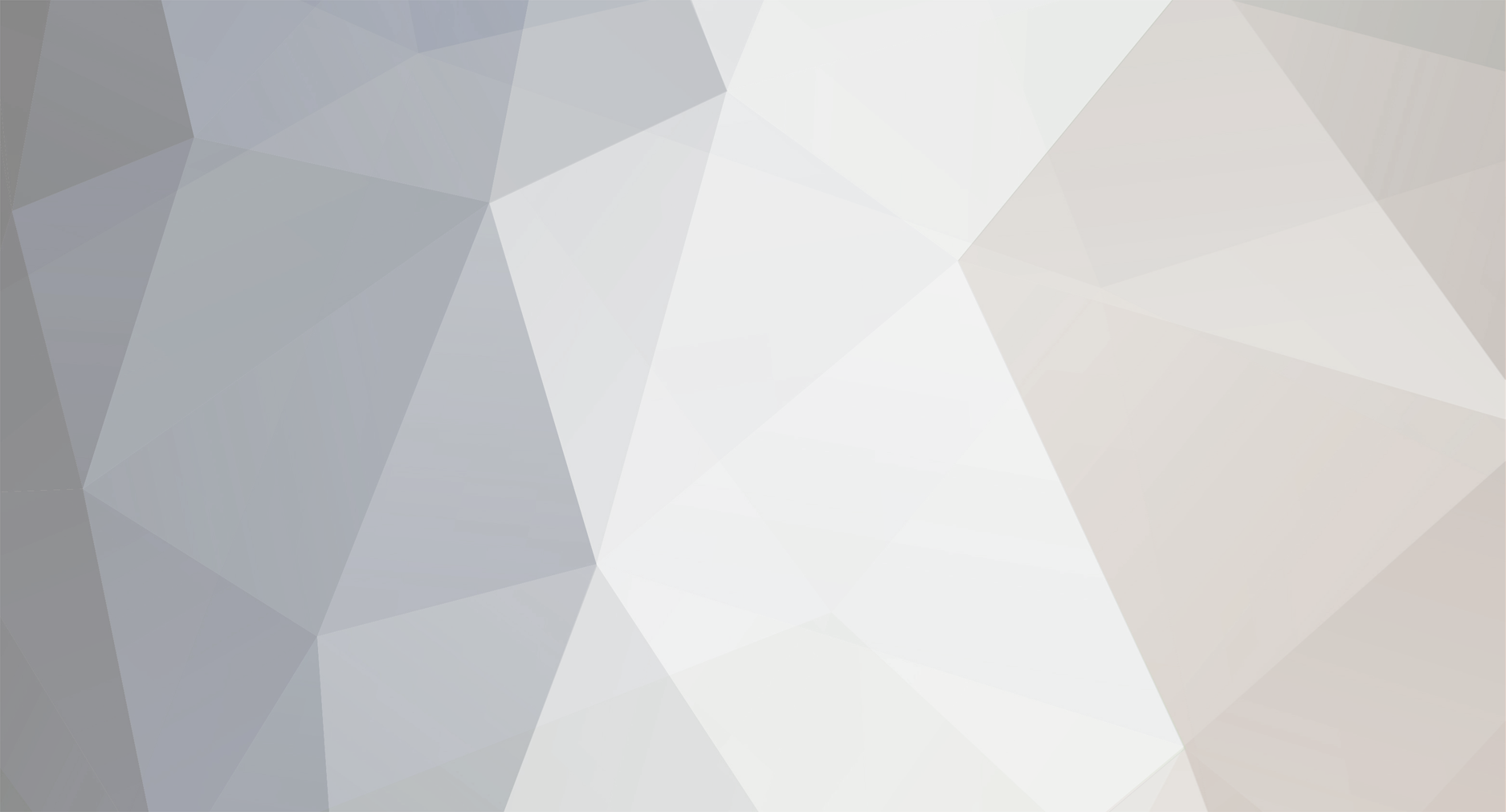
xtopolis
Members-
Posts
1,422 -
Joined
Everything posted by xtopolis
-
Basically, you go through line by line checking to make sure it behaves as expected. Your next step might be: <?php if (($_POST['op'] != "add") || ($_GET['master_id'] != "")) { var_dump($_POST['op']); var_dump($_GET['master_id']); die(); And check to see what those variables contain, etc.. keep going line by line or section.. and see that it works as intended.
-
At this point the best I can say is walk through it step by step. Start breaking the code down from the top to make sure everything is getting to it. So it says master_id is not defined, so at the very top debug like this: <?php echo $_GET['master_id']; die(); //... (rest of code) then access the page as: http://yourhost.com/addentry.php?master_id=24 and make sure it is printing 24 Go through it in small sections like that making sure the variables do indeed contain what you expect. I'd rather suggest redoing the form as it seems rather convoluted, but it seems like it's nearly there, so it's up to you how much you want to mess with it.
-
It means that nothing exists at $_POST['add'] because $_POST['add'] was never created. It's likely due to how you're making the form, and testing it. It's very hard for me to read what you're doing, but it seems like an "if they haven't submitted the form, show this, otherwise show that" or something.. I think the issue is that you're looking for variable that don't exist yet. Most of those errors will go away when you fix the root error it seems. The root error being the condition that look for if the form has been submitted. As far as I can see you look in all cases to see if $_POST['op'] == 'add' as your driving condition.. maybe the top one should be <?php if (!isset($_POST['op']) || ($_GET['master_id'] != "")) { meaning that the form has not been submitted (and therefor doesn't exist).(Line 2 error, probably) The line 8 error is because you are not accessing the page correctly as : yourpage.php?master_id=2, instead probably just : yourpage.php Line 24 and 67 should fix if you fix the line 2 and 8 errors.
-
No, the basic syntax is similar to: SELECT (column) FROM (table) WHERE (condition) Your query should (probably) be what I posted: $get_names = "SELECT CONCAT_WS(' ', f_name, l_name) as display_name FROM master_name WHERE id = $_GET[master_id]"; CONCAT_WS(' ', f_name, l_name) AS display_name == the column (combing two as one with the CONCAT_WS function), then naming it to "display_name" for easy reference.
-
I believe you would just add to the AND conditions ... ON (bad_rows.username = good_rows.username AND bad_rows.id > good_rows.id AND bad_rows.something = good_rows.something) Is that what you mean, or am I misunderstanding?
-
$get_names = "SELECT CONCAT_WS(' ', f_name, l_name) as display_name FROM master_name WHERE id = $_GET[master_id]"; SELECT (column) FROM (table) WHERE (condition) As for the rest.. :S , lets go a step at a time..
-
[SOLVED] Undefined Variable/Supplied Argument Is Not Valid
xtopolis replied to twilitegxa's topic in PHP Coding Help
Yes, as pointed out by eagle, you reference that id in $_GET[item_id], but never assign it as: $item_id = $_GET[item_id]; in the page in question. -
That would leave him with an extra ) inside the query, at the very end. Why can't you just make it a bit less confusing? $connect = mysql_connect("127.0.0.1", "root", "") or die('Could not connect'); mysql_select_db("MainDB", $connect) or die('Could not select database'); $sql = "DELETE bad_rows.* FROM account AS good_rows INNER JOIN account AS bad_rows ON (bad_rows.username = good_rows.username AND bad_rows.id > good_rows.id)"; $query = mysql_query($sql, $connect) or die(mysql_error()); mysql_close($connect) or die(mysql_error());
-
[SOLVED] Undefined Variable/Supplied Argument Is Not Valid
xtopolis replied to twilitegxa's topic in PHP Coding Help
$item_id is ONLY defined in the mysql statements. It doesn't have any value, you don't assign it any value. Where are you supposed to get $item_id from? -
I think this is the default behavior of JOIN. Without your query/database structure, it's hard to speculate.
-
edit - im confusing myself, post current code.
-
I suggest you show us your relevant code and describe specifically which part doesn't work.
-
Did you modify it's locations for dependent scripts accordingly after you moved it? require('config.php'); require('admin/global.php'); Do those paths still point to the right place now relative to it's new location? @The Eagle, nothing will change with your modification.
-
I think you're missing a ( to surround the ON condition. $query = mysql_query("DELETE bad_rows.* FROM account AS 'good_rows' INNER JOIN account AS 'bad_rows' ON (bad_rows.username = good_rows.username AND bad_rows.id > good_rows.id)", $connect) or die(mysql_error()); (Line4) , I modified your statement so it could be more easily read.
-
I'm not downloading it, but are you sure the javascript src files point to the right spot? Otherwise, narrow it down, and then debug it with alert() statements until you find the problem area.
-
Your includes are pointing to the wrong spot it seems. You probably just need to make it: include '../classes/config.php'; include '../classes/sessions.php'; (just a guess from the other errors) Find out where those scripts lay relative to your current page.
-
MySQL laughs in the face of your date calculations. I would say keep all the data gathering/sorting/crunching with MySQL and leave the displaying to PHP. IMO.
-
Show some relevant code please.
-
I would suggest reposting the query in the MySQL section to try to get what you want. I'm fairly certain it's entirely plausible. Glad it helped.
-
[SOLVED] nl2br or str_replace cannot replace new lines with <br />
xtopolis replied to obay's topic in PHP Coding Help
Try str_replace("\\n",'<br />',$text); -
CREATE TABLE ‘categories’ ( ‘id’ INT NOT NULL AUTO_INCREMENT , ‘name’ VARCHAR( 255 ) NOT NULL , ‘shortdesc’ VARCHAR( 255 ) NOT NULL , ‘longdesc’ TEXT NOT NULL , ‘status’ ENUM( ‘active’, ‘inactive’ ) NOT NULL , #this line has incorrect quotes ‘parentid’ INT NOT NULL , PRIMARY KEY ( `id` ) ) TYPE = MYISAM ; That line is using your version of single quotes which I'm sure it doesn't like. Use this version of single quote: ' So that line will now look like this: ‘status’ ENUM( 'active', 'inactive' ) NOT NULL , # mine instead of this ‘status’ ENUM( ‘active’, ‘inactive’ ) NOT NULL , # yours I changed the ‘active’, ‘inactive’ --to--> 'active', 'inactive' - I am using normal single quotes for those string values, you are not.
-
What happens when change JOIN to LEFT JOIN or RIGHT JOIN in the query? This is not my strong point, but if I remember correctly, one of those will retrieve null values as well. (only modified the last part for BETWEEN SELECT el.`loc`,dee.`pid`,dee.`rating`,dee.`thedate` FROM `lunch_locations` el JOIN `lunch_data` dee ON el.`id`=dee.`pid` WHERE MONTH(`thedate`)=MONTH(CURDATE()) AND DAY(`thedate`)=DAY(CURDATE()) AND ( YEAR(`thedate`) BETWEEN 2005 AND YEAR(CURDATE()) ) (edit: also I'm not sure which ordering of the AND statements would be more efficient)
-
The back tick ` is used to escape column/table names that use reserved words. It is the special identifier stating that `thetexthere` is the name of a table or column. Single quotes ' are used to denote strings , in this example for a "yet to be" column name. CREATE TABLE `categories` ( # `categories` refers to a table name `id` INT NOT NULL AUTO_INCREMENT , # `id` refers to a column name `name` VARCHAR( 255 ) NOT NULL , #etc `shortdesc` VARCHAR( 255 ) NOT NULL , #etc `longdesc` TEXT NOT NULL , #etc `status` ENUM( 'active', 'inactive' ) NOT NULL , # -- 'active', 'inactive' are strings for yet to be columns `parentid` INT NOT NULL , #`parentid` is a column name PRIMARY KEY ( `id` ) #refering to the column `id` ) TYPE = MYISAM ; The last one for PRIMARY KEY( `id` ) may be a little confusing, but that's just how it is. (Assuming it worked)
-
Try changing this line PRIMARY KEY ( 'id' ) to PRIMARY KEY ( `id` ) I did a global replace on your quotes, so I forgot to correct it. That should fix it I think.
-
Could it be the weird quote marks you're using? Try copy pasting this: CREATE TABLE `categories` ( `id` INT NOT NULL AUTO_INCREMENT , `name` VARCHAR( 255 ) NOT NULL , `shortdesc` VARCHAR( 255 ) NOT NULL , `longdesc` TEXT NOT NULL , `status` ENUM( 'active', 'inactive' ) NOT NULL , `parentid` INT NOT NULL , PRIMARY KEY ( 'id' ) ) TYPE = MYISAM ; and see if you get the same error?