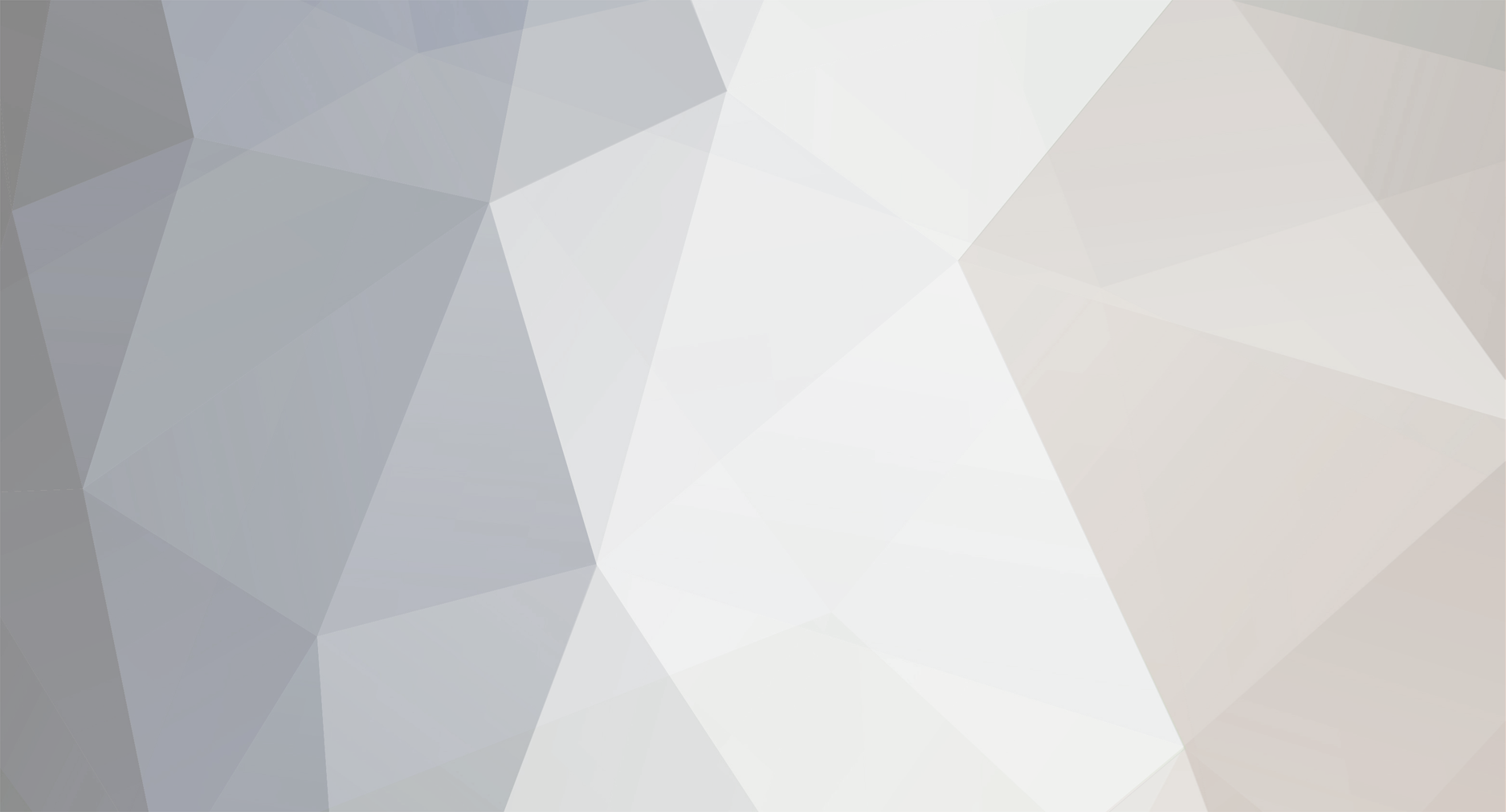
cbolson
Members-
Posts
139 -
Joined
-
Last visited
Never
Everything posted by cbolson
-
Hi, Firstly either your server needs to be configured to parse html pages as php (can be done via htaccess) or you need to change your extensions to .php. Secondly, assuming that the numeric value is always in the same place (ie first in your exampes) and that they are sperated by a hyphen you could do this: // get the file name from the url (note - there are several ways to get this) $file=basename($_SERVER["PHP_SELF"]); // split $file up using the hyphen - creates an array $file_parts=explode("-",$file); //define the first item in the array as your variable (maybe add controls to check it is numeric) $your_var=$file_parts[0]; Hope that helps Chris
-
[SOLVED] Need help with Displaying Checkboxes output after chosen
cbolson replied to Jnerocorp's topic in PHP Coding Help
If you take a look at the code, you will see that the +OR+ has been added manually: $link = "http://JneroCorp.com/?q=" . implode('+OR+', $typesAry) . "+OR+site:http://{$_POST['site']}/"; so just change that to this: $link = "http://JneroCorp.com/?q=" . implode('+OR+', $typesAry) . "+site:http://{$_POST['site']}/"; Chris. PS. using the implode() is clearly a better way to go, I just didn't want to get into too much detail of changing the original code -
[SOLVED] Need help with Displaying Checkboxes output after chosen
cbolson replied to Jnerocorp's topic in PHP Coding Help
$filetypes=""; if(count($_POST["filetype"]>0)){ foreach($_POST["filetype"] AS $key=>$val){ $filetypes.=$val; } $filetypes=substr($filetypes,0,-3); } should strip the last 3 characters off Chris -
[SOLVED] Need help with Displaying Checkboxes output after chosen
cbolson replied to Jnerocorp's topic in PHP Coding Help
OK, so you need to create a new variable rather than echoing the $var. Try this: // define filetypes from array $filetypes=""; if(count($_POST["filetype"]>0)){ foreach($_POST["filetype"] AS $key=>$val){ $filetypes.=$val; } } // define site url $url='site:'.$_POST["site"]; //put it together to make url $new_url='http://JneroCorp.com/?q='.$filetypes.$url; Personally I would consider removing the +OR+ part from the posted checkbox values and add them in the loop, but that won't actually make any difference. Chris -
[SOLVED] Need help with Displaying Checkboxes output after chosen
cbolson replied to Jnerocorp's topic in PHP Coding Help
once you submit the form you can see the array data for testing like this: print_r($_POST["filetype"]); This, in itself is not much use, just useful for showing the data that has been passed. How to use it would depend on what you want to do with the results. However, basically you need to loop through it to break it up into "usefull" data, something like this: if(count($_POST["filetype"]>0)){ foreach($_POST["filetype"] AS $key=>$val){ echo "Filetype selected: ".$val."<br>"; } } That will just give you a list of the filetypes checked, so you need to modify the line echo "Filetype selected: ".$val."<br>"; to get the code to do what you want it to with the user submitted data. Chris -
Hi, I think that you have got a little mixed up, either in your explanation or in the example of how the database data looks. You said: However, from the example data, it looks like the coinciding column is the "email" column. Also, it is a good idea to always have a unique numeric column in all tables and use this for referencing data between tables. (just a tip) Anyway, assuming you are using mysql database you could join the tables something like this: SELECT t1.friend, t2.email, t2.price FROM members AS t1 LEFT JOIN info AS t2 ON t2.email=t1.email WHERE t1.friend='user@hotemail.com' That might not be exactly right but should be a good starting point. Chris
-
[SOLVED] Need help with Displaying Checkboxes output after chosen
cbolson replied to Jnerocorp's topic in PHP Coding Help
Hi, You have the same name for all the checkboxes so it will only return the last one that is checked. You need to change them into an array and then access the array values in find2.php. <input type="checkbox" name="filetype[]" value=".txt+OR+"> .txt<br> <input type="checkbox" name="filetype[]" value=".bmp+OR+"> .bmp<br> <input type="checkbox" name="filetype[]" value=".gif+OR+"> .gif<br> <input type="checkbox" name="filetype[]" value=".jpg+OR+"> .jpg<br> Chris -
Sessions could be used once the variable for the menu has been sent (ie the menu item has been clicked) but I can't really see the benefit of this. You would still have to add the id menu item to the links for when the selected menu is changed. Personally I would actually use a different, more complicated but "cleaner" method. I would create a multidimensional array of all the menu items and their submenu items. I would use this to "draw" the menu rather than it being hardcoded. Then, on each page load I would check the url against this array to see which menu item is being shown (by checking it's herf value) and therefore "know" which item to open (as defined before). This has the advantage of keeping the urls as they are as you wouldn't need to add any variables to the links. The downside is that it would require a bit more programing Chris
-
Hi, Firstly I presume that you have an "id" field in the storedata table that is unique to each food type. This is what you should use to identify the various types rather than it's string value. For the form you create an array of food types (using the id) something like this: // get food types $list_types=''; $result=mysql_query("SELECT ID, TYPE FROM storedata"); while($row=mysql_fetch_assoc($result)){ $list_types.='<input type="checkbox" name="foodtypes['.$row["ID"].']" value="1">'.$row["TYPE"].'<br>'; } // make the form echo ' <form action="next_page.php" method='post'> '.$list_types.' <input type="submit" value="next"> </form> '; Then, in your next page you should have an array of food types as selected by the user, something like this: foodtypes=array(2,5,6) where each number is the id of the food type as selected by the user. Finally, you would need to modify your sql to only get these types, something like this: $sql_condition=""; if(count($_POST["foodtypes"])>0){ $sql_condition='' AND ("; // loop though items to define sql condition foreach($_POST["foodtypes"] AS $key=>$val{ $sql_condition.='' ID='".$key."' ||"; } // remove final pipes $sql_condition=substr($sql_condition,0,-3); // close condition bracket $sql_condition.=')'; } $result = mysql_query("SELECT * FROM storedata WHERE ID<>0 ".$sql_condition.""); while($row = mysql_fetch_assoc($result)){ ... output results.... { Note - I have not checked this code. I hope this makes sence Chris
-
Hi, not quite sure why this thread is in the mysql group.... Is there any reason why you are trying to do this with layers? I know everyone (including me) insists that layout should be done with layers rather than tables. However tables are the correct way to do things in some cases - ie when you want to display tabluar data. Here you are showing a list of items in various columns - surely that is tabluar data? Also, if you check in Opera and reduce the window width, you will see that it too messes up as the images float down below. I would suggest that, for the central list, you convert it to a table list. Chris
-
When the page is initially opend (ie nothing has been clicked), how is the menu shown? Are the items closed or open? Clearly I don't know what is in your css stylesheet, but, by looking at the html code the submenu items have the class "hidden" which would imply that this class is hiding the elements initially. So, when a link is clicked, all the items should be closed by default and then the javsascript (and php) would open only the menu item passed. Chris
-
Hi, not sure that it is the only problem with the script, but you are defining the textComponent by the id, however this is not defined. var textComponent = document.getElementById('outputtext'); For your textarea you have nly goiven it a name, if you want to use getElementById() you need to define the id attribute as well. <textarea id="outputtext" name="outputtext" cols="30" rows="25">Dear </textarea> Hope this helps you on your way Chris
-
Hi, To be able to keep the menu items open on the next page you need to "tell" the page which item was open on the previous page. You could do this be means of setting and resetting a cookie each time the user opens a menu, however, seeing as you are using php, I would opt to add a variable to the url to indicate which menu item to open. So, your links would look like this: <li class="nav"><a href="./totaloverview.php?id_menu=hidden1" target="_parent">Overview</a></li> where "hidden1" is the id of the parent menu item. Then, within the javsacript add some php to detect this value and, if defined, automatically open that menu item. Something like this: <script language="JavaScript" type="text/JavaScript"> <!-- menu_status = new Array(); function showHide(theid){ if (document.getElementById) { var switch_id = document.getElementById(theid); if(menu_status[theid] != 'show') { switch_id.className = 'show'; menu_status[theid] = 'show'; }else{ switch_id.className = 'hide'; menu_status[theid] = 'hide'; } } } <?php if($_GET["id_menu"]!=""){ // open this menu item echo showHide($_GET["id_menu"]); } ?> //--> </script> I haven't tested this, but I can't see any reason for it not to work. Of course, there are other methods, but this is probably the simplest solution. Chris
-
Hi, You have several syntax issues with your javascript that prevent it from "finishing" and that is why the code thinks that the functions are not defined. I have gone thorugh it and cleaned out the errors. Try replacing your javscript with this: currentIndx=0; MyImages=new Array(); MyImages[0]='vulcano.gif'; MyImages[1]='eye.gif'; MyImages[2]='ear.gif'; MyImages[3]='hand.gif'; Messages=new Array() Messages[0]='We learn about our world through the 5 senses'; Messages[1]='We use our eyes to see things'; Messages[2]='Our ears to hear things' Messages[3]='And our sense of touch to feel things.'; imagesPreloaded = new Array(4); for (var i = 0; i < MyImages.length ; i++){ imagesPreloaded[i] = new Image(120,120) imagesPreloaded[i].src=MyImages[i] } /*###### function to write image number in sequence, eg 1 of 4*/ function writeImageNumber(){ oSpan=document.getElementById("sp1"); oSpan.innerHTML="Image "+eval(currentIndx+1)+" of "+MyImages.length; } /* ####################### we create the functions to go forward and go back ####################### */ function Nexter(){ if (currentIndx<imagesPreloaded.length-1){ currentIndx=currentIndx+1; document.getElementById('text1').innerHTML=Messages[currentIndx]; }else { currentIndx=0 document.getElementById('text1').innerHTML=Messages[currentIndx]; } writeImageNumber(); } function Backer(){ if (currentIndx>0){ currentIndx=currentIndx-1; document.getElementById('text1').innerHTML = Messages[currentIndx]; }else { currentIndx=3 document.getElementById('text1').innerHTML = Messages[currentIndx]; } writeImageNumber(); } function automaticly() { document.getElementById('text1').innerHTML = Messages[currentIndx]; currentIndx=currentIndx+1; var delay = setTimeout("automaticly()",3500); } /*###### function to reload the images and text when refresh is pressed ##### */ function setCurrentIndex(){ currentIndx=0; document.getElementById('text1').innerHTML = Messages[0]; writeImageNumber(); } Chris PS. yes, it is me again - I'm following you