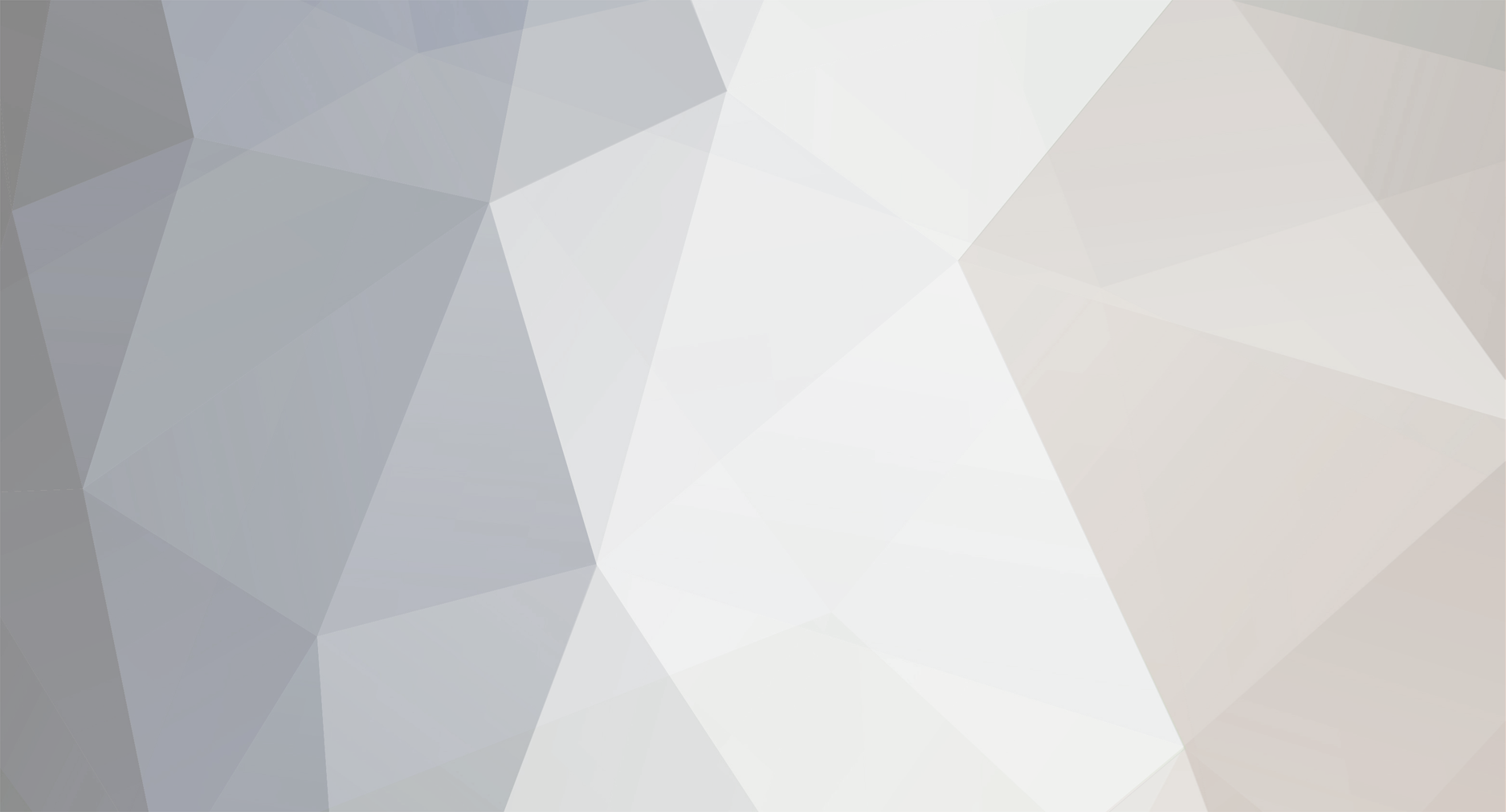
twilitegxa
-
Posts
1,020 -
Joined
-
Last visited
Posts posted by twilitegxa
-
-
I think I'm going to try it another way.
-
I have the following page:
<?php $conn = mysql_connect("localhost", "root", "") or die(mysql_error()); $db = mysql_select_db("smrpg", $conn) or die(mysql_error()); $location = $_GET['location']; $choice = $_GET['choice']; //ITEM SHOP if ($location == 'item_shop' && $choice == 'buy') { $get_items = "select * from shop_items where shop = 'Item'"; $get_items_res = mysql_query($get_items, $conn) or die(mysql_error()); echo "<h3>Item Shop</h3>"; echo "<form action=buy.php method=post><table border=0 cellspacing=3 cellpadding=3><th>Item</th><th>Price</th><th>Quantity</th>"; while ($show_item = mysql_fetch_array($get_items_res)) { $item = $show_item['item']; $price = $show_item['price']; echo "<tr><td>$item</td> <td align=center>$price S</td> <td><input type=text size=2 name=quantity></td> </tr>"; } echo "<tr><td colspan=3><input type=button value=Buy> <a href=location.php?location=item_shop><input type=button value=Back></a></td></tr></table></form>"; //WEAPON SHOP } else if ($location == 'weapon_shop' && $choice == 'buy') { $get_items = "select * from shop_items where shop = 'Weapon'"; $get_items_res = mysql_query($get_items, $conn) or die(mysql_error()); echo "<table border=0 cellspacing=3 cellpadding=3><th>Item</th><th>Price</th>"; while ($show_item = mysql_fetch_array($get_items_res)) { $item = $show_item['item']; $price = $show_item['price']; echo "<tr><td>$item</td><td align=center>$price S</td></tr>"; } echo "</table>"; } //get identity $get_identity = "select * from scouts where username = '".$_SESSION['userName']."' and active = '1'"; $get_identity_res = mysql_query($get_identity, $conn) or die(mysql_error()); while ($user_identity = mysql_fetch_array($get_identity_res)) { $identity = $user_identity['identity']; //gather gold $get_gold = "select * from gold where identity = '$identity'"; $get_gold_res = mysql_query($get_gold, $conn) or die(mysql_error()); while ($show_gold = mysql_fetch_array($get_gold_res)) { $gold = $show_gold['amount']; echo "<strong>Gold</strong>: $gold"; } } ?>
And what I would like to do is on my buy.php page, I want to display which items they chose to buy. If they didn't put a value in the input box or if they put a zero, then I want it to show nothing. But if they typed in a value, like 1 or 2, etc, then I want it to display the items they chose and the quantity, along with a total. Here's the basic idea:
<h1>Purchased Items</h1> Items you have purchased: <table> <th>Item</th> <th>Price</th> <th>Quantity</th> <th>Total</th> <tr> <td>Herb</td> <td>40 S</td> <td>5</td> <td>200 S</td> </tr> <tr> <td>Antidote</td> <td>20 S</td> <td>5</td> <td>100 S</td> </tr> <tr> <td colspan=3><strong>Total:</strong></td> <td><strong>300 S</strong></td> </tr> </table>
What I need to know is, how can I get the values of each item and quantity that the user wants to buy over to the buy.php page? Is it possible to do it the way I currently have the page? Or do I have to do it another way?
-
Thank you, I will try this out. Thanks for the suggestion.
-
Well, to answer your question, I'm using mostly PHP and MySQL to load all information onto my web pages. I'd love to use something like AJAX maybe in this particular instance probably, unless there's a better way, I just don't know how to code in AJAX very much yet. But your suggestion about storing all my PHP data into JavaScript variables, how could I go about doing that? I'm still new to JavaScript and am trying to learn more.
I'm not sure if the page needs to be real-time ran or not. What I want to do is have a sort of text-based browser game, kind of liek the ones you see on MySpace and Facebook. Similar, but also kind of different. The similarity is basically the fact that you have a character that can fight monsters and each attack lowers their hit points until their dead. So what I'm trying to do is have a list of characters that the user can pick from once they are logged in, then they choose an area to fight in, then a location within that area, then it would generate a random monster in that area and then they could attack, use an item, use magic skills, or defend. I'd like to be able to use like a PHP function for when the user clicks the attack button that it would run a PHP script that would update the monster's hit points, and then once the battle was over, it would reset the hits points back to full health for that monster so it could be fought again or something.
I'm not sure if this has to be done with something like AJAX, but I was wondering if anyone knew if it could be done with something I know a little better like PHP. Or I was hoping someone could at least point me in the right direction if i needed to use something like AJAX or JavaScript and how I might go about using it.
-
Thank you! I was just figuring it out as you were posting it. Thanks for the help!
-
I want to have links on my page that take the viewer to another page. On that second page, I want the PHP to display certain text if a certain link was clicked. Here is what I have so far, but I know something is wrong, I just don't know how to do it. Can anyone help?
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Burg Village</title> </head> <body> <h1>Burg Village</h1> <p>Locations:</p> <p>Hero's House<br /> Best Friend's House<br /> <a href="location.php?location=item_shop">Item Shop</a><br /> Weapon Shop<br /> Exit To Caldor Island</p> </body> </html>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> </head> <body> <h1>Burg Village</h1> <?php if (location == 'item_shop') { echo "Buy <br /> Sell <br /> Exit <br />"; } else { echo "Go somewhere else." ?> </body> </html>
Can anyone tell me how to do this correctly?
-
How can I get the value of the select list option chosen?
-
Can anyone tell me how I could alter my above code to make the identity equal to whatever identity the user chooses in my first select list?
-
I found this example:
<script type="text/javascript"> function test(){ document.getElementById("php_code").innerHTML="<?php $con = mysql_connect('localhost', 'root', ''); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("smrpg", $con); $sql = ('UPDATE derived_values SET health = 7, energy = 5 WHERE identity = "Sailor Reese"'); $result = mysql_query($sql); echo "it worked" ?>"; } </script> <input type="button" value="Attack" onClick="test(); return false;"> <span id="php_code"> </span>
I altered it to see if it would work, and it does, but I need some help getting my values because right now I'm just using constants. I need to get the $identity to equal the identity field first.
-
Is there a way to run a php function when a button is pressed? Or is that something that you do with JavaScript? I have a page that might be a little confusing. It uses JavaScript to run some PHP script to display different tables of information on my page. Here are the pages:
first page:
<?php $get_scouts = "select * from scouts where username = '".$_SESSION['userName']."'"; $get_scouts_res = mysql_query($get_scouts, $conn) or die(mysql_error()); echo "<select name=\"users\" onchange=\"showUser(this.value)\"> <option selected=\"selected\">None Selected</option>"; while ($list_scouts = mysql_fetch_array($get_scouts_res)) { $identity = ucwords($list_scouts['identity']); $topic_id = $list_scouts['id']; echo "<OPTION value=\"$identity\">$identity</OPTION>"; } echo "</select>"; ?> Select an area: <?php $get_map = "select * from monsters1 group by map"; $get_map_res = mysql_query($get_map, $conn) or die(mysql_error()); echo "<select name=\"maps\" onchange=\"showMap(this.value)\"> <option selected=\"selected\">None Selected</option>"; while ($list_maps = mysql_fetch_array($get_map_res)) { $map_id = $list_maps['id']; $map = $list_maps['map']; echo "<option value=\"$map\">$map</option>"; } echo "</select>"; ?> <div id="txtHint2"></div> <table> <tr> <td valign="top"> <div id="txtHint"></div> </td> <td valign="top"><div id="txtHint3"></div></td> </tr> </table> </form>
Here is the JavaScript:
//code to display maps var xmlhttp; function showUser(str) { xmlhttp=GetXmlHttpObject(); if (xmlhttp==null) { alert ("Browser does not support HTTP Request"); return; } var url="getuser.php"; url=url+"?q="+str; url=url+"&sid="+Math.random(); xmlhttp.onreadystatechange=stateChanged; xmlhttp.open("GET",url,true); xmlhttp.send(null); } function stateChanged() { if (xmlhttp.readyState==4) { document.getElementById("txtHint").innerHTML=xmlhttp.responseText; } } function GetXmlHttpObject() { if (window.XMLHttpRequest) { // code for IE7+, Firefox, Chrome, Opera, Safari return new XMLHttpRequest(); } if (window.ActiveXObject) { // code for IE6, IE5 return new ActiveXObject("Microsoft.XMLHTTP"); } return null; } //code to display locations var xmlhttp2; function showMap(str) { xmlhttp2=GetXmlHttpObject(); if (xmlhttp2==null) { alert ("Browser does not support HTTP Request"); return; } var url2="getlocations.php"; url2=url2+"?q="+str; url2=url2+"&sid="+Math.random(); xmlhttp2.onreadystatechange=stateChanged2; xmlhttp2.open("GET",url2,true); xmlhttp2.send(null); } function stateChanged2() { if (xmlhttp2.readyState==4) { document.getElementById("txtHint2").innerHTML=xmlhttp2.responseText; } } function GetXmlHttpObject2() { if (window.XMLHttpRequest2) { // code for IE7+, Firefox, Chrome, Opera, Safari return new XMLHttpRequest2(); } if (window.ActiveXObject) { // code for IE6, IE5 return new ActiveXObject("Microsoft.XMLHTTP"); } return null; } //code to display monsters var xmlhttp3; function showMonsters(str) { xmlhttp3=GetXmlHttpObject(); if (xmlhttp3==null) { alert ("Browser does not support HTTP Request"); return; } var url3="getmonsters.php"; url3=url3+"?q="+str; url3=url3+"&sid="; xmlhttp3.onreadystatechange=stateChanged3; xmlhttp3.open("GET",url3,true); xmlhttp3.send(null); } function stateChanged3() { if (xmlhttp3.readyState==4) { document.getElementById("txtHint3").innerHTML=xmlhttp3.responseText; } } function GetXmlHttpObject3() { if (window.XMLHttpRequest3) { // code for IE7+, Firefox, Chrome, Opera, Safari return new XMLHttpRequest3(); } if (window.ActiveXObject) { // code for IE6, IE5 return new ActiveXObject("Microsoft.XMLHTTP"); } return null; }
Here is the getuser.php:
<?php $q=$_GET["q"]; $con = mysql_connect('localhost', 'root', ''); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("smrpg", $con); $sql="SELECT * FROM derived_values WHERE identity = '".$q."'"; $result = mysql_query($sql); echo "<table border='0'>"; while($row = mysql_fetch_array($result)) { echo "<tr>"; echo "<td colspan=2><strong>" . $row['identity'] . "</strong></td></tr>"; echo "<tr><td align=right><i>Health</i></td><td>" . $row['health'] . "/" . $row['full_health'] . "</td></tr>"; echo "<tr><td align=right><i>Energy</i></td><td>" . $row['energy'] . "/" . $row['full_energy'] . "</td></tr>"; echo "<tr><td align=right><i>Attack</i></td><td>" . $row['acv1'] . "</td></tr>"; echo "<tr><td align=right><i>Defense</i></td><td>" . $row['dcv1'] . "</td>"; echo "</tr>"; } echo "</table>"; mysql_close($con); ?>
Here is the getlocations.php:
<?php $q=$_GET["q"]; $con = mysql_connect('localhost', 'root', ''); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("smrpg", $con); $sql="SELECT * FROM monsters1 WHERE map = '".$q."' group by location"; $result = mysql_query($sql); echo "<table border='0'>"; echo "Select a location: <select name=\"locations\" onchange=\"showMonsters(this.value)\"> <option selected=selected>None Selected</option>"; while($row = mysql_fetch_array($result)) { $location = $row['location']; echo "<option value=\"$location\">" . $row['location'] . "</option>"; } echo "</select>"; mysql_close($con); ?>
Here is the getmonsters.php:
<?php $q=$_GET["q"]; $con = mysql_connect('localhost', 'root', ''); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("smrpg", $con); $sql="SELECT * FROM monsters1 WHERE location = '".$q."' group by name order by rand() limit 1"; $result = mysql_query($sql); echo "<table border='0'>"; echo "<tr><td>"; while($row = mysql_fetch_array($result)) { echo "<strong>" . $row['name'] . "</strong></td>"; } echo "</tr></table><br />"; //function for Attack function attackMonster() { echo "test attack"; } echo "<form><input id=\"attack\" type=\"button\" value=\"Attack\" onlick=\"attackMonster()\"> <input type=\"button\" value=\"Magic\"> <input type=\"button\" value=\"Item\"></form>"; mysql_close($con); ?>
On this last part, the getmonsters.php page, I have three buttons: Attack, Item, and Magic. I would like to know if I can set the Attack button, for example, to run a php script that updates the $health and $energy fields based on some formula? I would need to take the $acv1 variable and create a formula that would figure out the attack damage amount and then the php function would need to update the $health field in the table. Is this possible to do with PHP? Can you set a button to run a php function upon being clicked? Or would I have to use a JavaScript function that ran the PHP functon, or how can I do this? Can anyone help me out with this?
-
And thank you for explaining it, too, so everyone else can understand what I was doing wrong! You are awesome!
-
Finally got it figured out:
<?php $comment_id = $_GET['comment_id']; //connect to server and select database $conn = mysql_connect("localhost", "root", "") or die(mysql_error()); $db = mysql_select_db("testimonials", $conn) or die(mysql_error()); if (isset($_POST['submit'])) { //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $reply=mysql_real_escape_string($_POST['reply']); $comment_id = $_GET['comment_id']; $error='';//initialize $error to blank if(trim($name)=='' ){ $error.="Please enter your name!<br />"; //concatenate the $error Message with a line break } if(trim($email)==''){ $error.="Plese enter your e-mail address!<br />"; } elseif (!preg_match("/^[_\.0-9a-zA-Z-]+@([0-9a-zA-Z][0-9a-zA-Z-]+\.)+[a-zA-Z]{2,6}$/i", $email)) { $error.="The e-mail you entered was not valid!<br />"; } if(trim($reply)=='' ){ $error.="Please enter your reply!<br />"; //concatenate the $error Message with a line break } if($error==''){ $sql="INSERT INTO replies (comment_id, reply, reply_create_time, reply_owner, reply_owner_email) VALUES ( '$comment_id', '$reply', now(), '$name','$email')"; mysql_query($sql,$conn) or die(mysql_error()); header('Location: testimonials.php'); // mysql_query($sql,$conn) or die(mysql_error()); } else{ echo "<div class=error><span style=color:red>$error</span><br /></div>"; } } else { $name= ''; $email= ''; $reply= ''; } ?> <form name="comment" id="comment" onsubmit="return validateFormOnSubmit(this)" action="reply.php?comment_id=<?php echo $comment_id; ?>" method="post"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="name"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" value="<?php echo $name; ?>"/></div></td> </tr> <tr> <td align="left"><label for="email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" value="<?php echo $email; ?>"/></div></td> </tr> <tr> <td align="left"> <label for="reply">Reply:</label></td> <td> <textarea name="reply" id="reply" rows="5" cols="30" value="<?php echo $reply; ?>"></textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div></td> <td width="2"></td> </tr> </table> </form>
Thanks for the help with this Hussam!
-
That suggestion also echos the correct comment_id, but the one i had previously did, too. It prints the correct comment id, it just won't INSERT the correct comment id.
-
How do I echo the sql statement?
-
Nope, didn't work. Still returning a zero value
-
Still inserting a zero value :'(
-
It prints the right value on the page, but it won't insert the value into the table for some reason. Can anyone figure out why?
-
Here is the reply page:
<?php $reply_comment_id = $_GET['comment_id']; echo $reply_comment_id; //connect to server and select database $conn = mysql_connect("localhost", "root", "") or die(mysql_error()); $db = mysql_select_db("testimonials", $conn) or die(mysql_error()); if (isset($_POST['submit'])) { //create and issue the first query $name=mysql_real_escape_string($_POST['comment_owner']); $email=mysql_real_escape_string($_POST['comment_owner_email']); $reply=mysql_real_escape_string($_POST['reply']); $reply_comment_id = $_GET['comment_id']; $error='';//initialize $error to blank if(trim($_POST['comment_owner'])=='' ){ $error.="Please enter your name!<br />"; //concatenate the $error Message with a line break } if(trim($_POST['comment_owner_email'])==''){ $error.="Plese enter your e-mail address!<br />"; } else { if(!preg_match("/^[_\.0-9a-zA-Z-]+@([0-9a-zA-Z][0-9a-zA-Z-]+\.)+[a-zA-Z]{2,6}$/i", $_POST['comment_owner_email'])) { $error.="The e-mail you entered was not valid!<br />"; } } if(trim($_POST['reply'])=='' ){ $error.="Please enter your reply!<br />"; //concatenate the $error Message with a line break } if($error==''){ $sql="INSERT INTO replies (comment_id, reply, reply_create_time, reply_owner, reply_owner_email) VALUES ( '$reply_comment_id', '$reply', now(), '$name','$email')"; mysql_query($sql,$conn) or die(mysql_error()); header('Location: testimonials.php'); // mysql_query($sql,$conn) or die(mysql_error()); } else{ echo "<div class=error><span style=color:red>$error</span><br /></div>"; } } else { $name= ''; $email= ''; $reply= ''; } ?> <form name="comment" id="comment" onsubmit="return validateFormOnSubmit(this)" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <table border="0" cellspacing="0" cellpadding="5" width="662" class="style2"> <tr> <td align="left"><label for="name"> Name:</label></td> <td> <div class="c2"><input type="text" name="comment_owner" id="comment_owner" size="30" value="<?php echo $name; ?>"/></div></td> </tr> <tr> <td align="left"><label for="email">E-mail:</label></td> <td> <div class="c2"><input type="text" name="comment_owner_email" id="comment_owner_email" size="30" value="<?php echo $email; ?>"/></div></td> </tr> <tr> <td align="left"> <label for="reply">Reply:</label></td> <td> <textarea name="reply" id="reply" rows="5" cols="30" value="<?php echo $reply; ?>"></textarea></td> </tr> <tr> <td colspan="4"> <div class="c1"><input name="submit" type="submit" value="Submit" /> <input type="reset" name="reset" id="reset" value="Reset" /></div></td> <td width="2"></td> </tr> </table> </form>
-
Dark, that doesn't bring anything because it's named $reply_comment_id, but if I change it, it still doesn't print any value. :-\
-
I tried echoing the comment id like this and it worked:
echo $_GET['comment_id'];
I added this right after the first php tag at the beginning and it printed the right comment_id.
-
I know it is getting the comment_id because I tried echoing it and it is getting it, so I can't figure out why I can't insert it???
-
Dark:
I think this example is basically what I am doing. On the first page, it displays all the comments and replies. There is a link to reply to each post, which does exactly what your suggested example does: it sends in the link to the url the comment id:
<a href=\"reply.php?comment_id=$comment_id\">Reply</a>
If you look at my page, when you hover over the link, it will show you the url:
Example: http://webdesignsbyliz.com/reply.php?comment_id=59
And when you click the actual link, you see in the url the comment has been sent through:
http://webdesignsbyliz.com/reply.php?comment_id=59
So, this should mean that I can use the $_GET['comment_id'] to send in the form for the reply to insert that value into my table when the form submits the rest of the posted data, but it's not able to access it for some reason. That is what we're trying to figure out.
-
I will look at your suggestion dark and try it out. Thanks!
-
So on the first page the viewer comes to (second code listed above), they can select a comment to reply to. The link sends with it to the next page (the third code listed above) the comment_id from the comment they have chosen to reply to. So the comment_id comes from the previous page but should be accessible from the reply page. I can't figure out why it's not working. I have another page that is similar and it works on that page. It is using the same basic principle, so I don't know what I'm doing wrong.
Checking For Existing Field Value??
in PHP Coding Help
Posted
How can I check my table for existing field values and update a field if the value exists or add a new record if the value does not? I have the following page:
Right now I have it checking for all the items in the character's inventory, but now I want to check that if the $_POST['sel_item'] value appears in the table inventory in field item, then I want to update the quantity field by the $_POST['sel_item_qty'], but if not, I need to add a new record, inserting the item and quantity into the table. Can anyone tell me how to check for the existing field value and then update or insert, according the result?