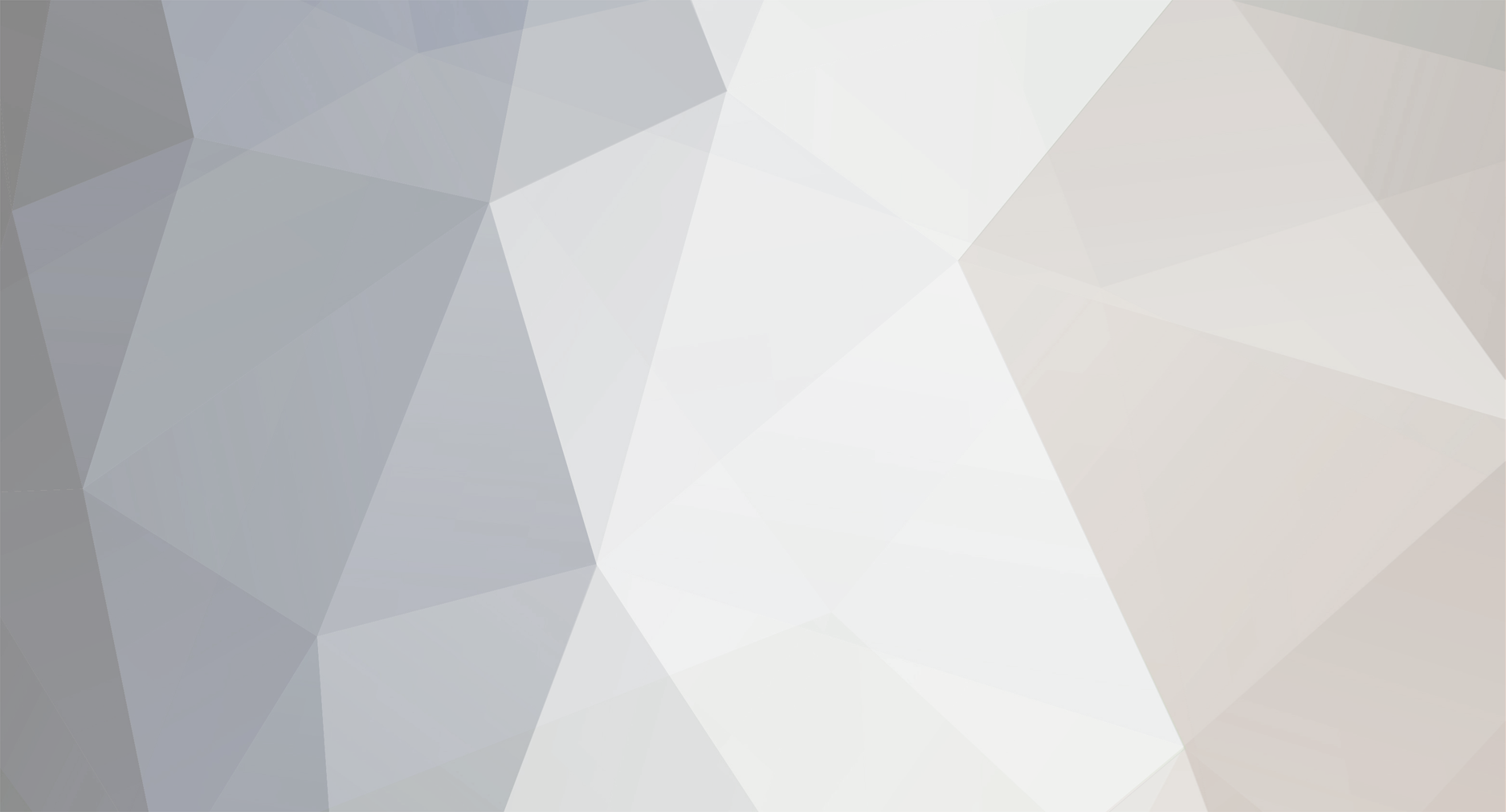
F1Fan
Members-
Posts
1,182 -
Joined
-
Last visited
Everything posted by F1Fan
-
[SOLVED] removing place values from a number - very easy Q
F1Fan replied to aebstract's topic in PHP Coding Help
If you want to round it, just use round($number,2) for 2 decimal places. If you just want to truncate the rest of the decimal places, you'll have to create a function that does that. -
I created a similar script. Try modding this to your needs: <?php function MoveDir2($empnum,$row,$dir){ global $db; if ($dir=="up") $new = $row-1; else $new = $row+1; $query = "SELECT sort_order, empnum, title, description, image, feature, action FROM custom_leftmenu WHERE " . "empnum = $empnum ORDER BY sort_order"; $items = $db->getAssoc($query); // Here's what should work for ya: $move1 = $items[$new]; $move2 = $items[$row]; $items[$row] = $move1; $items[$new] = $move2; ksort($items); // to here... $q = "DELETE FROM custom_leftmenu WHERE empnum = $empnum; "; $count = 1; foreach ($items as $row){ $q .= "INSERT INTO custom_leftmenu (sort_order, empnum, title, description, image, feature, action) " . "VALUES ($count, $empnum, '{$row['title']}', '{$row['description']}', '{$row['image']}', " . "'{$row['feature']}', '{$row['action']}'); "; $count++; } return pg_query($q); } ?>
-
If that's the case, you'll need to use AJAX. You'll need a javascript function that runs every X seconds or minutes that calls some PHP code to check the DB for any updates. If there are updates, the javascript function should update with the new value.
-
Yes, but that will only vertically align the elements in the body. Your problem is that the data needs to be vertically aligned in a specific div. But, since we don't know which div is the problem, I suggest either adding it to all of them, or one by one until you see something change.
-
Or you could use javascript's window.reload() function.
-
PHP won't automatically generate a table. I suggest you add the vertical-align: top; to ALL the divs. I think the more likely situation than PHP generating a table is that the divs are defaulting to vertical-align: middle;, because it's not explicitly set.
-
OK, so I have a frequent need to insert or update data into a table. But the problem is that I have to do a select to determine if it needs to be an update or an insert. This works perfectly, but it's a little bulky. So, my question is this: Is there a way to have ONE query run that inserts if the where statement in the update doesn't return any rows to update? Here's my working code: <?php $query = "SELECT c FROM feature_count WHERE f = '$f' AND a = '$a'"; $count = $db->getOne($query); if ($count>0){ $count++; $query = "UPDATE feature_count SET c = $count WHERE f = '$f' AND a = '$a'"; } else{ $query = "INSERT INTO feature_count (f, a, c) VALUES ('$f', '$a', 1)"; } $db->query($query); ?> Basically, this function runs on every page someone on my site hits. It counts the features that are used. I really just want something like this: <?php $query = "UPDATE feature_count SET c = (c+1) WHERE f = '$f' AND a = '$a'"; ?> And if there are no rows where f = '$f' AND a = '$a', I want it to insert a row with f = '$f' and a = '$a' and c = 1. Any ideas?
-
Mchl -> did you read the whole topic, or just the title and one post??
-
You have so many divs on that page, that I'm getting a little lost. Never the less, try adding the "vertical-align: top;" to the CSS of any element that those items are contained in. If that doesn't work, try playing around with it. Try messing it up. Sometimes that helps me see something that I was blind to before.
-
[SOLVED] GD resized(smaller) image has larger filesize than original
F1Fan replied to Darkstar's topic in PHP Coding Help
Have you tried phpThumb()? It's awesome! http://phpthumb.sourceforge.net/ I've taken 2MB full-size images and created thumbnails (and smaller representations) of it, and it loads WAY quicker than a 2MB file would. You can also set the quality, which affects speed. -
Yes, that is exactly what I meant. I'm glad it worked.
-
You need a session start on every page. Then, if they log in successfully, save their username in a $_SESSION variable. The next time the login script goes to authenticate, have it check to see if the $_SESSION username variable has been set. If it has, assume authentication. Otherwise, send them back to the login screen to log in.
-
Did you start a new topic with your "If" statement question? I can help you with it now, but I don't know where you want me to post it.
-
Have you gotten this working yet? I looked at the page, and I think you need to decide what percentage of the width you want each table to take up on the page. Then do something similar to what dropfaith's example, like this: <table width="100%"> <tr> <td width="50%" align="center" valign="top"> <!-- Your first table (left) here --> </td> <td width="50%" align="center" valign="top"> <!-- Your second table (right) here --> </td> </tr> </table> If you want them to appear center aligned on the page, alight the left one "right" and the right one "left." But that would only work if you keep the 50/50 split.
-
[SOLVED] $id = $_GET['id'] - can't get it to work :(
F1Fan replied to selliott's topic in Microsoft SQL - MSSQL
My bad, I missed a )... Try: if (!isset($_GET['id'])) die("ID not set..."); Also, where are you getting "$_GET"? A form I'm assuming? using the "get" method, not post? -
Yes, but I'm signing off for tonight. I'll help you with it tomorrow.
-
WOO HOO! Is that what you wanted?
-
Arg, I missed one thing: <?php echo "<table border='0' width='40%' id='stats'>\n"; echo "<tr><td colspan='7' id='th'>Character Attributes And Sub-Attributes</td></tr>\n"; echo "<tr class='sub'><td>Attribute/Sub-Attribute</td><td colspan='3' align='center'>Level</td><td colspan='3' align='center'>Points</td></tr>\n"; echo "<tr><td></td></tr>\n"; // keeps getting the next row until there are no more to get while($row = mysql_fetch_array( $result )) { echo "<tr><td>\n"; echo $row['attribute_id']; echo " "; echo $row['desc']; echo "</td><td width='2%'> </td><td width='1%' align='right'>\n"; echo $row['level']; echo "</td><td width='2%'> </td><td width='2%'> </td><td width='1%' align='right'>\n"; echo $row['points']; echo "</td><td width='2%'> </td></tr>\n"; } echo "</table>"; ?>
-
LOL, I see! No, after I saw your code, I decided the table within a table wouldn't do what we want. Try this one (it will at least make the code easier to look at when you view the source): <?php echo "<table border='0' width='40%' id='stats'>"; echo "<tr><td colspan='7' id='th'>Character Attributes And Sub-Attributes</td></tr>"; echo "<tr class='sub'><td>Attribute/Sub-Attribute</td><td colspan='3' align='center'>Level</td><td colspan='3' align='center'>Points</td></tr>"; echo "<tr><td></td></tr>"; // keeps getting the next row until there are no more to get while($row = mysql_fetch_array( $result )) { echo "<tr><td>"; echo $row['attribute_id']; echo " "; echo $row['desc']; echo "</td><td width='2%'> </td><td width='1%' align='right'>"; echo $row['level']; echo "</td><td width='2%'> </td><td width='2%'> </td><td width='1%' align='right'>"; echo $row['points']; echo "</td><td width='2%'> </td></tr>"; } echo "</table>"; ?> I missed some stuff.
-
Simple Problem! Not complex, well I don't think so!
F1Fan replied to stig1's topic in PHP Coding Help
Yup. -
OK, I think I completely understand now. I thought I did before, but looking at your page made me realize exactly what you want. This will end up a little weird, but here goes: <?php echo "<table border='0' width='40%' id='stats'>"; echo "<tr><td colspan='7' id='th'>Character Attributes And Sub-Attributes</td></tr>"; echo "<tr class='sub'><td>Attribute/Sub-Attribute</td><td colspan='3' align='center'>Level</td><td colspan='3' align='center'>Points</td></tr>"; echo "<tr><td><td></tr>"; // keeps getting the next row until there are no more to get while($row = mysql_fetch_array( $result )) { echo "<tr><td>"; echo $row['attribute_id']; echo " "; echo $row['desc']; echo "</td><td width='2%'></td><td width='1%' align='right'>"; echo $row['level']; echo "</td><td width='2%'></td><td width='1%' align='right'>"; echo $row['points']; echo "</td><td width='2%'></td></tr>"; } echo "</table>"; ?> That's an experimental try. Let me know how it goes.
-
Try changing this line: if ($curPageURL == "http://www.DOMAIN.com/"){ to this: if ($curPageURL() == "http://www.DOMAIN.com/"){
-
Which one is the one you want split up? If there's other data going into the table, you may have to include it in the existing table.
-
[SOLVED] $id = $_GET['id'] - can't get it to work :(
F1Fan replied to selliott's topic in Microsoft SQL - MSSQL
I disagree. I believe it is referring to the $_GET['id'], rather than the $id variable. To do it correctly, do this: <?php //--------define connectivity information if (!isset($_GET['id']) die("ID not set..."); $ConnString="DRIVER={SQL Server};SERVER=server_URL_here;DATABASE=database_name_here"; $DB_User="database_username_here"; $DB_Passwd="password_here"; $id = $_GET['id']; //--this is where my problem is $table = "photos$id"; //-------Query database $QueryString="SELECT * FROM $table"; //-------connect to database $Connect = odbc_connect( $ConnString, $DB_User, $DB_Passwd ); $Result = odbc_exec( $Connect, $QueryString ); // MAKE THE XML DOC $nl = "\r\n"; echo "<?xml version=\"1.0\" encoding=\"ISO-8859-1\"?>" . $nl; echo "<master>" . $nl; while($r = odbc_fetch_array( $Result ) ) { echo '<node photo="' . $r['photo'] . '" description="' . $r['description'] . '">' . $nl; echo '</node>' . $nl; } echo "</master>" . $nl; odbc_free_result( $Result ); odbc_close( $Connect ); ?> or, to just mask the notice, do this: <?php //--------define connectivity information $ConnString="DRIVER={SQL Server};SERVER=server_URL_here;DATABASE=database_name_here"; $DB_User="database_username_here"; $DB_Passwd="password_here"; $id = @$_GET['id']; //--this is where my problem is $table = "photos$id"; //-------Query database $QueryString="SELECT * FROM $table"; //-------connect to database $Connect = odbc_connect( $ConnString, $DB_User, $DB_Passwd ); $Result = odbc_exec( $Connect, $QueryString ); // MAKE THE XML DOC $nl = "\r\n"; echo "<?xml version=\"1.0\" encoding=\"ISO-8859-1\"?>" . $nl; echo "<master>" . $nl; while($r = odbc_fetch_array( $Result ) ) { echo '<node photo="' . $r['photo'] . '" description="' . $r['description'] . '">' . $nl; echo '</node>' . $nl; } echo "</master>" . $nl; odbc_free_result( $Result ); odbc_close( $Connect ); ?> -
Try adding your connection variable to it like so: <?php $dbh=mysql_connect ("localhost", "name", "password") or die ('I cannot connect to the database because: ' . mysql_error()); mysql_select_db ("database",$dbh); ?>