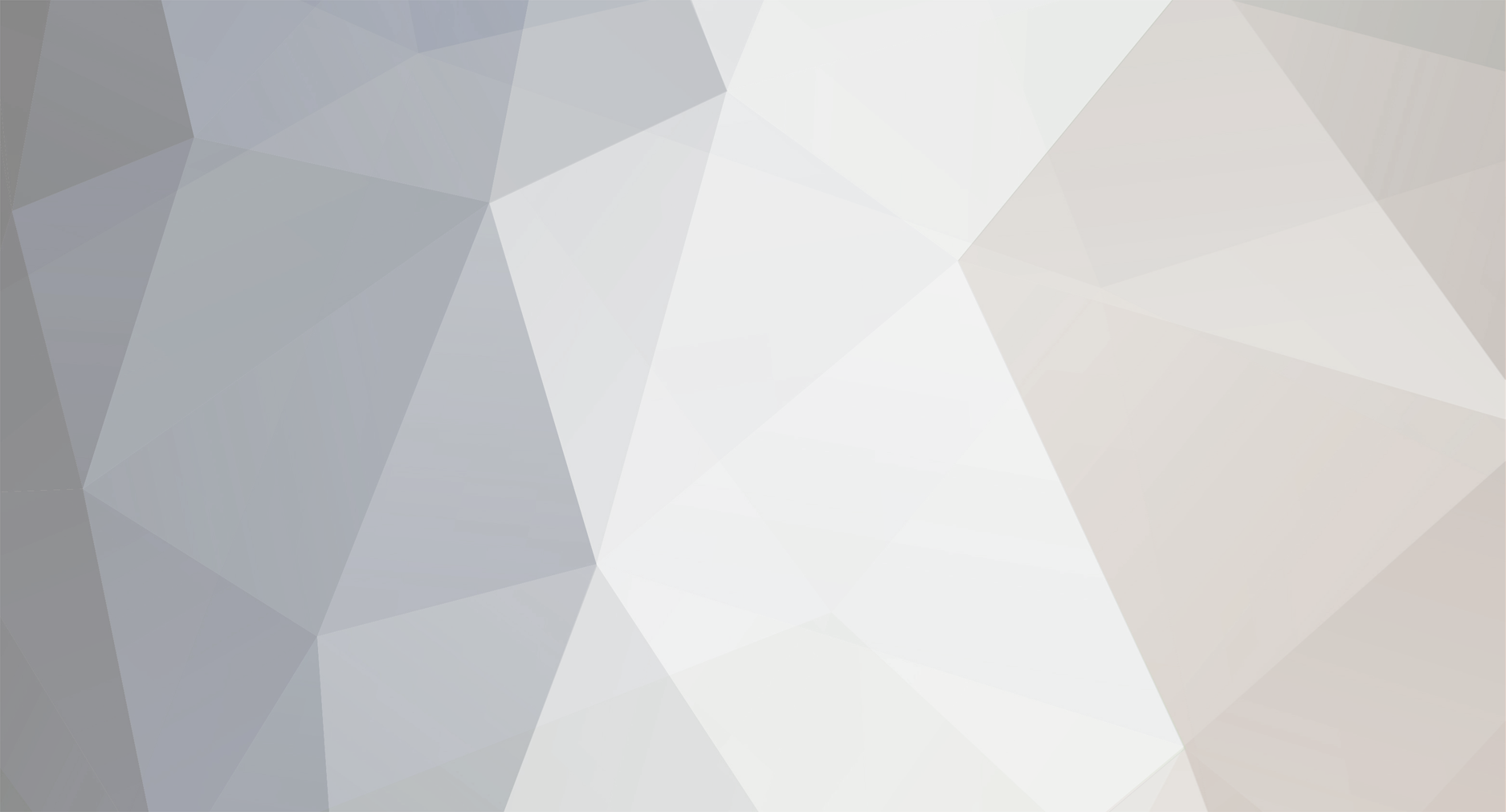
justAnoob
Members-
Posts
561 -
Joined
-
Last visited
Never
Everything posted by justAnoob
-
Where on my site am I able to use this php file? Should I just do an "include" on the top of all my scripts? On my site,, users can register, login, change their account info, post pictures with description of thier pictures, and send messages to other users. <?php /** @class: InputFilter (PHP4 & PHP5, without comments) * @project: PHP Input Filter * @date: 10-05-2005 * @version: 1.2.2_php4/php5 * @author: Daniel Morris * @contributors: Gianpaolo Racca, Ghislain Picard, Marco Wandschneider, Chris Tobin and Andrew Eddie. * @copyright: Daniel Morris * @email: dan@rootcube.com * @license: GNU General Public License (GPL) */ class InputFilter { var $tagsArray; var $attrArray; var $tagsMethod; var $attrMethod; var $xssAuto; var $tagBlacklist = array('applet', 'body', 'bgsound', 'base', 'basefont', 'embed', 'frame', 'frameset', 'head', 'html', 'id', 'iframe', 'ilayer', 'layer', 'link', 'meta', 'name', 'object', 'script', 'style', 'title', 'xml'); var $attrBlacklist = array('action', 'background', 'codebase', 'dynsrc', 'lowsrc'); function inputFilter($tagsArray = array(), $attrArray = array(), $tagsMethod = 0, $attrMethod = 0, $xssAuto = 1) { for ($i = 0; $i < count($tagsArray); $i++) $tagsArray[$i] = strtolower($tagsArray[$i]); for ($i = 0; $i < count($attrArray); $i++) $attrArray[$i] = strtolower($attrArray[$i]); $this->tagsArray = (array) $tagsArray; $this->attrArray = (array) $attrArray; $this->tagsMethod = $tagsMethod; $this->attrMethod = $attrMethod; $this->xssAuto = $xssAuto; } function process($source) { if (is_array($source)) { foreach($source as $key => $value) if (is_string($value)) $source[$key] = $this->remove($this->decode($value)); return $source; } else if (is_string($source)) { return $this->remove($this->decode($source)); } else return $source; } function remove($source) { $loopCounter=0; while($source != $this->filterTags($source)) { $source = $this->filterTags($source); $loopCounter++; } return $source; } function filterTags($source) { $preTag = NULL; $postTag = $source; $tagOpen_start = strpos($source, '<'); while($tagOpen_start !== FALSE) { $preTag .= substr($postTag, 0, $tagOpen_start); $postTag = substr($postTag, $tagOpen_start); $fromTagOpen = substr($postTag, 1); $tagOpen_end = strpos($fromTagOpen, '>'); if ($tagOpen_end === false) break; $tagOpen_nested = strpos($fromTagOpen, '<'); if (($tagOpen_nested !== false) && ($tagOpen_nested < $tagOpen_end)) { $preTag .= substr($postTag, 0, ($tagOpen_nested+1)); $postTag = substr($postTag, ($tagOpen_nested+1)); $tagOpen_start = strpos($postTag, '<'); continue; } $tagOpen_nested = (strpos($fromTagOpen, '<') + $tagOpen_start + 1); $currentTag = substr($fromTagOpen, 0, $tagOpen_end); $tagLength = strlen($currentTag); if (!$tagOpen_end) { $preTag .= $postTag; $tagOpen_start = strpos($postTag, '<'); } $tagLeft = $currentTag; $attrSet = array(); $currentSpace = strpos($tagLeft, ' '); if (substr($currentTag, 0, 1) == "/") { $isCloseTag = TRUE; list($tagName) = explode(' ', $currentTag); $tagName = substr($tagName, 1); } else { $isCloseTag = FALSE; list($tagName) = explode(' ', $currentTag); } if ((!preg_match("/^[a-z][a-z0-9]*$/i",$tagName)) || (!$tagName) || ((in_array(strtolower($tagName), $this->tagBlacklist)) && ($this->xssAuto))) { $postTag = substr($postTag, ($tagLength + 2)); $tagOpen_start = strpos($postTag, '<'); continue; } while ($currentSpace !== FALSE) { $fromSpace = substr($tagLeft, ($currentSpace+1)); $nextSpace = strpos($fromSpace, ' '); $openQuotes = strpos($fromSpace, '"'); $closeQuotes = strpos(substr($fromSpace, ($openQuotes+1)), '"') + $openQuotes + 1; if (strpos($fromSpace, '=') !== FALSE) { if (($openQuotes !== FALSE) && (strpos(substr($fromSpace, ($openQuotes+1)), '"') !== FALSE)) $attr = substr($fromSpace, 0, ($closeQuotes+1)); else $attr = substr($fromSpace, 0, $nextSpace); } else $attr = substr($fromSpace, 0, $nextSpace); if (!$attr) $attr = $fromSpace; $attrSet[] = $attr; $tagLeft = substr($fromSpace, strlen($attr)); $currentSpace = strpos($tagLeft, ' '); } $tagFound = in_array(strtolower($tagName), $this->tagsArray); if ((!$tagFound && $this->tagsMethod) || ($tagFound && !$this->tagsMethod)) { if (!$isCloseTag) { $attrSet = $this->filterAttr($attrSet); $preTag .= '<' . $tagName; for ($i = 0; $i < count($attrSet); $i++) $preTag .= ' ' . $attrSet[$i]; if (strpos($fromTagOpen, "</" . $tagName)) $preTag .= '>'; else $preTag .= ' />'; } else $preTag .= '</' . $tagName . '>'; } $postTag = substr($postTag, ($tagLength + 2)); $tagOpen_start = strpos($postTag, '<'); } $preTag .= $postTag; return $preTag; } function filterAttr($attrSet) { $newSet = array(); for ($i = 0; $i <count($attrSet); $i++) { if (!$attrSet[$i]) continue; $attrSubSet = explode('=', trim($attrSet[$i])); list($attrSubSet[0]) = explode(' ', $attrSubSet[0]); if ((!eregi("^[a-z]*$",$attrSubSet[0])) || (($this->xssAuto) && ((in_array(strtolower($attrSubSet[0]), $this->attrBlacklist)) || (substr($attrSubSet[0], 0, 2) == 'on')))) continue; if ($attrSubSet[1]) { $attrSubSet[1] = str_replace('&#', '', $attrSubSet[1]); $attrSubSet[1] = preg_replace('/\s+/', '', $attrSubSet[1]); $attrSubSet[1] = str_replace('"', '', $attrSubSet[1]); if ((substr($attrSubSet[1], 0, 1) == "'") && (substr($attrSubSet[1], (strlen($attrSubSet[1]) - 1), 1) == "'")) $attrSubSet[1] = substr($attrSubSet[1], 1, (strlen($attrSubSet[1]) - 2)); $attrSubSet[1] = stripslashes($attrSubSet[1]); } if ( ((strpos(strtolower($attrSubSet[1]), 'expression') !== false) && (strtolower($attrSubSet[0]) == 'style')) || (strpos(strtolower($attrSubSet[1]), 'javascript:') !== false) || (strpos(strtolower($attrSubSet[1]), 'behaviour:') !== false) || (strpos(strtolower($attrSubSet[1]), 'vbscript:') !== false) || (strpos(strtolower($attrSubSet[1]), 'mocha:') !== false) || (strpos(strtolower($attrSubSet[1]), 'livescript:') !== false) ) continue; $attrFound = in_array(strtolower($attrSubSet[0]), $this->attrArray); if ((!$attrFound && $this->attrMethod) || ($attrFound && !$this->attrMethod)) { if ($attrSubSet[1]) $newSet[] = $attrSubSet[0] . '="' . $attrSubSet[1] . '"'; else if ($attrSubSet[1] == "0") $newSet[] = $attrSubSet[0] . '="0"'; else $newSet[] = $attrSubSet[0] . '="' . $attrSubSet[0] . '"'; } } return $newSet; } function decode($source) { $source = html_entity_decode($source, ENT_QUOTES, "ISO-8859-1"); $source = preg_replace('/&#(\d+);/me',"chr(\\1)", $source); $source = preg_replace('/&#x([a-f0-9]+);/mei',"chr(0x\\1)", $source); return $source; } function safeSQL($source, &$connection) { if (is_array($source)) { foreach($source as $key => $value) if (is_string($value)) $source[$key] = $this->quoteSmart($this->decode($value), $connection); return $source; } else if (is_string($source)) { if (is_string($source)) return $this->quoteSmart($this->decode($source), $connection); } else return $source; } function quoteSmart($source, &$connection) { if (get_magic_quotes_gpc()) $source = stripslashes($source); $source = $this->escapeString($source, $connection); return $source; } function escapeString($string, &$connection) { if (version_compare(phpversion(),"4.3.0", "<")) mysql_escape_string($string); else mysql_real_escape_string($string); return $string; } } ?>
-
got session start on both of them,, just posting what worked.
-
hope you don't mind me using your name for the subject,,,, But,, thanks for not just giving me the answer to a problem that I was having... I did a lot of trial and error and finally found something that works... It feels so much better to have figured it out on my own......Everything works great so far(IE and FireFox).. Everything look ok? It retrieves whatever url is in the box on my browser,,, so then I can use it for a header statement... <?php $_SESSION['url'] = "http://".$_SERVER['HTTP_HOST'].$_SERVER['REQUEST_URI']."?".$_SERVER['QUERY_STRING']; ?> <?php $url = $_SESSION['url']; header("location:" . $url); exit(); ?>
-
Someone showed me this.... Save this as server_stuff.php,,,,, or whatever you want... Then upload to server or whatever you use and then view the page.... <?php echo "<table border=\"1\">"; echo "<tr><td>" .$_SERVER['argv'] ."</td><td>argv</td></tr>"; echo "<tr><td>" .$_SERVER['argc'] ."</td><td>argc</td></tr>"; echo "<tr><td>" .$_SERVER['GATEWAY_INTERFACE'] ."</td><td>GATEWAY_INTERFACE</td></tr>"; echo "<tr><td>" .$_SERVER['SERVER_ADDR'] ."</td><td>SERVER_ADDR</td></tr>"; echo "<tr><td>" .$_SERVER['SERVER_NAME'] ."</td><td>SERVER_NAME</td></tr>"; echo "<tr><td>" .$_SERVER['SERVER_SOFTWARE'] ."</td><td>SERVER_SOFTWARE</td></tr>"; echo "<tr><td>" .$_SERVER['SERVER_PROTOCOL'] ."</td><td>SERVER_PROTOCOL</td></tr>"; echo "<tr><td>" .$_SERVER['REQUEST_METHOD'] ."</td><td>REQUEST_METHOD</td></tr>"; echo "<tr><td>" .$_SERVER['REQUEST_TIME'] ."</td><td>REQUEST_TIME</td></tr>"; echo "<tr><td>" .$_SERVER['QUERY_STRING'] ."</td><td>QUERY_STRING</td></tr>"; echo "<tr><td>" .$_SERVER['DOCUMENT_ROOT'] ."</td><td>DOCUMENT_ROOT</td></tr>"; echo "<tr><td>" .$_SERVER['HTTP_ACCEPT'] ."</td><td>HTTP_ACCEPT</td></tr>"; echo "<tr><td>" .$_SERVER['HTTP_ACCEPT_CHARSET'] ."</td><td>HTTP_ACCEPT_CHARSET</td></tr>"; echo "<tr><td>" .$_SERVER['HTTP_ACCEPT_ENCODING'] ."</td><td>HTTP_ACCEPT_ENCODING</td></tr>"; echo "<tr><td>" .$_SERVER['HTTP_ACCEPT_LANGUAGE'] ."</td><td>HTTP_ACCEPT_LANGUAGE</td></tr>"; echo "<tr><td>" .$_SERVER['HTTP_CONNECTION'] ."</td><td>HTTP_CONNECTION</td></tr>"; echo "<tr><td>" .$_SERVER['HTTP_HOST'] ."</td><td>HTTP_HOST</td></tr>"; echo "<tr><td>" .$_SERVER['HTTP_REFERER'] ."</td><td>HTTP_REFERER</td></tr>"; echo "<tr><td>" .$_SERVER['HTTP_USER_AGENT'] ."</td><td>HTTP_USER_AGENT</td></tr>"; echo "<tr><td>" .$_SERVER['HTTPS'] ."</td><td>HTTPS</td></tr>"; echo "<tr><td>" .$_SERVER['REMOTE_ADDR'] ."</td><td>REMOTE_ADDR</td></tr>"; echo "<tr><td>" .$_SERVER['REMOTE_HOST'] ."</td><td>REMOTE_HOST</td></tr>"; echo "<tr><td>" .$_SERVER['REMOTE_PORT'] ."</td><td>REMOTE_PORT</td></tr>"; echo "<tr><td>" .$_SERVER['SCRIPT_FILENAME'] ."</td><td>SCRIPT_FILENAME</td></tr>"; echo "<tr><td>" .$_SERVER['SERVER_ADMIN'] ."</td><td>SERVER_ADMIN</td></tr>"; echo "<tr><td>" .$_SERVER['SERVER_PORT'] ."</td><td>SERVER_PORT</td></tr>"; echo "<tr><td>" .$_SERVER['SERVER_SIGNATURE'] ."</td><td>SERVER_SIGNATURE</td></tr>"; echo "<tr><td>" .$_SERVER['PATH_TRANSLATED'] ."</td><td>PATH_TRANSLATED</td></tr>"; echo "<tr><td>" .$_SERVER['SCRIPT_NAME'] ."</td><td>SCRIPT_NAME</td></tr>"; echo "<tr><td>" .$_SERVER['REQUEST_URI'] ."</td><td>REQUEST_URI</td></tr>"; echo "<tr><td>" .$_SERVER['PHP_AUTH_DIGEST'] ."</td><td>PHP_AUTH_DIGEST</td></tr>"; echo "<tr><td>" .$_SERVER['PHP_AUTH_USER'] ."</td><td>PHP_AUTH_USER</td></tr>"; echo "<tr><td>" .$_SERVER['PHP_AUTH_PW'] ."</td><td>PHP_AUTH_PW</td></tr>"; echo "<tr><td>" .$_SERVER['AUTH_TYPE'] ."</td><td>AUTH_TYPE</td></tr>"; echo "</table>" ?>
-
I have been trying for a while now.. And still nothing... I looked everywhere on google and stuff... Still can't get a grip on how to do this... Even talked to a few people that are looking for the same thing.
-
Is there any way to speed up the upload script??? Or is it the image size that is holding me back? <?php session_start(); include "connection.php"; $item_name = mysql_real_escape_string($_POST['item_name']); $description = mysql_real_escape_string($_POST['description']); $in_return = mysql_real_escape_string($_POST['in_return']); $category = mysql_real_escape_string($_POST['listmenu']); define ("MAX_SIZE","1500"); function getExtension($str) { $i = strrpos($str,"."); if (!$i) { return ""; } $l = strlen($str) - $i; $ext = substr($str,$i+1,$l); return $ext; } $errors=0; if(isset($_POST['submit'])) { $image=$_FILES['image']['name']; if($image) { $filename = stripslashes($_FILES['image']['name']); $extension = getExtension($filename); $extension = strtolower($extension); if (($extension != "jpg") && ($extension != "jpeg") && ($extension != "gif") && ($extension != "png")) { $_SESSION['badformat'] = "Your picture must be a .JPG .GIF or .PNG"; header("location: http://www.------.com/----.php"); $errors=1; exit(); } else { $size=filesize($_FILES['image']['tmp_name']); if ($size > MAX_SIZE*1024) { $_SESSION['toobig'] = "Your picture can not exceed 1.5 megabyte."; header("location: http://www.-------.com/-----.php"); $errors=1; exit(); } $image_name=time().'.'.$extension; $newname="userimages/$category/".$image_name; $copied = copy($_FILES['image']['tmp_name'], $newname); if (!$copied) { $_SESSION['notcopy'] = "There was an error posting your picture. Please try again later."; header("location: http://www.-----.com/--------.php"); $errors=1; exit(); } } } } // if everything is good, post new item for the user $mysqlcategory = $category; $imgpath = $newname; $findit = $_SESSION['id']; $result=mysql_query("SELECT id FROM members WHERE username = '$findit'"); $row=mysql_fetch_assoc($result); $user_id = $row['id']; $sql = "INSERT INTO abcxyz(item_name, description, in_return, imgpath, category, user_id)VALUES('$item_name','$description','$in_return', '$imgpath', '$mysqlcategory', '$user_id')"; mysql_query($sql) or die(mysql_error()); // go to confirmation page if upload is completed. if(isset($_POST['submit']) && !$errors) { $_SESSION['posted'] = $item_name; $_SESSION['picposted'] = $imgpath; header("location: http://www.------.com/-------.php"); exit(); } ?>
-
Also another question.. With my upload script it assigns each picture a name using time()...Each user can upload pictures. All the pictures are viewable, about 125x125 pixels. Then if the image is clicked,, a full size picture is viewable. The question is,,, my upload script uploads the picture at whatever size it is..Should I create thumbnail pics to have displayed in the 125x125 areas and then when they are clicked, it would open the full size images??? My folder setup on my server is like this.... userimages/category1 userimages/category2 etc........ So would I have to also have a thumnail folder for all the thumbs? userimages/thumbs/category1 userimages/thumbs/category2 etc....... Wouldn't this just take up more space on the server?? What is the best way to go about this?
-
Still no luck,,,I've searched google and it seems that others have pretty much what I got here... Everthing else works great other than checking the image size. I have tried $image and $filename for the getimagesize.. <?php session_start(); include "connection.php"; $item_name = mysql_real_escape_string($_POST['item_name']); $description = mysql_real_escape_string($_POST['description']); $in_return = mysql_real_escape_string($_POST['in_return']); $category = mysql_real_escape_string($_POST['listmenu']); define ("MAX_SIZE","1500"); function getExtension($str) { $i = strrpos($str,"."); if (!$i) { return ""; } $l = strlen($str) - $i; $ext = substr($str,$i+1,$l); return $ext; } $errors=0; if(isset($_POST['submit'])) { $image=$_FILES['image']['name']; if($image) { $filename = stripslashes($_FILES['image']['name']); list($width, $height, $type, $attr) = getimagesize($filename); if($height > $width) { echo "Your pictures height is larger than its width. Please resize your picture."; $errors=1; exit(); } $extension = getExtension($filename); $extension = strtolower($extension); if (($extension != "jpg") && ($extension != "jpeg") && ($extension != "gif") && ($extension != "png")) { $_SESSION['badformat'] = "Your picture must be a .JPG .GIF or .PNG"; header("location: http://www.--------.com/-------.php"); $errors=1; exit(); } else { $size=filesize($_FILES['image']['tmp_name']); if ($size > MAX_SIZE*1024) { $_SESSION['toobig'] = "Your picture can not exceed 1.5 megabyte."; header("location: http://www.---------.com/------.php"); $errors=1; exit(); } $image_name=time().'.'.$extension; $newname="userimages/$category/".$image_name; $copied = copy($_FILES['image']['tmp_name'], $newname); if (!$copied) { $_SESSION['notcopy'] = "There was an error posting your picture. Please try again later."; header("location: http://www.-------.com/--------.php"); $errors=1; exit(); } } } } // if everything is good, post picture for the user $mysqlcategory = $category; $imgpath = $newname; $findit = $_SESSION['id']; $result=mysql_query("SELECT id FROM members WHERE username = '$findit'"); $row=mysql_fetch_assoc($result); $user_id = $row['id']; $sql = "INSERT INTO abcxyz(item_name, description, in_return, imgpath, category, user_id)VALUES('$item_name','$description','$in_return', '$imgpath', '$mysqlcategory', '$user_id')"; mysql_query($sql) or die(mysql_error()); // go to confirmation page if upload is completed. if(isset($_POST['submit']) && !$errors) { $_SESSION['posted'] = $item_name; $_SESSION['picposted'] = $imgpath; header("location: http://www.-------.com/-------.php"); exit(); } ?>
-
Oh, sorry. I'm looking to keep images widescreen or square format... No crazy, tall stretch pictures. Also just check to make sure it is an image... What I have below is not working... A picture that is taller than its width is not catching the error that I have below. <?php list($width, $height, $type, $attr) = getimagesize("$image"); if($height > $width) { echo "Your pictures height is larger than its width. Please resize your picture."; $errors=1; exit(); } ?>
-
my size check is not working properly,,, am I missing something? <?php if(isset($_POST['submit'])) { $image=$_FILES['image']['name']; if($image) { $filename = stripslashes($_FILES['image']['name']); $extension = getExtension($filename); $extension = strtolower($extension); if (($extension != "jpg") && ($extension != "jpeg") && ($extension != "gif") && ($extension != "png")) { $_SESSION['badformat'] = "Your picture must be a .JPG .GIF or .PNG"; header("location: http://www.---------.com/--------.php"); $errors=1; exit(); } list($width, $height, $type, $attr) = getimagesize("$image"); if($height > $width) { echo "Your pictures height is larger than its width. Please resize your picture."; $errors=1; exit(); } else { $size=filesize($_FILES['image']['tmp_name']); if ($size > MAX_SIZE*1024) { $_SESSION['toobig'] = "Your picture can not exceed 1.5 megabyte."; header("location: http://www.------.com/---------.php"); $errors=1; exit(); } $image_name=time().'.'.$extension; $newname="userimages/$category/".$image_name; $copied = copy($_FILES['image']['tmp_name'], $newname); if (!$copied) { $_SESSION['notcopy'] = "There was an error posting your picture. Please try again later."; header("location: http://www.-------.com/-------.php"); $errors=1; exit(); } } } } ?>
-
session_set_cookie_params('900', '/', 'http://www.yoursite.com'); //15 minutes i think // keeps the user logged in until the browser closes time limit , path, domain I'm pretty sure the time limit is in seconds.
-
yes,,, how do you mean though?
-
Ok,, here is my setup now... and yes,, i use session_start Lets say this is what is in the url box http://www.mysite.com/mypage.php?pageno=2 I use this to get what is in the url box $_SESSION['getpage'] = $_SERVER['HTTP_REFERER']; I even echoed out that out to see what I get,, and it is the entire address in the url box. Then when I use this header,, it leaves out the page number and I get this... http://www.mysite.com/mypage.php $getpage = $_SESSION['getpage']; header("Location: $getpage"); exit(); Any input would be appreciated. Thanks...
-
http://ww23.rr.com/index.php?origURL=http://http//www.mysite.com/mypage.php That is what page I return with the following code below. I don't want all that,, just http//www.mysite.com/mypage.php It is also leaving out the page number.. Any ideas?? $_SESSION['url'] = 'http://'.$_SERVER['SERVER_NAME'].$_SERVER['PHP_SELF']; $url = $_SESSION['url']; header("Location: http://$url");
-
still having probs... how would you go about getting this url and saving it as a variable to pull later on. http://www.mysite.com/mypage.php?pageno=2
-
I'm working on retrieving the url and store it as a session so I can call it back with a header statement... Does this look right??? <?php $_SESSION['url'] = "http://".$_SERVER['HTTP_HOST'].$_SERVER['REQUEST_URI']; header("" . $_SESSION['url'] . ""); ?>
-
Hey I got it... Awesome,,, this was killing me... This works... I'm so happy. <?php include "connection.php"; $fluke = $_SESSION['id']; $flip = ("SELECT id FROM member_messages WHERE status = 'NEW' and sendto = '" . $fluke . "'"); $flop = mysql_query($flip); $freak = mysql_num_rows($flop); if(!$freak) echo ""; else echo "You have new messages."; ?>
-
Yes,,, NEW is capitalized in the database..... No one can figure this out... What is going on?? <?php include "connection.php"; $userid = $_SESSION['id']; $flip='SELECT id FROM member_messages WHERE status = "NEW" and user_id ="' . $userid . '"'; $flop = mysql_num_rows($flip); // this line if($flop >= 1) { echo "You have new messages."; } ?> Tried using 'id' instead of '*' but still get the same error Warning: mysql_num_rows(): supplied argument is not a valid MySQL result resource on commented line
-
<?php echo "Hello World!!!!"; ?>
-
About to run SELECT * FROM member_messages WHERE status = "NEW" and user_id ="admin" Okay,, that is what I get.
-
string(6) "timcin" int(0) Still giving a 0 when I use var_dump.
-
I have a table in mysql with a colum called 'status'.....The only thing that can go in the column is the word 'NEW', which is placed there when a member gets a new message. I am using the following code to get the number of new messages that a user has but it is not working. I tried using a var_dump to see what is going on and all i get is this... string(6) "timcin" int(0) ... Any ideas why it is not giving me the number of rows that I have. Is is something to do with the way that I have the word 'NEW' in the databae? <?php include "connection.php"; $userid = $_SESSION['id']; $sql=mysql_query('SELECT * FROM member_messages WHERE status = "NEW" and user_id = "$userid"'); $num_rows = mysql_num_rows($sql); var_dump($userid, $num_rows); echo "You have " . $num_rows . " new message(s)."; mysql_close(); ?>