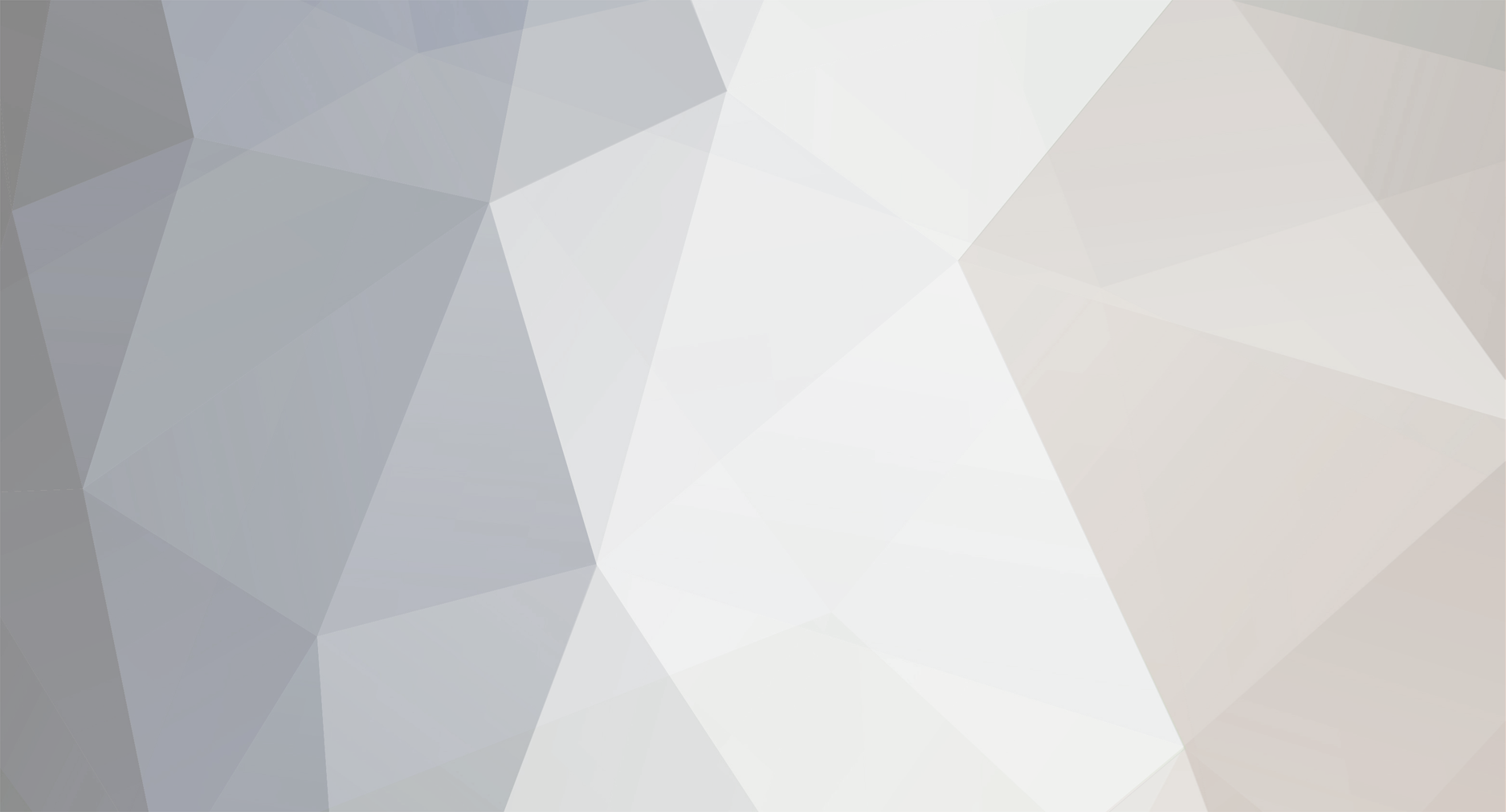
Philip
Staff Alumni-
Posts
4,665 -
Joined
-
Last visited
-
Days Won
20
Everything posted by Philip
-
Must be a sign the world is soon to collapse.
-
[SOLVED] image not appearing in ie, but does in firefox
Philip replied to harlequeen's topic in PHP Coding Help
No, it's not because you can't size images, but because the way you have your quotes: echo '<center><img src="http://www.morganite-netball-club.co.uk/images/playerpics/' . $row['playerimg'] . '" height=\"280\" width=\"400\"></center><br/>'; You're trying to escape a double quote, when you're echoing with single quotes. echo '<center><img src="http://www.morganite-netball-club.co.uk/images/playerpics/' . $row['playerimg'] . '" height="280" width="400"></center><br/>'; If you had done the following, you would have needed the escaping (as shown) : echo "<center><img src=\"http://www.morganite-netball-club.co.uk/images/playerpics/" . $row['playerimg'] . "\" height=\"280\" width=\"400\"></center><br/>"; -
$result = mysql_query("SELECT `email` FROM `members` WHERE `email`='{$email}' LIMIT 1"); if($mysql_num_rows($result)>0) { // you already have this user in the database } else { // You don't and should put an INSERT query here. } Of course, thats just an example.
-
Say what!? When he was calling $$a it was looking for $Hello, not $hello.
-
You're calling $$a, which looks for the variable with the value of $a or - $Hello. You obviously haven't set it up yet..... You could do: $a = 'Hello'; $hello = 'Hello everyone'; // ... $$a = 'Something'; echo $hello.'<br />'; echo $aa.'<br />';
-
For the easier way, take a look at the PHP class: http://www.phpclasses.org/browse/package/4150.html Otherwise look at their API: http://twiki.cpanel.net/twiki/bin/view/AllDocumentation/AutomationIntegration/XmlApi
-
@SetToLoki - if you're talking to me you can use commas instead of dots. Echo allows for multiple parameters to be passed, so PHP won't have to concatenate the strings before outputting them... it just outputs the strings. @Ken - see above, and I do believe it is slightly (very very slightly) faster, just because it does not have to concatenate the strings first. Again, this is the same as the ' vs " argument. Speed wise, it is practically the same. @Doctor - as SetToLoki said, look into javascript's window open command: http://www.pageresource.com/jscript/jwinopen.htm
-
You need to have quotes around the string: echo "<a href='http://en.utrace.de/?query={$result->fields['log_ip']}'>{$result->fields['log_ip']}</a>"; or echo '<a href="http://en.utrace.de/query=',$result->fields['log_ip'],'>',$result->fields['log_ip'],'</a>';
-
Or using one query: SELECT COUNT(*) FROM `table` GROUP BY `group`
-
Well that's one way to get the PTA off your back. If i was him i would do it. It wouldnt surprise me if someone found him a few years down the track.....as a woman That was my first thought actually.
-
Ehh, theres a few things about this I'll throw my $0.02 into. You're going to be querying the database a lot. No big deal - as long as you create a clean query. I mean, seriously, how do you think forums handle the load? At the bottom of this page: Anyways. I think after 15 minutes, the user should be pulled from the list - typically its about 5 minutes before they are considered inactive. Here's what I would do: Use a INSERT... ON DUPLICATE KEY UPDATE... for your query. Use their user ID as the primary key, and a timestamp. On your online list, have in your WHERE clause something like timestamp > date_sub(NOW(), INTERVAL 15 MINUTE) Don't use persistent connections
-
Like Ken said, you could use a static method, but it wouldn't be as portable as using global or as a parameter. (mainly because if you change your class name, the method, etc, you'd have to go into each function and change that rather than just one variable)
-
Globals is not the best way to go about this, but instead you should pass the variable by argument into the function: function fooBar($connection) { $result = $connection->query("SELECT `name` FROM `users`"); // ... more stuff here } $my = new db_class("192.168.1.2", "root", "password", "db"); fooBar($my);
-
Typically you would do it manually - at least I would. But remember, after you go through and check everything once or maybe even twice, then open it up to a group of users to 'beta' test it. You could automate the process, yes, but that would seem like more trouble than it is worth.
-
Kinda. Typically a class will be an organized structure of methods (functions), properties (variables), and other objects to perform a specific task or a range of tasks. I added a bit to the Guest class: <?php // Our Guest Class: class Guest { // Setup the properties of the class: public $firstName, $lastName, $carColor, $carMake, $age, $cups, $bags; // Add what happens when you create a new guest public function __construct($fn,$ln,$cc,$cm,$a) { // Set their properties $this->firstName = $fn; // Get this from the passed arguments $this->lastName = $ln; // Get this from the passed arguments $this->carColor = $cc; // Get this from the passed arguments $this->carMake = $cm; // Get this from the passed arguments $this->age = $a; // Get this from the passed arguments $this->cups = 0; // Set this to 0 as default $this->bags = 0; // Set this to 0 as default } // What if our guests get thirsty? public function drankSoda($cups) { // We should keep track of how many sodas they have $this->cups = $this->cups + $cups; } // But then they might get hungry too! public function ateChips($bags) { // So let's keep tally of who eats the most at my party $this->bags = $this->bags + $bags; } } // We normally would create an object like this: $Phil = new Guest('Phil', 'IsCool', 'green', 'Mini', 19); // Then, we could say I drank 2 cups of soda: $Phil->drankSoda(2); // I then got super hungry and had 5 bags of chips: $Phil->ateChips(5); // Finally, I had to wash those chips down and had one more cup of soda: $Phil->drankSoda(1); // In the end, let's see how I did: echo $Phil->firstName.' '.$Phil->lastName.' drove up in a '.$Phil->carColor.' '.$Phil->carMake.' and had '.$Phil->cups.' cups of soda and '.$Phil->bags.' bags of chips!'; // That would show on screen: // Phil IsCool drove up in a green Mini and had 3 cups of soda and 5 bags of chips. ?> Maybe that will make more sense?
-
An API is an application programming interface. http://en.wikipedia.org/wiki/API
-
I call fake. I doubt somebody like tigerdirect or godaddy would really be dumb enough to do that
-
Can you see your error once you put it inside code tags w/highlighting? <?php $curnum = 0; $username = $_POST['username']; $password = $_POST['password']; $gender = $_POST['gender']; $byear = $_POST['birth_year']; if(!$username) { $curnum ++; echo "<tr><td>".$curnum.". Please enter a username.</td></tr> } ?> (the missing "; at the end of the echo statement) PS - next time use code tags please
-
I think its similar to this concept (you pay for a chunk of pixel grid space to place your company's logo for instance). Sorry to the mods/admin, not trying to 'advertise' that link's service or anything.. just for illustrative purposes. Haha, just don't do it as bad as them:
-
[SOLVED] Revenue idea for phpfreaks (The first one)
Philip replied to dreamwest's topic in PHPFreaks.com Website Feedback
... -
wow people still go for that sort of thing? Second that - I thought that was a 90's thing?
-
[SOLVED] Revenue idea for phpfreaks (The first one)
Philip replied to dreamwest's topic in PHPFreaks.com Website Feedback
You probably think you're the pornstar profile right? -
http://www.phpfreaks.com/forums/index.php?action=stats Bottom of the page will show hits and general stats.
-
Because thats javascript not php??