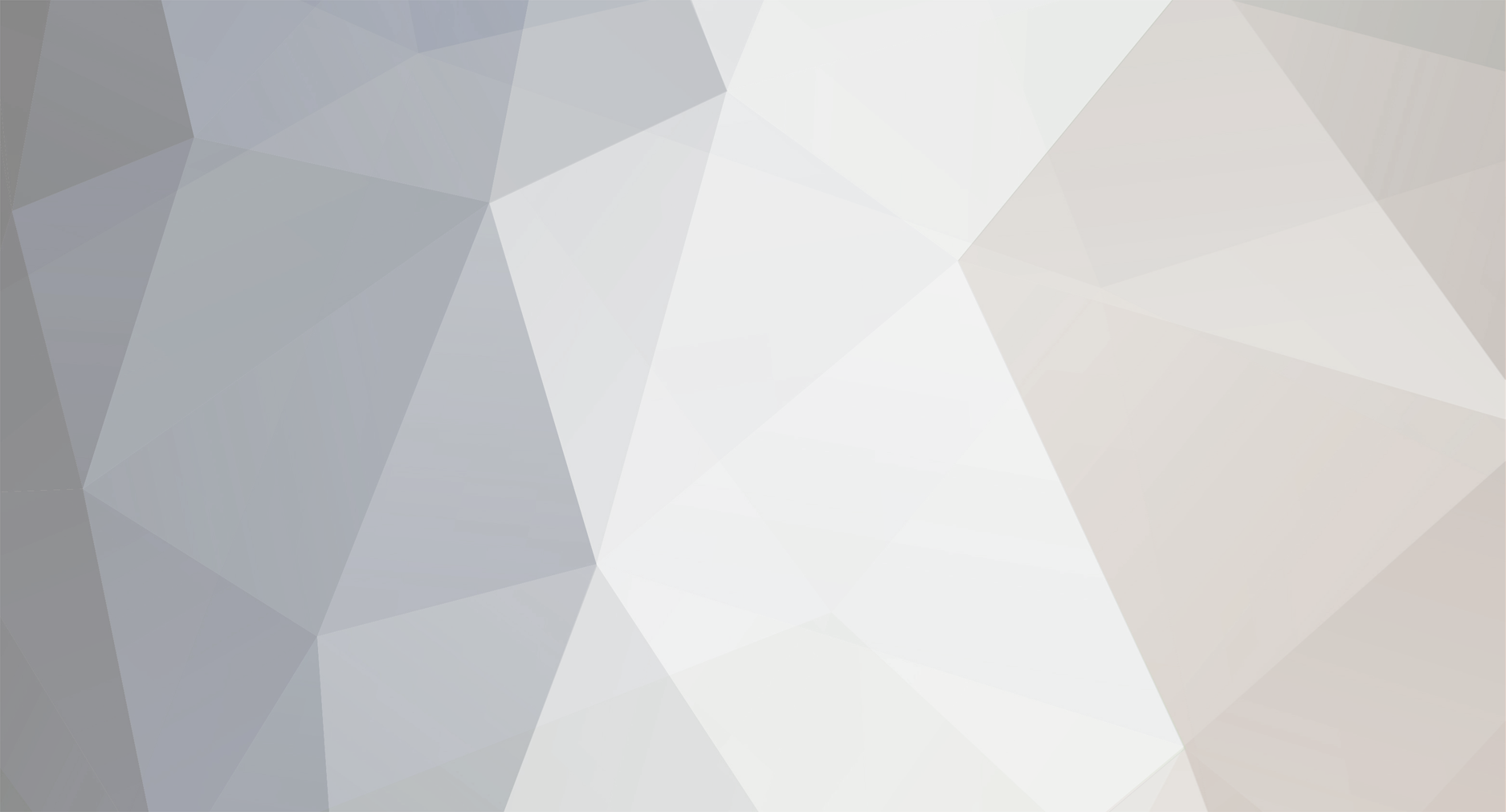
AngelicS
Members-
Posts
30 -
Joined
-
Last visited
Never
Everything posted by AngelicS
-
To only show a certain persons data, you'll need to add "WHERE - W3Schools" in your mysql query. Then just do a $user_data=mysql_fetch_array($mysql_query); Then if you want to echo the users name, you can do it like this: echo $user_data['name']; The "name" is a name column that you get from your database.
-
Try to use this code in the include: <?php class lastRSS { // ------------------------------------------------------------------- // Public properties // ------------------------------------------------------------------- var $default_cp = 'UTF-8'; var $CDATA = 'nochange'; var $cp = ''; var $items_limit = 0; var $stripHTML = false; var $date_format = ''; // ------------------------------------------------------------------- // Private variables // ------------------------------------------------------------------- var $channeltags = array ('title', 'link', 'description', 'language', 'copyright', 'managingEditor', 'webMaster', 'lastBuildDate', 'rating', 'docs'); var $itemtags = array('title', 'link', 'description', 'author', 'category', 'comments', 'enclosure', 'guid', 'pubDate', 'source', 'thumbnail', 'Listings'); var $imagetags = array('title', 'url', 'link', 'width', 'height'); var $textinputtags = array('title', 'description', 'name', 'link'); // ------------------------------------------------------------------- // Parse RSS file and returns associative array. // ------------------------------------------------------------------- function Get ($rss_url) { // If CACHE ENABLED if ($this->cache_dir != '') { $cache_file = $this->cache_dir . '/rsscache_' . md5($rss_url); $timedif = @(time() - filemtime($cache_file)); if ($timedif < $this->cache_time) { // cached file is fresh enough, return cached array $result = unserialize(join('', file($cache_file))); // set 'cached' to 1 only if cached file is correct if ($result) $result['cached'] = 1; } else { // cached file is too old, create new $result = $this->Parse($rss_url); $serialized = serialize($result); if ($f = @fopen($cache_file, 'w')) { fwrite ($f, $serialized, strlen($serialized)); fclose($f); } if ($result) $result['cached'] = 0; } } // If CACHE DISABLED >> load and parse the file directly else { $result = $this->Parse($rss_url); if ($result) $result['cached'] = 0; } // return result return $result; } // ------------------------------------------------------------------- // Modification of preg_match(); return trimed field with index 1 // from 'classic' preg_match() array output // ------------------------------------------------------------------- function my_preg_match ($pattern, $subject) { // start regullar expression preg_match($pattern, $subject, $out); // if there is some result... process it and return it if(isset($out[1])) { // Process CDATA (if present) if ($this->CDATA == 'content') { // Get CDATA content (without CDATA tag) $out[1] = strtr($out[1], array('<![CDATA['=>'', ']]>'=>'')); } elseif ($this->CDATA == 'strip') { // Strip CDATA $out[1] = strtr($out[1], array('<![CDATA['=>'', ']]>'=>'')); } // If code page is set convert character encoding to required if ($this->cp != '') //$out[1] = $this->MyConvertEncoding($this->rsscp, $this->cp, $out[1]); $out[1] = iconv($this->rsscp, $this->cp.'//TRANSLIT', $out[1]); // Return result return trim($out[1]); } else { // if there is NO result, return empty string return ''; } } // ------------------------------------------------------------------- // Replace HTML entities &something; by real characters // ------------------------------------------------------------------- function unhtmlentities ($string) { // Get HTML entities table $trans_tbl = get_html_translation_table (HTML_ENTITIES, ENT_QUOTES); // Flip keys<==>values $trans_tbl = array_flip ($trans_tbl); // Add support for ' entity (missing in HTML_ENTITIES) $trans_tbl += array(''' => "'"); $trans_tbl += array(''' => ","); // Replace entities by values return strtr ($string, $trans_tbl); } // ------------------------------------------------------------------- // Parse() is private method used by Get() to load and parse RSS file. // Don't use Parse() in your scripts - use Get($rss_file) instead. // ------------------------------------------------------------------- function Parse ($rss_url) { // Open and load RSS file if ($f = @fopen($rss_url, 'r')) { $rss_content = ''; while (!feof($f)) { $rss_content .= fgets($f, 4096); } fclose($f); // Parse document encoding $result['encoding'] = $this->my_preg_match("'encoding=[\'\"](.*?)[\'\"]'si", $rss_content); // if document codepage is specified, use it if ($result['encoding'] != '') { $this->rsscp = $result['encoding']; } // This is used in my_preg_match() // otherwise use the default codepage else { $this->rsscp = $this->default_cp; } // This is used in my_preg_match() // Parse CHANNEL info preg_match("'<channel.*?>(.*?)</channel>'si", $rss_content, $out_channel); foreach($this->channeltags as $channeltag) { $temp = $this->my_preg_match("'<$channeltag.*?>(.*?)</$channeltag>'si", $out_channel[1]); if ($temp != '') $result[$channeltag] = $temp; // Set only if not empty } // If date_format is specified and lastBuildDate is valid if ($this->date_format != '' && ($timestamp = strtotime($result['lastBuildDate'])) !==-1) { // convert lastBuildDate to specified date format $result['lastBuildDate'] = date($this->date_format, $timestamp); } // Parse TEXTINPUT info preg_match("'<textinput(|[^>]*[^/])>(.*?)</textinput>'si", $rss_content, $out_textinfo); // This a little strange regexp means: // Look for tag <textinput> with or without any attributes, but skip truncated version <textinput /> (it's not beggining tag) if (isset($out_textinfo[2])) { foreach($this->textinputtags as $textinputtag) { $temp = $this->my_preg_match("'<$textinputtag.*?>(.*?)</$textinputtag>'si", $out_textinfo[2]); if ($temp != '') $result['textinput_'.$textinputtag] = $temp; // Set only if not empty } } // Parse IMAGE info preg_match("'<image.*?>(.*?)</image>'si", $rss_content, $out_imageinfo); if (isset($out_imageinfo[1])) { foreach($this->imagetags as $imagetag) { $temp = $this->my_preg_match("'<$imagetag.*?>(.*?)</$imagetag>'si", $out_imageinfo[1]); if ($temp != '') $result['image_'.$imagetag] = $temp; // Set only if not empty } } // Parse ITEMS preg_match_all("'<item(| .*?)>(.*?)</item>'si", $rss_content, $items); $rss_items = $items[2]; $i = 0; $result['items'] = array(); // create array even if there are no items foreach($rss_items as $rss_item) { // If number of items is lower then limit: Parse one item if ($i < $this->items_limit || $this->items_limit == 0) { foreach($this->itemtags as $itemtag) { $temp = $this->my_preg_match("'<$itemtag.*?>(.*?)</$itemtag>'si", $rss_item); if ($temp != '') $result['items'][$i][$itemtag] = $temp; // Set only if not empty } // Strip HTML tags and other bullshit from DESCRIPTION if ($this->stripHTML && $result['items'][$i]['description']) $result['items'][$i]['description'] = strip_tags($this->unhtmlentities(strip_tags($result['items'][$i]['description']))); // Strip HTML tags and other bullshit from TITLE if ($this->stripHTML && $result['items'][$i]['title']) $result['items'][$i]['title'] = strip_tags($this->unhtmlentities(strip_tags($result['items'][$i]['title']))); // If date_format is specified and pubDate is valid if ($this->date_format != '' && ($timestamp = strtotime($result['items'][$i]['pubDate'])) !==-1) { // convert pubDate to specified date format $result['items'][$i]['pubDate'] = date($this->date_format, $timestamp); } // Item counter $i++; } } $result['items_count'] = $i; return $result; } else // Error in opening return False { return False; } } } $rss = new lastRSS; $searchx = $_GET["search"]; $search = $_GET["search"]; $start = $_GET["page"]; $replace = " "; $with = "+"; $search = str_replace($replace, $with, $search); $title = "+"; $title2 = " "; $titlefinal = str_replace($title, $title2, $s); //Paging $perpage = "1"; $next = $start + $perpage; $past = $start - $perpage; ?> <?php if ($rs = $rss->get("http://api.search.yahoo.com/WebSearchService/rss/webSearch.xml?appid=yahoosearchwebrss&query=originurlextension%3Adoc+$search&adult_ok=1&start=$start")) { } foreach($rs['items'] as $item) { $title = $item['title']; $description = $item['description']; $urllink = $item['guid']; //Include the layout which echos the result include "idea/resulttemplate.php"; echo "\n"; } ?> And then, in other.php, remove the echo.
-
I'll show you how to make it with a MySQL database =) Create a new table, named searches. Inside this table, add 2 fields. You should make sure that you make a variable for the thing they search for. In this case, I'll name the variable $searched_for Now, when a user presses the search button, run the following query. mysql_query("INSERT INTO `searches`(`name`) VALUES('{$searched_for}')") or die(mysql_error()); Now when you want to show the 10 latest searches, you can do a for loop, like this: $select=mysql_query("SELECT * FROM `searches` ORDER BY `id` DESC") or die(mysql_error()); for($i=1;$i <= 10;$i++) { $row=mysql_fetch_array($select); echo $row['name']; } I think this should work. Not tested, but try it, and tell me if any errors occurs =)
-
How to read and write to a file - Trap 17 Tutorial Check it out =) Best wishes //AngelicS
-
So is it working?
-
Try adding this row: $salt_npass1 = md5($npass1.$salt); and then change the if statement to this: if ($salt_passn != $salt_npass1) { echo "The two new password didn't match"; }
-
if $result['items'] is the news items, you can use the count to count them by doing the following. If not, I'd like to know how you get the number of search results, so that I can think clearly. $items=count($result['items']); Now to decide how many pages you want, you divide the $items, with the number of results you want on every page, in this case - 10. Like this. $pages=$items/10; There you got the max pages. Is this what you need? Best wishes //AngelicS
-
You are trying to match the old and the new password. Is that what you want?
-
You do know that you are not using the variable $result1, which contains the query to delete something, right? Or at least that is what I think. //AngelicS
-
$result = mysql_query("SELECT userpass FROM Users WHERE usname = '{$_SESSION['usname']}'") or die(mysql_error()); if you try to echo the variable $results, I think that you wont get anything =) Am I right? If I am, try doing it like this instead: $result = mysql_query("SELECT userpass FROM Users WHERE usname = '{$_SESSION['usname']}'") or die(mysql_error()); $row=mysql_fetch_assoc($result); And then when you get to the if statement, change it to this: if ($salt_passo != $row['userpass']) { echo "Your old password was entered incorrectly"; } Best wishes //AngelicS
-
Check the register script, and make sure that you are using the same encryption salt =) That's my tip.
-
Try this =) $salt_passo = md5($opass.=$salt); $salt_passn = md5($npass.=$salt); Best wishes //AngelicS
-
Try this =) <?php include 'config.php'; include 'opendb.php'; session_start(); $opass = $_POST["opass"]; $npass = $_POST["npass"]; $npass1 = $_POST["npass1"]; $salt = 's+(_a*'; $salt_passo = md5($opass.$salt); $salt_passn = md5($npass.$salt); $result = mysql_query("SELECT userpass FROM Users WHERE usname = '{$_SESSION['usname']}'") or die(mysql_error()); if(isset($_SESSION['usname'])) { if ($salt_passo != $result) { echo "Your old password was entered incorrectly"; } elseif ($salt_passo != $salt_passn) { echo "The two new password didn't match"; } else { mysql_query("UPDATE `Users` SET `userpass`='{$salt_passn}' WHERE `usname` = '{$_SESSION['usname']}'") or die("Error: ".mysql_error()); } } else { echo '<meta http-equiv="refresh" content="2;url=index.php">'; } ?> I think you've been programming another language for a while, and newly started PHP? Instead of doing the following else{ if{ And forget to close both of them Try using the elseif() {} =) Best wishes //AngelicS
-
Did you even try to read my last post? Because the code you need is there.
-
The following code is normal HTML DOM: <div id="test"> Lalalalalalala </div> <div> <input type="button" value="click here" onclick="getElementById('test').innerHTML+='testtesttest'" /> </div> What it does is, when the button is pressed, it adds the text "testtesttest" after the "lalalalalala.." You can do the same thing but with ajax. When the timer expires, run a PHP script, and make sure you add the following code in the end of the function: getElementById('TheDivId').innerHTML+=xmlhttp.responseText; Inside the PHP script, check if there are any new news. If there is any new news item, make sure that you echo it. Hope this isn't confusing. You'll need to define the xmlhttp before you can use this function. Here's a link that might help. W3Schools - Ajax http://www.w3schools.com/ajax/ajax_browsers.asp Best wishes //AngelicS
-
According to what I know, the only way to get something from the database, or from the server itself, without refreshing the page, is by using AJAX. I don't think there is any other way. Edit: What you'll have to do is set a JavaScript timer, and every time the timer reaches 0, run an AJAX function, and reset the timer. That will set it in a loop, and the AJAX function will get what you need from the database and insert it into the news div. Best wishes //AngelicS
-
This is simple math =) Let's say I have the variable 10. I'd write it like this $num=10; Now I want to increase the value of that variable by 25%. All I need to do is multiply the variable with 1.25, which is +25%. The code would look like this: $num=10; $num*=1.25; echo $num; The output would be 12.5, because 0.25(25%) out of 10 is 2.5, and + the 1 (100%), it becomes 12.5. Hope this helped =) Best wishes //AngelicS
-
Have you tried the link that corbin sent you? Because you have the answer there. Try using the update statement: Let's say I have selected the total views on my page, and then I want to echo it. I'd do it like this: $sel=mysql_query("SELECT * FROM `statistics` WHERE `id`=1") OR die(mysql_error()); $row=mysql_fetch_array($sel); echo $row['views']; Now everytime a user visits the page, I want to increase the $row['views'] with one. I'd need to add the following rows. $row['views']+=1; mysql_query("UPDATE `statistics` SET views='{$row['views']}' WHERE `id`=1") OR die(mysql_error()); The first row, $row['views']+=1; , increases the value of "views" by one. The second row, Updates the value of "views" inside the database, where the ID is one (of course you can change that). Now the final code looks like this: <?php include("config.php"); $sel=mysql_query("SELECT * FROM `statistics` WHERE `id`=1") OR die(mysql_error()); $row=mysql_fetch_array($sel); $row['views']+=1; mysql_query("UPDATE `statistics` SET views='{$row['views']}' WHERE `id`=1") OR die(mysql_error()); echo $row['views']; ?> Check this site for more information about the UPDATE statement: W3Schools http://www.w3schools.com/php/php_mysql_update.asp
-
Can you just show me the code for the form, please? Best wishes //AngelicS
-
You don't use it
-
You'll have to find a way to find out how many pages there are, and then do the following. Create a variable that contains the maximum number of pages. For example: $max_pages=10 //In this case, but you might want to check by looking in a database or something. Then change the following code: if ($start > 1 && <= $max_pages) { // because 1 is the first page //Echo the current page, and add a "next" and "previous" button. echo "Page #$start <br/>"; echo "<a href=\"?page=$past\">Previous</a> |"; echo " <a href=\"?page=$next\">Next</a> "; } And add the following code: if ($start <= $max_pages) { echo "<a href=\"?page=$past\">Previous</a>"; } And about the search thing, you just have to add it =) Best wishes //AngelicS
-
I just realized that you don't use $id = $_GET['id']; May it be that you are using $id_post = $_POST['id']; instead, by mistake?
-
Try adding brackets to the else statement =) { } Best Wishes //AngelicS
-
Try learning from this =) <?php //if the page is not set, set it to 1 if(!$_GET['page']) $_GET['page']=1; //ser the variable start to the current page. $start = $_GET['page']; $perpage = 1; $next = $start + $perpage; $past = $start - $perpage; //If the current page is less than or equal to 1 ($perpage) if ($start <= $perpage) { //Echo the current page, and add a "next" button. echo "Page #$start <br/>"; echo "<a href=\"?page=$next\">Next</a>"; } //If the current page is bigger than one if ($start > 1) { // because 1 is the first page //Echo the current page, and add a "next" and "previous" button. echo "Page #$start <br/>"; echo "<a href=\"?page=$past\">Previous</a> |"; echo " <a href=\"?page=$next\">Next</a> "; } ?> Best wishes //AngelicS