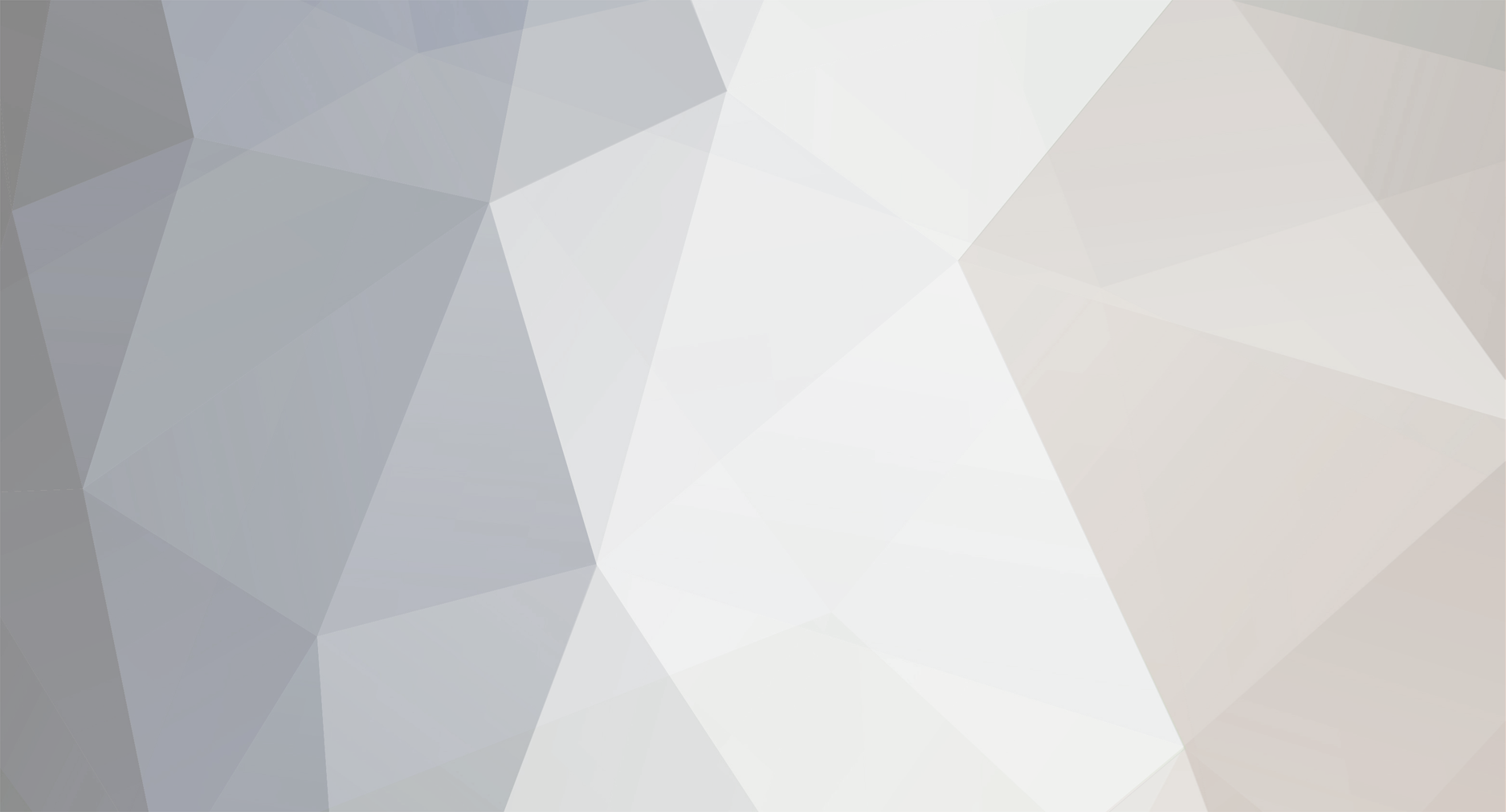
mds1256
Members-
Posts
259 -
Joined
-
Last visited
Everything posted by mds1256
-
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
Ok, I have switched to returning an array and using list as suggested and it works too it also like you suggests keeps my code readable. Thank you all who contributed to this, it is now working perfect -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
The passing by reference worked a treat! my amended code signin.php <?php require_once('_coreFunctions.php'); require_once('_signInFunctions.php'); sif_checkLoginForm($displayForm, $username, $error); // I am passing in the variables defined in _signInFuntions.php require_once('header.php'); ?> <div id="contentBoxWrapper"> <div id="contentBoxTop"> <div id="contentBoxTopInner"><a href="/" class="firstLink">Home</a> <span class="breadcrumbDivider">></span> Sign In<hr /></div> </div> <div id="contentBoxContent"> <div id="signInFormWrapper"> <h1>Sign In</h1> <?php if($displayForm) { echo " <form name=\"signInForm\" id=\"signInForm\" method=\"post\" action=\"\"> <label for=\"username\">Username:</label><br /> <input type=\"text\" name=\"username\" id=\"signInFormUsername\" class=\"formField\" value=\"{$username}\" /> <br /><br /> <label for=\"password\">Password:</label><br /> <input type=\"password\" name=\"password\" id=\"signInFormPassword\" class=\"formField\" /><br /><br /> <input type=\"submit\" id=\"signInSubmit\" name=\"signInSubmit\" value=\"Login\" /> </form> <br /> {$error} "; } ?> <br /> <a href="resetpassword">Forgotten password?</a> </div> </div> <div id="contentBoxBottom"></div> </div> <?php require_once('footer.php'); ?> _signInFunctions.php <?php require_once('_coreFunctions.php'); $displayForm = true; // define the variables that are needed on the signin.php page $username = ""; $error = ""; function sif_checkLoginForm(&$displayForm, &$username, &$error) // pass in references to these variables so they can be accessed { if(isset($_POST['signInSubmit'])) { $username = mysqli_real_escape_string(cf_dbConnect(), $_POST['username']); $password = mysqli_real_escape_string(cf_dbConnect(), $_POST['password']); $query = mysqli_query(cf_dbConnect(), "call sp_checkLoginDetails('{$username}', '{$password}')"); if(mysqli_num_rows($query) == 1) { $_SESSION['isLoggedIn'] = true; while($row = mysqli_fetch_object($query)) { $_SESSION['firstName'] = $row->firstName; } mysqli_query(cf_dbConnect(), "call sp_updateLoginDateTime('{$_SESSION['firstName']}')"); header("Location: ."); die(); } else { $error = "<div id=\"formMessages\">Username or Password is incorrect. Please try again.</div>"; if($username == "username...") { $username = ""; } } } } ?> -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
Ok, I have refactored my code just to try and get this working then I will start sorting the functions out to display the form. The problem is now that I am losing variables due to scope, I DONT want to create globals as I hear they are not good. Basically I need to use the $displayForm variable that is defined in the function to be accessible outside of the function and on the signin.php page, the same would go for the $error variable too? any ideas on the best way to do this? Thanks signin.php <?php require_once('_coreFunctions.php'); require_once('_signInFunctions.php'); sif_checkLoginForm(); // calling the check form function where $displayForm is defined require_once('header.php'); ?> <div id="contentBoxWrapper"> <div id="contentBoxTop"> <div id="contentBoxTopInner"><a href="/" class="firstLink">Home</a> <span class="breadcrumbDivider">></span> Sign In<hr /></div> </div> <div id="contentBoxContent"> <div id="signInFormWrapper"> <h1>Sign In</h1> <?php if($displayForm) // this variable isnt available due to scoping problems { echo " <form name=\"signInForm\" id=\"signInForm\" method=\"post\" action=\"\"> <label for=\"username\">Username:</label><br /> <input type=\"text\" name=\"username\" id=\"signInFormUsername\" class=\"formField\" value=\"{$username}\" /> <br /><br /> <label for=\"password\">Password:</label><br /> <input type=\"password\" name=\"password\" id=\"signInFormPassword\" class=\"formField\" /><br /><br /> <input type=\"submit\" id=\"signInSubmit\" name=\"signInSubmit\" value=\"Login\" /> </form> <br /> {$error} "; } ?> <br /> <a href="resetpassword">Forgotten password?</a> </div> </div> <div id="contentBoxBottom"></div> </div> <?php require_once('footer.php'); ?> _signInFunctions.php <?php require_once('_coreFunctions.php'); function sif_checkLoginForm() { $username = ""; $displayForm = true; // defined here, dont want to use GLOBALS if(isset($_POST['signInSubmit'])) { $username = mysqli_real_escape_string(cf_dbConnect(), $_POST['username']); $password = mysqli_real_escape_string(cf_dbConnect(), $_POST['password']); $query = mysqli_query(cf_dbConnect(), "call sp_checkLoginDetails('{$username}', '{$password}')"); if(mysqli_num_rows($query) == 1) { $_SESSION['isLoggedIn'] = true; while($row = mysqli_fetch_object($query)) { $_SESSION['firstName'] = $row->firstName; } mysqli_query(cf_dbConnect(), "call sp_updateLoginDateTime('{$_SESSION['firstName']}')"); header("Location: ."); die(); } else { $error = "<div id=\"formMessages\">Username or Password is incorrect. Please try again.</div>"; if($username == "username...") { $username = ""; } } } } ?> -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
So have one function that will check if form is posted (like the one I already have) and then also have another function to echo the form out? Do you have a very basic example on what you mean by the return construct to exit? -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
use an if() condition Thanks for the above code, that is what I was thinking towards, when you say use an IF(), i am guessing I would need to put the if condition in the signin.php around the form which is back in normal HTML, so wouldnt that be mixing layout with logic again e.g. <?php require_once('_coreFunctions.php'); // this is where DB functions are e.g. connection etc require_once('_signInFunctions.php'); $displayForm = true; $error = ""; $username = ""; if(isset($_POST['signInSubmit'])) { $username = mysqli_real_escape_string(cf_dbConnect(), $_POST['username']); $password = mysqli_real_escape_string(cf_dbConnect(), $_POST['password']); $query = mysqli_query(cf_dbConnect(), "call sp_checkLoginDetails('{$username}', '{$password}')"); if(mysqli_num_rows($query) == 1) { $_SESSION['isLoggedIn'] = true; while($row = mysqli_fetch_object($query)) { $_SESSION['firstName'] = $row->firstName; } mysqli_query(cf_dbConnect(), "call sp_updateLoginDateTime('{$_SESSION['firstName']}')"); header("Location: ."); // this is my redirect part } else { $error = "<div id=\"formMessages\">Username or Password is incorrect. Please try again.</div>"; if($username == "username...") { $username = ""; } } } require_once('header.php'); // this has HTML inside of it e.g. head tag etc ?> <div id="contentBoxWrapper"> <div id="contentBoxTop"> <div id="contentBoxTopInner"><a href="/" class="firstLink">Home</a> <span class="breadcrumbDivider">></span> Sign In<hr /></div> </div> <div id="contentBoxContent"> <div id="signInFormWrapper"> <h1>Sign In</h1> <?php if (isset($error)) {echo $error;} ?> <?php if(!isset($_POST['submit'])) { echo " <form name=\"signInForm\" id=\"signInForm\" method=\"post\" action=\"\"> <label for=\"username\">Username:</label><br /> <input type=\"text\" name=\"username\" id=\"signInFormUsername\" class=\"formField\" value=\"{$username}\" /><br /><br /> <label for=\"password\">Password:</label><br /> <input type=\"password\" name=\"password\" id=\"signInFormPassword\" class=\"formField\" /><br /><br /> <input type=\"submit\" id=\"signInSubmit\" name=\"signInSubmit\" value=\"Login\" /> </form>"; } ?> <br /> <a href="resetpassword">Forgotten password?</a> </div> </div> <div id="contentBoxBottom"></div> </div> <?php require_once('footer.php'); ?> -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
Ok, I have came up with an idea, using the principle of seperating out the business logic from the layout. Rather than echoing out the form code from within the function, I can call the function at the top of the page before any HTML e.g. before the header.php include. The fuction will still have the if - post so will still work. But.... Using this principle if I use the same idea for a contact form as an example and I then want to hide the form once submitted and show a response message of 'thank you for contacting us', how would I achieve this using this new method as the form code would always be hard coded into the html page? -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
I tried a few ideas which also didnt work by moving things about. I could call the function before any HTML output but how would I get the form to display where I want it rather than the top of the page before the header etc. At the moment I have signin.php <?php require_once('_coreFunctions.php'); // this is where DB functions are e.g. connection etc require_once('_signInFunctions.php'); require_once('header.php'); // this has HTML inside of it e.g. head tag etc ?> <div id="contentBoxWrapper"> <div id="contentBoxTop"> <div id="contentBoxTopInner"><a href="/" class="firstLink">Home</a> <span class="breadcrumbDivider">></span> Sign In<hr /></div> </div> <div id="contentBoxContent"> <div id="signInFormWrapper"> <h1>Sign In</h1> <?php sif_loginForm(); ?> // this is where I call the login form function...... <br /> <a href="resetpassword">Forgotten password?</a> </div> </div> <div id="contentBoxBottom"></div> </div> <?php require_once('footer.php'); ?> _signInFunctions.php <?php require_once('_coreFunctions.php'); function sif_loginForm() { $displayForm = true; $error = ""; $username = ""; if(isset($_POST['signInSubmit'])) { $username = mysqli_real_escape_string(cf_dbConnect(), $_POST['username']); $password = mysqli_real_escape_string(cf_dbConnect(), $_POST['password']); $query = mysqli_query(cf_dbConnect(), "call sp_checkLoginDetails('{$username}', '{$password}')"); if(mysqli_num_rows($query) == 1) { $_SESSION['isLoggedIn'] = true; while($row = mysqli_fetch_object($query)) { $_SESSION['firstName'] = $row->firstName; } mysqli_query(cf_dbConnect(), "call sp_updateLoginDateTime('{$_SESSION['firstName']}')"); header("Location: ."); // this is my redirect part } else { $error = "<div id=\"formMessages\">Username or Password is incorrect. Please try again.</div>"; if($username == "username...") { $username = ""; } } } if($displayForm) { echo " <form name=\"signInForm\" id=\"signInForm\" method=\"post\" action=\"\"> <label for=\"username\">Username:</label><br /> <input type=\"text\" name=\"username\" id=\"signInFormUsername\" class=\"formField\" value=\"{$username}\" /><br /><br /> <label for=\"password\">Password:</label><br /> <input type=\"password\" name=\"password\" id=\"signInFormPassword\" class=\"formField\" /><br /><br /> <input type=\"submit\" id=\"signInSubmit\" name=\"signInSubmit\" value=\"Login\" /> </form> <br /> {$error} "; } } ?> -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
I removed the register form but still with the same problem, I am misunderstanding what removing this web form is achieving? -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
Thanks for the tip The sif_loginForm function is used on the signin.php inside the signInFormWrapper DIV I have had a look at that artice and like I say I know why this is happening, just trying to get the best solution? -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
That part is working so not sure why you keep looking at that part? -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
Yeah I know, I didn't mean what does the code do, I know what that part does as I wrote it. What I meant was what is that going to achieve as it doesn't have anything to do with what I am asking. -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
very helpful. The login part works fine, its the re-direct that doesnt.... Like I said forget about the register form at the moment, it has no bearing on what I am trying to achieve. -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
Forget about the register form, it doesnt do anything at the moment and is just a placeholder for the form at the moment. the username is an email address. what does that code do? -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
Yeah, this is my current code... I cannot seem to find a way to call the function before HTML has been outputted due to the header and where in the HTML this function is being called. I had a bit of a mess about with ob_start() and ob flush and that worked but that is more of a sticking plaster rather than a solution. the _coreFunctions.php is being called in the whole find so it doesnt need to be re-called in the function. -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
Yeah. My whole code is as follows: signin.php <?php require_once('_coreFunctions.php'); // this is where DB functions are e.g. connection etc require_once('_signInFunctions.php'); require_once('header.php'); // this has HTML inside of it e.g. head tag etc ?> <div id="contentBoxWrapper"> <div id="contentBoxTop"> <div id="contentBoxTopInner"><a href="/" class="firstLink">Home</a> <span class="breadcrumbDivider">></span> Sign In<hr /></div> </div> <div id="contentBoxContent"> <div id="signInFormWrapper"> <h1>Sign In</h1> <?php sif_loginForm(); ?> // this is where I call the login form function...... <br /> <a href="resetpassword">Forgotten password?</a> </div> <div id="signInRegisterFormWrapper"> <h1>Register Now</h1> <form name="registerForm" method="post" action=""> <label for="username">Username:</label><br /><input type="text" name="username" class="formField" /><br /><br /> <label for="password">Password:</label><br /><input type="password" name="password" class="formField" /><br /><br /> <input type="submit" value="Register" /> </form> </div> </div> <div id="contentBoxBottom"></div> </div> <?php require_once('footer.php'); ?> _signInFunctions.php <?php require_once('_coreFunctions.php'); function sif_loginForm() { $displayForm = true; $error = ""; $username = ""; if(isset($_POST['signInSubmit'])) { $username = mysqli_real_escape_string(cf_dbConnect(), $_POST['username']); $password = mysqli_real_escape_string(cf_dbConnect(), $_POST['password']); $query = mysqli_query(cf_dbConnect(), "call sp_checkLoginDetails('{$username}', '{$password}')"); if(mysqli_num_rows($query) == 1) { $_SESSION['isLoggedIn'] = true; while($row = mysqli_fetch_object($query)) { $_SESSION['firstName'] = $row->firstName; } mysqli_query(cf_dbConnect(), "call sp_updateLoginDateTime('{$_SESSION['firstName']}')"); header("Location: ."); // this is my redirect part } else { $error = "<div id=\"formMessages\">Username or Password is incorrect. Please try again.</div>"; if($username == "username...") { $username = ""; } } } if($displayForm) { echo " <form name=\"signInForm\" id=\"signInForm\" method=\"post\" action=\"\"> <label for=\"username\">Username:</label><br /> <input type=\"text\" name=\"username\" id=\"signInFormUsername\" class=\"formField\" value=\"{$username}\" /><br /><br /> <label for=\"password\">Password:</label><br /> <input type=\"password\" name=\"password\" id=\"signInFormPassword\" class=\"formField\" /><br /><br /> <input type=\"submit\" id=\"signInSubmit\" name=\"signInSubmit\" value=\"Login\" /> </form> <br /> {$error} "; } } ?> -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
nope, my code.... will give it ago. -
Redirect to different page after successful login
mds1256 replied to mds1256's topic in PHP Coding Help
I know why its doing it as I am calling the header etc. e.g. <?php require_once('header.php'); ?> <p>some general login text here.....</p> <?php loginForm(); ?> -
Looking to redirect my user to a new page after successfully logging in via a form. I have refactored my code slightly to cut down on unnecessary code so the I was doing it no longer works (getting the headers already sent error), I know why this is happening but I need some ideas on how I can now achieve this? Any ideas would be great. function loginForm() { $displayForm = true; $error = ""; $username = ""; if(isset($_POST['signInSubmit'])) { $username = mysqli_real_escape_string(cf_dbConnect(), $_POST['username']); $password = mysqli_real_escape_string(cf_dbConnect(), $_POST['password']); $query = mysqli_query(cf_dbConnect(), "call sp_checkLoginDetails('{$username}', '{$password}')"); if(mysqli_num_rows($query) == 1) { $_SESSION['isLoggedIn'] = true; while($row = mysqli_fetch_object($query)) { $_SESSION['firstName'] = $row->firstName; } mysqli_query(cf_dbConnect(), "call sp_updateLoginDateTime('{$_SESSION['firstName']}')"); header("Location: ."); // this is the point where the headers are already sent I know why this happens, need an alternative way } else { $error = "<div id=\"formMessages\">Username or Password is incorrect. Please try again.</div>"; } } if($displayForm) { echo " <form name=\"signInForm\" id=\"signInForm\" method=\"post\" action=\"\"> <label for=\"username\">Username:</label><br /> <input type=\"text\" name=\"username\" id=\"signInFormUsername\" class=\"formField\" value=\"{$username}\" /><br /><br /> <label for=\"password\">Password:</label><br /> <input type=\"password\" name=\"password\" id=\"signInFormPassword\" class=\"formField\" /><br /><br /> <input type=\"submit\" id=\"signInSubmit\" name=\"signInSubmit\" value=\"Login\" /> </form> <br /> {$error} "; } }
-
Good idea! Thanks again for this
-
Thanks! That seems to work <?php function form() { $error = ""; $nameVal = ""; $emailVal = ""; $bool = true; if(isset($_POST['submit'])) { $nameVal = $_POST['name']; $emailVal = $_POST['email']; if($_POST['name'] <> "John") { $error = "not the correct name"; } else { $bool = false; echo "Posted Sucessfully"; } } if($bool == true) { echo " <form action=\"\" method=\"post\" name=\"form1\"> <input id=\"name\" name=\"name\" type=\"text\" value=\"{$nameVal}\" /><br /> <input id=\"email\" name=\"email\" type=\"text\" value=\"{$emailVal}\" /><br /> <input name=\"submit\" id=\"submit\" type=\"submit\" /> </form> <br /> {$error} "; } } ?> <html> <head> </head> <body> <?php form(); ?> </body> </html>
-
Hi I am looking to create a form that will display an error message with the fields still populated. I am after some advice on how I would achieve it. If the form contains errors then I want to display an error message underneath the form and have the fields populated with the data they have already entered. If the form submission was successful then I want to hide the form and show just a confirmation message (this is the part I am having trouble with as I can achieve this but It means I would need to duplicate the form code and have it twice in my function and I dont want that). My code I have chucked together (very roughly) to demonstrate my issue: <?php function form() { $error = ""; $nameVal = ""; $emailVal = ""; if(isset($_POST['submit'])) { $nameVal = $_POST['name']; $emailVal = $_POST['email']; if($_POST['name'] <> "John") { $error = "not the correct name"; } else { // hide form and display confirmation message here } } echo " <form action=\"\" method=\"post\" name=\"form1\"> <input id=\"name\" name=\"name\" type=\"text\" value=\"{$nameVal}\" /><br /> <input id=\"email\" name=\"email\" type=\"text\" value=\"{$emailVal}\" /><br /> <input name=\"submit\" id=\"submit\" type=\"submit\" /> </form> <br /> {$error} "; } ?> <html> <head> </head> <body> <?php form(); ?> </body> </html>
-
Not really sure what you mean so I would say no. the use of stored procedures is something that I have read removes the possibility of a SQL injection, I am not using them for everything but for crucial things like username and password checking etc. Is there a better way of preventing a SQL injection rather than using Stored Procedures?
-
I don't know, someone having more experience have to say.. Ok, thanks very much for your time.
-
yes. I have managed to hack about and do what I am after with the concat function, is there not an easier way to achieve this -- -------------------------------------------------------------------------------- -- Routine DDL -- Note: comments before and after the routine body will not be stored by the server -- -------------------------------------------------------------------------------- DELIMITER $$ CREATE DEFINER=`root`@`localhost` PROCEDURE sp_selectNewsArticles(IN input VARCHAR(255)) BEGIN SET @query = CONCAT('SELECT id, newsTitle FROM tbl_newsArticles order by ',input,' desc'); PREPARE stmt FROM @query; EXECUTE stmt; DEALLOCATE PREPARE stmt; END call sp_selectNewsArticles('id')