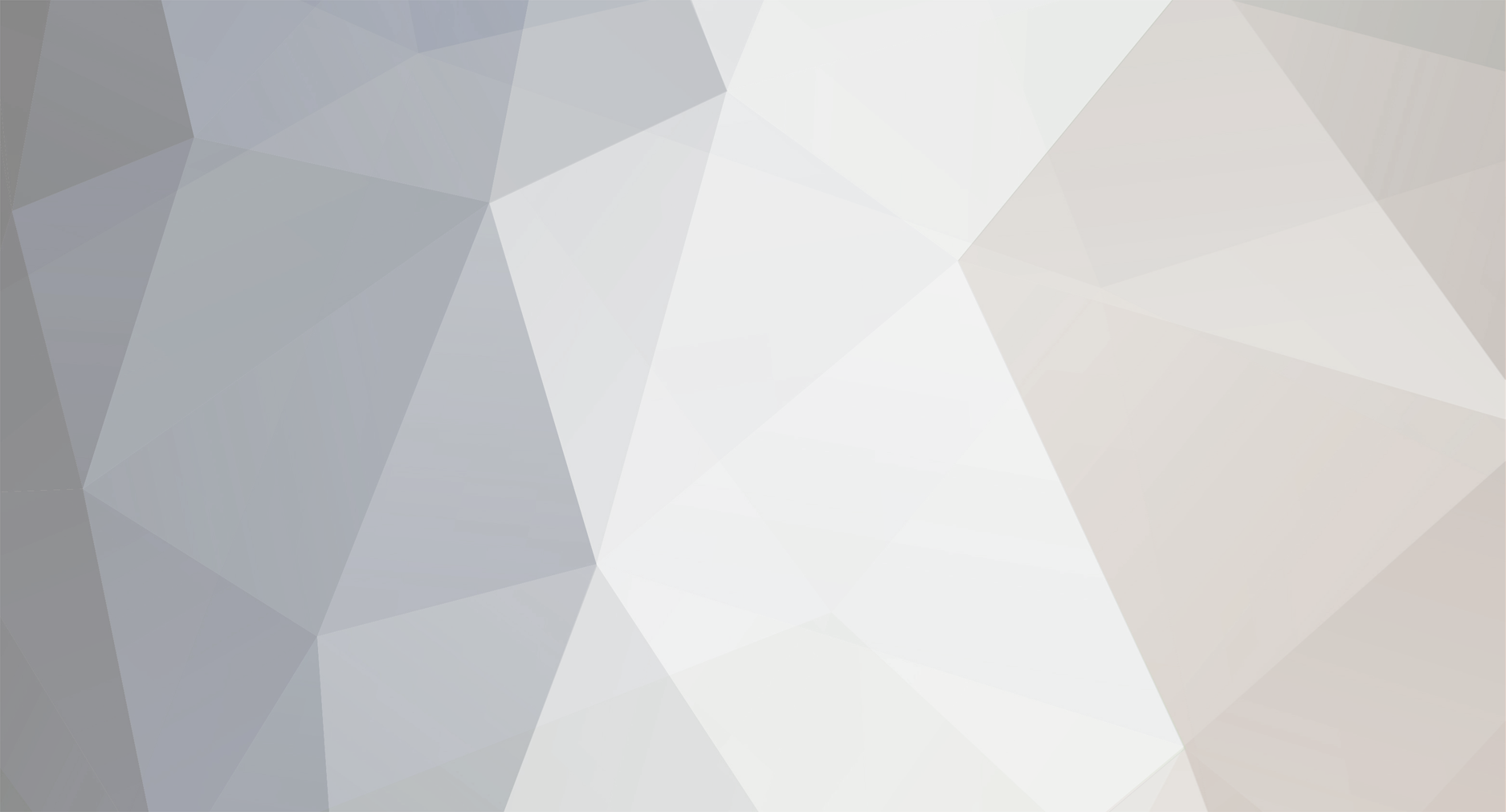
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
Most browsers now-a-days accept the CSS column style. http://https://developer.mozilla.org/en-US/docs/Web/Guide/CSS/Using_multi-column_layouts
-
I believe he is using the Mysqli library, or else he would have gotten a non-member function error. If so, the OP still needs to prepare the query before the bind_param. $sql_string = "INSERT INTO users SET username = ?, password = ?"; $stmt = $mysqli->prepare($sql_string); $stmt->bind_param('ss',$username,$password); $stmt->execute(); if($stmt->affected_rows == 1) { echo "Your account has been successfully created!"; }
-
Perhaps it isn't in the battle start, but in the battle end. As in "how do you end the battle?"
-
Call to undefined method PDOStatement::bind_param()
jcbones replied to Tom10's topic in PHP Coding Help
I believe you are looking for: PDOStatement.bindParam -
$sql = "SELECT User_Id,Username, Date, Time FROM $table WHERE `Date` = CURDATE() ORDER BY User_Id"; //query $table = '<table> <tr> <th>User_Id</th> <th>Username</th> <th>Date</th> <th>In</th> <th>Leave</th> <th>Back IN</th> <th>Out</th> </tr>'; //table headers. $lastId = NULL; //set variable, default value null. foreach($pdo->query($sql,PDO::FETCH_ASSOC) as $row) { loop through the query. if($lastId != $row['User_Id']) { //if the last id doesn't match the new id. if($lastId != NULL) { $table .= '</tr>'; } //but the last id isn't null, close the row. $table .= '<tr><td>' . $row['User_Id'] . '</td><td>' . $row['Username'] . '</td><td>' . $row['Date'] . '</td>'; //start a new row, with standard info. } $table .= '<td>' . $row['Time'] . '</td>'; //then show the time, Hope there isn't more than 5 rows. $lastId = $row['User_Id']; Change lastId to current ID. } //end loop. $table .= '</tr></table>'; //end table. echo $table; //print table to screen.
-
Change this line: (It shows it like this in the manual, but setting true returns the function as a string, so it wouldn't be printed in a die() or exit().) die( print_r( sqlsrv_errors(), true) ); To: die( print_r( sqlsrv_errors())); Then tell us what errors it is printing out.
-
You need to store ALL of the ID's that you send mail to, then you need to update those rows. while($row = mysql_fetch_assoc($sql)) { $ids[] = $row['idalert']; . . . } else { $sql = "UPDATE alert SET sent='1' WHERE idalert IN (" . implode(',',$ids) . ")";
-
I concur, you need to echo out the fully built search query to see what it says, if it is correct, and if it interacts with the database like you expect.
-
Hope this answers the latest question: <?php //data used for testing: $array1 = [1, 5, 10, 20, 40, 80]; $array2 = [6, 7, 20, 80, 100]; $array3 = [3, 4, 15, 20, 30, 70, 80, 120]; //declare a variable called values, empty array: $values = []; //combine arrays, assign them as $ar. foreach ([$array1, $array2, $array3] as $ar) { <-- take the 3 arrays and assign it to $ar - I get this //loop through each $ar index, pulling just the $value; foreach ($ar as $value) { <-- How do you get from 3 arrays in $ar to just the values from the arrays? //if there is not an index in the $values array, that is the same as the $value of the current $ar index. if (!isset($values[$value])) { <-- If the values array passing in the array values variable $value is not set then it equals 0 / why is this? //then set the index, and the counter to 0, this just declares the index so that it doesn't throw a notice. $values[$value] = 0; } //then we increment that value, so we have a count of the occurences. If this is the first occ, then we increment from 0 to 1. //*Note* no need to assign a variable name to this, it serves no purpose. $a = $values[$value]++; <-- Why do you increment this here for what purpose? } } //declare an empty array in variable $commonValues; $commonValues = []; //loop through the values array we created in the loop above. Remember that we are holding the numbers in index, and the count in value. foreach ($values as $value => $count) { <-- Can you explain what this foreach is doing? Stepping through it? //if the count is greater than 2, if ($count > 2) { //add it to the commonValues array, auto incrementing the index. $commonValues[] = $value; } } //print the array $commonValues to the screen, Note, there is a mis-spelling here. print_r($common_values);
-
Here is your problem: You want the directory of the file you choose in the form above. $path1 = pathinfo($_GET["pathw"]); $paths = $path1['dirname']; //selects directory name of the filepath you choose. You then copy the directory to a new variable: $pathw = $paths; You then refresh, setting the URL_QUERY_STRING as the current directory: echo "<meta http-equiv=\"refresh\" content=\"0;URL=mov1.php?pathw=$pathw\">"; When it refreshes, $path1 = pathinfo($_GET["pathw"]); Get the path info for the DIRECTORY. Since you sent the directory (not file name) in the URL_QUERY_STRING. $paths = $path1['dirname']; //Choose the directory ONE LEVEL UP.
-
All of this, and y'all still missed the connection as first param: mysqli_query($connection,$query); In this case: $insert = mysqli_query($fon,"INSERT INTO 1141650_sigma22.JoinDNO (ID, FirstName, LastName, City, State) VALUES (NULL, '$FirstName', '$LastName', '$City', '$State')"); This should work, provided you changed the $state variable declaration to $State.
-
Don't forget to unlink the page, after the site is set up, otherwise any idiot could change the site creds. Temporarily closing the site down.
-
help on designing CBT (computer based testing) in php
jcbones replied to valdes's topic in PHP Coding Help
Remember that relational databases need to be at least 3rd normal form. https://www.youtube.com/watch?v=yYioLVWgh64 -
Try changing: $prices = (array)$dataDecode->line_items[0]->tax_lines; To: $prices = (array)$obj->tax_lines; Oversight in translation.
-
On a side note, you need to check with your host. A lot of host will not send the mail unless it is FROM an address on the server.
-
hey, I'm not pimply faced.
-
One closer look, I believe this is what you are looking for. try { $dtbs = new PDO($dsn_dev, $pdo_user, $pdo_password, array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION)); } catch (Exception $e) { die('Error : ' . $e->getMessage()); } try { $stmt = $dtbs->prepare("INSERT INTO $tabledata ($mysql_email, $mysql_sku, $mysq_price, $mysql_price_with_tax, $mysql_price__tax1___line_items, $mysql_price__tax2___line_items ) VALUES (:email, :sku, :price, :price_with_tax, :price_tax1, :price_tax2)"); foreach ($dataDecode->line_items as $obj) { $prices = (array)$dataDecode->line_items[0]->tax_lines; $price1 = !empty($prices[0]) ? $prices[0]->price : 0.00; $price2 = !empty($prices[1]) ? $prices[1]->price : 0.00; $stmt->execute(array(':email'=>$email, ':sku'=>$obj->sku;, ':price'=> $obj->price, ':price_with_tax'=>$obj->price_with_tax, ':price_tax1'=>$price1, ':price_tax2'=>$price2 )); } } catch(Exception $e) { throw $e; }
-
Not sure what you are expecting for your "good" value. However, I think the code may be confusing you a bit. I've took the liberty to clean it up a might, with some notations. Hope it helps. try { $dtbs = new PDO($dsn_dev, $pdo_user, $pdo_password, array(PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION)); } catch (Exception $e) { die('Error : ' . $e->getMessage()); } try { $stmt = $dtbs->prepare("INSERT INTO $tabledata ($mysql_email, $mysql_sku, $mysq_price, $mysql_price_with_tax, $mysql_price__tax1___line_items, $mysql_price__tax2___line_items ) VALUES (:email, :sku, :price, :price_with_tax, :price_tax1, :price_tax2)"); foreach ($dataDecode->line_items as $obj) { foreach ($dataDecode->line_items[0]->tax_lines as $obj2) { $stmt->execute(array(':email'=>$email, ':sku'=>$obj->sku;, ':price'=> $obj->price, ':price_with_tax'=>$obj->price_with_tax, ':price_tax1'=>$obj2->title, //is this suppose to be rate? ':price_tax2'=>$obj2->price )); } } } catch(Exception $e) { throw $e; }
-
Email is a tricky thing, as it has a lot to do with white list, black list an so on. If you are on a shared server, the server could be blacklisted. Some servers also require the FROM address to be an address active on the server. I normally suggest that people use phpmailer, as they make it somewhat easier. The confusing part with your thread is that you have moved to a different set up, and/or you aren't giving enough info. Bottom line, using a mailing library like PHPMailer, SwiftMailer, Zend_Mail, eZcomponents etc. would have you already up and running.
-
IIRC CRLF is /r/n not /n/n. As a side note, you should be including a strlen in the fputs function, or it could be corrupted.
-
You would normalize the data to at least the 3rd form. That means you would have a: Users table, this holds all data relative to the user. Name, Address, Password, Group, etc. Fish table, this holds all data relative to a type of fish. Type, Description, WaterType, Schooling, etc. Catch table, this would hold each individual catch, tying back to the userID, and the fishID. userID, fishID, weight, length, etc. You could go further, and have a table with all the local, or territorial waters, then tie the fish back to it. Bottom line is that databases are not spreadsheets. Each individual entry gets its own line, there should be little to no repeated data.
-
It will be slow, especially if there are a lot of returns. Each call to the api function creates overhead. If you call it once, twice, thrice, there may never be a problem. You make that call ten times, you will notice it.
-
If every image is the same size, you can set the style attribute to float:left, and it will auto size for the width of the parent division.