (NOTHING) has been output to the page... you can also append your firstName data perhaps more confidently when cleaning it with urlencode and maybe htmlentities. $fn = htmlentities(urlencode($_POST['firstName'])); header('Location: http://www.examplesite.com/query=' . $fn); Please look into the functions in the manual as they will help out a lot.