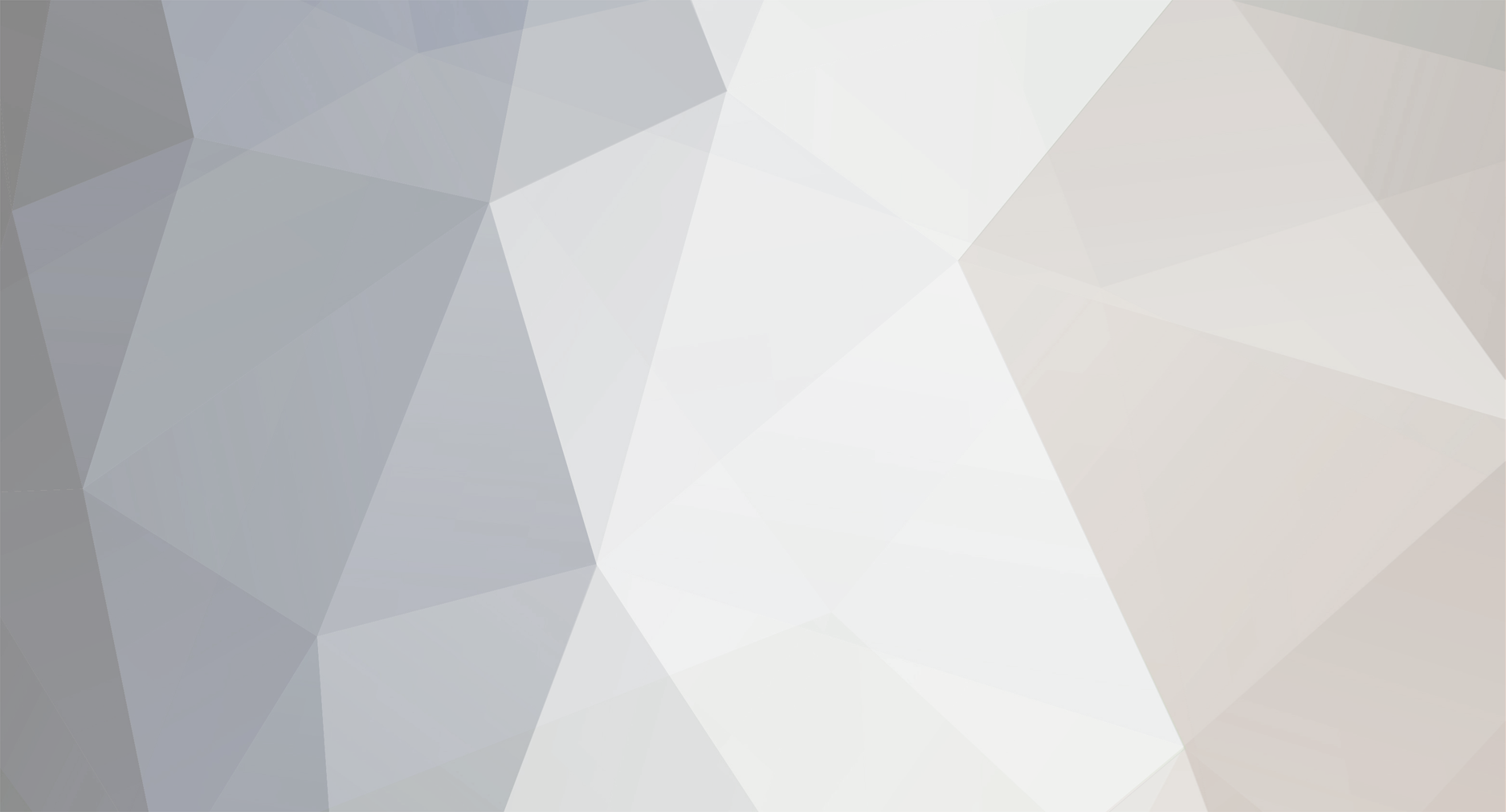
jdavidbakr
Members-
Posts
271 -
Joined
-
Last visited
Everything posted by jdavidbakr
-
If you're wanting our opinion on which publicly available script is best you might point us to what you're looking at, and maybe describe why you think one is better than the other. I apologize if I've come across too strong, I have found that generally there are two types of posters here - those who want to learn how to do something and those who want us to do it for them. I try to be very helpful with the former, but the latter typically receive my ire. When you said "I'm not asking for ideas or code that I can test, but rather for code that has already been tested and found to work correctly at all times." I categorized you into the latter.
-
You've got a number of versions of the form above - what does the form html actually look like right now?
-
Somebody please help me sort out this unlink thing!
jdavidbakr replied to Stevis2002's topic in PHP Coding Help
Change this: $deleteSQL2 = sprintf("SELECT Images FROM testimonials WHERE Testimonial_Id=%s", GetSQLValueString($_GET['del'], "int")); $result = mysql_query($deleteSQL2 , $localhost) or die(mysql_error()); //echo mysql_error(); to this: $deleteSQL2 = sprintf("SELECT Images FROM testimonials WHERE Testimonial_Id=%s", GetSQLValueString($_GET['del'], "int")); echo "<P>".$deleteSQL2; $result = mysql_query($deleteSQL2) or die(mysql_error()); //echo mysql_error(); First, you don't need the $localhost argument to mysql_query (that alone is probably breaking it). If it still doesn't work, look at the query that is printed via the echo command and make sure it's what you're expecting. -
is 'to' the name of your column? It's a reserved word, so you need to enclose it in back-ticks. $user=$_SESSION['username']; $get_messages = mysql_query("SELECT `id` FROM `inbox` WHERE `to`='$user' ORDER BY `id` DESC") or die("Error on line 9 - " . mysql_error());
-
Somebody please help me sort out this unlink thing!
jdavidbakr replied to Stevis2002's topic in PHP Coding Help
That query will find any rows in the Images table that have the Testimonial_Id column ending in the letter 's'. So if your Testimonial_Id is numeric, it will find nothing. If your testimonial_id has values like "Blue","Cars","Dogs", it would find "Cars" and "Dogs." If you're intending to replace %s with a variable, you need to use sprintf - otherwise, your query contains a literal "%s" -
Are you sure this block of code is being executed: // ++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ // 2. performing jqUploader flash upload if ($_FILES['Filedata']['name']) { if (move_uploaded_file ($_FILES['Filedata']['tmp_name'], $uploadFile)) { mysql_query("insert into Profile_Photos (filename) values ('{$uploadFile}')"); // delete the file // @unlink ($uploadFile); return $uploadFile; } try echo mysql_error() immediately after the query. You might also try pulling the query out into a variable and echoing that as well, just to make sure the query is what you expect. I.e. if ($_FILES['Filedata']['name']) { if (move_uploaded_file ($_FILES['Filedata']['tmp_name'], $uploadFile)) { $statement = "insert into Profile_Photos (filename) values ('{$uploadFile}')"; echo "<P>".$statement; mysql_query($statement); echo "<P>".mysql_error(); // delete the file // @unlink ($uploadFile); return $uploadFile; }
-
Somebody please help me sort out this unlink thing!
jdavidbakr replied to Stevis2002's topic in PHP Coding Help
What is the result of the query if you execute it in PHPMyAdmin? SELECT Images FROM testimonials WHERE Testimonial_Id='%s' I'm going to guess that you're needing to set Testimonial_Id to a numeric value instead of '%s' -
Somebody please help me sort out this unlink thing!
jdavidbakr replied to Stevis2002's topic in PHP Coding Help
Your script is ending before you do any deleting - which is probably good at this point, as you have this code in: echo $image_name; die(); Is an image name being echoed? Verify that it's the file you want to delete, then remove the line and the rest of your script should run. -
The fact that no one has given you an answer probably means that we don't know of an existing script that does what you ask. The fact that you're unhappy that instead of giving you a "robust script" we're giving you "ideas" on how to create your own gives off the impression that you are trying to leech your code from people who know how to do it. A good programmer doesn't indiscriminately throw in blocks of code that "work" to create their application. While there are libraries and APIs and functions you can get to speed your development, in general you're better off if you fully understand what every part of code is doing and often times that means writing it yourself.
-
Somebody please help me sort out this unlink thing!
jdavidbakr replied to Stevis2002's topic in PHP Coding Help
You've got an error in your mysql. Add "echo mysql_error()" immediately after your query. You don't need mysql_query twice - just keep the one with $result = ... And your error is probably in the %s - if that's the string you're searching for, you need to enclose it in single quotes. If you're intending to do something with sprintf to populate that as a string, you need to include that function to get your query to be correct. -
Somebody please help me sort out this unlink thing!
jdavidbakr replied to Stevis2002's topic in PHP Coding Help
In your code above: if ((isset($_GET['del'])) && ($_GET['del'] != "")) { unlink($img_dir . $row['Images']); $row is never defined, so $row['Images'] is empty. That's what I was pointing out, you need to define $row from your query result. -
This section of code: if (!isset($visitortitle)) { echo $visitortitle; } is doing absolutely nothing - it's saying "If $visitortitle is nothing then echo nothing" and then continues on. So your code will do exactly the same thing whether visitortitle is selected or not.
-
Somebody please help me sort out this unlink thing!
jdavidbakr replied to Stevis2002's topic in PHP Coding Help
You're just executing the select statement into thin air - you're not capturing the rows that are being selected. $result = mysql_query(...); while($row = mysql_fetch_array($result)) { --- delete $row['Images'] --- } -
A transaction is probably not what you're looking for, assuming you're wanting to do something along the lines of Wikipedia where you can revert to a previous version. Transaction just gives you the opportunity to roll back to where you started, akin to "Revert to saved." Once saved, "Revert to Saved" no longer gets you further back than the previous saved state. If you're using a web-based interface to update the database this pretty much hoses any use of a transaction unless you do something to persist the database connection between http requests. Also not sure what you would do with a stored procedure to accomplish this. Maybe triggers, but you probably are going to be better off just adding the logic to your application code just because triggers can be kind of hairy if you aren't familiar with them. The problem you are presenting is not exactly trivial. The more power you want the rollbacks to have, the more complicated the solution would be. If you want to be able to roll back just sections of the text, you're going to have to have a more complex data structure than if you just need to roll back to a date/time for a blog. If the latter is sufficient, here is what I would probably do: You have your blog table. In the blog table is the primary key, we'll call it blog_id. Have a column in this table that contains the date and time of the most recent update. You have another table called blog_versions. Its primary key could be the blog_id and datetime (or timestamp). Whenever you update the blog table, first copy the existing row into the blog_versions, including the previous update time. Then update the blog table, making sure that you update the timestamp column. Now, you can present the user a list of previous versions and give them the opportunity to choose one (showing the publish time) to override the current version. It actually wouldn't be too difficult to populate the blog_versions with a trigger whenever the blog table is updated. Look up the mysql docs on creating triggers if you feel up to the task.
-
Data from 2 tables, for multiple vehicles but limited by a another field
jdavidbakr replied to BCAV_WEB's topic in MySQL Help
Let's look just at your query logic and ignore the PHP formatting and strip away all the columns that aren't necessary for the logic. Here's a basic situation: two cars, three colors, colors 1 and 2 go to car 1 and colors 2 and 3 go to car 2. Your tables, without all the extra info, would look something like this: Car table: car_id car_name 1 Car 1 2 Car 2 Color table: color_id color_name 1 Red 2 Blue 3 Green Car_to_color table: car_id color_id 1 1 1 2 2 2 2 3 So, basically, Car 1 can be either red or blue, and car 2 can be either blue or green. The primary key for the car_to_color table should be car_id and color_id together. Now, you're looping through the cars: select * from car Each row you are using $car_row with mysql_fetch_array(), so to get the colors for the car whose row you're on: select color.* from color join car_to_color on (car_to_color.color_id = color.color_id) where car_to_color.car_id = '{$car_row['car_id']}' Be sure you understand the logic of how it should work before you try to put it into your code. You might try making a simple page that just lists the car id and its color id's just to simplify the code and give you a chance to make sure you know exactly how it's supposed to work. -
Data from 2 tables, for multiple vehicles but limited by a another field
jdavidbakr replied to BCAV_WEB's topic in MySQL Help
Just add a column in your colors table that is car_id. Or, if you need to have more than one color per car, have a table that is car_to_color with car_id and color_id as the two columns. Then, to get the list of colors for car_id 1 you would either: select * from colors where car_id = 1 or, if you use the second method, select colors.* from colors join car_to_color on (color.id = car_to_color.color_id) where car_id = 1 -
Data from 2 tables, for multiple vehicles but limited by a another field
jdavidbakr replied to BCAV_WEB's topic in MySQL Help
if your tables are MyISAM there is no internal foreign key mechanism - but you can still use the concept, there's just not a way for the database to validate them. if your tables are InnoDB then, in the "Structure" view there is a link for "Relation View" which is where you set up the foreign keys. -
Data from 2 tables, for multiple vehicles but limited by a another field
jdavidbakr replied to BCAV_WEB's topic in MySQL Help
Put a field in your colors table that is a foreign key to the car table - then you can look up just the colors for the car by filtering the color table by the car's ID, and you can do joins between the two tables. -
I'd suggest looking at a calendar application you want to emulate and seeing the fields that they use to control this behavior (in the UI, not necessarily in the database). Like in iCal, you set the frequency of the event and when it ends. When you edit an event it breaks it into two or more events (depending on if you choose 'only this occurrence' or 'all future occurrences'). Then think about how you can structure your tables to accomplish this same data. Obviously the database schema and the logic involved to display the calendar would be more complex than if you just have a single table of events, but it should be a good exercise in building relational tables But, depending on how much control you want to give the user, it could get very complicated very quickly.
-
If you want to order by date you'll need to change the column to DATE. If you sort by that you'll get the order by whatever the sort collation is set to, which is most likely not the order you're looking for. Any reason it's in there as a VARCHAR?
-
Are you asking if you should use your administrator account or connect to the database with a different user in your application? I always create a separate user for each application database - I have dozens of applications on my site, each running on their own database, and each has their own user. I do that so that if one of the users somehow gets compromised they only have access to the single database. Could be overkill but it makes me feel better. I would never put an administrator's password into any PHP connection code, though.
-
So do you know that the query to pull the info is actually pulling a row?
-
Your answer is on line 129, whatever is causing the filename to be /home/bob/public_html/mysite/profiles/write.php instead of what you're expecting it to be.
-
strtotime() takes a string and converts it to a time. It's more flexible than mktime, it actually parses an english string. Check the php manual for strtotime() to see what it's capable of.