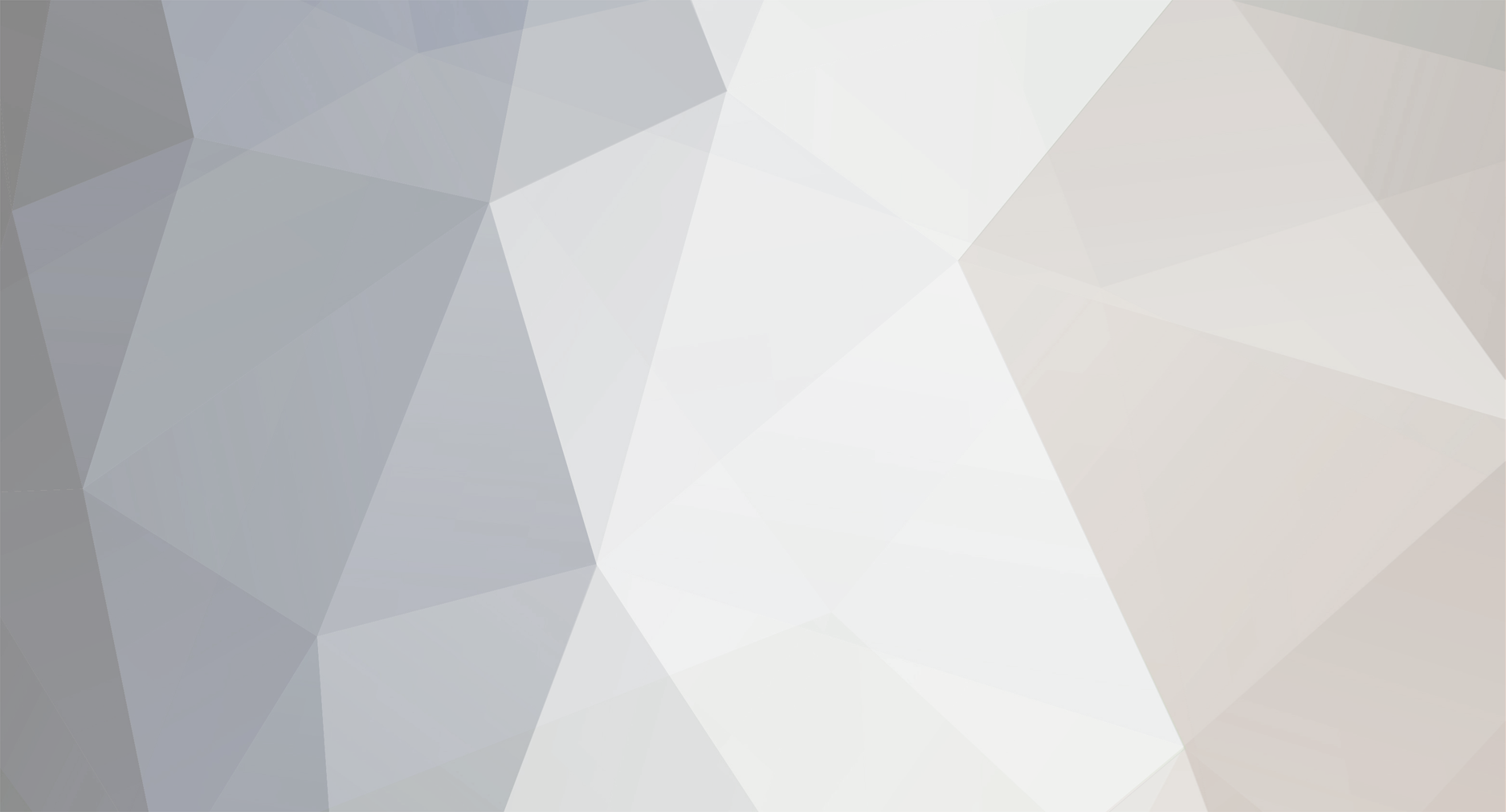
Philip
Staff Alumni-
Posts
4,665 -
Joined
-
Last visited
-
Days Won
20
Everything posted by Philip
-
I don't understand why you're having trouble with that, when the rest of your code does it just fine. echo '<td width="10%" valign="top" bgcolor="'.$bg_color.'"><a href="contact_edit.php?id='.$rows['id'].'">Update</a>';
-
// Technically, you won't need trim() if(trim($_POST['age']) < 18) { echo '{lease Download the parental consent form <a target="_blank" href="www.wokinghambikeathon.co.uk/documents/parentalconsentform.pdf">here</a><br>then click <a href="www.wokinghambikeathon.co.uk/register2.php">here</a> to continue'; // I find it easiest to use single quotes on echo and double quotes in my html. // it requires less escaping }else{ header("Location: http://www.wokinghambikeathon.co.uk/register2.php"); ?> Should be: // Technically, you won't need trim() if(trim($_POST['age']) < 18) { echo 'Please Download the parental consent form <a target="_blank" href="www.wokinghambikeathon.co.uk/documents/parentalconsentform.pdf">here</a><br>then click <a href="www.wokinghambikeathon.co.uk/register2.php">here</a> to continue'; // I find it easiest to use single quotes on echo and double quotes in my html. // it requires less escaping }else{ header("Location: http://www.wokinghambikeathon.co.uk/register2.php"); } // you were missing this! ?> Edit: fixed typo with { instead of P
-
Are you on shared hosting? - If so, you might have used too many resources, and your host shut you off.
-
Of course, this is untested, and I'm sure someone will come up with a better way, but yeah it should you get you started. <?php // put at top: set_time_limit(60); // that will allow for 60 seconds of execution, you can up it if needed // get count of how many rows we'll be dealing with $total_rows = mysql_num_rows(mysql_query("SELECT `isbn` FROM `BOOKS`")); // how many rows per loop execution - you can up this too, probably like a 1000 is a good number $rows_per_loop = 250; // Get the most amount of loops possible. $total_loops = ceil($total_rows/$rows_per_loop); // just make it look nice: echo 'Running rows: <br />'; // run the loop, while loop counter is less than the total number of loops: for($i=0; $i<$total_loops; $i++) { // get the numbers for the limit, // start is just the current loop number multiplied by the rows per loop // and end is loop counter + 1, multiplied by the rows per loop $limit_start = $rows_per_loop*$i; $limit_end = $rows_per_loop*($i+1); // Remember, only call the columns you need, not * -> that'll help a lot $result = mysql_query("SELECT `price`, `isbn` FROM `BOOKS` LIMIT $limit_start, $limit_end") or die(mysql_error()); // now run the while loop while ($myrow = mysql_fetch_assoc($result)) { // ***a side note, I change mysql_fetch_array to assoc since you're calling just the assoc names, not their index #'s. $item = $myrow['isbn']; // get DOM from URL or file $html = file_get_html('http://mysite.com/price.aspx?ItemNo=$item'); // ***how big is this? // find all div tags with id=gbar foreach($html->find('div#price') as $e) $num= $e->innertext; $new_num = preg_replace ('~[^0-9.]~','', $num); $margin_num=$new_num*1.15; // ***IS THIS SUPPOSED TO BE IN THE LOOP TOO? YOU FORGOT THE { } ON FOREACH if($margin_num!=0) mysql_query("UPDATE BOOKS set `price` = '$margin_num' WHERE `isbn` ='$item'", $db); } // end while loop. // now that we've run those, let's clear the results so that we don't run out of memory. mysql_free_result(); // show where we are at echo $limit_start,' - ',$limit_end,'<br />'; sleep(1); // give PHP a little nap to keep from overloading server ob_flush(); // as recommended by MadTechie, in case we go beyond the max execution time flush(); // add flush, to make sure it is outputted } //end for loop echo 'Rows updated!'; ?> A few things I would add in: make sure nobody else can run this script possibly add input where you can start/stop the updating (kinda like what you were trying to do with pagination) - update rows 500-2500
-
I can give you some basic pseudo code: main mysql query get total number of rows free result number of loops = row count/execute in each loop loop [ number of loops-- ] { mysql query with limit(start row, end row) do something with results, while loop free results sleep for a second }
-
Okay, well, another way to do it is.... ini_set("memory_limit","12M"); Increase memory limit or, Break up your query, run it X rows at a time (use mysql LIMIT), free the result, run the next, repeat. edit: of course I'm just throwing 12M as an example
-
Err, I'm sorry - typo: if($i/%250==0) { Change to: if(($i%250)==0) {
-
Good point.
-
<?php class test { public $var1; public $var2; function testlol($var) { $this->var1 = $var; $this->var2 = 'haha'; } } $myClass = new test; $myClass->testlol("hi"); print_r($myClass); ?> Will show:
-
$i=0; while ($myrow = mysql_fetch_array($result)) { ++$i; if($i/%250==0) { echo $i; sleep(1); // give PHP a break, if you have 43,000 rows } $item = $myrow["isbn"]; // get DOM from URL or file $html = file_get_html("http://mysite.com/price.aspx?ItemNo=$item"); // find all div tags with id=gbar foreach($html->find('div#price') as $e) $num= $e->innertext; $new_num = preg_replace ('~[^0-9.]~','', $num); $margin_num=$new_num*1.15; if($margin_num!=0) mysql_query("UPDATE BOOKS set `price` = $margin_num WHERE `isbn` =$item", $db); } That will show when your on rows that are multiples of 250. It'll also give PHP a little break each time, so you don't overload the server. Of course, that number could be higher, like every 1000.
-
In regards to your edit: You don't need to do <?php ?> tags, because heredoc is a type of string and will parse variables automatically. See the example on the php.net page: <?php $str = <<<EOD Example of string spanning multiple lines using heredoc syntax. EOD; /* More complex example, with variables. */ class foo { var $foo; var $bar; function foo() { $this->foo = 'Foo'; $this->bar = array('Bar1', 'Bar2', 'Bar3'); } } $foo = new foo(); $name = 'MyName'; echo <<<EOT My name is "$name". I am printing some $foo->foo. Now, I am printing some {$foo->bar[1]}. This should print a capital 'A': \x41 EOT; ?> Will output:
-
<?php $test = "value"; echo <<<EOF <script language="JavaScript" type="text/JavaScript"> <!-- some javascript --> </script> <form> <input type="text" value="$test" /> </form> EOF; ?> Works just fine - outputs the correct code in the page source
-
You're still missing something, namely in trim() // Technically, you won't need trim() if(trim($_POST['age']) < 18) { echo '{lease Download the parental consent form <a target="_blank" href="www.wokinghambikeathon.co.uk/documents/parentalconsentform.pdf">here</a><br>then click <a href="www.wokinghambikeathon.co.uk/register2.php">here</a> to continue'; // I find it easiest to use single quotes on echo and double quotes in my html. // it requires less escaping }else{ header("Location: http://www.wokinghambikeathon.co.uk/register2.php"); }
-
[SOLVED] Advise: Private PHP forum. Any suggestions?
Philip replied to webmaster1's topic in PHP Coding Help
Hey, I never said it was a GOOD idea....LOL... BTW, I am new to these boards, how the heck do you respond to someone while "quoting" them but being able to access the full REPLY options? Everytime I click on QUOTE, it takes me to a QUICK REPLY and l lose the extra options... What gives? Thanks! Quote -> Preview, or as Mchl said -- @OP: Another option, not as great as relying on the forum to hide itself, but using .htaccess. -
Yeah, you can use array_combine, but it would still be an array, not variables like the OP wanted.
-
'Folk' If you're getting \'Folk\', then you need to use stripslashes()
-
can't get table cells consistent in MySQL data display
Philip replied to kevinritt's topic in PHP Coding Help
See above - I already changed it for you -
can't get table cells consistent in MySQL data display
Philip replied to kevinritt's topic in PHP Coding Help
<table cellspacing="1" class="mainTable"> <tr> <td width="50px" class="display">ID</td> <td width="150px" class="display">Name</td> <td width="150px" class="display">Name (2)</td> <td width="450px" class="display">Address</td> <td width="70px" class="display">Phone</td> <td width="200px" class="display">Email</td> <td width="80px" class="display">Exp Date</td> <td width="80px" class="display">Membership Type</td> <td width="120px" class="display">Child(ren)'s Name(s)</td> <td width="300px" class="display">Interests</td> <td width="80px" class="display">Date added</td> <td width="80px" class="display">added</td> <td width="150px" class="display">Additional</td> </tr> <?php $sql = mysql_query( "SELECT * FROM memberships" ) or die("SELECT Error: ".mysql_error()); // start the while statement to get results from database while ($row = mysql_fetch_array($sql)) { // foreach row in the while loop.. foreach($row as $key=>$value){ // convert the long winded $row[''] to simple variable // also cleaning the data of slashes for security ValidateOutput() (look at clean.php) $$key = ValidateOutput($value); } // display data in an HTML table for viewing echo ' <tr> <td class="display">'.$memberID.'</td> <td class="display">'.$firstName.' '.' '.$lastName.'</td> <td class="display">'.$firstName2.' '.' '.$lastName2.'</td> <td class="display">'.$address.' '.' '.$city.' '.' '.$state.' '.' '.$zip.'</td> <td class="display">'.$telephone.'</td> <td class="display">'.$email.'</td> <td class="display">'.$expiration.'</td> <td class="display">'.$type.'</td> <td class="display">'.$childName.'</td> <td class="display">'.$interests.'</td> <td class="display">'.$dateAdded.'</td> <td class="display">'.$added.'</td> <td class="display">'.$additionalNotes.'</td> </tr> '; } ?> </table> Use rows (<tr>) instead of new tables for each mysql row -
I'm confused on what you mean. Adding: <?php if(!defined(THEME_PATH)) define(THEME_PATH, ''); ?>/ at the top of the theme pages, would allow for you to view the page on its own without the Themes/default
-
Granted, this would only work if you didn't use keys in your arrays: <?php $array1 = array("car","truck","bike"); $array2 = array("first","second","third"); foreach($array1 as $key => $value) { $$value = $array2[$key]; echo $$value,' => ',$value,'<br />'; // show what the values are } ?> Would print:
-
So, in the second code: <?php if(!defined(THEME_PATH)) define(THEME_PATH, ''); ?> <html> <head> <!-- CSS Stylesheet --> <link rel="stylesheet" type="text/css" href="style.css" /> <!-- / CSS Stylesheet --> <title><?php echo $pagetitle; ?></title> </head> <body> <div class="id1"> <img src="<?php echo THEME_PATH; ?>images/header.png"> <?php loopecho("1","45"); ?> </div> </body>
-
Something like this? <?php $pagename = "Homepage"; define(THEME_PATH,'Themes/default/'); function loopecho($start,$finish); { while($start <= $finish) { echo $start; $start++; } } include(THEME_PATH.'index.php'); ?> <html> <head> <!-- CSS Stylesheet --> <link rel="stylesheet" type="text/css" href="style.css" /> <!-- / CSS Stylesheet --> <title><?php echo $pagetitle; ?></title> </head> <body> <div class="id1"> <img src="<?php echo THEME_PATH; ?>images/header.png"> <?php loopecho("1","45"); ?> </div> </body>
-
Additionally, make sure to set $i equal to 1 or 0 to begin with - somewhere above your while loop. P.S. - don't forget to mark as solved
-
[SOLVED] Checking if Username Already Exists in the DB
Philip replied to Akenatehm's topic in PHP Coding Help
You're checking if it's greater than one row, not equal to: if(mysql_num_rows($checkuser) > '1') should be: if(mysql_num_rows($checkuser) > 0) -
[SOLVED] How do I make my site stretch to each side?
Philip replied to Akskater1000's topic in PHP Coding Help
http://w3schools.com/css/default.asp Read up