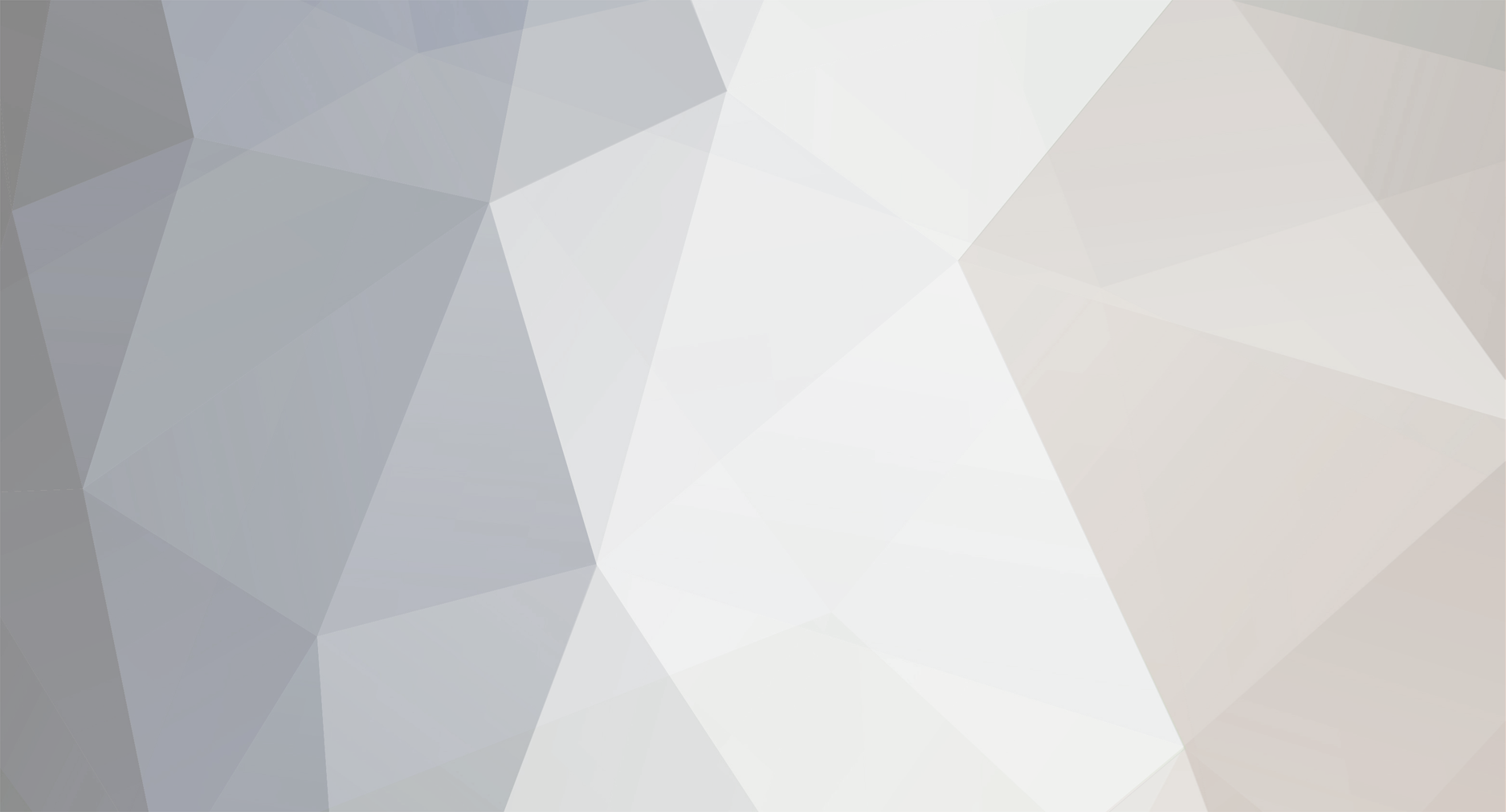
twilitegxa
-
Posts
1,020 -
Joined
-
Last visited
Posts posted by twilitegxa
-
-
Nope, it is still returning a zero value for comment_id :-(
Can it have anything to do with my PHP_SELF action value? I don't know what else could be causing it.
-
It's saying:
[pre]Array
(
)
[/pre]
-
This is what is printed:
INSERT INTO replies (comment_id, reply, reply_create_time, reply_owner,reply_owner_email) VALUES ('', 'fkfkhfmb', now(), 'test','test@aol.com')
I again had to put single quotes around the $reply_comment_id.
-
I got this:
INSERT INTO replies (comment_id, reply, reply_create_time, reply_owner,reply_owner_email) VALUES ('', 'test', now(), 'BriannaCarter','brianna@aol.com')
But I had to add single quotes around the $_GET[comment_id] or else it didn't work at all.
-
Okay, thanks. :-)
-
That's what I was trying to tell you. The information IS inserting, everything except for the comment_id. Every time a record inserts, the comment_id field is inserting as a '0'.
And when I used your last example, it printed out this:
INSERT INTO replies (comment_id, reply, reply_create_time, reply_owner,reply_owner_email) VALUES ('', 'test', now(), 'BriannaCarter','brianna@aol.com')
yes
For some reason, comment_id is inserting as a blank value. And I think I gave you my actual table structure earlier, so I guess I don't need to post it again, right? And yes, each comment can have many replies.
-
Yes, the reply is being inserted into the table in the database, but it isn't showing up on the display page because it displays the replies based on what comment it was replied to. When the posted data inserts, it inserts a '0' into the comment_id field instead of the actual comment_id that is sent through the web address by clicking the link. Can anyone figure out what is wrong?
And I tried your example, Hussam, but nothing changed. :-(
-
And here is the link if you want to test it:
http://webdesignsbyliz.com/testimonials.php
You can just post a new testimonial, then post a reply, or simply post a reply to an existing testimonial and when you click the reply button, you can see that the comment_id is sent along with the link through the address.
-
No, I have two tables. One is for comments and one is for replies. For the replies page, which is the one in question, I need to pull the comment_id from the previous page. I send the comment_id through the link that takes the user to the reply form. For the reply page, when it inserts I have the reply_id set to auto increment, but not the comment_id because it was set to auto increment for the comment table. I hope that made sense. Here are my two tables:
Comment:
CREATE TABLE IF NOT EXISTS `user_comments` ( `comment_id` int(11) NOT NULL auto_increment, `comment` longtext, `comment_create_time` datetime default NULL, `comment_owner` varchar(150) default NULL, `comment_owner_email` varchar(150) default NULL, `url` longtext NOT NULL, PRIMARY KEY (`comment_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=58 ;
Replies:
CREATE TABLE IF NOT EXISTS `replies` ( `reply_id` int(11) NOT NULL auto_increment, `comment_id` int(11) NOT NULL, `reply` longtext, `reply_create_time` datetime default NULL, `reply_owner` varchar(150) NOT NULL, `reply_owner_email` varchar(255) NOT NULL, PRIMARY KEY (`reply_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=28 ;
And here is my first page that sends the comment_id when going to the reply page:
<?php //connect to server and select database $conn = mysql_connect("localhost", "root", "") or die(mysql_error()); mysql_select_db("testimonials", $conn) or die(mysql_error()); //gather the comments $get_comments = "select comment_id, comment, date_format(comment_create_time, '%b %e, %Y at %r') as fmt_comment_create_time, comment_owner, comment_owner_email, url from user_comments order by comment_create_time desc"; $get_comments_res = mysql_query($get_comments, $conn) or die(mysql_error()); if (mysql_num_rows($get_comments_res) < 1) { //there are no comments, so say so $display_block = "<p><em>No comments currently exist. Please submit your testimonial!</em></p>"; } else { //create the display string $display_block = " <table cellpadding=3 cellspacing=2 border=0 width=100%> <tr> <th>COMMENT</th> <th># OF REPLIES</th> </tr>"; while ($comment_info = mysql_fetch_array($get_comments_res)) { $comment_id = $comment_info['comment_id']; $comment = stripslashes($comment_info['comment']); $comment_create_time = $comment_info['fmt_comment_create_time']; $comment_owner = stripslashes($comment_info['comment_owner']); $comment_owner_email = stripslashes($comment_info['comment_owner_email']); $url = stripslashes($comment_info['url']); //get number of replies $get_num_posts = "select count(reply_id) from replies where comment_id = $comment_id"; $get_num_posts_res = mysql_query($get_num_posts, $conn) or die(mysql_error()); $num_posts = mysql_result($get_num_posts_res, 0, 'count(reply_id)'); if ($num_posts == '0') { $num_posts = ' ';} //add to display $display_block .= " <tr> <td><b>Comment By: $comment_owner</b><br> <em>Created on $comment_create_time</em><br> URL: <a href=\"$url\" target=\"_blank\">$url</a><br><br> $comment </td> <td align=center valign=top>$num_posts <a href=\"reply.php?comment_id=$comment_id\">Reply</a></td> </tr> <tr> <td colspan=2><hr></td> </tr>"; //gather the replies $get_replies = "select reply_id, comment_id, reply, date_format(reply_create_time, '%b %e, %Y at %r') as fmt_reply_create_time, reply_owner, reply_owner_email from replies where comment_id = $comment_id order by reply_create_time desc"; $get_replies_res = mysql_query($get_replies, $conn) or die(mysql_error()); while ($reply_info = mysql_fetch_array($get_replies_res)) { $reply_id = $reply_info['reply_id']; $reply = stripslashes($reply_info['reply']); $reply_create_time = $reply_info['fmt_reply_create_time']; $reply_owner = stripslashes($reply_info['reply_owner']); $reply_owner_email = stripslashes($reply_info['reply_owner_email']); //add to display $display_block .= " <tr> <td><div id=reply>Reply</div><div class=reply><b>Comment By: $reply_owner</b><br> <em>Created on $reply_create_time</em><br><br> $reply</div> </td> <td> </td> </tr> <tr> <td colspan=2><hr> </td> </tr>"; } } //close up the table $display_block .= "</table>"; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Web Designs By Liz - Testimonials</title> <style type="text/css" media="screen"> /*<![CDATA[*/ @import url(global.css); /*]]>*/ </style> <script type="text/javascript"> //<![CDATA[ <!-- function MM_preloadImages() { //v3.0 var d=document; if(d.images){ if(!d.MM_p) d.MM_p=new Array(); var i,j=d.MM_p.length,a=MM_preloadImages.arguments; for(i=0; i<a.length; i++) if (a[i].indexOf("#")!=0){ d.MM_p[j]=new Image; d.MM_p[j++].src=a[i];}} } function MM_findObj(n, d) { //v4.01 var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) { d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);} if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n]; for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document); if(!x && d.getElementById) x=d.getElementById(n); return x; } function MM_nbGroup(event, grpName) { //v6.0 var i,img,nbArr,args=MM_nbGroup.arguments; if (event == "init" && args.length > 2) { if ((img = MM_findObj(args[2])) != null && !img.MM_init) { img.MM_init = true; img.MM_up = args[3]; img.MM_dn = img.src; if ((nbArr = document[grpName]) == null) nbArr = document[grpName] = new Array(); nbArr[nbArr.length] = img; for (i=4; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = args[i+1]; nbArr[nbArr.length] = img; } } } else if (event == "over") { document.MM_nbOver = nbArr = new Array(); for (i=1; i < args.length-1; i+=3) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = (img.MM_dn && args[i+2]) ? args[i+2] : ((args[i+1])? args[i+1] : img.MM_up); nbArr[nbArr.length] = img; } } else if (event == "out" ) { for (i=0; i < document.MM_nbOver.length; i++) { img = document.MM_nbOver[i]; img.src = (img.MM_dn) ? img.MM_dn : img.MM_up; } } else if (event == "down") { nbArr = document[grpName]; if (nbArr) for (i=0; i < nbArr.length; i++) { img=nbArr[i]; img.src = img.MM_up; img.MM_dn = 0; } document[grpName] = nbArr = new Array(); for (i=2; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = (args[i+1])? args[i+1] : img.MM_up; nbArr[nbArr.length] = img; } } } //--> //]]> </script> <script type="text/javascript" language="JavaScript"> //<![CDATA[ <!-- function HideContent(d) { document.getElementById(d).style.display = "none"; } function ShowContent(d) { document.getElementById(d).style.display = "block"; } function ReverseDisplay(d) { if(document.getElementById(d).style.display == "none") { document.getElementById(d).style.display = "block"; } else { document.getElementById(d).style.display = "none"; } } //--> //]]> </script> </head> <body onload="MM_preloadImages('home_selected.png','home_hover.png','resume.png','resume_hover.png','resume_selected.png','contact_selected.png','contact_hover.png','portfolio_selected.png','portfolio_hover.png','testimonials.png','testimonials_hover.png','testimonials_selected.png')"> <script language="JavaScript" type="text/javascript"> //<![CDATA[ function doClock() { window.setTimeout( "doClock()", 1000 ); today = new Date(); self.status = today.toString(); } doClock() //]]> </script> <div class="c1" id="logo"><img src="weblogo.png" title="Web Designs By Liz Logo" alt="Web Designs By Liz Logo" /></div> <table align="center" border="0" cellpadding="0" cellspacing="0"> <tr> <td><a href="index.html" target="_top" onclick="MM_nbGroup('down','group1','home','home_selected.png',1)" onmouseover="MM_nbGroup('over','home','home_hover.png','home_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="home.png" alt="Home Page" name="home" border="0" id="home" /></a></td> <td><a href="resume.html" target="_top" onclick="MM_nbGroup('down','group1','resume','resume_selected.png',1)" onmouseover="MM_nbGroup('over','resume','resume_hover.png','resume_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="resume.png" alt="Resume" name="resume" border="0" id="resume" /></a></td> <td><a href="contact.html" target="_top" onclick="MM_nbGroup('down','group1','contact','contact_selected.png',1)" onmouseover="MM_nbGroup('over','contact','contact_hover.png','contact_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="contact.png" alt="Contact Page" name="contact" border="0" id="contact" /></a></td> <td><a href="portfolio.php" target="_top" onclick="MM_nbGroup('down','group1','portfolio','portfolio_selected.png',1)" onmouseover="MM_nbGroup('over','portfolio','portfolio_hover.png','portfolio_selected.png',1)" onmouseout="MM_nbGroup('out')"><img name="portfolio" src="portfolio.png" border="0" alt="Portfolio" /></a></td> <td><a href="testimonials.php" target="_top" onClick="MM_nbGroup('down','group1','testimonials','testimonials_selected.png',1)" onMouseOver="MM_nbGroup('over','testimonials','testimonials_hover.png','testimonials_selected.png',1)" onMouseOut="MM_nbGroup('out')"><img name="testimonials" src="testimonials_selected.png" border="0" alt="Testimonials" onLoad="MM_nbGroup('init','group1','testimonials','testimonials.png',1)" /></a></td> </tr> </table> <div id="resume_title" class="body"><div class="c1"></div> <table width="900" align="center"> <tr> <td class="style2" align="center"> <h1>Testimonials</h1> <p>Post your testimonial <a href="testimonials_form.php">here</a>.</p> </td> </tr> <tr> <td class="style2"> <?php print $display_block; ?> </td> </tr> </table> </div> <div id="footer" class="style2 c1"><br /> <em>Adobe Photoshop, Illustrator, InDesign, Dreamweaver, and Flash logos<br /> are trademarks of Adobe Systems Incorporated.<br /> <br /> Copyright © 2009 Liz Kula. All rights reserved.</em> <div id="foot-nav"> <ul> <li><a href="http://validator.w3.org/check?uri=http://webdesignsbyliz.com/resume.html" target="_blank"><img src="http://www.w3.org/Icons/valid-xhtml10-blue" alt="Valid XHTML 1.0 Transitional" height="31" width="88" /></a></li> <li><a href="http://jigsaw.w3.org/css-validator/validator?uri=http://webdesignsbyliz.com/global.css" target="_blank"><img class="c2" src="http://jigsaw.w3.org/css-validator/images/vcss-blue" alt="Valid CSS!" /></a></li> </ul> </div> </div> </body> </html>
And here is the page that submits the reply form again:
<?php session_start(); //connect to server and select database $conn = mysql_connect("localhost", "root", "") or die(mysql_error()); $db = mysql_select_db("testimonials", $conn) or die(mysql_error()); if (isset($_POST['submit'])) { //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $reply=mysql_real_escape_string($_POST['reply']); $reply_comment_id = $_GET['comment_id']; $error='';//initialize $error to blank if(trim($_POST['comment_owner'])=='' ){ $error.="Please enter your name!<br />"; //concatenate the $error Message with a line break } if(trim($_POST['comment_owner_email'])==''){ $error.="Plese enter your e-mail address!<br />"; } else { if(!preg_match("/^[_\.0-9a-zA-Z-]+@([0-9a-zA-Z][0-9a-zA-Z-]+\.)+[a-zA-Z]{2,6}$/i", $_POST['comment_owner_email'])) { $error.="The e-mail you entered was not valid!<br />"; } } if(trim($_POST['reply'])=='' ){ $error.="Please enter your reply!<br />"; //concatenate the $error Message with a line break } if($error==''){ header('Location: testimonials.php'); $sql="INSERT INTO replies (reply_id, comment_id, reply, reply_create_time, reply_owner, reply_owner_email) VALUES ('', '$reply_comment_id', '$reply', now(), '$name','$email')"; mysql_query($sql,$conn) or die(mysql_error()); } else{ echo "<div class=error><span style=color:red>$error</span><br /></div>"; } } else { $name= ''; $email= ''; $reply= ''; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Web Designs By Liz - Testimonials</title> <style type="text/css" media="screen"> /*<![CDATA[*/ @import url(global.css); /*]]>*/ </style> <script type="text/javascript"> //<![CDATA[ <!-- function MM_preloadImages() { //v3.0 var d=document; if(d.images){ if(!d.MM_p) d.MM_p=new Array(); var i,j=d.MM_p.length,a=MM_preloadImages.arguments; for(i=0; i<a.length; i++) if (a[i].indexOf("#")!=0){ d.MM_p[j]=new Image; d.MM_p[j++].src=a[i];}} } function MM_findObj(n, d) { //v4.01 var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) { d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);} if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n]; for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document); if(!x && d.getElementById) x=d.getElementById(n); return x; } function MM_nbGroup(event, grpName) { //v6.0 var i,img,nbArr,args=MM_nbGroup.arguments; if (event == "init" && args.length > 2) { if ((img = MM_findObj(args[2])) != null && !img.MM_init) { img.MM_init = true; img.MM_up = args[3]; img.MM_dn = img.src; if ((nbArr = document[grpName]) == null) nbArr = document[grpName] = new Array(); nbArr[nbArr.length] = img; for (i=4; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = args[i+1]; nbArr[nbArr.length] = img; } } } else if (event == "over") { document.MM_nbOver = nbArr = new Array(); for (i=1; i < args.length-1; i+=3) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = (img.MM_dn && args[i+2]) ? args[i+2] : ((args[i+1])? args[i+1] : img.MM_up); nbArr[nbArr.length] = img; } } else if (event == "out" ) { for (i=0; i < document.MM_nbOver.length; i++) { img = document.MM_nbOver[i]; img.src = (img.MM_dn) ? img.MM_dn : img.MM_up; } } else if (event == "down") { nbArr = document[grpName]; if (nbArr) for (i=0; i < nbArr.length; i++) { img=nbArr[i]; img.src = img.MM_up; img.MM_dn = 0; } document[grpName] = nbArr = new Array(); for (i=2; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = (args[i+1])? args[i+1] : img.MM_up; nbArr[nbArr.length] = img; } } } //--> //]]> </script> <script type="text/javascript" language="JavaScript"> //<![CDATA[ <!-- function HideContent(d) { document.getElementById(d).style.display = "none"; } function ShowContent(d) { document.getElementById(d).style.display = "block"; } function ReverseDisplay(d) { if(document.getElementById(d).style.display == "none") { document.getElementById(d).style.display = "block"; } else { document.getElementById(d).style.display = "none"; } } //--> //]]> </script> <!-- Validation Script --> <script type="text/javascript"> function validateFormOnSubmit(theForm) { var reason = ""; reason += validateUsername(theForm.comment_owner); reason += validateEmail(theForm.comment_owner_email); reason += validateEmpty(theForm.reply); if (reason != "") { alert("Some fields need correction:\n" + reason); return false; } } function validateUsername(fld) { var error = ""; var illegalChars = /\W/; // allow letters, numbers, and underscores if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your name.\n"; } return error; } function trim(s) { return s.replace(/^\s+|\s+$/, ''); } function validateEmail(fld) { var error=""; var tfld = trim(fld.value); // value of field with whitespace trimmed off var emailFilter = /^[^@]+@[^@.]+\.[^@]*\w\w$/ ; var illegalChars= /[\(\)\<\>\,\;\:\\\"\[\]]/ ; if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your e-mail address.\n"; } else if (!emailFilter.test(tfld)) { //test email for illegal characters fld.style.background = 'Yellow'; error = "Please enter a valid e-mail address.\n"; } else if (fld.value.match(illegalChars)) { fld.style.background = 'Yellow'; error = "Your e-mail address contains illegal characters.\n"; } else { fld.style.background = 'White'; } return error; } function validateEmpty(fld) { var error = ""; if (fld.value.length == 0) { fld.style.background = 'Yellow'; error = "Please enter your reply.\n" } else { fld.style.background = 'White'; } return error; } </script> <!--End Validation Script --> </head> <body onload="MM_preloadImages('home_selected.png','home_hover.png','resume.png','resume_hover.png','resume_selected.png','contact_selected.png','contact_hover.png','portfolio_selected.png','portfolio_hover.png')"> <script language="JavaScript" type="text/javascript"> //<![CDATA[ function doClock() { window.setTimeout( "doClock()", 1000 ); today = new Date(); self.status = today.toString(); } doClock() //]]> </script> <div class="c1" id="logo"><img src="weblogo.png" title="Web Designs By Liz Logo" alt="Web Designs By Liz Logo" /></div> <table align="center" border="0" cellpadding="0" cellspacing="0"> <tr> <td><a href="index.html" target="_top" onclick="MM_nbGroup('down','group1','home','home_selected.png',1)" onmouseover="MM_nbGroup('over','home','home_hover.png','home_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="home.png" alt="Home Page" name="home" border="0" id="home" /></a></td> <td><a href="resume.html" target="_top" onclick="MM_nbGroup('down','group1','resume','resume_selected.png',1)" onmouseover="MM_nbGroup('over','resume','resume_hover.png','resume_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="resume.png" alt="Resume" name="resume" border="0" id="resume" /></a></td> <td><a href="contact.html" target="_top" onclick="MM_nbGroup('down','group1','contact','contact_selected.png',1)" onmouseover="MM_nbGroup('over','contact','contact_hover.png','contact_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="contact.png" alt="Contact Page" name="contact" border="0" id="contact" /></a></td> <td><a href="portfolio.php" target="_top" onclick="MM_nbGroup('down','group1','portfolio','portfolio_selected.png',1)" onmouseover="MM_nbGroup('over','portfolio','portfolio_hover.png','portfolio_selected.png',1)" onmouseout="MM_nbGroup('out')"><img name="portfolio" src="portfolio.png" border="0" alt="Portfolio" /></a></td> <td><a href="testimonials.php" target="_top" onclick="MM_nbGroup('down','group1','testimonials','testimonials_selected.png',1)" onmouseover="MM_nbGroup('over','testimonials','testimonials_hover.png','testimonials_selected.png',1)" onmouseout="MM_nbGroup('out')"><img name="testimonials" src="testimonials_selected.png" border="0" alt="Testimonials" /></a></td> </tr> </table> <div id="resume_title" class="body"><div class="c1"></div> <table width="900" align="center"> <tr> <td class="style2" align="center"> <h1>Give Your Testimonial</h1> </td> </tr> <tr> <td class="style2"> <table class="style2" align="center"> <tr> <td> <form name="comment" id="comment" onsubmit="return validateFormOnSubmit(this)" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="name"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" value="<?php echo $name; ?>"/></div></td> </tr> <tr> <td align="left"><label for="email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" value="<?php echo $email; ?>"/></div></td> </tr> <tr> <td align="left"> <label for="reply">Reply:</label></td> <td> <textarea name="reply" id="reply" rows="5" cols="30" value="<?php echo $reply; ?>"></textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div></td> <td width="2"></td> </tr> </table> </form> </td> </tr> </table> </td> </tr> </table> <?php echo $reply_comment_id; ?> </div> <div id="footer" class="style2 c1"><br /> <em>Adobe Photoshop, Illustrator, InDesign, Dreamweaver, and Flash logos<br /> are trademarks of Adobe Systems Incorporated.<br /> <br /> Copyright © 2009 Liz Kula. All rights reserved.</em> <div id="foot-nav"> <ul> <li><a href="http://validator.w3.org/check?uri=http://webdesignsbyliz.com/resume.html" target="_blank"><img src="http://www.w3.org/Icons/valid-xhtml10-blue" alt="Valid XHTML 1.0 Transitional" height="31" width="88" /></a></li> <li><a href="http://jigsaw.w3.org/css-validator/validator?uri=http://webdesignsbyliz.com/global.css" target="_blank"><img class="c2" src="http://jigsaw.w3.org/css-validator/images/vcss-blue" alt="Valid CSS!" /></a></li> </ul> </div> </div> </body> </html>
So when the viewer selects a post to reply to, it should send along with it the comment_id, and then when the reply is submitted, it should insert that comment_id with the rest of the reply information. Is it because I am using the PHP_SELF in the action value? Or did I do something else wrong?
-
I have the following page:
<?php session_start(); //connect to server and select database $conn = mysql_connect("localhost", "root", "") or die(mysql_error()); $db = mysql_select_db("testimonials", $conn) or die(mysql_error()); if (isset($_POST['submit'])) { //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $reply=mysql_real_escape_string($_POST['reply']); $reply_comment_id = $_GET['comment_id']; $error='';//initialize $error to blank if(trim($_POST['comment_owner'])=='' ){ $error.="Please enter your name!<br />"; //concatenate the $error Message with a line break } if(trim($_POST['comment_owner_email'])==''){ $error.="Plese enter your e-mail address!<br />"; } else { if(!preg_match("/^[_\.0-9a-zA-Z-]+@([0-9a-zA-Z][0-9a-zA-Z-]+\.)+[a-zA-Z]{2,6}$/i", $_POST['comment_owner_email'])) { $error.="The e-mail you entered was not valid!<br />"; } } if(trim($_POST['reply'])=='' ){ $error.="Please enter your reply!<br />"; //concatenate the $error Message with a line break } if($error==''){ header('Location: testimonials.php'); $sql="INSERT INTO replies (reply_id, comment_id, reply, reply_create_time, reply_owner, reply_owner_email) VALUES ('', '$reply_comment_id', '$reply', now(), '$name','$email')"; mysql_query($sql,$conn) or die(mysql_error()); } else{ echo "<div class=error><span style=color:red>$error</span><br /></div>"; } } else { $name= ''; $email= ''; $reply= ''; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Web Designs By Liz - Testimonials</title> <style type="text/css" media="screen"> /*<![CDATA[*/ @import url(global.css); /*]]>*/ </style> <script type="text/javascript"> //<![CDATA[ <!-- function MM_preloadImages() { //v3.0 var d=document; if(d.images){ if(!d.MM_p) d.MM_p=new Array(); var i,j=d.MM_p.length,a=MM_preloadImages.arguments; for(i=0; i<a.length; i++) if (a[i].indexOf("#")!=0){ d.MM_p[j]=new Image; d.MM_p[j++].src=a[i];}} } function MM_findObj(n, d) { //v4.01 var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) { d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);} if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n]; for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document); if(!x && d.getElementById) x=d.getElementById(n); return x; } function MM_nbGroup(event, grpName) { //v6.0 var i,img,nbArr,args=MM_nbGroup.arguments; if (event == "init" && args.length > 2) { if ((img = MM_findObj(args[2])) != null && !img.MM_init) { img.MM_init = true; img.MM_up = args[3]; img.MM_dn = img.src; if ((nbArr = document[grpName]) == null) nbArr = document[grpName] = new Array(); nbArr[nbArr.length] = img; for (i=4; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = args[i+1]; nbArr[nbArr.length] = img; } } } else if (event == "over") { document.MM_nbOver = nbArr = new Array(); for (i=1; i < args.length-1; i+=3) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = (img.MM_dn && args[i+2]) ? args[i+2] : ((args[i+1])? args[i+1] : img.MM_up); nbArr[nbArr.length] = img; } } else if (event == "out" ) { for (i=0; i < document.MM_nbOver.length; i++) { img = document.MM_nbOver[i]; img.src = (img.MM_dn) ? img.MM_dn : img.MM_up; } } else if (event == "down") { nbArr = document[grpName]; if (nbArr) for (i=0; i < nbArr.length; i++) { img=nbArr[i]; img.src = img.MM_up; img.MM_dn = 0; } document[grpName] = nbArr = new Array(); for (i=2; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = (args[i+1])? args[i+1] : img.MM_up; nbArr[nbArr.length] = img; } } } //--> //]]> </script> <script type="text/javascript" language="JavaScript"> //<![CDATA[ <!-- function HideContent(d) { document.getElementById(d).style.display = "none"; } function ShowContent(d) { document.getElementById(d).style.display = "block"; } function ReverseDisplay(d) { if(document.getElementById(d).style.display == "none") { document.getElementById(d).style.display = "block"; } else { document.getElementById(d).style.display = "none"; } } //--> //]]> </script> <!-- Validation Script --> <script type="text/javascript"> function validateFormOnSubmit(theForm) { var reason = ""; reason += validateUsername(theForm.comment_owner); reason += validateEmail(theForm.comment_owner_email); reason += validateEmpty(theForm.reply); if (reason != "") { alert("Some fields need correction:\n" + reason); return false; } } function validateUsername(fld) { var error = ""; var illegalChars = /\W/; // allow letters, numbers, and underscores if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your name.\n"; } return error; } function trim(s) { return s.replace(/^\s+|\s+$/, ''); } function validateEmail(fld) { var error=""; var tfld = trim(fld.value); // value of field with whitespace trimmed off var emailFilter = /^[^@]+@[^@.]+\.[^@]*\w\w$/ ; var illegalChars= /[\(\)\<\>\,\;\:\\\"\[\]]/ ; if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your e-mail address.\n"; } else if (!emailFilter.test(tfld)) { //test email for illegal characters fld.style.background = 'Yellow'; error = "Please enter a valid e-mail address.\n"; } else if (fld.value.match(illegalChars)) { fld.style.background = 'Yellow'; error = "Your e-mail address contains illegal characters.\n"; } else { fld.style.background = 'White'; } return error; } function validateEmpty(fld) { var error = ""; if (fld.value.length == 0) { fld.style.background = 'Yellow'; error = "Please enter your reply.\n" } else { fld.style.background = 'White'; } return error; } </script> <!--End Validation Script --> </head> <body onload="MM_preloadImages('home_selected.png','home_hover.png','resume.png','resume_hover.png','resume_selected.png','contact_selected.png','contact_hover.png','portfolio_selected.png','portfolio_hover.png')"> <script language="JavaScript" type="text/javascript"> //<![CDATA[ function doClock() { window.setTimeout( "doClock()", 1000 ); today = new Date(); self.status = today.toString(); } doClock() //]]> </script> <div class="c1" id="logo"><img src="weblogo.png" title="Web Designs By Liz Logo" alt="Web Designs By Liz Logo" /></div> <table align="center" border="0" cellpadding="0" cellspacing="0"> <tr> <td><a href="index.html" target="_top" onclick="MM_nbGroup('down','group1','home','home_selected.png',1)" onmouseover="MM_nbGroup('over','home','home_hover.png','home_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="home.png" alt="Home Page" name="home" border="0" id="home" /></a></td> <td><a href="resume.html" target="_top" onclick="MM_nbGroup('down','group1','resume','resume_selected.png',1)" onmouseover="MM_nbGroup('over','resume','resume_hover.png','resume_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="resume.png" alt="Resume" name="resume" border="0" id="resume" /></a></td> <td><a href="contact.html" target="_top" onclick="MM_nbGroup('down','group1','contact','contact_selected.png',1)" onmouseover="MM_nbGroup('over','contact','contact_hover.png','contact_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="contact.png" alt="Contact Page" name="contact" border="0" id="contact" /></a></td> <td><a href="portfolio.php" target="_top" onclick="MM_nbGroup('down','group1','portfolio','portfolio_selected.png',1)" onmouseover="MM_nbGroup('over','portfolio','portfolio_hover.png','portfolio_selected.png',1)" onmouseout="MM_nbGroup('out')"><img name="portfolio" src="portfolio.png" border="0" alt="Portfolio" /></a></td> <td><a href="testimonials.php" target="_top" onclick="MM_nbGroup('down','group1','testimonials','testimonials_selected.png',1)" onmouseover="MM_nbGroup('over','testimonials','testimonials_hover.png','testimonials_selected.png',1)" onmouseout="MM_nbGroup('out')"><img name="testimonials" src="testimonials_selected.png" border="0" alt="Testimonials" /></a></td> </tr> </table> <div id="resume_title" class="body"><div class="c1"></div> <table width="900" align="center"> <tr> <td class="style2" align="center"> <h1>Give Your Testimonial</h1> </td> </tr> <tr> <td class="style2"> <table class="style2" align="center"> <tr> <td> <form name="comment" id="comment" onsubmit="return validateFormOnSubmit(this)" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="name"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" value="<?php echo $name; ?>"/></div></td> </tr> <tr> <td align="left"><label for="email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" value="<?php echo $email; ?>"/></div></td> </tr> <tr> <td align="left"> <label for="reply">Reply:</label></td> <td> <textarea name="reply" id="reply" rows="5" cols="30" value="<?php echo $reply; ?>"></textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div></td> <td width="2"></td> </tr> </table> </form> </td> </tr> </table> </td> </tr> </table> </div> <div id="footer" class="style2 c1"><br /> <em>Adobe Photoshop, Illustrator, InDesign, Dreamweaver, and Flash logos<br /> are trademarks of Adobe Systems Incorporated.<br /> <br /> Copyright © 2009 Liz Kula. All rights reserved.</em> <div id="foot-nav"> <ul> <li><a href="http://validator.w3.org/check?uri=http://webdesignsbyliz.com/resume.html" target="_blank"><img src="http://www.w3.org/Icons/valid-xhtml10-blue" alt="Valid XHTML 1.0 Transitional" height="31" width="88" /></a></li> <li><a href="http://jigsaw.w3.org/css-validator/validator?uri=http://webdesignsbyliz.com/global.css" target="_blank"><img class="c2" src="http://jigsaw.w3.org/css-validator/images/vcss-blue" alt="Valid CSS!" /></a></li> </ul> </div> </div> </body> </html>
I need to send the $reply_comment_id along with the form when it inserts, but it's not inserting. It looks like it is sending it in the address when you click the reply button on a post on the previous page:
http://webdesignsbyliz.com/reply.php?comment_id=57
As you can see above, it does send the ID, but it won't insert it. Can anyone help? What am I doing wrong?
-
I tried putting the php script before my html script since you can't have anything in the browser before the header redirect thing, and I just put a div tag around the error messages and used css to display them where I wanted, and that worked fine. Here is the revised code:
<?php //connect to server and select database $conn = mysql_connect("localhost", "webdes17_twilite", "minimoon") or die(mysql_error()); $db = mysql_select_db("webdes17_testimonials", $conn) or die(mysql_error()); if (isset($_POST['submit'])) { //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $url=mysql_real_escape_string($_POST['url']); $comment=mysql_real_escape_string($_POST['comment']); $error='';//initialize $error to blank if(trim($_POST['comment_owner'])=='' ){ $error.="Please enter your name!<br />"; //concatenate the $error Message with a line break } if(trim($_POST['url'])==''){ $error.="Please enter your web address!<br />";//concatenate more to $error } else { if(!preg_match('|^http(s)?://[a-z0-9-]+(.[a-z0-9-]+)*(:[0-9]+)?(/.*)?$|i', $_POST['url'])) { $error.="The URL you entered was not valid!<br />"; } } if(trim($_POST['comment_owner_email'])==''){ $error.="Plese enter your e-mail address!<br />"; } else { if(!preg_match("/^[_\.0-9a-zA-Z-]+@([0-9a-zA-Z][0-9a-zA-Z-]+\.)+[a-zA-Z]{2,6}$/i", $_POST['comment_owner_email'])) { $error.="The e-mail you entered was not valid!<br />"; } } if(trim($_POST['comment'])=='' ){ $error.="Please enter your comment!<br />"; //concatenate the $error Message with a line break } if($error==''){ header( 'Location: testimonials.php' ) ; $sql="INSERT INTO user_comments (comment_id, comment, comment_create_time, comment_owner, comment_owner_email, url) VALUES ('', '$comment', now(), '$name','$email', '$url')"; mysql_query($sql,$conn) or die(mysql_error()); } else{ echo "<div class=error><span style=color:red>$error</span><br /></div>"; } } else { $name= ''; $email= ''; $url= ''; $comment= ''; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Web Designs By Liz - Testimonials</title> <style type="text/css" media="screen"> /*<![CDATA[*/ @import url(global.css); /*]]>*/ </style> <script type="text/javascript"> //<![CDATA[ <!-- function MM_preloadImages() { //v3.0 var d=document; if(d.images){ if(!d.MM_p) d.MM_p=new Array(); var i,j=d.MM_p.length,a=MM_preloadImages.arguments; for(i=0; i<a.length; i++) if (a[i].indexOf("#")!=0){ d.MM_p[j]=new Image; d.MM_p[j++].src=a[i];}} } function MM_findObj(n, d) { //v4.01 var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) { d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);} if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n]; for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document); if(!x && d.getElementById) x=d.getElementById(n); return x; } function MM_nbGroup(event, grpName) { //v6.0 var i,img,nbArr,args=MM_nbGroup.arguments; if (event == "init" && args.length > 2) { if ((img = MM_findObj(args[2])) != null && !img.MM_init) { img.MM_init = true; img.MM_up = args[3]; img.MM_dn = img.src; if ((nbArr = document[grpName]) == null) nbArr = document[grpName] = new Array(); nbArr[nbArr.length] = img; for (i=4; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = args[i+1]; nbArr[nbArr.length] = img; } } } else if (event == "over") { document.MM_nbOver = nbArr = new Array(); for (i=1; i < args.length-1; i+=3) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = (img.MM_dn && args[i+2]) ? args[i+2] : ((args[i+1])? args[i+1] : img.MM_up); nbArr[nbArr.length] = img; } } else if (event == "out" ) { for (i=0; i < document.MM_nbOver.length; i++) { img = document.MM_nbOver[i]; img.src = (img.MM_dn) ? img.MM_dn : img.MM_up; } } else if (event == "down") { nbArr = document[grpName]; if (nbArr) for (i=0; i < nbArr.length; i++) { img=nbArr[i]; img.src = img.MM_up; img.MM_dn = 0; } document[grpName] = nbArr = new Array(); for (i=2; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = (args[i+1])? args[i+1] : img.MM_up; nbArr[nbArr.length] = img; } } } //--> //]]> </script> <script type="text/javascript" language="JavaScript"> //<![CDATA[ <!-- function HideContent(d) { document.getElementById(d).style.display = "none"; } function ShowContent(d) { document.getElementById(d).style.display = "block"; } function ReverseDisplay(d) { if(document.getElementById(d).style.display == "none") { document.getElementById(d).style.display = "block"; } else { document.getElementById(d).style.display = "none"; } } //--> //]]> </script> <!-- Validation Script --> <script type="text/javascript"> function validateFormOnSubmit(theForm) { var reason = ""; reason += validateUsername(theForm.comment_owner); reason += validateEmail(theForm.comment_owner_email); reason += validatePhone(theForm.url); reason += validateEmpty(theForm.comment); if (reason != "") { alert("Some fields need correction:\n" + reason); return false; } } function validateUsername(fld) { var error = ""; var illegalChars = /\W/; // allow letters, numbers, and underscores if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your name.\n"; } return error; } function trim(s) { return s.replace(/^\s+|\s+$/, ''); } function validateEmail(fld) { var error=""; var tfld = trim(fld.value); // value of field with whitespace trimmed off var emailFilter = /^[^@]+@[^@.]+\.[^@]*\w\w$/ ; var illegalChars= /[\(\)\<\>\,\;\:\\\"\[\]]/ ; if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your e-mail address.\n"; } else if (!emailFilter.test(tfld)) { //test email for illegal characters fld.style.background = 'Yellow'; error = "Please enter a valid e-mail address.\n"; } else if (fld.value.match(illegalChars)) { fld.style.background = 'Yellow'; error = "Your e-mail address contains illegal characters.\n"; } else { fld.style.background = 'White'; } return error; } function validatePhone(fld) { var error = ""; var illegalChars = /\W/; // allow letters, numbers, and underscores var urlfld = trim(fld.value); // value of field with whitespace trimmed off var urlFilter = /http:\/\/[A-Za-z0-9\.-]{3,}\.[A-Za-z]{3}/; if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your web address.\n"; } else if (!urlFilter.test(urlfld)) { //test url for illegal characters fld.style.background = 'Yellow'; error = "Please enter a valid web address.\n"; } return error; } function validateEmpty(fld) { var error = ""; if (fld.value.length == 0) { fld.style.background = 'Yellow'; error = "Please enter your comment.\n" } else { fld.style.background = 'White'; } return error; } </script> <!--End Validation Script --> </head> <body onload="MM_preloadImages('home_selected.png','home_hover.png','resume.png','resume_hover.png','resume_selected.png','contact_selected.png','contact_hover.png','portfolio_selected.png','portfolio_hover.png')"> <script language="JavaScript" type="text/javascript"> //<![CDATA[ function doClock() { window.setTimeout( "doClock()", 1000 ); today = new Date(); self.status = today.toString(); } doClock() //]]> </script> <div class="c1" id="logo"><img src="weblogo.png" title="Web Designs By Liz Logo" alt="Web Designs By Liz Logo" /></div> <table align="center" border="0" cellpadding="0" cellspacing="0"> <tr> <td><a href="index.html" target="_top" onclick="MM_nbGroup('down','group1','home','home_selected.png',1)" onmouseover="MM_nbGroup('over','home','home_hover.png','home_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="home.png" alt="Home Page" name="home" border="0" id="home" /></a></td> <td><a href="resume.html" target="_top" onclick="MM_nbGroup('down','group1','resume','resume_selected.png',1)" onmouseover="MM_nbGroup('over','resume','resume_hover.png','resume_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="resume.png" alt="Resume" name="resume" border="0" id="resume" /></a></td> <td><a href="contact.html" target="_top" onclick="MM_nbGroup('down','group1','contact','contact_selected.png',1)" onmouseover="MM_nbGroup('over','contact','contact_hover.png','contact_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="contact.png" alt="Contact Page" name="contact" border="0" id="contact" /></a></td> <td><a href="portfolio.php" target="_top" onclick="MM_nbGroup('down','group1','portfolio','portfolio_selected.png',1)" onmouseover="MM_nbGroup('over','portfolio','portfolio_hover.png','portfolio_selected.png',1)" onmouseout="MM_nbGroup('out')"><img name="portfolio" src="portfolio.png" border="0" alt="Portfolio" /></a></td> <td><a href="testimonials.php" target="_top" onclick="MM_nbGroup('down','group1','testimonials','testimonials_selected.png',1)" onmouseover="MM_nbGroup('over','testimonials','testimonials_hover.png','testimonials_selected.png',1)" onmouseout="MM_nbGroup('out')"><img name="testimonials" src="testimonials_selected.png" border="0" alt="Testimonials" /></a></td> </tr> </table> <div id="resume_title" class="body"><div class="c1"></div> <table width="900" align="center"> <tr> <td class="style2" align="center"> <h1>Give Your Testimonial</h1> </td> </tr> <tr> <td class="style2"> <table class="style2" align="center"> <tr> <td> <form name="comment" id="comment" onsubmit="return validateFormOnSubmit(this)" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="name"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" value="<?php echo $name; ?>"/></div> </td> </tr> <tr> <td align="left"><label for="email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" value="<?php echo $email; ?>"/></div> </td> </tr> <tr> <td align="left"><label for="url">URL:</label></td> <td> <div class="c2"><input type="text" name="url" id="url" size="30" value="<?php echo $url; ?>"/></div> </td> </tr> <tr> <td align="left"> <label for="comment">Comments:</label> </td> <td> <textarea name="comment" id="comment" rows="5" cols="30" value="<?php echo $comment; ?>"> </textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div> </td> <td width="2"></td> </tr> </table> </form> </td> </tr> </table> </td> </tr> </table> </div> <div id="footer" class="style2 c1"><br /> <em>Adobe Photoshop, Illustrator, InDesign, Dreamweaver, and Flash logos<br /> are trademarks of Adobe Systems Incorporated.<br /> <br /> Copyright © 2009 Liz Kula. All rights reserved.</em> <div id="foot-nav"> <ul> <li><a href="http://validator.w3.org/check?uri=http://webdesignsbyliz.com/resume.html" target="_blank"><img src="http://www.w3.org/Icons/valid-xhtml10-blue" alt="Valid XHTML 1.0 Transitional" height="31" width="88" /></a></li> <li><a href="http://jigsaw.w3.org/css-validator/validator?uri=http://webdesignsbyliz.com/global.css" target="_blank"><img class="c2" src="http://jigsaw.w3.org/css-validator/images/vcss-blue" alt="Valid CSS!" /></a></li> </ul> </div> </div> </body> </html>
Thanks guys!
-
Here is what I have for that page with the error:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Web Designs By Liz - Testimonials</title> <style type="text/css" media="screen"> /*<![CDATA[*/ @import url(global.css); /*]]>*/ </style> <script type="text/javascript"> //<![CDATA[ <!-- function MM_preloadImages() { //v3.0 var d=document; if(d.images){ if(!d.MM_p) d.MM_p=new Array(); var i,j=d.MM_p.length,a=MM_preloadImages.arguments; for(i=0; i<a.length; i++) if (a[i].indexOf("#")!=0){ d.MM_p[j]=new Image; d.MM_p[j++].src=a[i];}} } function MM_findObj(n, d) { //v4.01 var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) { d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);} if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n]; for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document); if(!x && d.getElementById) x=d.getElementById(n); return x; } function MM_nbGroup(event, grpName) { //v6.0 var i,img,nbArr,args=MM_nbGroup.arguments; if (event == "init" && args.length > 2) { if ((img = MM_findObj(args[2])) != null && !img.MM_init) { img.MM_init = true; img.MM_up = args[3]; img.MM_dn = img.src; if ((nbArr = document[grpName]) == null) nbArr = document[grpName] = new Array(); nbArr[nbArr.length] = img; for (i=4; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = args[i+1]; nbArr[nbArr.length] = img; } } } else if (event == "over") { document.MM_nbOver = nbArr = new Array(); for (i=1; i < args.length-1; i+=3) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = (img.MM_dn && args[i+2]) ? args[i+2] : ((args[i+1])? args[i+1] : img.MM_up); nbArr[nbArr.length] = img; } } else if (event == "out" ) { for (i=0; i < document.MM_nbOver.length; i++) { img = document.MM_nbOver[i]; img.src = (img.MM_dn) ? img.MM_dn : img.MM_up; } } else if (event == "down") { nbArr = document[grpName]; if (nbArr) for (i=0; i < nbArr.length; i++) { img=nbArr[i]; img.src = img.MM_up; img.MM_dn = 0; } document[grpName] = nbArr = new Array(); for (i=2; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = (args[i+1])? args[i+1] : img.MM_up; nbArr[nbArr.length] = img; } } } //--> //]]> </script> <script type="text/javascript" language="JavaScript"> //<![CDATA[ <!-- function HideContent(d) { document.getElementById(d).style.display = "none"; } function ShowContent(d) { document.getElementById(d).style.display = "block"; } function ReverseDisplay(d) { if(document.getElementById(d).style.display == "none") { document.getElementById(d).style.display = "block"; } else { document.getElementById(d).style.display = "none"; } } //--> //]]> </script> <!-- Validation Script --> <script type="text/javascript"> function validateFormOnSubmit(theForm) { var reason = ""; reason += validateUsername(theForm.comment_owner); reason += validateEmail(theForm.comment_owner_email); reason += validatePhone(theForm.url); reason += validateEmpty(theForm.comment); if (reason != "") { alert("Some fields need correction:\n" + reason); return false; } } function validateUsername(fld) { var error = ""; var illegalChars = /\W/; // allow letters, numbers, and underscores if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your name.\n"; } return error; } function trim(s) { return s.replace(/^\s+|\s+$/, ''); } function validateEmail(fld) { var error=""; var tfld = trim(fld.value); // value of field with whitespace trimmed off var emailFilter = /^[^@]+@[^@.]+\.[^@]*\w\w$/ ; var illegalChars= /[\(\)\<\>\,\;\:\\\"\[\]]/ ; if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your e-mail address.\n"; } else if (!emailFilter.test(tfld)) { //test email for illegal characters fld.style.background = 'Yellow'; error = "Please enter a valid e-mail address.\n"; } else if (fld.value.match(illegalChars)) { fld.style.background = 'Yellow'; error = "Your e-mail address contains illegal characters.\n"; } else { fld.style.background = 'White'; } return error; } function validatePhone(fld) { var error = ""; var illegalChars = /\W/; // allow letters, numbers, and underscores var urlfld = trim(fld.value); // value of field with whitespace trimmed off var urlFilter = /http:\/\/[A-Za-z0-9\.-]{3,}\.[A-Za-z]{3}/; if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your web address.\n"; } else if (!urlFilter.test(urlfld)) { //test url for illegal characters fld.style.background = 'Yellow'; error = "Please enter a valid web address.\n"; } return error; } function validateEmpty(fld) { var error = ""; if (fld.value.length == 0) { fld.style.background = 'Yellow'; error = "Please enter your comment.\n" } else { fld.style.background = 'White'; } return error; } </script> <!--End Validation Script --> </head> <body onload="MM_preloadImages('home_selected.png','home_hover.png','resume.png','resume_hover.png','resume_selected.png','contact_selected.png','contact_hover.png','portfolio_selected.png','portfolio_hover.png')"> <script language="JavaScript" type="text/javascript"> //<![CDATA[ function doClock() { window.setTimeout( "doClock()", 1000 ); today = new Date(); self.status = today.toString(); } doClock() //]]> </script> <div class="c1" id="logo"><img src="weblogo.png" title="Web Designs By Liz Logo" alt="Web Designs By Liz Logo" /></div> <table align="center" border="0" cellpadding="0" cellspacing="0"> <tr> <td><a href="index.html" target="_top" onclick="MM_nbGroup('down','group1','home','home_selected.png',1)" onmouseover="MM_nbGroup('over','home','home_hover.png','home_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="home.png" alt="Home Page" name="home" border="0" id="home" /></a></td> <td><a href="resume.html" target="_top" onclick="MM_nbGroup('down','group1','resume','resume_selected.png',1)" onmouseover="MM_nbGroup('over','resume','resume_hover.png','resume_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="resume.png" alt="Resume" name="resume" border="0" id="resume" /></a></td> <td><a href="contact.html" target="_top" onclick="MM_nbGroup('down','group1','contact','contact_selected.png',1)" onmouseover="MM_nbGroup('over','contact','contact_hover.png','contact_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="contact.png" alt="Contact Page" name="contact" border="0" id="contact" /></a></td> <td><a href="portfolio.php" target="_top" onclick="MM_nbGroup('down','group1','portfolio','portfolio_selected.png',1)" onmouseover="MM_nbGroup('over','portfolio','portfolio_hover.png','portfolio_selected.png',1)" onmouseout="MM_nbGroup('out')"><img name="portfolio" src="portfolio.png" border="0" alt="Portfolio" /></a></td> <td><a href="testimonials.php" target="_top" onclick="MM_nbGroup('down','group1','testimonials','testimonials_selected.png',1)" onmouseover="MM_nbGroup('over','testimonials','testimonials_hover.png','testimonials_selected.png',1)" onmouseout="MM_nbGroup('out')"><img name="testimonials" src="testimonials_selected.png" border="0" alt="Testimonials" /></a></td> </tr> </table> <div id="resume_title" class="body"><div class="c1"></div> <table width="900" align="center"> <tr> <td class="style2" align="center"> <h1>Give Your Testimonial</h1> </td> </tr> <tr> <td class="style2"> <table class="style2" align="center"> <tr> <td> <?php //connect to server and select database $conn = mysql_connect("localhost", "webdes17_twilite", "minimoon") or die(mysql_error()); $db = mysql_select_db("webdes17_testimonials", $conn) or die(mysql_error()); if (isset($_POST['submit'])) { //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $url=mysql_real_escape_string($_POST['url']); $comment=mysql_real_escape_string($_POST['comment']); $error='';//initialize $error to blank if(trim($_POST['comment_owner'])=='' ){ $error.="Please enter your name!<br />"; //concatenate the $error Message with a line break } if(trim($_POST['url'])==''){ $error.="Please enter your web address!<br />";//concatenate more to $error } else { if(!preg_match('|^http(s)?://[a-z0-9-]+(.[a-z0-9-]+)*(:[0-9]+)?(/.*)?$|i', $_POST['url'])) { $error.="The URL you entered was not valid!<br />"; } } if(trim($_POST['comment_owner_email'])==''){ $error.="Plese enter your e-mail address!<br />"; } else { if(!preg_match("/^[_\.0-9a-zA-Z-]+@([0-9a-zA-Z][0-9a-zA-Z-]+\.)+[a-zA-Z]{2,6}$/i", $_POST['comment_owner_email'])) { $error.="The e-mail you entered was not valid!<br />"; } } if(trim($_POST['comment'])=='' ){ $error.="Please enter your comment!<br />"; //concatenate the $error Message with a line break } if($error==''){ header( 'Location: testimonials.php' ) ; $sql="INSERT INTO user_comments (comment_id, comment, comment_create_time, comment_owner, comment_owner_email, url) VALUES ('', '$comment', now(), '$name','$email', '$url')"; mysql_query($sql,$conn) or die(mysql_error()); } else{ echo "<span style=color:red>$error</span><br />"; } } else { $name= ''; $email= ''; $url= ''; $comment= ''; } ?> <form name="comment" id="comment" onsubmit="return validateFormOnSubmit(this)" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="name"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" value="<?php echo $name; ?>"/></div> </td> </tr> <tr> <td align="left"><label for="email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" value="<?php echo $email; ?>"/></div> </td> </tr> <tr> <td align="left"><label for="url">URL:</label></td> <td> <div class="c2"><input type="text" name="url" id="url" size="30" value="<?php echo $url; ?>"/></div> </td> </tr> <tr> <td align="left"> <label for="comment">Comments:</label> </td> <td> <textarea name="comment" id="comment" rows="5" cols="30" value="<?php echo $comment; ?>"> </textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div> </td> <td width="2"></td> </tr> </table> </form> </td> </tr> </table> </td> </tr> </table> </div> <div id="footer" class="style2 c1"><br /> <em>Adobe Photoshop, Illustrator, InDesign, Dreamweaver, and Flash logos<br /> are trademarks of Adobe Systems Incorporated.<br /> <br /> Copyright © 2009 Liz Kula. All rights reserved.</em> <div id="foot-nav"> <ul> <li><a href="http://validator.w3.org/check?uri=http://webdesignsbyliz.com/resume.html" target="_blank"><img src="http://www.w3.org/Icons/valid-xhtml10-blue" alt="Valid XHTML 1.0 Transitional" height="31" width="88" /></a></li> <li><a href="http://jigsaw.w3.org/css-validator/validator?uri=http://webdesignsbyliz.com/global.css" target="_blank"><img class="c2" src="http://jigsaw.w3.org/css-validator/images/vcss-blue" alt="Valid CSS!" /></a></li> </ul> </div> </div> </body> </html>
-
:-( When I tried to upload this code to my server, I got this error when the form is submitted:
Warning: Cannot modify header information - headers already sent by (output started at testimonials_form.php:7) in testimonials_form.php on line 237
Also, when this error occurs, all the fields are pre-filled in except for my comment. I think that might be a problem for someone if they got an error and they had written a lot in the comment field. Can you guys help?
-
Well, I figured out the part about the insert statement. I had to add it to the if part where it redirects.
if($error==''){ header( 'Location: testimonials.php' ) ; $sql="INSERT INTO user_comments (comment_id, comment, comment_create_time, comment_owner, comment_owner_email, url) VALUES ('', '$comment', now(), '$name','$email', '$url')"; mysql_query($sql,$conn) or die(mysql_error()); } else{ echo "<span style=color:red>$error</span><br />"; }
Now I just need to try adding in my JavaScript and test it. Thanks for all the help guys!
-
Okay, I need a little bit of help still. I have everything on one page now, except for the JavaScript, but I will add it in later, after I get the PHP working properly. Okay, so I have it set up like this:
<?php //connect to server and select database $conn = mysql_connect("localhost", "root", "") or die(mysql_error()); $db = mysql_select_db("smrpg", $conn) or die(mysql_error()); // if the form is submitted which means that this is not the first time visit for this user, // the user did put all values and hit the submit button and inside this if statement we do what we //want to happen in the second page in your code if (isset($_POST['submit'])) { //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $url=mysql_real_escape_string($_POST['url']); $comment=mysql_real_escape_string($_POST['comment']); $error='';//initialize $error to blank if(trim($_POST['comment_owner'])=='' ){ $error.="Please enter your name!<br />"; //concatenate the $error Message with a line break } if(trim($_POST['url'])==''){ $error.="Please enter your web address!<br />";//concatenate more to $error } else { if(!preg_match('|^http(s)?://[a-z0-9-]+(.[a-z0-9-]+)*(:[0-9]+)?(/.*)?$|i', $_POST['url'])) { $error.="The URL you entered was not valid!<br />"; } } if(trim($_POST['comment_owner_email'])==''){ $error.="Plese enter your e-mail address!<br />"; } else { if(!preg_match("/^[_\.0-9a-zA-Z-]+@([0-9a-zA-Z][0-9a-zA-Z-]+\.)+[a-zA-Z]{2,6}$/i", $_POST['comment_owner_email'])) { $error.="The e-mail you entered was not valid!<br />"; } } if(trim($_POST['comment'])=='' ){ $error.="Please enter your comment!<br />"; //concatenate the $error Message with a line break } if($error==''){ header( 'Location: testimonials.php' ) ; } else{ echo "<span style=color:red>$error</span><br /> <p>Please press the back button and fill in the form properly and submit again.</p>"; } $sql="INSERT INTO user_comments (comment_id, comment, comment_create_time, comment_owner, comment_owner_email, url) VALUES ('', '$comment', now(), '$name','$email', '$url')"; mysql_query($sql,$conn) or die(mysql_error()); // grab the data from the $_POST array and put it in a variables // $email = $_POST['email']; // validate the data and make sure they are all correct or have a value or whatever // send the data to the database and check if its entered successfully // don't forget the error handling here, you might wanna see if the data was entered or not // do NOT print any thing before redirecting otherwise it won't work, so you collect the errors here and display them right before the form if there is any errors // NOW redirect } else { // this means the form is NOT submitted and the user is visiting this page for the // first time (not a submitted page because $_POST['submit'] doesn't have a value //display the form right here, you might want to cut off the php tags here and start html but // don't forget the last curly brace of else and also don't forget to initialize variables to fill // the form with them because you don't want the user to submit all the data again if he // had just one error //so do like this in the php $name= ''; $email= ''; $url= ''; $comment= ''; } ?> <form name="comment" id="comment" onsubmit="return validateFormOnSubmit(this)" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="name"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" value="<?php echo $name; ?>"/></div> </td> </tr> <tr> <td align="left"><label for="email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" value="<?php echo $email; ?>"/></div> </td> </tr> <tr> <td align="left"><label for="url">URL:</label></td> <td> <div class="c2"><input type="text" name="url" id="url" size="30" value="<?php echo $url; ?>"/></div> </td> </tr> <tr> <td align="left"> <label for="comment">Comments:</label> </td> <td> <textarea name="comment" id="comment" rows="5" cols="30" value="<?php echo $comment; ?>"> </textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div> </td> <td width="2"></td> </tr> </table> </form>
But whenever I fill in one of the fields incorrectly and submit to check if my validation is working, it doesn't redirect (like it's supposed to), and the errors are showing (like they are supposed to), but each time submit is pressed, it is still inserting the data, even without the fields being properly filled in. Where is my insert statement supposed to go? I thought it went inside the first if statement, like if all validation passes, insert data, if not, show form with posted data pre-filled. Also, that pre-filled part is working also, so that's good. :-) Can you help me with where the insert statement is supposed to go, please? Also, I think I need to modify the if/else statement that says "Press back button on your browser, etc" because I don't need it to say anything, sinec the error messages display if there is an error present, but how do I write that if statement to where it displays the errors if there are any but redirects if there aren't any? I think I've got it a little mixed up.
-
I will try your example and I will probably add the JavaScript that I have working and see how that goes because I want to accommodate both client-side as well as server-side validation, just in case JavaScript is disable, as you pointed out. I will be back if I get stuck! LOL
-
Well, I'm still learning PHP, so I don't know how to do everything yet. LOL But the way I learned to insert information from a form into a table is to have the form on one page, then when submitted it goes to the next page that inserts it and display a message. Then I redirect from there because I want it to go back to the viewing page. I'd like to do all the validation one page, but I don't know how exactly. So, my JavaScript works the way it is now, but when I had the action pointing to the insert page, even when the JavaScript gives an error, it would continue to the insert page. So maybe someone can help me out here. I have the form with the JavaScript:
<!-- Validation Script --> <script type="text/javascript"> function validateFormOnSubmit(theForm) { var reason = ""; reason += validateUsername(theForm.comment_owner); reason += validateEmail(theForm.comment_owner_email); reason += validatePhone(theForm.url); reason += validateEmpty(theForm.comment); if (reason != "") { alert("Some fields need correction:\n" + reason); return false; } //redirect window.location = "validation.php" return false; } function validateUsername(fld) { var error = ""; var illegalChars = /\W/; // allow letters, numbers, and underscores if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your name.\n"; } return error; } function trim(s) { return s.replace(/^\s+|\s+$/, ''); } function validateEmail(fld) { var error=""; var tfld = trim(fld.value); // value of field with whitespace trimmed off var emailFilter = /^[^@]+@[^@.]+\.[^@]*\w\w$/ ; var illegalChars= /[\(\)\<\>\,\;\:\\\"\[\]]/ ; if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your e-mail address.\n"; } else if (!emailFilter.test(tfld)) { //test email for illegal characters fld.style.background = 'Yellow'; error = "Please enter a valid e-mail address.\n"; } else if (fld.value.match(illegalChars)) { fld.style.background = 'Yellow'; error = "Your e-mail address contains illegal characters.\n"; } else { fld.style.background = 'White'; } return error; } function validatePhone(fld) { var error = ""; var illegalChars = /\W/; // allow letters, numbers, and underscores var urlfld = trim(fld.value); // value of field with whitespace trimmed off var urlFilter = /http:\/\/[A-Za-z0-9\.-]{3,}\.[A-Za-z]{3}/; if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your web address.\n"; } else if (!urlFilter.test(urlfld)) { //test url for illegal characters fld.style.background = 'Yellow'; error = "Please enter a valid web address.\n"; } return error; } function validateEmpty(fld) { var error = ""; if (fld.value.length == 0) { fld.style.background = 'Yellow'; error = "Please enter your comment.\n" } else { fld.style.background = 'White'; } return error; } </script> <!--End Validation Script --> <form name="comment" id="comment" onsubmit="return validateFormOnSubmit(this)" action="" method="post"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="comment_owner"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" /></div> </td> </tr> <tr> <td align="left"><label for="comment_owner_email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" /></div> </td> </tr> <tr> <td align="left"><label for="url">URL:</label></td> <td> <div class="c2"><input type="text" name="url" id="url" size="30" /></div> </td> </tr> <tr> <td align="left"> <label for="comment">Comments:</label> </td> <td> <textarea name="comment" id="comment" rows="5" cols="30"> </textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div> </td> <td width="2"></td> </tr> </table> </form>
But how can I put my PHP validation and insert statement and message all on the same page? Here is my PHP validation:
<?php if(isset($_POST['submit'])){ $error='';//initialize $error to blank if(trim($_POST['comment_owner'])=='' ){ $error.="Please enter your name!<br />"; //concatenate the $error Message with a line break } if(trim($_POST['url'])==''){ $error.="Please enter your web address!<br />";//concatenate more to $error } else { if(!preg_match('|^http(s)?://[a-z0-9-]+(.[a-z0-9-]+)*(:[0-9]+)?(/.*)?$|i', $_POST['url'])) { $error.="The URL you entered was not valid!<br />"; } } if(trim($_POST['comment_owner_email'])==''){ $error.="Plese enter your e-mail address!<br />"; } else { if(!preg_match("/^[_\.0-9a-zA-Z-]+@([0-9a-zA-Z][0-9a-zA-Z-]+\.)+[a-zA-Z]{2,6}$/i", $_POST['comment_owner_email'])) { $error.="The e-mail you entered was not valid!<br />"; } } if(trim($_POST['comment'])=='' ){ $error.="Please enter your comment!<br />"; //concatenate the $error Message with a line break } if($error=='') header('location:yourpage.php'); } else{ echo "<span style=color:red>$error</span><br /> <p>Please press the back button and fill in the form properly and submit again.</p>"; } } ?>
And here is my insert page:
<?php //connect to server and select database $conn = mysql_connect("localhost", "webdes17_twilite", "minimoon") or die(mysql_error()); $db = mysql_select_db("webdes17_testimonials", $conn) or die(mysql_error()); //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $url=mysql_real_escape_string($_POST['url']); $comment=mysql_real_escape_string($_POST['comment']); $sql="INSERT INTO user_comments (comment_id, comment, comment_create_time, comment_owner, comment_owner_email, url) VALUES ('', '$comment', now(), '$name','$email', '$url')"; mysql_query($sql,$conn) or die(mysql_error()); //create nice message for user $msg = "<p>Your comment has been added. Thank you for your testimonial! You will be redirected to the Testimonials page in a moment. If you aren't forwarded to the new page, please click <a href=\"http://www.webdesignsbyliz.com/testimonials.php\"> here</a>. </p>"; ?>
Right now, these are three separate pages. How can I put the PHP validation and insert statement on the same page? Would I use an if statement? How would I write it? Can anyone help? Or are you saying that ALL teh validation can go on one page, the same page as the form? Can someone please help me?
-
I don't understand what you mean by line 4. It doesn't seem to make sense to put it on line 4. Can you show me where you mean please about the _$POST['submit']='submit';? Actually LOL Neither part looked right to me. Because I ready had if ($error=='') with the redirect:
if($error==''){ header('location:yourpage.php'); }
And yes, I have a page named "yourpage.php" for testing purposes. If it redirects, I will change it to the page that inserts the form data. But anyway, can you please show me what you mean and where you made those changes you mentioned because I'm having a hard time understanding what you meant. Thanks!
-
I have a form that validates with JavaScript, which then redirects to my page that validates with PHP, just in case JavaScript is disabled. I'm having trouble getting it to redirect if there are no PHP or JavaScript errors to my page that inserts it into my table and displays my success message. Can anyone help get my page to redirect properly? Here is my form:
<?php session_start(); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Web Designs By Liz - Give Your Testimonial</title> <style type="text/css" media="screen"> /*<![CDATA[*/ @import url(global.css); /*]]>*/ </style> <script type="text/javascript"> //<![CDATA[ <!-- function MM_preloadImages() { //v3.0 var d=document; if(d.images){ if(!d.MM_p) d.MM_p=new Array(); var i,j=d.MM_p.length,a=MM_preloadImages.arguments; for(i=0; i<a.length; i++) if (a[i].indexOf("#")!=0){ d.MM_p[j]=new Image; d.MM_p[j++].src=a[i];}} } function MM_findObj(n, d) { //v4.01 var p,i,x; if(!d) d=document; if((p=n.indexOf("?"))>0&&parent.frames.length) { d=parent.frames[n.substring(p+1)].document; n=n.substring(0,p);} if(!(x=d[n])&&d.all) x=d.all[n]; for (i=0;!x&&i<d.forms.length;i++) x=d.forms[i][n]; for(i=0;!x&&d.layers&&i<d.layers.length;i++) x=MM_findObj(n,d.layers[i].document); if(!x && d.getElementById) x=d.getElementById(n); return x; } function MM_nbGroup(event, grpName) { //v6.0 var i,img,nbArr,args=MM_nbGroup.arguments; if (event == "init" && args.length > 2) { if ((img = MM_findObj(args[2])) != null && !img.MM_init) { img.MM_init = true; img.MM_up = args[3]; img.MM_dn = img.src; if ((nbArr = document[grpName]) == null) nbArr = document[grpName] = new Array(); nbArr[nbArr.length] = img; for (i=4; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = args[i+1]; nbArr[nbArr.length] = img; } } } else if (event == "over") { document.MM_nbOver = nbArr = new Array(); for (i=1; i < args.length-1; i+=3) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = (img.MM_dn && args[i+2]) ? args[i+2] : ((args[i+1])? args[i+1] : img.MM_up); nbArr[nbArr.length] = img; } } else if (event == "out" ) { for (i=0; i < document.MM_nbOver.length; i++) { img = document.MM_nbOver[i]; img.src = (img.MM_dn) ? img.MM_dn : img.MM_up; } } else if (event == "down") { nbArr = document[grpName]; if (nbArr) for (i=0; i < nbArr.length; i++) { img=nbArr[i]; img.src = img.MM_up; img.MM_dn = 0; } document[grpName] = nbArr = new Array(); for (i=2; i < args.length-1; i+=2) if ((img = MM_findObj(args[i])) != null) { if (!img.MM_up) img.MM_up = img.src; img.src = img.MM_dn = (args[i+1])? args[i+1] : img.MM_up; nbArr[nbArr.length] = img; } } } //--> //]]> </script> <script type="text/javascript" language="JavaScript"> //<![CDATA[ <!-- function HideContent(d) { document.getElementById(d).style.display = "none"; } function ShowContent(d) { document.getElementById(d).style.display = "block"; } function ReverseDisplay(d) { if(document.getElementById(d).style.display == "none") { document.getElementById(d).style.display = "block"; } else { document.getElementById(d).style.display = "none"; } } //--> //]]> </script> <!-- Validation Script --> <script type="text/javascript"> function validate_required(field,alerttxt) { with (field) { if (value==null||value=="") { alert(alerttxt);return false; } else { return true; } } } function validate_form(thisform) { with (thisform) { if (validate_required(comment_owner_email,"E-mail address must be filled out!")==false) {email.focus();return false;} } } </script> <!--End Validation Script --> </head> <body onload="MM_preloadImages('home_selected.png','home_hover.png','resume.png','resume_hover.png','resume_selected.png','contact_selected.png','contact_hover.png','portfolio_selected.png','portfolio_hover.png','testimonials.png','testimonials_hover.png','testimonials_selected.png')"> <script language="JavaScript" type="text/javascript"> //<![CDATA[ function doClock() { window.setTimeout( "doClock()", 1000 ); today = new Date(); self.status = today.toString(); } doClock() //]]> </script> <!-- Validation Script --> <script type="text/javascript"> function validateFormOnSubmit(theForm) { var reason = ""; reason += validateUsername(theForm.comment_owner); reason += validateEmail(theForm.comment_owner_email); reason += validatePhone(theForm.url); reason += validateEmpty(theForm.comment); if (reason != "") { alert("Some fields need correction:\n" + reason); return false; } //redirect window.location = "validation.php" return false; } function validateUsername(fld) { var error = ""; var illegalChars = /\W/; // allow letters, numbers, and underscores if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your name.\n"; } return error; } function trim(s) { return s.replace(/^\s+|\s+$/, ''); } function validateEmail(fld) { var error=""; var tfld = trim(fld.value); // value of field with whitespace trimmed off var emailFilter = /^[^@]+@[^@.]+\.[^@]*\w\w$/ ; var illegalChars= /[\(\)\<\>\,\;\:\\\"\[\]]/ ; if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your e-mail address.\n"; } else if (!emailFilter.test(tfld)) { //test email for illegal characters fld.style.background = 'Yellow'; error = "Please enter a valid e-mail address.\n"; } else if (fld.value.match(illegalChars)) { fld.style.background = 'Yellow'; error = "Your e-mail address contains illegal characters.\n"; } else { fld.style.background = 'White'; } return error; } function validatePhone(fld) { var error = ""; var illegalChars = /\W/; // allow letters, numbers, and underscores var urlfld = trim(fld.value); // value of field with whitespace trimmed off var urlFilter = /http:\/\/[A-Za-z0-9\.-]{3,}\.[A-Za-z]{3}/; if (fld.value == "") { fld.style.background = 'Yellow'; error = "Please enter your web address.\n"; } else if (!urlFilter.test(urlfld)) { //test url for illegal characters fld.style.background = 'Yellow'; error = "Please enter a valid web address.\n"; } return error; } function validateEmpty(fld) { var error = ""; if (fld.value.length == 0) { fld.style.background = 'Yellow'; error = "Please enter your comment.\n" } else { fld.style.background = 'White'; } return error; } </script> <!--End Validation Script --> <div class="c1" id="logo"><img src="weblogo.png" title="Web Designs By Liz Logo" alt="Web Designs By Liz Logo" /></div> <table align="center" border="0" cellpadding="0" cellspacing="0"> <tr> <td><a href="index.html" target="_top" onclick="MM_nbGroup('down','group1','home','home_selected.png',1)" onmouseover="MM_nbGroup('over','home','home_hover.png','home_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="home.png" alt="Home Page" name="home" border="0" id="home" /></a></td> <td><a href="resume.html" target="_top" onclick="MM_nbGroup('down','group1','resume','resume_selected.png',1)" onmouseover="MM_nbGroup('over','resume','resume_hover.png','resume_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="resume.png" alt="Resume" name="resume" border="0" id="resume" /></a></td> <td><a href="contact.html" target="_top" onclick="MM_nbGroup('down','group1','contact','contact_selected.png',1)" onmouseover="MM_nbGroup('over','contact','contact_hover.png','contact_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="contact.png" alt="Contact Page" name="contact" border="0" id="contact" /></a></td> <td><a href="portfolio.php" target="_top" onclick="MM_nbGroup('down','group1','portfolio','portfolio_selected.png',1)" onmouseover="MM_nbGroup('over','portfolio','portfolio_hover.png','portfolio_selected.png',1)" onmouseout="MM_nbGroup('out')"><img name="portfolio" src="portfolio.png" border="0" alt="Portfolio" /></a></td> <td><a href="testimonials.php" target="_top" onClick="MM_nbGroup('down','group1','testimonials','testimonials_selected.png',1)" onMouseOver="MM_nbGroup('over','testimonials','testimonials_hover.png','testimonials_selected.png',1)" onMouseOut="MM_nbGroup('out')"><img name="testimonials" src="testimonials_selected.png" border="0" alt="Testimonials" onLoad="MM_nbGroup('init','group1','testimonials','testimonials.png',1)" /></a></td> </tr> </table> <div id="resume_title" class="body"><div class="c1"></div> <table width="900" align="center"> <tr> <td class="style2" align="center"> <h1>Give Your Testimonial</h1> </td> </tr> <tr> <td class="style2"> <table class="style2" align="center"> <tr> <td> <form name="comment" id="comment" onsubmit="return validateFormOnSubmit(this)" action="" method="post"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="comment_owner"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" /></div> </td> </tr> <tr> <td align="left"><label for="comment_owner_email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" /></div> </td> </tr> <tr> <td align="left"><label for="url">URL:</label></td> <td> <div class="c2"><input type="text" name="url" id="url" size="30" /></div> </td> </tr> <tr> <td align="left"> <label for="comment">Comments:</label> </td> <td> <textarea name="comment" id="comment" rows="5" cols="30"> </textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div> </td> <td width="2"></td> </tr> </table> </form> </td> </tr> </table> </td> </tr> </table> </div> <div id="footer" class="style2 c1"><br /> <em>Adobe Photoshop, Illustrator, InDesign, Dreamweaver, and Flash logos<br /> are trademarks of Adobe Systems Incorporated.<br /> <br /> Copyright © 2009 Liz Kula. All rights reserved.</em> <div id="foot-nav"> <ul> <li><a href="http://validator.w3.org/check?uri=http://webdesignsbyliz.com/resume.html" target="_blank"><img src="http://www.w3.org/Icons/valid-xhtml10-blue" alt="Valid XHTML 1.0 Transitional" height="31" width="88" /></a></li> <li><a href="http://jigsaw.w3.org/css-validator/validator?uri=http://webdesignsbyliz.com/global.css" target="_blank"><img class="c2" src="http://jigsaw.w3.org/css-validator/images/vcss-blue" alt="Valid CSS!" /></a></li> </ul> </div> </div> </body> </html>
Here is my PHP validation page:
<style type="text/css" media="screen"> /*<![CDATA[*/ @import url(global.css); /*]]>*/ </style> <body onload="MM_preloadImages('home_selected.png','home_hover.png','resume.png','resume_hover.png','resume_selected.png','contact_selected.png','contact_hover.png','portfolio_selected.png','portfolio_hover.png','testimonials.png','testimonials_hover.png','testimonials_selected.png')"> <script language="JavaScript" type="text/javascript"> //<![CDATA[ function doClock() { window.setTimeout( "doClock()", 1000 ); today = new Date(); self.status = today.toString(); } doClock() //]]> </script> <div class="c1" id="logo"><img src="weblogo.png" title="Web Designs By Liz Logo" alt="Web Designs By Liz Logo" /></div> <table align="center" border="0" cellpadding="0" cellspacing="0"> <tr> <td><a href="index.html" target="_top" onclick="MM_nbGroup('down','group1','home','home_selected.png',1)" onmouseover="MM_nbGroup('over','home','home_hover.png','home_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="home.png" alt="Home Page" name="home" border="0" id="home" /></a></td> <td><a href="resume.html" target="_top" onclick="MM_nbGroup('down','group1','resume','resume_selected.png',1)" onmouseover="MM_nbGroup('over','resume','resume_hover.png','resume_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="resume.png" alt="Resume" name="resume" border="0" id="resume" /></a></td> <td><a href="contact.html" target="_top" onclick="MM_nbGroup('down','group1','contact','contact_selected.png',1)" onmouseover="MM_nbGroup('over','contact','contact_hover.png','contact_selected.png',1)" onmouseout="MM_nbGroup('out')"><img src="contact.png" alt="Contact Page" name="contact" border="0" id="contact" /></a></td> <td><a href="portfolio.php" target="_top" onclick="MM_nbGroup('down','group1','portfolio','portfolio_selected.png',1)" onmouseover="MM_nbGroup('over','portfolio','portfolio_hover.png','portfolio_selected.png',1)" onmouseout="MM_nbGroup('out')"><img name="portfolio" src="portfolio.png" border="0" alt="Portfolio" /></a></td> <td><a href="testimonials.php" target="_top" onClick="MM_nbGroup('down','group1','testimonials','testimonials_selected.png',1)" onMouseOver="MM_nbGroup('over','testimonials','testimonials_hover.png','testimonials_selected.png',1)" onMouseOut="MM_nbGroup('out')"><img name="testimonials" src="testimonials_selected.png" border="0" alt="Testimonials" onLoad="MM_nbGroup('init','group1','testimonials','testimonials.png',1)" /></a></td> </tr> </table> <div id="resume_title" class="body"><div class="c1"></div> <table width="900" align="center"> <tr> <td class="style2" align="center"> <h1>Give Your Testimonial</h1> <?php /* this is guarunteed to work it is possible to use <? (short tags but this style works everywhere).*/ /*Only verify/validate form when it is submitted program name: form.php */ if(isset($_POST['submit'])){ $error='';//initialize $error to blank if(trim($_POST['comment_owner'])=='' ){ $error.="Please enter your name!<br />"; //concatenate the $error Message with a line break } if(trim($_POST['url'])==''){ $error.="Please enter your web address!<br />";//concatenate more to $error } else { if(!preg_match('|^http(s)?://[a-z0-9-]+(.[a-z0-9-]+)*(:[0-9]+)?(/.*)?$|i', $_POST['url'])) { $error.="The URL you entered was not valid!<br />"; } } if(trim($_POST['comment_owner_email'])==''){ $error.="Plese enter your e-mail address!<br />"; } else { if(!preg_match("/^[_\.0-9a-zA-Z-]+@([0-9a-zA-Z][0-9a-zA-Z-]+\.)+[a-zA-Z]{2,6}$/i", $_POST['comment_owner_email'])) { $error.="The e-mail you entered was not valid!<br />"; } } if(trim($_POST['comment'])=='' ){ $error.="Please enter your comment!<br />"; //concatenate the $error Message with a line break } if($error==''){//Hmmmm no text is in $error so do something else, the page has verified and the email was valid // so uncomment the line below to send the user to your own success page or wherever (swap yourpage.php with your files location). header('location:yourpage.php'); } else{ echo "<span style=color:red>$error</span><br /> <p>Please press the back button and fill in the form properly and submit again.</p>"; } } ?> </td> </tr> </table> </div> <div id="footer" class="style2 c1"><br /> <em>Adobe Photoshop, Illustrator, InDesign, Dreamweaver, and Flash logos<br /> are trademarks of Adobe Systems Incorporated.<br /> <br /> Copyright © 2009 Liz Kula. All rights reserved.</em> <div id="foot-nav"> <ul> <li><a href="http://validator.w3.org/check?uri=http://webdesignsbyliz.com/resume.html" target="_blank"><img src="http://www.w3.org/Icons/valid-xhtml10-blue" alt="Valid XHTML 1.0 Transitional" height="31" width="88" /></a></li> <li><a href="http://jigsaw.w3.org/css-validator/validator?uri=http://webdesignsbyliz.com/global.css" target="_blank"><img class="c2" src="http://jigsaw.w3.org/css-validator/images/vcss-blue" alt="Valid CSS!" /></a></li> </ul> </div> </div>
Here is the page that inserts my form results:
<?php //connect to server and select database $conn = mysql_connect("localhost", "root", "") or die(mysql_error()); $db = mysql_select_db("webdes17_testimonials", $conn) or die(mysql_error()); //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $url=mysql_real_escape_string($_POST['url']); $comment=mysql_real_escape_string($_POST['comment']); $sql="INSERT INTO user_comments (comment_id, comment, comment_create_time, comment_owner, comment_owner_email, url) VALUES ('', '$comment', now(), '$name','$email', '$url')"; mysql_query($sql,$conn) or die(mysql_error()); //create nice message for user $msg = "<p>Your comment has been added. Thank you for your testimonial! You will be redirected to the Testimonials page in a moment. If you aren't forwarded to the new page, please click <a href=\"http://www.webdesignsbyliz.com/testimonials.php\"> here</a>. </p>"; ?>
Can anyone help me out? I just need the second page to redirect to the third page if the form has no PHP errors. What am I doing wrong? I also have the third page redirecting to another page automatically after 5 seconds, so I really need the second page to redirect to the third page. can anyone help?
-
Thanks, though. The absolute position seems to be doing the trick. I knew it was something simple. Thanks so much!!!
-
LOL I'm not sure. I was just trying different values until it looked right, but it still looks wrong anyway. :-\
-
When I select the first option, this is how it looks:
Then the second option does this:
Then the third option does this:
So now I have the first and second div tags lining up after everything has been selected, but they are all messed up between selections. Can anyone help?
-
I'm having some trouble displaying my divs the way I want to. I'm not sure what codes I have wrong, or what I might need to add. Here is what I have for the three div tags I'm trying to get situated (right now I don't have anything set for the first div tag):
#txtHint { } #txtHint2 { margin-left: 790px; margin-top: -35px; clear:none; } #txtHint3 { margin-left: 550px; margin-top: -1px; clear: none; }
And here is the HTML page where it displays:
<?php $get_map = "select * from monsters1 group by map"; $get_map_res = mysql_query($get_map, $conn) or die(mysql_error()); echo "<select name=\"maps\" onchange=\"showMap(this.value)\"> <option selected=\"selected\">None Selected</option>"; while ($list_maps = mysql_fetch_array($get_map_res)) { $map_id = $list_maps['id']; $map = $list_maps['map']; echo "<option value=\"$map\">$map</option>"; } echo "</select>"; ?> <div id="txtHint2"></div> <br /> <div id="txtHint"></div> <div id="txtHint3"></div> </form> </div>
These div tags are being filled by a JS/PHP combination. I don't know if that makes a difference or not, but the PHP code that populates the three tags is below:
txtHint:
echo "<table border='0'>"; while($row = mysql_fetch_array($result)) { echo "<tr>"; echo "<td colspan=2><strong>" . $row['identity'] . "</strong></td></tr>"; echo "<tr><td align=right><i>Health</i></td><td>" . $row['health'] . "</td></tr>"; echo "<tr><td align=right><i>Energy</i></td><td>" . $row['energy'] . "</td></tr>"; echo "<tr><td align=right><i>Attack</i></td><td>" . $row['acv1'] . "</td></tr>"; echo "<tr><td align=right><i>Defense</i></td><td>" . $row['dcv1'] . "</td>"; echo "</tr>"; } echo "</table>";
txtHint2:
echo "<table border='0'>"; echo "Select a location: <select name=\"locations\" onchange=\"showMonsters(this.value)\"> <option selected=selected>None Selected</option>"; while($row = mysql_fetch_array($result)) { $location = $row['location']; echo "<option value=\"$location\">" . $row['location'] . "</option>"; } echo "</select>";
txtHint3:
echo "<table border='0'>"; echo "<tr><td>"; while($row = mysql_fetch_array($result)) { echo "<strong>" . $row['name'] . "</strong></td>"; } echo "</tr></table>";
And this is what is happening to my divs when the div contents are displayed:
I need to keep everything from moving as the user selects options, and currently when the user selects the first option, it places it, but then when the user selects each additional option from the other select lists, the contents move lower and lower. I want the first and third div tags to line up with each other, but they aren't. Wat can I do?
-
I figured out the problem. Some of my name values have apostrophes in them. How do I use the msql_real_escape_string with the statements that display the names? Here's where is displays the name:
$sql="SELECT * FROM monsters1 WHERE location = '".$q."' group by name"; $result = mysql_query($sql); echo "<table border='0'>"; echo "Select a monster: <select name=\"monsters\"> <option selected=selected>None Selected</option>"; while($row = mysql_fetch_array($result)) { echo "<option>" . $row['name'] . "</option>"; } echo "</select>";
Reply To Post ID Question
in PHP Coding Help
Posted
Here is the first form, that gets the comment along with generating the comment_id:
Here's the page that displays all the comments and replies, and you can see in the link for the reply that it sends the comment id:
And here is again the reply form, that should take the comment_id from the last page and send it along with this form data upon submit:
I haven't made any of the suggested modifications yet.