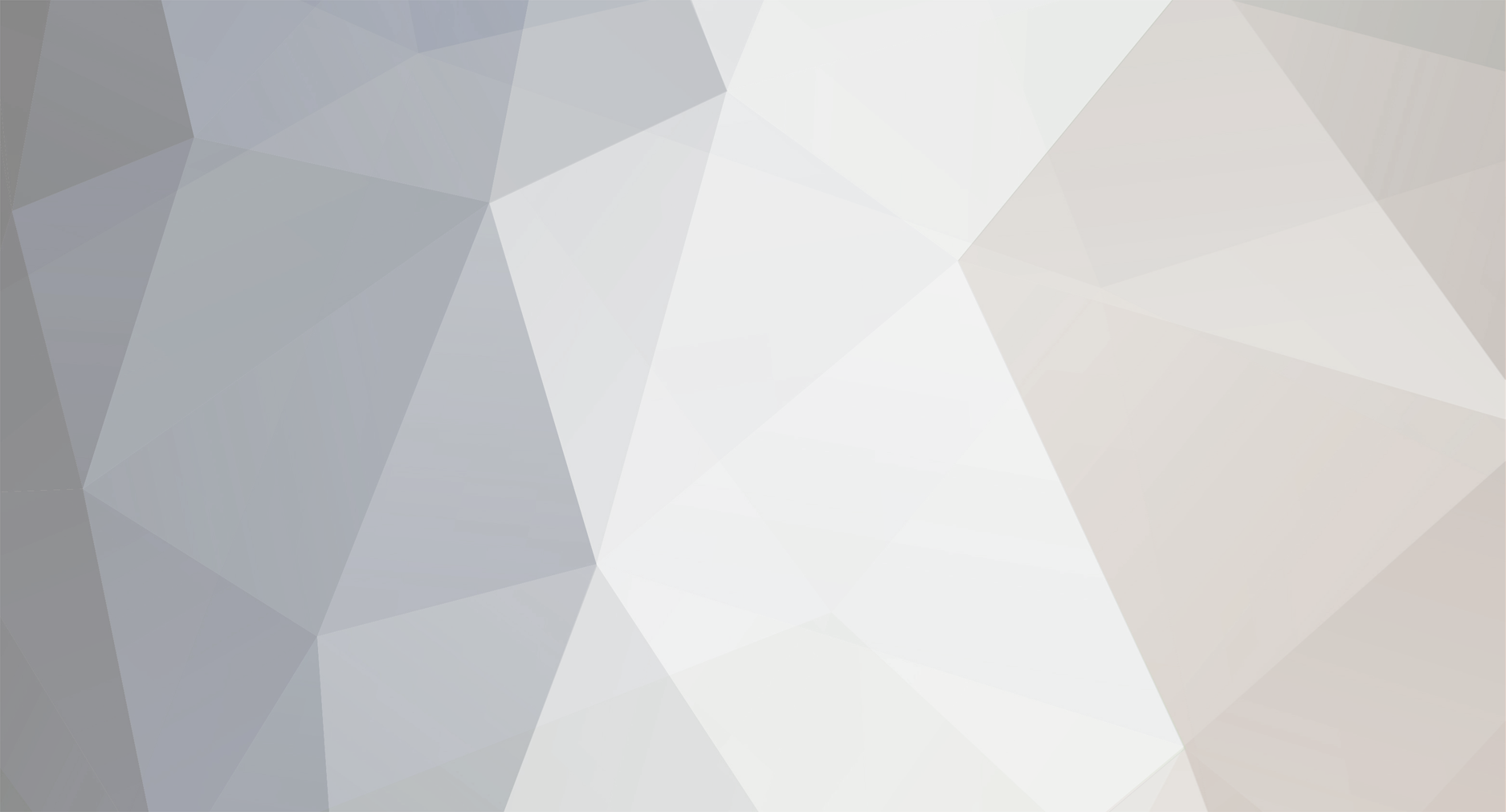
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
Sorry, I usually try to explain my solutions, as I was writing that I was about to go do something so I was kind of rushing.
-
I made an example, but I can't edit my previous post.. Anyway here it is: <?php header('Content-type: image/gif'); $original = imagecreatefromgif('http://www.google.com/intl/en_ALL/images/logo.gif'); //Create an image resource of your original image $gray = imagecreatetruecolor(imagesx($original), imagesy($original)); // Same size as original image imagefill($gray, 0, 0, imagecolorallocate($gray, 100, 100, 100)); // Fill the new image resource with gray imagecopymerge($original, $gray, 0, 0, 0, 0, imagesx($original), imagesy($original), 80); //Merge them together imagegif($original); ?> If you test that example out it'll output a grayed out version of the Google logo.
-
Yes, it's possible. Using PHP GD.First you'll need to create a new image resource using imagecreatetruecolor(). Then fill it with some gray color using imagefill(). Finally you'll merge your original image resource with the second using imagecopymerge() (utilizing the last parameter of that function to change the alpha transparency). If you need an example just ask.
-
I'm assuming you want something like this:? $numbers=array("1","2","3","4","5"); $numbers_not_wanted=array("3","5"); for($i = 0;$i < count($numbers);$i++) { if(in_array($numbers[$i], $numbers_not_wanted)) continue; echo $numbers[$i]; } Assuming I'm right in guessing (Because you didn't specify what you want..) the reason yours didn't work is because you were only comparing the current element of $numbers to one element of $numbers_not_wanted. If I'm completely wrong then tell me what you really wanted.
-
No problem. For now and future reference once your problem is solved remember to press the "topic solved" button on the bottom left.
-
[SOLVED] Getting Apache's log file data using PHP?
Alex replied to random1's topic in PHP Coding Help
Np, just press the "topic Solved" button on the bottom left. -
echo "<table width='100' align='left' border='1'>"; for($i = 1;$i <= 5;$i++) echo "<tr><td>" . $i . "</td><td>" . (pow($i, 2)) . "</td></tr>"; echo "</table>";
-
[SOLVED] News article - using the subject as a hyperlink
Alex replied to beerguts's topic in PHP Coding Help
First you'd get the article id from the URL using the $_GET super-global, then after securing the variable (with mysql_real_escape_string()) setting up a query to return the information from that article. Like so: $article_id = mysql_real_escape_string($_GET['article']); $result = mysql_query("SELECT news_title, news_body, news_id, DATE_FORMAT(news_date, '%D %b %Y') AS formattedRacedate FROM news WHERE news_id='$article_id'")or die(mysql_error()); $row = mysql_fetch_assoc($result); // Not a loop because it's only 1 record echo $row['news_body']; // The whole article You can then format it anyway you want. Preferably you should also preform a check first to make sure that an article by that id really exists, like so: $article_id = mysql_real_escape_string($_GET['article']); $result = mysql_query("SELECT news_title, news_body, news_id, DATE_FORMAT(news_date, '%D %b %Y') AS formattedRacedate FROM news WHERE news_id='$article_id'")or die(mysql_error()); if(!mysql_num_rows($result)) { //Article doesn't exist } else { $row = mysql_fetch_assoc($result); // Not a loop because it's only 1 record echo $row['news_body']; // The whole article } -
There's no normal reason that shouldn't be working. That's the correct syntax for getting a $_POST variable. I tested it, and it works.
-
~^[A-Za-z0-9]*$~ Edit: You don't need regex to check if a string contains only numbers and letters (Is alphanumeric), you could just as well use ctype_alnum(). Personally I'd use that. It would be much faster as well, not that it would matter if you're only using it once.. But running a benchmarking test shows that ctype_alnum is about 6-12 times faster.
-
[SOLVED] Getting Apache's log file data using PHP?
Alex replied to random1's topic in PHP Coding Help
I was able to do it this way: <?php $file = '../../Apache/logs/error.log'; //Change to your path $fp = fopen($file, 'r'); fseek($fp, filesize($file)-2500); $content = array_slice(array_reverse(explode("\n",fread($fp, 2500))), 0, 21); array_shift($content); echo "<pre>"; print_r($content); echo "</pre>"; -
People constantly overuse word press.
-
Using header() one of the headers you can send is essentially a redirect: header('Location: http://phpfreaks.com'); Would redirect to phpfreaks.com, just put there were you want to redirect.
-
My Mistake :-\ Use a empty() to make sure it has a value (And not that it was just submitted like you'd do with isset()). <?php require '/home/xxxxxxxxxxxxx/Includes/connect.php'; if(empty($_POST['Name']) || empty($_POST['Email'])) { echo 'Required fields missing'; exit; } /* Assign all variables passed from the form */ $client = mysql_real_escape_string($_POST['Name']); $email = mysql_real_escape_string($_POST['Email']); /* Sending email with information to us */ $to = "[email protected]"; $subject = "Quote"; $random_hash = md5(date('r', time())); $headers = 'From: kerry.nz'; $headers .= "\r\nContent-Type: multipart/alternative; boundary=\"PHP-alt-".$random_hash."\""; ob_start(); ?>
-
Firstly, for future reference please post all code either inside of [ code ] or [ php ] (without the spaces), just makes it easier to read. Now, here's how you'd apply what I just posted: <?php require '/home/xxxxxxxxxxxxx/Includes/connect.php'; if(!isset($_POST['Name']) || !isset($_POST['Email'])) { echo 'Required fields missing'; exit; } /* Assign all variables passed from the form */ $client = mysql_real_escape_string($_POST['Name']); $email = mysql_real_escape_string($_POST['Email']); /* Sending email with information to us */ $to = "[email protected]"; $subject = "Quote"; $random_hash = md5(date('r', time())); $headers = 'From: kerry.nz'; $headers .= "\r\nContent-Type: multipart/alternative; boundary=\"PHP-alt-".$random_hash."\""; ob_start(); ?> Usually using exit like that is a sign of bad logic and planning, but in less complicated situations like this it doesn't really matter.
-
You could use an else if Like so: if($_GET['password'] == 'somepass1') { echo 'Some text 1'; } else if($_GET['password'] == 'somepass2') { echo 'Some text 2'; } else { echo 'Wrong password'; } Tip: Brackets around conditional statements aren't required unless you're evaluating more than one expression between them. Since you're only preforming one echo in the above you could also do this (with the same exact effect). if($_GET['password'] == 'somepass1') echo 'Some text 1'; else if($_GET['password'] == 'somepass2') echo 'Some text 2'; else echo 'Wrong password'; That being said, just addressing your specific question about conditionals, I would actually do it another way; utilizing a PHP switch statement. Like so: switch($_GET['password']) { case 'pass1': echo 'Something'; break; case 'pass2': echo 'Something else'; break; default: echo 'Wrong password'; break; }
-
Well, in the code you posted you didn't even have an action set for the form (the action attribute for the form tells the form where to submit the data too.) Additionally you need to specify the method, which in your case would be post. Use this: <form action="somefile.php" method="post"> <table border="1"> <tr><td>Your Name </td><td><input type="text" name="Name" /></td></tr> <tr><td>Email Address</td><td><input type="text" name="Email" /></td></tr> <tr><td colspan="2"> <input type="submit" value="Submit for Quote" /></td></tr> </table> </form> Then inside "somefile.php" (Use a different filename if you want, just make sure to edit that in the form then). somefile.php: if(!isset($_POST['Name']) || !isset($_POST['Email'])) { echo "Required fields were left blank"; } else { echo "Name: {$_POST['Name']} <br />\n Email: {$_POST['Email']}"; }
-
The is used to get variables from the query string in the URL. Basic example: if($_GET['password'] == 'somepassword') { echo 'Text..'; } else { echo 'Incorrect password'; }
-
If you want to make sure before it's submitted you'll have to use JavaScript. Either way you will need to do PHP validation (Because people can turn off JavaScript). Here's an example of how to do it: if(!isset($_POST['Name']) || !isset($_POST['Email'])) { echo "Required fields were left blank"; } else { //Process as usual. } isset() Use this to check if the variables are set and aren't NULL.
-
http://shouldiusetablesforlayout.com/ Seriously. Tables are good sometimes, but for the most part they cause more problems than they're worth. You'd be better off learning alternative techniques that are more cross-browser friendly. You can start here: http://www.mardiros.net/liquid-css-layouts.html
-
Remove last line in a file PHP/Store all lines except the last one
Alex replied to ktsirig's topic in PHP Coding Help
If you use file() you can get all the data of a file into an array (each line has it's own element). Then using array_pop() you can remove the last element from that array. Finally if you want the contents of the file in a string rather than an array use implode(). $file = file('somefile.txt'); array_pop($file); $content = implode("\n", $file); -
Need a good book that has pagnation examples in it
Alex replied to Redlightpacket's topic in PHP Coding Help
Well, I don't know any books, but if you haven't already you might want to check out a nice pagination tutorial made by Crayon Violent, http://www.phpfreaks.com/tutorial/basic-pagination -
store php in mysql and then execute it? is thi possible?
Alex replied to work_it_work's topic in PHP Coding Help
It's often not a good idea because if just anyone can enter whatever they wish into the database it would be very easy for them to insert something maliciousness that could cause problems. If access to inserting things into the database that will be processed by eval() is limited and not public it would be fine, in that it probably won't cause any problems, just not necessarily a good practice. To give you a suggestion for an alternative I'd probably need to know more information. -
store php in mysql and then execute it? is thi possible?
Alex replied to work_it_work's topic in PHP Coding Help
It's possible, although in most cases it's not a good idea and there's an alternative solution. To execute a string as PHP anyway use eval()