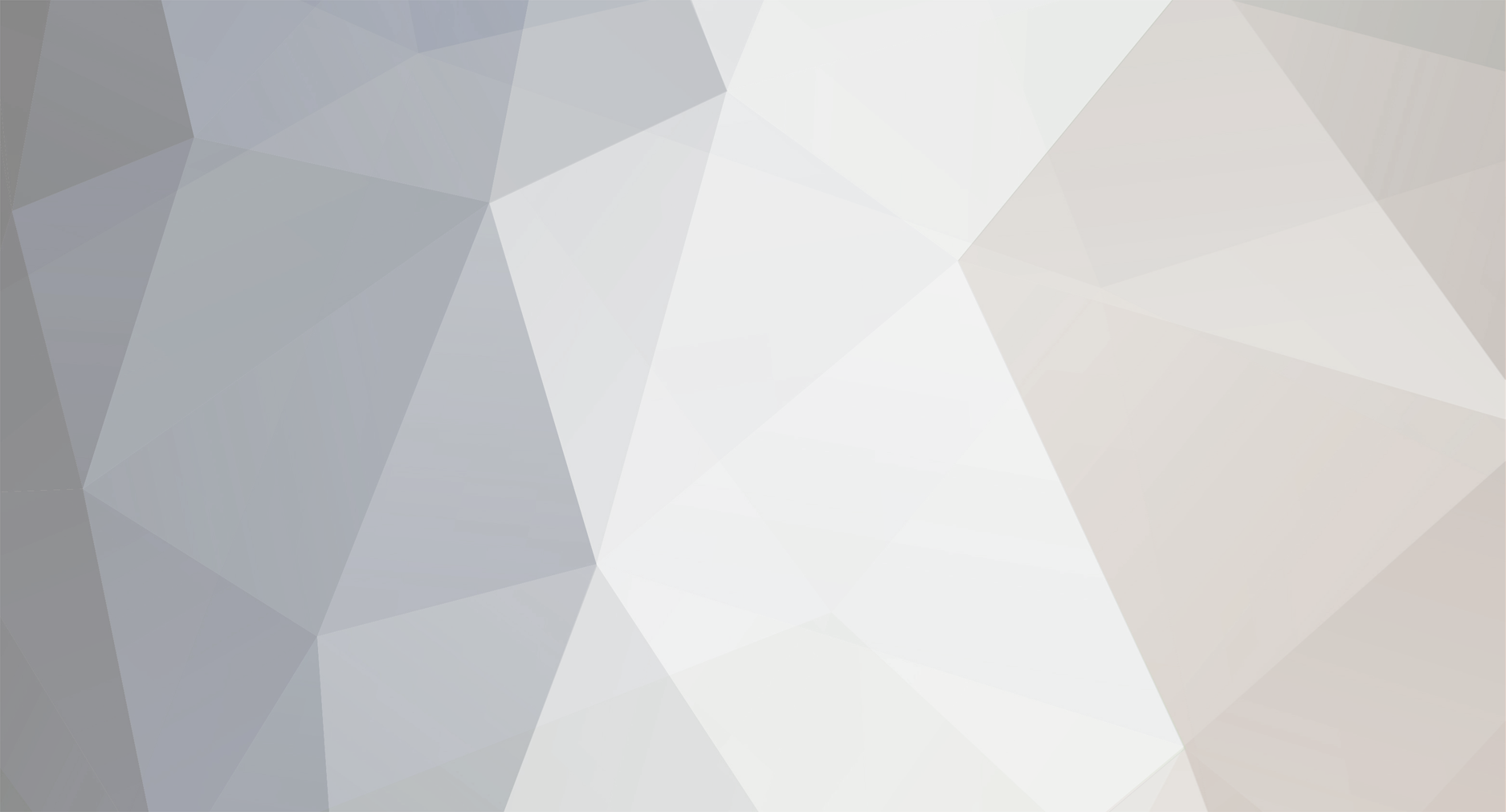
ChemicalBliss
Members-
Posts
719 -
Joined
-
Last visited
Never
Everything posted by ChemicalBliss
-
You need a recursive loop/function. <?php function recur_in_array($array,$string){ for($i=0;$i<count($array);$i++){ if(is_array($array[$i])){ $found = recur_in_array($array[$i],$string); if($found === true){ return $found; } }else{ if (in_array($string, $product_rows[0])) { return true; } } } } if(recur_in_array($array_var) === true){ echo("xxx found"); } ?> -CB-
-
Debug your code; 1. add "or die()" function: mysql_query("...") or die(mysql_error()); 2. Echo Specific Variables to check their values. // Before the loop echo($num); // Inside the loop echo($i); 3. Try the query using phpmyadmin. You may find that there is a typo (Case-sensitive, o doesnt equal O). -CB-
-
You want to login/logout and create accounts? So you need to make these 3 functions. For your level i would suggest seperate files: login.php <?php [b]// Check if logging in:[/b] if(isset($_POST['login_name'])){ // login // Grab File (accounts.txt) // Explode Data (Get each individual User/Pass) // Check if the user/pass exists. // If it does, then set a cookie or use sessions to store the data. // redirect to some other page } // otherwise. // Display login form. ?> register.php <?php // Check if registering if(isset($_POST['register_name'])){ // Santize Variables (Make sure it is safe to use them/Display them) // Check if the email or username already exists in the accounts.txt file. // If it doesn't then add the account to the list and redirect to login.php } // otherwise // Display the registration form. ?> logout.php <?php // Delete cookies or sessions, redirect to another page, or login.php ?> That is the basic logic of multiple user logins. To change your code, you will have to check your logic on your page, as just adding the necessary parts could change your script logic in a way that would be easier to rewrite the whole thing. You may be able to get away with just adding a "register_user" function, and changing your login function to accomadate multiple users. IMHO, i would rewrite the whole thing (would be much quicker and easier). -CB-
-
function / page reloaded after header redirect
ChemicalBliss replied to juriaan79's topic in PHP Coding Help
Sounds as if the page your redirecting also has the same error or something. Echo the Redirect string, make sure it points to the right file. Make sure that file is not causing the problem, empty it (backup first), so u get a blank page on redirect, if u get 1 email then it's the file. -CB- -
How do you mean it didnt work? What was it supposed to do? If you want a newline, in html use '<br />', in plain text use '\n'. Check over the HTML source output (right-click in IE/Mozilla and click 'View Source'). Make sure it's what you expect. -CB-
-
How to make changing account? If you want the accounts to be stored in a mysql database, you need to setup a database portion. Check out some MySQL-PHP tutorials on google for a quick overview. If you have any problems following the tutorials or coding your own part then let us know . Thing is you need to know about MySQL if you want to use it, and there are plenty of tutorials out there that will save both of us time . -CB-
-
Using $_POST with UPDATE query?.. think it's easy.
ChemicalBliss replied to oni-kun's topic in PHP Coding Help
you have one too many commas If you ever get MySQL Syntax errors, Echo/Print the query and make sure it is what you expect . -CB- -
Maybe if you explain what your data is, and exactly your planning to do with the data, we can provide the logic you need . Then you can code it . -CB-
-
Yay at least there is results . Ok if you use 'AND' instead of 'OR', you will only match results that contain _BOTH_ search phrases. The OR lets it match any result that has Either of those phrases. What you can do, is supply a checkbox next to the search, where it will ask "Match all phrases", if checked, it will switch the OR's to AND's, otherwise it will leave the query as is. There are methods that can sort results into a more accurate result set. But this is slightly beyond me for now as i havn't ventured down that route yet . Good Luck though . (This thread i guess has been solved as your original question is answered, it's also quite big, i'd suggest starting a new thread about search optimization etc if you want that). -CB-
-
lol, correct the spelling, see what it does . (Correct the spelling in the array above). -CB-
-
lol i'm not getting it right at all tonight . forgot the bracket on the end (end of die() command). $numresults=mysql_query($query) or die("ERROR - ".mysql_error()."<br />Query: ".$query; should be $numresults=mysql_query($query) or die("ERROR - ".mysql_error()."<br />Query: ".$query); EDIT: Oh and add echo($query); just above that line too. -CB-
-
strange, its not matching any records. make sure the query is working. Change this: $numresults=mysql_query($query); to this: $numresults=mysql_query($query) or die("ERROR - ".mysql_error()."<br />Query: ".$query; Tell us what it says .
-
loop_max will be the number of emails you want to send that night, you can use a mysql query to count the amount of users if u want to send an email to everyone., but loop_max lets you put a maximum amount of emails to be sent (so u dont get IP banned or suspended or flooding servers or overloading your host.)
-
In Fact that code is wrong lol, was wrong one - this is the correct one: <?php // Get the search variable from URL $var = @$_GET['q'] ; $trimmed = trim($var); //trim whitespace from the stored variable // rows to return $limit=10; // check for an empty string and display a message. if ($trimmed == "") { echo "<p>Please enter a search...</p>"; exit; } // check for a search parameter if (!isset($var)) { echo "<p>We dont seem to have a search parameter!</p>"; exit; } //connect to your database ** EDIT REQUIRED HERE ** mysql_connect("host","username","password"); //specify database ** EDIT REQUIRED HERE ** mysql_select_db("database_name") or die("Unable to select database"); // Build SQL Query $search_words = explode(' ', $trimmed); // MODIFIED BELOW $search_fields_array = array( "Organisation", "Locatation", "Prices", "Toddlers", "Indoor_Outdoor" ); $sfc_count = count($search_fields_array); // Loop each table union. For($i=0;$i<$sfc_count;$i++){ // Turn that item into an array (easier this way) if(!is_array($search_fields_array[$i])){ $this_where = $search_fields_array[$i]; $search_fields_array[$this_where] = array(); } // Loop each search word (for each table union) Foreach($search_words As $Word){ // Append an array item containing the where statement $search_fields_array[$this_where][] = "`".$this_where."` LIKE '%".mysql_real_escape_string($Word)."%'"; } } $query = "select * from `table` where ".implode(" OR ",$search_fields_array['Organisation']) ." UNION select * from `table` where ".implode(" OR ",$search_fields_array['Locatation']) ." UNION select * from `table` where ".implode(" OR ",$search_fields_array['Prices']) ." UNION select * from `table` where ".implode(" OR ",$search_fields_array['Toddlers']) ." UNION select * from `table` where ".implode(" OR ",$search_fields_array['Indoor_Outdoor']) ." order by `Organisation`"; // MODIFIED ABOVE $numresults=mysql_query($query); $numrows=mysql_num_rows($numresults); // If we have no results, offer a google search as an alternative if ($numrows == 0) { echo "<h4>Results</h4>"; echo "<p>Sorry, your search: "" . $trimmed . "" returned zero results</p>"; // google echo "<p><a href=\"http://www.google.com/search?q=" . $trimmed . "\" target=\"_blank\" title=\"Look up " . $trimmed . " on Google\">Click here</a> to try the search on google</p>"; } // next determine if s has been passed to script, if not use 0 if (empty($s)) { $s=0; } // get results $query .= " limit $s,$limit"; $result = mysql_query($query) or die("Couldn't execute query"); // display what the person searched for echo "<p>You searched for: "" . $var . ""</p>"; // now you can display the results returned // begin to show results set echo "Results <br /><br />"; $count = 1 + $s ; // now you can display the results returned while ($row= mysql_fetch_array($result)) { $title = $row["Result"]; $col = $row["Organisation"]; $col1 = $row["Location"]; $col2 = $row["Indoor_Outdoor"]; $col3 = $row["Prices"]; $col4 = $row["Toddlers"]; $col5 = $row["Primary"]; $col6 = $row["Junior"]; $col7 = $row["High_School"]; $col8 = $row["All_Family"]; $col9 = $row["Telephone"]; $col10 = $row["Website_Address"]; echo '<div class=\"results\">'; echo "<div class=\"title\"> $col</div>"; echo "<div class=\"subheadings\">Indoors or Outdoors? $col2</div>"; echo "<div class=\"subheadings\">Price Range in £'s: $col3</div>"; echo "<div style=\"clear:both\"></div>"; echo "<div class=\"suitable\">Suitable for toddlers? $col4<br /> Suitable for primary schools? $col5 <br />Suitable for junior schools? $col6 <br />Suitable for high schools?$col7<br /> Suitable for all the family? $col8</div>"; echo "<div class=\"lo\">Location: $col1</div>"; echo "<div style=\"clear:both\"></div>"; echo "<div class=\"lo\">Telephone Number: $col9</div>"; echo "<div style=\"clear:both\"></div>"; echo "<div class=\"website\">Website Addess: <a href=\"$col10\">$col10</a></div>"; echo "<br /><br />"; echo "</div>\n"; $count++ ; } $currPage = (($s/$limit) + 1); //break before paging echo "<br />"; // next we need to do the links to other results if ($s>=1) { // bypass PREV link if s is 0 $prevs=($s-$limit); print " <a href=\"$PHP_SELF?s=$prevs&q=$var\"><< Prev 10</a>  "; } // calculate number of pages needing links $pages=intval($numrows/$limit); // $pages now contains int of pages needed unless there is a remainder from division if ($numrows%$limit) { // has remainder so add one page $pages++; } // check to see if last page if (!((($s+$limit)/$limit)==$pages) && $pages!=1) { // not last page so give NEXT link $news=$s+$limit; echo " <a href=\"$PHP_SELF?s=$news&q=$var\">Next 10 >></a>"; } $a = $s + ($limit) ; if ($a > $numrows) { $a = $numrows ; } $b = $s + 1 ; echo "<p>Showing results $b to $a of $numrows</p>"; ?> Sorry for the confusion. -CB-
-
oops, sorry, forgot to concatenate that line. put a . (period) before the quoted string . -CB-
-
How are you executing the script? Cron jobs? If the script is executed at exactly midnight, and ytou only want to send a specific amount of emails in that one execution. Thyen logic like this should suffice: Loop (while $loop_count is less than $loop_max) Get Next Row From Mysql (that "Sent" = false) Send Email On Successfull Send, Set Row Field "Sent" = true end Loop
-
Limit number of users signup, count db entries
ChemicalBliss replied to blesseld's topic in PHP Coding Help
Just use a mysql count query. http://dev.mysql.com/doc/refman/5.1/en/counting-rows.html eg: $query = "SELECT COUNT(*) FROM `adccmemebers`"; $number_of_users = mysql_result(mysql_query($query),0); -CB- -
Change the way this page displays results:
ChemicalBliss replied to roldahayes's topic in PHP Coding Help
It's because the code is generated off-site, you would have to write your own javascript code, even then, not sure if you could use custom map.js with the google API's anyway. Well, that's the extent of my knowledge in this area, your moving onto javascript now . Good Luck though! -CB- -
This code works for a single search term, correct? <?php // Get the search variable from URL $var = @$_GET['q'] ; $trimmed = trim($var); //trim whitespace from the stored variable // rows to return $limit=10; // check for an empty string and display a message. if ($trimmed == "") { echo "<p>Please enter a search...</p>"; exit; } // check for a search parameter if (!isset($var)) { echo "<p>We dont seem to have a search parameter!</p>"; exit; } //connect to your database ** EDIT REQUIRED HERE ** mysql_connect("host","username","password"); //specify database ** EDIT REQUIRED HERE ** mysql_select_db("database_name") or die("Unable to select database"); // Build SQL Query $search_words = (explode(' ', $trimmed)); foreach ($search_words as $word) { $query = "select * from table where Organisation like '%$word%' UNION select * from table where Location like '%$word%' UNION select * from table where Prices like '%$word%' UNION select * from table where Toddlers like '%$word%' UNION select * from table where Indoor_Outdoor like '%$word%' order by Organisation"; } $numresults=mysql_query($query); $numrows=mysql_num_rows($numresults); // If we have no results, offer a google search as an alternative if ($numrows == 0) { echo "<h4>Results</h4>"; echo "<p>Sorry, your search: "" . $trimmed . "" returned zero results</p>"; // google echo "<p><a href=\"http://www.google.com/search?q=" . $trimmed . "\" target=\"_blank\" title=\"Look up " . $trimmed . " on Google\">Click here</a> to try the search on google</p>"; } // next determine if s has been passed to script, if not use 0 if (empty($s)) { $s=0; } // get results $query .= " limit $s,$limit"; $result = mysql_query($query) or die("Couldn't execute query"); // display what the person searched for echo "<p>You searched for: "" . $var . ""</p>"; // now you can display the results returned // begin to show results set echo "Results <br /><br />"; $count = 1 + $s ; // now you can display the results returned while ($row= mysql_fetch_array($result)) { $title = $row["Result"]; $col = $row["Organisation"]; $col1 = $row["Location"]; $col2 = $row["Indoor_Outdoor"]; $col3 = $row["Prices"]; $col4 = $row["Toddlers"]; $col5 = $row["Primary"]; $col6 = $row["Junior"]; $col7 = $row["High_School"]; $col8 = $row["All_Family"]; $col9 = $row["Telephone"]; $col10 = $row["Website_Address"]; echo '<div class=\"results\">'; echo "<div class=\"title\"> $col</div>"; echo "<div class=\"subheadings\">Indoors or Outdoors? $col2</div>"; echo "<div class=\"subheadings\">Price Range in £'s: $col3</div>"; echo "<div style=\"clear:both\"></div>"; echo "<div class=\"suitable\">Suitable for toddlers? $col4<br /> Suitable for primary schools? $col5 <br />Suitable for junior schools? $col6 <br />Suitable for high schools?$col7<br /> Suitable for all the family? $col8</div>"; echo "<div class=\"lo\">Location: $col1</div>"; echo "<div style=\"clear:both\"></div>"; echo "<div class=\"lo\">Telephone Number: $col9</div>"; echo "<div style=\"clear:both\"></div>"; echo "<div class=\"website\">Website Addess: <a href=\"$col10\">$col10</a></div>"; echo "<br /><br />"; echo "</div>\n"; $count++ ; } $currPage = (($s/$limit) + 1); //break before paging echo "<br />"; // next we need to do the links to other results if ($s>=1) { // bypass PREV link if s is 0 $prevs=($s-$limit); print " <a href=\"$PHP_SELF?s=$prevs&q=$var\"><< Prev 10</a>  "; } // calculate number of pages needing links $pages=intval($numrows/$limit); // $pages now contains int of pages needed unless there is a remainder from division if ($numrows%$limit) { // has remainder so add one page $pages++; } // check to see if last page if (!((($s+$limit)/$limit)==$pages) && $pages!=1) { // not last page so give NEXT link $news=$s+$limit; echo " <a href=\"$PHP_SELF?s=$news&q=$var\">Next 10 >></a>"; } $a = $s + ($limit) ; if ($a > $numrows) { $a = $numrows ; } $b = $s + 1 ; echo "<p>Showing results $b to $a of $numrows</p>"; ?> If so, this code should work for multiple search terms. <?php // Get the search variable from URL $var = @$_GET['q'] ; $trimmed = trim($var); //trim whitespace from the stored variable // rows to return $limit=10; // check for an empty string and display a message. if ($trimmed == "") { echo "<p>Please enter a search...</p>"; exit; } // check for a search parameter if (!isset($var)) { echo "<p>We dont seem to have a search parameter!</p>"; exit; } //connect to your database ** EDIT REQUIRED HERE ** mysql_connect("host","username","password"); //specify database ** EDIT REQUIRED HERE ** mysql_select_db("database_name") or die("Unable to select database"); // Build SQL Query $search_words = explode(' ', $trimmed); // MODIFIED BELOW $search_fields_array = array( "Organisation", "Locatation", "Prices", "Toddlers", "Indoor_Outdoor" ); // Loop each table union. For($i=0;$i<count($search_fields_array);$i++){ // Turn that item into an array (easier this way) if(!is_array($search_fields_array[$i])){ $this_where = $search_fields_array[$i]; $search_fields_array[$this_where] = array(); } // Loop each search word (for each table union) Foreach($search_words As $Word){ // Append an array item containing the where statement $search_fields_array[$this_where][] = "`".$this_where."` LIKE '%".mysql_real_escape_string($Word)."%'"; } } $query = "select * from `table` where ".implode(" OR ",$search_fields_array[0]) ."UNION select * from `table` where ".implode(" OR ",$search_fields_array[1]) ."UNION select * from `table` where ".implode(" OR ",$search_fields_array[2]) ."UNION select * from `table` where ".implode(" OR ",$search_fields_array[3]) ."UNION select * from `table` where ".implode(" OR ",$search_fields_array[4]) "order by `Organisation`"; // MODIFIED ABOVE $numresults=mysql_query($query); $numrows=mysql_num_rows($numresults); // If we have no results, offer a google search as an alternative if ($numrows == 0) { echo "<h4>Results</h4>"; echo "<p>Sorry, your search: "" . $trimmed . "" returned zero results</p>"; // google echo "<p><a href=\"http://www.google.com/search?q=" . $trimmed . "\" target=\"_blank\" title=\"Look up " . $trimmed . " on Google\">Click here</a> to try the search on google</p>"; } // next determine if s has been passed to script, if not use 0 if (empty($s)) { $s=0; } // get results $query .= " limit $s,$limit"; $result = mysql_query($query) or die("Couldn't execute query"); // display what the person searched for echo "<p>You searched for: "" . $var . ""</p>"; // now you can display the results returned // begin to show results set echo "Results <br /><br />"; $count = 1 + $s ; // now you can display the results returned while ($row= mysql_fetch_array($result)) { $title = $row["Result"]; $col = $row["Organisation"]; $col1 = $row["Location"]; $col2 = $row["Indoor_Outdoor"]; $col3 = $row["Prices"]; $col4 = $row["Toddlers"]; $col5 = $row["Primary"]; $col6 = $row["Junior"]; $col7 = $row["High_School"]; $col8 = $row["All_Family"]; $col9 = $row["Telephone"]; $col10 = $row["Website_Address"]; echo '<div class=\"results\">'; echo "<div class=\"title\"> $col</div>"; echo "<div class=\"subheadings\">Indoors or Outdoors? $col2</div>"; echo "<div class=\"subheadings\">Price Range in £'s: $col3</div>"; echo "<div style=\"clear:both\"></div>"; echo "<div class=\"suitable\">Suitable for toddlers? $col4<br /> Suitable for primary schools? $col5 <br />Suitable for junior schools? $col6 <br />Suitable for high schools?$col7<br /> Suitable for all the family? $col8</div>"; echo "<div class=\"lo\">Location: $col1</div>"; echo "<div style=\"clear:both\"></div>"; echo "<div class=\"lo\">Telephone Number: $col9</div>"; echo "<div style=\"clear:both\"></div>"; echo "<div class=\"website\">Website Addess: <a href=\"$col10\">$col10</a></div>"; echo "<br /><br />"; echo "</div>\n"; $count++ ; } $currPage = (($s/$limit) + 1); //break before paging echo "<br />"; // next we need to do the links to other results if ($s>=1) { // bypass PREV link if s is 0 $prevs=($s-$limit); print " <a href=\"$PHP_SELF?s=$prevs&q=$var\"><< Prev 10</a>  "; } // calculate number of pages needing links $pages=intval($numrows/$limit); // $pages now contains int of pages needed unless there is a remainder from division if ($numrows%$limit) { // has remainder so add one page $pages++; } // check to see if last page if (!((($s+$limit)/$limit)==$pages) && $pages!=1) { // not last page so give NEXT link $news=$s+$limit; echo " <a href=\"$PHP_SELF?s=$news&q=$var\">Next 10 >></a>"; } $a = $s + ($limit) ; if ($a > $numrows) { $a = $numrows ; } $b = $s + 1 ; echo "<p>Showing results $b to $a of $numrows</p>"; ?> Hope this helps, -CB-
-
If it's a contact form that any guest can use, then i would advise; 1. Spam Control (Time limit between sending messages, ip/cookie etc). 2. Email Verification (A little overkill with spam control), when user sends a message, he must confirm the message in his own email, sort of like a registration type thing. 3. Only allow certain number of messages/day, if the limit is reached then send an email to you, so you can check it out and reset the limit if everything is ok. 4. Of course you will need to only allow Plain Text in the message body/name/email and subject. 5. Anything going into mysql from those fields needs to be properly escaped (mysql_real_escape_string). -CB-
-
Change the way this page displays results:
ChemicalBliss replied to roldahayes's topic in PHP Coding Help
You can change the style, i doubt you can change it into table format, without some really crazy coding. -
You only need to update, if you are updating an existing row in the mysql table. If you are adding a new row (like a new person/log entry) then you can insert . So it's more like this: <?php // connect to to mysql // loop each id in the post array foreach($_POST['row_id_array'] As $thisid){ // Query, Uses the value of the ID fields to find the row (if there is one), in MySQL $query = "SELECT `id` FROM `mytable` WHERE `log_id`='".$thisid; $result = mysql_query($query) or die(mysql_error()); // Count number of rows that matched the query. $rows = mysql_num_rows($result); // What to do. if($rows == 0){ // Insert }else if($rows == 1){ // Update }else{ // More than one match. Umm - Shouldnt happen with unique id's } } ?> <!-- FORM --> <form action=".." method="post"> First Record <input type="hidden" name="row_id_array[0]" value="56" /> <input type="text" name="length[0]" value="" /> <input type="text" name="weight[0]" value="" /> Second Record <input type="hidden" name="row_id_array[1]" value="165" /> <input type="text" name="length[1]" value="" /> <input type="text" name="weight[1]" value="" /> ... </form> So, each row, will have a hidden "ID" field that tells the script the ID number of that row. It uses a POST array so that you can submit as many rows to update as you want. Then you just go through the loop (above), for each row_id_array, check if the row exists and act accordingly. (you can change the query only to match if the results are exactly the same, then update only those changed, but would require to recode the conditional statements). Hope it makes sense to you . -CB-
-
Forum - PHP Help - 0 replies Search
ChemicalBliss replied to ChemicalBliss's topic in PHPFreaks.com Website Feedback
Yeah, lol - i fell this has been thoroughly discussed, i can't think of anything new to bring to the matter, seems that we won't need it . Thanks for the response though guys (and gals) . -CB- -
In MySQL, Being able to insert (create a new row) with _blank_ values depends entirely on how you made the table (more specifically, what options and default values you gave the column you want to be blank). You can pass blank values, and you will have to use UPDATE, if you want to change a row. -CB-
-
The easiest and safest method by far is to use a HTML DOM Parser Class. (Some Free, Some Commercial). This page coud help you a little: http://www.onderstekop.nl/articles/114/ If the link get's removed then just google PHP HTML PARSER Good Luck -CB-