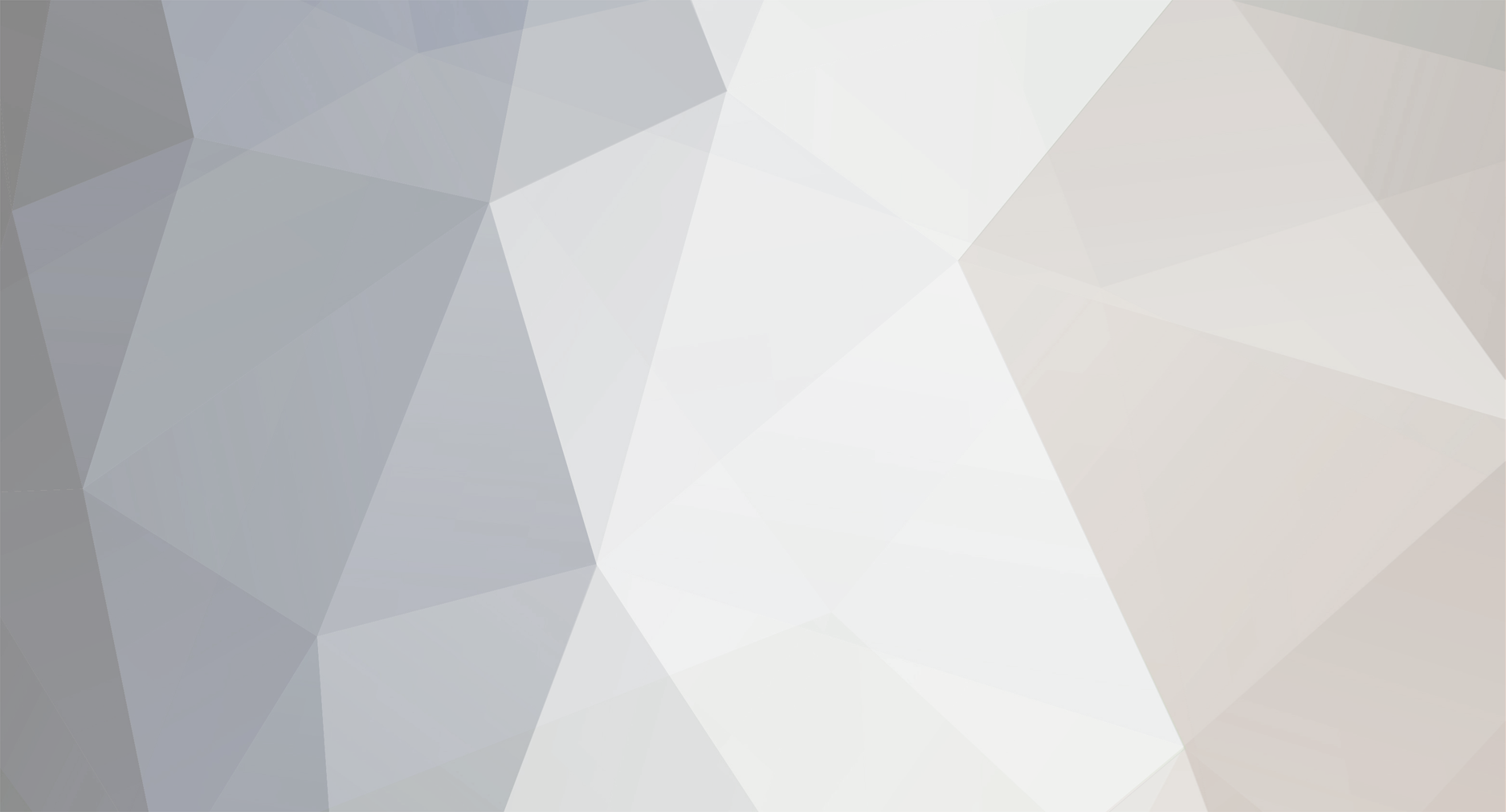
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
So, give us something to work with. Form Insert script We need to see updated scripts with everything you try. I pointed out a problem with your insert script, I assume that was taken care of.
-
You are pulling random names and then sorting them in list of 4 players for each team? <?php $sql = "select * from players ORDER BY Rand() LIMIT 32"; $res = mysql_query($sql) or die (mysql_error()); $i = 0; $team = 0; while ($row = mysql_fetch_array($res)) { if($i++ % 4 == 0) { echo '<br />Team: ' . ++$team . '<br />'; } echo $row['player'] . '<br />'; }
-
To clarify, these are the extra two tables. CREATE TABLE `postcodes` ( `id` int(11) NOT NULL AUTO_INCREMENT, `postcode` int(4) NOT NULL, PRIMARY KEY (`id`) ) CREATE TABLE `events_postcodes` ( `event_id` int(11) NOT NULL, `postcode_id` int(11) NOT NULL )
-
MySQL is relational, so you need a relational table, in fact, a many to many. So, you would have a table with id and postcode. And, a table with postcode_id and event_id that would tie both of these tables together. This way postcode and event is stored once, and then a list of the relationships between the events and postcodes is stored. Which is easily sorted. SELECT event.* FROM event WHERE event.id IN (SELECT event_id FROM events_postcodes JOIN postcodes ON events_postcodes.postcode_id = postcodes.id WHERE postcodes.postcode = '5432') I think that is close, un-tested though.
-
So your problem is, the script checks to see if the id is in the database, but then doesn't pass it to the second query. Yep, no need to run any error checking.
-
For some reason your query is returning 0 rows, thereby not ever making the array. You need to find out exactly under what circumstances this happens. I would surmise that the $id isn't being set properly (is empty). Check the arrays, and throw in an echo on it if it isn't set. $sqlquery = "SELECT * FROM table WHERE id='$id'"; 24. $sqlarray = ($sql); 25. $resultarray = mysql_query($sqlarray,$con)or trigger_error('<p>There seems to be a problem, please try again soon.</p>'); 26. while($rowarray=mysql_fetch_assoc($resultarray)){ 27. $lat[] = $rowarray['lat']; 28. $long[] = $rowarray['long']; 29. } if(is_array($lat) && is_array($long)) { $averagelat= array_sum($lat) / count($lat); $averagelong= array_sum($long) / count($long); } else { echo $sqlquery . ' <<has failed>>'; }
-
You need to decide how you want to tie these together. I would assume (dangerous) that you want all 3 of those heater images tied to only one product (?). The same going for the filters? You should be getting the product id back from mysql with mysql_insert_id, then you can insert it with the rest of your queries. I wouldn't combine the queries into a multi-query (you can, but it would take more knowledge of mysql functions). Let us know, we can get this sorted quickly for you.
-
Date Issue 01/01/2012 (not > then 12/31/2011)
jcbones replied to Failing_Solutions's topic in PHP Coding Help
You can use MySQL's DATE_FORMAT to format the date into how you want, which reduces overhead on PHP(trivial). <?php $sql = "SELECT DATE_FORMAT(date_column,%m-%d-%Y) FROM table"; ?> -
While convention has you list the column first, it will work either way.
-
Your insert query does not contain a topicsID.
-
It was an example, and the first <?php was just so the code block would highlight the code. In essence, it was me being lazy!
-
It is hard to help you when you don't post code. You should be hyper-linking by setting the data to an anchor element, and passing a query string to the end of the URI. <?php echo '<a href="a/link/to/somewhere.php?find=' . $me . '">LINKING</a>'; //to get the value: if(isset($_GET['find'])) { echo $_GET['find']; } ?> $_GET
-
The reason your first attempt could not connect is because you were using an array to assign the data, a constant to call the data. EXAMPLES: <?php //define an array, and it's index. $config['DB_HOST'] = 'localhost'; $config['DB_USER'] = 'root'; $config['DB_PASS'] = ''; $config['DB_NAME'] = 'form'; //call the array into a functions arguments. mysql_connect($config['DB_HOST'],$config['DB_USER'], $config['DB_PASS']); mysql_select_db($config['DB_NAME']); //set constants. define('DB_HOST','localhost'); define('DB_USER','root'); define('DB_PASS',''); define('DB_NAME','form'); //call the constants into a functions arguments. mysql_connect(DB_HOST, DB_USER, DB_PASS); mysql_select_db(DB_NAME); I would write your insert as: <?php require ('resources/DB_connect.php'); if ($_SERVER['REQUEST_METHOD'] == 'POST') { //if your page receives a post. if(empty($_POST['title'])) { //if the title is empty, include the error. $error[] = 'You must include a Title!'; } elseif(empty($_POST['post'])) { //if the post is empty, include the error. $error[] = 'You must include a Post!'; } else { //if everything is filled out, process the insert. $title = mysql_real_escape_string(htmlentities($_POST['title'])); $post = mysql_real_escape_string(htmlentities($_POST['post'])); $sql = "INSERT INTO posts(title,post) VALUES ('$title','$post')"; //I stick with the common insert syntax, nothing wrong with the other update/insert syntax though. if(!mysql_query($sql)) { //if the query fails. trigger_error($sql . ' has encountered an error<br />' . mysql_error()); //trigger an error. } } } ?> <html> <head> <title> Post something! </title> </head> <body> <?php echo (isset($error)) ? implode('<br />',$error) : NULL; //if either of the inputs are blank, post the errors here, if there is an error here, there was no database insert. ?> <div id="form"> <form method="POST" action= ""> <label for="title">Title:</label><br> <input type="text" name="title" id="title" /><br> <label for="post">Post:</label><br> <textarea name="post" id="post" rows="15" cols="50"></textarea><br> <input type="submit" value="Submit" /> </form> </div> </body> </html>
-
I suppose that you are wanting to preserve new lines? As HTML formatting should remain intact (unless you are using htmlentities). If so, you need to look at nl2br.
-
You still need to get the data with one of the fetch functions. mysql_fetch_array mysql_fetch_row mysql_fetch_assoc
-
You include connect.php in your poll.php file with a relative file path. This will work if you are accessing poll.php itself, but will not work if poll.php is included in another file, as that file will do the include. So, PHP is looking in the pages/ folder for the file connect.php. You should put an absolute file path to the connect file. require_once ('absolute/path/to/connect.php'); This is the exact reason that so many of us stress that you must use absolute file paths to every included file.
-
So you want to allow 1 space, but no more? The above code given will remove ALL spaces. 1 space but no more is: preg_replace('~\s\s+~','',$username);
-
You will also be setting slaves to 1, so your users will never have more than 1 slave. I would suggest leaving it up to the database. UPDATE users2 SET slaves=slaves+1, date2=DATE_ADD(NOW(),INTERVAL 20 SECOND) This will do the same as all of your code above, Although, I think you would want to specify a row for a specific user.
-
I discourage the mysql_fetch_array function in favor of the mysql_fetch_row() or mysql_fetch_assoc(), depending on what you want returned. Mysql_fetch_array() just returns both the row and the association thereby using unnecessary memory, when you plan on only using one of them anyway. I also discourage Global keywords in function, pass them in the arguments, you will have less confusion and errors this way. I also discourage storing items in a comma delimited manner inside a table, Mysql is a relational database, and has powerful functionality to tie information together. You should have a 3rd table that has a many to many relationship, and ties the item to the user. CREATE TABLE userItem ( userID int(11), itemID int(11), itemQuantity int(3) ) Then look into using the JOIN syntax.
-
MySqli prepared Statements inside custom class
jcbones replied to gork4life's topic in PHP Coding Help
Try this: <?php error_reporting(-1); ini_set('display_errors',1); include_once 'User.php'; //Creating new User Object $newUser = new User(); $fname = $_POST['fname']; $lname = $_POST['lname']; $email = $_POST['email']; $username = $_POST['username']; $password = $_POST['password']; $conf_pass = $_POST['conf_pass']; $newUser->regUser($fname, $lname, $email,username, $password, $conf_pass); ?> And, <?php include '../includes/Constants.php'; ?> <?php /** * Description of User * * @author Eric Evas */ class User { var $id, $fname, $lname, $email, $username, $password, $conf_pass; protected static $db_conn; //declare variables public function __construct() { $host = DB_HOST; $user = DB_USER; $pass = DB_PASS; $db = DB_NAME; //Connect to database $this->db_conn = new mysqli($host, $user, $pass, $db); //Check database connection if ($this->db_conn->connect_error) { echo 'Connection Fail: ' . mysqli_connect_error(); } else { echo 'Connected'; } } function regUser($fname, $lname, $email, $username, $password, $conf_pass) { if ($stmt = $this->db_conn->prepare("INSERT INTO USERS (user_fname,user_lname, user_email,username,user_pass) VALUES (?,?,?,?,?)")) { $stmt->bind_param('sssss', $fname, $lname, $email, $username, $password); if(!$stmt->execute()) { printf("Error: %s.\n", $stmt->error); } elseif($stmt->affected_rows > 0) { printf('Your query was successful!'); } //$stmt->store_result(); //there is nothing to store here. $stmt->close(); } } } ?> -
Is your slaves columns suppose to increment every 20 seconds, or only every 20 seconds while the user is online? Is there a maximum number of slaves at one time? The reason all these questions are asked, is we can suggest a more efficient way, and possibly keep it in the database itself.
-
MySqli prepared Statements inside custom class
jcbones replied to gork4life's topic in PHP Coding Help
Variables must be set as class properties before trying to access them as class properties. Since your variables are passed into the function as arguments, then they must be accessed inside the function as function variables. In other words lose the $this->, or declare them as properties with $this->. In your second script you are assigning the variables as class properties inside your script. If you do that, there is no need to pass them as function arguments, and you can forget the rules above. In this script your flow will read as: assign these variables to the class as properties, then retrieve the same properties from the class, and pass them to a function that resides in that same class. Or, as I like to say, leave by the front door, and run around the neighborhood to get to the back door. So, do this: function regUser($fname, $lname, $email, $username, $password, $conf_pass) { if ($stmt = $this->db_conn->prepare("INSERT INTO USERS (user_fname,user_lname, user_email,username,user_pass) VALUES (?,?,?,?,?)")) { $stmt->bind_param('sssss', $fname, $lname, $email, $username, $password); $stmt->execute(); $stmt->store_result(); $stmt->close(); } } AND //Creating new User Object $newUser = new User(); $fname = $_POST['fname']; $lname = $_POST['lname']; $email = $_POST['email']; $username = $_POST['username']; $password = $_POST['password']; $conf_pass = $_POST['conf_pass']; $newUser->regUser($fname, $lname, $email, $username, $password, $conf_pass); ?> If this doesn't produce the results you desire, it is time to find the database errors. For this you will want to look at: mysqli::error() -
Here you go: project.php <?php //session start must come BEFORE any output to the page. session_start(); //start session; if($_SERVER['REQUEST_METHOD'] == 'POST') { //if there was a form posted. $_SESSION['post_data'] = $_POST; //store all post data to the session array. } //debugging: //echo '<pre>' . print_r($_SESSION,true) . '</pre>'; ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Frameset//EN" "http://www.w3.org/TR/html4/frameset.dtd"> <html> <head> <title>Title of the document</title> <meta name="description" content="Type a Short Description Here" /> <meta name="keywords" content="type, keywords, here" /> <meta name="author" content="Your Name" /> <meta http-equiv="content-type" content="text/html;charset=UTF-8" /> <link rel="stylesheet" type="text/css" href="mystyle.css" /> </head> <body> <?php $number_of_pizzas_to_order = 3; //tell the form how many pizza's you would like to offer. echo "Order Type: <br/> \n"; echo"<form method='post' action='project2.php'>"; //set the variables. $DEL = NULL; $TAKE = NULL; $EAT = NULL; //check the appropriate box. if(isset($_SESSION['post_data']['ORDER'])){ if ($_SESSION['post_data']['ORDER'] == 'delivery') { $DEL='checked' ; }elseif ($_SESSION['post_data']['ORDER'] == 'takeout') { $TAKE='checked' ; }elseif ($_SESSION['post_data']['ORDER'] =='eatin') { $EAT='checked' ; } } else { $TAKE = 'checked'; } echo "Delivery <input type='radio' name='ORDER' value='delivery' $DEL> \n Take Out <input type='radio' name='ORDER' value='takeout' $TAKE> \n Eat in <input type='radio' name='ORDER' value='eatin' $EAT>\n"; //set the variables. //build form dynamically. Allows for edits. for($i = 1; $i <= $number_of_pizzas_to_order; $i++) { //preset variables. $no_pizza = NULL; $cheese = NULL; $pepporoni = NULL; $veggie = NULL; $meat_lovers = NULL; $Small = NULL; $Medium = NULL; $Large = NULL; if(!empty($_SESSION['post_data']['pizza'][$i])) { //if this is an edit. $$_SESSION['post_data']['pizza'][$i]['type'] = 'selected="selected"'; //only select the right pizza type $$_SESSION['post_data']['pizza'][$i]['size'] = 'selected="selected"'; //and size } //echo a heredoc (cause I love em). echo <<<EOF <br/>Pizza {$i}: <select name='pizza[{$i}][type]'>\n <option></option>\n <option $no_pizza value="no_pizza">no pizza</option>\n <option $cheese>cheese</option>\n <option $pepporoni>pepporoni</option>\n <option $veggie>veggie</option>\n <option $meat_lovers value="meat_lovers">meat lovers</option>\n </select> \n Pizza size: <select name='pizza[{$i}][size]'>\n <option></option> <option $Small>Small</option> <option $Medium>Medium</option> <option $Large>Large</option> </select> EOF; } //Build drink section dynamically, allowing for edits. //just like above. echo '</br> <br/>What drink would you like? <br />'; for($i = 1; $i <= $number_of_pizzas_to_order; $i++) { $No_drink = NULL; $Bottled_Pepsi = NULL; $Bottled_Fanta = NULL; $Bottled_Sprite = NULL; $Bottled_RootBeer = NULL; $halfLiter = NULL; $liter = NULL; $doubleLiter = NULL; if(!empty($_SESSION['post_data']['drink'][$i])) { $$_SESSION['post_data']['drink'][$i]['type'] = 'selected="selected"'; $$_SESSION['post_data']['drink'][$i]['size'] = 'selected="selected"'; } echo <<<EOF Drink {$i}:<select name='drink[{$i}][type]'>\n <option></option>\n <option $No_drink value="No_drink">No drink</option>\n <option $Bottled_Pepsi value="Bottled_Pepsi">Bottled Pepsi</option>\n <option $Bottled_Fanta value="Bottled_Fanta">Bottled Fanta</option>\n <option $Bottled_Sprite value="Bottled_Sprite">Bottled Sprite</option>\n <option $Bottled_RootBeer value="Bottled_RootBeer">Bottled RootBeer</option>\n </select>\n Drink size: <select name='drink[{$i}][size]'>\n <option></option>\n <option $halfLiter value="halfLiter">.5 liter</option>\n <option $liter value="liter">1 liter</option>\n <option $doubleLiter value="doubleLiter">2 liter</option>\n </select>\n EOF; } echo "<br/> <br/> <input type='submit' value='submit your order' name='submit'> </form>"; ?> </body> </html> project2.php <?php session_start(); //always update the session post data. $_SESSION['post_data'] = $_POST; ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Frameset//EN" "http://www.w3.org/TR/html4/frameset.dtd"> <html> <head> <title>Title of the document</title> <meta name="description" content="Type a Short Description Here" /> <meta name="keywords" content="type, keywords, here" /> <meta name="author" content="Your Name" /> <meta http-equiv="content-type" content="text/html;charset=UTF-8" /> <link rel="stylesheet" type="text/css" href="mystyle.css" /> </head> <body> <?php //pizza's are in array now, you can add, delete, and change prices all in one place. $pizza = array( 'cheese' => array(10=>'Small',13=>'Medium',15=>'Large'), 'pepporoni' => array(12=>'Small',14=>'Medium',16=>'Large'), 'veggie' => array(12=>'Small',14=>'Medium',16=>'Large'), 'meat lovers' => array(13=>'Small',15=>'Medium',17=>'Large') ); //drinks are in array, had to make it multi-dem to overcome a problem with spaces, and variable naming conventions. $drink = array( 'halfLiter' => array('.69' => '.5 liter'), 'liter' => array('.99' => '1 liter'), 'doubleLiter' => array('1.69' => '2 liter') ); $total = 0; //start our total at 0. echo "<br/><br/>Your Order is:<br/>"; foreach($_SESSION['post_data']['pizza'] as $k => $v) { //go through our posted data. (pizza section). $type = str_replace('_',' ',$v['type']); //handling variable naming by adding and removing underscores for spaces. if(array_key_exists($type,$pizza)) { //if the pizza type is in the pizza array. $_SESSION['post_data']['pizza'][$k]['price'] = array_search($v['size'],$pizza[$type]); //return the key (price) from that array, building another index into the session array. } else { $_SESSION['post_data']['pizza'][$k]['price'] = 0.00; //else set it at 0. } //string below is the individual output for each pizza product. $pizs[] = ucwords($type) . ' ' . $v['size'] .' (' . $_SESSION['post_data']['pizza'][$k]['price'] . ')'; //build our output string, and add it to an array. $total += $_SESSION['post_data']['pizza'][$k]['price']; //add our price to the total. } foreach($_SESSION['post_data']['drink'] as $k => $v) { //go through the drink section of the posted data. if(array_key_exists($v['size'],$drink)) { //if the size is in the drink array. $keys = array_keys($drink[$v['size']]); //get the keys of that array (only 1 exists), this key is the price. $size = $drink[$v['size']][$keys[0]]; //now we request the contents of that key, which is the real size (had to be done to allow for editing the original form). $_SESSION['post_data']['drink'][$k]['price'] = $keys[0]; //now add the price to a new index in the drink array. } else { $_SESSION['post_data']['drink'][$k]['price'] = 0.00; //unless it doesn't exist, then we make it 0. } //string below is the individual output for each drink product $drks[] = str_replace('_',' ',$v['type']) . ' ' . $size .' (' . $_SESSION['post_data']['drink'][$k]['price'] . ')'; //the string for each drink now goes to the drks array. $total += $_SESSION['post_data']['drink'][$k]['price']; //and the price gets added to the total. } //next we tie the pizs array together with a comma, break the rule, then tie the drks array together with a comma. Finally sending our total to the screen. echo implode(',',$pizs) . '<br />' . implode(',',$drks) . '<br /><br /> <br/><br/>Your total price is: ' . $total . '<br/> <br/>'; //debugging. //echo '<pre>' . print_r($_SESSION,true) . '</pre>'; //pretty much the same as you had, just changed where to look for it. if ($_SESSION['post_data']['ORDER']=='delivery') { echo"Please enter the information required for delivery <br/>" ; echo "Address: <input type=text><br/>"; echo "City: <input type=text><br/>"; echo "Zip Code: <input type=text><br/>"; } elseif ($_SESSION['post_data']['ORDER']=='takeout') { echo "Your order will be ready for pickup in 30 min"; } elseif ($_SESSION['post_data']['ORDER']=='eatin') { echo "Your order will be ready in 30 min"; } echo"<form action=asdf.php>"; echo"<br/> <input type=submit value='confirm this order'>"; echo"</form>"; //we don't have to send hidden fields, all the info is in the session. As long as it is a GET form. echo "<form action='project.php'> <br/> <br/><input type=submit value='edit this order'> </form>"; ?> </body> </html> Fully commented up. There are ways to tighten it up a bit, I'll let you work on that.
-
The reason to do it the second way is for error handling. $safe4 = "UPDATE banned SET time"; mysql_query($safe4) or trigger_error($safe4 . ' has encountered and error<br />' . mysql_error()); is much more descriptive than: mysql_query("UPDATE banned SET time") or trigger_error(mysql_error();
-
It would be quite simple to condense all of that into one single script. But, you will need to divide the logic and the display. When you do this, you notice that you can lose a lot of the code that you have. <?php session_start(); //start the session. $message = NULL; //set a message variable. $login = FALSE; //login is false on every page start. if($_SERVER['REQUEST_METHOD'] == 'POST') { //if the form was submitted. function clean($data) //generic clean function. { $data = trim($data); $data = stripslashes($data); $data = htmlspecialchars($data); return $data; } $user = clean($_POST['username']); //"cleaned" post data. $pass = clean($_POST['password']); //"cleaned" post data. if ($user == "webdeveloper" && $pass == "master") //if the user and password fits the hardcoded strings. { $_SESSION['username'] = $user; //set user in the session array. $_SESSION['password'] = $pass; //set password in the session array. $_SESSION['ON'] = TRUE; //session ON is now true. $lifetime=600; setcookie(session_name(),session_id(),time()+$lifetime); //setting a cookie ?? } else { $message = 'Wrong Username or Password'; //if login failed, send a message to the user. } } if (empty($_SESSION['ON']) || $_SESSION['ON'] == FALSE || isset($_GET['Logout'])) { //if session is not ON, or LOGOUT is requested. $login = FALSE; //set the login to false. session_unset(); //unset the session. session_destroy(); //terminate the session. } elseif(!empty($_SESSION['ON']) && $_SESSION['ON'] == TRUE) { //else if the session is NOT empty, and is TRUE. $login = TRUE; //LOGIN is also true. } if($login == TRUE) { //if LOGIN is true, print the block below to the page. ?> <html><head><title>LOGIN WEB SESSION</title> <link rel="stylesheet" type="text/css" href="loginsession.css" /> </head> <body><div>LOGIN WELCOME</div> <div> HERE IS YOUR USER DATA:<br /> <label class="data">username:<?php echo $_SESSION['username'] ?><br> password: <?php echo $_SESSION['password'] ?><br></label> HERE IS YOUR SESSION DATA:<br /> <label class="data"> <?php echo (session_name().": ".session_id()) ?><br> <?php echo ("SESSION DATA: ".session_encode() )?> </label> </div> <div><a href="?Logout=1">Logoff</a></div> </body> </html> <?php } else { //else login will be false, so show the form. ?> <html><head><meta http-equiv="content-type" content="text/html; charset=iso-8859-1"> <!--USERID: webdeveloper PASSWORD: master --> <title>LOGIN WEB SESSION</title> <link rel="stylesheet" type="text/css" href="loginsession.css" /> </head> <body> <form action="?Login=1" method="post"> <?php echo $message; //show a message if it exists.?> <table> <tr><td width="100">Username:</td><td width="200"><input type="text" name="username"></td></tr> <tr><td width="100">Password:</td><td width="200"><input type="password" name="password"></td></tr> <tr><td colspan="2" align="center"><input type="submit" value="Login"></td></tr> </table> </form> </body> </html> <?php } ?> Don't know why copy/paste from Geany double spaces!