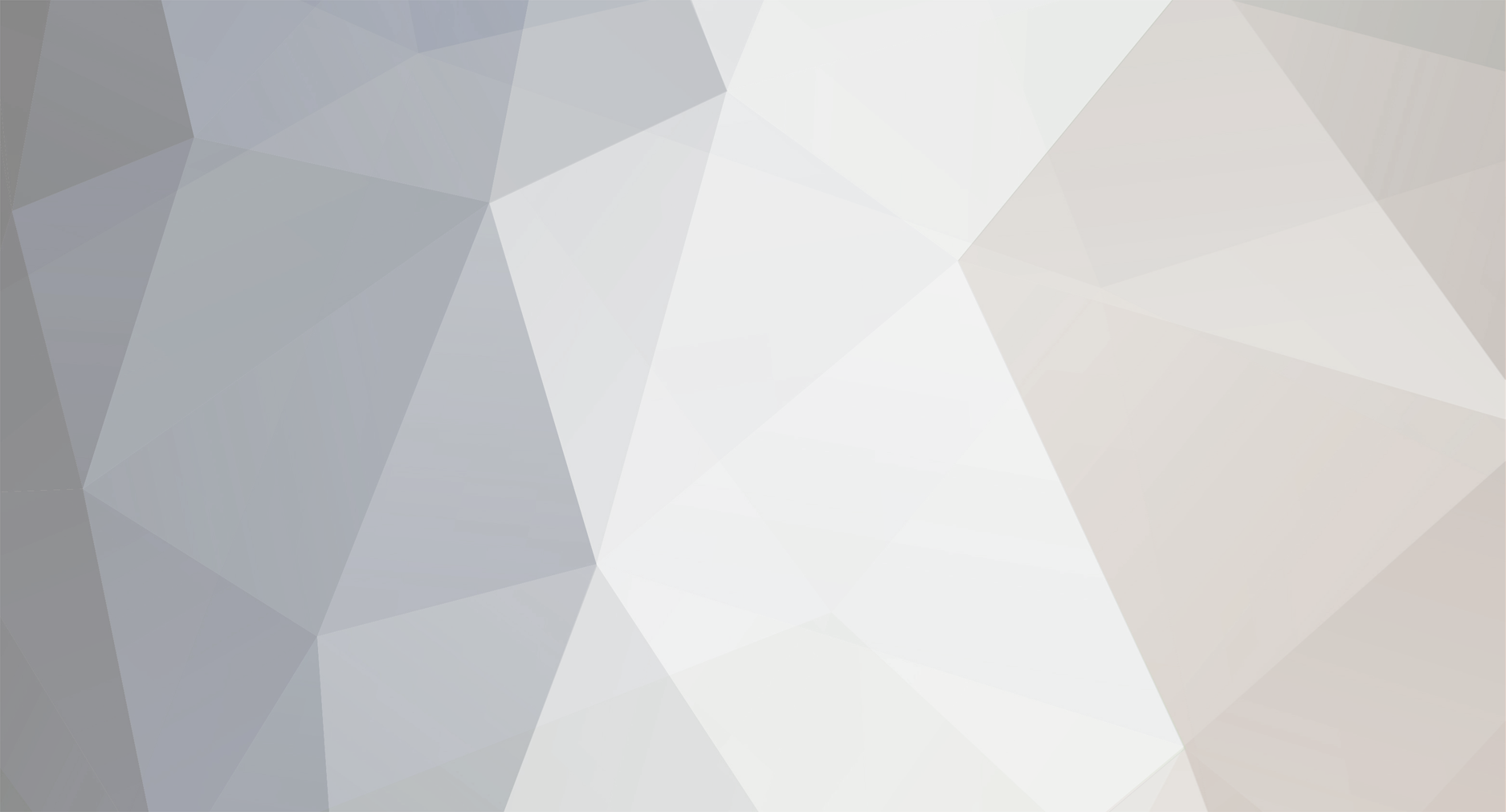
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
The full details of U.S. phone numbers are available at the North American Numbering Plan Administration website: http://www.nanpa.com/ The basis are as Psycho stated: 10 digits (although some people can still get away with dialing only seven digits to reach someone in their own area code) - 3-digit area code, 3-digit exchange, and 4-digit number. The area code can't start with 0 (zero) or 1 (one); and certain combinations are reserved. The 1- you often see at the begining of a 11-digit number is used to indicate a long-distance call (toll or toll-free). It is not part of the assigned number. All numbers in the NANP are 10 digits.
-
session_start() sends a header. You can NOT send any header() after sending output to the browser. The error message says the output started at line 14 of db_connect.php. The db_connect.php code you posted only has 8 or 9 lines, so there must be something after the closing PHP tag ("?>"). Anything after that tag will be sent as output to the browser. If you have blank lines there, you need to remove them. If fact, I don't event put the closing PHP tag in my include files (unless there actually IS HTML to be sent to the browser). PHP is smart enough to know that the end of the file is the end of the PHP in the file.
-
Could not open stream from different folder
DavidAM replied to thientanchuong's topic in PHP Coding Help
include('.../models/database.php'); should only be TWO DOTS not THREE. But that's not going to fix it. When using relative paths for include (the ../) it is relative to the SCRIPT THAT IS RUNNING not the file doing the include. If the running script is admin/addNewCategory.php then the include would be include('models/database.php'). Of course using relative paths can be troublesome. Once you fix it as above, if you later try to include the controller from, oh say "admin/secret/hideFromUser.php", then the relative path would need to be include('../models/database.php'); which would then cause the other script to fail. I usually define an set of constants in my bootstrap (or other common include file) that has absolute references and then use the constant for all includes: bootstrap.php define('C_PHP_INCLUDE_DIR', '/path/to/include/base/');all php scripts include('./bootstrap.php'); include(C_PHP_INCLUDE_DIR . 'models/database.php');Then I put a link (not a copy) to the bootstrap file in all execution directories. This way, I can maintain the absolute paths in a single file and all code uses the same path. -
You are looping through the 36 columns and trying to insert the entire row each time. If you get the INSERT working, you will be inserting the same data 36 times for every (valid) row in the file. As Barand has said: you are missing the closing parenthesis for the VALUES clause, and you are using a semi-colon instead of a comma between the data elements. If you are going to use IMPLODE (which IS the recommended way to do this), you do NOT need the FOR loop. $sql = "INSERT IGNORE INTO " .$table. "VALUES (" . implode("','", $data) . ")";
-
Need Help with Cubecart/SQL Database Error Code!
DavidAM replied to mangodesign's topic in Applications
The error message in your last post, still shows the query WITHOUT a value supplied for the LIMIT. This is causing the mySQL error. Most likely, this error is causing the PHP error about the invalid argument to foreach. We don't know because you have not posted any code. You were asked to show the code, but you haven't. The error message says the error occurred at line 1082 in functions.inc.php. If we could see the 10 or 20 lines before and after that line of code, we might be able to say WHY the query is incomplete. In post # 10 you executed the query in phpMyAdmin (or whatever). THAT QUERY IS CORRECT, you have a value for the LIMIT. The query itself did not return any rows, which means there is no data in the database that matches the query. So you may have two problems here: 1) the query is not written correctly, or there's no data; and 2) you are not assigning a value IN THE CODE (that we can not see) to the LIMIT CLAUSE. -
Need Help with Cubecart/SQL Database Error Code!
DavidAM replied to mangodesign's topic in Applications
The foreach error is likely caused by an attempt to iterate over an array of data you thought was returned, when in fact, the query failed and no data was returned. Your SQL string is incomplete. There needs to be a numeric value (or two) after the LIMIT keyword - to specify the limit. i.e. LIMIT 10 will limit the result to 10 records. -
This IF (elseif) will be FALSE if the "/" is the first character. strpos returns the position of the found character and the first position is 0 (zero) which will evaluate to false. You would needelseif(strpos($_POST['firstname'], '/') !== false) (yes, two equal-signs after the bang).
- 10 replies
-
- special
- characters
-
(and 3 more)
Tagged with:
-
fsockopen() and fgets() is slowing page load almost 3mins
DavidAM replied to pramodh's topic in PHP Coding Help
Moved to PHP Coding Help forum. Please use code tags (the <> button in the editor or ) when posting code. I would recommend using curl instead of managing the socket yourself. When do you have performance problems? GET or POST How is the function being called and under what circumstances? $fp is a resource and is not a valid target of foreach. Why did you take out the while statement? You need to turn on error reporting so you can see the errors, warnings and notices. Fix them all.- 1 reply
-
- fsockopen()
- fgets()
-
(and 1 more)
Tagged with:
-
Numerous addition and subtraction elements in one query
DavidAM replied to Steve_NI's topic in MySQL Help
The quotes in that message are surrounding the part of the SQL that it had a problem with -- Line 1 refers to line 1 of the query (it's all on one line, so that is not helpful). Your query appears to be missing a closing parenthesis at the end. So the quotes in the error are referring to the nothing it found when looking for that closing parenthesis. Here is what your query looks like - I split it out so the groupings are more visible: Select Sum ( (Select SUM(Balance) from account) - (Select sum( (select sum(Balance) from credit_card) - (select sum(Amount) FROM bills where Date > 12) ) ) -
777 is "world writable", which means anyone who can logon to the server can read or write the file. This setting is NOT recommended even on private servers, because it means any user you now have or add in the future has full access to the file. It is NOT Best Practice. Since the issue was viewing the file, the web-server already has write access to the directory. To be able to view the file, you only need to set the READ flag, so 444 (on the file) would allow the world to read it. You do NOT need write permission on a file in order to DELETE it -- deleting a file is a WRITE to the directory. Since we are talking about images here, unless you are planning to modify the uploaded file, no one needs write access to it; and since it is not a program, no one needs EXECUTE access. Uploaded files are going to be owned by the effective userid of the web-server, NOT the site owner/administrator (usually). Since any website access to the file will use that same userid, you could go with 400 on the file, if you want to be strict.
-
Because of Operator Precedence (operators.precedence), the original code (with respect to $query) is equivalent to: $left = "SELECT * FROM $_DB[DIR] WHERE ( `flags` & ". DF_DIR_MADE; $right = DF_V5 ." ) = ". DF_DIR_MADE ." ORDER BY `id` ASC;"; $query = $left | $right; which produces the results you saw.
-
JOIN Select all the data you need from both tables in a single query: SELECT title, content, p.id, imageUrl FROM posts AS p LEFT JOIN postImages as i ON p.id = i.postid -- YADA YADA YADA The LEFT JOIN will ensure you get POSTS even if they do not have an associated image. Leave LEFT out of the JOIN if you only want posts that have images. The problem with this approach is that any POST that has more than one image associated with it, will be in the resultset multiple times (once for each image).
-
Making radio buttons sticky in a foreach array loop
DavidAM replied to sh89's topic in PHP Coding Help
Please use tags. For multiple radio buttons, the HTML name attribute needs to represent/produce an array. name="magtype[]". In your loop, you need to check for the value in the array: //Creating foreach loop for magazine selection foreach ($magSubs as $mag => $SUB_PRICE) { echo "<input type='radio' name='magtype[]' value='$mag' "; if (isset($_GET['magtype'])) { if (in_array($mag, $_GET['magtype'])) { echo "checked='checked'"; } } echo " >$mag $$SUB_PRICE per month<br>"; // WHAT IS ^^ THIS? Is that supposed to be a variable-variable? } -
Why run the same query twice. Just build the table in a variable, then echo and send the same variable: $table = "<table border='1'> <tr> <th>Date</th> <th>Time</th> <th>Machine</th> <th>Action</th> <th>User</th> </tr>"; while($row = mysql_fetch_array($result)) { $table . = "<tr>"; $table . = "<td>" . $row['Date'] . "</td>"; $table . = "<td>" . $row['Time'] . "</td>"; $table . = "<td>" . $row['Machine'] . "</td>"; $table . = "<td>" . $row['Action'] . "</td>"; $table . = "<td>" . $row['USER'] . "</td>"; $table . = "</tr>"; } $table . = "</table>"; echo $table; $msg = $table;
-
Missing $ on the array subscript. You have the same problem in both echo statements. Turn on error reporting, these statements should be issuing errors (notices) about an undefined constant.
-
Are you sure it is running as CGI? Make sure that the user running the webserver has write access to the log directory, so it can create the log file. Again, check the Apache error and access logs to see if it is reporting any errors there.
-
Have a look at the Apache Error/Access logs. If I remember correctly, you can only set PHP flags/values in the .htaccess if you are running PHP as an Apache module. Those settings are throwing an Apache 500 error. If you are going to use CGI, change the setting in the php.ini file.
-
The (mySql) database is completely separate from the PHP process. Unless you are using an Asynchronous Query (which I do NOT recommend), PHP has already retrieved ALL of the data and the database is done with the query. Unlike MS Access, there is no concept of the "current row". You will need to include the row's unique identifier in then original query, and the issue the update using that identifier (as in your example above). Note that executing queries in a loop is resource intensive. If you have to do it, then you have to do it. But the Relational Database is designed around SETs (of data). If you can express the conditions of the updates in SQL, you would be better off using an UPDATE statement to update multiple rows at a time rather than walking the entire table. For instance: UPDATE Employees SET Salary = Salary * 1.05 WHERE IsActive = 1 AND IsManager =1 followed by UPDATE Employees SET Salary = Salary * 1.01 WHERE IsActive = 1 and IsManager = 0, would give everybody a raise -- a 5% raise to the managers and a 1% raise to the others.
-
PHP - MySQL field string manipulation and value replacement
DavidAM replied to RCurtis's topic in PHP Coding Help
I'm not sure that will work if "1" is the ONLY value in the column (there's no comma to match). If I were stuck with this data, I would either use the explode/implode sequence: $list = '1,3,5,2,8,10'; $list = explode(',', $list); if (($sub = array_search(1, $list)) !== false) unset($list[$sub]); $list = implode(',', $list); OR add a comma to the beginning and the end of the string and then replace ",1," with ",", and then trim the leading/trailing commas: $list = '1,3,5,2,8,10'; $newlist = ',' . $list . ','; $newlist = str_replace(',1,', ',', $newlist); $newlist = trim($newlist, ','); -
Is $this->db->query() a custom method of a custom database class? In the mySql extensions, the query() method returns a resource (or object) that must be used with one of the fetch_*() functions (or methods). The "undefined offset 1" error is because list($number,$sum) = $this->db->query('SELECT COUNT(*), SUM(amount) FROM dc_donations'); // Is essentially the same as: $temp = $this->db->query('SELECT COUNT(*), SUM(amount) FROM dc_donations'); $sum = $temp[1]; $number = $temp[0]; (yes, it assigns from right to left). So the error means that method (query()) is NOT returning an array with two elements.
-
PHP - MySQL field string manipulation and value replacement
DavidAM replied to RCurtis's topic in PHP Coding Help
Fix your database design! Normalize the table so you do NOT have multiple values in a single column. There should be a parent table and a child table with a one-to-many relationship. Then the "update" you want to do becomes a simple DELETE statement. Otherwise, you will need a query (SELECT), a for loop, explode(), array_search(), implode(), and another query (UPDATE). -
Getting file sizes for files in another directory
DavidAM replied to Zanzibarjones's topic in PHP Coding Help
It would be the path they navigate to. The code you showed does not provide enough information about where the filenames came from or even what is in the array. If you are using glob or readdir, you will need to prepend the same path you gave those functions to the filename you are checking. Is your $namehref variable getting the correct value? If so, maybe you want to use the same thing you did there. Also, your calls to filemtime should be having the same problem as the call to filesize. -
Do I need to escape the return of mysqli_error()?
DavidAM replied to Mig21's topic in PHP Coding Help
It depends on the application and the audience. In general, you log the error message, and any pertinent information (such as date-time, page, user input, etc) that might help you determine the cause and fix the problem. You review the log periodically. Since the log is a file, if you are reviewing it in a text editor, you do NOT want the HTML escaped -- you want to see the raw data. If you write a browser-based front-end to review the log, you would escape the data AS YOU WRITE IT TO THE HTML PAGE (NOT THE LOG). This way, you will SEE what the user entered NOT the escaped encoding. If you are talking about an in-house application where the users might actually call you while the message is displayed, you MIGHT choose to display the error. IF you display it, you WOULD escape it WHEN YOU WRITE IT TO THE PAGE. However, even in this case, I would write it to a log and display a "There was a problem" message. It is my experience that users do NOT read error messages anyway, even when they immediately call me, the answer is always "I didn't read it". Write it to a log, and when they call you, you can open the log to see the message. Truth be told, you WANT to see the error message yourself; all users interpret or paraphrase messages when they read them, or skip words, or read them wrong, or make them up so they don't look dumb for not having read it. -
Getting file sizes for files in another directory
DavidAM replied to Zanzibarjones's topic in PHP Coding Help
blahblahblah is throwing the error on line blah. Have a look at that file and that line (and a couple of lines before). You could debug this by printing the $file value when $size becomes false (i.e. the call failed). Most likely, the problem is the path. filesize requires the full path of the file to be checked. If a relative path (or just a filename) is provided, it will look in the current working directory. It might also be a permission issue, but since only you saw the error message, we can't really say. Note: When posting error messages on this site, post the entire message. You can X-out any sensitive information if you want. In my experience, PHP has very few (if any) cryptic error messages, if you read the message it tells you what, when, where and why. -
For completeness: When doing comparisons you use two equal-signs (or three for strict comparisons). A single equal-sign does an assignment. So the statement above is assigning $username to the $customer->username property and testing its value. It will always be true unless $username is empty or otherwise evaluates to false (zero, null, false). if ($customer->username == $username)