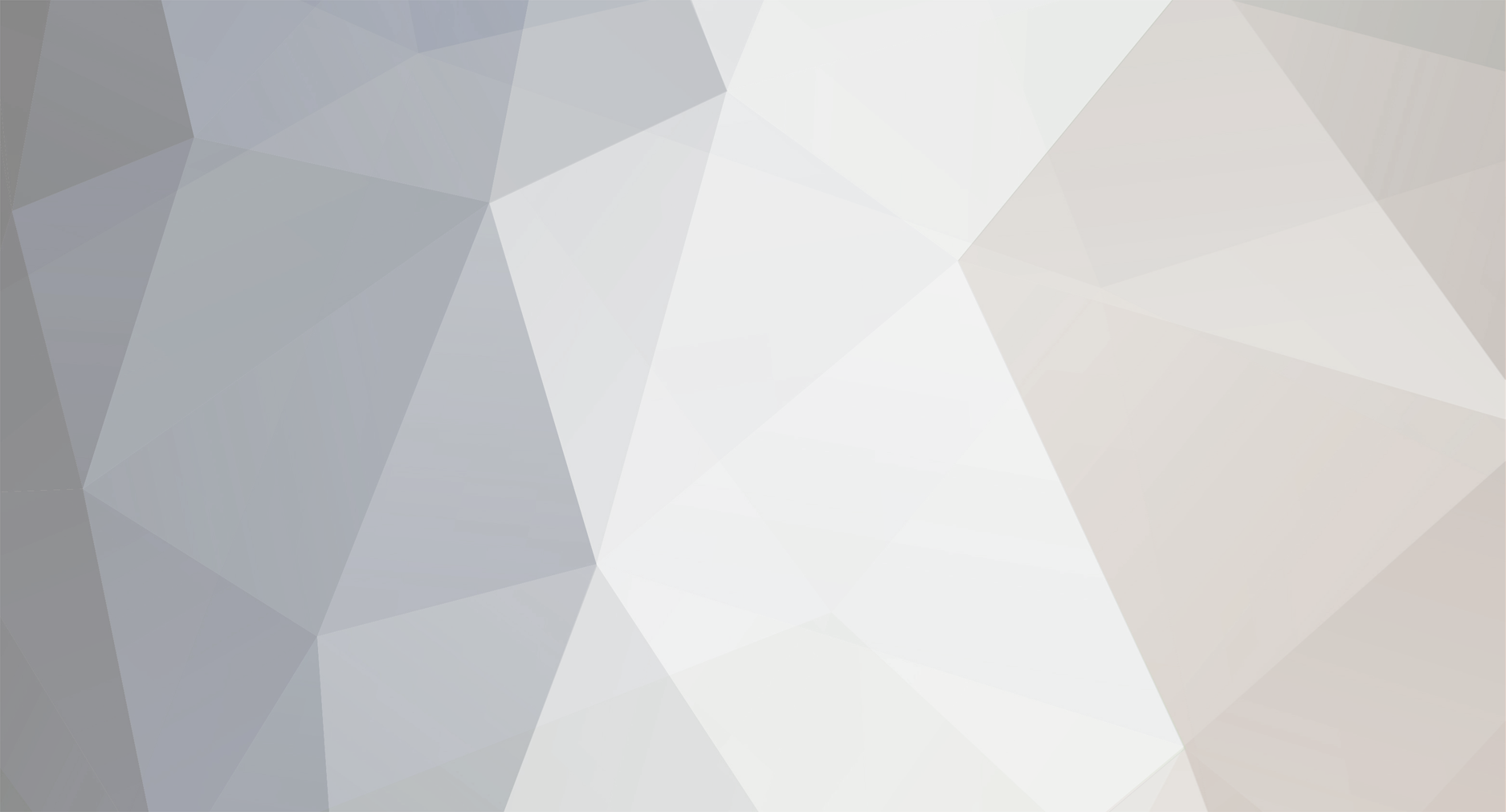
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
First, lets migrate you over to PDO. It is faster, and more secure than the old mysql functions. First Set up the connection: I like to store this in a script to include, so if I change the connection, I will only have to change the details in one place. config.php //Change these definitions as needed for your database connection. define('DSN','mysql:host=localhost;dbname=test'); //pdo dsn define('USER','root'); //pdo user define('PW',''); //pdo password //connect to the database: $pdo = new PDO(DSN,USER,PW,array(PDO::ATTR_DEFAULT_FETCH_MODE => PDO::FETCH_ASSOC)); //setting the default fetch mode to associative array.
-
The reason global variables are bad, is because they can change. What happens if you start coding a new script and call the PDO variable $pdo instead of $dbh? However there are much better ways of doing things. $var1 = 'blah'; $var2 = 'blah'; function phpfreaks($v1,$v2) { return $v1 . ' ' . $v2; } echo phpfreaks($var1,$var2); If you want to make sure the correct type of variable is passed to the function, you can use type hinting. $var1 = 'blah'; $var2 = 'blah'; function phpfreaks(PDO $v1, $v2) { return $v1 . ' ' . $v2; } echo phpfreaks($var1,$var2); //return: error, as $v1 is not an object of PDO You can also pass by reference, so that the function has control of the variable outside of the function scope. $var1 = 'blah'; $var2 = 'blah'; function phpfreaks(&$v1, &$v2) { $v1 = 'Not Blah'; $v2 = 'Not Blah'; return; } if(phpfreaks($var1,$var2)) { echo $var1 . ' ' . $var2; } //output: Not Blah Not Blah
-
Command Line curl and PHP curl Different results
jcbones replied to codeinphp's topic in PHP Coding Help
This is the result: Chromium**** <div class="row thead"> <input class="fullgrid_btn" type="button" value="View All Channels" /> Daily Programming on <span class="channel_name">BRAVO</span> for Sunday, May 31, 2015 thru Sunday, June 7, 2015 </div> <div class="tbody"> <div class="row"> <div class="col th"> 12:00 AM <br/> May 31, 2015 </div> <div class="prog_cols" > <div class="col ts prog_910899 movies" data-progid="910899" data-catid="movies" > <span class="prog_name">Enough</span> <div class="prog_time">May 31, 2015, 12:00 am - 2:00 am</div> <a class="btn_watchlist disabled" href="javascript:void(0)" data-progid="910899">(+) add to watchlist</a> <div class="prog_desc">A woman on the lam with her young daughter takes drastic steps when her abusive husband tracks them down.</div> </div> </div> <a class="watchnow" href="http://www.streamlive.to/premium">Watch Now</a> </div> <div class="row"> <div class="col th"> 2:00 AM <br/> May 31, 2015 </div> <div class="prog_cols" > <div class="col ts prog_910900 " data-progid="910900" data-catid="" > <span class="prog_name">The Real Housewives of Atlanta</span> <div class="prog_time">May 31, 2015, 2:00 am - 3:00 am</div> <a class="btn_watchlist disabled" href="javascript:void(0)" data-progid="910900">(+) add to watchlist</a> <div class="prog_desc">Kandi Burruss and Todd Tucker plan a family ski trip to Colorado. </div> </div> </div> <a class="watchnow" href="http://www.streamlive.to/premium">Watch Now</a> </div> <div class="row"> <div class="col th"> 3:00 AM <br/> May 31, 2015 </div> <div class="prog_cols" > <div class="col ts prog_910901 " data-progid="910901" data-catid="" > <span class="prog_name">Blood, Sweat & Heels</span> <div class="prog_time">May 31, 2015, 3:00 am - 4:00 am</div> <a class="btn_watchlist disabled" href="javascript:void(0)" data-progid="910901">(+) add to watchlist</a> <div class="prog_desc">Chantelle has a fast-paced blind date with a sightly bachelor while Mica has an intimate date at her apartment. In other developments, Demetria's cake-tasting gathering takes a sour turn; the crew heads to the Hamptons; and a painful moment tests Daisy.</div> </div> </div> <a class="watchnow" href="http://www.streamlive.to/premium">Watch Now</a> </div> <div class="row"> <div class="col th"> 4:00 AM <br/> May 31, 2015 </div> <div class="prog_cols" > <div class="col ts prog_910902 " data-progid="910902" data-catid="" > <span class="prog_name">Paid Programming</span> <div class="prog_time">May 31, 2015, 4:00 am - 6:00 am</div> <a class="btn_watchlist disabled" href="javascript:void(0)" data-progid="910902">(+) add to watchlist</a> <div class="prog_desc">No details are there for this program.</div> </div> </div> <a class="watchnow" href="http://www.streamlive.to/premium">Watch Now</a> </div> <div class="row"> <div class="col th"> 6:00 AM <br/> May 31, 2015 </div> <div class="prog_cols" > <div class="col ts prog_910903 " data-progid="910903" data-catid="" > <span class="prog_name">Million Dollar Listing New York</span> <div class="prog_time">May 31, 2015, 6:00 am - 8:00 am</div> <a class="btn_watchlist disabled" href="javascript:void(0)" data-progid="910903">(+) add to watchlist</a> <div class="prog_desc">Fredrik's reputation is tested when developers threaten to pull out of premium finishes they promised. Elsewhere, Ryan reunites with a familiar blonde, and Luis pursues one of the city's top developers.</div> </div> </div> <a class="watchnow" href="http://www.streamlive.to/premium">Watch Now</a> </div> <div class="row"> ***Truncated for posting*** -
Command Line curl and PHP curl Different results
jcbones replied to codeinphp's topic in PHP Coding Help
Yes. This is the script I used: <?php $headers = array(); $headers[]= 'Host: www.streamlive.to'; $headers[]= 'Connection: keep-alive'; //$headers[]= 'Content-Length: 27'; $headers[]= 'Pragma: no-cache'; $headers[]= 'Cache-Control: no-cache'; $headers[]= 'Accept: */*'; $headers[]= 'Origin: http://www.streamlive.to'; $headers[]= 'X-Requested-With: XMLHttpRequest'; $headers[]= 'User-Agent: Mozilla/5.0 (Windows NT 6.1) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/43.0.2357.81 Safari/537.36'; $headers[]= 'Content-Type: application/x-www-form-urlencoded; charset=UTF-8'; $headers[]= 'Referer: http://www.streamlive.to/tv-guide'; $headers[]= 'Accept-Encoding: gzip, deflate, sdch'; $headers[]= 'Accept-Language: en-US,en;q=0.8'; $headers[]= 'Cookie: PHPSESSID=f7mqqpgm3beto9a462fn8a2pl3; _gat=1; _ga=GA1.2.1850053691.1432824439'; $ch = curl_init('http://www.streamlive.to/tv-ajax.php'); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_COOKIESESSION, true); curl_setopt($ch, CURLOPT_FRESH_CONNECT, true); //curl_setopt($ch, CURLOPT_HEADER,true); curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); curl_setopt($ch, CURLOPT_VERBOSE, true);//0-FALSE 1 TRUE curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, 'action=getchannelsched&id=14'); $html = curl_exec($ch); $html = gzdecode($html); curl_close($ch); echo '<pre>'; var_dump($html); echo '</pre>'; if (file_put_contents ('data.txt', $html) !== false) { echo 'Success!'; } else { echo 'Failed'; } -
Command Line curl and PHP curl Different results
jcbones replied to codeinphp's topic in PHP Coding Help
Well, I played with it a bit, and found the problem. curl_setopt($ch, CURLOPT_POSTFIELDS, urlencode('getchannelsched&id=9')); Change to: curl_setopt($ch, CURLOPT_POSTFIELDS, 'action=getchannelsched&id=9'); -
Command Line curl and PHP curl Different results
jcbones replied to codeinphp's topic in PHP Coding Help
If this request relies on sessions, then perhaps the garbage collector has already gobbled that up. Meaning the sessionID doesn't exist on the server. -
Command Line curl and PHP curl Different results
jcbones replied to codeinphp's topic in PHP Coding Help
I notice you have a Content-Length in your PHP script, but not in your command line. Not sure if the page is cutting the data off, or waiting for more. Are you sure the passed data is 27 octets? -
Well, first you would have to wrap it in a form, and specify the form action and method: <form method="post" action"page_to_submit_to"><div class="login"> <input type="text" placeholder="username" name="user"><br> <input type="password" placeholder="password" name="password"><br> <input type="submit" value="Login"> </form> </div> Which you would then get the values by: echo $_POST['user']; echo $_POST['password']; Since you wouldn't want to tell the user what they typed in, instead you would want to log them in. You would send the values to a database, and see if they match up. /*PDO Connection required*/ //Get the password from the database, that is associated with the user. (Dependent on database design). $sql = 'SELECT password FROM user WHERE username = :user LIMIT 1'; //Using PDO we can prepare the query. $stmt = $pdo->prepare($sql); //Then bind the username to the query. $stmt->bindParam(':user', $_POST['user'], PDO::PARAM_STR); //Then execute the query. $stmt->execute(); //and get the results. No while() here, as there is only one row. $result = $stmt->fetch(PDO::FETCH_ASSOC); //we tell pdo to return an associative array //we now verify that the password matches the hased password we stored in the database. //we always hash passwords in the database for security reasons. //we would have run the password through password_hash() function before storing. if(password_verify($_POST['password'],$result['password'])) { //if the passwords match. /*Login successful Do Stuff*/ } else { //if the passwords do NOT match /*Login error Do Stuff*/ }
-
http://https://cloud.google.com/translate/docs Google has it.
-
Changing the encoding to utf-8 will not change the Unicode character set. The character is what the character is, in other words. To change from Chinese to English, you would have to translate it.
-
Need help figuring out values that I need
jcbones replied to NalaTheFurious's topic in PHP Coding Help
So: $pageNumber = (int)$_GET['page']; $stop = $pageNumber * 10; $start = $stop - 9; ?? -
You are assigning values, not comparing them. = (assignment) == (comparison)
-
So you are looking to merge two images. function watermark_image($oldimage_name, $new_image_name=null,$watermark){ //global $image_path; //don't use global, as it leads to hard to maintain code. list($owidth,$oheight) = getimagesize($oldimage_name); $width = $height = 500; //this should be set as an option for code reusability. //create main image. $im = imagecreatetruecolor($width, $height); $img = imagecreatefromjpeg($oldimage_name); imagecopyresampled($im, $img, 0, 0, 0, 0, $width, $height, $owidth, $oheight); //create watermark image. $img_src = imagecreatetruecolor($width,$height); $wm = imagecreatefrompng($watermark); list($wmWidth,$wmHeight) = getimagesize($watermark); imageAlphaBlending($img_src, false); imageSaveAlpha($img_src, true); imagecopyresampled($img_src,$wm,0,0,0,0,$width,$height,$wmWidth,$wmHeight); //merge the images, we will use imagecopy() instead of imagecopymerge() because we don't need to set trans. imagecopy($img, $img_src, 0, 0, 0, 0, $width,$height); //if there is a new image name, save the image. if(!empty($new_image)) { imagejpeg($img,$new_image_name,100); imagedestroy($im); return true; } else { //if there isn't a new image name, output the image. imagejpeg($img,null,100); imagedestroy($im); return true; } return false; } //set content header. header('Content-Type: image/jpeg'); //call and output the function. (since we didn't send an image name.) watermark_image($image_path.$image, null,$image_path.$watermark);
-
Start with this Tutorial http://www.phpfreaks.com/tutorial/php-add-text-to-image. It's a little dated, but I don't think much has changed. There are also some pretty good script samples in the manual on imagettftext.
-
Perhaps start with mcrypt_encrypt and mcrypt_decrypt.
-
The best for what? There is no best, everything is relative.
-
To point out further: or, question? $stmt->bind_param('issssssib', $escola, $form, $data, $horas,$local,$dest, $datas, $visto, $file_path ); Why are you sending the file path as a blob? If you need it to be a blob, you couldn't send it with mysqli-stmt.execute. You would need to send it with mysqli-stmt.send-long-data, this fact is noted in the manual on mysqli-stmt.bind-param.
-
Yes, buy a Ferrari, and all should be well.
-
You're right Barand, if I have to have an excuse, I'll go with cross-eyed!
-
Barand, I'm guessing that he has the usernames multiple times in the permissions table, for the different levels. It is a bad design with bad logic, but with the example that the OP put forward, this is how it looks to be.
-
The function in_array doesn't accept multiple arrays. How about a simple: "SELECT username FROM permissions WHERE level = 'Admin' AND level = 'Super User'";
-
We do not advocate w3schools around here. A LOT of their information is outdated, or just plain wrong. For javascript, I suggest the jquery library. http://http://www.jquery-tutorial.net/ For PHP, I suggest the default manual tutorial http://http://php.net/manual/en/tutorial.php
-
If you decide to do this by AJAX, then here is a tut for you. http://http://www.jquery-tutorial.net/ajax/introduction/
-
I'll bite: <?php //expected data: 45:16.2432432;19:2465467;12;4 //file_path to the saved file. $file_path = 'uploads/'; //file name of the temp file: $file_name = sys_get_tmp_dir() . $_SERVER['uploaded_file']['tmp_name']; //name of the saved file. $new_file = $_SERVER['uploaded_file']['tmp_name']; //if temp file doesn't exist. if(!file_exists($file_name)) { exit('The requested file was not uploaded.'); } //if the file exists, then get it's contents into a string. $contents = file_get_contents($file_name); //test for expected data. $contents = preg_replace('/[^0-9:.;]/','',$contents); //append it to the saved file, testing to make sure it saved. if(file_put_contents($file_path . $new_file,$contents . PHP_EOL, FILE_APPEND) !== FALSE) { echo 'success.'; }