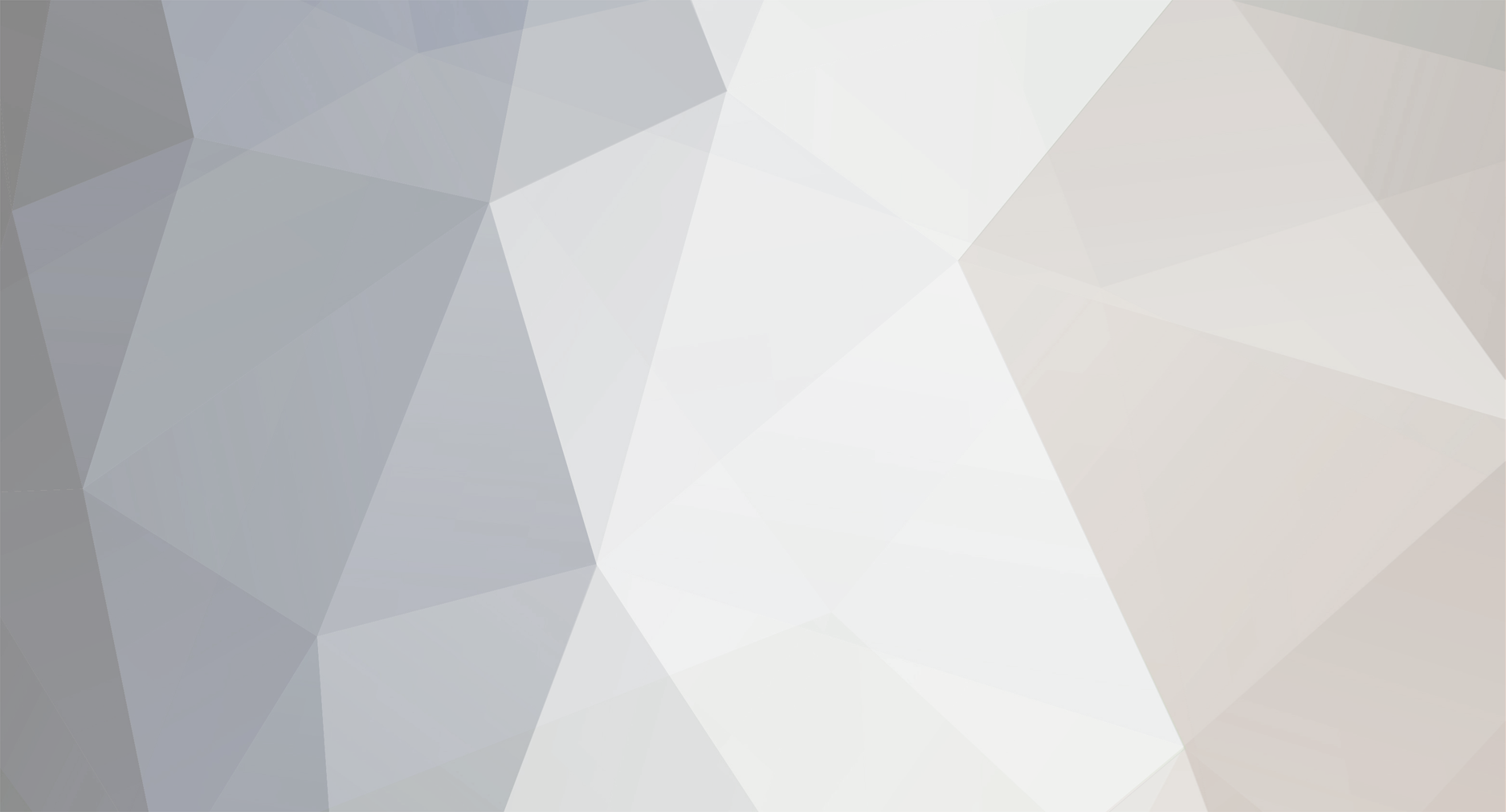
twilitegxa
-
Posts
1,020 -
Joined
-
Last visited
Posts posted by twilitegxa
-
-
I have this page:
<?php session_start(); //connect to server and select database $conn = mysql_connect("localhost", "root", "") or die(mysql_error()); $db = mysql_select_db("smrpg", $conn) or die(mysql_error()); //show scouts characters $get_scouts = "select * from scouts where username = '".$_SESSION['userName']."'"; $get_scouts_res = mysql_query($get_scouts, $conn) or die(mysql_error()); while ($list_scouts = mysql_fetch_array($get_scouts_res)) { $identity = ucwords($list_scouts['identity']); $topic_id = $list_scouts['id']; echo "<ul class=\"character_list\"><li><a href=\"fight.php?identity=$identity\">$identity</li></ul> "; } ?>
And it goes to this page:
<?php session_start(); //connect to server and select database $conn = mysql_connect("localhost", "root", "") or die(mysql_error()); $db = mysql_select_db("smrpg", $conn) or die(mysql_error()); //check for required info from the query string if (!$_GET['identity']) { header("Location: train_fight.php"); exit; } //get derived values $derived = "select * from derived_values where identity = $_GET[identity]"; $derived_res = mysql_query($derived, $conn) or die(mysql_error()); $display_block = "<ul>"; while ($derived_info = mysql_fetch_array($derived_res)) { $derived_id = $derived_info['id']; $derived_identity = $derived_info['identity']; $derived_health = $derived_info['health']; $derived_energy = $derived_info['energy']; $derived_acv1 = $derived_info['acv1']; $derived_acv2 = $derived_info['acv2']; $derived_dcv1 = $derived_info['dcv1']; $derived_dcv2 = $derived_info['dcv2']; $derived_total_cp = $derived_info['total_cp']; $display_block .= "<li>$derived_identity</li>"; } $display_block .= "</ul>"; ?>
But I am getting this error:
You have an error in your SQL syntax; check the manual that correspondsto your MySQL server version for the right syntax to use atline 1
What am I doing wrong here? If I change my where statement or take it out, it is displaying the information, but I can't figure out what's wrong with my where statement or where I'm getting my "identity" from. Something's wrong but I can't find it. Can anyone help?
-
I am looking for any information on how I can go about creating a PHP/JavaScript jigsaw puzzle program that automatically creates jigsaw puzzles from a folder of images. Does anyone know what I can look up to get started on something like this?
-
Sorry. :-) I want to make a puzzle that people can put together and that would have a timer. Then when the puzzle is complete, stop the timer, and then give them a score based on their time. Does that help?
-
Can anyone help point me in the right direction for help in creating a puzzle program in PHP? Or if anyone knows any other better way to create one, please, let me know. Thanks!
-
Thank you so much! That works perfectly!
-
As you can see, I've tried using
$reply_comment_id = $_GET['comment_id'];
But it's not getting the comment_id from the previous page. When I send the user from the main page to the reply form, I send the comment id with the link, so it should be able to be picked up on the reply form page, but I can't remember how to do this. Can anyone help?
-
Okay, my insert statement is working except for my $comment_reply_id, which I send from the main page to the second page, but I need to know how I can send it to the third page from the second page. I send it to the second page from the first page like this:
<a href=\"reply.php?comment_id=$comment_id\">Reply</a>
How do I send it from the second page to the third? The second page is the form:
<form name="comment" id="comment" action="reply_form.php" method="post"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="name"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" /></div> </td> </tr> <tr> <td align="left"><label for="email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" /></div> </td> </tr> <tr> <td align="left"> <label for="reply">Reply:</label> </td> <td> <textarea name="reply" id="reply" rows="5" cols="30"> </textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div> </td> <td width="2"></td> </tr> </table> </form>
And the third page is the insert page:
<?php //connect to server and select database $conn = mysql_connect("localhost", "username", "password") or die(mysql_error()); $db = mysql_select_db("webdes17_testimonials", $conn) or die(mysql_error()); //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $reply=mysql_real_escape_string($_POST['reply']); $reply_comment_id = $_GET['comment_id']; $sql="INSERT INTO replies (reply_id, comment_id, reply, reply_create_time, reply_owner, reply_owner_email) VALUES ('', '$reply_comment_id', '$reply', now(), '$name','$email')"; if (mysql_query($sql,$conn)) { // code to do if insert succeeds } else { print('Could not INSERT comment to database. Error given: '.mysql_error().'<br/>'.'Query sent: '.$sql.'<br/>'."\n"); // other code to do if insert fails (if any) } //create nice message for user $msg = "<p>Your comment has been added. Thank you for your feedback! You will be redirected to the Testimonials page in a moment. If you aren't forwarded to the new page, please click <a href=\"http://www.webdesignsbyliz.com/testimonials.php\"> here</a>. </p>"; ?>
-
I am trying to make a reply type of form, and I want the reply form to insert the form data to the table and detect what post it is replying to. So far I have this form:
<td> <form name="comment" id="comment" action="reply_form.php" method="post"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="name"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" /></div> </td> </tr> <tr> <td align="left"><label for="email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" /></div> </td> </tr> <tr> <td align="left"> <label for="reply">Reply:</label> </td> <td> <textarea name="reply" id="reply" rows="5" cols="30"> </textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div> </td> <td width="2"></td> </tr> </table> </form> </td>
And I have this insert statement on my page:
<?php //connect to server and select database $conn = mysql_connect("localhost", "username", "password") or die(mysql_error()); $db = mysql_select_db("webdes17_testimonials", $conn) or die(mysql_error()); //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $reply=mysql_real_escape_string($_POST['reply']); $reply_comment_id = $_GET['comment_id']; $sql="INSERT INTO replies (reply_id, comment_id, reply, reply_create_time, reply_owner, reply_owner_email) VALUES ('', '$reply_comment_id', '$reply', now(), '$name','$email')"; mysql_query($sql,$conn) or die(mysql_error()); //create nice message for user $msg = "<p>Your comment has been added. Thank you for your feedback! You will be redirected to the Testimonials page in a moment. If you aren't forwarded to the new page, please click <a href=\"http://www.webdesignsbyliz.com/testimonials.php\"> here</a>. </p>"; ?>
And I have a link on the main page that takes the user to the reply page that sends the comment_id like this:
<a href=\"reply.php?comment_id=$comment_id\">Reply</a>
But my insert statement is not inserting anything. What am I doing wrong? It's not giving the error; It's outputting my message saying that the reply has been added. Here is my table sql statement, just in case:
CREATE TABLE IF NOT EXISTS `replies` ( `reply_id` int(11) NOT NULL auto_increment, `comment_id` int(11) NOT NULL, `reply` longtext, `reply_create_time` datetime default NULL, `reply_owner` varchar(150) NOT NULL, `reply_owner_email` varchar(255) NOT NULL, PRIMARY KEY (`reply_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=4 ;
Can anyon help me with what I am doing wrong this time? I know I must be missing something simple, but I can figure it out! Please help!
-
Thank you! Removing the enctype from the form worked perfectly! Thank you so much!
-
I tried changing the insert statement to this:
<?php //connect to server and select database $conn = mysql_connect("localhost", "username", "password") or die(mysql_error()); $db = mysql_select_db("database_name", $conn) or die(mysql_error()); //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $url=mysql_real_escape_string($_POST['url']); $comment=mysql_real_escape_string($_POST['comment']); $sql="INSERT INTO user_comments (comment_id, comment, comment_create_time, comment_owner, comment_owner_email, url) VALUES ('', '$comment', now(), '$name','$email', '$url')"; mysql_query($sql,$conn) or die(mysql_error()); //create nice message for user $msg = "<p>Your comment has been added. Thank you for your testimonial!</p>"; ?>
But it's still generating blank fields when I submit the form. Any ideas?
-
Well, it's been a while since I have worked with PHP and MySQL, so maybe I don't need to get the field values from the session. LOL I was just pulling the same basic principle from another "working" message board I have created in the past, but I just couldn't get this one to work for some reason. How should I change it? I will first try changing to put the quotes around the ones you suggested, but should I be changing them from being session values?
-
I have the following form:
<table class="style2" align="center"> <tr> <td> <form name="comment" id="comment" action="comment_form.php" method="post" enctype="text/plain"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="name"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" /></div> </td> </tr> <tr> <td align="left"><label for="email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" /></div> </td> </tr> <tr> <td align="left"><label for="url">URL:</label></td> <td> <div class="c2"><input type="text" name="url" id="url" size="30" /></div> </td> </tr> <tr> <td align="left"> <label for="comment">Comments:</label> </td> <td> <textarea name="comment" id="comment" rows="5" cols="30"> </textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div> </td> <td width="2"></td> </tr> </table> </form> </td> </tr> </table>
And for some reason, my php insert statement is inserting blank data from the form into the table in my database. All it's inserting is the date correctly. Here is my sql statement:
<?php session_start(); //connect to server and select database $conn = mysql_connect("localhost", "webdes17_twilite", "minimoon") or die(mysql_error()); mysql_select_db("webdes17_testimonials",$conn) or die(mysql_error()); //create and issue the first query $add_comment = "insert into testimonials values ('', '".mysql_real_escape_string($_POST['comment'])."', now(), '$_SESSION[comment_owner]', '$_SESSION[comment_owner_email]', '$_SESSION[url]')"; mysql_query($add_comment,$conn) or die(mysql_error()); //create nice message for user $msg = "<p>Your comment has been added. Thank you for your testimonial!</p>"; ?>
Can anyone help me with what I'm doing wrong? Here is my table statement as well, in case I have something wrong:
CREATE TABLE IF NOT EXISTS `user_comments` ( `comment_id` int(11) NOT NULL auto_increment, `comment` longtext, `comment_create_time` datetime default NULL, `comment_owner` varchar(150) default NULL, `comment_owner_email` varchar(150) default NULL, `url` longtext NOT NULL, PRIMARY KEY (`comment_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=25 ;
I can't figure out what I'm doing wrong? :-( The page directs to the php page properly and displays the message for the user stating that the comment was successfully added, so it THINKS it's being added, but the fields are coming in blank. Can anyone help me with why?
-
Can anyone help me figure out why my data is not inserting from my form?
<%@ Page Title="Dorknozzle Help Desk" Language="C#" MasterPageFile="~/Dorknozzle.master" AutoEventWireup="true" CodeFile="HelpDesk.aspx.cs" Inherits="HelpDesk" %> <asp:Content ID="Content1" ContentPlaceHolderID="head" Runat="Server"> </asp:Content> <asp:Content ID="Content2" ContentPlaceHolderID="ContentPlaceHolder1" Runat="Server"> <h1>Employee Help Desk Request</h1> <asp:Label ID="dbErrorMessage" ForeColor="Red" runat="server" /> <p> Station Number:<br /> <asp:TextBox ID="stationTextBox" runat="server" CssClass="textbox" /> <asp:RequiredFieldValidator ID="stationNumReq" runat="server" ControlToValidate="stationTextBox" ErrorMessage="<br />You must enter a station number!" Display="Dynamic" /> <asp:CompareValidator ID="stationNumCheck" runat="server" ControlToValidate="stationTextBox" Operator="DataTypeCheck" Type="Integer" ErrorMessage="<br />The value must be a number!" Display="Dynamic" /> <asp:RangeValidator ID="stationNumRangeCheck" runat="server" ControlToValidate="stationTextBox" MinimumValue="1" MaximumValue="50" Type="Integer" ErrorMessage="<br />Number must be between 1 and 50." Display="Dynamic" /> </p> <p> Problem Category:<br /> <asp:DropDownList ID="categoryList" runat="server" CssClass="dropdownmenu" /> </p> <p> Problem Subject:<br /> <asp:DropDownList ID="subjectList" runat="server" CssClass="dropdownmenu" /> </p> <p> Problem Description:<br /> <asp:TextBox ID="descriptionTextBox" runat="server" CssClass="textbox" Columns="40" Rows="4" TextMode="MultiLine" /> <asp:RequiredFieldValidator ID="descriptionReq" runat="server" ControlToValidate="descriptionTextBox" ErrorMessage="<br />You must enter a description!" Display="Dynamic" /> </p> <p> <asp:Button ID="submitButton" runat="server" CssClass="button" Text="Submit Request" onclick="submitButton_Click" /> </p> </asp:Content>
Code-behind:
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Data.SqlClient; using System.Configuration; public partial class HelpDesk : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { SqlConnection conn; SqlCommand categoryComm; SqlCommand subjectComm; SqlDataReader reader; string connectionString = ConfigurationManager.ConnectionStrings["Dorknozzle"].ConnectionString; conn = new SqlConnection(connectionString); categoryComm = new SqlCommand("SELECT CategoryID, Category FROM HelpDeskCategories", conn); subjectComm = new SqlCommand( "SELECT SubjectID, Subject FROM HelpDeskSubjects", conn); try { conn.Open(); reader = categoryComm.ExecuteReader(); categoryList.DataSource = reader; categoryList.DataValueField = "CategoryID"; categoryList.DataValueField = "Category"; categoryList.DataBind(); reader.Close(); reader = subjectComm.ExecuteReader(); subjectList.DataSource = reader; subjectList.DataValueField = "SubjectID"; subjectList.DataValueField = "Subject"; subjectList.DataBind(); reader.Close(); } finally { conn.Close(); } } } protected void submitButton_Click(object sender, EventArgs e) { if (Page.IsValid) { SqlConnection conn; SqlCommand comm; string connectionString = ConfigurationManager.ConnectionStrings["Dorknozzle"].ConnectionString; conn = new SqlConnection(connectionString); comm = new SqlCommand("INSERT INTO HelpDesk (EmployeeID, StationNumber, " + "CategoryID, SubjectID, Description, StatusID) " + "VALUES (@EmployeeID, @StationNumber, @CategoryID, " + "@SubjectID, @Description, @StatusID)", conn); comm.Parameters.Add("@EmployeeID", System.Data.SqlDbType.Int); comm.Parameters["@EmployeeID"].Value = 5; comm.Parameters.Add("@StationNumber", System.Data.SqlDbType.Int); comm.Parameters["@StationNumber"].Value = stationTextBox.Text; comm.Parameters.Add("@CategoryID", System.Data.SqlDbType.Int); comm.Parameters["@CategoryID"].Value = categoryList.SelectedItem.Value; comm.Parameters.Add("@SubjectID", System.Data.SqlDbType.Int); comm.Parameters["@SubjectID"].Value = subjectList.SelectedItem.Value; comm.Parameters.Add("@Description", System.Data.SqlDbType.NVarChar, 50); comm.Parameters["@Description"].Value = descriptionTextBox.Text; comm.Parameters.Add("@StatusID", System.Data.SqlDbType.Int); comm.Parameters["@StatusID"].Value = 1; try { conn.Open(); comm.ExecuteNonQuery(); Response.Redirect("HelpDesk.aspx"); } catch { dbErrorMessage.Text = "Error submitting the help desk request! Please " + "try again later, and/or change the entered data!"; } finally { conn.Close(); } } } }
It only display my error message.
-
Thank you so much! I will work with this some more so I can understand it better. I've wanted to switch to C# anyway, I was just being taught VB from my book I was learning from. :-) Thanks so much for the help!
-
I need to display it back to the user. Say they select a color, like red, from the option list. Then it will say, next to the Suggested Pet Name, whatever value I set to be displayed. Like, if the user chooses red, I'd like to display the name, Sunny. How would I do that? I added the dropdown list in place of the other one:
<asp:DropDownList ID="DropDownList1" runat="server">
<asp:ListItem>Red</asp:ListItem>
<asp:ListItem>Blue</asp:ListItem>
<asp:ListItem>Yellow</asp:ListItem>
<asp:ListItem>Green</asp:ListItem>
</asp:DropDownList>
I could do it in C# if you could show me how!
-
How can I add a value to what displays from a function in my class when a user chooses an option from my drop down menu?
Here is my drop down menu:
<select id="dd">
<option>Red</option>
<option>Yellow</option>
<option>Green</option>
</select>
Then here is my class:
<script runat="server">
Class PetName
Public FirstName As String
Function GetName() As String
Dim Whole As String
Whole = FirstName
Return (Whole)
End Function
End Class
</script>
And here is where I set the value to be displayed (I currently have it set to a literal value, but I would like to set it to a value that is set when the user chooses an option in the select menu):
<%
Dim pet1 As PetName
pet1 = New PetName
pet1.FirstName = "Bob"
%>
And here is where it displays the value on the page:
<p>Suggested Pet Name: <%=pet1.GetName%></p>
I know I can probably use an if/else statement, but how do I set it up? Do I set it within my Dim statement? If so, how?
Say I want to do this:
if dd.SelectedItem.Text = "Red" Then pet1.FirstName = "Sunny"
elseif dd.SelectedItem.Text = "Blue Then pet1.FirstName = "Ices"
Am I on the right track? How do I actually do this?
-
I figured out a way to do what I wanted:
<script runat="server"> sub Page_Load if Not Page.IsPostBack then Dim petInformation = New Hashtable petInformation.Add("G", "Grooming") petInformation.Add("H", "Health") petInformation.Add("F", "Feeding") petInformation.Add("E", "Exercise") petInformation.Add("S", "Species") dd.DataSource = petInformation dd.DataValueField="Key" dd.DataTextField="Value" dd.DataBind() end if end sub Sub displayMessage(ByVal s As Object, ByVal e As EventArgs) If dd.SelectedItem.Text = "Grooming" Then lbl1.Text = "<p>Grooming your pet is specific to each kind of pet. For<br> example, you can give your dog a bath in the bathtub, while<br> cats require a special foaming cleanser instead. Some pets<br> require more grooming than others, so be sure to read the <br>information thoroughly. Some grooming might even be <br>covered by a pet's insurance, especially those that require <br>excessive grooming. Choose your pet type below for more<br> specific information:</p>" ElseIf dd.SelectedItem.Text = "Health" Then lbl1.Text = "Keeping your pet healthy is very important. Ensuring they <br>have the proper nutrition for their age, exercise, and <br>supplements is an important to ensure good health for your <br>pet. Choose your pet type below for appropriate preventive <br>and supplemental health products and exercise we <br>recommend:" ElseIf dd.SelectedItem.Text = "Feeding" Then lbl1.Text = "Feeding your pet the proper amounts is very crucial in <br>keeping your pet at a healthy weight. Too much can cause <br>him to be overweight, while too little can cause him to be <br>malnourished. Choose your pet type below for charts <br>showing proper nutrition according to age and weight:" ElseIf dd.SelectedItem.Text = "Exercise" Then lbl1.Text = "Getting the right amount of exercise ensures that your pet<br> stays healthy and doesn't become overweight. This also <br>helps to keep his joints and muscles strong and in good <br>condition. Choose your pet type below to see some <br>recommendations for exercise for your pet:" ElseIf dd.SelectedItem.Text = "Species" Then lbl1.Text = "Each pet has unique characteristics. Some pets <br>require special care because of their species. Others, a <br>special diet. Knowing more about your pet will help <br>you to take better care of him. Select your pet's species<br> below to learn more about your pet:" End If End Sub </script> <html> <body> <form id="Form1" runat="server"> <asp:DropDownList id="dd" runat="server" AutoPostBack="True" onSelectedIndexChanged="displayMessage" /> <p><asp:label id="lbl1" runat="server" /></p> </form> </body> </html>
-
I need help with the following form:
page one:
form
page two:
summary of form values,
confirm button (takes to default.aspx),
cancel button (takes back to form with values still filled in)
I have these pages currently:
page one:
<!-- UserForm.aspx --> <%@ Page Language="VB" ClassName="SenderClass" %> <script runat="server"> ' Readonly property for name Public ReadOnly Property Name() As String Get Return USerName.Text End Get End Property 'Readonly Property for phone Public ReadOnly Property Phone() As String Get Return UserPhone.Text End Get End Property 'Event to transfer page control to Result.aspx Sub Page_Transfer(sender As Object, e As EventArgs) Server.Transfer("Result.aspx") End sub </script> <html> <head> </head> <body> <form id="Form1" runat="server"> User Name: <asp:TextBox ID="UserName" runat="server" /> Phone: <asp:TextBox ID="UserPhone" runat="server" /><br> <asp:Button ID="Button1" Text="Submit" OnClick="Page_Transfer" runat="server" /> </form> </body> </html>
page two:
<!-- Result.aspx --> <%@ Page Language="VB" %> <%@ Reference Page="UserForm.aspx" %> <script runat="server"> Dim result As SenderClass Sub Page_load(obj as Object, e as EventArgs) Dim content As String If Not IsPostBack Then result = CType(Context.Handler, SenderClass) content = "Name: " + result.Name + "<br>" _ + "Phone: " + result.Phone Label1.text = content End If End Sub </script> <html> <head> </head> <body> <h1>Summary:</h1> <i><form id="Form1" runat="server"> <asp:Label id="Label1" runat="server" /></i><br /><br /> <asp:Button ID="Confirm" runat="server" Text="Confirm" PostBackUrl="default.aspx" /> <asp:Button ID="Cancel" runat="server" Text="Cancel" /></form> </body> </html>
Page two displays the summary, and has the confirm and cancel button. I have confirm set to go to the default.aspx page, but how do I get the cancel button to take the user back to the form (userform.aspx) with the form fields filled in with the previous data the user had filed in?
-
Is there a way to have a hash table as a select list that, when the user selects an option, it populates a paragraph for that option in a label? I have the code that displays the selected item's value, but I don't know how to add a paragraph or sentence that doesn't show up in the select list. I want a few options, and then wen they pick an option, it says something else (like something that wasn't in the list, like a paragraph or sentence about the option picked). Can anyone help with that?
I'm working with this:
<script runat="server"> sub Page_Load if Not Page.IsPostBack then dim mycountries=New Hashtable mycountries.Add("G", "Grooming") mycountries.Add("H", "Health") mycountries.Add("F", "Feeding") mycountries.Add("E", "Exercise") mycountries.Add("S", "Species") dd.DataSource=mycountries dd.DataValueField="Key" dd.DataTextField="Value" dd.DataBind() end if end sub sub displayMessage(s as Object,e As EventArgs) lbl1.text="Your favorite country is: " & dd.SelectedItem.Text end sub </script> <html> <body> <form id="Form1" runat="server"> <asp:DropDownList id="dd" runat="server" AutoPostBack="True" onSelectedIndexChanged="displayMessage" /> <p><asp:label id="lbl1" runat="server" /></p> </form> </body> </html>
How can I do this? Any help?
-
Why does username have to be ids? My usernames are the username the visitor registers. I haven't read the normalization article yet. I don't think I'm going to get this. :-( Thanks for the help anyway.
I have the id in both tables set to the same number, but I'm not sure the right logic to do this the right way. :-(
-
I tried this on my login page:
<?php $user_area_location = 'account.php'; // Location of the user area # # $error = array(); if(isset($_GET['action'])) { switch($_GET['action']) { case 'logoff': unset($_SESSION['loggedIn']); array_push($error, 'You were logged off.'); $sql = "update mdark_warrior SET active = 0 WHERE id = '$_SESSION[userID]';"; $sql .= "update scouts SET active = 0 WHERE id = '$_SESSION[userID]';"; $sql .= "update knights SET active = 0 WHERE id = '$_SESSION[userID]';"; $sql .= "update fdark_warrior SET active = 0 WHERE id = '$_SESSION[userID]';"; $result = mysql_query($sql); //Poof...characters disabled break; } } if(!$error) { if(empty($_POST['username'])) { array_push($error, 'You didn\'t supply a username'); } if(empty($_POST['password'])) { array_push($error, 'You didn\'t supply a password'); } } if(!$error){ $result = @mysql_query('SELECT id, username, email, name FROM `users` WHERE username = \''.mysql_real_escape_string($_POST['username']).'\' AND password = \''.mysql_real_escape_string(md5($_POST['password'])).'\''); if($row = @mysql_fetch_array($result)) { $_SESSION['loggedIn'] = true; $_SESSION['userName'] = $row['username']; $_SESSION['userMail'] = $row['email']; $_SESSION['name'] = $row['name']; $_SESSION['userID'] = $row['id']; header('Location: '.$user_area_location); die('<a href="'.$user_area_location.'">Go to your user account</a> or go back to <a href=choose_character.php>choose_character.php</a>'); }else{ array_push($error, 'The username or password you provided were not correct'); } } ?>
But it's not updating. What did I do wrong?
-
Well, okay, that sounds good I think. LOL Can you tell I'm still confused on how to do it? How will I get the id at this point? Sorry I asking for so much help!
-
Okay, one more question: where you have WHERE user = 56, how am I going to set this up? Should it be something like:
WHERE username = '$_SESSION[userName]' ?
I'm confused about how to do this exactly?
-
zanus:
This is my login page. I don' have a logout page, so where would I put the update script?
<?php session_start(); //Access Tracking Snippet //set up static variables $page_title = "login.php"; $user_agent = getenv("HTTP_USER_AGENT"); $date_accessed = date("Y-m-d"); include("connect_db.php"); //create and issue query $sql = "insert into access_tracker values ('', '$page_title', '$user_agent', '$date_accessed')"; mysql_query($sql,$conn); ?> <?php $user_area_location = 'account.php'; // Location of the user area # # $error = array(); if(isset($_GET['action'])) { switch($_GET['action']) { case 'logoff': unset($_SESSION['loggedIn']); array_push($error, 'You were logged off.'); break; } } if(!$error) { if(empty($_POST['username'])) { array_push($error, 'You didn\'t supply a username'); } if(empty($_POST['password'])) { array_push($error, 'You didn\'t supply a password'); } } if(!$error){ $result = @mysql_query('SELECT username, email, name FROM `users` WHERE username = \''.mysql_real_escape_string($_POST['username']).'\' AND password = \''.mysql_real_escape_string(md5($_POST['password'])).'\''); if($row = @mysql_fetch_array($result)) { $_SESSION['loggedIn'] = true; $_SESSION['userName'] = $row['username']; $_SESSION['userMail'] = $row['email']; $_SESSION['name'] = $row['name']; header('Location: '.$user_area_location); die('<a href="'.$user_area_location.'">Go to your user account</a> or go back to <a href=choose_character.php>choose_character.php</a>'); }else{ array_push($error, 'The username or password you provided were not correct'); } } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Sailor Moon RPG - Login</title> <!-- Source File --> <style type="text/css" media="screen"> /*<![CDATA[*/ @import url(global.css); /*]]>*/ </style> </head> <body> <!-- HEADER --> <h1 class="logo">Sailor Moon RPG</h1> <!-- /HEADER --> <?php include("topnav.php"); ?> <div id="main"> <?php include("includes/log.php"); ?> <?php include("mainnav.php"); ?> <table cellspacing="2" cellpadding="0" border="0"> <form method="post" action="login.php"> <?php if(isset($error) && $error) { ?> <tr> <td colspan="2"> <ul><?php foreach($error as $key => $value) echo '<li>'.$value.'</li>'; ?></ul> </td> </tr><?php } ?> <tr> <td>Username:</td> <td><input type="text" name="username" /></td> </tr> <tr> <td>Password:</td> <td><input type="password" name="password" /></td> </tr> <tr> <td> </td> <td><input type="submit" name="submit" value="Login!" /> <a href="forgot.php">I forgot my username or password</a></td> </tr> </form> </table> </div> <?php include("bottomnav.php"); ?> <!-- FOOTER --> <div id="footer_wrapper"> <div id="footer"> <p>Sailor Moon and all characters are<br> trademarks of Naoko Takeuchi.</p> <p>Copyright © 2009 Liz Kula. All rights reserved.<br> A product of <a href="#" target="_blank">Web Designs By Liz</a> systems.</p> <div id="foot-nav"><!-- <ul> <li><a href="http://validator.w3.org/check?uri=http://webdesignsbyliz.com/digital/index.php" target="_blank"><img src="http://www.w3.org/Icons/valid-xhtml10-blue" alt="Valid XHTML 1.0 Transitional" height="31" width="88" /></a></li> <li><a href="http://jigsaw.w3.org/css-validator/validator?uri=http://webdesignsbyliz.com/digital/global.css" target="_blank"><img class="c2" src="http://jigsaw.w3.org/css-validator/images/vcss-blue" alt="Valid CSS!" /></a></li> </ul> --></div> </div> </div> <!-- /FOOTER --> </body> </html>
Error in SQL Syntax HELP!!!
in PHP Coding Help
Posted
Okay, that worked! Thank you! But now I get this error:
Notice: Use of undefined constant identity - assumed 'identity'
How can I get rid of this error?