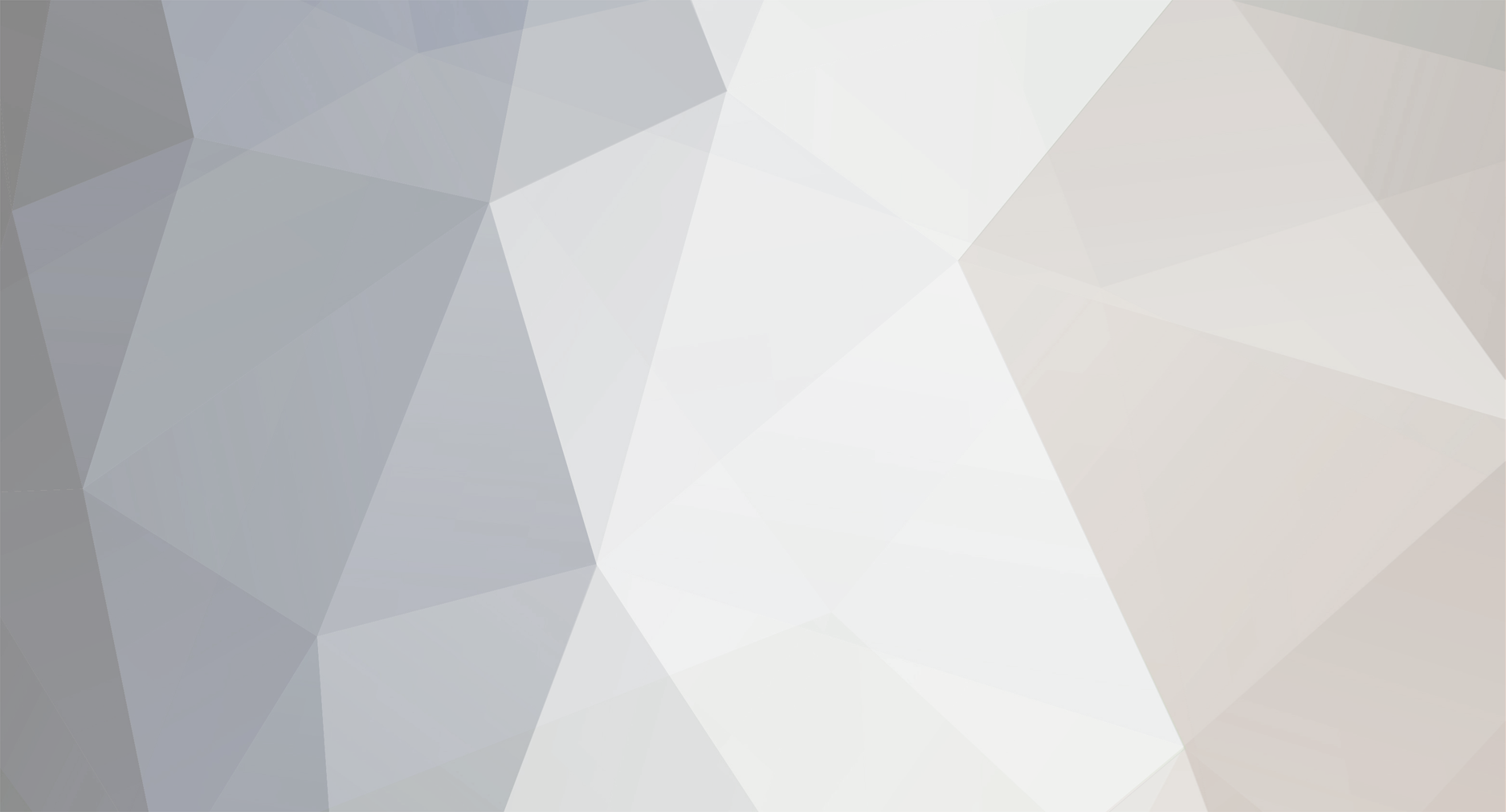
jdavidbakr
Members-
Posts
271 -
Joined
-
Last visited
Everything posted by jdavidbakr
-
You're missing the curly braces around the $_POST['room_id']
-
Need a suggested improvement to the following code...
jdavidbakr replied to loco41211's topic in PHP Coding Help
This should match either single or double quotes. (Note that it will not match single quotes within double quotes and vice versa) preg_match_all("/[\"']([^\"']+)[\"']/", $line, $matches); $pass = $matches[1][0]; return $pass; Inside the []'s it will match any of the characters, inside [^] it will match anything but the characters listed. The []+ will match one or more characters. So this will match either a single or double quote, then any non-zero number of anything except single or double quotes, followed by a single or double quote. The parentheses determine what will be passed to $matches. Technically this will also match a string with a double-quote open and a single-quote close, i.e. 'the string' "the string" "the string' 'the string" but won't match "don't match this" or 'I said, "Do not match this"' -
Something like this? The fields in the form (method=post): <input type="checkbox" name="user[]" value="1"> <input type="checkbox" name="user[]" value="2"> The php code to identify which users were checked: if (is_array($_POST['user'])) { foreach($_POST['user'] as $id) { // $id contains the value of each checked box, process as you wish } }
-
Need a suggested improvement to the following code...
jdavidbakr replied to loco41211's topic in PHP Coding Help
You have identified the line and you just want to extract the value within the quotes, right? preg_match_all("/\"([^\"]+)\"/", $string, $matches); $value = $matches[1][0]; -
Most professional way to create php dynamic website?
jdavidbakr replied to shortysbest's topic in PHP Coding Help
Lots of ways to do it, I don't know that any one is the best. If you want to use query strings, you could do a switch() statement: switch($_GET['page']) { case 'home': include "home.html"; break; default: include "index.html"; break; } Or you could have each .php page contain the same set of includes: <? include "header.html"; // content here include "footer.html"; ?> or any number of other methods. You could go so far as to build classes that handle each page element and have a master class that draws the page. It's really up to you and how you plan to maintain your site's content. -
Need a suggested improvement to the following code...
jdavidbakr replied to loco41211's topic in PHP Coding Help
If including the file isn't a viable option, you might try regular expressions. Check out preg_match or preg_match_all, one of those should give you the tools you need to parse that kind of data. -
What's the output of mysql_error() when the query fails?
-
Select multiple keywords/wildcards from one field
jdavidbakr replied to xuykin's topic in MySQL Help
I'd start by breaking up your query into pieces and gradually adding conditions, i.e. start with the 'plane' and then add 'nitro' and then add the price condition. But you are doing this the hard (and slow) way - you might consider adding some other tables to link against, i.e. Product |-|| Product-To-Attribute ||-| Attribute where Attribute has the attributes you'll want to search against ('plane' and 'nitro') and you'd then run a query joining the product and product-to-attribute tables with the where clause limiting the attribute id. Doing wildcard searches at the beginning of the string eliminates any usage of indexes and therefore requires the db to examine every row; not a big problem if you have a small number of rows, but if you get a large table it could become very slow. -
Your "where" clause isn't doing any comparison. You need something like SELECT * FROM table WHERE date >= now() and date <= date_add(now(), interval 7 day)
-
Try echoing your query instead of executing it - that may lead you to what is happening.
-
If you're likely going to have a varying number of videos for each product, you're definitely better off if you create a separate table and link to the item via a foreign key as you described.
-
$_GET from URL, Logical Operator Problem
jdavidbakr replied to firedealer's topic in PHP Coding Help
So are you getting nothing or are you getting the wrong result? Try adding echo $_GET['time'] to the top to make sure you really are getting passed what you think you're getting passed. You might also try removing the second condition (checking $current_time) to make sure that it is indeed the $_GET condition that's breaking your logic. -
Trying to find a real time ad script rotator
jdavidbakr replied to sfraise's topic in Javascript Help
I'd start by looking for a javascript prototype that makes sense to you - I use MooTools but jQuery has less of a learning curve and lots of folks use that too. That will give you lots of built-in functionality and should be able to do what you're wanting to do very easily, plus will give you lots of tools to do stuff with in the future. -
User 1: "Hi, I want to build myself a house. I bought a lot by a pond and a bunch of wood, pipe, wire, and bricks. Can someone tell me where to start?" User 2: "You might want to hire an architect and a builder." User 1: "Thanks for your answer, too bad it's completely useless. All I want to know is how to build a house."
-
Need help -- working in IE but not Firefox
jdavidbakr replied to melindaSA's topic in PHP Coding Help
You might want to look into a framework to handle your ajax calls (mootools is my favorite but there are others that are probably just as good) - ajaxy stuff can get very complicated when you're dealing with cross-browser compatibility. -
You might also look at boolean mode, which will return more results. Also, the minimum string length (I think, off the top of my head) is 4 characters - so "901" wouldn't ever match in that case. FullText is very cool but it is definitely not as straightforward as other conditions.
-
Run that insert on the other database?
-
I think what you're wanting to do is use aliases select Judge1.name, Judge2.name from TrialClass, person Judge1, person Judge2 where Judge1.ID = TrialClass.Judge1ID and Judge2.ID = TrialClass.Judge2ID Basically that gives you two copies of the "person" table named "Judge1" and "Judge2" that act independently of each other.
-
You're form has method="post" - the variables will show up in $_POST[] instead of $_GET[].
-
Try this: <? if ($_SERVER['SERVER_NAME'] != 'www.example.com') { header("Location: http://www.example.com/"); exit; } ?>