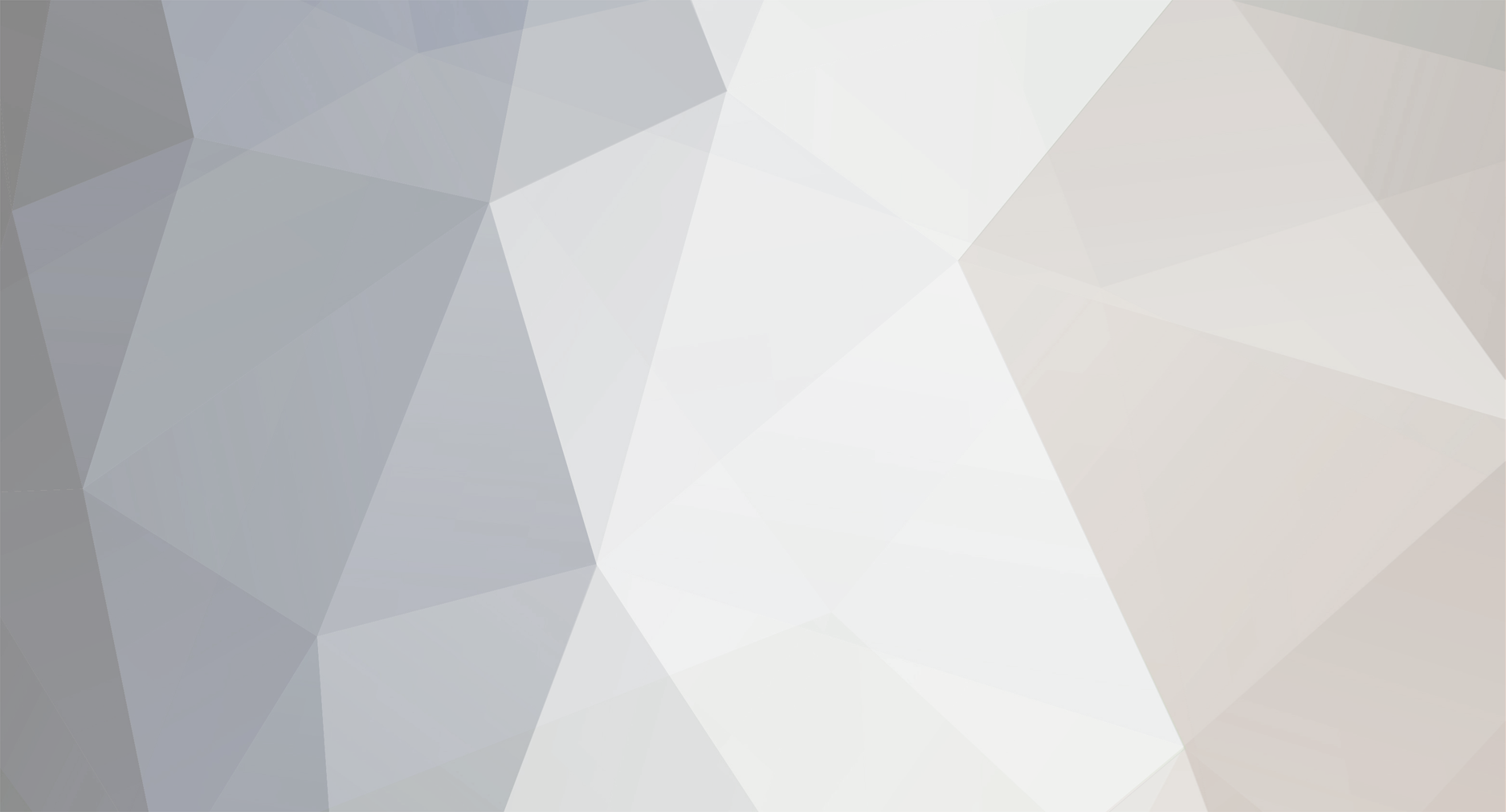
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
1) Just store the unique formid, and make it a key on the table 2) When the user clicks the link and lands on your page, lookup the formid ($_GET['formid']) in the database and retrieve the creation date 3) If the current date is more than 28 days (or whatever) display a message saying the survey has expired (or whatever). 4) If the formid does not exist in the database, display a message saying it has expired or was entered incorrectly. You will probably want a cron job to delete old entries. That all depends on how much space you have and how many links are generated and what data is important to you. Or you might want to track whether the user responded to the link and maybe when so you can determine if 28 days is too much or too little or just right. EDIT: Forgot to mention: I do not think there is any way to affect the email in the user's mailbox. They are going to be stuck with an expired formid or a stale link. That's why I suggested #4 above, atleast if they click it next month, they get your website.
-
Are you saying you have a table like this: Notes ===== Apple Raisin Banana Apple, Raisin Apple, Banana Mango, Apple and you want to remove the word apple from the notes field leaving the rest of the field as is? Notes ===== <BLANK> Raisin Banana , Raisin , Banana Mango, If so, you need to use the REPLACE function UPDATE locations SET notes = REPLACE(notes, 'Apple', '') WHERE notes LIKE '%Apple%'
-
limit users to only 3 logins using php mysql ;ogin system?
DavidAM replied to rgriffin3838's topic in MySQL Help
First, you need to review your code. You have a block to "protect MySQL injection" but it is AFTER you have already sent the POSTed data to the database. Kinda like closing the barn door after the horse got out. For the check you asked, you have already seleted the login counter (using SELECT *) so I would test it like this: if($count==1) { // Found Only one record with user/password - cool $row = mysql_fetch_array($result); if ($row['nmbrlogin'] >= 3) { echo "Sorry you have already logged in 3 times. If this is a mistake please contact customer support."; } else { // I wouldn't send the password again, but that assumes username is unique (which it should be) $query="UPDATE members SET nmbrlogin=nmbrlogin+1 WHERE username='$myusername'"; $result1=mysql_query($query); // Register $myusername, $mypassword and redirect to file "tilt_activate.php" session_register("myusername"); // I would NOT put the password in the session. Sessions are stored in a file system somewhere and are probably world readable. //session_register("mypassword"); header("location:URL.php"); exit(); // Don't load the rest of the page } } else { echo "Wrong Username or Password"; } You could do it in the WHERE clause of the SELECT, but then you can't tell the user why you rejected their login. -
You have an extra closing brace in the if (empty ... part, which closes the if(isset ... part and orphans the else (at the bottom). I don't see how that produces the results you indicate, but it does need to be fixed. <?php if(isset($_POST['submit'])) { if (empty($_POST['name']) || empty($_POST['phone']) || empty($_POST['email']) || empty($_POST['message']) ){ header( "Location: form-error.php"); } } // <--- This closes the "if(isset" above which will cause the form to send mail even if not posted You should turn on error reporting to see what other errors you might be getting. Place this inside the first openning tag (<?php) in every PHP file: error_reporting(E_ALL); also, add exit() after the header() call. That will prevent the remaining code from executing while the browser receives and processes the header you sent. Without it, it is possible the page might send the email after sending the header(). You should also check to see if $_POST['check'] exists before looping through it: $check_msg = ''; if (isset($_POST['check'])) { foreach($_POST['check'] as $value) { $check_msg .= "\n$value\n"; } } Post any error messages you get along with the updated code and we'll see where we are.
-
[SOLVED] Problems wiht Time Out Script.
DavidAM replied to overlordofevil's topic in PHP Coding Help
That means that something was sent to the browser between the session_start() and the header() calls. It could be blank space in the include file. Make sure the openning tag (<?php) in the include file starts in the first position of the first line; also make sure there is no carriage return (or other white-space) after the closing tag (?>) (some people just leave the closing tag out all together). By the way, you are referring to $_SESSION['ses_time']; unconditionally at the beginning of the script. If this is your actual code, I foresee problems when the user first visits the site (with a brand new session). That element of the array will not exist, there will be another notice and the variable will evaluate to zero, which will cause your page to timeout. -
This will probably require an array formula (sometime called "control-enter" formulas). If you can post the layout of your data (column headings and a couple of rows), I might be able to help.
-
It looks like you are moving the file twice. move_uploaded_file() will MOVE the file from the temporary location that it was uploaded to, so it is not there the next time you call move_uploaded_file(), and so you get an error. Also, you are creating a $target value, but not using it anywhere. To streamline the code, I would do something like this (this is an example): // You don't really need basename() here, the name only contains the filename (with extension) (I think) $target = basename($_FILES['uploaded']['name']); // Assuming $fileDest is the base directory for your images // AND Assuming it already has the / on the end of it $dest = $fileDest . $partNumber . '/'; // Make the PartNumber directory if it is not already there if (!is_dir($dest)) { mkdir ($dest, $mode = 0777, $recursive = false); } // Move the uploaded file if (move_uploaded_file($_FILES['uploaded']['tmp_name'], $dest . $target )) { echo "File uploaded!"; } else { $errMsg .= 'An unexpected error has occured. Please try again and contact the Information Management Systems Administrators if the problem persists.'; } You may have to check to see if the destination file ($dest . $target) already exists before you try to move it. If it does, you may want to use unlink() to delete it. Or perhas rename it to *.old (in case the move fails, you can then rename it back).
-
any way to ignore a value from $result = select * from 'tableName'; ?
DavidAM replied to schalfin's topic in MySQL Help
How about: select id, entry_date, entry_text from myTable WHERE entry_date < (SELECT MAX(entry_date) FROM myTable) If you don't want certain data, tell the database not to send it. Don't try to code around it. Of course, this will ignore ALL rows that have the greatest entry_date. If two rows end up with the same entry_date, they will both be ignored. You could use select id, entry_date, entry_text from myTable WHERE id < (SELECT MAX(id) FROM myTable) if the id column is an auto-increment column. It's not best-practice, but it produces exactly the result you specified. -
Your search string "WIX 24061" does not have a comma in it, so the explode is not being executed. Shouldn't you change the strpos() and explode() to be looking for a space? if(strpos($_SESSION['search'], ' ') !== false) { $parts1 = explode(' ', $_SESSION['search']); Or did I misunderstand the issue?
-
[SOLVED] php throwing off my javascript function
DavidAM replied to justAnoob's topic in PHP Coding Help
In your "working" example, there are quotes around the name. But when you changed it to the echo, they got left out. Don't you need them so JS will see the string rather than a variable name? echo '<a href="#" onclick="clicked(\'' . $row['item_name'] . '\')">' . $row['item_name'] . '</a>'; -
[SOLVED] IF Statement & Two Tables With Different Echoes
DavidAM replied to EternalSorrow's topic in PHP Coding Help
In the first snippet, the reason that you don't get anything for the second table is because '$table2' will never be defined from the query. Change that IF statement to test $table1, and it should work. if (isset($table1) && $table1 == 'people') { echo '<li><a href="biography.php?name='.$name.'"><img src="images/thumbnails/'.$img.'.jpg" alt="" title="'.$name.' ('.$occupation.')"></a></li>'; } I do not see why the second snippet is not working. ... OHH I SEE IT ... if ($result['table1'] == 'film') should actually be if ($row['table1'] == 'film') Same for the second IF. By the way: 1) Since you are using extract(), you don't need to use $row[], you can just refer to that as $table1. 2) If you are going to use extract(), you really should use mysql_fetch_assoc() instead of mysql_fetch_array(). The mysql_fetch_array() returns an array that is keyed by column names AND by numeric indexes, so you get something like this: array( 0 => titleValue, 1 => imageValue, 2 => idValue, 3 => occupationValue, 4 => yearValue, 5 => table1Value, 'title' => titleValue, 'image' => imageValue, 'id' => idValue, 'occupation' => occupationValue, 'year' => yearValue, 'table1' => table1Value) in $row. The numeric keys can create problems (or at least used to create problems) when using extract(). -
[SOLVED] Need help importing data from txt file
DavidAM replied to jim.davidson's topic in MySQL Help
I've never done this, and I have no test environment here at work, but, since you are on a Windows platform, do you need to specify that the LINES TERMINATED BY "\r\n"? -
[SOLVED] IF Statement & Two Tables With Different Echoes
DavidAM replied to EternalSorrow's topic in PHP Coding Help
Unless I am terribly mistaken (I'm at work and I don't have a system here to test with), a UNION only uses the column names from the first query. In the subsequent query (or queries) the columns are taken in the same order as the first. So in your query: <?php $query = "SELECT title, image, year, id, 'film' AS table1 FROM film UNION ALL SELECT name, img, id, occupation, 'people' AS table2 FROM people"; The rows returned will be named: title, img, year, id, and table1 (in that order). When you get a row from the people table, the data from column name will be in title, data from img will be in image, id will be in year, occupation will be in id and table2 will be in table1. Some of those pairings for columns look like they will have different datatypes; specifically, occupation and id. This may be causing a problem on the server side; although the "or die" should be reporting the error. You should re-arrange the second query to line up the columns better. If you want different column names, you can use NULLs to fill columns that do not apply to one query or the other: <?php $query = "SELECT title, image, id, NULL AS occupation, year, 'film' AS source FROM film UNION ALL SELECT name, img, id, occupation, NULL, 'people' FROM people"; in this way, film.title and people.name will end up in the same column (whose name will be title). Likewise film.image and people.img will be in the same column (named image). There will be a column named source that will identify the row's source table. The occupation column will always be NULL (empty) for a row from the film table; and year will always be NULL for a row from the people table. The ORDER BY should be affecting the entire UNION (although, as stated above, the id column may not be what you think it is in your query). As for the LIMIT phrase, I think it applies after the ORDER BY (so it applies to the UNION'd results), but I can't test it right now. I do not see why you are getting a blank page, though. It looks like you should get something. Can you post the code you are actually using now? -
See the blank lines outside of the php tags? ?> <?php Those are sent to the browser. So you cannot send any more header information to the browser because you have already sent content. Remove the blank lines. In fact, there is no reason to close and re-open the php tag, so remove them too.
-
It's not real clear where your problem lies. which script is storing the value and which script is not seeing it? When I did this it seems like I ran into a problem because both scripts could be executing at the same time. If the main script has not finished before the browser requests the captcha script, the session has not been updated and the captcha script does not see it. I resolved this problem by putting everything into the session variables and calling session_close() BEFORE starting the output to the browser. That way the session data is written to the session file and the captcha script can get it when it calls session_start().
-
Let's say your query returns three rows, with ID's of 4, 9, and 16. The checkboxes will have HTML names of: name="completed[4]" name="completed[9]" name="completed[16]" If we check the first two boxes, then the posted array will look like this (I'm at work and do not have a PHP engine to play on, so pardon any typos): $_POST => array ( completed => array ( 4 => yes, 9 => yes ) ) (well, ok, it will also have the submit button in it, but you know that already) so, we can walk this array like this: foreach ($_POST['completed'] as $ID => $value) { echo 'User Checked the box for ' . $ID . PHP_EOL; } We don't really care what $value is because checkboxes do NOT get posted unless they are checked. So the fact that it is there means it was checked. This will output: User Checked the box for 4 User Checked the box for 9 Now the easiest way to do the update you need would be something like this: $IDs = ''; if (isset($_POST['completed'])) { // Nothing to do if nothing is checked foreach ($_POST['completed'] as $ID => $Value) { $IDs .= (empty($IDs) ? '' : ',') . $ID; } // Now $IDs is a string like "4,9" $sql = 'UPDATE `online-form` SET completed = "yes" WHERE ID IN (' . $IDs . ')';
-
You realize you just took the lazy route again! Right? This too will come back and bite you in the back-end someday.
-
How to stop a user from hitting back button and it not reloading
DavidAM replied to SEVIZ's topic in PHP Coding Help
There is a referrer -- $_SERVER['HTTP_REFERER'] -- but you can NOT rely on it. The browser sends it, so it can be spoofed. What you need to do is make sure the page is not cached, see header(). I'm not real sure of the best way to do it. But, if the page has expired, then the browser has to request it from the server again. Since you extract the money from the database, the user should now get the NEW (updated) value of what is available to spend. If you are putting the amount they have available in the form (maybe as a hidden field) and using that to determine if they have enough to cover their request, DON'T!!!!! It is very easy to modify a form and send any value I want. When the form is submitted, you MUST check against the database again (or a session variable) NOT the form. Hopefully, someone will chime in here with the correct headers to force a page to not be cached. EDIT: Or you can search the forums for expire and cache; I'm sure there have been one or two topics on that. -
[*]Always put your code in [ code ] tags (without the spaces) so it is displayed properly; [*]Make sure you have error reporting turned on -- error_reporting(E_ALL); -- at the beginning of your scripts; [*]You did not state a problem. What is your code doing? Do you get any error messages? From a cursory glance, you are creating multiple checkbox fields with the same name ("completed"). You will not be able to tell which one is checked when the user submits the form. The hidden fields will not help since ALL of them will be posted regardless of whether the associated checkbox is checked or not. Actually, they are only associated in our minds, the browser makes no such association. To make this work, remove the hidden fields (you don't need them) and change the checkbox to: <input type="checkbox" name="completed[<?php echo $ID;?>]" value="yes" /> This will allow the user to check any number of checkboxes. And will produce an array, when submitted, of $_POST['completed'][##] = 'yes' where the key ("##") will be the ID of the line checked. You can walk through this array, and update multiple records with one submit. If you want to prevent the user from checking multiple boxes, you will have to use either Javascript (which will be a pain) or use an INPUT TYPE="radio" button. In this case, all of the radios have to have the same name (as they are now) but you can specify the value to be the ID. You still do not need the hidden fields.
-
Something along the lines of // Output TO SCREEN //imagejpeg($image_p, null, 100); // Output TO FILE if ($fileh = fopen('NewFilenameHere', 'w')) { if (! fwrite($fileh, $image_p)) { echo 'Failed to write to output file' . PHP_EOL; } fclose($fileh); } else { echo 'Failed to open output file' . PHP_EOL; }
-
The PHP manual for fgets() (http://us2.php.net/manual/en/function.fgets.php) says: If your input file has newlines, then they are in the $phpLine after you read the file, and are being written to the new file. You can get rid of them by using one of the trim() functions either on the read ... $phpLine = trim(fgets($file)); or on the write ... fwrite($wfile, trim($phpLine));
-
[SOLVED] Quering Multiple Databases for a single field.
DavidAM replied to Errant_Shadow's topic in MySQL Help
The "!==" can be thought of as NOT EXACTLY THE SAME AS. It checks the datatypes as well as the value. For instance; the number zero (0) evaluates to false in a test. So if you set "$var = 0", then test it "if ($var == 0)" the IF is true. If you set "$var = false", and test "if ($var == 0)" the IF is also true. However, "if ($var === 0)" [note three equal signs], then it is false, because the datatypes are different (integer vs. boolean). See the PHP manual on comparison operators (http://us3.php.net/manual/en/language.operators.comparison.php) This is especially handly with the strpos() function. This function will return false if the value is not found. If it finds the value, it returns the offset position. So, if the value is found at the first character, it returns 0 (zero). An equal test "if ($pos == 0)" or "if ($pos != 0)" will not provide the results you expect. For example: $Sent = 'Now is the time for all good men to come to the aid of their country.'; $Find = 'Now'; $Pos = strpos($Sent, $Find); // $Pos is now equal to zero if ($Pos) { // We found something - except since $Pos is zero, and zero evaluates to false, this does not execute echo 'Found it at: ' . $Pos . PHP_EOL; } if (! $Pos) { // We did not find it - except since $Pos is zero, and zero evaluates to false, this IS executed echo 'Did NOT find it.' . PHP_EOL; } if ($Pos !== false) { // We found something - this will work echo 'Really Found it at: ' . $Pos . PHP_EOL; } if ($Pos === false) { // We did not find it - this works too echo 'It is NOT there at all' . PHP_EOL; } Note: I wrote this code off-the-cuff, so it may have typos in it, but I think it shows the intent. Many functions may return zero or false with different meanings. Also note that zero in a string "$var = '0';" may also evaluate to the number zero or false, since PHP is not a strongly typed language. So, sometimes you want to make sure that a value is infact the string or numeric or boolean value you are testing it against. In those cases, you need to use the additional equal sign. EDIT: The !== operator was not strictly necessary in the code I posted last time. I used it because I was looking for a specific value I had assigned to the array. In that code, the variable we were testing is either an array or it is false, so there should not be any ambiguity. -
[SOLVED] Quering Multiple Databases for a single field.
DavidAM replied to Errant_Shadow's topic in MySQL Help
while ($row = mysql_fetch_assoc($result)) { $_SCRIPS[$myDatabases[$i]][] = $row; // would this need that extra [] to work right? } Yes, you need that extra [] at the end to add the $row array to the [db] array. As a result, the output will have one additional level from your sample: $_SCRIPS = array ( ['red'] => array ( [0] => array ( 'ID' => 4, 'Name' => John Doe, 'Title' => PHP Weekly, 'StartDate' => 2009-10-01, 'ExpireDate' => 2010-09-30 ) ), ['blue'] => array ( [0] => array ( 'ID' => 4, 'Title' => PHP Weekly, 'PaidDate' => 2008-09-12, 'IssueCount' => 12 ) ) ) If more than one row is returned from the query then [ 0 ] will be [ 1 ] for the second record and so on. If you KNOW for a fact, that there will be only 1 record from each query, you can leave off the extra [] and get the output as you suggested in your post (without the extra level). Just be aware that $_SCRIPS['blue'] will not exist if no rows were returned for that query. If there is to be only one record (because ID is the primary key and there can not be more than one) or if you only want the first record, you could eliminate the loop and do something along these lines: if ($result) { $_SCRIPS[$myDatabases[$i]] = mysql_fetch_assoc($result); } mysql_fetch_assoc will return false if there is no row. So then you can check it like this: if ($_SCRIPS['blue'] !== false) { // Process data from BLUE } Also note, the $myDatabases array values are in lower-case so your resulting array keys will be lower-case. -
There's an extra comma just before the WHERE clause. So the query is probably failing. You should check each query when you execute it: if (! mysql_query("UPDATE shop SET item='$item', size='$size', shape='$shape', colour='$colour', quantity='$quantity', image='$image', price='$price', description='$description' WHERE id='$id'")) { echo 'Database update failed: ' . mysql_error(); } else { echo "<BR>The item '$item' has been updated successfully!<BR><BR>"; }
-
I'm not sure what the problem is now. Did the error message go away? Is the form being built? Does it have the values selected from the database in it? The only thing I see is that you are using short tags "<?" in the form where you echo the data. You should always use full tags "<?php". If your server is not configured to allow short tags, then the variables are not getting echo'd.