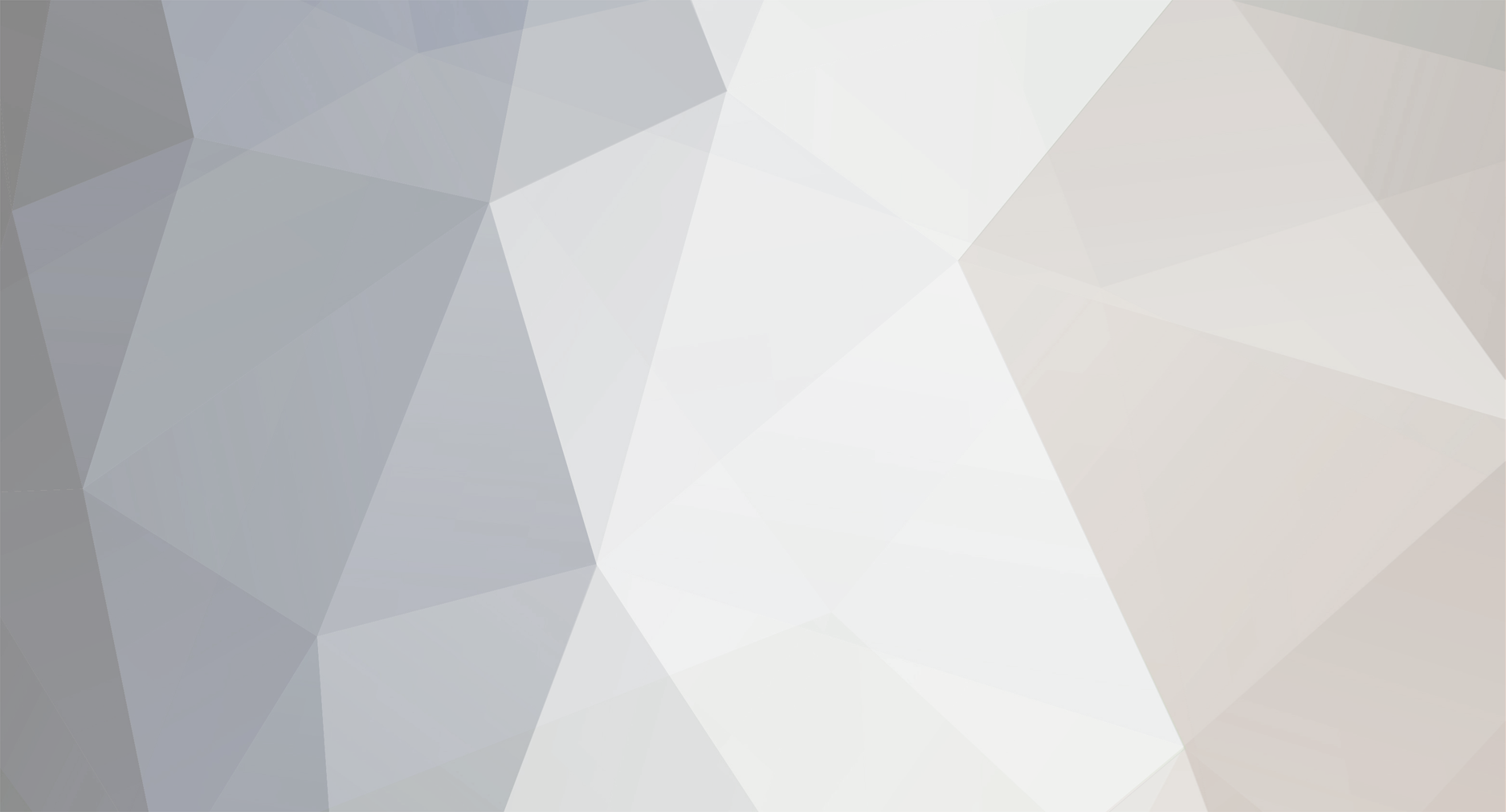
jdavidbakr
Members-
Posts
271 -
Joined
-
Last visited
Everything posted by jdavidbakr
-
check date variable not between 2 dates in database
jdavidbakr replied to dragon_sa's topic in MySQL Help
Are your column types "DATE" or "DATETIME"? If so, it's trivial select * from table where date < '{$startDATE}' or date > '{$finishDATE}'; -
Creating usernames and passwords based on filenames
jdavidbakr replied to richardmilne's topic in MySQL Help
You have the ID/PIN/etc by selecting them from the database; just use those values to construct the filename. <? $filename = $id.'.'.$pin; ?> <img src="[image directory]/<?=$filename?>" alt="Profile Picture"> -
"using password: NO" means that no password was passed to the connect function. Double-check to make sure $password has been correctly initialized. In your sample code you're not initializing any of the connection variables, if you just removed them for security reasons you'll want to make sure you have them set correctly. If the sample code is indeed your entire code, you're not able to connect because you haven't defined any of the connection parameters.
-
Properly Cropping Images without affecting image content...
jdavidbakr replied to iPixel's topic in PHP Coding Help
There are a whole slew of function for handling images. Here's a function I use to resize the image to a max size (the resulting image will be no wider than $max_w and no taller than $max_h) It's pulled out of a class, $this->location is the filename for the image in question. function resize_image($max_w,$max_h) { // This function resizes this image to fit in the box max_w bi max_h $image_size = getimagesize(kImagesDirectory.'/'.$this->location); $ratio = $image_size[0]/$image_size[1]; $goal_ratio = $max_w/$max_h; // Calculate the new image size if ($ratio < $goal_ratio) { // Tall image $height = $max_h; $width = $max_w*$ratio; } else { // Wide image $width = $max_w; $height = $max_h/$ratio; } // Create a new image for the resized image $newimage = imagecreatetruecolor($width,$height); $oldimage = imagecreatefromjpeg(kImagesDirectory.'/'.$this->location); imagecopyresampled($newimage,$oldimage,0,0,0,0,$width,$height,$image_size[0],$image_size[1]); imagejpeg($newimage,kImagesDirectory.'/'.$this->location); } -
that's awesome, ignace. I love it when I discover a new useful function :-) Mundane, probably the easiest thing to do is to store your row in another variable and then operate on that: $title = strip_tags($info['post_title']); Then replace any usage of $info['post_title'] with $title.
-
Are you sure you have a row in all three tables that match all your criteria? Show the echoed query, plus the rows from each table you are expecting the query to deliver.
-
You'll also want to test your back-end code to make sure your queries work correctly too. I think it was when I migrated a database from 4 to 5 that I got hammered with a whole bunch of incorrectly formatted sql statements that were using a list of tables and then a left join, such as: select * from table_1, table_2 left join table_3 on (table_3.id = table_1.id) ... that worked in the old version but not in the new.
-
I think you're on the right track with having a relational table between each user and the articles they like. Using a comma-separated field is most always a no-no; if your table is indexed properly, you can get the count of how many people like an article very quickly and efficiently: select count(*) from liketable where article_id = 1
-
Also you might want to describe what you are expecting the code to do, and what it is actually doing. Not too many people will read through your code to try to figure that out.
-
It'd be a lot easier to store the fields without the HTML and add them when you display them That said, it can be done using regex, but gets scary if you don't have control over the data. This regex will strip anything that starts with "<" and ends with ">": $result = preg_replace("/<[^>]*>/","",$data); but will break if you have something like <p>I just learned that 2 < 3!</p> as your string. There's probably a more robust HTML removing regex, someone may even be able to give it to you ... but I'm going to stick with my original answer which is to avoid putting the HTML into the field in the first place.
-
Why a Parse error: syntax error, unexpected T_STRING
jdavidbakr replied to bbram's topic in PHP Coding Help
. = the concatenate function, it must be between two strings or it will not work. You also need to put the $ before 'rows' - so you're correct syntax should be <? echo $rows['faq_subject'] ?> -
There are a lot of ways you can go about it. Like hamza said, you could show products in the same category. You could build a table that links products with related products, and have a place in the admin where you can link products together (like if you have a DVD player as a product, you could link various cables that would be in a different category but could be useful for the DVD player). Whatever you do, you'll need to come up with how you will define the related products and that will probably get you started with what you'll need to do.
-
No, you check to see if the username exists before you attempt to insert it. Your psudocode should be something like: 1) check to see if the user name exists. If it does, tell the user that the username already exists and require them to choose another one. 2) If the user name doesn't exist, insert it into the database with the 'active' flag set to false. Send the user the e-mail with the link to activate the account. 3) When the user clicks on the link, that page will change the 'active' flag to true. 4) Include a check for the 'active' flag when the user logs in to their account.
-
More or less. Obviously you don't want to execute $sql1 until after you've verified that the username doesn't exist, and $sql2 is just a copy of my code which was just to illustrate how you use 'true' and 'false,' it doesn't do anything; instead, in $sql1 include the varified column and set it to false.
-
Yes, although with MySQL you can use a TINYINT (you can define it as BOOL and it will make it a TINYINT) and use the following, it makes more logical sense than using 1 and 0 to me when you're reading through the code: update user set user_active = true where USER_ID = 1; select * from user where user_active = false; etc. Just don't put quotes around 'true' and 'false'.
-
PLEASE HELP: export csv from php table not working
jdavidbakr replied to dilby's topic in PHP Coding Help
Here's my CSV code, it's from a larger class that I use to draw tables but it should give an idea. There's more going on here than you would need, because the class itself is pretty detailed and specific, but hopefully this will help you start in the right direction. function export_mailmerge($statement,$filename) { // Print the headers $data = array(); $final = ''; foreach($this->header as $header=>$values) { $data[] = '"'.$values['text'].'"'; } $final .= implode(",",$data)."\r\n"; $result = mysql_query($statement); $current = 0; $last = mysql_num_rows($result); while ($current < $last) { $data = array(); foreach($this->header as $header=>$values) { $data[] = '"'.$this->get_data($result, $values, $current, $header).'"'; } $final .= implode(",",$data)."\r\n"; $current ++; } // Send the csv file as an attachment header("Content-type: application/octet-stream"); header("Content-Disposition: attachment; filename=".$filename); header("Pragma: no-cache"); header("Expires: 0"); echo $final; } -
I wouldn't use a temp table, instead have a column "user_verified" that is initially set to false, and is switched to true when they've verified. Then just add a test against all three columns - user name, password, and user verified - instead of just user name and password, when logging the user in.
-
It will do every row that matches the condition in one statement.
-
Retrieving field names and values from database
jdavidbakr replied to PeggyBuckham's topic in MySQL Help
$key should contain the field names in your code. -
PLEASE HELP: export csv from php table not working
jdavidbakr replied to dilby's topic in PHP Coding Help
Any quotes or anything in your csv_output field? That's kind of a strange way to do it, could you not just have a separate script that creates the CSV instead of storing the CSV in the form? If the table is huge you're passing a lot of data back and forth unnecessarily. -
die() doesn't just print an error. It kills the script after it prints the error. Your script isn't passing the die() line. I'm not sure where $_REQUEST['error'] is coming from, it won't be set to 1 if your query fails. But BlueSkyIS is correct anyway, you should check for the username and send the error if it exists rather than intentionally tripping an error.
-
best way to handle a lost username/password
jdavidbakr replied to webguync's topic in PHP Coding Help
Not much to it // Generate a new 7-character password $new_password = substr(md5(uniqid(rand())),0,7); // Get the md5 hash for the database $new_password_hash = md5($new_password); // Insert into the db $statement = "update user set password = '$new_password_hash' where user_id = '$user_id'"; mysql_query($statement); // E-mail password to user mail($user_email,"Your password has been reset","Here is your new password: $new_password"); -
Then don't use "die" because that will stop the script. Do something like this: mysql_query($statement); if (mysql_error()) { echo "That already exists"; }
-
Simple If Then Statement, but it doesn't work
jdavidbakr replied to teisenhood's topic in PHP Coding Help
Yes, putty is a good SSH client. But it doesn't do a whole lot if you don't know your password.