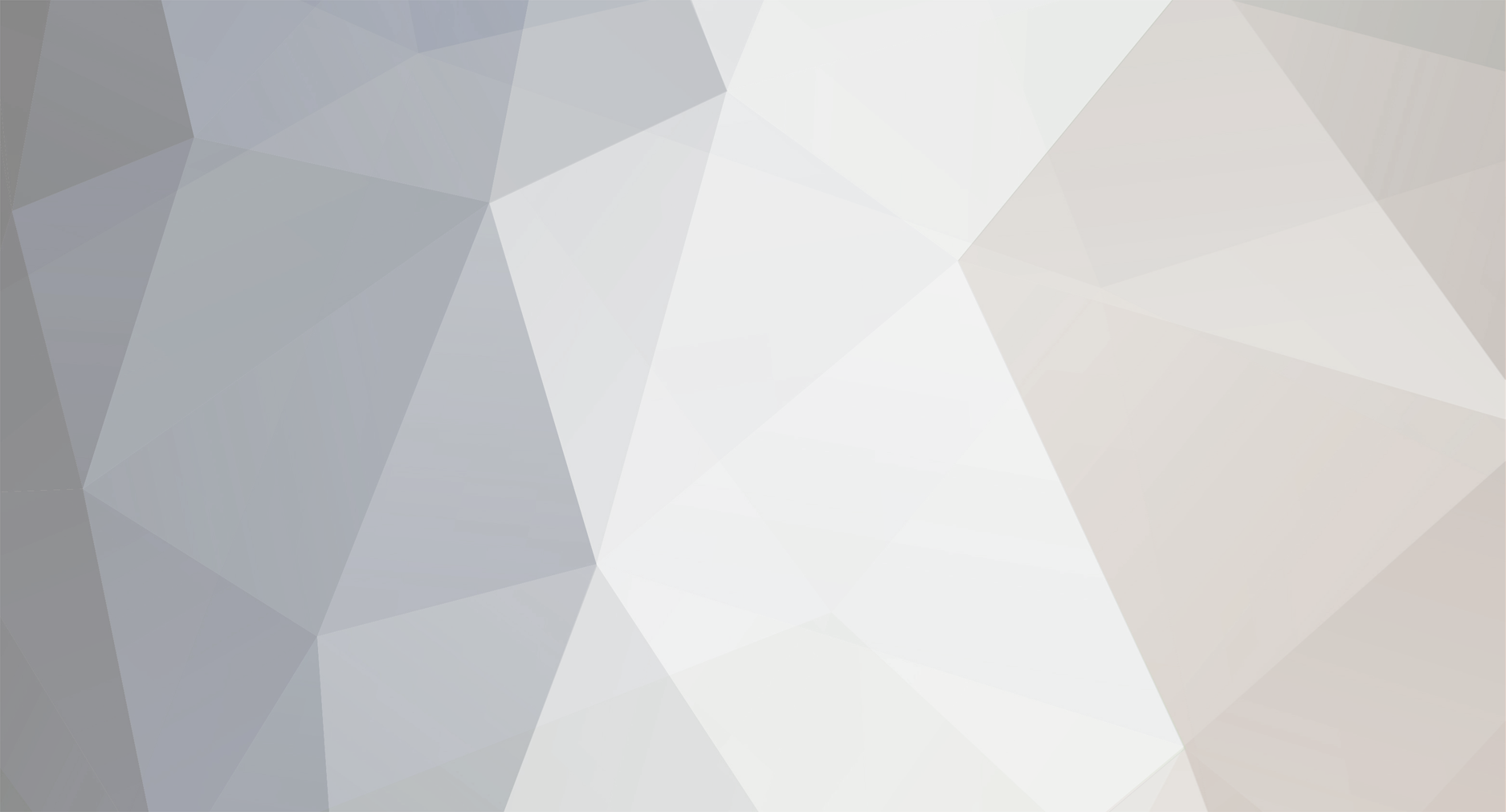
Philip
Staff Alumni-
Posts
4,665 -
Joined
-
Last visited
-
Days Won
20
Everything posted by Philip
-
SOmehow I disagree with that point of view... Mostly agreed, while it might be "a bunch of methods (functions) and variables put in a single place" there should be some organization/meaning behind why those functions/variables are there. Not everything needs to be in OOP format, I've seen quite a few good rules of when to use OOP... I'll quote some from one list: When your design is large, or is likely to become large. When types of data form a natural hierarchy that lets us use inheritance. When the system design is already object-oriented. When implementation of components is likely to change, especially in the same program. When huge numbers of clients use the same code When you have a piece of data on which many different operations are applied When the kinds of operations have standard names (check, process, etc).
-
You're going to want to use GET. This is a very basic example of what you want: <?php // Use get to retrieve the value of a variable in a url. // pagename.php?variable=value $pageID = $_GET['pageID']; // Now you can use the value ($pageID) in that script echo '<div class="page" id="post-',$pageID,'">'; // That'll show: // <div class="page" id="post-2"> // If you want to include a file, based on the page, I'd recommend checking it against an array // since the GET variables can be easily modified. ?>
-
[SOLVED] FTP site problem after upload. [ PHP and MYSQL base lang ]
Philip replied to tayhaithian's topic in PHP Coding Help
Make sure that mysql user has the correct privileges (permissions). You can check this with your hosting account's site admin typically, or via command line... see here about it: http://www.iis-aid.com/articles/how_to_guides/assigning_mysql_privileges_via_the_command_line -
HTML-Kit is pretty good, it's what I use. Take a look at this thread: http://www.phpfreaks.com/forums/index.php/topic,54859.0.html It has a list of editors, people have voted, etc.
-
Put this at the top: printf("%s\n", $_GET['dat']); What does that output?
-
Session value failed to retrieve from another page .
Philip replied to tayhaithian's topic in PHP Coding Help
Change your first script to: <?php session_start(); ?> <html> <head> </head> <body> <br /><br /><br /> <center><h1> Please choose only the available left Tables . Sorry for any inconvenience . </h1></center> <?php if(isset($_POST['Submit'])) { $_SESSION['table_id']=$_GET['table_id']; //print_r ($_SESSION['table_id']); header ('location:special.php'); } include('conn.php'); $data=mysql_query("SELECT table_id FROM restab WHERE NOT EXISTS ( SELECT table_id FROM payments WHERE STATUS='pending' AND restab.table_id = payments.table_id )") or die(mysql_error()); echo '<center>'; echo '<form method="POST" action="table.php">'; echo '<select name="table_id">'; for($i=0;$i<mysql_num_rows($data);$i++) { $row=mysql_fetch_assoc($data); echo '<option value="'.$row['table_id'].'">'.$row['table_id'].'</option>'; } echo '</select>'; echo '<br /><br /><input type="submit" name="Submit" value="GO ==>>"/>'; echo '</form>'; echo '</center>'; ?> </body> </html> I'm honestly surprised you're not getting any errors -
Okay, there are a few things that are wrong here <?php $to = "julie.birdsong@gmail.com"; $from_header = "From: $from"; if($contents != "") { //send mail - $textfield come from surfer input mail($to, $textfield, $from_header); // redirect back to url visitor came from header("Location: $HTTP_REFERER"); } else { print("<HTML><BODY>Error, no comments were submitted!"); print("</BODY></HTML>"); } ?> Where is the $from defined in: $from_header = "From: $from"; And the $textfield needs to be $_POST['textfield'] since you're getting it from the form. So: <?php // Always be sure to use long tags (<?php, not <?) // Setup basic info $to = "julie.birdsong@gmail.com"; $from_header = "From: $from"; // Where is this coming from? // Check contents, again where is variable that coming from? if($contents != "") { //send mail - $textfield come from surfer input // dont forget $_POST mail($to, $_POST['textfield'], $from_header); // redirect back to url visitor came from // This isn't the most secure way, but that's okay for now. header("Location: $HTTP_REFERER"); } else { print("<HTML><BODY>Error, no comments were submitted!"); print("</BODY></HTML>"); } ?>
-
If you're wanting to delete the actual file, take a look at unlink()
-
Take a look at: http://www.tizag.com/mysqlTutorial/mysqldelete.php Syntax is "DELETE FROM `tablename` WHERE `column`='value' "
-
$q1 = mysql_query("SELECT * FROM Affiliates WHERE AffiliateID = '".$_POST['deleteuser']."' "«»); You have the ' and the " mixed up, try this: $q1 = mysql_query("SELECT * FROM Affiliates WHERE AffiliateID = '".$_POST['deleteuser'].'");
-
Mixing GET/POST are we? I don't really understand why you're trying to use GET variables when you have the same variables in hidden POST fields.
-
[SOLVED] Using PHP to highlight the current page in a list
Philip replied to biwerw's topic in PHP Coding Help
Change it to this: <!--BODY--> <div id="layout-body"> <!--NAVIGATION--> <?php // Simplify the variable name $pageID = $_GET['id']; // Define our array of allowed $_GET values $pass = array( 'page1' => 'Page 1', 'page2' => 'Page 2', 'page3' => 'Page 3', ); // Include the navigation file include ("navigation.php"); ?> <!--CONTENT--> <div id="content"> <?php // If the page is allowed, include it: if(array_key_exists($pageID, $pass)) { include_once('work/' . $pageID . '.php'); } // If there is no $_GET['id'] defined, then serve the homepage: elseif (!isset($pageID)) { include ('home.php'); } // If the page is not allowed, send them to an error page: else { // This send the 404 header header("HTTP/1.0 404 Not Found"); // This includes the error page include ('error.php'); } ?> </div><!--content end--> </div><!--layout-body end--> -
[SOLVED] Using PHP to highlight the current page in a list
Philip replied to biwerw's topic in PHP Coding Help
Mind posting code from index.php? I'm thinking that you're defining $pass after you've included the navigation file. -
echo '<td align="left"><a href="mailto:' . $row['email'] . '">'.$row['email'].'</a></td>';
-
[SOLVED] Using PHP to highlight the current page in a list
Philip replied to biwerw's topic in PHP Coding Help
I just tested it on my server, and the code works. Mind posting more code? -
[SOLVED] Using PHP to highlight the current page in a list
Philip replied to biwerw's topic in PHP Coding Help
Okay, that's odd. Maybe I'm misunderstanding the question. <ul class="nav"> <?php // For all of the array items, foreach($pass as $key => $value) { // Show the basic structure echo '<li><a href="index.php?id=',$key,'" title="',$value,'"'; // And if it is the current page (if the GET var is the same as the one in the loop) // We should show id=current. if($pageID == $key) echo ' id="current"'; // Finish showing basic structure echo '>',$value,'</a></li>'; } ?> </ul> That code is on the included page (page1, page2, etc), correct? -
[SOLVED] Using PHP to highlight the current page in a list
Philip replied to biwerw's topic in PHP Coding Help
<ul class="nav"> <?php print_r($pass); // For all of the array items, /*foreach($pass as $key => $value) { // Show the basic structure echo '<li><a href="index.php?id=',$key,'" title="',$value,'"'; // And if it is the current page (if the GET var is the same as the one in the loop) // We should show id=current. if($pageID == $key) echo ' id="current"'; // Finish showing basic structure echo '>',$value,'</a></li>'; }*/ ?> </ul> What does that output? -
[SOLVED] If-else statement using isset creates blank output
Philip replied to yotamono's topic in PHP Coding Help
<?php echo $_GET['s']; if (isset($_GET['s'])) { ?> <li class="selected"><a href="<?php the_permalink(); ?>">special page</a></li> <?php } ?> <?php elseif (isset($_GET['show'])) { ?> <li><a href="<?php the_permalink(); ?>">article</a></li> <li class="selected"><a href="?show=comments">discussion</a></li> <li class="menu-break"><?php edit_post_link('edit this page'); ?></li> <?php } ?> <?php else { ?> <li class="selected"><a href="<?php the_permalink(); ?>">article</a></li> <li><a href="?show=comments">discussion</a></li> <li class="menu-break"><?php edit_post_link('edit this page'); ?></li> <?php } ?> Does that output anything? It might help to post the entire page - if its part of a template, because I don't see anything else wrong from a quick glance. -
[SOLVED] If-else statement using isset creates blank output
Philip replied to yotamono's topic in PHP Coding Help
You're forgetting the semicolon on the end of the function call. <?php the_permalink() ?> should be: <?php the_permalink(); ?> -
I've used this one, and it's not too bad IMO http://www.brandspankingnew.net/specials/ajax_autosuggest/ajax_autosuggest_autocomplete.html There are quite a few that are additions to frameworks if you're already using one (jQuery, mooTools, etc)
-
Well, I'm not sure how you did it when they registered. However, it is not necessary to stripslashes on something that will be encrypted. Give this a shot, I simplified it a bit: <?php if (isset($_POST['submit'])) { // if form has been submitted // makes sure they filled it in if(!$_POST['username'] | !$_POST['pass']) die('You did not fill in a required field.'); // checks it against the database if (!get_magic_quotes_gpc()) $_POST['email'] = addslashes($_POST['email']); // Are you sure you meant to do 'username', or was it supposed to be 'email' $check = mysql_query("SELECT * FROM users WHERE email = '".$_POST['username']."'")or die(mysql_error()); //Gives error if user dosen't exist if(mysql_num_rows($check)==0) die('That is not a vaild username.'); // otherwise, get info $info = mysql_fetch_assoc($check); // this isn't needed, unless you did this when they signed up. // remember, to NOT change $_POST values. // so we'll setup a new variable, named password $password = stripslashes($_POST['pass']); $password = md5($password); //gives error if the password is wrong if ($password != $info['password']) { $test = 'In the DB: '.$info['password'].'<br />'; $test.= 'MD5\'d: '.md5($_POST['pass']).'<br />'; $test.= 'MD5\'d + stripslashes: '.$password.'<br />'; $test.= 'Do any of these match?'; echo $test; die("Invalid password"); } ?>
-
A few things I notice: <?php if(!$_POST['username'] | !$_POST['pass']) { // should be: if(!$_POST['username'] || !$_POST['pass']) { ?> and: <?php // you don't need this, since md5 returns alphanumeric only $_POST['pass'] = stripslashes($_POST['pass']); // you don't need the next line $info['password'] = stripslashes($info['password']); $_POST['pass'] = md5($_POST['pass']); ?>
-
Put session start at the very top of the script, right after <?php
-
It'll give you the same thing everytime if the input is exactly the same. Btw, <?php $pass = md5($_POST['pass']); if ($pass) != $info['password']) { die('Incorrect password, please try again.'); } You won't be able to set a $_POST variable to a different variable - and if you can, it's bad practice.
-
Try this: <?php $body = "We have received the following information:\n\n"; echo '<pre>'; print_r($fields); echo '</pre>'; /*foreach($fields as $a => $b) { $body .= sprintf("%20s: %s\n",$b,$_REQUEST['$a']); }*/ ?>