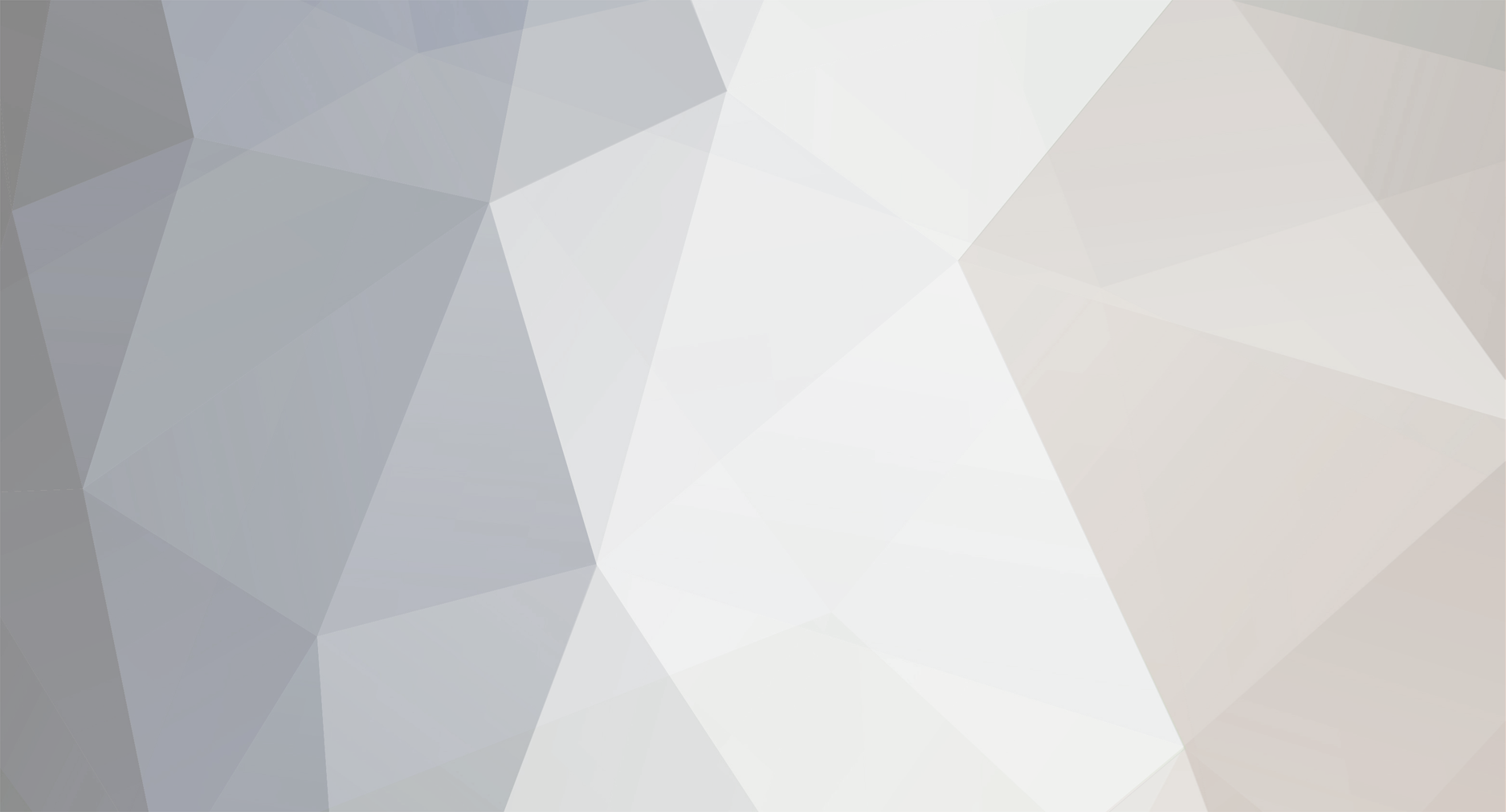
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
In short, your query is failing. replace $query = mysql_query("SELECT user.username, student.student_fn, student.student_ln, student.student_mi, section.year, section.section FROM ubhsocr.user INNER JOIN (ubhsocr.section INNER JOIN student ON section.section_id = student.section_id) ON user.user_id = student.user_id where user.type='Student' ORDER BY user.username LIMIT $startResults, $resultsPerPage"); with $query = mysql_query("SELECT user.username, student.student_fn, student.student_ln, student.student_mi, section.year, section.section FROM ubhsocr.user INNER JOIN (ubhsocr.section INNER JOIN student ON section.section_id = student.section_id) ON user.user_id = student.user_id where user.type='Student' ORDER BY user.username LIMIT $startResults, $resultsPerPage") or trigger_error(mysql_error()); Make sure: To of script <?php error_reporting(-1); ini_set('display_errors','1'); This should tell you why the query is failing. PS. You should be using the mysqli or PDO libraries, and not mysql for compatibility and security issues.
-
hiding a menu option based on user role. Not WP
jcbones replied to laflair13's topic in PHP Coding Help
The easiest route I can see off hand is: <?PHP session_start(); if (isset($_SESSION['loggedin']) && $_SESSION['loggedin'] == true) { // User still logged $role = $row['role']; // You can then use that variable later in page // If $role == 1, Admin, show menu, prevent function access, ect } else { header ("Location: index.php"); } if ($_SESSION['role'] == '2') { $showdiv = 'supernavbar'; } else if ($_SESSION['role'] == '1') { $showdiv = 'navbar'; } //echo "<script type=\"text/javascript\">document.getElementById('".$showdiv."').style.display = 'block';</script>"; ?> <div class="mainbar"> <div id="menu"> <?php include($showdiv . '.php'); ?> </div> </div> <!-- /.mainbar --> -
Mysql column to array with batches what am I doing wrong?
jcbones replied to cobusbo's topic in PHP Coding Help
Thanks Barand, I stand corrected. For some reason I was thinking chunk returned a single dimension. Sometimes, I need to quit thinking. -
Mysql column to array with batches what am I doing wrong?
jcbones replied to cobusbo's topic in PHP Coding Help
The first thing that crosses my mind when you say that you want to put a database column into an array is, "You are storing your data wrong". That being said, $batch would never be an array, since it would be sent as a string from the database, so you cannot join it. -
There are a couple of things to note. 1. You should learn JOIN's, you can pull all of this form data with one query. 2. You should migrate to the MySQLi or PDO libraries. <?php $connection=mysql_connect('localhost', '',''); mysql_select_db('db'); $username= $_SESSION['username']; $query = "SELECT g.g_code FROM i_group AS g JOIN instructor AS i ON g.i_id = i.id WHERE i.username = '$username'"; $query2 = mysql_query($query) or trigger_error(mysql_error()); $row2 = mysql_fetch_assoc($query2); <---PROBLEM STARTS HERE (not part of the code)---> echo 'Select a group :'; echo '<form method="post"><select name="code1">'; echo '<br>'; while($row2=mysql_fetch_array($query2)){ echo '<br>'; echo "<option value=\"" . $row2['g_code'] . "\">" . $row2['g_code'] . "</option> "; } echo '</select>'; echo '<input type="submit" name="submit" value="GO">'; echo '</form>'; if(!empty($_POST['code1'])){ $go = $_POST['code1']; $query = "SELECT * FROM student WHERE `group`='$go'"; ///blah,blah } else { echo 'You didn\'t receive a go code!'; }
-
Is the incoming calls voip? If they are, then most voip carriers have call logging for a fee.
-
You are trying to add two values to the database, but you have 5 columns listed. Yes, the database is going to puke on that one. It doesn't know what columns you want to add to. You will either have to shorten the columns, or add to the values. You should be using prepared statements to interact with the database. Couple that with error logging, you will see the problem pretty quick. Edit: prepared statements will cure the data type problem as well.
-
Change: @mail($email_to, $email_subject, $email_message, $headers); To: mail($email_to, $email_subject, $email_message, $headers); See if you get errors. To do this right, you should be using something like PHPmailer -> http://http://phpmailer.worxware.com/index.php
-
Q1. Yes. Q2. You can build a function to test it. function sessionVar($key) { return isset($_SESSION[$key]) ? $_SESSION[$key] : null; } echo 'Now using an empty session ' . sessionVar('index') . ' will not throw an error.'; Although, in this case the function would need to be more robust, as it would throw an error on an empty session variable being sent to is_array().
-
Adding a break rule would separate put each anchor onto a new line. echo "<a href='editUser.php?id={$row['id']}'> Change Password </a><br />";
-
Have you found any JavaScript errors? You can check then using Firefox developer tools.
- 3 replies
-
- google charts
- mssql
-
(and 3 more)
Tagged with:
-
Select them through an anchor, passing the id through the the uri, and receiving the id back through the $_GET array. Then you can display the user on the receiving page.
-
No it instantiates an existing class, and creates an object. In order to access the values, you need to call the indexes. $events = $_POST['test']; PS. Don't use $_REQUEST, it opens up insecurities that you could do without.
-
Help with removing required confirmation on snippet
jcbones replied to PHPJoey89's topic in PHP Coding Help
Sorry, I stopped before I should. My recommended way: Create a new column on the table, int(1) default value 0. When you delete change the value to 1. Change the queries to match the new column (WHERE deleted != 1). This in turn will allow you to reverse a mistake, then you would catch (archive) the deleted rows, to a log file, after a set amount of time. -
Help with removing required confirmation on snippet
jcbones replied to PHPJoey89's topic in PHP Coding Help
You could do this two ways. 1. find the confirm code, and take it out. It may be JS code, that appends the confirm=1 onto the URL query string. 2. Change your delete link by appending confirm=1 onto the URL query string. The correct way: 1. Change the delete link to send a POST to the action page. Only thing that should be passed in a URL query string should be for reading from the database. This change could be done via javascript (ajax), or by creating a form. -
Help with removing required confirmation on snippet
jcbones replied to PHPJoey89's topic in PHP Coding Help
Perhaps admin.php is the page you should be showing. -
Or, if you want something to run if it isn't in the array. if(!in_array($id1,$id)) //if NOT in array.
-
Things you should be doing differently. 1. You should be using mysqli or PDO as stated, they support prepared statements that eliminate the need to structure your query inputs, as well as making sure they are the right type. 2. You should be sanitizing and validating your inputs, you should never trust the client to send the correct data. You must verify the data is correct. 3. You should be using $_POST or $_GET arrays, as you expect them. Never use $_REQUEST. I could make this form submit by sending an URL 'email' query. 4. Your line If(isset($_REQUEST['submit'])!='') will always be true. isset() returns a boolean value, so it is never an empty string. Should probably be: if(isset($_POST['submit'])) { //or better yet: if($_SERVER['REQUEST_METHOD'] == 'POST')
-
Blocking the Access to Multiple Files with .htaccess?
jcbones replied to glassfish's topic in PHP Coding Help
Yes, just that line in a .htaccess file will work. As for the first question, above the htdocs folder. example /home/myfile/htdocs/gallerysite you could store in any folder in /home/myfile and no one would be able to call it with a url, because a url automatically points to the htdocs folder. The server still has access to all folders though. -
Blocking the Access to Multiple Files with .htaccess?
jcbones replied to glassfish's topic in PHP Coding Help
If you don't want them called by a url, then why not move them above the public html files? -
How to print a string that also contains an array?
jcbones replied to E_Leeder's topic in PHP Coding Help
Do you mean like: function printArray(array $arr) { $op = null; foreach($arr as $key=>$val) { if(is_array($val)) { $op .= printArray($val); } else { $op .= $key . ': ' . $val . '<br />'; } return $op; } -
Also, header() locations should be a fully qualified URI and not a relative path.
-
PHP: Insert an associated array variable into a table
jcbones replied to mstevens's topic in PHP Coding Help
So basically, echo "<table>"; $i = 0; foreach ($ZodiacArray as $image => $zodiac) { echo ($i % 4 == 0) ? <tr> : null; echo '<td><img src="$image" alt="$zodiac" style="width:68px;height:65px"></td>'; echo ($i++ % 4 == 0) ? </tr> : null; } echo '</table>'; I think that is error free, but as always, I could be wrong. -
Simply adding an isset doesn't change the fact that the index isn't set. Which is why your photo isn't showing. You have just told it to not even try to load any photos, if the index isn't set. While that is correct, you still must fix the original problem. To debug, add the following to your script. echo '<pre>' . print_r($sk,true) . '</pre>';
-
How to print a string that also contains an array?
jcbones replied to E_Leeder's topic in PHP Coding Help
Use the second argument to print array. echo 'These are the books: ' . print_r($userArray,true) . ' and these are the pages: ' . print_r($pagesArray,true);