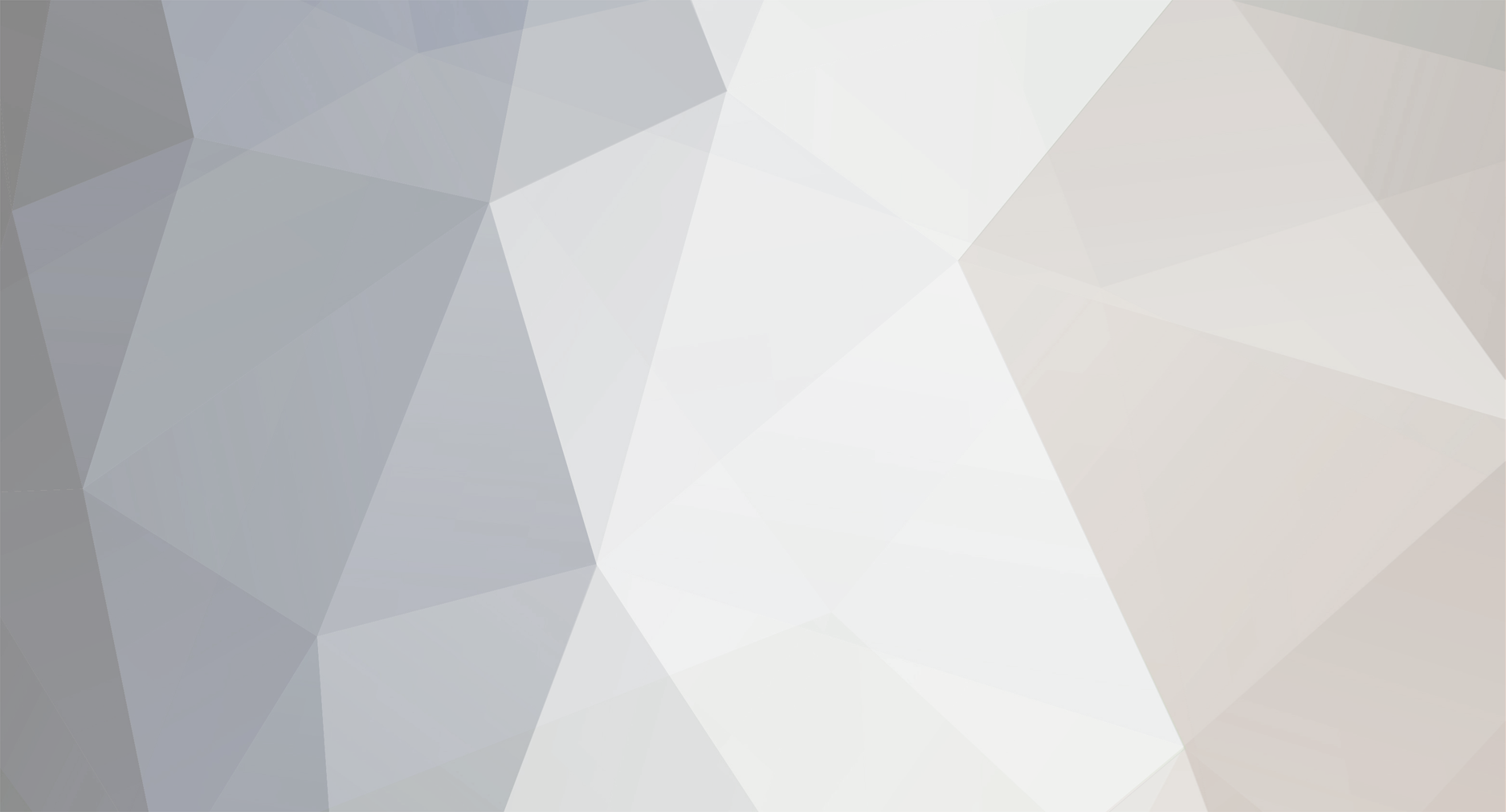
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
PHP Help with multiple choice for search page (mySQL)
jcbones replied to WebsiteDesigner's topic in PHP Coding Help
Your logic doesn't have the right flow, try this: <?php } else{ if(!empty($_GET['latt']) && !empty($_GET['long'])) { $condition="SELECT ( 3959 * acos( cos( radians( '".$_GET['latt']."' ) ) * cos( radians( latitude ) ) * cos( radians( longitude ) - radians( '".$_GET['long']."' ) ) + sin( radians( '".$_GET['latt']."' ) ) * sin( radians( latitude ) ) ) ) as distance,id,membership FROM notary_members "; //declare an array, this will hold our columns if they are in the search params. $queryCon = array(); //set the columns to an array ONLY if they exist in our search params. if(!empty($_GET['edoc'])) { $queryCon[] = 'edoc=\'yes\''; } if(!empty($_GET['esign'])) { $queryCon[] ="esign= 'yes'"; } if(!empty($_GET['laserprinter'])) { $queryCon[] ="laserprinter= 'yes'"; } if(!empty($_GET['dualtray'])) { $queryCon[] ="dualtray= 'yes'"; } if(!empty($_GET['error_in'])) { $queryCon[] ="error_in= 'yes'"; } if(!empty($_GET['bg_checked'])) { $queryCon[] ="bg_checked= 'yes'"; } if(!empty($_GET['home_insp'])) { $queryCon[] ="home_insp= 'yes'"; } if(!empty($_GET['fingerprint'])) { $queryCon[] ="fingerprint= 'yes'"; } if(!empty($_GET['hosp_signing'])) { $queryCon[] ="hosp_signing= 'yes'"; } if(!empty($_GET['jail_signing'])) { $queryCon[] ="jail_signing= 'yes'"; } if(!empty($_GET['im_docs'])) { $queryCon[] ="im_docs= 'yes'"; } //since you have a constant column called status, then make your WHERE. $condition .= ' WHERE '; //if the array isn't empty (search params exist ) then implode the columns. if(!empty($queryCon)) { $condition .= implode(' AND ',$queryCon) . ' AND '; } //set the last part of the query, which would be the status column, and order by. $condition .= 'status = 1 ORDER BY distance ASC'; $sql=mysql_query($condition); $num=mysql_num_rows($sql); $getmile=explode(' ',$_GET['miles']); $c=0; while($getidres=mysql_fetch_array($sql)) { if($getidres['distance']<=$getmile[0]) { if($getidres['membership']=='premium') { $prefirst[$getidres['id']]=$getidres['distance']; $c++; } else { $basicfirst[$getidres['id']]=$getidres['distance']; $c++; } } } if(!empty($sql)) { if($c!=0) { if(!empty($prefirst) || !empty($basicfirst)) { ?> Functions used: implode empty -
Yes, buddski, you are correct. Didn't think that one through.
-
1. Make sure your variables are what you think they are... echo them. 2. Do not limit an update, you should specify EXACTLY which row you want to update, otherwise you might be getting a different row than you want. 3. Separate processing and display. Processing should always be done first, then the display. 4. Echo something in your loops, that will let you know where the script is. 5. Make sure your error reporting is set to it's highest, and display errors is on. 6. If all of that fails, take the bare essentials that you desire, and run it in a test script. This will weed out all of the display's etc.
-
To answer your question: variable variables <?php $key = "apple"; $arr = array($$key => "fruit"); ?> Although, I prefer keys and values like: <?php $arr = array('fruit' => 'apple'); echo 'Hmmm, what kind of fruit do we have? Oh an, ' . $arr['fruit'] . '!'; ?>
-
Current time isn't displaying the correct hour.
jcbones replied to D.Rattansingh's topic in PHP Coding Help
If you have PHP5 you should get prompted to set the date_default_timezone_set in any script that runs a date function. Of course, this is if you have error_reporting and display_errors turned on in your development enviroment. -
The current script will update the database everytime it loads. You should put all form processing at the top of your scripts. If you process forms at the bottom of scripts, when the page reloads it looks as if nothing happened (and that is correct, because it happens after page load).
-
how to show the option based on the value in the table?
jcbones replied to tayys's topic in PHP Coding Help
Just start another thread, so that anyone looking at this one will not confuse the two different issues. -
The 2 queries can be made into 1, and is basically there anyway. "SELECT s.statusid, s.status, s.time_posted, s.userid, u.username, u.avatar_url FROM statuses AS s LEFT JOIN users AS u ON s.userid = u.id ORDER BY s.statusid DESC" Get rid of the second while loop, and run everything in the first. Don't forget to point your $row2 array back to $row.
-
<?php $con = mysql_connect("test","test","test"); // $con = mysql_connect("localhost","root",""); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("test", $con); // mysql_select_db("test", $con); //need sanitation and validation on these varaibles. $id = (!empty($_POST['id'])) ? (int)$_POST['id'] : NULL; $demo = (!empty($_POST['demo']) && $_POST['demo'] == 'N') ? 'N' : 'Y'; //limit is out of place for a pull from the database. $limit = 1; //set a default for limit, so that it will not EVER be empty. $limit = $row["unique_recp"]; //limit is not even required in this script, unless email_list has more than 1 email per user id. } if(empty($id)) { //exit the script if ID isn't set. exit('There is no ID to query'); } $res = mysql_query("SELECT emails FROM email_list WHERE custid = '$id' and demo = 'N' LIMIT $limit"); while ($row = mysql_fetch_array($res)) { echo "<div id=\"left\">".$row['emails']."</div><div id=\"clr\"></div>"; } //mysql_query($res); //You already performed this query, you cannot query a resource. $res2 = "update email_list set demo = 'Y' WHERE custid = '$id' LIMIT $limit"; //not sure you want to limit an update. mysql_query($res2); mysql_close($con);
-
how to show the option based on the value in the table?
jcbones replied to tayys's topic in PHP Coding Help
array_keys just returns the keys of the array that is passed to it. This is done so that we can find what items were sent in the post field. -
oop classes & methods - why can i echo some and not others?
jcbones replied to ricky spires's topic in PHP Coding Help
I think you are getting a little confused on the way Objects work, and work together. I would like to point you to a tutorial here, Hopefully, this will answer all of your questions. Remember that __construct() is called whenever an object is instantiated. Using a function by any other name would mean it doesn't get called, unless you specifically call it. -
Have you tried a scalar subquery? SELECT * FROM Customers WHERE CID = (SELECT DISTINCT(CID) FROM Reports LIMIT 1) ORDER BY Company
-
Good job, page is validating.
-
Try this: <?php function csv_file_to_mysql_table($source_file, $target_table, $max_line_length=10000) { if (($handle = fopen($source_file, "r")) !== FALSE) { $columns = fgetcsv($handle, $max_line_length, ","); foreach ($columns as &$column) { $column = str_replace(".","",$column); } while (($data = fgetcsv($handle, $max_line_length, ",")) !== FALSE) { while(count($data) < count($columns)) { array_push($data, NULL); } $c = count($data); for($i = 0; $i < $c; $i++) { $data[$i] = "'{$data[$i]}'"; } $sql[] = '(' . implode(',',$data) . ')'; } fclose($handle); $query = "INSERT INTO $target_table (".implode(",",$columns).")VALUES " . implode(',',$sql) . "\n"; mysql_query($query) or trigger_error(mysql_error()); } } $file = 'test.csv'; //path name to your CSV file; $table = 'test'; //name of your database table. **NOTE *** column names must match EXACTLY. csv_file_to_mysql_table($file,$table); //calling the function. ?> If nothing shows up in your database table, let me know. This should work if the file is less than ~1,000 rows.
-
oop classes & methods - why can i echo some and not others?
jcbones replied to ricky spires's topic in PHP Coding Help
<?php class Bear { // define properties protected $name; public $weight=100; public $age= 0; public $sex = "Male"; public $colour= "Brown"; public $type = 'Brown Bear'; // constructor function __construct($name) { $this->name = $name; } // define methods public function eat($units) { echo $this->name." is eating ".$units." units of food... <br/>"; $this->weight += $units; } public function run() { echo $this->name." is running... <br/>"; } public function kill() { echo $this->name." is killing prey... <br/>"; } public function sleep() { echo $this->name." is sleeping... <br/>"; $this->age++; } public function description() { $output = $this->name . ' is a ' . $this->type . ', with a beautiful ' . $this->colour . ' coat.'; $output .= ($this->sex == 'Male') ? ' He ' : ' She '; $output .= 'is ' . $this->age . ' days old, and weighs in at ' . $this->weight . ' lbs.<br />'; return $output; } } class PolarBear extends Bear { //if we make a constructor here, we will need to call the parent constructor if //we want to set the name of the bear. we of course could set it using $this, but //that would defeat the purpose of showing this. function __construct($name) { parent::__construct($name); $this->colour = 'White'; $this->type = 'Polar Bear'; } // define methods public function swim() { echo $this->name." is swimming... "; } } $bear = new Bear('Winney the Pooh'); //calling just the bear class. $polar = new PolarBear('Snowman'); //calling the polar bear extension $polar->eat(100); $bear->eat(10); $bear->sleep(); echo '<hr />' . $bear->description() . '<hr />' . $polar->description(); //using a foreach loop to look at the variables of the Polar Bear. //you will notice that the name is not in there, that is because //the name variable is protected. Changing it to public will make it appear here. echo 'The polar bear is:'; foreach($polar as $var => $value) { echo $var . ' is ' . $value . '<br />'; } -
The problem is that you will have to know the exact CSV format. Is there any way you could give a sample CSV file. This way we could see if the first line contains column names, etc. Given that, I would be glad to help you out.
-
how to show the option based on the value in the table?
jcbones replied to tayys's topic in PHP Coding Help
Sorry, I didn't even pay attention to the form element. Change: <form> To: <form action="" method="post"> -
Post name 'notes' is skewed during actual post
jcbones replied to n1concepts's topic in PHP Coding Help
By using cURL, you are submitting the form, not the user. You have total control over where the user goes after the form submission. -
Well, it sure looks that way! But, the free version will only get you a simple word document.
-
Post name 'notes' is skewed during actual post
jcbones replied to n1concepts's topic in PHP Coding Help
Oh, I was trying to help you by suggesting some simple de-bugging. But, I did as you suggested, and loaded the form. Filled it out and submitted it. I found that it passed this string: contact_title=Big Cahuna&first_name=Jerry&last_name=Bones¬es=Show me the money&submit=submit I would also suggest that it is working on your end. Perhaps your "source" is mis-leading you. -
I understand what you are wanting to do, and have worked up a solution. Please note, that the current database design only allows one parent per child. To correct this, you would need a relational table. Here is what I have currently. <?php include 'config.php'; //database connection. $sql = "SELECT * FROM `table`"; //select all rows. $result = mysql_query($sql) or trigger_error(mysql_error()); //complete or error. while($r = mysql_fetch_assoc($result)) { //fetch if($r['Parent'] == 0) { //if the parent is 0. $arr[$r['ID']][0] = $r['Name']; //set them to the first index in the array indexed by their id. } else { $arr[$r['Parent']][1][] = $r['Name']; //children get set to the second index in the parents array. We //made this an array, so that multiple children can reside in it. } } //BELOW is just an example of how to output it. echo '<ol>'; //start an ordered list. foreach($arr as $id => $array) { //itenerate the array. echo '<li>' . $array[0]; //remember the first index is the parent. if(isset($array[1]) && is_array($array[1])) { //if a second index is set, and is an array, there is a child. echo '<ol>'; //start a nested ordered list. foreach($array[1] as $children) { //itenerate through the children array. echo '<li>' . $children . '</li>'; //put them in a line item. } echo '</ol>'; //close the children ordered list. } echo '</li>'; //close the parent line item. } echo '</ol>'; //close the whole ordered list. ?>
-
Post name 'notes' is skewed during actual post
jcbones replied to n1concepts's topic in PHP Coding Help
What does post data actually hold? <?php echo '<pre>' . print_r($_POST,true) . '</pre>'; -
I would second the suggestion of using number_format.
-
how to show the option based on the value in the table?
jcbones replied to tayys's topic in PHP Coding Help
See if this works. Un-tested: <?php require_once '../../connectDB.php'; require_once '../include/functions.php'; if(isset($_POST['change'])) { $itm_keys = array_keys($_POST['change']); foreach($itm_keys as $id) { if(!empty($_POST['cboSub'][$id])) { $sub_id = (int) $_POST['cboSub'][$id]; $sql = "UPDATE tb_item SET sub_id = '$sub_id' WHERE itm_id = '$id'"; mysql_query($sql) or trigger_error($sql . ' has an error: ' . mysql_error()); } } } $sql = " SELECT * FROM tb_item ORDER BY itm_id asc"; $result = mysql_query($sql) or die(mysql_error()); ?> <form> <h1>Items</h1> <table width="75%" border="1" align="left" cellpadding="5" cellspacing="1"> <tr> <th width="100" align="left">Item ID</th> <th width="100" align="left">Parts</th> <th width="100" align="left">Sub-Process</th> <th width="100" align="left">Confirmation</th> </tr> <tr> <?php while ($row = mysql_fetch_array($result)) { extract($row); ?> <td><?php echo $itm_id; ?></td> <td><?php echo $itm_parts; ?></td> <td> <select name='cboSub[<?php echo $itm_id; ?>]' id='cbosub'> <option value='' selected>---- Choose Process ----</option> <?php createComboBox($sub_id); ?> </select> </td> <td> <input type="submit" name="change[<?php echo $itm_id; ?>]" value="Modify" /> </a> </td> </tr> <?php }; ?> </table> </form> <?php /** * Create combo box for sub-process */ function createComboBox($id) { $sql = " SELECT * FROM tb_sub_process ORDER BY sub_id asc"; $result = mysql_query($sql) or die(mysql_error()); while ($row = mysql_fetch_array($result)) { extract($row); $select = ($id == $sub_id) ? 'selected="selected"' : NULL; echo "<option value='$sub_id' $select>$sub_name</option>"; } } ?> -
how to show the option based on the value in the table?
jcbones replied to tayys's topic in PHP Coding Help
What 'earlier page'?