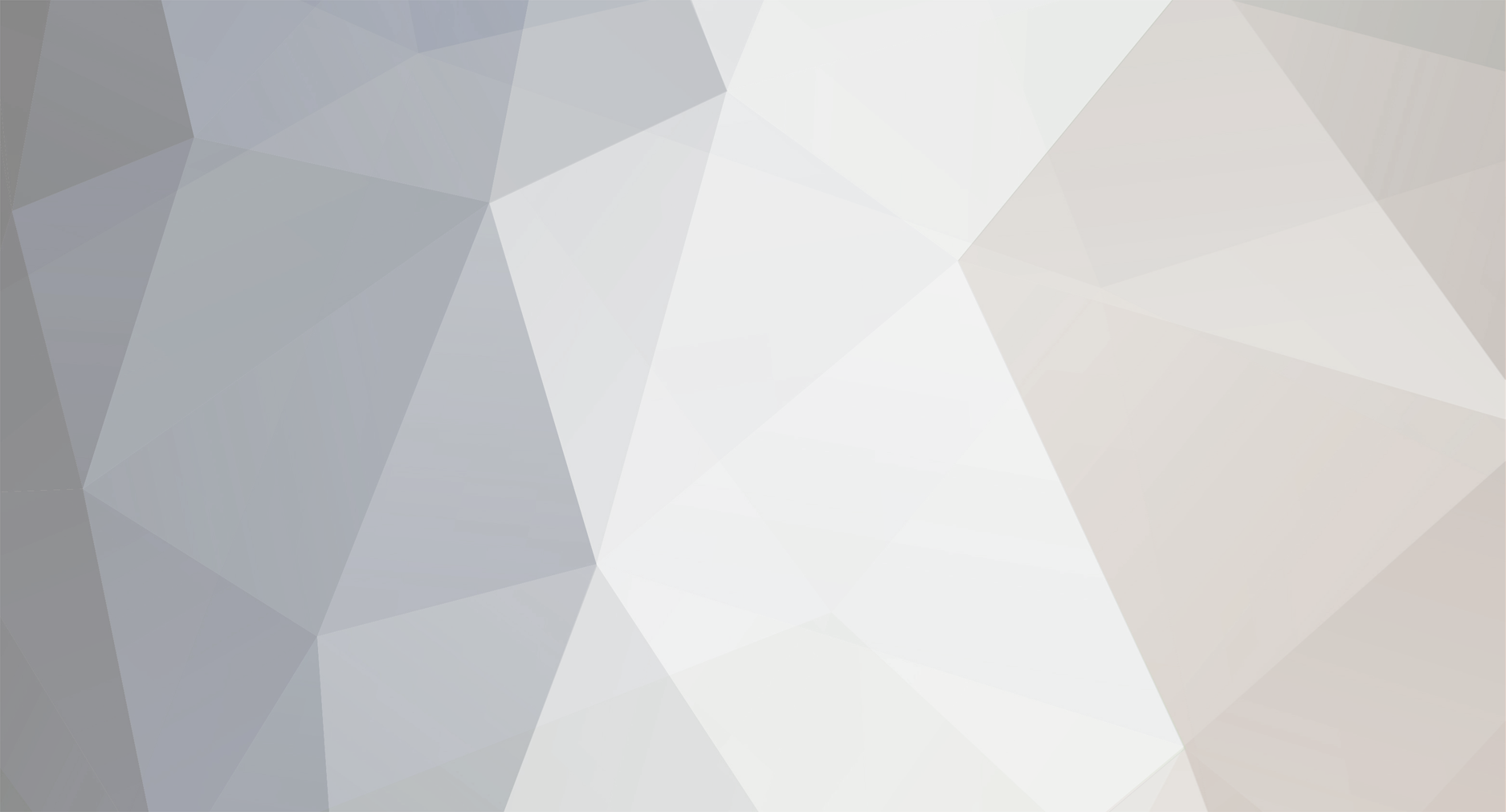
ialsoagree
Members-
Posts
406 -
Joined
-
Last visited
Everything posted by ialsoagree
-
In the final code you posted, are the variables you're using ($start, etc.) defined? Should they be $_POST variables instead?
-
[SOLVED] Help With Showing Users On the Index Page
ialsoagree replied to shorty3's topic in PHP Coding Help
Without knowing the structure of your database, there isn't an exact solution anyone can give you. You're selecting all the columns from the table, so perhaps something like this will work: <?php $start = TRUE; while ($online_array = mysql_fetch_assoc($select)) { if ($start) { echo $online_array['username']; $start = FALSE; } else echo ', '.$online_array['username']; } echo ' were online recently'; ?> -
Just took a quick glance but... <?php $query = 'INSERT INTO users (user_id, username, password) VALUES ("", "$username", "$password")'; ?> Your variables are inside single quotes, therefor they're not being parsed by PHP as variables and instead are being sent as literal text. Instead, you should be using: <?php $query = 'INSERT INTO users (user_id, username, password) VALUES ("", "'.$username.'", "'.$password.'")'; ?> (Notice how the variables appear in red above - literal text - and blue here - PHP variables?) You might also want to notice that you're submitting the user_id as "", if user_id is being set by automatically by the database, you shouldn't submit a value for the user_id at all, simply skip it in the first and second list. You propagate this error further: <?php $query = 'INSERT INTO users_details (user_id, username, first_name, last_name, email, city, state) VALUES ("$user_id","$username", "$first_name", "$last_name", "$email", "$city", "$state") '; ?>
-
Can you be more specific about the problem you're having?
-
Read up on preg_replace: http://us.php.net/preg_replace Subjects must be strings, but the patterns and replaces can be arrays.
-
You could try splitting it into an array of strings. It may or may not work, I can't honestly say as I'm not even sure why this isn't working.
-
For some odd reason, it no longer wants to find matches that it was finding before. The only difference from before is that the formatted string is being used every time instead of the original string. I don't see why this would change anything, so there's probably something small going on that we're just missing.
-
Can you confirm that the original script outputted text that was partly formatted, but not entirely formatted (when it was repeating the text over and over again), or was it entirely unformatted?
-
I've confirmed that the preg_replace is not finding the keywords in the text but haven't yet come up with a reason. Something seems amiss here. I can't promise I'll keep working on it much more tonight though, about to start drinking.
-
Your database appears to have an error. The 510th keyword is actually a URL: [509] => http://www.rawguru.com/store/raw-food/wild_peanut_caramel_chocolate.html Fix this, and see if the issue is resolved.
-
Is the id column in your table a string? If not, try this: $sql1="UPDATE $tbl_name SET employer='$_POST[employer][$i]' WHERE id=$id[$i]";
-
Okay, now we're on to something. Run this for me. <?php $pull_url = mysql_query("SELECT * FROM `jos_autolinks`"); $theviddetails2 = $recipe->ingredients; echo $theviddetails2; $i=0; while ($url = mysql_fetch_array($pull_url)) { $keyword[$i] = $url['phrase']; $link = $url['link']; $target = $url['target']; $theviddetails2 = preg_replace("/$keyword/", "<a href='$link' target='$target' style='text-decoration:none; border-bottom: 1px dotted;'>$keyword</a>", $theviddetails2); $i++; } print_r($keyword); print $theviddetails2; ?>
-
As for this warning: "Warning: Cannot modify header information - headers already sent by (output started at /home/content/n/f/l/nflcars/html/flashmarquee/application10.php:34) in /home/content/n/f/l/nflcars/html/flashmarquee/application10.php on line 67" You're outputting data: ?> </p> <table width="500" border="0" cellspacing="1" cellpadding="0"> <form name="form1" method="post" action=""> <tr> <td> <table width="100%" border="0" align="center" cellpadding="0" cellspacing="1"> <tr> <td colspan="5" align="left" valign="top"><br /> <br /></td> </tr> <?php while($rows=mysql_fetch_array($result)){ ?> <tr> <td width="25%" align="left" valign="top">Employer</td> <td width="34%" align="left" valign="top"><input name="employer[]" type="text" id="employer" value="<? echo $rows['employer']; ?>"></td> <td colspan="3" align="left" valign="top"> </td> </tr> <tr> <td colspan="5" align="left" valign="top"><hr /></td> </tr> <?php } ?> <tr align="left" valign="top"> <td colspan="3"><input type="submit" name="Submit" value="Submit"></td> </tr> </table> </td> </tr> </form> </table> <div align="center"> <?php Then trying to change a header: if(isset($result1)){ header("location:application10.php"); } Header's must be edited BEFORE anything is sent to the browser.
-
ipwnzphp, try one more thing for me: <?php $pull_url = mysql_query("SELECT * FROM `jos_autolinks`"); $theviddetails2 = $recipe->ingredients; while ($url = mysql_fetch_array($pull_url)) { $keyword = $url['phrase']; $link = $url['link']; $target = $url['target']; echo strlen($theviddetails2).' '; $theviddetails2 = preg_replace("/$keyword/", "<a href='$link' target='$target' style='text-decoration:none; border-bottom: 1px dotted;'>$keyword</a>", $theviddetails2); } print $theviddetails2; ?>
-
Solar, no. The slashes will break it. Using single quotes inside double quotes (or visa versa, double quotes inside single) will cause the single (or double) quote to be printed normally. ipwnzphp, I really don't know what to tell you. The code appears to lose scope on the variable without any particular reason. While the loop does create a new block context, I've never used a PHP parser that caused scope issues except when changing functions or classes. You can try this, it's a lot of unnecessary processing, but maybe it'll work: <?php $pull_url = mysql_query("SELECT * FROM `jos_autolinks`"); $some_flag = FALSE; while ($url = mysql_fetch_array($pull_url)) { if (!$some_flag) { $theviddetails2 = $recipe->ingredients; $some_flag = TRUE; } $keyword = $url['phrase']; $link = $url['link']; $target = $url['target']; $theviddetails2 = preg_replace("/$keyword/", "<a href='$link' target='$target' style='text-decoration:none; border-bottom: 1px dotted;'>$keyword</a>", $theviddetails2); } print $theviddetails2; ?>
-
Is this the exact context of your code? This is a copy+paste from what is actually running on your server?
-
You're getting those notices because the variables you've used ("$Submit" and "$result1") are undefined. It seems you've copied this code from somewhere else. This code appears to be created before a major change in PHP, the register_globals directive was defaulted from "on" to "off". In PHP 4.1.0 and up you can refer to data submitted via a post request with: $_POST['html_name_attribute'] Whenever data isn't submitted, $result1 is not defined and thus will throw this notice. A solution would be to initialize it to false before you check if data was submitted.
-
The problem is that, where ever you're doing this: "$theviddetails2 = $recipe->ingredients;" $theviddetails2 becomes out of scope by the time you do this: "$theviddetails2 = preg_replace(..." inside of the loop. The only solution I can offer you is to define $theviddetails2 as a global variable whenever you use it inside a function or method (a class function).
-
This is PHP's manual entry for variable scope, if this doesn't help I can try to help you further: http://us.php.net/global
-
That website goes over how to do exactly what you describe: <?php $formatted_submission = nl2br($unformatted_submission); ?>
-
http://us.php.net/nl2br
-
There's a scope issue between "$theviddetails2 = $recipe->ingredients;" and "$theviddetails2 = preg_replace(...". Without knowing the exact context of the script, it'll be difficult to fix that issue for you. The most direct solution would be to declare $theviddetails2 outside the scope of any functions/classes. Then, within any functions/objects you want to use it, you'll have to declare it as a global variable using: global $theviddetails2;
-
Welp, it's not a spelling error now. Try running this for me: <?php $pull_url = mysql_query("SELECT * FROM `jos_autolinks`"); echo (empty($recipe->ingredients)) ? 'Ingredients is empty ' : ''; $theviddetails2 = $recipe->ingredients; echo (empty($theviddetails2)) ? 'Vid Details is empty ' : ''; while ($url = mysql_fetch_array($pull_url)) { $keyword = $url['phrase']; $link = $url['link']; $target = $url['target']; echo (empty($theviddetails2)) ? 'Vid2 Details is empty ' : ''; $theviddetails2 = preg_replace("/$keyword/", "<a href='$link' target='$target' style='text-decoration:none; border-bottom: 1px dotted;'>$keyword</a>", $theviddetails2); } echo (empty($theviddetails2)) ? 'Vid3 Details is empty ' : ''; print $theviddetails2; ?>
-
Can you confirm that the database lists the changed id number as 7 and not 11?
-
Bah, I feel like an amateur. Just a spelling error, sorry: <?php $pull_url = mysql_query("SELECT * FROM `jos_autolinks`"); $theviddetails2 = $recipe->ingredients; while ($url = mysql_fetch_array($pull_url)) { $keyword = $url['phrase']; $link = $url['link']; $target = $url['target']; $theviddetails2 = preg_replace("/$keyword/", "<a href='$link' target='$target' style='text-decoration:none; border-bottom: 1px dotted;'>$keyword</a>", $theviddetails2); } print $theviddetails2; ?>