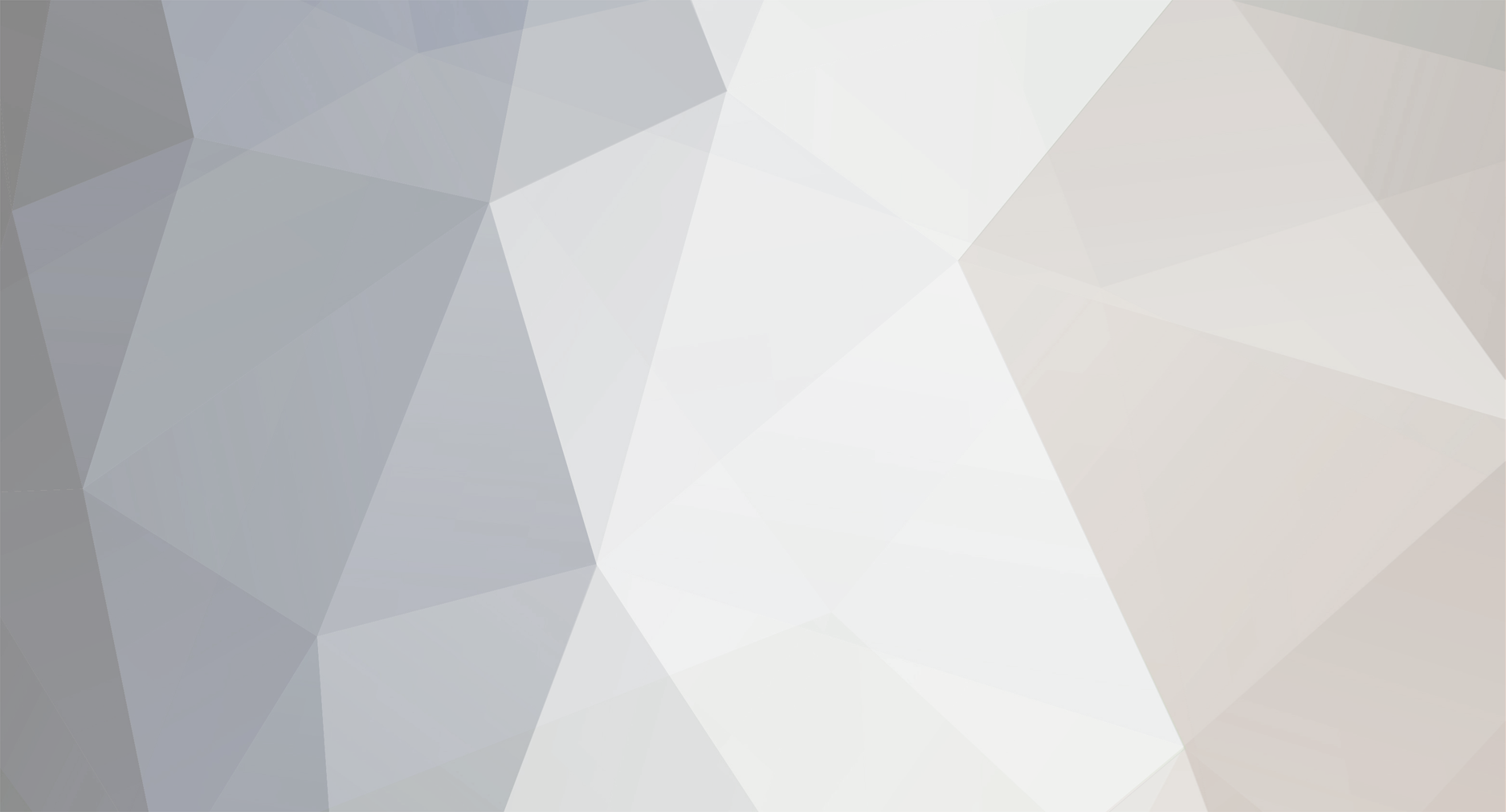
ialsoagree
Members-
Posts
406 -
Joined
-
Last visited
Everything posted by ialsoagree
-
[SOLVED] Copying MySQL Table Data To Another Server
ialsoagree replied to Monadoxin's topic in PHP Coding Help
Is it possible to create a mysql connection to both databases from the same PHP script? -
When given the year, if you've checked and know that the booking spans more than one moth, I would do something like this: $end_of_month = mktime(11, 59, 59, $month, $last_day_in_month, $year); // get the unix timestamp for the end of the month $start_of_booking_in_month = mktime(0, 0, 0, $month, $day_booking_starts, $year); // get the unix timestamp for the start of booking $time_in_first_month = $end_of_month - $start_of_booking_in_month; // get the seconds between the end of the month and the start of booking $days_in_first_month = ceil($time_in_first_month / 60 / 60 / 24); // convert the seconds to days rounding fractions up With a little innovation you can figure out how to do this over multiple months using a loop. To get you started, you'll want to do something like this: $what_year = $year; $next_month = $month; // You could set this to the previous month (checking to see if you need to subtract a year) which would allow you to process // the entire booking inside of the loop below, just remember that inside the loop, you're going to have to check if the booking starts in the middle // of the month, or if starts at the beginning of the month. if ($month == 12) { $next_month = 1; $what_year = $year+1; } else $next_month++; // If $end_of_booking is a UNIX timestamp while ($end_of_booking >= time(0, 0, 0, 1, $next_month, $what_year)) { /* Process the payment at next month's rate, you can use the above code to help you with this keep in mind though - the above works specifically for the 1st month in the booking, you'll have to to check to see if the booking ends during the "$next_month" variable or if it goes past it. If it goes past just process from the begining of $next_month to the end of $next_month, otherwise process from the beginning of $next_month to the end of the booking. */ if ($next_month == 12) { $next_month = 1; $what_year = $what_year+1; } else $next_month++; } Hope that helps.
-
[SOLVED] Using While Statement to Insert numbers
ialsoagree replied to Colton.Wagner's topic in PHP Coding Help
Is this the entire code? If nothing it happening when the code is run you should almost certainly be getting an error message. Have you tried using view source to see what if any HTML is written to the browser? -
[SOLVED] Using While Statement to Insert numbers
ialsoagree replied to Colton.Wagner's topic in PHP Coding Help
Having trouble remembering, doesn't a MySQL error need to be reported explicitly? In other words, if you tried to insert a non-unique value into a unique column, wouldn't you need to report the returned MySQL error explicitly in PHP? -
$apps_html.= '<td><a href="window.html" onclick="popUp(this.href,'200','400'); return false;"></a></td>'; should be $apps_html.= '<td><a href="window.html" onclick="popUp(this.href,\'200\',\'400\'); return false;"></a></td>'; Have to escape the single quotes or they tell PHP to end the string.
-
Just so you're clear, AJAX doesn't load things "all at once." AJAX uses HTTP and loads things on demand. AJAX is stateless, like all HTTP requests. If you have an array and you want to get access to it again, the simplest way would be to save it in a session variable if you're using sessions. You could save it into a cookie but it would be very easy for the user to manipulate. You could save it into a database, but since you need a way to identify the user this wouldn't be much better than just using a session.
-
[SOLVED] Accessing methods of objects in an array
ialsoagree replied to Anzeo's topic in PHP Coding Help
The code you have written implies that "array" is a property of a class, and the use of "$this" implies that you are inside that class. If this is wrong, then that's your problem. If it is right, are you sure that array[1] has been defined as a new class? -
Corbin, the problem is that you're comparing 1 number to the list, and not every number in the list to every other number in the list. So in a group of 50 random numbers, we're not looking at the probability that a single given number will match the other numbers in the set, we're looking at the probability that any number will be the same as any other number in the set. If we talk about it as pairings, you're creating 49 pairs (1 number compared to each of the 49 others). But in reality, there's 1225 pairs (50*49/2). The pairs aren't statistically independent, but it gives you some perspective as to the fact that you can't simply compare 1 number to the entire set.
-
Would be pretty helpful if we could see the HTML too.
-
[SOLVED] Embedding flash object in Else statement
ialsoagree replied to zero742's topic in PHP Coding Help
This appears to be a code snippet rather than the whole page (I'm basing this off the fact that you're ending your code with multiple brackets). Is it possible that you have an if condition that could be met but wouldn't produce output, or is it possible that you have an if condition that doesn't have an else? Checking the HTML source might provide a clue as to the problem. -
Because variables will interpolate within double quotes so you don't have to use concatenation. This way is cleaner and faster (AFAIK). I actually prefer this format: echo "<a href='view-listing.php?contact_id={$row['contact_id']}'>stripslashes({$row['business_name']})</a><br/>"; Except that he already concatenated his string as I point out in the post you quoted. Its wasting processing cycles.
-
That'd work, although the += and -= shouldn't be necessary. Also, this is the type of thing that may look a little weird. IE. if I start on page 1 I can see page 5, but once I get to page 5, I can not only see page 1, but I can see page 9 as well. Kinda makes you wonder why you couldn't see page 9 from page 1. But, that's just an issue of convenience.
-
[SOLVED] Embedding flash object in Else statement
ialsoagree replied to zero742's topic in PHP Coding Help
I guess it's good that I said the exact same thing then? "Instead, you need to ... change them back to double quotes ... so PHP knows the difference:" EDIT: Show should be print or echo? -
The anchor tag is not self closing: <a href="some link" /> This is invalid HTML! Anchor tag does not close itself! <a href="some link">Link text</a> This is correct HTML. Anchor tag needs a closing tag. By the way, if you're using double quotes in PHP and then concatenating your variables to them, why use double quotes at all? Double quotes tell PHP to parse the string for PHP variables, classes, etc. If you remove all those variables and classes from the string, then you're telling PHP to parse a string that doesn't have anything to parse in it! You're wasting processing cycles parsing a string that you already know has nothing to parse because you've explicitly removed all the PHP from those strings! Use single quotes instead, it saves processing time!
-
This is a complex problem and there's a few solutions depending on what you want to accomplish. What you should immediately realize is that what pages get displayed needs to depend on what page you're on, and how many you have. But it's not that straight forward. Users need to go back as much as they need to go forward. So displaying 2-11 when you're on page 2 isn't useful. I can't get back to page 1! Instead, you need to define the range of pages that will be shown. So, for example, you might show the next 9 pages and the previous 9 pages. Then you have to validate that those pages exist. Then you have to decide what you want to do with "left over" space. Case in point, should page 1 only show the next 9 pages where as page 10 will show pages 1-20? Or should page 1 recognize that the 9 previous pages aren't being used, and switch them to next pages instead. So that page 1 also shows pages 1-20 (or at least 1-19) instead of just showing pages 1-10. If you don't recycle left over room, by the time a user gets to page 10 they can see twice as many pages as they could from page 1 (including page 1). Creates a bit of a weird feel when clicking on page 2 suddenly shows page 11, clicking page 3 suddenly shows page 12 etc. Makes the user wonder why pages 11 and 12 weren't just shown in the first place.
-
[SOLVED] Embedding flash object in Else statement
ialsoagree replied to zero742's topic in PHP Coding Help
You're getting the same error because you replace ALL the double quotes with single quotes. To give you a clue as to what's going on: PHP recognizes all of this normally: print '<object codebase=' PHP throws an error when it sees this: http://download.macromedia.com/pub/shockwave/cabs/flash/swflash.cab#version=9,0,0,0 It's not a string (you've closed your single quote, so PHP doesn't recognize it as being in the string), you didn't end your line with a semicolon, and you didn't concatenate to your string. Thus, it's a syntax error, PHP throws an error. This would be another string, but once again since it's not concatenated, it would result in a PHP parse error: 'doc_804151193636402' Now your outside the string again: name= Now you're inside the string again: 'doc_804151193636402' etc. Instead, you need to escape your single quotes (or change them back to double quotes) so PHP knows the difference: <?php print '<object codebase=\'http://download.macromedia.com/pub/shockwave/cabs/flash/swflash.cab#version=9,0,0,0\' id=\'doc_804151193636402\' name=\'doc_804151193636402\' classid=\'clsid:d27cdb6e-ae6d-11cf-96b8-444553540000\' align=\'middle\' height=\'500\' width=\'100%\' >'; ?> -
[SOLVED] Embedding flash object in Else statement
ialsoagree replied to zero742's topic in PHP Coding Help
Also, if you have no PHP written in your strings, you shouldn't be using double quotes. Double quotes tell PHP to parse the string for PHP syntax (IE. look for and interpret variables, functions, etc.). This uses processing cycles. If you know before hand that there's no PHP in the strings, why waste processing cycles processing PHP where there is none? I've personally abandoned the use of double quotes simply because it makes PHP programming as a whole easier for me. I always know that variables and functions have to be concatenated to the string and that single quotes have to be escaped. I also never waste processing cycles parsing a string for PHP that doesn't have any PHP in it. -
You say you hit the "GET DATA" button and nothing happens. What I think you mean is that you hit the "GET DATA" button and you're taken to the page you're already at. This is a logic error in your program as this is what you've written it to do: $extract = mysql_query ("SELECT * FROM cy_equ WHERE udf = '$udf_form' LIMIT 1 "); The "LIMIT 1" tells MySQL only to ever return 1 result set. If you're looking to get more than one result set, you can't use the limit. In addition, removing the limit isn't going to be enough: <?php while ($row = mysql_fetch_assoc($extract)) { $id = $row['id']; $equ = $row['equ']; $ext = $row['ext']; $udf = $row['udf']; $udf2 = $row['udf2']; $udf3 = $row['udf3']; }?> On each iteration of this loop, all the data from one result is replaced by the next result. When the 1st result is processed (currently only 1 result can ever be returned), $id is equal to the 1st results id data, $equ is equal to the equ data etc. However, on the 2nd iteration (for the 2nd result) those same variables, $id, $equ, etc. are set to the 2nd results data (and the 1st result is lost). This will repeat until it gets to the last result. In the end, every result prior to the last one isn't saved. But even if you were saving all the data from every result, your HTML is only outputting one set of data: <div class="boxextqry"> <h3> UDF Query </h3> <form name="form1" method="post" action="../index1.php?page=udfsearch"> <p> Input UDF: <input type="number" name="udf" size="1" maxlength="4"/></p><br> <p> ID = <font color="red"><?php echo $id; ?> </font> </p> <p> EXT = <font color="red"><?php echo $ext; ?> </font> </p> <p> EQU = <font color="red"><?php echo $equ; ?> </font></p> <p> UDF = <font color="red"><?php echo $udf; ?> </font></p> <p> UDF2 = <font color="red"><?php echo $udf2; ?> </font></p> <p> UDF3 = <font color="red"><?php echo $udf3; ?> </font></p> <p><input type="submit" name="submit" value="GET DATA"/></p> <input type="hidden" name="udf" value= "<?php echo $udf_form; ?>" /> <p><input type="submit" name="next" value="NEXT"/></p> <p><input type="submit" name="prev" value="PREV"/></p> </form> </div> All your HTML happens exactly 1 time and therefor can only output 1 set of data no matter how many you collect and processes. You would have to make a loop with this HTML inside it to display each result. Truth be told, it's hard to tell exactly what you're trying to do here because it's not thoroughly planned out. Your program is doing exactly what you've told it to. There doesn't appear to be an error with the syntax, it's a logic error in the program. That is, you need to think more about what you're trying to accomplish and the steps you need to take in order to accomplish it. If you provide a more detailed idea of what you're trying to do it may be possible to explain where your code has gone wrong.
-
As I don't plan to download the zip file and do your homework for you, if you throw the codes I requested up I can point you in the right direction (maybe even give an example or two if there's a lot of work).
-
You're improperly concatenating the string: <?php echo '<p class="error">'$error'</p>'; // this throws an unexpected variable error ?> ...should be... <?php echo '<p class="error">'.$error.'</p>'; ?>
-
As for number 6, that's pretty easy. If you don't know how to do it, you might want to read up on the PHP's manual entry for Class Basics. As for 7 and 8, can you post the Customer class using code tags as well as the BikeSite class and BikeSiteTester.php file?
-
Wanted to edit this in but couldn't: http://en.wikipedia.org/wiki/Birthday_attack With 4.3B possibilities (yes, yours has more, but this gives you an idea) it only takes 110,000 entries before a truly random generator has a 75% chance of producing duplicates. That is, once you've used a mere 0.26% of the possibilities (out of 4.3 billion), 3/4 of all randomly generated numbers will be duplicates. Attempting to generate the barcodes randomly is going to be a time consuming process. On the scale you need them, it's unreasonable to do in real time.
-
I agree, I also stand by the idea that there's absolutely no reason to generate these barcodes when they're needed. Everything will run faster and smoother if they're generated before hand. Unlike your typical PHP request, it doesn't matter who, when, or where the request is coming from. The need for a unique barcode is exactly the same. Do the work BEFORE the request comes in and you save the end user time.
-
This would be my suggestion for a solution. Generate ~50000 unique barcodes and put them into a database table. Have MySQL remove from that table every barcode already in use. Have a CRON run a script every few seconds that checks how many unique barcodes are in the table and, if it is lower than 50,000, attempts to create 1 new barcode once. If the script ran once a second you would have 86,400 attempts to generate a new barcode per day. If you need more attempts, you could have the CRON run, say, once a minute, and have the PHP script try to generate 1 new bar code until it times out. Any kind of combination there in that works for your system.
-
I'm not so sure that will work either. If he needs to generate thousands of unique ID's in a single request, you're going to time out long before you come up with a fraction of the solution. As I said before, my approach does create size issues, but provides a computationally possible solution. The scale of your problem demands minimal processing time. The only way to minimize the processing time is to guarantee a correct result every time you generate a barcode. The only way to guarantee a correct barcode upon request is to draw the barcodes from a list that only contains valid barcodes. If you try to generate thousands of unique barcodes randomly that have never been used, there's no way you're ever going to generate them before PHP times out. I think it's also worth pointing out that my solution becomes FASTER the more bar codes you have. Other solutions will become SLOWER the more bar codes you have. Further, if you're only going to need 5M barcodes total, you can reduce the size by only including a list of 5M unique barcodes and then removing from it those barcodes already in use. Other solutions don't take advantage of this fact and utilize the complete list of barcodes while trying to quickly generate thousands of both random and unique barcodes - a task PHP is never going to be able to complete. Take this a step further, my solution focuses on limiting the pool of random barcodes you generate to a set that only contains valid barcodes. You can use this to your advantage in multiple ways to reduce the size of the pool. For example, only store, say, 10,000 unique barcodes (that aren't in use). Whenever a barcode is used, you can let the server do background tasks to generate a new unique random barcode and add it to the list. This way, you have immediate access to unique barcodes that are random (and you're not waiting for them to be generated), you can reduce the size of the table containing those unique values, and you can utilize the servers downtime to populate that list instead of trying to do it right when the user requests.