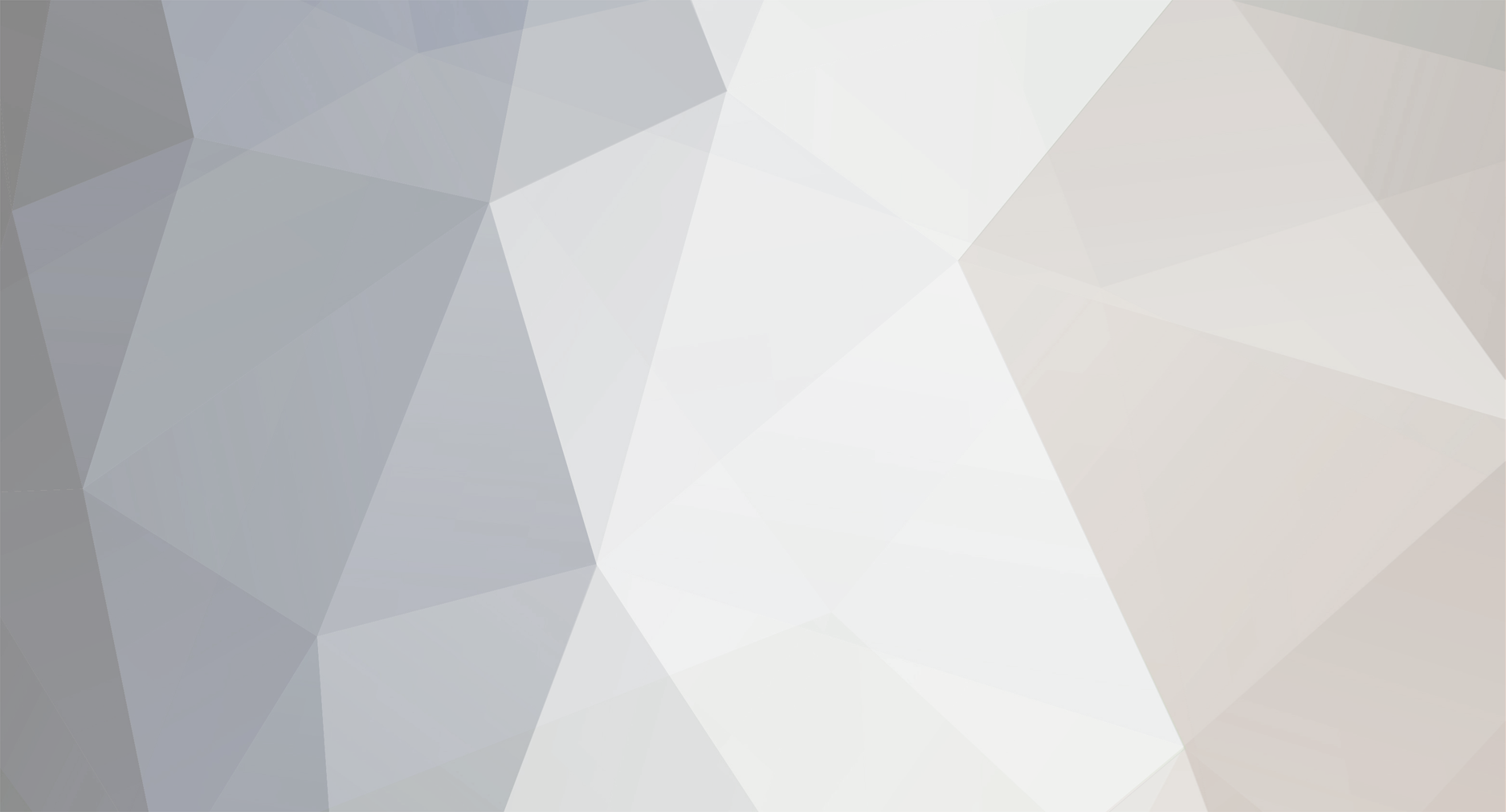
cpd
Members-
Posts
883 -
Joined
-
Last visited
-
Days Won
5
Everything posted by cpd
-
Excuse me if this is ridiculously simple but if I understand you right this is your database, your password, your username. Even if you've forgotten both username and password surely you can search for your first name, last name or email then write an UPDATE script encoding the password, as per Christians post, updating the row of your email/first name & last name/id; then log in with your new password...
-
Before Christians suggestion I'd validate the integer as intval() returns 0 on failure which could, in theory, give unexpected results. mysql_real_escape_string() is most definitely not sufficient security. In this case it shouldn't matter though as the integer shouldn't be wrapped in apostrophes. And to your last post Swarfega, no that's a bad idea. Casting is what you should be doing and is effectively what intval() does.
-
Just to echo Jessica's comments. You've done nothing anyone seems to have suggested and given little information about the problem. I've given you a direction to start with and you've not perused it at all... PFMaBiSmAd pointed stuff out and you just pasted more code without any output from your query. See the link in Jessica's signature "How To Ask Questions": read it, understand it, and if you don't like it don't ever expect a good reply.
-
Looping through an array setting child arrays in position
cpd replied to jkkenzie's topic in PHP Coding Help
It looks like an index naming convention gone wrong to me. What's generating this array? -
You have 2 conditions in your query which both have to be met for a result to be returned. MySQL won't decide to return something that doesn't match so the short answer is you have rows in your table that meet the criteria. By selecting the email and ok columns you'll prove this and be able to begin working out what rows match the conditions. This isn't a permanent thing, its just to get you to realise what's happening.
-
Run your current query but include: SELECT img_name, email, ok .... Dump the results and review what's been retrieved
-
Qu'est-ce que tu essaies de faire. Parler anglais s'il vous plaƮt.
-
I am getting this message and not sure how to fix it.
cpd replied to acarder488's topic in PHP Coding Help
Good man. Well done! -
I just wanted to give you a cleaner and slightly easier, in some respects, to understand method for doing this. Using the following classes I wrote in about 30-45 minutes you can easy get grades without any trouble. If something goes wrong it'll spit an error out at you which you can handle however you want. /** * A wrapper for a single grade. Requires upper and lower limits with which it * will compare a mark and determine if the mark is within the range. */ class Grade { /** * @var string The letter this grade represents */ private $letter = null; /** * @var int The lower limit of the grade */ private $lowerLimit = 0; /** * @var nit The upper limit of the grade */ private $upperLimit = 0; /** * Sets the grade, lower limit and upper limit boundaries. * * @param type $letter The grade letter * @param type $lowerLimit The lower grade limit * @param type $upperLimit The upper grade limit */ public function __construct($letter, $lowerLimit, $upperLimit) { $this->setLetter($letter); $this->setLowerLimit($lowerLimit); $this->setUpperLimit($upperLimit); } /** * Retrieves the grade letter * * @return string The grade letter */ public function getLetter() { return $this->letter; } /** * Determines if a mark is within this grade range. * * @param int|double $mark The mark to test against * @return boolean true if in range, else false. */ public function isInRange(Mark $mark) { if($mark->getValue() >= $this->lowerLimit && $mark->getValue() <= $this->upperLimit) { return true; } return false; } /** * Sets the grade letter * * @param string $letter The grade letter. Can be A+ or B- if you like. * @throws IllegalArgumentException */ private function setLetter($letter) { $this->letter = $letter; } /** * Sets the lower grade limit * * @param int $limit The lower grade limit * @throws IllegalArgumentException */ private function setLowerLimit($limit) { if(!is_numeric($limit)) { throw new InvalidArgumentException("Lower limit must be numeric"); } $this->lowerLimit = (int) $limit; } /** * Sets the upper grade limit * * @param int $limit The upper grade limit * @throws IllegalArgumentException */ private function setUpperLimit($limit) { if(!is_numeric($limit)) { throw new InvalidArgumentException("Upper limit must be numeric"); } $this->upperLimit = (int) $limit; } } /** * Mark represents a single mark received from 0 to 100. This class acts as a wrapper object. */ class Mark { /** * @var double The mark given */ private $mark = 0; /** * Performs validation and sets the mark * * @param int|double $mark The mark received * @throws IllegalArgumentException */ public function __construct($mark) { // Is the mark numeric? if(!is_numeric($mark)) { throw new InvalidArgumentException("Mark must be integer or double"); } // Cast it as a double $mark = (double) $mark; // Is the mark within the range if($mark < 0 || $mark > 100) { throw new InvalidArgumentException("A mark must be between 0 and 100"); } $this->mark = $mark; } /** * Retreives the mark * * @return double The mark */ public function getValue() { return $this->mark; } } /** * Grader will return a grade based on the available grades it is given */ class Grader { /** * * @var array An array of possible grades */ private $grades = array(); public function addGrade(Grade $grade) { $this->grades[] = $grade; return $this; } public function addGrades(array $grades) { // Cycles through the grades and attempts to add them foreach($grades as $grade) { if(!($grade instanceof Grade)) { throw new InvalidArgumentException("Grades must be of type Grade"); } $this->addGrade($grade); } } /** * Finds the approriate grade for the mark. * * The first instance of a satisfactory grade will be returned. This means if * there is an overlap between limits in grades the first grade to of been added * via the addGrades() or addGrade() methods will be returned. * * * @param Mark $mark The mark received * @return Grade|null A Grade object if found with satisfactory parametes. If nothing is found null is returned. */ public function getGrade(Mark $mark) { foreach($this->grades as $grade) { if($grade->isInRange($mark)) { return $grade; } } return null; } } You can then implement the following code to use it. If you don't care for how it functions ignore the above and just review what's below. It has been tested and works quite well as is but there's always improvements to be made. // Has the form been submitted? if(isset($_POST['submissionButtonName'])) { // Create a grader and add marks to it. $grader = new Grader(); $grader->addGrade(new Grade("A+", 96, 100))->addGrade(new Grade("A", 92, 95))->addGrade(new Grade("A-", 88, 91)); try { // Create a mark object using the $_POST data. // The post field name used here should be the same as the one used to enter the mark $mark = new Mark($_POST['mark']); // Was a grade found? If so echo it else echo a message saying we couldnt find a grade based on the available grades. if(($grade = $grader->getGrade($mark)) === null) { echo "Could not find a grade"; } else { echo $grade->getLetter(); } } catch(InvalidArgumentException $e) { // Change how you handle the error... var_dump($e->getMessage()); } } ?> <html> <body> <form method="post"> <input type="text" name="mark" /> <input type="submit" name="submissionButtonName" value="Mark" /> </form> </body> </html> I just thought another view on it would be helpful as I ranted before about how I disliked the code.
-
I am getting this message and not sure how to fix it.
cpd replied to acarder488's topic in PHP Coding Help
With all due respect, can you apply a bit of common sense and look at your configuration as I pointed out before. I gave you the answer once, ChristianF has pointed you in the right direction, now figure it out for yourself. -
Just wanted to echo salathe's comments: learn both. Ultimately you're going to pick up generic programming skills understanding control flow/loops etc so it really doesn't matter. Both are scripting languages and aren't strongly typed so learn both.
-
I couldn't agree more with KevinM1. Which one you choose to learn (first) is completely dependent on your goal. There's no reason why you can't learn C++ first; It inherits everything from C. People seem to think C -> C++ because its a "step up" but in reality its not, its two different languages one being a superset of the other.
-
I can spot a load of things that are unnecessary or can be done a whole lot better in that, sorry BagoZonde. For a start the if($_POST) { doesn't test if the form was submitted. The $_POST superglobal is generated by PHP regardless of whether POST data was submitted or not so this isn't a true test for a form submission. If you both bother looking and synthesising my previous post you'll see pointers on how to improve your code. If you don't bother synthesising it you are wasting my, and anybody else who posts, time. If you don't understand something, bloody well ask.
-
From looking at the code I can see your control flow is a little ski-wiff. Lets work through this systematically: I can't see in your mark-up where you've defined a "reset" button and even if you had there's no need to test for it in PHP as it won't refresh the page. To test if something's submitted use isset($_POST['submitButtonName'] Instead of setting each variable as you've done, use extract($_POST), it'll save a lot of time. You query if the value is_numeric() in ever if statement, why not have a single if statement wrapping all other logic that requires is_numeric. This is far better control flow. Consider using range() to determine if a grade is within a range: if(in_array($finalgrade, range(0, 59))) { Use the HTML Entity code for plus and minus: plus = + and minus = + If the problem persists use the Regex to test if the input is an integer: if(preg_match('/[0-9]{1,3}/', $finalgrade)) { // Code omitted }
-
You would need to store the key value pair somewhere else. I would then use the usort() function to make a comparison and have PHP sort it. For example, if you rework the array to form another array in the format array(0 => "key:value", 1 => "key:value") you can use: usort($array, function($a, $B) { $a = explode(":", $a); $b = explode(":", $B); if($a[1] < $b[1]) { return -1; } elseif($a[1] == $b[1]) { return 0; } else { return 1; } });
-
If the code above is your actual code you could of used the sort() function; I appreciate you said you can't but never the less. To print the code use: foreach($arr as $k => $v) echo $k . " => " . $v . "<br />"; This sentence makes absolutely no sense to me.
-
Exceptions are generally used when something unexpected occurs. The to throw an exception you've defined yourself you must extend the Exception class, if it doesn't you'll get a fatal error. A typical exception you could throw is an InvalidArgumentException which could be used when a variable is of an incorrect type. Personally I've never used the Exception class on its own as I find it a little to broad and I certainly wouldn't use any exception class for all exceptions. Where you log errors is entirely up to you. People often used text files. You can write a logging class which could be dependency injected into any class that needs it.
-
I am getting this message and not sure how to fix it.
cpd replied to acarder488's topic in PHP Coding Help
You're trying to access the variables as if they are constants. E.g. define("DB_USER", "MyDatabaseUsername"); When you've actually defined the configuration as an array. To use your current set-up you should do: mysqli_connect($config['DB_HOST'], $config['DB_USER'], $config['DB_PASS']); -
HI, nice to have you here. One thing though: Drupal is a CMS, with its next release having some of the Symfony2 components, not a framework. Symfony2 on the other hand is a framework although I may have misinterpreted what you meant by "framework" .
-
Consider "building" the string instead of writing it all in one go. $row = '<tr> <td>'.$date_added.'</a></td> <td>'.$title.'</a></td> <td><a href="view.php?id='.$id.'">View Details</a></td>'; if($c->is_admin == 'Yes') { $row.= '<td><a href="update.php?id='.$id.'">Update</a></td>'; } $row.= '<td><a href="delete.php?id='.$id.'">Delete</a></td></tr>'; The $row.= means append to the variable.
-
You can convert the encoding using http://php.net/mb_convert_encoding . If you use this in your get_content.php page all should be well. I've noticed you don't sanitise or prepare your SQL before execution. Consider using the mysqli_prepare function as opposed to just running a query. In its current state you are open to injection.
-
I am getting this message and not sure how to fix it.
cpd replied to acarder488's topic in PHP Coding Help
Your insert() function uses a variable named $type but doesn't define $type anywhere. You then use an if statement after setting the $query variable that has no definition rendering it pointless. By the look of it you need to research "variable scope" because it appears as though you're not sure what it is. -
Playing all images from folder, with pause in between
cpd replied to TheDutchBeast's topic in PHP Coding Help
Google should give you a nice solution. There is a jQuery "Cycle" plugin found at http://jquery.malsup.com/cycle/ which you could use. There are loads available, find one that suits you and implement it A Mod may move the topic to Javascript help. -
Your mark-up looks wrong as you have no list item tags in your sub unordered list tags. If you can't be bothered understanding the script just use google to find another. Its a waste of everybodies time explaining what's wrong.
-
What do you understand about the script above specifically?