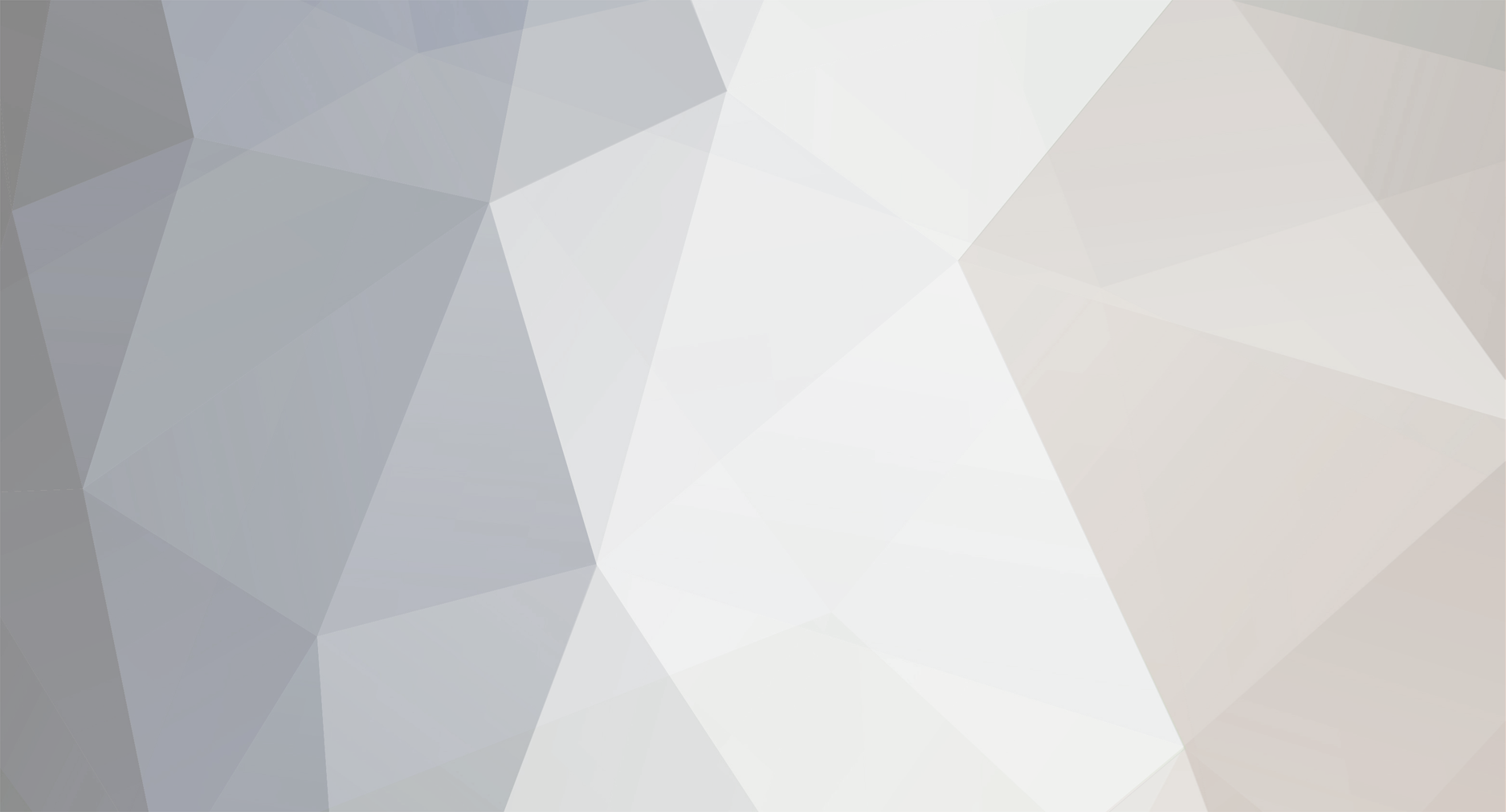
DavidAM
Staff Alumni-
Posts
1,984 -
Joined
-
Days Won
10
Everything posted by DavidAM
-
After reading that other topic (you know the one I'm talking about) I felt I needed to say something that has been on my mind for a while: THIS IS A GREAT SITE!! I want to especially thank the Administrators for managing this site and keeping it going. It is, by far, the best peer-help site I have ever had the privilege of visiting. I know you guys work hard to maintain the site; hardware, software, users, etc. And you deserve a big thanks. The moderators, I'm sure, also work hard keeping topics where they belong, and generally trying to bring order out of chaos. Thank you! To all the PFR's and Guru's, Thanks! I appreciate the fact that you freely share your knowledge. I have learned a lot from this site. Just reading through the topics, I pick up ideas, suggestions, and warnings that help make me a better programmer. I've tried to give back to the community, but I don't know how I can ever keep the books balanced. There is so much to learn here. I have not posted many questions -- in fact only one, and although no one had an answer for me, I posted the solution once I found it, to help anyone else who came across a similar problem -- but I read a lot of topics. I've learned a great deal about RegEx (which has always been my nemesis) from this site. I've picked up quite a few security pointers in areas that I never would have thought could be a problem. I've even picked up a couple of SQL pointers. To all you guys (and gals?) that help keep this place running smoothly, and all you members who ask questions that make people think -- KUDOS! -- Keep up the good work. And a Great Big THANKS to Eric 'phpfreak' Rosebrock for founding this site! If it wasn't for you, I'd probably be surfing through idiotic videos on Utube. And before anyone comments, I intentionally left out the first "f", not the second one.
-
Man you really had me going! Sometimes I hate PHP and equality tests. You are missing two characters //This code actually CHANGES these two variables to empty strings if ($user="" or $pass=""){ // it should be this (two equal-signs to test for equality) if ($user=="" or $pass==""){
-
First, Indent your code, it makes it much easier to see what is happening <?php include('config.php'); if (isset($_POST['set'])){ $user=mysql_real_escape_string($_POST['user']); $pass=mysql_real_escape_string(md5($_POST['pass'])); if ($user="" or $pass=""){ echo 'Invalid Username/Password'; } else{ mysql_select_db("db_mycountdown",$db_connect); $sql=mysql_query("SELECT * FROM users WHERE username='$user'",$db_connect); $fetch = mysql_num_rows($sql); if ($fetch>0){ unset($user); echo 'Error: This user already exists!<br>'; } else{ mysql_select_db("db_mycountdown",$db_connect); $sql = mysql_query("INSERT INTO users (username, password) VALUES ('$user','$pass')",$db_connect); echo 'Account Successfully Created!'; } } } echo '<a href="Index.php">Already have an account? Log in!</a> <form action="" method="post"> Username:<br/> <input type="text" name="user"/><br /> Password:<br/> <input type="password" name="pass"/><br /> Confirm Password:<br/> <input type="password" name="passconfirm"/><br /><br /> <input type="submit" name="set" value="Register" /> <br /> </form>'; ?> 2) $pass is never going to be empty. You ran MD5 on a possibly empty string, and it will produce results (maybe). I would test for empty($_POST['user']) or empty($_POST['pass']) before processing them. 3) there is no database connection, unless you do that in config.php 4) you do not need to select the database before every query (I do it immediately after connecting and it stays that way unless I select a different one). 5) you should check that pass and passconfirm have the same value before you insert into the database. That's the whole reason for having two password fields. 6) (Actually, this should always be first!) Turn on error reporting to see if any errors are being thrown and ignored: Add error_reporting(E_ALL); at the beginning of your scripts. Also, add ini_set('display_errors', 1); if it is not set in the ini file. 7) After $sql = mysql_query(...), try adding this line: $sql = mysql_query(...); if ($sql === false) { echo mysql_error(); } to see if the query is failing. Let us know how things go and post any error messages you get so we can help further.
-
WHERE comes before GROUP BY. The WHERE tells what you want to get, and the GROUP BY tells how you want to see it.
-
You could use the suggestion taquitosensei gave you. ($minutes % 60) will return the number of minutes (between 0 and 59) with the hours, days, etc. stripped off. But it you want all of the components (which you don't seem to be using) you have to subtract out each level as you go through: function getTIME($time) { ## example start/end dates $startdate = $time; $enddate = time(); ## difference between the two in seconds $time_period = ( $enddate - $startdate ); $day = '86400'; $hour = '3600'; $minute = '60'; $second = '1'; $days = intval($time_period / $day); $time_period -= ($days * $day); $hours = ($time_period / $hour); $time_period -= ($hours * $hour); $minutes = ($time_period / $minute); $time_period -= ($minutes * $minute); $seconds = ($time_period / $second); echo number_format($hours).":".number_format($minutes); } and I wouldn't use number_format, instead use: printf('%2d:%02d, $hours, $minutes) or, the quickest way, might be echo date($time_period, 'H:i'); I think that would work, haven't tried it.
-
My first reaction was to use an IF statement as in : UPDATE table SET Service='$Service',Provider='$provider',Date=IF('$date' = '', NULL, '$date') WHERE id = '$id'; Which seems to work. But only if $date returns an empty string. I decided to try something a little more robust to see if we could set NULL if the date was invalid. So I tried $date + INTERVAL 0 DAY, and strangely enought that returns NULL if $date is empty. I had expected it to return the zero date. So, in MySql 4.1 (yes I know, I'm WAY behind the times here), the following should always set Date to NULL if $date does not contain a valid MySql date. UPDATE table SET Service='$Service',Provider='$provider',Date='$date' + INTERVAL 0 DAY WHERE id = '$id';
-
Acutally, unless they have changed something, you can set a DateTime field to NULL. But, take a look at the example code: UPDATE table SET Service='$Service',Provider='$provider',Date='$date' WHERE id = '$id'; This is explicitly assigning a value to the Date column. If $date is an empty string or 0 (zero) or NULL, you are still assigning a value to the column. What would this look like if we assigned some values to those variables? UPDATE table SET Service='HardWork',Provider='LazyBoy',Date='' WHERE id = '$id'; Look at that, we are assigning an empty string to Date. That is not a valid DateTime value. And, according to the documentation, the server will set the column to that special "zero date" (0000-00-00 00:00:00). If you want to set the column to NULL, you have to do so just like setting any other column to NULL: UPDATE table SET Service='HardWork',Provider='LazyBoy',Date=NULL WHERE id = '$id'; NO QUOTES around that.
-
Something like this: SELECT users.dispname, users.country, scores.email, scores.month, SUM(scores.correct) AS TotalCorrect, SUM(scores.time) AS TotalTime FROM scores JOIN users ON scores.email = users.email GROUP BY users.dispname, users.country, scores.email, scores.month ORDER BY TotalCorrect DESC, TotalTime ASC LIMIT 10
-
Man this new layout is hard to read! OK. Your problem is that you are loading the $prices array inside the foreach loops (stores -- products). After printing the $prices array, you do not clear it, so the values are still in it. Then you add more elements to the array in the next run through the products/stores loop and you print the ENTIRE array. Try clearing the $prices array ( $prices = array() ) just before the while($price = mysql_fetch_object($resultt)) loop.
-
It works when run manually from the command line ... it does not work when run from cron. This would indicate a difference in the shell environment when cron is running it vs. when logged in and running it. $pdf-Output() is not specifying an absolute pathname for the file to be created. Unless that method is prepending a path name, it is trying to create the file in the "current working directory". What is your current working directory when running a cron script? Typically, cron will mail any output (including error messages) from a cron job to the user. I don't know how this worksout on a hosted site. Are you getting any strange emails that might be indicating an error in the job? Try adding to the end of your cron command >>/var/www/reports/errors.txt 2>&1 (use a path that you have write access to) then after the cron job runs, take a look at errors.txt and see if you have any error messages there.
-
4) you never called session_start() I don't know how I missed that ... not my fault though ... I don't make mistakes ... I thought I did once, but I was wrong ...
-
It looks like some of those echos are for debugging, but I see a couple of other problems with the code: 1) Make sure the openning tag (<?php) starts at the very first character of the very first line. You can NOT have any whitespace before it because that whitespace will be sent to the browser. 2) Set your session variables before calling the header() function. Code after the header() function (with a location) is probably not going to execute. That being said, always put an exit() after the header() call (with a location) so that the code after it will definitely NOT execute. 3) session_register() is depricated. You should use $_SESSION['variableName'] instead.
-
from the manual http://us.php.net/manual/en/function.array-unique.php array_unique() (emphasis added) So the keys are not going to be consecutive. Which means you can't walk the resulting array with an incrementing index. What are you expecting from countwords()? The way it is written you should get a numerically indexed array with a count assigned to each value, but you have no way of knowing which word that count applies to. I think $tempcount has what you really want; an array indexed by words with a count of the number of times that word is found in the original array. You can print it using foreach: foreach ($tempcount as $word => $count) { print $word . ' occurs ' . $count . ' time(s)<BR>' .PHP_EOL; }
-
Just a couple of things I've noticed that may have some bearing on this: [*]I have trouble with mysqladmin assigning a DEFAULT clause when creating a table with NOT NULL columns. Or removing the default from a column so that there is NO default (seems mysql wants a default if the column is not null, but it should NOT) [*]I have trouble changing a value in the mysqlbrowser to NULL. [*]When you are adding $dob to the query string, how are you doing it? I'm at work and don't have access to an environment to check things on, so this is all from memory. // Example 1 $dob = (empty($_POST['dob']) ? 'NULL' : $_POST['dob']); $sql = "UPDATE myTable SET myDOB = '" . $dob . "' WHERE myID = " . $id . "'"; // Example 2 $dob = (empty($_POST['dob']) ? NULL : date($_POST['dob'])); $sql = "UPDATE myTable SET myDOB = '" . $dob . "' WHERE myID = " . $id . "'"; // Example 3 $sql = "UPDATE myTable SET myDOB = " . (empty($_POST['dob']) ? 'NULL' : "'" . date($_POST['dob']) . "'") . . " WHERE myID = " . $id . "'"; Ex 1-- is going to put the string 'NULL' into a date field, which is illegal and results in a "zero date". Ex 2-- is going to put an empty string in there, also illegal Ex 3--should put a NULL in the field since the resulting query will not have quotes around the word NULL.
-
If id is a unique column in bidding_details, then we can update using that column's value: // Get the top 50 valid bids sorted from highest to lowest $query="SELECT id, reg_id, bid_price FROM `bidding_details` where `bid_id`='$bid_id' and `sortbid` = '1' order by bid_price DESC limit 50"; $result=mysql_query($query) or die('Failed selecting applications: ' . mysql_error()); // Walk the result set to check for users with more than 3 in a row $lastU = ''; // The last user we found in the result $inRow = 0; // How many this user has in a row $reject = array(); // ID's of entries to be rejected while($row = mysql_fetch_array($result)) { if ($lastU == $row['reg_id']) { // If same user as last row increment count $inRow++; if ($inRow >= 3) { // If the user has more than 3 in a row, we reject the ID $reject[] = $row['id']; } } else { // Not same user, so change the last user we saw $lastU = $row['reg_id']; $inRow = 1; } } // If any entries were put into the reject array, update them all at once. if (count($reject) > 0) { $sqlU = "UPDATE bidding_details SET sortbid = '0', rank = '0' WHERE id IN (" . implode(',', $reject) . ")"; mysql_query($sqlU); } This collects the unique id's of the third (or fourth or fifth, etc) in a row and then updates them at the end of the loop.
-
Depends on what kind of programmer you are. E_NOTICE are errors that the parser can recover from after making certain assumptions. If you write: $B = 100; $A = $b + 1; you will get an E_NOTICE that $b does not exist. PHP will treat it as 0 (zero) and continue. So, in this case, $A will be set to 1 and the code continues. However, if you meant to write: $B = 100; $A = $B + 1; (note the second $b is now capitalized). $A should be 101. But with E_NOTICE off, if you typed my first example, you don't know that there was a problem and your script is running with an incorrect value. You should always declare all variables before using them. And you should always have E_ALL (which includes E_NOTICE) turned on. If you have it on and are getting notices, you should fix the code NOT change the level of reported errors. The following code will work and produce what is expected: for ($i = 0; $i < 10; $i++) { $out .= $i . ', '; } print $out; // prints: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, but it will also produce an E_NOTICE the first time through the loop ($out does not exist, but PHP treats it as an empty string and runs on. However, if later on, you modify the code and add the following somewhere ABOVE that: $out = implode(':', range(A-E)); print $out; // prints: A:B:C:D:E then $out is defined going into the for loop and at the end of the for loop it will contain: A:B:C:D:E0, 1, 2, 3, 4, 5, 6, 7, 8, 9, which is probably NOT what you wanted. The correct fix to the for loop is not to turn off E_NOTICE but to define the variable: $out = ''; for ($i = 0; $i < 10; $i++) { $out .= $i . ', '; } print $out; // prints: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, and now the code will work correctly even after you come back later and re-use the $out variable elsewhere.
-
But, in the select you are ordering ASC so you are NOT getting the top 50 bids. You are getting the bottom 50 bids that are still open. When you the update them, you still have bids in the top 50 that need to be considered. Even if you do the first select DESC, when you mark some bids as not valid, that will allow other bids to float up into the now top 50 that may need to be considered. If bid_details had a unique column (such as bid_detail_id integer auto_increment) the whole process would be simple. Is that a possibility? Without that, you probably need to mark everything that is not in the top 50 as not valid, but that would leave you with less than 50 valid bids after marking duplicates as not valid.
-
any way to ignore a value from $result = select * from 'tableName'; ?
DavidAM replied to schalfin's topic in MySQL Help
True. In this particular case. But why not get in the habit of writing effecient code so when a case comes up that requires 50 records to be ignored, you won't even consider using the front-end to skip over them? I've been in the programming and database world for more years than I care to mention. And one of my pet peeves, is lazy programmers. In fact this whole world of interpreted languages that allow everybody and their brother to write code drives me nuts. I blame Bill Gates! Macros were fine until he got a hold of them, and turned it into a language and made everyone think they could write code. People who have no idea what logic is, are writing sloppy code and thinking they are "programmers". One of my philosophies of life is: Of course, if you own M$ or Intel, you love bloated code, because the users will have to buy more computing power to run it, which you are glad to sell, with a bloated O/S that requires more computing power ... ... climbs down off soapbox :-\ -
I apologize if you were offended by my questions. I did not intend to imply you were doing something stupid. I was offering help (which is what we do here) in the form of an alternative solution. If our alternative solution was acceptable to you, then your problem would be solved. But, we don't know the logic of your application, so we did not know the alternative was not acceptable. Sometimes, people do not ask the right question; sometimes you have to "think outside of the box"; sometimes a new perspective on a problem produces an elegant solution. Without understanding the problem, we are offering band-aids for bullet-holes at best. When the issues are not understood, it is possible to provide a "solution" that creates more problems later or just does not fit with the "logic" of the application. So we ask questions. But hey, if you don't want a "real" solution, then I'll stick to your last question (which I did not fully understand, but I am not allowed to ask questions ...): The query is ordering by bid_price asc so it did NOT produce those results. Actually, the code had no output statements so it did not produce those results AT ALL. If you only want the highest 50 bids, you need to sort DESCENDING not ASCENDING. LIMIT 1 does not start at row 100 and should not start at row 50; so I don't know what you are saying here.
-
OK. This may be a little more difficult than I expected. I have a couple more questions. You want to record the names ... - where and in what format? Are you looking for a list (i.e. Tom, Dick, Harry)? Or do you have a summary sheet that lists the players and you're looking to make a mark or count in the player's row for "quick games" (I'm not familiar with darts terminology, what do you call these games?)? Are you looking for a count of "quick legs" in a game or just any game where any leg is "quick" the basic formua (per leg) would be =if(and(T8<17,isnumber(U8)),"QuickWin","-") But I'm a little confused by the forumla in your previous post as compared to your spreadsheet: =IF(COUNTIF(G8:U8,">99")=0,"-",COUNTIF(G8:U8,">99")) G8-R8 look like scores. But T8 is the number of darts used so I don't see why it is in the forumla (although, I guess they will not be using 99 darts so it doesn't matter). And U8 is the won or lost column (according to your last post) so why is it in that forumla?
-
First, I agree with Zanus on this one. If the rule says you can't have more than 3 bids in a row, then you check when they enter the bid, and you reject it if it violates the rule. (Select the highest three bids in the table, if they all have the same user as the one placing the bid, they are trying to break the rule and you reject the bid). I'm really confused by the logic here. Why would user1 enter a higher bid when he has the highest bid? He's just raising the price for himself. Is this some kind of multi-winner auction? The top 5 bids ALL win, but a specific user can only have 3 winning bids? If that is the case, I can understand the problem. However, if there is only 1 winning bid (as your last post seems to state) then why would a user out-bid himself. If I put in the highest bid, at say $12 why would I then bid $13? I just increased the cost to myself since I already have the highest bid. And why do you care how many bids I put in a row if the only bid that matters is the absolute highest bid?
-
any way to ignore a value from $result = select * from 'tableName'; ?
DavidAM replied to schalfin's topic in MySQL Help
Why waste resources (database and php) retrieving and processing data that is going to be ignored? It is much more efficient not to send the data "across the wire" in the first place. Yes, the code is perfectly capable of skipping a row; and for a one-time query, it probably makes no difference. But in a page, that could be requested by any number of users all over the world concurrently, we should write the most efficient code possible. Database engines are designed to store and retrieve data, they do it very efficiently. Use the correct tool for the job. -
@zanus: The SELECT COUNT(*) solution would be better if he was looking for people with 3 entries; but the post indicated he was looking for 3 consecutive entries, and I couldn't see a way to do that in a query. @canabatz: By the way, I did not think about this before, but that query may not return what you expect. I have not really read through the complete specs for mySql, but without an ORDER BY clause, the order of the rows may be random. What I'm saying is that you may get 3 consecutive entries with the query, but the rows may not actually be consecutive in the database. I'm still not sure what you are trying to accomplish, but if you have a create_date column or an auto_increment column, you should probably be ordering the results by that column to make sure you get the same sequence as when the rows went into the database.
-
You will have to determine is unique about the row. Then you can do an update with a WHERE clause specifying the uniqueness of the row. I don't know what your table looks like, so i can't tell you how to do that. What is the table structure? and what are you trying to update?
-
Your code is not collecting the users in an array, so I'm not sure what you mean by But this should show which users have 3 entries in a row: $query="SELECT * FROM `my_table` where id='1233' LIMIT 10"; $result=mysql_query($query) or die('Failed selecting applications: ' . mysql_error()); $lastU = ''; $inRow = 0; while($row = mysql_fetch_array($result)) { if ($lastU == $row['first_name']) { $inRow++; } else { $lastU = $row['first_name']; } if ($inRow == 3) { echo $lastU . ' 3 in a row'; } }