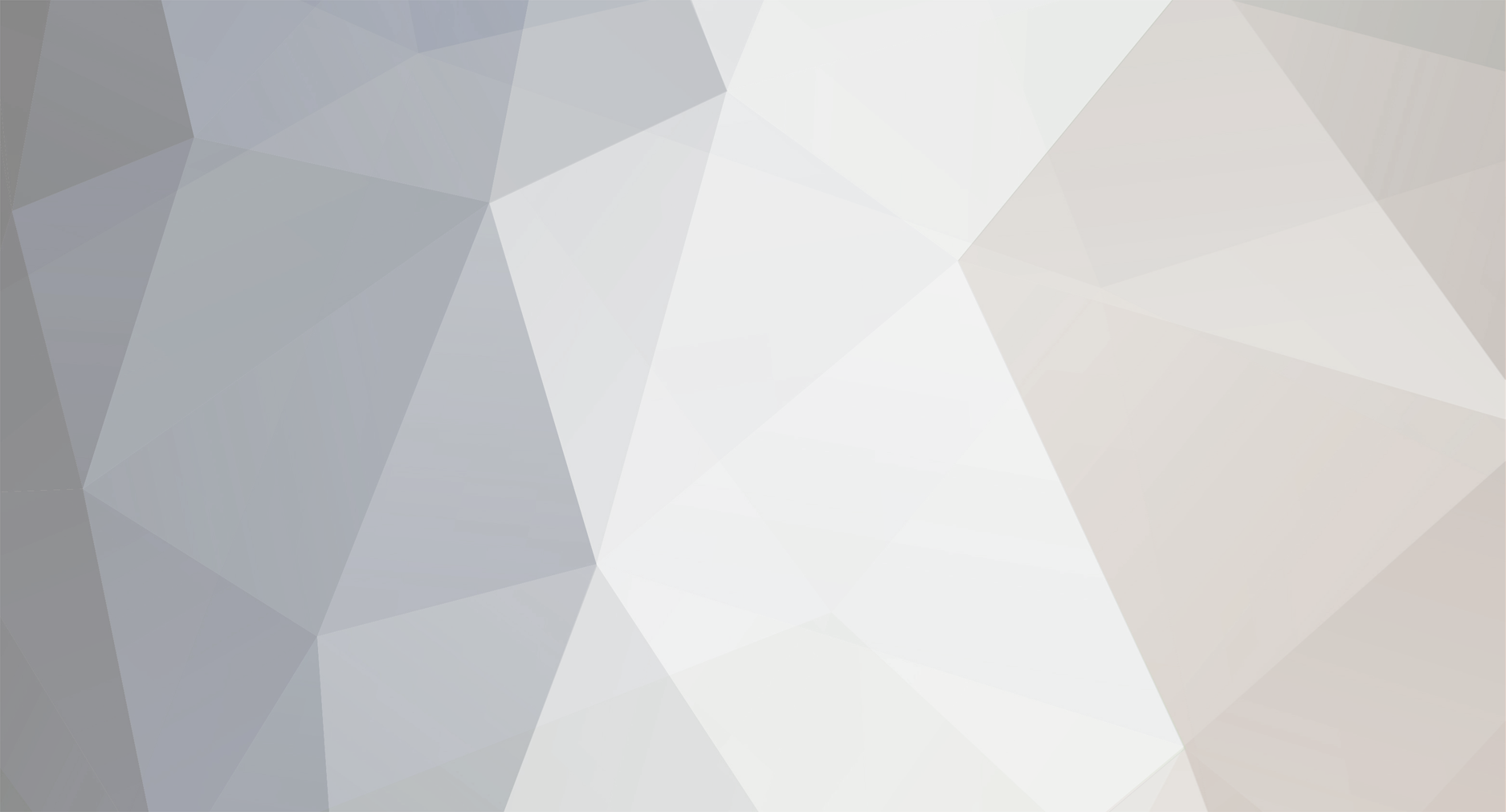
mds1256
Members-
Posts
259 -
Joined
-
Last visited
Everything posted by mds1256
-
That makes sense - so you can forbid a client APP but still allow a user - for example you release two applications which can authenticate using the same user details but then you retire one of the apps so you would then remove that Client id from the allowed list which prevents the app from working with the API but still allowing the other APP access. The goal is to create a mobile app that authenticates with a back end to using tokens - the user first logs into the mobile app using a username and password which will send off a request for a token - the server will response with the token if the details are correct and then the mobile app will record the token and send this in each request to the server. The part i was struggling with was why do i need a client id and secret as well as a username and password - but i guess if i want to control the client app access as well as the user access this is why i need the two. Can you confirm that this thought is correct?
-
Ok so the test OAuth2 server i have created must have the following provided in order to provide the access token: 1. header - grant_type = password 2. header - username 3. header - password 4. Authorization header - client id:client secret otherwise it wont issue an access token. So what I am confused over is why do you need to provide a username and password and also a client id and client secret? Why not just authenticate with the username and password?
-
Ah, I see - thanks for this it makes it very clear! I know its good practice to use this already existent header but is there any reason why I couldn't create my own headers and use these, e.g. access_token and refresh_token? On a slightly different note: Looking at the Oauth that seems to have a client id and a client secret passed in the http authorization header and then the access/refresh token passed in to the post body - what is the advantage of having a client id and secret as well as the access tokens? Is it the case that this controls which 'application' can use the endpoint?
-
I am wanting to pass over the access token in an authentication header for an API I am creating (learning) and I have read that the authorization header should have a value of 'Bearer aTokenStringHere'. What is the best way of getting this header value and parsing it, is it just the case of getting the Authorization header form the request and then stripping out the Bearer part of the string? and leaving the token value and then using that? Also can you explain why we must use Bearer as part of the value for Authorization header? Thanks
-
Sorry didn't make myself fully clear, i used the example of using pdo and prepared statements prevents sql injection. However i did fail to spot that EMULATE_PREPARES was not switched off so good spot.
-
Using PDO and prepared statements negates the use of mysqli_real_escape_string etc as it will automatically escape any characters that would cause issues.
-
See - https://forums.phpfreaks.com/topic/302964-pdo-safe-inserting-data-into-database/
-
Looks fine to me, using prepared statements does the escaping for you so you don't need to run the input through any functions. There are a couple of ways to secure your form a little better, which validates the inputted data, e.g. function to make sure that the email address is formed correctly and that the password is complex (contains upper and lower case as well as numbers etc). The other thing you could look at is the introduction of form tokens which tell your PHP based on a session that the form was submitted from your website rather than someone just copying your html code and creating a spoofed register form
-
Thats what I was thinking, just want to make sure I do it correctly to save any problems in the future, I did look into the HTMLPurifier and it does look like a good idea. because I am using PDO and prepared statements then I should be ok with any sql injections. The one issue I seen was that single quotes seem to be converted to a slash and a number when json_encode is used? Is this normal as the html then isn't formed correctly in the json output? The news articles contain markup from the WYSIWYG editor, e.g. headings, paragraph, styes etc. the metadata of the news article is stored in different fields in the database but the content itself it stored in html due to the formatting from the WYSIWYG editor. Never really done much with markdown, but it looks like a good way to go so I will look into this - any chance you could give me the basics of how markdown works? I guess we store the markdown in the database but how is it converted back to html when retrieved?
-
Hi Just wanting to know how exactly I should be doing the following: I am looking to store HTML in a MySQL database, this will be added via a WYSIWYG editor on a webpage and posted via php to a MySQL database. This HTML is used to display news articles on a website, so what is the correct way of storing and returning this? I don't want to remove HTML so I guess it's a straight insert via pdo bind parameters and then just select and display on the webpage when returning? Or should I be using any functions to encode/ make safe the HTML code. Also as part of this I also need to return the news articles for a mobile app in a JSON api, I will use json_encode function on the returned data but should I be running any make safe functions on that too? Thanks
-
Yeah true, i suppose it would be no different than someone just randomly creating a token value and submitting that - the chances of getting it correct is very slim!
-
I see, so one last question (I hope). Do you know if OAUTH 2 prevents use of already issued tokens, e.g. user1 had token 'aaabbb' 2 years ago and that expired but user2 now has that same token 'aaabbb' (really unlikely) but that token got compromised 2 years ago and someone just happens to test this token at exactly same time as user2 now having this valid token. I think what I am getting at; does OAUTH 2 do a lookup of tokens already issued from the past before issuing a new one to prevent the same token value being issued?
-
I see that I just extend the appropriate classes - which allows me to override the current behaviour with my own - thanks both for the above comments, this has really helped.
-
So I have been playing around with the framework from: bshaffer. and have a few questions if you could help? Reading the documentation I have managed to create a test auth system but is it advisable to not edit the frameworks source files? e.g. do I need to use the user table that it looks for by default or should I edit the code to look at my user table? my auth page looks like and it takes post data of user name and password (as well as client name and client secret) and provides an access code which I can then use the api page (below the auth page) to validate the access code and provide the content, I can also submit a request to this page with the refresh token to then generate the new access token which I can store in my app for future requests: <?php // error reporting (this is a demo, after all!) ini_set('display_errors',1);error_reporting(E_ALL); // Autoloading (composer is preferred, but for this example let's just do this) require_once('src/OAuth2/Autoloader.php'); OAuth2_Autoloader::register(); $dsn = "mysql:dbname=xxxxxxx;host=localhost"; $username = "xxxxxxx"; $password = "xxxxxxx"; // $dsn is the Data Source Name for your database, for exmaple "mysql:dbname=my_oauth2_db;host=localhost" $storage = new OAuth2_Storage_Pdo(array('dsn' => $dsn, 'username' => $username, 'password' => $password)); // Pass a storage object or array of storage objects to the OAuth2 server class $server = new OAuth2_Server($storage); // Add the "Client Credentials" grant type (it is the simplest of the grant types) $server->addGrantType(new OAuth2_GrantType_UserCredentials($storage)); $server->addGrantType(new OAuth2_GrantType_RefreshToken($storage)); // Handle a request for an OAuth2.0 Access Token and send the response to the client $server->handleTokenRequest(OAuth2_Request::createFromGlobals(), new OAuth2_Response())->send(); ?> api page - post with passing in access token as well as client id and client secret: <?php // error reporting again ini_set('display_errors',1);error_reporting(E_ALL); // Autoloading again require_once('src/OAuth2/Autoloader.php'); OAuth2_Autoloader::register(); // create your storage again $dsn = "mysql:dbname=xxxxxxx;host=localhost"; $username = "xxxxxxx"; $password = "xxxxxxxxx"; $storage = new OAuth2_Storage_Pdo(array('dsn' => $dsn, 'username' => $username, 'password' => $password)); // create your server again $server = new OAuth2_Server($storage); // Handle a request for an OAuth2.0 Access Token and send the response to the client if (!$server->verifyResourceRequest(OAuth2_Request::createFromGlobals(), new OAuth2_Response())) { $server->getResponse()->send(); die; } echo json_encode(array('success' => true, 'message' => 'You accessed my APIs!')); ?> So the basis of OAUTH 2 it looks like what I was trying to do but I am only wanting to use a subset of its functionality so do I need to remove any files from the framework or is it safe to just leave them all and only implement the functionality I need to? If I want the application to permanently stay logged in do I change the expiry date of the refresh token to like 6 months as the refresh token expiry seems to only come into play if there hasn't been any new request to refresh the access token (so if a user is actively using the app then it will generate a new refresh token frequently anyway - each time the access token expires) - so if the user hasn't used the app in 6 months then prompt them to login again? This seems reasonable to me. It actually wasn't as difficult to implement compared to what I was reading on the internet (assuming the above code is ok - as its based on the documentation)
-
I do agree, however there are many frameworks that can be added to the project and these add complexity if things go wrong - for example if i switch to a newer version of PHP and that framework is not compatible and the its not updated for several months, Is there a single source for the framework for PHP as the places I have looked (OAUTH site) seemed to have been created by someone and not an official framework? In essence I want the most supposed framework there is for this - any pointers where to look.
-
Thanks for that. The example I am going from is my Gas and Electric phone application - without knowing how it works exactly it seems that I login once and haven't had to log in again (been over 6 months since first logged in). Also I believe for example Facebook app works the same way where you login once and it doesn't ask you again? So just wondering how this would be implemented - I can see how using the refresh tokens like you have explained but I have also read that refresh tokens should expire at some point too? Also what should an access token be made up from - could I just generate a totally random string or should it do some hashing based on a user id and time generated for example and then use the server to decode this to see if it matches the data or should it just check to see if the access token matches the same string in the access_token column in the database. Sorry if these questions are basic but just trying to get my head around the concept of a properly scalable authentication system for an API
-
Hi I am trying to get my head around how to properly implement token authentication for my JSON api. It seems like the concept is: use username and password on a /login POST api over SSL to then compare with the values in the DB for username and hashed password. If it authenticates ok then generate a random access token (stored in the DB against the user and have an expiry date/time associated with it) and return this access token to the user Then on every client request send the access token to the server and check this value with that is stored and validate against the expiry If this matches and is still valid then process the API request and return the data requested If it doesn't match then generate a 401 error and return this to the client and handle re-logging in. So I get how that part of it works but I see apps etc that use APIs that stay logged in for ever basically. So do you think they hold onto the username and password in the client app for when the server access token expires and it then resends the username and password to get a new access token? I have read that the client application should never remember the username and password and you should use a concept of 'refresh tokens'. How do these work? Also refresh tokens should also expire (after a longer period of time than the access token) so how does this concept work? Thanks
-
Hi I have a question about API keys and security. I am building a mobile app (learning) and will be using a PHP/MySQL JSON Rest API (designed myself) and I am new to APIs in general so some best practices would be appreciated if you have any? My real question is to do with securing these APIs. For example I was thinking of using user name and password that the user logs into the application with to be send over HTTPS for each request to validate the user is authorised and authenticated. However I have read that I should also be using API keys, so how would I integrate this in? Would each user have their own unique API key or would each system that uses this API have a unique key? If its each user that has their own key would I send all three pieces of data with the request (API Key, username and password). Any advice would be great. Thanks
-
Hi Looking to implement this ER Diagram but not entirely sure how I would implement the relationship between the Parking and the AuthorisedCars entity. Basically the Parking entity will record the parking event in that it will record the reg number, the time and date of the event as well as if the car left or entered the car park. The Reg number along with the Time and date are down as the primary key. In the AuthorisedCar entity has the Reg number along with the make, model and colour of the car and this will be used to record all the authorised cars that are allowed to park. The relationship shows that a Parking event may or may not be for an Authorised Car, and that an Authorised Car may or may not have Many Car Parking Events. How would I represent this relationship in a Relational Model for the database implementation. I thought of a foreign key for Reg Number in the Parking entity as a car that is NOT on the authorised list may enter / exit the car park so a foreign key is not acceptable as there will not be a matching entry in the AuthorisedCar entity. How would you implement this? Thanks
-
Thanks, I see what you mean, I guess you probably do read more than you write when thinking about it. Next question is how do you set up the environment where all reads are sent to the slaves automatically or do you have to programatically have to tell it to connect to the slaves when running a select and then swap connections to the master when doing an insert / update?
-
I see that you can do Master to Master replication and then adjust the increment offset to stop PK collisions. I have also read that an idea is to have multiple web servers connected to their own MySQL db server so that said web server node will have its own dedicated db server which has a master to master replication so any updates will populate to the others. Not sure if this is a good idea or not, just seems that most sites I have looked at recommend Load balancing the web servers and then they all point to ONE db server which seems a little daft as you may have a bottle neck if the DB server is overloaded..... I can see how Master - Slave replication can work when most times when people are reading then it can always provide additional nodes for reading from (slaves) but not much information about a busy website that needs to perform lots of writes.......
-
That is more for Java connections rather than PHP. Will have a look through the manual for PHP and see if it offers the same but if anyone has any suggestions then please post here
-
Hi Just messing about with MySQL and would like to try and set up (for educational purposes) a MySQL Load Balancing environment. Just like you do with Apache where you round robin requests between two or more servers for the web app, I want to also set up this for the database setup. I have looked on the internet and havent found much help or answers but surely this is possible. I am guessing from setting up the apache load balancer that you will need shared storage (for the database files / config files) and then also two nodes each being a DB server which is connected to the shared storage. If this is not possible then how do people set up environments that need many servers due to load? Thanks
-
Nice! Thank you!
-
I have the following string (the parts between the double quotes is dynamic so not the same length or content each time) and I need to pick out who is logged on only, don't need any of the other content. "explorer.exe","2012","Console","1","30,832 K","Running","MYDOMAIN\Administrator","0:00:02","N/A" Now, I need to get the MYDOMAIN\Administrator part only (not including the quotes) and keep this in a variable. Could someone help me out with this, i'm sure it is fairly easy for one of you regex experts to do. Thank you.