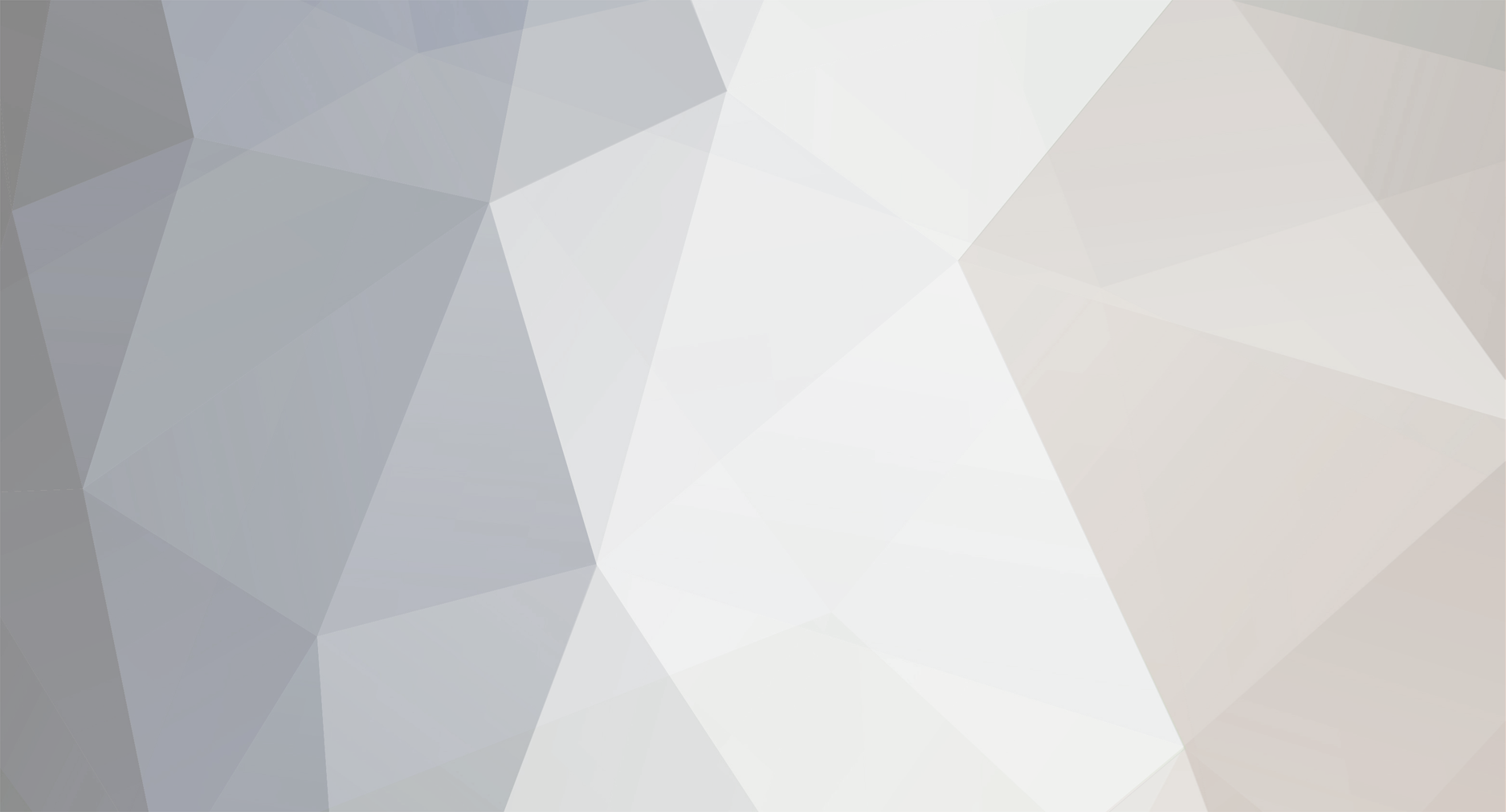
GKWelding
Members-
Posts
268 -
Joined
-
Last visited
Everything posted by GKWelding
-
Can you post here what your serialized array looks like? I'd be able to help you more if you did. Cheers.
-
insert/update functions for mysql, what do you think?
GKWelding replied to Goat's topic in PHP Coding Help
the only major problem I can see is that you have no support for boolean datatypes. Is there any particular reason for this? -
can you explain what you're hoping to do with this script? what does test.php contain?
-
managed to solve it myself by doing the following... RewriteCond %{REQUEST_URI} ^/clinics/(dashboard)([a-zA-Z0-9_-\.]+)$ RewriteRule ^(.*)$ index.php?/frontend/index/$1$2 [L] RewriteCond %{REQUEST_URI} ^/clinics/dashboard.* RewriteRule ^(.*)$ index.php?$1[L] The first rewrite condition determines whether alphanumeric character, _, - or a . is the first character after the url. If it is then redirect to the first function. If it isn't then assume the url is either url.com/dashboard or url.com/dashboard/ rather than url.com/dashboard.html or url.com/dashboard-view.html.
-
Parse error: syntax error, unexpected T_ELSEIF
GKWelding replied to strago's topic in PHP Coding Help
that was just me being lazy and not checking my code... dammit... -
ok, have just read your reply. I get what you're trying to do, but this is the completely wrong way to go about it. You should do the following. if($screen_resolution =="1024x768") { $max = 9; }else if($screen_resolution =="1440x900") { $max = 14; } for($col = 0; $col < $max; $col++) { // code in your for loop... }
-
Random code, the sane way of doing what your code does above is the following. if($screen_resolution =="1024x768") { $col = 8; }else if($screen_resolution =="1440x900") { $col = 13; }
-
Parse error: syntax error, unexpected T_ELSEIF
GKWelding replied to strago's topic in PHP Coding Help
for a start I'm not a huge fan of the syntax you're using, secondly, I thought it was a colon rather than a semi colon after the if statement, and thirdly you need to put a conditional after your elseif. It needs to be elseif ($a == $b). If you wanted the elseif to be a default incase the if statement wasn't true then you should just do else. I would change your syntax to: $download_link = $tube->get('http://www.youtube.com/watch?v='.$_GET['v']); $download_link2 = $tube->get($_GET['v']); if($download_link) { echo '2. Right click <a href='.$download_link.'>this link</a>.'; } if(!($download_link2)) { echo '<BR>2. Right click <a href='.$download_link2.'>this link</a>.'; } else if { echo '<P>Error locating download URL.</p>'; } else { echo '<center><H1>Last 20 Downloads</H1></center>'; include('downloads.html'); } ?> This is much clearer for people to read and understand in my opinion. If you don't agree then that's fine, I'd try the suggestions I made above to get your code working. -
I have the following code in my htaccess file... RewriteCond %{REQUEST_URI} ^/clinics/dashboard/.* RewriteRule ^(.*)$ index.php?$1 [L] This works fine when I got to http://www.{myurl}.com/clinics/dashboard/ and redirects me to the correct controller. However, if I do http://www.{myurl}.com/clinics/dashboard then I get a 404 error. Regex isn't my strong point at all, so does anybody know how I could add a regular expression to look to see if the character after dashboard is either a / or whether it's the end of the string. I know that to find out if the next character isn't a / or a . is... RewriteCond %{REQUEST_URI} ^/clinics/dashboard[^/\.].* RewriteRule ^(.*)$ index.php?$1 [L] and that to match a / at the end of the url string is... RewriteCond %{REQUEST_URI} ^/clinics/dashboard[/$].* RewriteRule ^(.*)$ index.php?$1 [L] However, my limited regex knowledge has failed me and I can't seem to modify this to do match either a / at the end of the string or nothing at all, and trust me, I've googled my little heart out... Thanks in advance for your replies, they will be much appreciated.
-
Geocoding An Address To Get Coordinates For Google Maps API
GKWelding replied to sintax63's topic in PHP Coding Help
How are you passing the data to the google maps API? If you're using the cURL library or something similar Google will automatically send you back a response within the request. So for my script's it's as follows (excuse the pseudo code): $xml = curl_exec($data); // parse the string response from google $response = simplexml_load_string($xml); // response is now an object so output the required properties echo $response->lat; echo $response->long; -
In fact, I'm feeling so generous here's the modified function for you. function combineArrays($array1,$array2) { // this has been added in for testing $array1 = Array ( '0' => Array ( '0' => 0 ), '100' => Array ( '0' => 1 ), '105' => Array ( '0' => 1 ), '106' => Array ( '0' => 6 ) ); $array2 = Array ( '0' => Array ( '0' => 0 ), '100' => Array ( '0' => 2 ), '103' => Array ( '0' => 4 ), '104' => Array ( '0' => 1 ), '105' => Array ( '0' => 1 ) ); // get differences $arr1diffs = array_diff_key($array1,$array2); $arr2diffs = array_diff_key($array2,$array1); // new var $newArray = ''; // add unique keys to new array for both arrays foreach($arr1diffs as $key=>$val) { $newArray[$key] = $val['0']; unset($array1[$key],$key,$val); } foreach($arr2diffs as $key=>$val) { $newArray[$key] = $val['0']; unset($array1[$key],$key,$val); } // sum duplicate keys and add to new array foreach($array1 as $key=>$val) { $newArray[$key] = $val['0']+$array2[$key]['0']; unset($key,$val); } // tidy up unset($array1,$array2,$arr1diffs,$arr2diffs); // remove unwanted 0 element unset($newArray['0']); // return new array return $newArray; } which outputs... Array ( [106] => 6 [103] => 4 [104] => 1 [100] => 3 [105] => 2 ) I assume this was what you were trying to do?
-
look at php.net for array_diff_key and array_keys to do what you want. Use array_diff_key to find out the array keys that don't have duplicates, add them to a separate array, unset them as you do this. This way the original arrays now only contain matching array keys. loop through one array and add the values together. The code below works, and I have just tested it too so I'm sure you can modify it to work with multi-dimensional arrays. function combineArrays($array1,$array2) { // this has been added in for testing $array1 = array('0'=>'30','1'=>'10','5'=>'60'); $array2 = array('0'=>'3','2'=>'10','5'=>'6'); // get differences $arr1diffs = array_diff_key($array1,$array2); $arr2diffs = array_diff_key($array2,$array1); // new var $newArray = ''; // add unique keys to new array for both arrays foreach($arr1diffs as $key=>$val) { $newArray[$key] = $val; unset($array1[$key],$key,$val); } foreach($arr2diffs as $key=>$val) { $newArray[$key] = $val; unset($array1[$key],$key,$val); } // sum duplicate keys and add to new array foreach($array1 as $key=>$val) { $newArray[$key] = $val+$array2[$key]; unset($key,$val); } // tidy up unset($array1,$array2,$arr1diffs,$arr2diffs); // remove unwanted 0 element unset($newArray['0']); // return new array return $newArray; }
-
Geocoding An Address To Get Coordinates For Google Maps API
GKWelding replied to sintax63's topic in PHP Coding Help
As above. Sign up, get an API key, pass in the address string or whichever method you choose and get a return that will include long and lat. You can also choose the return type but I tend to parse it as XML. -
If the session data is saved as an unencrypted cookie then it is very easy to edit. If it's saved as an encrypted cookie or saved in a database then it is much more secure. However, please remember nothing is ever fool proof or completely safe. You have to take security on a per project basis. You need to make cracking your security protocols more effort that the data you hold is worth. For example, no point in using SSL certificates and https if the only thing your holding is food recipes.
-
2 seconds searching google gave me this, it has documentation, and an example. http://www.phpinsider.com/php/code/SmartyPaginate/
-
try this instead. $query = "SELECT h.login, h.forumnname, b.charactername FROM efed_handler AS h JOIN handler_characters AS c ON h.id = c.handler_id JOIN efed_bio AS b ON c.bio_id = b.id";
-
that old Malformed Headers problem again!!!!! HELP!!!!!!
GKWelding replied to funkychunky's topic in PHP Coding Help
can you paste the exact error message you receive? if it's blank then make sure you have error reporting level set to highest. -
Click counter to ignore traffic from search bots
GKWelding replied to HaLo2FrEeEk's topic in PHP Coding Help
code for your click counter might help? without seeing your code the below is just a suggestion. if(!eregi("Googlebot",$_SERVER['HTTP_USER_AGENT'])){ {code for doing count} } -
$path ios self explanatory, $filemode should be your chmod values in octal format, eg. 0755. function chmodr($path, $filemode) { if (!is_dir($path)) return chmod($path, $filemode); $dh = opendir($path); while (($file = readdir($dh)) !== false) { if($file != '.' && $file != '..') { $fullpath = $path.'/'.$file; if(is_link($fullpath)) return FALSE; elseif(!is_dir($fullpath) && !chmod($fullpath, $filemode)) return FALSE; elseif(!chmodr($fullpath, $filemode)) return FALSE; } } closedir($dh); if(chmod($path, $filemode)) return TRUE; else return FALSE; }
-
I honestly don't knwo what your scripts tryign to do, but a good place to start would be getting your jQuery right. $('username') is wrong. If you're trying to find an input box with the name attribute of username your should be doing this $("input[name=username]") or $("[name=username]"). Same for password etc...
-
You're missing your connection resource from your mysql_query. mysql_query should be done like this: $link = mysql_connect("localhost", "mysql_user", "mysql_password"); mysql_select_db("database", $link); $result = mysql_query("SELECT * FROM table1", $link); notice the link after the query. without that $result will not be a valid result resource and therefore mysql_num_rows will fail too.
-
Also, try just not posting then entire code, especially when it's unformatted and none specific, you're not going to get an answer to thsi question like this.
-
Unable to retreve the values from Mysql Query
GKWelding replied to surajkandukuri's topic in PHP Coding Help
just as a pointer, I knew the answer to this straight off, but if you didn't then try googling it first, I just googled mysql_fetch_array to test this and the first page back was http://php.net/manual/en/function.mysql-fetch-array.php which shows you exactly what I've just shown you above. -
Unable to retreve the values from Mysql Query
GKWelding replied to surajkandukuri's topic in PHP Coding Help
$sql_query= "Select Count(*) from (Select Cat_Num from cat_completiondate where `EmployeeNumber`=$employeenumber and `CategoryJobPosition` =$jobid) as temp"; $query_result2=mysql_query($sql_query); while($result_row=mysql_fetch_array($query_result2){ $value = $result_row['temp']; echo($value); } also, try using code tags. -
I was talking more about the method Gareth said, set the header type as csv or xls.