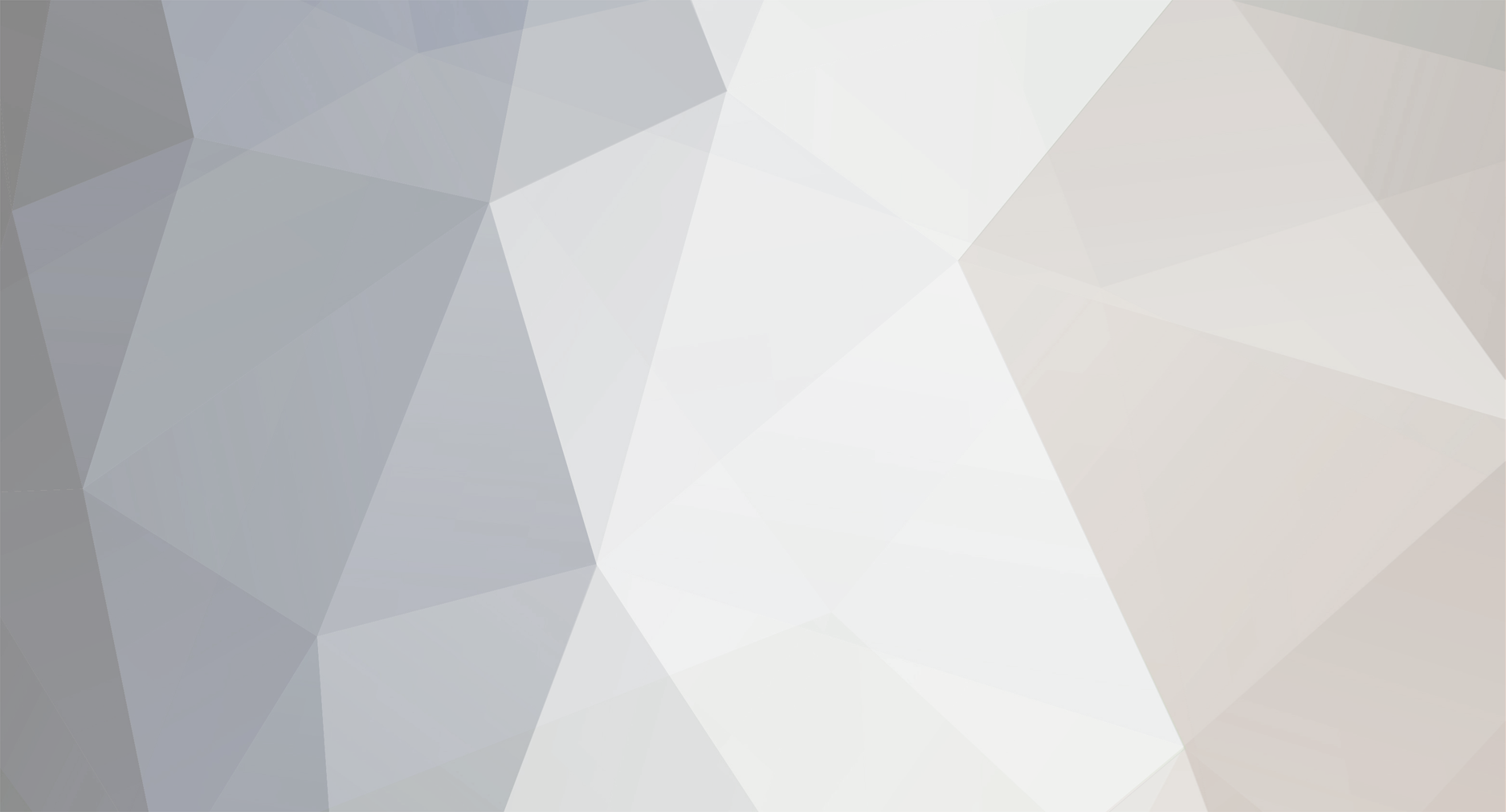
acidpunk
Members-
Posts
45 -
Joined
-
Last visited
Profile Information
-
Gender
Not Telling
acidpunk's Achievements

Newbie (1/5)
0
Reputation
-
Yeah, sorry I mistyped it. But thank you for the response, I actually originally did do exactly what you said, but it did not work.
-
Hey everyone. So i'm trying to json_encode one of my arrays like such: heres an example of how the array is being created (From the array results, everything seems to be fitting OK into the actual array creation) but it's just the actual array creating a multi demension after each result. $num = 1; $num2 = 1; $i = 0; if ($num > 0) { $array[$i][1] = 40; } if ($num2 > 0) { $array[$i][2] = 50; } ++$i; I'm trying to achive this: var array_one_results = new Array(); array_one[1][0] = 40; array_one[1][0] = 50; array_one[2][1] = 40; array_one[2][1] = 50; right now, this is my results: var array_name = new Array(); array_name["0"] = new Array();array_taken["0"]["0"] = "10"; array_taken["0"]["1"] = "20"; array_name["1"] = new Array();array_taken["1"]["0"] = "10"; array_taken["1"]["1"] = "20"; array_name["2"] = new Array();array_taken["2"]["0"] = "10"; array_taken["2"]["1"] = "20"; array_name["3"] = new Array();array_taken["3"]["0"] = "10"; array_taken["3"]["1"] = "20"; array_name["4"] = new Array();array_taken["4"]["0"] = "10"; array_taken["4"]["1"] = "20"; It's creating a new array for each. If anyone could help me through this, i'd really appreciate it. I'm encoding it with this: function js_str($s) { return '"'.addcslashes($s, "\0..\37\"\\").'"'; } function js_array($array, $keys_array) { foreach ($array as $key => $value) { $new_keys_array = $keys_array; $new_keys_array[] = $key; if(is_array($value)) { echo 'array_name'; foreach($new_keys_array as $key) { echo '["'.$key.'"]'; } echo ' = new Array();'; js_array($value, $new_keys_array); } else { echo 'array_taken'; foreach($new_keys_array as $key) { echo '["'.$key.'"]'; } echo ' = '.js_str3($value).";\n"; } } }
-
I read your post and: noones going to hand you everything you're looking for, it ruins the whole purpose of learning, but. i figured i'd shoot you in the right direction, so I whipped up a quick little example of how a basic registration process works. I used "name" and "email" you can problably go from there, by filling in a password, or whatever else you'd wan't. I showed you an example by using rowCount() for checking if a user exists in the database. Lastly, if no errors exist, it gets posted in the database. //connection to the database. $user = 'dbuserhere'; $pass = 'dbpasshere'; $dbh = new PDO('mysql:host=localhost;dbname=dbnamehere', $user, $pass); //creat account. if (isset($_POST['register'])) //triggered from the user clicking on 'Create Account' { if (empty($_POST['name'])) { $errors[] = 'Error: Name may not be empty.'; } elseif(empty($_POST['email'])) { $errors[] = 'Error: E-mail may not be empty.'; } //check if the name being entered already exists in the database or not. $name = htmlentities($_POST['name']); $query = $dbh->prepare('SELECT `name` FROM `users` WHERE `name` = :name'); $query->execute(array( ':name' => $name )); if ($query->rowCount() > 0) { $errors[] = 'Error: The name you entered already exists.'; } //check if there were any errors during the process. if (!empty($errors)) { foreach ($errors as $error) { echo $error; } } else { //no errors, so insert the data. $email = htmlentities($_POST['email']); $query = $dbh->prepare('INSERT INTO `users` (`name`,`email`) VALUES(:name,:email)'); $query->execute(array( ':name' => $name, ':email' => $email )); echo 'Thank you '.$name.' for signing up!'; } } //form for your create account. echo '<form method="POST"> Name: <input required type="text" name="name"><br /> E-mail: <input required type="email" name="email"> <input type="submit" name="register" value="Create Account"> </form>'; ?> The login is basically the same thing as the register page. You have your form, <form method="POST"> Name: <input required type="text" name="name"> Pass: <input reuired type="password" name="pass"> call your form if (isset($_POST['login'])) { // 1. error check // 2. check if the user exists. // 3. if the user exits, fetch the users data. // 4. check the user pass against the form pass. // 5. create your session. } $name = htmlentities($_POST['name']); $query = $dbh->prepare('SELECT `pass`,`id`,`name` FROM `users` WHERE `name` = :name'); $query->execute(array( ':name' = $name )); if ($query->rowCount() > 0) //user exists. else, error. { $row = $query->fetch(); $user_pass = $row['pass']; $form_pass = htmlentities(['pass']); if ($form_pass != $user_pass) { echo 'oops! your passwords do not match!'; } else { //passwords match! $_SESSION['userid'] = $row['id']; //creating your session variable. header('Location: members.php'); } On your members page, just check to see if the $_SESSION is empty or not, if it' empty, direct the user back to the login page, if the session is not empty, stay logged in.. to query the logged user: $query = $dbh->prepare('SELECT * FROM `users` WHERE `id` = :id'); $query->execute(array(':id'=>$_SESSION['userid'])); $user = $query->fetch(); $username = $user['name']; $userid = $user['id']; $email = $user['email']; I got bored, decided to hopefully steer you in the right direction.
-
Loop unsets once, but not the 2nd time around
acidpunk replied to acidpunk's topic in PHP Coding Help
I thought i needed the & operator before $value, but nope. -
So i'm writing a script for a game online, basically it's a clan battle. What happens is, users within the clan attack (each seperatly) at the end of the round, all the users attacks get added up, then subtracts from the boss' health. if the boss isn't dead, the boss attacks. now this is the part that isn't working properly whats supposed to be happening is: after the boss attacks, if any of the users health within the clan attack falls to 0 or below, they get dropped from the array, now this works, but only during the 1st round. foreach ($attackers as $key => $value) { $value['hp'] -= $boss_atk; if ($value['hp'] < 1) { unset($attackers[$key]); } } After it checks if anyones health falls below 0, it than checks if anyones left in the array. If someone is left, then it sets back to the users attack, and redoes the loop. I'm hoping fresh eyes could lend me a hand! Heres the whole script: <?php require 'db.connection.php'; $raidid = 1; $raidover = 0; $attackers = array(); $user_query = $dbh->prepare('SELECT `hp`,`attack`,`criticalhit`,`block`,`username` FROM `stats` WHERE `raidid` = :raidid'); $user_query->execute(array(':raidid'=>$raidid)); $boss_hp = 5000; //testing purposes. $boss_atk = 100; //testing purposes. foreach ($user_query as $row) { $attackers[] = $row; //sets the array for users in attack. } while ($raidover == 0) { /* * clan's turn to attack. * each user within the clan attacks seperatly * they will continue to attack as long as their HP is over 0. */ static $attackTurn = "p"; if($attackTurn === "p") { $totalAttack = 0; foreach ($attackers as $key => $value) { echo $value['username'].' attacks for '.$value['attack'].'<br />'; $totalAttack += $value["attack"]; } } $boss_hp -= $totalAttack; if ($boss_hp < 1) { echo 'Crew has won!'; $raidover = 1; break; } /* * boss' turn to attack. */ $attackTurn = "b"; if($attackTurn === "b") { echo 'Boss attacks for '.$boss_atk.'<br />'; } /* * if any of the users have less than 0 health points * delete them from the loop. */ foreach ($attackers as $key => $value) { $value['hp'] -= $boss_atk; if ($value['hp'] < 1) { unset($attackers[$key]); } } /* * if there arn't any users left in the array * the raid is over, the boss wins. */ if (empty($attackers)) { echo 'Boss has won!'; $raidover = 1; break; } $attackTurn = "p"; //back to the top of the loop. } ?>
-
Help Joining These Queries (A Cron Job That Is Destroying My Site)
acidpunk replied to acidpunk's topic in PHP Coding Help
Honestly what I did was took the whole cron job away.I realized it probably wasn't even needed, and was kind of a worthless script. What I did was add the necessary updates during the time the item was being equipped. So when it was being equipped, the stats +, and when it de-equipped the stats - this brought my server back to life.! I guess case is solved Thank you for the help everyone who tried, psycho thank you for actually trying to show me examples instead of telling me to go read about something lol. My server isn't running 4 CPU'S anymore. anyways for shits and giggles this is how worthless this script is. $userQuery = mysql_query("SELECT * FROM users"); while ($user = mysql_fetch_array($userQuery,MYSQL_ASSOC)) { $item = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Weapon'")); $item11 = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Body'")); $item22 = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Shield'")); $item33 = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Head'")); $item4 = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Belt'")); $item5 = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Foot'")); $item6 = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Pants'")); $item7 = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Ring'")); $item8 = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Neck'")); $itemorb1 = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Orb1'")); $itemorb2 = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Orb2'")); $itemorb3 = mysql_fetch_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Orb3'")); //$jewel = mysql_real_array(mysql_query("select * from user_equipment where equipped='Yes' and owner='$user[id]' and slot='Jewel'")); $level = mysql_query("select * from level"); while ($lvl = mysql_fetch_array($level)){ $fat = $item[attack]+$item11[attack]+$item22[attack]+$item33[attack]+$item4[attack]+$item5[attack]+$item6[attack]+$item7[attack]+$item8[attack]+$itemorb1[attack]+$itemorb2[attack]+$itemorb3[attack]+$lvl[attack]+$user[jewel_attack]; $fhp = $item[hp]+$item11[hp]+$item22[hp]+$item33[hp]+$item4[hp]+$item5[hp]+$item6[hp]+$item7[hp]+$item8[hp]+$itemorb1[hp]+$itemorb2[hp]+$itemorb3[hp]+$lvl[hp]+$jewel[hp]; $fexp = $item[exp_per_hour]+$item11[exp_per_hour]+$item22[exp_per_hour]+$item33[exp_per_hour]+$item4[exp_per_hour]+$item5[exp_per_hour]+$item5[exp_per_hour]+$item8[exp_per_hour]+$itemorb1[exp_per_hour]+$itemorb2[exp_per_hour]+$itemorb3[exp_per_hour]+$lvl[eph]+$user[jewel_eph]; $crit = $item[critical]+$item11[critical]+$item22[critical]+$item33[critical]+$item4[critical]+$item5[critical]+$item6[critical]+$item7[critical]+$item8[critical]+$itemorb1[critical]+$itemorb2[critical]+$itemorb3[critical]+$user[jewel_critical]; $frage = $item[attacks_per_hour]+$item11[attacks_per_hour]+$item22[attacks_per_hour]+$item33[attacks_per_hour]+$item4[attacks_per_hour]+$item5[attacks_per_hour]+$item6[attacks_per_hour]+$item7[attacks_per_hour]+$item8[attacks_per_hour]+$itemorb1[attacks_per_hour]+$itemorb2[attacks_per_hour]+$itemorb3[attacks_per_hour]+$lvl[aph]+$user[jewel_aph]; $fmr = $item[max_attacks]+$item11[max_attacks]+$item22[max_attacks]+$item33[max_attacks]+$item4[max_attacks]+$item5[max_attacks]+$item6[max_attacks]+$item7[max_attacks]+$item8[max_attacks]+$itemorb1[max_attacks]+$itemorb2[max_attacks]+$itemorb3[max_attacks]+$lvl[max_attacks]+$userl[jewel_max]; $cap = $item[attack_cap]+$item11[attack_cap]+$item22[attack_cap]+$item33[attack_cap]+$item4[attack_cap]+$item5[attack_cap]+$item6[attack_cap]+$item7[attack_cap]+$item8[attack_cap]+$itemorb1[attack_cap]+$itemorb2[attack_cap]+$itemorb3[attack_cap]+$lvl[attack_cap]+$user[jewel_cap]; if($user['brewofdesolance'] == 1) { $fat = $fat+50; $fhp = $fhp+100; } if($user[blackmarketEXP] = 0){ $exp = $fexp; } if($user[blackmarketEXP] == 1){ $fexp = $fexp+50; } if($user[blackmarketEXP] == 2){ $fexp = $fexp+100; } if($user[blackmarketFURY] = 1){ $fmr+=250; } if($user[blackmarketFURY] = 2){ $fmr+=250; } if($user[blackmarketFURYCAP] = 1){ $cap+=1; } if($user[blackmarketFURYCAP] = 2){ $cap+=1; } if($user[blackmarketFURYCAP] = 3){ $cap+=1; } if($user[blackmarketFURYCAP] = 4){ $cap+=1; } if($user[blackmarketFURYCAP] = 5){ $cap+=1; } if($user[blackmarketFURYCAP] = 6){ $cap+=1; } if($user[blackmarketFURYCAP] = 7){ $cap+=1; } if($user[blackmarketFURYCAP] = { $cap+=1; } if($user[blackmarketFURYCAP] = 9){ $cap+=1; } if($user[blackmarketFURYCAP] = 10){ $cap+=1; } mysql_query("update users set critical=$crit where id=$user[id]"); mysql_query("update users set exp_per_turn=$fexp where $user[level] = $lvl[id] and id=$user[id]"); mysql_query("update users set attacks_per_turn=$frage where $user[level] = $lvl[id] and id=$user[id]"); mysql_query("update users set attack=$fat where $user[level] = $lvl[id] and id=$user[id]"); mysql_query("update users set hp=$fhp where $user[level] = $lvl[id] and id=$user[id]"); mysql_query("update users set max_attacks=$fmr where $user[level] = $lvl[id] and id=$user[id]"); mysql_query("update users set attacks_per_day_on_a_person=$cap where $user[level] = $lvl[id] and id=$user[id]"); } } echo "updated everyones stats."; ^^^ is the worthless script i was trying to optimize below is what i added when the updates were needed. if($_GET['action']) { //begin unequip functions. if ($_GET['action'] == 'unequip') { $id = (int) $_GET["id"]; $owner = (int) $_GET["owner"]; $userid = $stat["id"]; if ($userid != $owner) { echo "<script>alert(\"Error: You cannot be here!\");</script>"; die(); } $item_query = mysql_query("SELECT name,slot,attack,max_attacks,attack_cap,hp,exp_per_hour,attacks_per_hour FROM user_equipment where id = '".$id."'"); $name = mysql_result($item_query,0,"name"); $slot = mysql_result($item_query,0,"slot"); $attack = mysql_result($item_query,0,"attack"); $max_attacks = mysql_result($item_query,0,"max_attacks"); $attack_cap = mysql_result($item_query,0,"attack_cap"); $hp = mysql_result($item_query,0,"hp"); $eph = mysql_result($item_query,0,"exp_per_hour"); $aph = mysql_result($item_query,0,"attacks_per_hour"); $username = $stat["name"]; echo "<center><br />".$username." you have un-equipped you're ".$slot." ".$name."</center>"; //run the updates (hopefully speed it up.) mysql_query("update users set attack=attack-'$attack' where id = '$owner'"); mysql_query("update users set max_attacks=max_attacks-'$max_attacks' where id = '$owner'"); mysql_query("update users set attacks_per_day_on_a_person=attacks_per_day_on_a_person-'$attack_cap' where id = '$owner'"); mysql_query("update users set hp=hp-'$hp' where id = '$owner'"); mysql_query("update users set exp_per_turn=exp_per_turn-'$eph' where id = '$owner'"); mysql_query("update users set attacks_per_turn=attacks_per_turn-'$aph' where id = '$owner'"); mysql_query("UPDATE user_equipment SET equipped='N' WHERE id = '".$id."' AND owner = '$owner'"); } basically the same as action=equip for action=dequip.. sorry for rambling. ty -
Help Joining These Queries (A Cron Job That Is Destroying My Site)
acidpunk replied to acidpunk's topic in PHP Coding Help
Psyco thank you! And ya, i am using all those fields, i just didnt post that part (its for when i add all them together) so thats how i do a join query. I've been wondering how to do that for some time now, everything i ask how someone tells me to read about it on google lol. I'm going to try this, thank you so much. its weird, the site i'm running worked fine for over a month, and then all of a sudden it started to crash. I've been told its my queries. So i'm trying to fix all of them, and this page i posted is one of the culprits. Let me see if i can implement this. I also realized I really did a terrible job providing information for anyone to help me. :/ lol -
Help Joining These Queries (A Cron Job That Is Destroying My Site)
acidpunk replied to acidpunk's topic in PHP Coding Help
I'm really just adding everything together. user jewel_atttack, jewel_hp, jewel_eph say its attack i'm adding equipment (weapon,shield, ect) + user jewel_attack + level_attack, then i'd add all the HP'S together. Then the final resullt, update the users -
Help Joining These Queries (A Cron Job That Is Destroying My Site)
acidpunk replied to acidpunk's topic in PHP Coding Help
The user table has jewel_attack,jewel_hp,jewel_eph. The level; has attack,hp (for their level) user_equipment has the values stored aswell. What do you want me to post (only reason i didnt post is usually people think i'm just expecting it to be remade) but that isn't the case, just need some guidance on how to optimize this the correct way. if your willing to help, tell me what I need to post. -
Help Joining These Queries (A Cron Job That Is Destroying My Site)
acidpunk replied to acidpunk's topic in PHP Coding Help
Thank you for the replies. Kitchen, yes your right i'm trying to gather all the information about the users 'items' he has, its an RPG game. Basically he has 9 slots (head, weapon, shield ect) And each slot has data like 'attack, hp, critical, ect' Now, what this script does is every 5 minutes check to users equipment, along with his level, and gives him the correct stats based off what equipment he has on + his level. The script i have is completely lagging my site down. I get stuck with a 'website is currently offline' error from cloudflare. I've had my server admin tell me its this script running 100% of 4 CPU'S every 5 minutes. Its a useless script basically and i'm not familiar enough with joining tables to create this script to get faster.I really appreciate the responses. Lol, it sucks this 1 script can cause my whole site to fail. -
Help Joining These Queries (A Cron Job That Is Destroying My Site)
acidpunk replied to acidpunk's topic in PHP Coding Help
I love more puzzles. -
Help Joining These Queries (A Cron Job That Is Destroying My Site)
acidpunk replied to acidpunk's topic in PHP Coding Help
wait, wouldn't it work if i took out the AND slot='weapon' part, and just did mysql_query("SELECT from user_equipment WHERE equipped=Yes and owner=$userid) lol?! it would be basically doing the same exact thing, wouldn't it.. if i'm wrong tell me please, but i think that might be the solution here. -
Help Joining These Queries (A Cron Job That Is Destroying My Site)
acidpunk replied to acidpunk's topic in PHP Coding Help
The only way i know how too. Which is what i'm trying to figure out, there has to be a better solution. -
Help Joining These Queries (A Cron Job That Is Destroying My Site)
acidpunk replied to acidpunk's topic in PHP Coding Help
$item = mysql_fetch_array(mysql_query("select SUM(attack,hp,critical,attacks_per_hour,max_attacks,attack_cap) from user_equipment where equipped='Yes' and owner='$userid' and slot='Weapon'")); $attack = $item['SUM(attack)']; $hp = $item['SUM(hp)']; $critical = $item['SUM(critical)']; $aph = $item['SUM(attacks_per_hour)']; $max = $item['SUM(max_attacks)']; $cap = $item['SUM(attack_cap)']; $item2 = mysql_fetch_array(mysql_query("select SUM(attack,hp,critical,attacks_per_hour,max_attacks,attack_cap) from user_equipment where equipped='Yes' and owner='$userid' and slot='Shield'")); $attack2 = $item2['SUM(attack)']; $hp2 = $item2['SUM(hp)']; $critical2 = $item2['SUM(critical)']; $aph2 = $item2['SUM(attacks_per_hour)']; $max2 = $item2['SUM(max_attacks)']; $cap2 = $item2['SUM(attack_cap)']; $total_attack = $attack+attack2; so this is what I got from what you said to do (i think this is how you meant anyways, i tried.) (so now i have like 10 other queries.) So now, how can the problem with these useless 10 queries. :/ -
Help Joining These Queries (A Cron Job That Is Destroying My Site)
acidpunk replied to acidpunk's topic in PHP Coding Help
I see about the sum. Can i wrap everything inside 1 sum? like sum(attack,hp,critical) ect