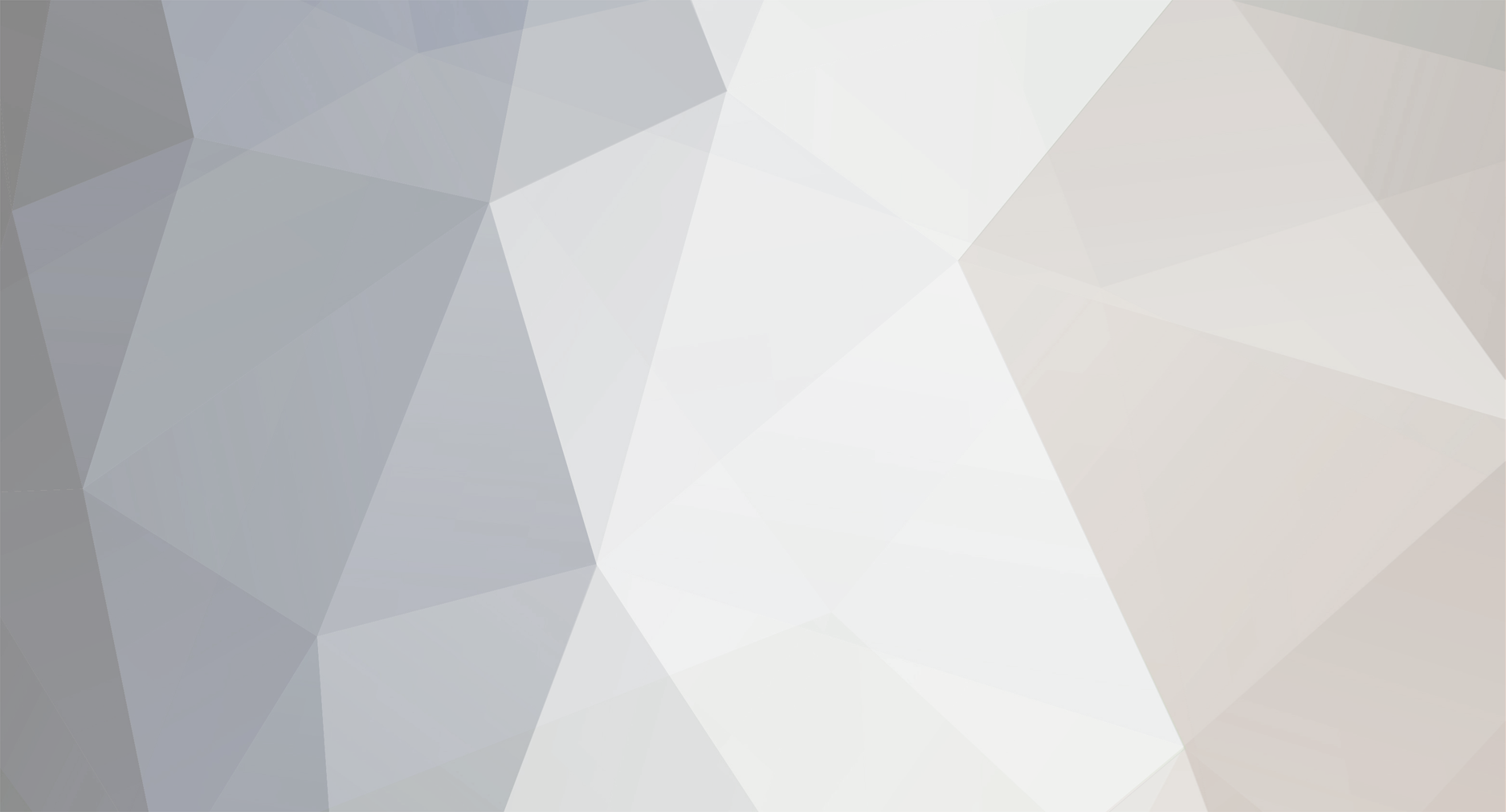
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
1) When you first load the page $_POST['submit'] is not defined, so change that IF to if (isset($_POST['submit'])) { 2) $SCRIPT_NAME is undefined. You are not assigning it anywhere in the code you posted.
-
Is it possible that one of the lines is more than 4096 characters long and so you only get part of it, then, for a while, you are getting data from two partial rows? Is it possible that one of the lines does not have matching opening and closing quotes in one or more fields? Try adding this just before the array_combine and see what you get: if (count($data) != count($fields)) { echo 'Incorrect column count in record: '; print_r($data); exit; } what output do you get?
-
[SOLVED] Largeblob image from mysql to website - corrupted?
DavidAM replied to KBBach's topic in MySQL Help
A couple of minor things: You are missing the '.' in the query to combine the two strings @mysql_query("select PIC from products where ID=" . $id); After mysql_query, you still have to fetch the row: $res = @mysql_query("select PIC from products where ID="$id); $img = mysql_fetch_array($res); echo $img[0]; And a couple of side notes: Since the output from this script is going to an image tag, it can be difficult to see any error messages or problems. If you just get a broken image placeholder on your page, you can request this script by typing it in the browser's address bar ( getProdImg.php?id=2 ), and then you will either see the image if it works, or an error message or something. For debugging, you can echo stuff out - which will break the image tag, but let you see what's happening. Just take out all the debug echos in the final script. There is nothing magical about this script. It can be requested from the browser (as I just suggested). So you need to sanitize the input to prevent SQL injection attacks, etc. -
[SOLVED] fopen(...txt,'"a+') not creating file and writing to it
DavidAM replied to phpnewbieca's topic in PHP Coding Help
Is error reporting on? Are you getting any error messages? Put error_reporting(E_ALL); ini_set("display_errors", 1); at the beginning of your scripts. Then post any errors you get so we can help. Also, do you realize that rewind simply moves the pointer to the beginning of the file? The fwrite will OVERWRITE existing content in the file, it will NOT INSERT the new data into the beginning of the file. -
[SOLVED] Largeblob image from mysql to website - corrupted?
DavidAM replied to KBBach's topic in MySQL Help
Yes, you need to write a new script that sends an image to the browser. Usually, when you want an image, you use <IMG src="ImageFile.jpg"> The browser then sends a request to the server for it to send "ImageFile.jpg" and the browser puts the image data where the IMG tag is. Since the request for the image file is a standard GET request, we can use a script to send an image <IMG src="getImage.php?file=ImageFile.jpg"> then write the script to display the image header('Content-Type: image/jpeg'); $data = file_get_contents($_GET['file']); echo $data; [ Of course there's no error checking or security checking there, this is just an example ] This script can do database access; or anything else you want. However, the output can ONLY be image data. That's why my previous post suggested echo '<IMG src="getProdImg.php?id=' . $row['ID'] . '">'; // id=# or whatever your unique identifier is I don't know the layout of your table, or what your primary key is called; so I suggested 'ID' which is fairly common. You need to write getProdImg.php to retrieve the image data from the database using the ID (or whatever you pass in as the identifier) and output the header and the data. -
[SOLVED] Largeblob image from mysql to website - corrupted?
DavidAM replied to KBBach's topic in MySQL Help
Wise words # 1 - Turn on error reporting (error_reporting(E_ALL)) at the beginning of ALL scripts. You cannot send a header in the middle of a page, and you can't dump image data to the browser without the header. (Well, you can, but it looks like crap). I don't know any way to do this in one pass. If anyone does, I'd be interested to see it. To accomplish this, make that an IMG tag that will run a script to dump the image data: echo '<td width=200>'; #echo 'billede her'; //** REMOVE THESE TWO LINES AND ADD THE ONE BELOW // header('Content-Type: image/jpeg'); // echo $row['PIC']; echo '<IMG src=getProdImg.php?id=' . $row['ID'] . '>'; // id=# or whatever your unique identifier is echo '</td>'; Then you write getProdImg.php to send the header(), retrieve the image data from the database and send it to the browser. Do not send any HTML from this script, it is only sending an image. -
It looks like you have the logic correct to show the current image in the select list for($i=0; $i<$c; $i++) { $selected = (isset($image) && $image == $imgArray[$i]) ? "selected" : ""; echo "<option value='{$imgArray[$i]}' $selected>{$imgArray[$i]}</option>\n"; } (by the way, use code tags to make your code easier to read) To make this work, you need to assign the image name to the $image variable before the loop somewhere. I don't see you doing that in the portion of code you posted.
-
I have never tried this before, so I can't speak about whether it will work or not. But I did notice, that in the middle of your function you have the directory "./In/" hard-coded in the ftp_nlist function call. Shouldn't that be $dir?
-
Without seeing the code for the getMatches method, I have to guess that it is returning an array of matches. The parameter looks like an SQL WHERE clause. If the method does in fact return all of the matches that satisfy the WHERE clause, then you probably need to get the last entry in the array: $prev_matches = $leaguemanager->getMatches("( `home_team` = {$team->id} OR `away_team` = {$team->id} ) AND DATEDIFF(NOW(), `date`) > 0"); $prev_match = $prev_matches[count($prev_matches)-1]; // or maybe 'end' would be better $prev_match = end($prev_matches);
-
I think thorpe is correct. The original code looks OK except that the openning tag before the else is a short tag. Maybe the OP's server does not support short tags. Apparently, this site's '[ code ]' tags don't support short tags either. Good for you! It's a nasty habit. Hey, can we get the government to ban short tags in public places? They are hazardous to the internet's well-being.
-
AND's take precedence over OR's so you have to add the parenthesis (below). This query will return all rows where user = $user AND any of the six tests AND any of the four results. SELECT * FROM bb_off WHERE user='$user' AND (test1='test1' OR test2='test' OR test3='test3' OR test4='test4' OR test5='test5' OR test6='test6') AND (Result1='result1' OR Result2='result2' OR Result3='result3' OR Result4='result4') Without the parenthesis it acts like this: SELECT * FROM bb_off WHERE (user='$user' AND test1='test1') OR test2='test' OR test3='test3' OR test4='test4' OR test5='test5' OR (test6='test6' AND Result1='result1') OR Result2='result2' OR Result3='result3'OR Result4='result4' Which is clearly NOT what you want.
-
can you post your code as it is not so we can have a look?
-
If I was doing this, I would create a table with two columns: BadWord and FixWord. Make BadWord a unique index (or the primary key). Then load the words like this (this code assumes an existing connection to the database): $filter = array(); // empty filter array to start with $res = mysql_query('SELECT BadWord, FixWord FROM WordFilter'); while ($row = mysql_fetch_array($res)) { $filter[$row[0]] = $row[1]; } mysql_free_result($res); // Now you have a filter array with the key being the bad word // Get the user's input $inString = $_GET['txtInput']; // or whatever $words = explode(' ', $inString); $outString = ''; // walk the array of words looking for bad words foreach($words as $word) { if (array_key_exists($word, $filter)) { $outString .= $filter[$word] . ' '; else $outString .= $word . ' '; } // Now $outString contains the censored input // (with an extra space at the end) echo $outString . PHP_EOL; This code will do what you asked. With a couple of caveats. It is not case insensitive (i.e. Damn and damn are two different words) It will not catch words followed by punctuation (i.e. Damn, I left the bitch.) I leave those exercises to the reader. I'm just wondering if this is the best way to do it. If your badwords table gets big, you are loading a lot of data into an array that you will not use. However, I can't think of a way to accomplish this in SQL right now. I might consider testing something along these lines: SELECT BadWord, FixWord FROM WordFilter WHERE '$UserInput' LIKE '%' + BadWord + '%'; If I could get this syntax to work, the select would only return the words that need to be replaced. That would reduce the amount of data returned to PHP and reduce the overhead of the page. Of course, I don't have spaces around the BadWord, so 'class' might get censored (easily fixed); but the table can be setup to be insensitive to case.
-
//loop through to add options to select html for ($x=0; $x<count($emparray); $x++) { $tableselect .= "<option value='$emparray[0]'>$emparray[0]</option>"; } You have collected the table names in an array ($emparray). In the loop, you are always referring to the first element of the array ($emparray[0]), so you are just getting the first table. Change that to: //loop through to add options to select html for ($x=0; $x<count($emparray); $x++) { $tableselect .= "<option value='$emparray[$x]'>$emparray[$x]</option>"; }
-
[SOLVED] Like/Or/And statement that almost works - Need help
DavidAM replied to leafer's topic in Microsoft SQL - MSSQL
Precedence! (is that spelled right?) wrap all the OR's in parenthesis so if ANY one is true AND the league is true ... WHERE ( position LIKE '%$position1%' OR position LIKE '%$position2%' OR position LIKE '%$position3%' OR position LIKE '%$position4%' ) AND league = '$league' otherwise the AND is attached to the last position condition (#4) so it is acting like this: WHERE position LIKE '%$position1%' OR position LIKE '%$position2%' OR position LIKE '%$position3%' OR ( position LIKE '%$position4%' AND league = '$league' ) -
AND (DATE_FORMAT(FROM_UNIXTIME(`cbdate`), '%Y/%m') = '2009/10') AND (DATE_FORMAT(FROM_UNIXTIME(`cbdate`), '%Y/%m') = '2009/11') AND The column cannot be BOTH October AND November. I think you meant to use OR AND ( (DATE_FORMAT(FROM_UNIXTIME(`cbdate`), '%Y/%m') = '2009/10') OR (DATE_FORMAT(FROM_UNIXTIME(`cbdate`), '%Y/%m') = '2009/11') ) AND Also added a set of parenthesis around the two DATE_FORMAT tests since the OR would otherwise breakup the condition. By the way, is cbdate actually stored as a unix timestamp? If you used a mySql DateTime column, this is not the correct way to query it.
-
If the first and last name is all that you are interested in, the query would be: SELECT DISTINCT fname, lname FROM titles WHERE ... that will give you only rows where the combination is unique. If you want to summarize some of the other data, you could do something like: SELECT fname, lname, COUNT(title) as Articles FROM titles WHERE ... GROUP BY fname, lname which will return one row for each unique combination of first and last name along with a count of the number of titles associated with the combination.
-
It is true that the From header of an email message needs to have a valid email address. Your system's mail handler sees the name you put there (for instance, "John") and is trying to turn it into a valid address. So it is adding the domain your server is configured to send mail from, so you end up with John@yahoo.com (or whatever). You need to specify an email address associated with your server. However, you can add a display name to that. Some (most?) email clients will display this part rather than the actual email address if it is specified correctly. You can try it like this: $siteEmail = 'mySite@yahoo.com'; // or whatever your website's designated email address is mail( "tech_for_all@yahoo.com", "$subject", $message, "From: \"$name\" <$siteEMail>\r\nReply-to: $email" ); Do not put the user's email address in the From:, since, as thorpe said, most mail servers will treat it as spam, and some will just refuse to send it. But you can cause the client to display just about anything in the from column.
-
I think you need to specify the From: header as a valid formatted email address (with optional display name). So it should really look like this: "John Doe" <JohnDoe@Mymail.net>. Otherwise the mail servers processing it are going to take liberties with an invalid email address. Be aware, however, that you might have problems trying to send an email using a "from" address that is not your domain. Some will consider it a spam, some servers will not send it. I think it best to use your website's email address in the from (although you can still use the User's display name) "John Doe" <Mywebsite@domain.com> and then put the user's email in the ReplyTo header (as you are doing now).
-
Your list_pages function returns the text to be output. You need to echo it to the page: <?php echo list_pages();?>
-
Unable to retreve the values from Mysql Query
DavidAM replied to surajkandukuri's topic in PHP Coding Help
mysql_fetch_array returns an associative array or numeric array or both. However, your query does not return a column named 'temp' so your assignment: $value=$result_row2['temp']; is not assigning anything to $value. 1. Turn on error reporting at the beginning of your script error_reporting(E_ALL);. Then you will see the error message about referring to a non-existant key. 2. Either specifically assign the column a name or refer to the numeric index $sql_query= "Select Count(*) AS temp from (Select Cat_Num from cat_completiondate where `EmployeeNumber`=$employeenumber and `CategoryJobPosition` =$jobid) as temp"; $query_result2=mysql_query($sql_query); $result_row2=mysql_fetch_array($query_result2); $value=$result_row2['temp']; $value=$result_row2[0]; // Should return the same value echo($value) -
retrieve value of array based on textbox array
DavidAM replied to sherric's topic in PHP Coding Help
If I understand correctly, you are putting out a bunch of text boxes for the user to enter a quantity, and you want to associate the quantity entered by the user with the appropriate "item". The way you are going about it will probably work, but since different browsers seem to do whatever they want, I would not depend on it. Maybe somebody will write a browser that chooses not to send empty text boxes. What you have: while ( $row = mysql_fetch_array($result2) ) { $i++; print "<tr>\n"; echo "<td>{$row['name']}</td>\n"; print "<td><input name=txtQty[] type=text/ size=5></td>"; print "<input type=\"hidden\" name=\"Item[]\" value=$row[id]>\n"; print "<input type=\"hidden\" name=\"Name[]\" value=$row[name]>\n"; echo "</tr>\n"; }//end while when posted will give you three arrays in $_POST. They are "txtQty", "Item", and "Name". Choose one of the keys from any of the arrays, and use the same key to access the associated entries from the other arrays: txtQty[1] is the quantity for Item[1] with Name[1] However, just to be safe, when I do this, I force the unique ID from the database as the key to the index of data I want: while ( $row = mysql_fetch_array($result2) ) { $i++; print "<tr>\n"; echo "<td>{$row['name']}</td>\n"; print "<td><input name=txtQty[{$row['id']}] type=text/ size=5></td>"; print "<input type=\"hidden\" name=\"Item[{$row['id']}]\" value=$row[id]>\n"; print "<input type=\"hidden\" name=\"Name[{$row['id']}]\" value=$row[name]>\n"; echo "</tr>\n"; }//end while this will force the key to all three arrays within the $_POST array to be the ID from the database so I can then go straight to the database with something like this: foreach ($_POST['txtQty'] as $key => $value) { $sql = "UPDATE someTable SET qtyCOl = {$value} WHERE id = '$key'"; // then execute the sql statement } actually, if you use this method, the hidden fields are not necessary at all. -
If you are using the InnoDB Storage Engine, you should define the ID in the articles table as a Foreign Key to the Catagories table. Part of the FKey definition is how to handle DELETES or UPDATES of the key. ON UPDATE CASCADE should cause the ID to be updated in the "foreign" table (Articles) when you update the ID in the Categories table.