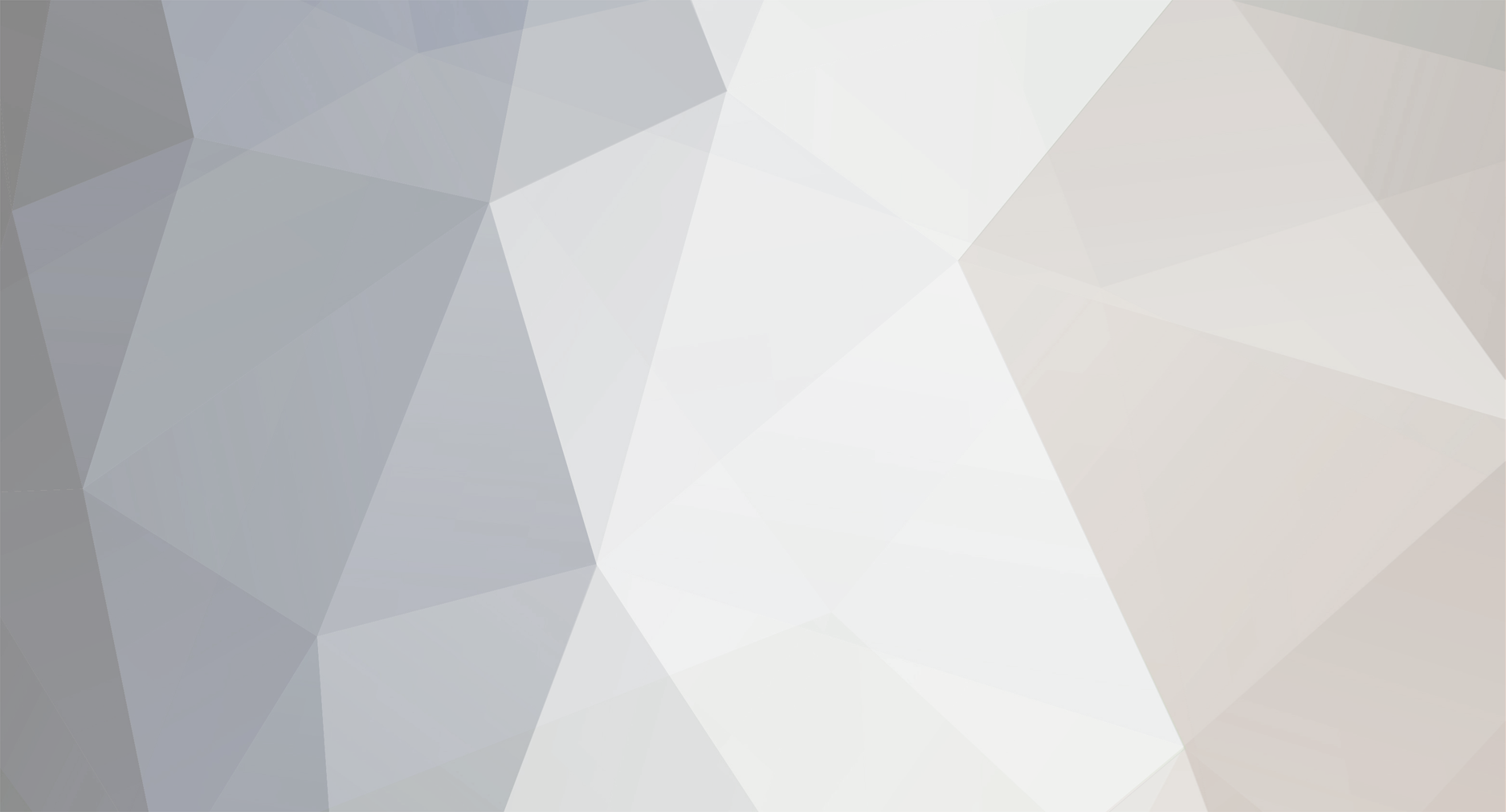
AtomicRax
Members-
Posts
69 -
Joined
-
Last visited
Everything posted by AtomicRax
-
I found a function online to auto link any variable containing text. It looks for http:// or www. in the text and then automatically adds the HTML code to turn it into a link. I have a problem though.. I have some html already in some of the variables that contain IMG tags. This function tries to link the url of the image.. so I get something like this: <img src="<a rel="nofollow" href="IMAGEURL" target="_blank">IMAGEURL</a>" border="0" /> This creates a problem and the images don't load! Is there anyway to stop it from doing this? function auto_link($text) { $pattern = '#\b(([\w-]+://?|www[.])[^\s()<>]+(?:\([\w\d]+\)|([^[:punct:]\s]|/)))#'; return preg_replace_callback($pattern, 'auto_link_callback', $text); } function auto_link_callback($matches) { $max_url_length = 50; $max_depth_if_over_length = 2; $ellipsis = '…'; $url_full = $matches[0]; $url_short = ''; if (strlen($url_full) > $max_url_length) { $parts = parse_url($url_full); $url_short = $parts['scheme'] . '://' . preg_replace('/^www\./', '', $parts['host']) . '/'; $path_components = explode('/', trim($parts['path'], '/')); foreach ($path_components as $dir) { $url_string_components[] = $dir . '/'; } if (!empty($parts['query'])) { $url_string_components[] = '?' . $parts['query']; } if (!empty($parts['fragment'])) { $url_string_components[] = '#' . $parts['fragment']; } for ($k = 0; $k < count($url_string_components); $k++) { $curr_component = $url_string_components[$k]; if ($k >= $max_depth_if_over_length || strlen($url_short) + strlen($curr_component) > $max_url_length) { if ($k == 0 && strlen($url_short) < $max_url_length) { // Always show a portion of first directory $url_short .= substr($curr_component, 0, $max_url_length - strlen($url_short)); } $url_short .= $ellipsis; break; } $url_short .= $curr_component; } } else { $url_short = $url_full; } return "<a rel=\"nofollow\" href=\"$url_full\" target=\"_blank\">$url_short</a>"; }
-
Creating temp file and transfering through ftp
AtomicRax replied to AtomicRax's topic in PHP Coding Help
I've changed $thumb_path to this: $thumb_path = tempnam("/tmp", $new_file_name); and then added an unlink() after it uploads the file, and it works now! Thanks. -
Try this: <?php // rotate images randomly but w/o dups on same page - format: // <img src='rotate.php?i=0'> - rotate image #0 - use 'i=1' // for second, etc // (c) 2004 David Pankhurst - use freely, but please leave in my credit $images=array( // list of files to rotate - add as needed "images/1.jpg", "images/2.jpg", "images/3.jpg", "images/4.jpg", "images/5.jpg", "images/6.jpg", "images/7.jpg", "images/8.jpg", "images/9.jpg" ); $total=count($images); $secondsFixed=2; // seconds to keep list the same $seedValue=(int)(time()/$secondsFixed); srand($seedValue); for ($i=0;$i<$total;++$i) // shuffle list 'randomly' { $r=rand(0,$total-1); $temp =$images[$i]; $images[$i]=$images[$r]; $images[$r]=$temp; } $index=(int)($_GET['i']); // image index passed in $i=$index%$total; // make sure index always in bounds $file=$images[$i]; header("Cache-Control: no-cache, must-revalidate"); header("Expires: Sat, 26 Jul 1997 05:00:00 GMT"); header ("Location: $file"); header ("Content-Length: 0"); exit; ?> Also header("Pragma: no-cache") is another no cache header..
-
From the beginning, the user accesses my website and uploads an image. Currently I'm using SimpleImage.php available at http://www.white-hat-web-design.co.uk/articles/php-image-resizing.php to resize uploaded pictures. I create two copies, a regular size and a thumbnail size. Then I transfer the converted files through FTP to my storage server. The regular size works great, but the thumbnail transfers as a blank file. I don't want to save the files on the non-storage server at all, so I'm trying to work with temporary files. include('./SimpleImage.php'); // A few variables $file_name = basename($_FILES['photo1']['name']); $random_digit=mt_rand(111111111,2147483647); // New file name $new_file_name = $userInfo['id'].'_'.$random_digit.$random_digit.'_'.substr($file_name, -5); // Paths to temporary files $target_path = $_FILES['photo1']['tmp_name']; $thumb_path = tmpfile(); // Start SimpleImage and load temp file $simImage = new SimpleImage(); $simImage->load($target_path); // Get the temp image dimensions list($width, $height) = getimagesize($target_path); // Resize the file if it's too tall if ($height > $var['maxPhotoHeight']) { $simImage->resizeToHeight($var['maxPhotoHeight']); $simImage->save($target_path); } // Get the newly resized image dimensions list($width, $height) = getimagesize($target_path); // Resize the image if it's too wide if ($width > $var['maxPhotoWidth']) { $simImage->resizeToWidth($var['maxPhotoWidth']); $simImage->save($target_path); } // Resize for thumbnail width $simImage->resizeToWidth($var['thumbWidth']); $simImage->save($thumb_path); // Get thumbnail dimensions list($width, $height) = getimagesize($thumb_path); // Resize the thumbnail if it's too tall if ($height > $var['thumbHeight']) { $simImage->resizeToHeight($var['thumbHeight']); $simImage->save($thumb_path); } // Was told this was important, but I've tried with and without it and got the same result fseek($thumb_path,0); // Perform File Transfer ftpTransfer($target_path, "$new_file_name"); ftpTransfer($thumb_path, "thumbs/$new_file_name"); // My Simple FTP Transfer Function: function ftpTransfer($source, $name) { global $ftp; $ftpMeta = "ftp://" . $ftp['user_name'] . ":" . $ftp['user_pass'] . "@" . $ftp['server'] . $ftp['dir'] . $name; $ftpData = file_get_contents($source); file_put_contents($ftpMeta, $ftpData); } Like I said, the regular sized image works great!! the thumbnail file is only created on the storage server but it has a 0kb file size. I'm sure it has to do with me working with a temporary file. tmpfile() claims to remove the file after it's closed, so maybe by using the Save feature in SimpleImage, it's closing the file and then the server automatically removes the temporary file? SimpleImage has a feature where I can write the image output [possibly to a file] using $simImage->output() for the data. If that helps with a solution? I don't know. I know it might be complicated, but any help is appreciated!
-
Instead of a hidden element, why not just assign the value to the button? <form action='findMovie.php' method='post'> <table> <?php $result = mysql_query ("SELECT genre FROM genres ORDER BY genre"); while ($row = mysql_fetch_array($result)) { $genre = $row['genre']; echo "<tr><td>" .$genre ."</td><td><input type='submit' name='listMovies' value='" . $genre ."'></td></tr>"; } ?> </table> </form> That way when you click a button, the genre (value of the button) can be read from $_POST['listMovies'] Or like btherl said with a drop down list... <form action='findMovie.php' method='post'> <select name="listMovies"> <?php $result = mysql_query ("SELECT genre FROM genres ORDER BY genre"); while ($row = mysql_fetch_array($result)) { $genre = $row['genre']; echo "<option value='" .$genre ."'>" . $genre ."</option>"; } ?> </select> </form>
-
While not too helpful, it's not recommended that you use or store passwords unencrypted.. This might be considered a "preference" but it's definitely a more secure way of handling them.
-
That's what I was thinking, since it's actually meant for file transfers, but I just wanted to make sure. Thanks! And for others who are curious, here's two snippets I found online at http://davidwalsh.name/send-files-ftp-php $connection = ftp_connect($server); $login = ftp_login($connection, $ftp_user_name, $ftp_user_pass); if (!$connection || !$login) { die('Connection attempt failed!'); } $upload = ftp_put($connection, $dest, $source, $mode); if (!$upload) { echo 'FTP upload failed!'; } ftp_close($connection); $upload = copy($source, 'ftp://user:password@host/path/file'); if (!$upload) { echo 'FTP upload failed!'; }
-
When the user accesses logout.php directly and they click on the "Yes" to logout, they go straight to logout_yes.php never get their session destroyed. Since you're using two files, try something like this: logout.php <? session_start(); ?> <html> <body> <tr><td colspan="3"><p>Logout</p></td></tr><tr><table> <center>Are you sure you want to logout?</center><br /> <center><a href=logout_yes.php>Yes</a> | <a href=javascript:history.back()>No</a> </body> </html> logout_yes.php <html> <body> <? session_destroy(); if(!session_is_registered('first_name')){ echo "<center><font color=red><strong>You are now logged out!</strong></font></center><br />"; echo "<center><strong>Login:</strong></center><br />"; include 'login_form.php'; } ?> </body> </html>
-
I'm not asking for specific coding help, but what I am looking for is an answer from someone who might have dealt with this in the past and can help shed some light on the subject. I'm working on a website that allows the user to upload several images at a time. I have serverA that hosts the website and database and serverB that hosts the image uploads. I'm looking for the best (preferably the fastest) method to transfer image files from serverA to serverB. I know I can transfer the files through FTP in PHP and I can also do it via HTTP by making a small script on serverB that would accept _POST transfers of each file.. Are there other methods? Remember, there are 2-3 images sent each time.. so just because a method might be "preferred," I don't want to make the user wait longer than they have to. Thanks for your time.
-
I've made some progress; I've managed to add the distance for each array in the mysql data array. Now it appears I'm stuck on sorting a multidimensional array based on two key values of arrays inside of them... I have this array: Array ( [0] => Array ( [superstore] => 0 [name] => My nonSuperstore [address] => ... [city] => ... [zip_code] => 70601 [phone] => ... [website] => ... [distance] => 0 ) [1] => Array ( [superstore] => 1 [name] => My Superstore [address] => ... [city] => ... [state] => ... [zip_code] => 70648 [phone] => ... [website] => ... [distance] => 27.29 ) [2] => Array ( [superstore] => 0 [name] => My nonSuperstore [address] => ... [city] => ... [state] => ... [zip_code] => 70651 [phone] => ... [website] => ... [distance] => 24.45 ) ) I've been browsing the sort, usort, asort, multisort, etc... manual pages and different tutorials but I can't figure it I need to sort the multidimensional array first by "superstore" then by "distance" .. making sure the superstores are listed above all else, and then the distance is sorted by the closest location to furthest location.. keeping the most outer key value is not important. Meaning I need to sort the above array into this: Array ( [0] => Array ( [superstore] => 1 [name] => My Superstore [address] => ... [city] => ... [state] => ... [zip] => 70648 [phone] => ... [website] => ... [distance] => 27.29 ) [1] => Array ( [superstore] => 0 [name] => My nonSuperstore [address] => ... [city] => ... [zip] => 70601 [phone] => ... [website] => ... [distance] => 0 ) [2] => Array ( [superstore] => 0 [name] => My nonSuperstore [address] => ... [city] => ... [state] => ... [zip] => 70651 [phone] => ... [website] => ... [distance] => 24.45 ) )
-
My deepest apologizes, I did leave something out. I'm also trying to sort the super stores on top of the regular stores, but still maintain the original zip code sorting. The column "superstore" is equal to 1 if it is a superstore, and 0 if it's not.. so the real query is: $query = query("SELECT * FROM " . $db['prefix'] . "stores $WHERE ORDER BY superstore DESC LIMIT $from, $maxResults"); which puts the super stores on top, but nothing is sorted correctly by zip code
-
I'm working on a store locator style program. I first get and sort all zip codes based on a their distance to an original location. I then use that zip code array to find all stores in the mysql database, but when I get the results, they're no longer arranged by distance from the original location.. and I can't just sort them ascending or descending based on their zip codes because that doesn't sort them correctly either. So I need to sort the data I get from the mysql database based on their "zip_code" column and in order from the $zip array.. Here's an example of what $zip looks like.. the zip code is the key and the distance from the original zip is the value [meaning the first key is the $originalZip]: Array ( [70601] => 0 [70616] => 1.12 [70629] => 1.2 [70612] => 1.24 [70602] => 1.31 [70615] => 1.88 [70609] => 3.41 [70605] => 4.4 [70669] => 4.62 [70606] => 4.9 [70611] => 7.23 [70607] => 9.68 [70663] => 9.74 ) and then I create the $WHERE variable by each key supplied: $WHERE = "WHERE zip_code='$originalZip'"; foreach ($zip as $key => $value) { if ($key == $originalZip) {} else { $WHERE .= " OR zip_code='$key'"; } } } Then I do the query [with a paginator]: $query = query("SELECT * FROM " . $db['prefix'] . "stores $WHERE LIMIT $from, $maxResults"); but when I print the data, it doesn't keep the correct sorting format.. if (mysql_num_rows($query) > 0) { for ($i = 0; $i<mysql_num_rows($query); $i++) { $storeData = mysql_fetch_array($query, MYSQL_ASSOC); // print store information here such as $storeData['name'] $storeData['address'] $storeData['zip_code'] } } Is there an easy way to sort the mysql results based on the $zip array?
-
Which PHP Editor do you think is the best? [v2]
AtomicRax replied to Daniel0's topic in Miscellaneous
I like Notepad++ because it works great, but also keeps it simple. It doesn't try to incorporate too much at once.. and honestly it was the first one I found when searching. so yeah.. I was going to look at NetBeans, but the website looks down right now? but I'll definitely try out Eclipse since I can access it's website -
or do I need to find the domain's IP address and then query it that way? Either way is fine because I think there's an easy way to do that in php.. also, I'd prefer free lookups.. not wanting to pay for this service..
-
I've set up my website to automatically link any posted URLs.. however, I want to check to see if the domain is blacklisted somewhere for spam, fraud, etc, and then alert the user before they continue to the website.. Does anyone know of an API I can use to search for this? I know I can manually search websites like http://www.spamhaus.org/ for blacklisted information, but what I'm looking for is something where my script can automatically query http://somewebsite.com/blaclistapi?domain=otherdomain.com and it would return information if the domain is blacklisted or not.. Anyone know of any resources like this?
-
I've used http://www.micahcarrick.com/php-zip-code-range-and-distance-calculation.html which is a php class for zip code search, radius search, etc... But the download contains a MySQL table structure and data with all [or I haven't found any missing yet] zip codes, with their city, state, lat, long, and some other information
-
I use $body = str_replace("{TITLE}", $title, $body); to replace the page title.. but that doesn't apply in this case either because I'm trying to get specific information from between the { } to use while modifying the same tag
-
I'm trying to develop a website file manager. I want to allow SSI, and would like to handle it specifically.. $regexp = "<!--#include\s[^>]*virtual=(\"??)([^\" >]*?)\\1[^>]*\/-->"; if(preg_match_all("/$regexp/siU", $body, $matches, PREG_SET_ORDER)) { foreach($matches as $match) { $includeFile = $match[2]; } } This snippet shows how you would get the included file path in the SSI code of the website body [ the $body tag ], but I don't need to just find it- I want to replace the SSI code with a simpler code in the HTML that appears in the editor for easy management.. Then switch back from my easier code to the actual SSI code.. Example: Replace <!--#include virtual="/newsManager/output.php"--> With {INCLUDE=/newsManager/output.php} THEN when the user saves the page, Replace {INCLUDE=/newsManager/output.php} With <!--#include virtual="/newsManager/output.php"--> Is there an easy fix or should I just switch to a full blown template engine.. even though this is the only required feature?
-
For reference, I believe I've got it. <!--#include virtual="/output.php?${QUERY_STRING}" -->
-
I have a file called myfile.html and it includes the following line: <!--#include virtual="/output.php" --> The SSI statement works great, but I want more... I would like to be able to link to myfile.html?var=val and use the var string in the php file. However $_GET[var] equals nothing when I try this Is this not possible?
-
For some reason it needed to be: mysql_query("INSERT INTO ads_entries (`adID`, `uid`, `username`, `date`, `timestamp`, `enddate`, `category`, `parish`, `title`, `description`, `pictureA`, `pictureB`, `pictureC`, `tnPicture`) VALUES (\"\", \"$uid\", \"$user\", \"$date\", \"$time\", \"$enddate\", \"$category\", \"$parish\", \"$title\", \"$description\", \"$file1\", \"$file2\", \"$file3\", \"$thumb\")"); Either way, I got it now.
-
This is about to drive me insane! I've double checked all of my variables, they're all assigned OK and everything (each variable has the correct value)...but for some reason, this code will NOT insert a new row! $file1 = "/path/to/picture.jpg"; $file2 = "/path/to/picture.gif"; $file3 = "/path/to/picture.png"; $thumb = "/path/to/tn_picture.jpg"; $date = date('m/d/Y'); $enddate = date('m/d/Y', strtotime("+30 days")); $uid = $mySite->user['uid']; $user = $mySite->user['username']; $time = time(); $category = $_POST[category]; $parish = $_POST[parish]; $title = $_POST[title]; $description = strip_tags($_POST[description]); $description = substr($description, 0, 2000); mysql_query("INSERT INTO ads_entries (`adID`, `uid`, `username`, `date`, `timestamp`, `enddate`, `category`, `parish`, `title`, `description`, `pictureA`, `pictureB`, `pictureC`, `tnPicture`) VALUES ('', '$uid', '$user', '$date', '$time', '$enddate', '$category', '$parish', '$title', '$description', '$file1', '$file2', '$file3', '$thumb')");
-
For the record, I got what I wanted by using the following: $f = 0; $d = 0; // Open $var['path'] directory if ($handle = opendir($var['path'])) { while (false !== ($file = readdir($handle))) { if ($file != "." && $file != ".." && is_dir("$var[path]/$file")) { // $file is a directory $dirList[$d] = $file; $d++; } elseif ($file != "." && $file != "..") { // $file is not a directory $fileList[$f] = $file; $f++; } } closedir($handle); } @sort($fileList); @sort($dirList); for ($x = 0; $x < $d; $x++) { $html .= "<a href=\"{$var['url']}/fileDir.php?dir=$dirList[$x]\">$dirList[$x]/</a><br>\n"; } for ($x = 0; $x < $f; $x++) { $html .= "<a href=\"{$var['url']}/fileEdit.php?file=$fileList[$x]\">$fileList[$x]</a><br>\n"; }
-
You have two concurrent else statements. The following will redirect to index.php if NOT logged in...is that what you're trying to achieve? function procCreate(){ global $session, $form; if($session->logged_in){ $dir = preg_replace("/(.*)(\/)$/","\\1", $dir); $dir = $session->username; $absolute_dir = $_SERVER['DOCUMENT_ROOT'].''.$dir; if (!is_dir($absolute_dir)) { mkdir($absolute_dir, 0777); chmod($absolute_dir, 0777); return $absolute_dir; } else { echo "There was an error processing your request"; } } else { header("Location: index.php"); } }
-
Currently I have: $i = 0; if ($handle = opendir($var['path'])) { while (false !== ($file = readdir($handle))) { if ($file != "." && $file != "..") { $fList[$i] = $file; $i++; } } closedir($handle); } @sort($fList); for ($x = 0; $x < $i; $x++) { if(is_dir("{$var['path']}/$fList[$x]")) { $html .= "<a href=\"{$var['url']}/fileDir.php?dir=$fList[$x]\">$fList[$x]/</a><br>\n"; } else { $html .= "<a href=\"{$var['url']}/fileEdit.php?file=$fList[$x]\">$fList[$x]</a><br>\n"; } } Which opens a directory and lists all the files and folders inside. Using sort(), the listing is in alphabetical order. Everything is one alphabetized list, which looks like this: I'm trying to group the directories in alphabetical order on top of the file listing, so it would sort like this: Any ideas?