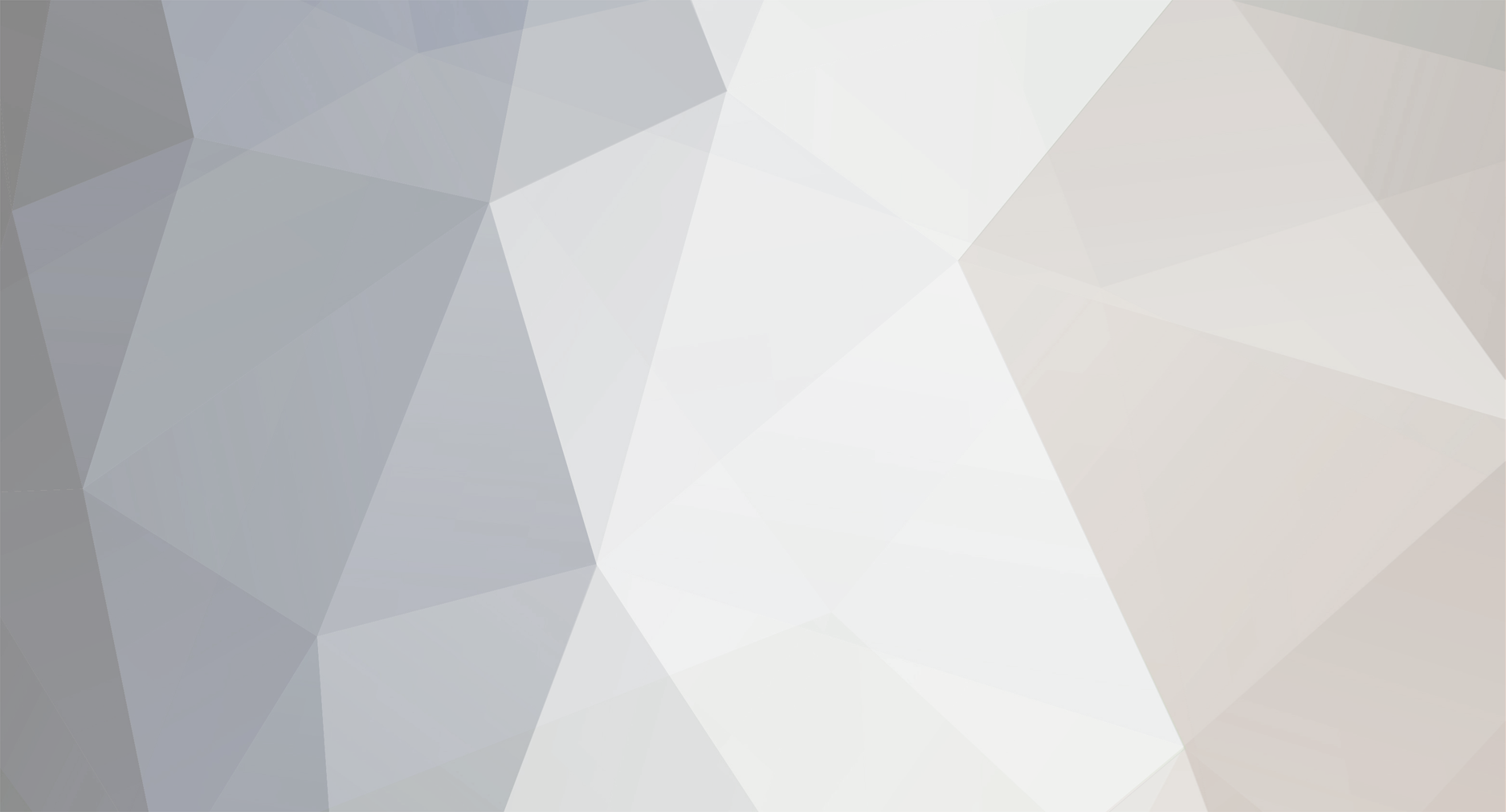
mds1256
Members-
Posts
259 -
Joined
-
Last visited
Everything posted by mds1256
-
Hi Looking to create a web site using php OOP, I understand the concepts of objects and classes etc. But I am wanting to achieve the following: class for retrieving news articles on a website - so I have a news content box that contains around 5 news articles titles that are links to the main articles. How would I do this in regard to methods? I am thinking: functions.php <?php class functions { public static function dbConnect() { mysql_connect('localhost', 'user', 'pass'); mysql_select_db('database'); } } ?> news.php <?php class news { public function getNews() { $query = mysql_query("select * from news limit 5"); return $query; } } ?> index.php <html> <?php require_once('functions.php'); require_once('news.php'); ?> <body> <?php functions::dbConnect(); $news = new news(); $newsresultset = $news->getNews(); while($row = mysql_fetch_assoc($newsresultset)) { echo $row['title']."<br />"; } ?> </body> </html> Is the above the best way to create and use the methods or is there a better way? Thanks
-
That is great! You are on fire, you have helped me out on a few things over the past few days. Thanks very much
-
The below scenario works fine but why shouldnt you do this? Why can I not just run the mysql_connect and mysql_select_db at the top of each page and then run my queries under all of this. What I have read is that the mysql_connection will die after the script finishes (at the end of the page) any way so why do people create mysql_connection objects as php is stateless so it doesnt save this any way. <?php mysql_connect('localhost', 'user', 'pass'); mysql_select_db('test'); ?> <html> <body> <?php $query = mysql_query("Select * from data"); while($row = mysql_fetch_array($query)) { echo $row['name']."<br />"; } ?> -------------- OTHER HTML HERE --------------- <?php $query = mysql_query("Select * from addresses"); while($row = mysql_fetch_array($query)) { echo $row['postcode']."<br />"; } ?> </body> </html>
-
Yes. Make the connection first and pass it into your object upon instantiation. Your querying for two different users so not really. As I said, a "Person" represents a single "person". The latter is correct, I have forgot I am working with objects woops... Do you have example of passing a connection object into this object. Also can you use array attributes on methods eg.: $person = getName()['firstname']
-
Hi, thanks for the reply. I am using singleton way so it doesnt create a new database connection / object each time i need to query the database? Is there a better way of achieving this. In the end I would like a database class that has a query method that I can use for each time I need to pull back results. so for example I need the query method to be dynamic and allow a return of an array, I then need to know the best way of allowing me to dynamically handling this array (maybe with another database method). I can do the following but if you look at the index page you will see that I need to store the returned array in a variable first then echo the variable with the array element. Looks a bit messy, is there a way to do this in a one liner? Database class: <?php class Database { private static $dbInstance; private $hostname; private $username; private $password; private $database; private function __construct() { $this->hostname = 'localhost'; $this->username = 'user'; $this->password = 'pass'; $this->database = 'test'; mysql_connect($this->hostname, $this->username, $this->password); mysql_select_db($this->database); } public static function getDBInstance() { if (!self::$dbInstance) { self::$dbInstance = new Database(); } return self::$dbInstance; } public function query($q) { return mysql_query($q); } } ?> person class: <?php require_once('Database.php'); class person { public function getName($id) { $con = Database::getDBInstance(); $query = $con->query("select name from data where id = ".$id); return mysql_fetch_assoc($query); } } ?> index page: <?php require_once('person.php'); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Untitled Document</title> </head> <body> <?php $person = new person(); $name = $person->getName(1); echo $name['name']; echo "<br />"; $name = $person->getName(2); echo $name['name']; ?> </body> </html>
-
Hi I have created a Database class that uses the singleton method. I have a query method within this class so i would call this to return an array of results. What i need to know is what would be the best way to use this returned array Database Class: <?php class Database { private static $dbInstance; private $hostname; private $username; private $password; private $database; private function __construct() { $this->hostname = 'localhost'; $this->username = 'user'; $this->password = 'pass'; $this->database = 'test'; mysql_connect($this->hostname, $this->username, $this->password); mysql_select_db($this->database); } public static function getDBInstance() { if (!self::$dbInstance) { self::$dbInstance = new Database(); } return self::$dbInstance; } public function query($q) { return mysql_query($q); } } ?> Person Class: <?php require_once('Database.php'); class person { public function getName($id) { $con = Database::getDBInstance(); $query = $con->query("select name from data where id = ".$id); $result = mysql_fetch_assoc($query); echo $result['name']; } } ?> Index page: <?php require_once('person.php'); ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Untitled Document</title> </head> <body> <?php $person = new person(); $person->getName(1); echo "<br />"; $person->getName(2); ?> </body> </html>
-
What is the best way to backup a MySQL database which has 24 hours access to clients. With ms SQL I have setup maintenance plans that do a full backup every night and creates logs every hour. This would allow me to restore database Up to a certain point and could even look at the logs and use them to rebuilt to that point in time if I needed to. I need to do this on a MySQL server and I know you can use mysqldump but how can I get it to backup nightly and create logs ever hour? Any good software for setting these up, on a windows environment.
-
Thanks for the above that is exactly what I thought but needed someone to confirm this. Thanks again
-
Thanks, so is this secure in that no one can just tamper with anything to add a session variable of logged_in with a value of 1? Also how do you then retrieve data about a user from the database it will need some type of reference number, should that be stored in the session too?
-
Hi What is the best way of handling a login system with sessions, I have read that you should never hold the password in a session, so what should you hold in the session in order to access a users data?
-
I am also not being rude either but I copied and pasted their code and amended as required so the style tag was originally copied. I am not going to spend hours on this as they only wanted an idea of how to do it. There is nothing wrong with the code posted and you don't know how the rest of the design will shape up so using relative is not really a problem.
-
Try the below! <style> #container { width:177px; float:left; margin-right:50px; } #div1 { background: yellow; position:relative; width:177px; } #div2 { background: green; position:relative; width:177px; margin-top:20px; } #div3 { float:left; background: blue; position:relative; width:500px; } </style> <div id="container"> <div id="div1"> <p><strong>DIV1:</strong> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus quis ante leo. Fusce at lacus risus. Fusce felis tortor, vehicula ut vulputate sit amet, ultricies sit amet risus.</p> </div> <div id="div2"> <p><strong>DIV2:</strong>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus quis ante leo. Fusce at lacus risus. Fusce felis tortor, vehicula ut vulputate sit amet, ultricies sit amet risus.</p> </div> </div> <div id="div3"> <p><strong>DIV3:</strong>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus quis ante leo. Fusce at lacus risus. Fusce felis tortor, vehicula ut vulputate sit amet, ultricies sit amet risus. Donec venenatis, nulla et ultrices varius, ligula sapien pharetra dolor, eget vulputate justo felis ac leo. Morbi nec ullamcorper purus. Nulla mollis dignissim nisi in imperdiet. Phasellus convallis ante a felis tincidunt hendrerit. Praesent ipsum lorem, mollis sed dignissim non, consectetur non ipsum. Nam euismod, nisi ut ultricies aliquet, lorem erat pretium tortor, eget volutpat lectus tellus at magna. Curabitur a est ante, interdum faucibus ipsum. Mauris vitae tincidunt nisl. Praesent semper erat sit amet augue pharetra tristique. Morbi imperdiet, magna et malesuada interdum, massa ipsum venenatis est, sit amet egestas risus sem a purus.</p> <p>Duis in sagittis justo. Quisque sit amet felis in tortor pharetra bibendum. Mauris eget purus sit amet magna vulputate ultricies. Nam non dignissim nisl. Suspendisse pretium malesuada dolor, non lacinia tellus imperdiet ullamcorper. Sed commodo placerat lacus eu bibendum. In id elit sed velit iaculis pulvinar et id elit. Cras sagittis porttitor tristique. Vivamus sed sodales lectus. Nulla interdum, mi sit amet volutpat feugiat, nunc erat consectetur neque, rutrum rutrum lorem nisl pellentesque magna. Maecenas mollis consequat fringilla. Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas. Etiam eu mi pellentesque turpis faucibus vestibulum quis in ligula. Donec mollis tempor velit, eget vestibulum ante elementum ac. In gravida, purus sed faucibus rhoncus, arcu augue pretium nibh, ut faucibus justo ante vitae metus. Nunc sit amet mauris urna.</p> </div>
-
not done much with ternary operators, but they are quite useful i have learnt
-
You are a legend!!!!! excatly what i was after! THANKS!
-
Creating dynamic drop down from db without repeat
mds1256 replied to schmidt82's topic in PHP Coding Help
This defintley works as i have just tested it. Make sure your database is all good as well, e.g. make sure you dont have a city name in twice with different 'city_id' <?php header('Content-Type: text/html; charset=iso-8859-1'); include 'connect.php'; $city_query = "SELECT DISTINCT city, city_id FROM barer"; $city_result = mysql_query($city_query); $optionsid = ""; while($row = mysql_fetch_array($city_result)) { $optionsid.="<option value=\"$row[city_id]\">".$row[city]."</option>"; } ?> <html> <select name="city"> <?php echo $optionsid ?> </select> </html> -
Thats fine but i want it to default to its default value is nothing is passed in not just display nothing
-
bump, any one?
-
kind of but when the errorID is null or blank it doesnt give any value from the function. I was also hoping to all the checking inside the function instead of on the page where i am going to use this function <?php function error($errorID=0) { echo $errorID; } if(isset($_GET['errorID'])) { error($_GET['errorID']); } else { error(); } ?>
-
Hello After some advice I have the following code but need to get the function to have a default value if a GET variable is not set. So if i use error(); i get the default function value which is fine and is what i want but when i add an argument of GET and the GET is not set then it doesnt work and displays blank. <?php function error($errorID=0) { echo $errorID; } error($_GET['errorID']); ?>
-
Very true! but if you change the location of the matching id field onto the address table you could have multiple address entries with the same userID and it would give you the same result So what is the benefit, I guess if you want a many to many relationship this will justify it
-
Just wondering what people's views are on bridging tables. Should I use them rather than creating a new field in the table to reference the other tables row number. e.g. Bridging table method: Users table: userID userName userPassword 1 abcd 1234 Address table: AddressID houseNo streetName postCode 5 52 New Street AA2 4DD Bridge table: bridgeID userID addressID 1 1 5 Same table method: Users table: userID userName userPassword addressID 1 abcd 1234 5 Address table: AddressID houseNo streetName postCode 5 52 New Street AA2 4DD
-
Thanks! Have ordered it, will give it a read
-
What about sessions? My idea is that when someone logs in it keeps there details in a session, which will be used for queries. e.g. username so when I select i can use the username from the session in the where clause. What about storing passwords (Salted and md5 hash) in a session, should I be doing this? And is it ok to pass user details like the above into my custom functions e.g. function getDetails($username, $password) { ............................. }
-
Ok thanks. Also what about mysql_connect(), should i open a connection at the top of each page or open and close in each function? I have read that once the page has loaded any way that the connection ends?
-
Was just looking for generic should know stuff. Had a question around mysql queries, one of the things I have to implement is to log all access attemps to an audit table within the database. So from the login page the php will run 2 queries, one checking (selecting) the details against the ones in the database and also at the same time logging them details (username, attempt time, ip address and authentication result). The only way I can see to do this is to have multiple mysql_query()'s happening. Is this ok to do or is there a better way of doing this? Thanks you for all the replies! Will all come in handy