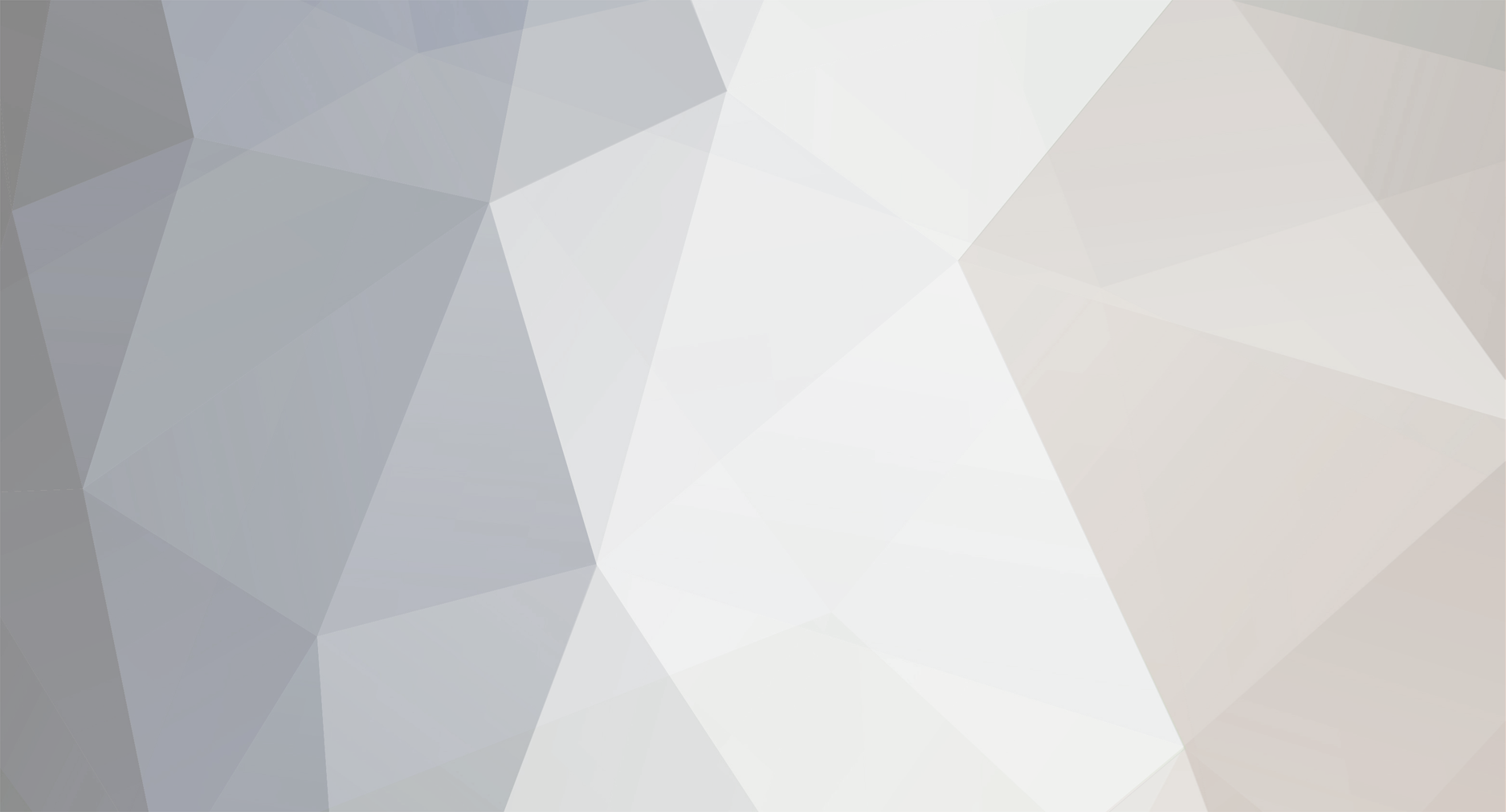
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
POST vars suddenly not working through jQuery based AJAX calls
Alex replied to jeffkee's topic in PHP Coding Help
Maybe you can find a solution here. It seems like exactly you're experiencing, and even if that's not your specific problem there's a lot of discussion in the comments for other possible causes and solutions to those causes. -
POST vars suddenly not working through jQuery based AJAX calls
Alex replied to jeffkee's topic in PHP Coding Help
Strange.. What exactly did the server upgrade entail? Was the PHP version updated? If so, from what version? For debugging purposes, you can try creating a new file that is completely blank besides printing out the POST data and seeing if you still have the problem with that file. That way you can rule out it being anything in your code that's causing this issue. -
Happy Birthday!
-
POST vars suddenly not working through jQuery based AJAX calls
Alex replied to jeffkee's topic in PHP Coding Help
What does start.php do? -
I like your idea to use array_reverse to order who goes first and who goes second. I knew my method for that was clunky and could have been done better, but are you using it correctly? In this code: function fight (&$warriors) { // figure out who goes first $w1s = $warriors[0]->get_stat('speed','current'); $w2s = $warriors[1]->get_stat('speed','current'); // check speed switch (true) { case ($w1s>$w2s) : break; case ($w1s<$w2s) : array_reverse($warriors); ... Are you sure array_reverse is doing what you intend? I don't think you can do it like that (you would have to do $warriors = array_reverse($warriors);) even though you passed $warriors into that function by reference. Maybe I'm wrong, or I'm just missing what you're doing entirely. But I thought you were doing something like this: function test(&$arr){ array_reverse($arr); print_r($arr); } $var = array('1', '2'); test($var); // Array ( [0] => 1 [1] => 2 ) Which you can see doesn't work.
-
Seemed like fun so I decided to give it a shot myself. Attempted an OOP approach. Took me just under an hour and a half. The output from mine could be formatted a lot nicer, I just got lazy on that aspect. There also isn't form validation and stuff like that, I just focused on the OOP design mostly. It was only an hour and a half so of course things could be nicer, but I don't think it's too bad. There could be bugs; I didn't really rigorously test it. Because the deadline for submitting this test to the potential employer has passed I don't see any harm in providing the code. If you want to attempt it on your own still, then just don't look <?php class Battle { private $_warrior1; private $_warrior2; public function __construct(Warrior $warrior1, Warrior $warrior2) { $this->_warrior1 = $warrior1; $this->_warrior2 = $warrior2; } public function start_battle() { $round = 0; /* If either's warrior's health is zero, the product of their healths will be zero. */ while($this->_warrior1->get_health() * $this->_warrior2->get_health() > 0 && $round < 30) { $battling_warriors = array($this->_warrior1, $this->_warrior2); if($this->_warrior1->get_speed() < $this->_warrior2->get_speed()) { $battling_warriors = array_reverse($battling_warriors); } else if(!($this->_warrior1->get_speed() > $this->_warrior2->get_speed())){ /* This is a bit ambiguous, the rules don't specify what happens if they have the same speed and defence, so we're assuming it won't happen */ if($this->_warrior1->get_defence() > $this->_warrior2->get_defence()) { $battling_warriors = array_reverse($battling_warriors); } } for($i = 0;$i < 2;++$i) { $damage = $battling_warriors[$i % 2]->attack() - $battling_warriors[($i + 1) % 2]->get_defence(); if($battling_warriors[($i + 1) % 2]->take_damage($damage)) { echo $battling_warriors[$i % 2]->get_name() . " has done " . $damage . " damage! " . $battling_warriors[($i+ 1) % 2]->get_name() . " has " . $battling_warriors[($i + 1) % 2]->get_health() . " left!<br />";; } else { echo $battling_warriors[($i + 1) % 2]->get_name() . " has evaded the attack!<br />"; } } ++$round; } } } class WarriorFactory { public static $types = array('Ninja', 'Samurai', 'Brawler'); public static function factory($type, $name) { if(in_array($type, self::$types)) { return new $type($name); } else { // error } } } class Warrior { /* Health, Attack, Defence and Speed are integer values between 0 and 100. Evade is a number between 0 and 1. */ protected $_name; protected $_max_health; protected $_health; protected $_attack; protected $_defence; protected $_speed; protected $_evade; public function __construct($name, $health, $attack, $defence, $speed, $evade) { $this->_name = $name; $this->_health = $this->_max_health = $health; $this->_attack = $attack; $this->_defence = $defence; $this->_speed = $speed; $this->_evade = $evade; } public function get_name() { return $this->_name; } public function get_health() { return $this->_health; } public function get_max_health() { return $this->_max_health; } public function get_speed() { return $this->_speed; } public function get_defence() { return $this->_defence; } /* Returns true on success hit, false on evade */ public function take_damage($attack) { if(rand(1, 100) <= $this->_evade * 100) { // attack blocked return false; } else { if($attack >= $this->get_health()) { $this->_health = 0; } else { $this->_health -= $attack; } } return true; } public function attack() { return $this->_attack; } } class Ninja extends Warrior { public function __construct($name) { parent::__construct( $name, rand(40, 60), rand(60, 70), rand(20, 30), rand(90, 100), rand(3, 5) / 10 ); } public function attack() { if(rand(0, 19) == 0) { echo $this->get_name() . " has double damage!<br />"; return $this->_attack * 2; } else { return $this->_attack; } } } class Samurai extends Warrior { public function __construct($name) { parent::__construct( $name, rand(60, 100), rand(75, 80), rand(35, 40), rand(60, 80), rand(3, 4) / 10 ); } public function take_damage($attack) { if(!($result = parent::take_damage($attack))) { if(rand(0, 9) == 0) { $this->_health += 10; echo $this->get_name() . " has gained 10 health!<br />"; } } return $result; } } class Brawler extends Warrior { private $_has_defence_bonus = false; public function __construct($name) { parent::__construct( $name, rand(90, 100), rand(65, 75), rand(40, 50), rand(40, 65), round(rand(30, 35) / 100, 2) ); } public function take_damage($attack) { if($result = parent::take_damage($attack) && $this->get_health() < 0.2 * $this->get_max_health() && !$this->_has_defence_bonus) { $this->_defence += 10; $this->_has_defence_bonus = true; echo $this->get_name() . "'s defence has been increased by 10!<br />"; } return $result; } } if(!isset($_POST['check'])) { ?> <form action="" method="POST"> <input type="hidden" name="check" value="1" /> Name <input type="text" name="warrior1_name" value="Warrior 1" /> Type <select name="warrior1_type"> <option value="Ninja">Ninja</option> <option value="Samurai">Samurai</option> <option value="Brawler">Brawler</option> </select> <br /> Name <input type="text" name="warrior2_name" value="Warrior 2" /> Type <select name="warrior2_type"> <option value="Ninja">Ninja</option> <option value="Samurai">Samurai</option> <option value="Brawler">Brawler</option> </select> <br /> <input type="submit" value="Battle!" name="submit" /> </form> <?php } else { $warrior1 = WarriorFactory::factory($_POST['warrior1_type'], $_POST['warrior1_name']); $warrior2 = WarriorFactory::factory($_POST['warrior2_type'], $_POST['warrior2_name']); $battle = new Battle($warrior1, $warrior2); $battle->start_battle(); } ?> Edit: I wish I had more to contribute to the thread question more directly, but all I can offer is speculation and secondhand anecdotal evidence. In many cases it seems like formal education and experience (learning it on your own, whatever) simply offer different things, but both can be valuable to fill in where the other missed out. I think the best option is to get a lot of experience in the field on your own, but to also get the formal education so that you're exposed to certain things that you might not have otherwise been exposed to. Personally, I've never had a formal education in anything computer related, besides a few high school courses on things unrelated to programming, and nothing was really learned, or taught for that matter, anyway. I've been programming for several years now completely self-taught, and I like to think that I've learned a lot, but I know there's still a ton to learn, and that's why this upcoming academic year I'm off to university to study computer science and mathematics, now that I've graduated high school.
-
Here's one way you can do it (the original problem, btw): $('p.box a.edit').click(function() { var p = $(this).parents('p.box'); $('form#editForm input#id').val(p.find('> .id').html()); $('form#editForm input#title').val(p.find('> .title').html()); }); edited twice for nicer solutions.. Can't think tonight.
-
This topic has been moved to Third Party PHP Scripts. http://www.phpfreaks.com/forums/index.php?topic=336743.0
-
is_numeric not returning true for 0 in form data
Alex replied to edpatterson's topic in PHP Coding Help
Sorry, I confused functions. The reason your code isn't working isn't because of the is_numeric() part at all, that's correct. The issue is here: if((isset($key)) && (!$value == '')){ namely the (!$value == '') portion. If $value == 0, then that statement will evaluate to false because 0 is evaluated to false, and !false == '' (or true == '') is false. Instead use: if((isset($key)) && $value != ""){ Alternatively, you could use: if((isset($key)) && !($value == '')){ Notice the position of the ! operator. -
is_numeric not returning true for 0 in form data
Alex replied to edpatterson's topic in PHP Coding Help
0 is different from "0" (string). Any data from a form will be a string. Instead of is_numeric which checks the datatype of the argument, you're looking for ctype_digit. I confused functions, nevermind. Solution in my next post.. -
Help with subtracting years from the time() function
Alex replied to Nightmareinhell's topic in PHP Coding Help
Well, that looks like the correct date. So the problem must be somewhere else and you haven't provided us with enough information to help. -
Help with subtracting years from the time() function
Alex replied to Nightmareinhell's topic in PHP Coding Help
Did you really run it again or just copy and paste the old result? That's not the formatting that should be returned at all. -
Help with subtracting years from the time() function
Alex replied to Nightmareinhell's topic in PHP Coding Help
That's really strange, both that the server time is off (and they won't change it) and that you're getting that result now. Are you sure it's still off by 10 years? Try this and tell us what output it gives back now: echo date("d-m-y H:i:s"); -
That's not exactly how you would achieve what you're looking for. Here's one way you can get the effect that you're after: When the page loads have all the current entries listed output through PHP, and store the ID, for example, of the most recent entry in JavaScript. Create a PHP file that given an ID will return any entries added after that (e.g. SELECT ... FROM table WHERE id > $last_id). Then you can periodically query that page via AJAX, get any new entries and update the JavaScript variable that holds the ID of the last entry being displayed.
-
From the starting value ($i = 0) what do you need to do to get to the next even number? Also, in the future please use [[/tt]php] or [[tt]code] tags.
-
Well, first off you need to define $i with the initial value of 1 outside the loop, otherwise it will reset to 1 every loop. Then you need to increment $i every loop (++$i): <?php $i = 1; while ($row = mysqli_fetch_array($result, MYSQLI_ASSOC)) { ?> <dl> <dt style="width: 190px;"><label for="answer[<?php echo $row['id']; ?>]"><?php echo $row['question'] ?></label></dt> <dd><input type="text" name="answer<?php echo $i ?>[<?php echo $row['id']; ?>]" class="answers[]" size="54" /></dd> </dl> <?php ++$i; } ?>
-
If I understand what you're asking.. You're never incrementing $i at the end of the loop, as I suspect you intended.
-
Are you getting any errors or what? You should have error reporting all the way up; put this at the top of the file: error_reporting(E_ALL);
-
It's not a PHP problem. Your issue is that when accessing the page from the other URLs the relative path for the JavaScript file gets messed up. For example, when accessing from another URL the file it tries to access is: http://www.rintlbd.com/update4.php/js/ckeditor/ckeditor.js Which isn't the right file. To fix this you can change it to an absolute path: <script type="text/javascript" src="/js/ckeditor/ckeditor.js"></script> /js/ckeditor/ckeditor.js instead of js/ckeditor/ckeditor.js
-
This topic has been moved to Miscellaneous. http://www.phpfreaks.com/forums/index.php?topic=336690.0
-
Using JavaScript, you can simply hide the form after it has been submitted and display a loading symbol or, simply a message, expressing that the file is being uploaded and to be patient.
-
You're never getting the data from the result. You need to do something like this: $result = mysql_query($pilotnum); $row = mysql_fetch_assoc($result); echo $row['max(pilotnum)']; Instead of echoing the query.
-
This topic has been moved to HTML Help. http://www.phpfreaks.com/forums/index.php?topic=336554.0
-
$_VERSION = new version(); I'm not sure that will give you an error, but in PHP 5 objects are passed by reference by default so you don't need the &.