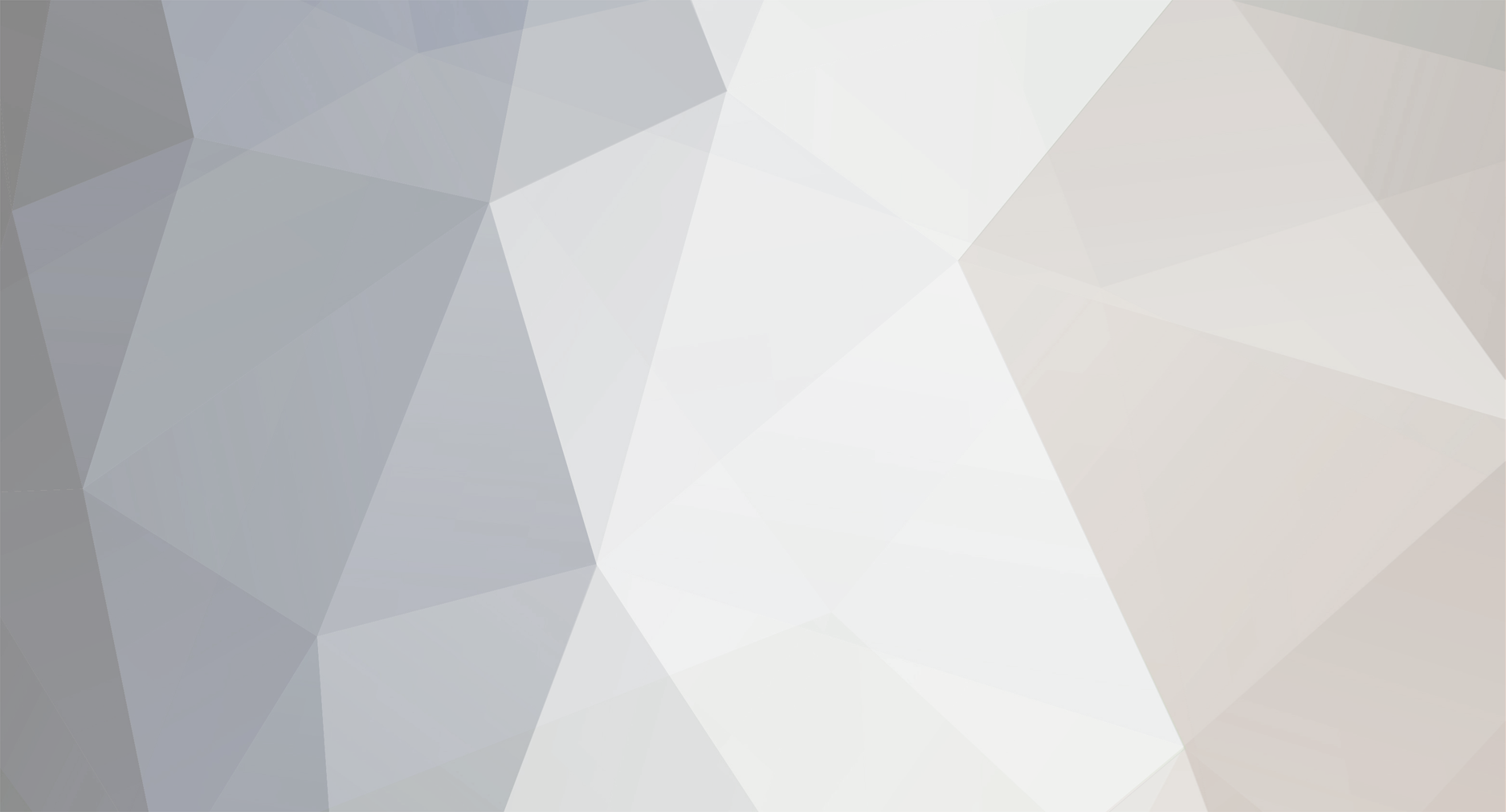
Bman900
Members-
Posts
184 -
Joined
-
Last visited
Never
Everything posted by Bman900
-
Alright so I used a pre made script for a secure log in for my admin panel but I want to add a few more security things to it. Basically I want to make it if a user get his or her password wrong 5 times the account will be locked and and email will be dispatched with a new password. Here is part of the code where the log in errors are handled: function procLogin(){ global $session, $form; /* Login attempt */ $retval = $session->login($_POST['user'], $_POST['pass'], isset($_POST['remember'])); /* Login successful */ if($retval){ header("Location: admin/admin.php"); } /* Login failed */ else{ $_SESSION['value_array'] = $_POST; $_SESSION['error_array'] = $form->getErrorArray(); header("Location: adminlogin.php"); } }
-
There we go! $double = mysql_query("SELECT question, COUNT(id) AS cnt FROM question WHERE id IN(SELECT id FROM question GROUP BY question HAVING count(id) > 1)"); I finally go something that makes sense but I only get one entry and I know there are more doubles and triples in my table. My while statement is: <?php while($row2 = mysql_fetch_assoc( $double )) { echo "<tr>"; echo "<td>".$row2['question']."</td>"; echo "<td>".$row2['cnt']."</td>"; echo "</tr>"; } ?>
-
Ah this is frustrating, it got me a value but it is still not right. I need to combine this: $double = mysql_query("SELECT question FROM question GROUP BY question HAVING count(*) > 1") or die(mysql_error()); with: $double = mysql_query("SELECT COUNT(id) AS cnt FROM question WHERE id IN(SELECT id FROM question GROUP BY question HAVING count(id))"); Please help me, am ripping my hair out here
-
I see what you mean now but I can't seem to do it right. So far I have: $double = mysql_query("SELECT question AND COUNT(id) AS cnt FROM question WHERE id IN(SELECT id FROM question GROUP BY question HAVING count(id))"); What am i doing wrong?
-
Well I am displaying my question, here is the code: $double = mysql_query("SELECT COUNT(id) AS cnt FROM question WHERE id IN(SELECT id FROM question GROUP BY question HAVING count(id))"); ?> <table width="500" border="2" cellspacing="0" cellpadding="0"> <tr> <th>Name</th> <th>Number</th> </tr> <?php while($row2 = mysql_fetch_assoc( $double )) { echo "<tr>"; echo "<td>".$row2['question']."</td>"; echo "<td>".$row2['cnt']."</td>"; echo "</tr>"; } ?> </tr> </table> Or are you talking about the selecting it in my query?
-
I did that and got: Question Number 10
-
Ok that looks like it might work but I need just a bit more help. Here is the code I used to display the query I ran: <table width="500" border="2" cellspacing="0" cellpadding="0"> <tr> <th>Question Asked 2 times</th> </tr> <?php while($row2 = mysql_fetch_assoc( $double )) { echo "<tr>"; echo "<td>".$row2['question']."</td>"; echo "</tr>"; } ?> </tr> </table> Obviously I need to make some changes to that but when I added echo "<td>".$row2['id']."</td>"; But that didn't work. So how would I display this correctly?
-
Ok I got the following code to check for doubles: Now is there a way to then display how many times those records are displayed in the database?
-
Well the thing is I wont be looking for a specific question, am just seeing if there are repeats and how many times it is repeated.
-
Is there a way to run a query that checks if a table has multipes of the samething and than print those out?
-
And for the questions, they are user inputed. So lets say user A asks: How was my day? and user B also asks: How was my day? I want a report saying that How was my day? has been asked twice.
-
Well what if I do how many times a keyword has redirected to a page? could that be simpler?
-
Alright so I have a script that takes in questions and stores them into a database. What I want to do is have like a Most asked question's report. Basicly I want to look trough the databse and if there are two questions that are the same I want to print it in a table with a number next to it identifying how many times it apears. Next I redirect the user based on keywords found in their questions which is also stored in the database. I then want to make a report on how many times each keyword apears in all of the questions in the databse. Where would I even start on this complicated journey?
-
SELECT * FROM question WHERE id IN(SELECT id FROM question ORDER BY id DESC LIMIT 10) ORDER BY id ASC But then I changed it to: SELECT * FROM question ORDER BY [id] DESC LIMIT 10 which then worked.
-
Alright I solved it by making the code look like this: echo "<tr>"; echo "<td>".$row['name']."</td>"; echo "<td>".$row['question']."</td>"; echo "<td>".$row['email']."</td>"; echo "</tr>"; But I get this: This version of MySQL doesn't yet support 'LIMIT & IN/ALL/ANY/SOME subquery' I am running MySQL 5.0
-
Thats exactly what I want to do. Also Maq I see what you are doing but once I run it gives me an error like: Parse error: syntax error, unexpected T_ENCAPSED_AND_WHITESPACE, expecting T_STRING or T_VARIABLE or T_NUM_STRING in /homepages/44/d272374679/htdocs/draft/admin/report.php on line 102 Don't you have to like put a \ infront of <td> to escape the php for that second?
-
Alright two questions how do I make PHP create my table and then how would I flip the order if I do SELECT * FROM question ORDER BY [id] DESC LIMIT 10 I don't want it to do 1-10 but rather 10-1.
-
I basically have a table in my database called 'question' with columns called 'name' 'question' 'email' Than I have a table in my PHP page: <table width="500" border="0" cellspacing="0" cellpadding="0"> <tr> <td>Question</td> <td>Name</td> <td>Email</td> </tr> <tr> <td> </td> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> </tr> <tr> <td> </td> <td> </td> <td> </td> </tr> </table> I want to show the last 10 question in my database along with email and names. Here is the code I have so far which just spits it out: $result = mysql_query("SELECT * FROM question") or die(mysql_error()); while($row = mysql_fetch_assoc( $result )) { echo "Name: ".$row['name']; echo " Question: ".$row['question']; echo " Email: ".$row['email']; } Than I want to make another page that just spit every row out in a table like the first one but that automatically expands as more entries are added. Where do I start?
-
I found it, I never included my variables for the original values. Wow am getting old or something...
-
Sorry about that, I will do it like that next time. I went back and did what you told me and got: Array ( [titlecolor] => #00FF00 [headercolor] => #00FF00 [headerlinks] => #00FF00 [navbackround] => #00FF00 [navlinks] => #00FF00 [leftside] => #00FF00 [footercolor] => #00FF00 [footertext] => #00FF00 [sumbit] => Submit ) It looks like the error is with the update code, but everything matches up and it should give me an error if it doesnt. Any guesses?
-
Here is my code: mysql_connect("$dbhost", "$dbusername", "$dbpassword") or die(mysql_error()); mysql_select_db("$databasename") or die(mysql_error()); $newtitlecolor = $_POST['titlecolor']; $newheadercolor = $_POST['headercolor']; $newheaderlink = $_POST['headerlinks']; $newnavcolor = $_POST['navbackround']; $newnavigationlink = $_POST['navlinks']; $newleftsidecolor = $_POST['leftside']; $newfootercolor = $_POST['footercolor']; $newfootertext = $_POST['footertext']; mysql_query("UPDATE style_options SET styleinput='$newheadercolor' WHERE styleinput='$headercolor' AND id = '1'") or die(mysql_error()); mysql_query("UPDATE style_options SET styleinput='$newnavcolor' WHERE styleinput='$navcolor' AND id = '2'") or die(mysql_error()); mysql_query("UPDATE style_options SET styleinput='$newleftsidecolor' WHERE styleinput='$leftsidecolor' AND id = '3'") or die(mysql_error()); mysql_query("UPDATE style_options SET styleinput='$newfootercolor' WHERE styleinput='$footercolor' AND id = '4'") or die(mysql_error()); mysql_query("UPDATE style_options SET styleinput='$newheaderlink' WHERE styleinput='$headerlink' AND id = '5'") or die(mysql_error()); mysql_query("UPDATE style_options SET styleinput='$newnavigationlink' WHERE styleinput='$navigationlink' AND id = '6'") or die(mysql_error()); mysql_query("UPDATE style_options SET styleinput='$newfootertext' WHERE styleinput='$footertext' AND id = '7'") or die(mysql_error()); mysql_query("UPDATE style_options SET styleinput='$newtitlecolor' WHERE styleinput='$titlecolor' AND id = '8'") or die(mysql_error()); echo "Update succesfull!<br />"; echo "<center>[<a href=\"editstyle.php\">Go BACK</a>]</center>"; The Update successful message comes trough but nothing really gets updated. Whats wrong here?
-
PERFECT! Thank you!
-
Well I want to make it simple for the user. Basically this is what I want to do: .css file #header { color: #003399; background: #8CA8E6; } And then my admin panel would have: Backround: ___________ SUBMIT Can this be done in .css? <?php $backround = "#8CA8E6"; ?> #header { color: #003399; background: <?php echo"$backround"; ?>; }
-
Basicly I have an admin panel where I want to edit certain colors in a .css file. Can I include PHP in the .css to make this work?