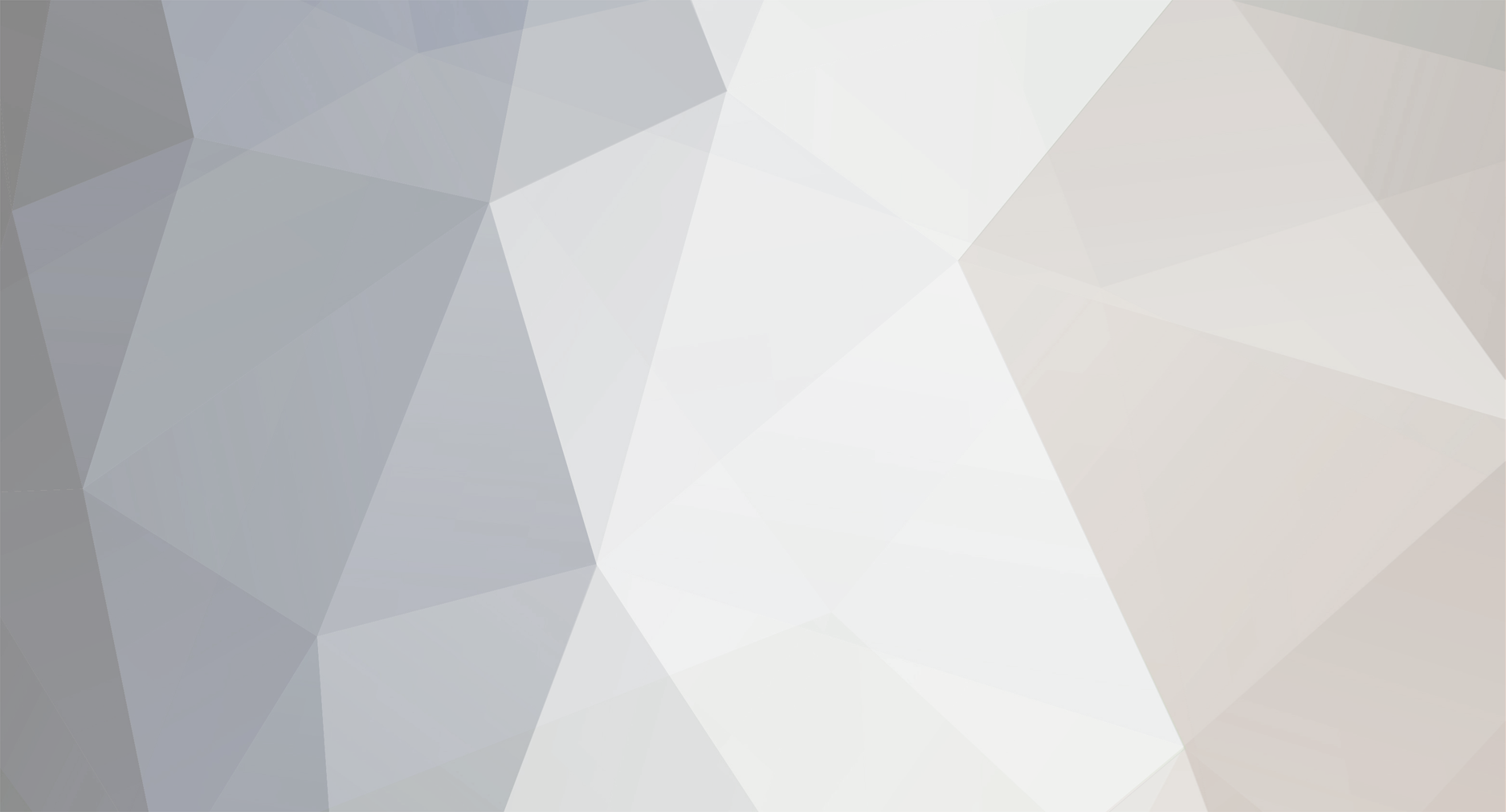
soak
Members-
Posts
219 -
Joined
-
Last visited
Never
Everything posted by soak
-
Can you please post the code snippet that renders the select box?
-
@AE117 that won't matter at all. $search= trim($search); is fine.
-
I don't see how trim could possibly be doing that. Try var_dump($search); after you've trimmed it, pretty sure it must be coming later in your code.
-
The flash file will be able to read the content but I would expect it will require valid xml. Your browser is displaying the error message. Whether it can parse that content is a question that probably only you can answer.
-
And a view source shows: <song path='16' title='95 Ford Prode GT'/> <song path='17' title='1997 Eagle Talon'/> <song path='18' title='1997 Eagle Talon'/> <song path='19' title='300zx Twin Turbo vs ZX10R'/> Which implies that you've somehow lost the lines that echo the <player ...> and </player>.
-
Can you post the first 4 lines of the resulting XML file please? View source in your browser should let you see it.
-
You're checking to see if a $_GET submit button was clicked and your insert is expecting $_POST data. The only way this would work is if your form has an action of "?submit=blah". Also, if $_GET['submit'] is set it will be a string so strictly speaking will never === true. If you've set the submit button value to "true" then you ought to check for $_GET['submit'] == "true".
-
You can't just blindly copy what jesushax wrote, read the comments next to the closing brackets.
-
You could display a random page as the first page displayed to the user but again that's not ideal. TBH, if it were me I think I would just start with the A's as you are doing at the moment. It's what the user would expect, they will expect the results to be in order (probably alpha) and that the first page will be displayed first.
-
It's not really ideal because you're querying the order_line table for every order that you do. You could get all of that from one query if you need to. Say you only need id and name from the main orders table and id, productname, cost from the line items table: $order = $db->getAssoc('SELECT o.id, o.name, oli.id as line_id, oli.productname, oli.cost FROM orders AS o INNER JOIN order_line_item AS oli ON oli.order_id = o.id'); You may have a bit more work sorting it when it comes out though. Also, you'll have o.id and o.name for each of your order lines. A couple of critiques: Select * is "bad". If possible list just the fields that you need in your SQL query. This may make your results array smaller and will ensure that you get exactly the fields that you were expecting. Do you use the whole array that is returned (i.e. do you display all of it at once)? If not then you ought to add a limit clause to your select query and just grab the data that you need. Add some pagination and incorporate that with your select and limit clause. Another way to do it would be to keep your first query as is and get the data for your second query all at once, indexing it by order id. This way you could use just 2 queries and get the same data that you do at the moment. Saying that though I assume that you aren't getting all the data from the table as in your example query and that you are limiting it in some way so this may not be possible.
-
All I've done is indented it properly to make it easier to read and moved the closing bracket at line 55 to the end of the code. It all looks about right but I've not tested obviously. <?php function pf_validate_number($value, $function, $redirect) { if(isset($value) == TRUE) { if(is_numeric($value) == FALSE) { $error = 1; } if($error == 1) { header("Location: " . $redirect); } else { $final = $value; } } else { if($function == 'redirect') { header("Location: " . $redirect); } if($function == "value") { $final = 0; } } return $final; } function showcart() { if($_SESSION['SESS_ORDERNUM']) { $custsql = "SELECT id, status from orders WHERE customer_id = " . $_SESSION['SESS_USERID'] . " AND status < 2;"; $custres = mysql_query($custsql); $custrow = mysql_fetch_assoc($custres); $itemssql = "SELECT products.*, orderitems.*, orderitems.id AS itemid FROM products, orderitems WHERE orderitems.product_id = products.id AND order_id = " . $custrow['id']; $itemsres = mysql_query($itemssql); $itemnumrows = mysql_num_rows($itemsres); } else { $custsql = "SELECT id, status from orders WHERE session = '" . session_id() . "' AND status < 2;"; $custres = mysql_query($custsql); $custrow = mysql_fetch_assoc($custres); $itemssql = "SELECT products.*, orderitems.*, orderitems.id AS itemid FROM products, orderitems WHERE orderitems.product_id = products.id AND order_id = " . $custrow['id']; $itemsres = mysql_query($itemssql); $itemnumrows = mysql_num_rows($itemsres); // If no SESS_ORDERNUM variable is available, the $itemnumrows variable is set to 0: $itemnumrows = mysql_num_rows($itemsres); } if(isset($itemnumrows) && $itemnumrows == 0) { echo "You have not added anything to your shopping cart yet."; } else { echo "<table cellpadding='10'>"; echo "<tr>"; echo "<td></td>"; echo "<td><strong>Item</strong></td>"; echo "<td><strong>Quantity</strong></td>"; echo "<td><strong>Unit Price</strong></td>"; echo "<td><strong>Total Price</strong></td>"; echo "<td></td>"; echo "</tr>"; while($itemsrow = mysql_fetch_assoc($itemsres)) { $quantitytotal = $itemsrow['price'] * $itemsrow['quantity']; echo "<tr>"; if(empty($itemsrow['image'])) { echo "<td><img src='./productimages/dummy.jpg' width='50' alt='" . $itemsrow['name'] . "'></td>"; } else { echo "<td><img src='./productimages/" . $itemsrow['image'] . "' width='50' alt='" . $itemsrow['name'] . "'></td>"; } echo "<td>" . $itemsrow['name'] . "</td>"; echo "<td>" . $itemsrow['quantity'] . "</td>"; echo "<td><strong>£" . sprintf('%.2f', $itemsrow['price']) . "</strong></td>"; echo "<td><strong>£" . sprintf('%.2f', $quantitytotal) . "</strong></td>"; echo "<td>[<a href='" . $config_basedir . "delete.php?id=" . $itemsrow['itemid'] . "'>X</a>]</td>"; echo "</tr>"; $total = $total + $quantitytotal; $totalsql = "UPDATE orders SET total = " . $total . " WHERE id = " . $_SESSION['SESS_ORDERNUM']; $totalres = mysql_query($totalsql); } echo "<tr>"; echo "<td></td>"; echo "<td></td>"; echo "<td></td>"; echo "<td>TOTAL</td>"; echo "<td><strong>£" . sprintf('%.2f', $total) . "</strong></td>"; echo "<td></td>"; echo "</tr>"; echo "</table>"; echo "<p><a href='checkout-address.php'>Go to the checkout</a></p>"; } }
-
Just a quick note, to get an array returned as the post data set the name field on each of your checkboxes like name="NAME[]" where NAME is the name of the field. The square brackets should make $_POST[NAME] return an array (note that you should still sanity check it before attempting processing in case none are selected). Just seen your update, like this: echo "<tr><td>".$id."</td><td>".$name."</td><td>".$subject."</td>"; echo "<td><input type='checkbox' name='whatever[]' value=".$id."/></td></tr>";
-
The showcart function stops at line 55. The rest of the code below that is being executed when you include that page and not just when you call that function.
-
I just wrote out a lengthy way to work around this and realised that you don't need to at all. When you perform update number 2 the `type` field for update number 1 will already have been updated from 'none' to 'Farm' so it won't be possible to overwrite your new rows.
-
I suspect it may be content after the last ?> in your included header page or your classes. If so, either strip the excess or do what I do and remove the last ?> completely. PHP will work fine without it and that way you NEVER get any extraneous spaces appearing.
-
Looking over your code there are a few places where things could be going wrong. Does it give you any sort of error message? If not pop these two lines at the very top of your file and try again: ini_set('display_errors', 'On'); error_reporting(E_ALL);
-
I think youi're nearly there. How about something like this: It's not the prettiest code but it should do what you need. <?php require_once("includes/connection.php"); require_once("includes/functions.php"); include("includes/header.php"); $query = "SELECT key_id, words FROM keywords"; $result = mysql_query($query); confirm_query($result); $options = array(); $number_of_uploads = 3; // Initialise your options strings for ($i = 1; $i <= $number_of_uploads; $i++) { $options[$i] = ''; } // build the checkboxes while($row = mysql_fetch_array($result)){ for ($i = 1; $i <= $number_of_uploads; $i++) { $options[$i] .= $row[1] . ':<input type="checkbox" value="'.$row[0].'" name="section'.$i.'[]]" />'; } } // now echo the form to the screen for ($i = 1; $i <= $number_of_uploads; $i++) { ?> <p> <b>Image:</b> <textarea name="title<?= $i ?>" cols="30" rows="1"></textarea> </p> <p> <b>Sections:</b> <?php echo $options[$i] ;?> </p> <?php } You can recover the data from the form using: <?php $sections = array(); $titles = array(); for ($i = 1; $i <= $number_of_uploads; $i++) { $sections[$i] = $_POST['section'.$i]; $titles[$i] = $_POST['title'.$i]; } I hope that all makes sense! -- A digital agency