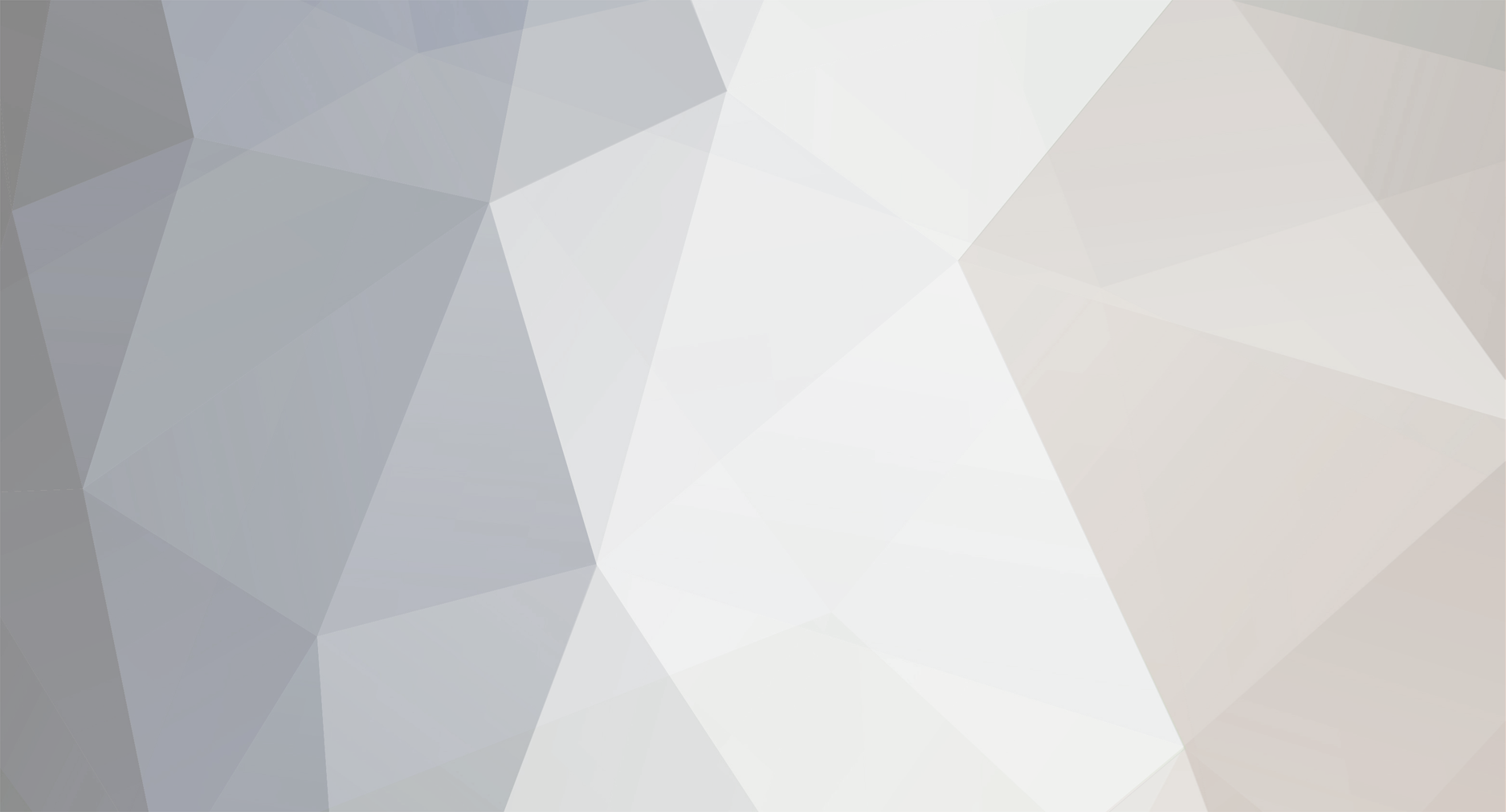
ialsoagree
Members-
Posts
406 -
Joined
-
Last visited
Everything posted by ialsoagree
-
You misinterpreted my response. There is going to be size issues (especially with my method) but there isn't going to be search issues. I'm NOT suggesting you create an array with the barcodes in use. I AM suggesting you create an array with barcodes NOT in use. You could use a DB table instead. Eitherway, the solution isn't to look around for what barcodes are in use, but rather to make a list of the barcodes not in use, and delete them once they are. The volume of barcodes you use isn't really relevant when you use my solution. You can search for 10,000 barcodes at a time if you want. You just need to use methods that select barcodes from a list of available barcodes, and never duplicate the choice. Then just delete the selections from that list.
-
Okay, touche, this is true. But this is more an issue of name space rather than variable scope.
-
You could create an array of every possible barcode, remove from that array every barcode that's currently being used, and save the array. Then, use array_rand to choose a barcode and remove it from the array when it's chosen.
-
Use "SELECT DISTINCT" instead of "SELECT". The distinct keyword tells MySQL not to include the same row more than once in the result set.
-
You can do a refresh with HTML. Read up on it here (just remember, you have to re-identify the user!): http://webdesign.about.com/od/metataglibraries/a/aa080300a.htm Doing it with javascript involves the setTimeOut function. You can read about it here: http://www.sean.co.uk/a/webdesign/javascriptdelay.shtm As far as youtube goes, that's done via AJAX (Asynchronous Javascript and XML). AJAX allows Javascript to send an HTTP request to the server (without loading a new browser page or creating a new entry in the browser's history - just remember, it's a regular HTTP request, you still have to identify the user, you also may need to identify that it's an AJAX request and not a regular request depending on how you set your webpage up!), get a response from the server, and then process that response and update the page the user is currently viewing without refreshing it. AJAX is a big beast. It's extremely powerful, extremely useful to learn, and a lot of work to perfect (from my experience, anyway - and no, I have not perfected it, I still consider myself a beginner). W3 School can get you started using AJAX. There are other tutorials on the web too. As for count down timers, this could be done with a variety of javascript and HTML (possibly AJAX). It can also be done with Flash or even Java. I couldn't tell you exactly how they tackle that project.
-
I may be wrong, but I'm leading toward no, this is not possible. HTTP is a stateless protocol. It sends complete text files containing all headers/content that the browser needs, and the browser sends complete text requests. You can use javascript to accomplish this, but all the data will be submitted instantly and it'll just be the user's browser delaying it. You could use AJAX to delay it. This would prevent you from sending the data, but you would have to have a way of identifying the original request and sending the appropriate information. Since HTTP is stateless, you need something like sessions to re-identify the same user.
-
This is about the best that I know of, is it what you're looking for? http://us2.php.net/manual/en/function.htmlspecialchars.php
-
At a quick glance, it looks like all your IP processing is done in functions, but there's never actually any call to those functions so the $ip variable remains undefined in the global scope through the duration of your script. I think you just need to call one of your IP functions to actually return the IP to a variable. Maybe?: <?php $write = mysql_query("INSERT INTO posts VALUES ('','$name','$message', 'getip()')") or die(mysql_eror()); ?>
-
POST should be GET? <?php $itemNumber=$_GET['number']; echo "<p>Item Number: ".$itemNumber."</p>"; ?> PS. because you're using double quotes you don't need to remove the variable from the string. In fact, if you're going to remove the variable from the string, you shouldn't use double quotes because it takes (marginally) longer to process (the string has to be parsed by PHP, but the variable was removed from the string so it's parsing that string for no reason wasting processing cycles). This works the same way, and makes the double quotes not redundant. <?php $itemNumber=$_POST['number']; echo "<p>Item Number: $itemNumber</p>"; ?>
-
Multiple Mysql Inserts - Point me in the right direction?
ialsoagree replied to MasterK's topic in PHP Coding Help
I would start by changing this: <?php <td><input size=10 type=text name=AnimeN<? echo"$i";?>></td> <td><input size=5 type=text name=SnNumber<? echo"$i";?>></td> <td><input size=5 type=text name=EpNumber<? echo"$i";?>></td> <td><input size=30 type=text name=EpName<? echo"$i";?>></td> <td><input size=30 type=text name=VidURL<? echo"$i";?>></td> ?> ...to this... <?php <td><input size=10 type=text name=AnimeN[]></td> <td><input size=5 type=text name=SnNumber[]></td> <td><input size=5 type=text name=EpNumber[]></td> <td><input size=30 type=text name=EpName[]></td> <td><input size=30 type=text name=VidURL[]></td> <td> <select name=Host[]> <option value=Myspace>Myspace</option> <option value=MegaVideo>Megavideo</option> <option value=Veoh>Veoh</option> <option value=clip.vn>clip.vn</option> <option value=Other>Other</option> <option value=MP4>MP4</option> </select></td></tr> ?> (I would also go through and add HTML quotes around your attribute settings, not having them is bad HTML). You can then use the foreach loop on one of the arrays, $_POST['AnimeN'] for example, define both the $key and $value parameters, and you can use $key to refer to all other arrays for that submission: <?php foreach ($_POST['AnimeN'] as $key => $value) { /* do stuff if you want */ $_POST['SnNumber'][$key]; // the SnNumber for the current submission /* more stuff here */ } ?> Unfortunately, you will have to do multiple inserts to the database (unless someone knows another way of doing inserts), fortunately using this method you can format all the submissions individually as you go through the loop and run the insert statement at the end of each loop. -
You use this if statement: "if (is_int($cat_id))" If $cat_id is being passed to the server via the browser, it will always be text (everything sent via HTML is interpreted as a variable type of string). If this is how $_REQUEST['cat_id'] is defined (by an HTML submission) try using is_numeric instead.
-
Defining variables as global is terrible advice. Globals are considered bad practice in many programming languages including PHP. I think that's highly debatable. When dealing with MySQL connections, far better to define 1 connection and use it consistently through a script then make several connections to the MySQL database (quite a bit of wasted over head and can even lead to scripts failing due to too many connections). If you know of any significant security issues addressed by passing a database connection to functions/methods that are not addressed by using a global variable to refer to that connection please let me know, might be worth updating some of my PHP if this is the case! I use classes to handle database interactions, webpage interactions, permissions, validations etc. and let methods and functions refer to these objects using the global keyword as they need. With proper use of privacy I see no security that could be breached that couldn't be breached with other methods.
-
"Keep it simple stupid" is a saying I tend to follow and didn't in my previous post, I apologize for that. Here's a better way of getting at my previous question. In the statement "$cat_id = $_REQUEST['cat_id'];" what is defining $_REQUEST['cat_id']? Is it defining that array index as the category name?
-
The explanation was hard to follow, I have an idea of what might be wrong though, let me know if I have it right: Are you defining a connection to a database and then you are unable to access that connection inside of class methods? If this is the case, make sure you are defining the database in a global scope (that is, outside of any functions or classes - or if it is in a function or class, the database is being assigned to a variable that has been initialized in that function or class using the global keyword). If you are, start your class method by recognizing the database as a global variable: <?php function some_class_method() { global $db; /* Rest of code */ } ?> Does that help?
-
Are you using the category name to allow the user to get to these pages? Perhaps a different page is linking the category name instead of the ID, and this page is expecting the ID instead of the name. If your ID's are numeric, you could make an OO function that checks if cat_id's are numeric and if so, converts them to ints. If they're not numeric, it finds the category ID based on the string (assuming it's a name - with proper escaping you should be safe from SQL injection). If not, I think you'd have to find the source of the problem.
-
It's going to be a fair amount of work no matter how you do it. There's no function in MySQL (that I've ever heard of anyway) or in PHP that handles this type of check. It leaves you with 2 choices, one choice requires less processing each time the script runs, and the other requires more processing each time the script runs. Any time that you can hard code a check (that is, define a set S, and then check if x is in set S) the check is going to have the minimum amount of processing necessary (that's what I've given you above). Any time you have to generate the set S (by running a query, for example) you have to tell PHP to produce the set (this is going to be a decent amount of coding, and all that code has to be processed every time the script runs). But you're not done, all you've done is created the S set. Now you have to go through and see if x is in it. In the end, my suggestion is (probably) the best. It might be more tedious, but the script will run faster and you really don't do any more coding. In the end, no, there's no easy way to code this (at least that I know of). If you are really unwilling to write out the list of tables and columns yourself, write a script to do this for you, then copy and paste it into your code. I'm not willing to do all the work for you, but I can give you the jist of how to do it: <?php $result = mysql_query('SELECT * FROM some_table LIMIT 1'); $array = mysql_fetch_assoc($result); foreach ($array as $key => $value) { echo "\n".' $database_array[\'some_table\'][] = $key;'; } ?> I will admit, there is a small improvement you could make to my code. Instead of using numbered indexes and seeing if the column value is in that table (requiring the use of in_array), you could use the columns as indexes and check if it's defined: <?php $result = mysql_query('SELECT * FROM some_table LIMIT 1'); $array = mysql_fetch_assoc($result); foreach ($array as $key => $value) { echo "\n".' $database_array[\'some_table\'][\''.$key.'\'] = TRUE;"; } ?> (there appears to be an error dispalying this, the 2nd index should be [\''.$key.'\'] but it does not appear to display this way inside the code box - this is an error with the forum and not with the way the code is written, make sure to apply this correction if you copy and paste this code). ...and then to check if a given column exists... <?php if ($database_array['table_to_search']['column_to_sort_by'] === TRUE) ?>
-
How do I replace any number of character occurences with one occurrence?
ialsoagree replied to OM2's topic in PHP Coding Help
You might want to have a look at str_replace: http://www.php.net/manual/en/function.str-replace.php If you check out the optional $count variable you should be able to figure out a way of creating a loop to do this for you. -
On MySQL: <?php $sql = 'SELECT location.locationName, room.roomName, call.* FROM location, room, call WHERE call.roomID = room.roomID AND room.locationID = location.locationID ORDER BY locationID ASC, roomID ASC, callID ASC'; ?> If I screwed up anything, it may be that the order by columns need a table prefix, in which case you'll probably want to use room.locationID, call.roomID, and call.callID but I don't think you should need the prefixes. If my logic is right, you should wind up getting arrays with all the calls for a particular room in a particular location, followed by all the call's for the next room in the same location etc., followed by all the calls for the first room in the next location, followed by all the calls for the second room in the second location etc. Record the location and room for the first query (building divs and tables as necessary) and insert calls as long as the room doesn't change. If the room changes, make sure the location is the same and if it is, build a new table, otherwise, build a new div. If you don't want the highest ID's last, then change every occurance of ASC to DESC (ascending, small to large, vs. DESC, large to small).
-
That seems pretty simple and efficient to me, although not hard to spoof. It depends on the security necessary for your website. I've personally adopted the habit of saving session ID's in the database, and then saving any appropriate information there (like what user is logged in - and if they are logged in for that matter, IP's, etc.). This puts all the authentication server side and the only thing that the user submits is a session ID (much harder to spoof, although not impossible, hence the IP is checked too). If the code you made works for you and you just want an easy way of including it all your scripts without copy and pasting it, you could put it into a file such as session.php and then have all your scripts run: require_once('session.php');
-
I'm not on a computer I can test so you might want to check to make sure that this is parsing the variable correctly: <?php echo "<div class='paginleft'><a href='pages.php?action=get_entries&cat_id=$cat_id¤tpage=1'>First</a></div>"; ?> With $cat_id surrounded by text, is PHP parsing that variable correctly? Worth checking the HTML.
-
This seems like a check that would be better to do BEFORE you run an SQL query, here's why: -If you do the check first, you can run 1 SQL query that orders everything for you. -Having SQL order the data for you saves PHP from having to do it (SQL is going to be better at it anyway, with PHP it's going to take 2 loops). You should know all the column names for all of your tables so my solution would be this: Build an array with indexes with the name of each of your tables: $database_array['table1'] $database_array['table2'] $database_array['tablex'] And then for each table, include the names of each column like so: $database_array['table1'][] = 'colum_name1' $database_array['table1'][] = 'colum_name2' $database_array['table1'][] = 'colum_namex' $database_array['table2'][] = 'colum_name1' $database_array['table2'][] = 'colum_name2' etc. Then you can use the in_array function to determine if an order by criteria would work on a specific table, more on in_array here: http://us2.php.net/manual/en/function.in-array.php
-
Your question is a little bit confusing. Would this work for you? <?php $query2 = "SELECT $srt FROM telephone WHERE signoff_status = '$so'"; ?>
-
If you're looking for an if, elseif, else syntax: <?php if (/*IE condition*/) { /* Code to Execute */ } elseif (/*FF condition*/) { /* Code to Execute */ } else { /* Code to Execute */ } ?> If you're looking for the conditions themselves, you might want to read up on this PHP variable: $_SERVER['HTTP_USER_AGENT'] You can set up a javascript that check's the user's browser and then loads a CSS file as an alternative
-
[SOLVED] Help With Showing Users On the Index Page
ialsoagree replied to shorty3's topic in PHP Coding Help
Just wanted to point out, mikesta707's earlier suggestion of including brackets around the else is NOT necessary. When brackets are left out, the else is interpreted for 1 following line. Your code could work fine as I suggested it, to add the image, do this: <?php $start = TRUE; while ($online_array = mysql_fetch_assoc($select)) { if ($start) { echo $online_array['username'].' <img src="'.$online_array['image'].'" />'; $start = FALSE; } else echo ', '.$online_array['username'].' <img src="'.$online_array['image'].'" />'; } echo ' were online recently'; ?> If you want to limit the number of displayed users without limiting the count on the total users, drop off the LIMIT clause in the SELECT statement, and change my above loop as follows: <?php $start = TRUE; $max = ($num > 5) ? 5 : $num; // Replace 5 with the max number of users you want to display for ($i=0; $i<$max; $i++) { $online_array = mysql_fetch_assoc($select); if ($start) { echo $online_array['username'].' <img src="'.$online_array['image'].'" />'; $start = FALSE; } else echo ', '.$online_array['username'].' <img src="'.$online_array['image'].'" />'; } echo ' were online recently'; ?> -
Not necessarily, when you are refering to an associated array inside double quotes you can leave off single quotes to address the indexes: <?php echo "$some_array[some_index] - The correct way to address some_index in the array inside double quotes."; ?> dsp77 You write this code: <?php require_once('config.php'); $order = "UPDATE intrari SET start='$start', sfarsit='$sfarsit', nume='$nume', prioritate='$prioritate', descriere='$descriere WHERE id='$id'"; mysql_query($order); header("location:edit.php"); ?> Where is $start, $sfarsit, $nume, $prioritate, $descriere, and $id defined?