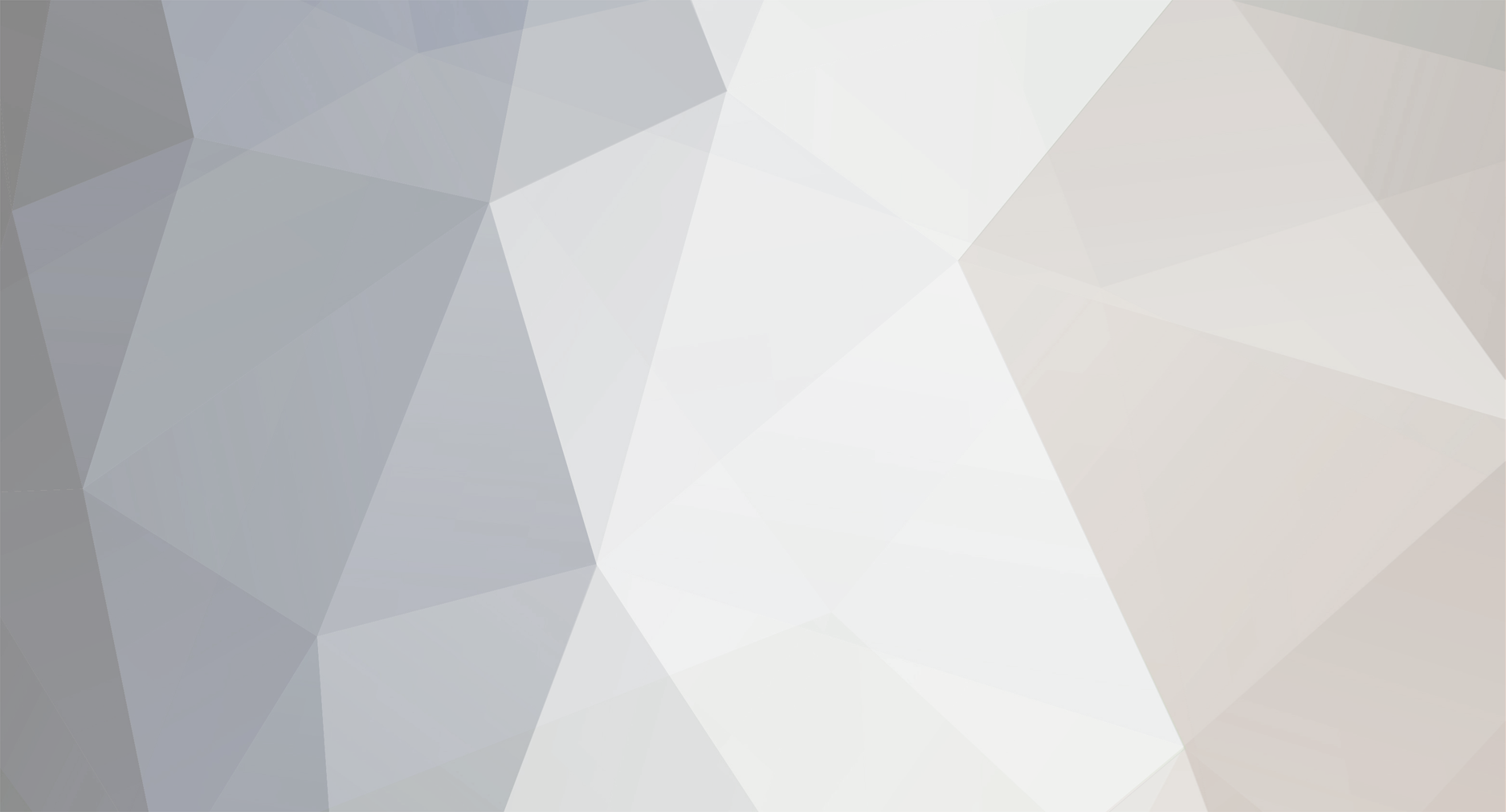
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
Escape the quotes or use single quotes $capa = "a:1:{s:10:\"subscriber\";b:1;}"; // or $capa = 'a:1:{s:10:"subscriber";b:1;}';
-
Building an Array from an Array, within a loop. Ideas?
Alex replied to monkeytooth's topic in PHP Coding Help
What does the data represent? Will each item be a separate record in the database or what? Here's an example of doing what you stated: <?php $text = <<<TEXT abc|def|ghi|jkl|mno|pqr|stu|vwx|ya abc|def|ghi|jkl|mno|pqr|stu|vwx|ya abc|def|ghi|jkl|mno|pqr|stu|vwx|ya abc|def|ghi|jkl|mno|pqr|stu|vwx|ya abc|def|ghi|jkl|mno|pqr|stu|vwx|ya abc|def|ghi|jkl|mno|pqr|stu|vwx|ya abc|def|ghi|jkl|mno|pqr|stu|vwx|ya TEXT; //$file = file('...'); $file = explode("\n", $text); $arr2 = Array(); foreach($file as $line) { $arr[] = $line; $arr2 = array_merge($arr2, explode('|', $line)); } print_r($arr); print_r($arr2); ?> -
The reason why it's not working the way you might expect is because "\n" != '\n'. '\n' will be the literal \n and "\n" will be a line break. $search = array("\r\n","\n","\r"); I doubt that'll work the way you intended though, it doesn't make much sense.. If you had: Text Text Text Text Text Text That would change it into: Text Text Text<p></p>Text Text Text edit: Whoops, forgot to post the solution to what I think you might be after.. I think you might be after something like this: $text = "Text Text Text\nText Text Text"; $text = preg_replace('~^(.+)$~m', "<p>$1</p>", $text); echo $text; Output: <p>Text Text Text</p> <p>Text Text Text</p>
-
[SOLVED] How to escape/ kill/ abort from within a function?
Alex replied to physaux's topic in PHP Coding Help
Oh sorry, I thought you wanted to terminate the entire script. If you just want to break out of the current function use return -
Create a link to previous page without using back button.
Alex replied to poleposters's topic in PHP Coding Help
You could use JavaScript, example: <a href="#" onclick="history.go(-1);">Back</a> You should also make sure that they actually came from your search page though, and they didn't just click a link like www.domain.com/users.php?id=1 -
You need delimiters. $phoneNum= preg_replace("~[^0-9]~", "", $phone);
-
array_unique
-
You can use a LIKE, and within a like you can use _ to match one character and % to match 0 or more, so something like this should do: SELECT column FROM table WHERE column LIKE '_8%'
-
[SOLVED] How to escape/ kill/ abort from within a function?
Alex replied to physaux's topic in PHP Coding Help
You mean like die or exit? -
move_uploaded_file, parameter 2 is the destination of the file (including what to name it)
-
You need to actually get the record from the mysql resource with a function such as mysql_fetch_assoc. You also are using an undefined constant on this line: $ekeep=$array[user_email]; An error might not be thrown depending on how high your error reporting is turned up, but it's still not good to do. $sql="SELECT user_email FROM $tbl_name WHERE user_login='$myusername' and user_pass='$mypassword'"; $result=mysql_query($sql); $row = mysql_fetch_assoc($result); $ekeep=$row['user_email']; setcookie("useremail", $ekeep, time()+60*60*24*100, "/", '.timingishstudios.com');
-
You have a couple of problems wrong inside of your foreach loop, and if you check the contents of $e I'm sure you'll see it's empty. Try this: if ($_POST['Submit']) { $e = array(); $para = $_POST['para']; $emails = preg_split( '@[ <>;:,\\\\/"{}*!?]@', $para ); foreach ($emails as $email) { if(empty($email) || stristr($email, '@') === FALSE) continue; $e[]= $email;//.'<br \>'; } foreach($e as &$v) { $v = preg_replace('%\.\s*[^\s\.]+\K\s+[^\.]+$%', '', $v); echo $v.'<br \>'; } } You should put the new foreach inside of the if(isset($_POST['Submit'])), so you don't get any errors if the form wasn't submitted. If you're using the continue keyword to skip an iteration there's no need for the else in the first place because if it skips the iteration it won't execute the below code anyway. Similarly you don't need to but a continue at the bottom of the foreach because it does that automatically. Finally, one other note is that you don't need to declare $e = array(); if you're using it as an array ($e[] = ..., it's optional.
-
You generally get that error when the first argument supplied is not an array, and you took out the only part of the code that would be useful for identifying your issue - the part where you actually defined the contents of the array. Please post the full code.
-
I'm sure there's a way to accomplish what you want, but it depends on your specific situation. Can you explain what you're trying to do a bit better? I understand you're trying to output an image then unlink it, but in what context?
-
PHP just sends the HTML to the browser, it doesn't interpret the HTML, so it doesn't load the image. That's the job of the browser. So the reason it's not working is because by the time the browser tries to load the image (the php script has already sent the output and stopped executing) the image was unlinked and does no exist anymore.
-
That's why you're supposed to use an ESD mat (Although, I doubt someone one buy one just for a single use), or at very least ground yourself to the machine by touching the power supply.
-
I feel although I might have extremely over-complicated it.. Maybe because I just donated blood a few hours ago I'm not thinking straight.. :-\ <?php $arr = Array( 'this is the string.checking for period. hello1 hello2 hello3', 'this is second string, want to delete. Hi Bye. Bye1 Bye2 3 4 5 6' ); foreach($arr as &$val) $val = substr($val, 0, - strpos(strrev($val), '.')) . ' ' . current(explode(' ', trim(substr($val, -strpos(strrev($val), '.'))))); print_r($arr); Output: Array ( [0] => this is the string.checking for period. hello1 [1] => this is second string, want to delete. Hi Bye. Bye1 )
-
Inside of a class ([ ]) ^ means 'not'. So [^a-zA-Z0-9]+ means anything that is not a-zA-Z0-9.
-
You should do some research about how functions work and how to utilize them, check out the manual. The way to use this function would be like so: function remove_nonAtoZ($input) { return preg_replace('~[^A-Z]~', '', $input); } echo remove_nonAtoZ("BA'3Ndf$%^A&*(nN)A");
-
~[^A-Z]~ will work. function remove_nonAtoZ($input) { return preg_replace('~[^A-Z]~', '', $input); } edit: In preg_replace you also need to specify the replacement, which you forgot.
-
That's not a good idea either, because JavaScript can be easily edited. It's not really an issue of communication, when the form is submitted there's only 1 request to the server, and one response. The amount of time that some quick PHP validation will take is insignificant.
-
The bottom line is that JavaScript can be disabled. Although JavaScript validation is often a nice touch, there should always be server-side validation in place just in case.
-
You can't exactly do that in PHP. You can in JavaScript, but if that's not what you're looking for you can use the $_GET superglobal with similar functionality. Example: <?php $var = isset($_GET['var']) ? $_GET['var'] : 'Not set'; echo '$var is: ' . $var; ?> <a href="?var=somethingelse">Click!</a>